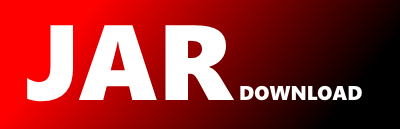
org.bouncycastle.jsse.provider.ProvSSLParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bctls-fips Show documentation
Show all versions of bctls-fips Show documentation
The Bouncy Castle Java APIs for the TLS, including a JSSE provider. The APIs are designed primarily to be used in conjunction with the BC FIPS provider. The APIs may also be used with other providers although if being used in a FIPS context it is the responsibility of the user to ensure that any other providers used are FIPS certified and used appropriately.
package org.bouncycastle.jsse.provider;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import org.bouncycastle.jsse.BCSNIMatcher;
import org.bouncycastle.jsse.BCSNIServerName;
class ProvSSLParameters
{
private static List copyList(Collection list)
{
if (list == null)
{
return null;
}
if (list.isEmpty())
{
return Collections.emptyList();
}
return Collections.unmodifiableList(new ArrayList(list));
}
private final ProvSSLContextSpi context;
private String[] cipherSuites;
private String[] protocols;
private boolean needClientAuth = false;
private boolean wantClientAuth = false;
private Object algorithmConstraints; // object not introduced till 1.6
private String endpointIdentificationAlgorithm;
private boolean useCipherSuitesOrder;
private List sniMatchers;
private List sniServerNames;
ProvSSLParameters(ProvSSLContextSpi context, String[] cipherSuites, String[] protocols)
{
this.context = context;
this.cipherSuites = cipherSuites;
this.protocols = protocols;
}
ProvSSLParameters copy()
{
ProvSSLParameters p = new ProvSSLParameters(context, cipherSuites, protocols);
p.needClientAuth = needClientAuth;
p.wantClientAuth = wantClientAuth;
p.algorithmConstraints = algorithmConstraints;
p.endpointIdentificationAlgorithm = endpointIdentificationAlgorithm;
p.useCipherSuitesOrder = useCipherSuitesOrder;
p.sniMatchers = sniMatchers;
p.sniServerNames = sniServerNames;
return p;
}
public void setCipherSuites(String[] cipherSuites)
{
if (!context.isSupportedCipherSuites(cipherSuites))
{
throw new IllegalArgumentException("'cipherSuites' cannot be null, or contain unsupported cipher suites");
}
this.cipherSuites = cipherSuites.clone();
}
public void setProtocols(String[] protocols)
{
if (!context.isSupportedProtocols(protocols))
{
throw new IllegalArgumentException("'protocols' cannot be null, or contain unsupported protocols");
}
this.protocols = protocols.clone();
}
void setProtocolsArray(String[] protocols)
{
// NOTE: The mechanism of ProvSSLContextSpi.updateDefaultProtocols depends on this not making a copy
this.protocols = protocols;
}
public void setNeedClientAuth(boolean needClientAuth)
{
this.needClientAuth = needClientAuth;
this.wantClientAuth = false;
}
public void setWantClientAuth(boolean wantClientAuth)
{
this.needClientAuth = false;
this.wantClientAuth = wantClientAuth;
}
public String[] getCipherSuites()
{
return cipherSuites.clone();
}
public String[] getProtocols()
{
return protocols.clone();
}
String[] getProtocolsArray()
{
// NOTE: The mechanism of ProvSSLContextSpi.updateDefaultProtocols depends on this not making a copy
return protocols;
}
public boolean getNeedClientAuth()
{
return needClientAuth;
}
public boolean getWantClientAuth()
{
return wantClientAuth;
}
public Object getAlgorithmConstraints()
{
return algorithmConstraints;
}
public void setAlgorithmConstraints(Object algorithmConstraints)
{
this.algorithmConstraints = algorithmConstraints;
}
public String getEndpointIdentificationAlgorithm()
{
return endpointIdentificationAlgorithm;
}
public void setEndpointIdentificationAlgorithm(String endpointIdentificationAlgorithm)
{
this.endpointIdentificationAlgorithm = endpointIdentificationAlgorithm;
}
public boolean getUseCipherSuitesOrder()
{
return useCipherSuitesOrder;
}
public void setUseCipherSuitesOrder(boolean honorOrder)
{
this.useCipherSuitesOrder = honorOrder;
}
public List getServerNames()
{
return copyList(sniServerNames);
}
public void setServerNames(List serverNames)
{
this.sniServerNames = copyList(serverNames);
}
public Collection getSNIMatchers()
{
return copyList(sniMatchers);
}
public void setSNIMatchers(Collection matchers)
{
this.sniMatchers = copyList(matchers);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy