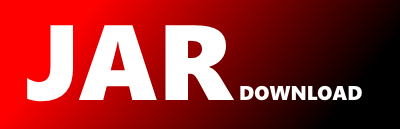
org.bouncycastle.jsse.BCSSLParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bctls-fips Show documentation
Show all versions of bctls-fips Show documentation
The Bouncy Castle Java APIs for the TLS, including a JSSE provider. The APIs are designed primarily to be used in conjunction with the BC FIPS provider. The APIs may also be used with other providers although if being used in a FIPS context it is the responsibility of the user to ensure that any other providers used are FIPS certified and used appropriately.
package org.bouncycastle.jsse;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* A BCJSSE-specific interface providing access to extended SSL parameters in earlier JDKs.
*/
public final class BCSSLParameters
{
private static String[] clone(String[] a)
{
return a == null ? null : (String[])a.clone();
}
private static List copyList(Collection list)
{
if (list == null)
{
return null;
}
if (list.isEmpty())
{
return Collections.emptyList();
}
return Collections.unmodifiableList(new ArrayList(list));
}
private String[] cipherSuites;
private String[] protocols;
private boolean wantClientAuth;
private boolean needClientAuth;
private List serverNames;
private List sniMatchers;
public BCSSLParameters()
{
}
public BCSSLParameters(String[] cipherSuites)
{
setCipherSuites(cipherSuites);
}
public BCSSLParameters(String[] cipherSuites, String[] protocols)
{
setCipherSuites(cipherSuites);
setProtocols(protocols);
}
public String[] getCipherSuites()
{
return clone(cipherSuites);
}
public void setCipherSuites(String[] cipherSuites)
{
this.cipherSuites = clone(cipherSuites);
}
public String[] getProtocols()
{
return clone(protocols);
}
public void setProtocols(String[] protocols)
{
this.protocols = clone(protocols);
}
public boolean getWantClientAuth()
{
return wantClientAuth;
}
public void setWantClientAuth(boolean wantClientAuth)
{
this.wantClientAuth = wantClientAuth;
this.needClientAuth = false;
}
public boolean getNeedClientAuth()
{
return needClientAuth;
}
public void setNeedClientAuth(boolean needClientAuth)
{
this.needClientAuth = needClientAuth;
this.wantClientAuth = false;
}
public void setServerNames(List serverNames)
{
if (serverNames == null)
{
this.serverNames = null;
}
else
{
List copy = copyList(serverNames);
Set types = new HashSet();
for (BCSNIServerName serverName : copy)
{
int type = serverName.getType();
if (!types.add(type))
{
throw new IllegalArgumentException("Found duplicate SNI server name entry of type " + type);
}
}
this.serverNames = copy;
}
}
public List getServerNames()
{
return copyList(this.serverNames);
}
public void setSNIMatchers(Collection sniMatchers)
{
if (sniMatchers == null)
{
this.sniMatchers = null;
}
else
{
List copy = copyList(sniMatchers);
Set types = new HashSet();
for (BCSNIMatcher sniMatcher : copy)
{
int type = sniMatcher.getType();
if (!types.add(type))
{
throw new IllegalArgumentException("Found duplicate SNI matcher entry of type " + type);
}
}
this.sniMatchers = copy;
}
}
public Collection getSNIMatchers()
{
return copyList(this.sniMatchers);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy