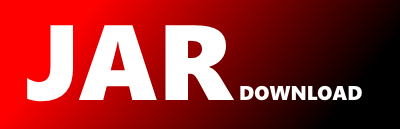
org.bouncycastle.tls.DefaultTlsServer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bctls-fips Show documentation
Show all versions of bctls-fips Show documentation
The Bouncy Castle Java APIs for the TLS, including a JSSE provider. The APIs are designed primarily to be used in conjunction with the BC FIPS provider. The APIs may also be used with other providers although if being used in a FIPS context it is the responsibility of the user to ensure that any other providers used are FIPS certified and used appropriately.
package org.bouncycastle.tls;
import java.io.IOException;
import org.bouncycastle.tls.crypto.TlsCrypto;
public abstract class DefaultTlsServer
extends AbstractTlsServer
{
private static final int[] DEFAULT_CIPHER_SUITES = new int[]
{
/*
* TLS 1.3
*/
CipherSuite.TLS_CHACHA20_POLY1305_SHA256,
CipherSuite.TLS_AES_256_GCM_SHA384,
CipherSuite.TLS_AES_128_GCM_SHA256,
/*
* pre-TLS 1.3
*/
CipherSuite.TLS_ECDHE_RSA_WITH_CHACHA20_POLY1305_SHA256,
CipherSuite.TLS_ECDHE_RSA_WITH_AES_256_GCM_SHA384,
CipherSuite.TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256,
CipherSuite.TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA384,
CipherSuite.TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA256,
CipherSuite.TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA,
CipherSuite.TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA,
CipherSuite.TLS_DHE_RSA_WITH_CHACHA20_POLY1305_SHA256,
CipherSuite.TLS_DHE_RSA_WITH_AES_256_GCM_SHA384,
CipherSuite.TLS_DHE_RSA_WITH_AES_128_GCM_SHA256,
CipherSuite.TLS_DHE_RSA_WITH_AES_256_CBC_SHA256,
CipherSuite.TLS_DHE_RSA_WITH_AES_128_CBC_SHA256,
CipherSuite.TLS_DHE_RSA_WITH_AES_256_CBC_SHA,
CipherSuite.TLS_DHE_RSA_WITH_AES_128_CBC_SHA,
CipherSuite.TLS_RSA_WITH_AES_256_GCM_SHA384,
CipherSuite.TLS_RSA_WITH_AES_128_GCM_SHA256,
CipherSuite.TLS_RSA_WITH_AES_256_CBC_SHA256,
CipherSuite.TLS_RSA_WITH_AES_128_CBC_SHA256,
CipherSuite.TLS_RSA_WITH_AES_256_CBC_SHA,
CipherSuite.TLS_RSA_WITH_AES_128_CBC_SHA,
};
public DefaultTlsServer(TlsCrypto crypto)
{
super(crypto);
}
protected TlsCredentialedSigner getDSASignerCredentials()
throws IOException
{
throw new TlsFatalAlert(AlertDescription.internal_error);
}
protected TlsCredentialedSigner getECDSASignerCredentials()
throws IOException
{
throw new TlsFatalAlert(AlertDescription.internal_error);
}
protected TlsCredentialedDecryptor getRSAEncryptionCredentials()
throws IOException
{
throw new TlsFatalAlert(AlertDescription.internal_error);
}
protected TlsCredentialedSigner getRSASignerCredentials()
throws IOException
{
throw new TlsFatalAlert(AlertDescription.internal_error);
}
protected int[] getSupportedCipherSuites()
{
return TlsUtils.getSupportedCipherSuites(getCrypto(), DEFAULT_CIPHER_SUITES);
}
public TlsCredentials getCredentials()
throws IOException
{
SecurityParameters securityParameters = context.getSecurityParametersHandshake();
switch (securityParameters.getKeyExchangeAlgorithm())
{
case KeyExchangeAlgorithm.DHE_DSS:
return getDSASignerCredentials();
case KeyExchangeAlgorithm.ECDHE_ECDSA:
return getECDSASignerCredentials();
case KeyExchangeAlgorithm.DHE_RSA:
case KeyExchangeAlgorithm.ECDHE_RSA:
return getRSASignerCredentials();
case KeyExchangeAlgorithm.NULL:
throw new TlsFatalAlert(AlertDescription.internal_error,
securityParameters.getNegotiatedVersion() + " credentials unhandled");
case KeyExchangeAlgorithm.RSA:
return getRSAEncryptionCredentials();
default:
/* Note: internal error here; selected a key exchange we don't implement! */
throw new TlsFatalAlert(AlertDescription.internal_error);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy