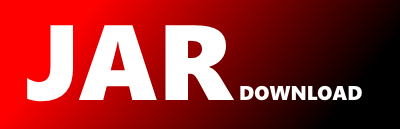
org.bouncycastle.tls.ServerName Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bctls-fips Show documentation
Show all versions of bctls-fips Show documentation
The Bouncy Castle Java APIs for the TLS, including a JSSE provider. The APIs are designed primarily to be used in conjunction with the BC FIPS provider. The APIs may also be used with other providers although if being used in a FIPS context it is the responsibility of the user to ensure that any other providers used are FIPS certified and used appropriately.
package org.bouncycastle.tls;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
public class ServerName
{
protected short nameType;
protected Object name;
public ServerName(short nameType, Object name)
{
if (!isCorrectType(nameType, name))
{
throw new IllegalArgumentException("'name' is not an instance of the correct type");
}
this.nameType = nameType;
this.name = name;
}
public short getNameType()
{
return nameType;
}
public Object getName()
{
return name;
}
public String getHostName()
{
if (!isCorrectType(NameType.host_name, name))
{
throw new IllegalStateException("'name' is not a HostName string");
}
return (String)name;
}
/**
* Encode this {@link ServerName} to an {@link OutputStream}.
*
* @param output
* the {@link OutputStream} to encode to.
* @throws IOException
*/
public void encode(OutputStream output) throws IOException
{
TlsUtils.writeUint8(nameType, output);
switch (nameType)
{
case NameType.host_name:
byte[] asciiEncoding = ((String)name).getBytes("ASCII");
if (asciiEncoding.length < 1)
{
throw new TlsFatalAlert(AlertDescription.internal_error);
}
TlsUtils.writeOpaque16(asciiEncoding, output);
break;
default:
throw new TlsFatalAlert(AlertDescription.internal_error);
}
}
/**
* Parse a {@link ServerName} from an {@link InputStream}.
*
* @param input
* the {@link InputStream} to parse from.
* @return a {@link ServerName} object.
* @throws IOException
*/
public static ServerName parse(InputStream input) throws IOException
{
short name_type = TlsUtils.readUint8(input);
Object name;
switch (name_type)
{
case NameType.host_name:
{
byte[] asciiEncoding = TlsUtils.readOpaque16(input);
if (asciiEncoding.length < 1)
{
throw new TlsFatalAlert(AlertDescription.decode_error);
}
name = new String(asciiEncoding, "ASCII");
break;
}
default:
throw new TlsFatalAlert(AlertDescription.decode_error);
}
return new ServerName(name_type, name);
}
protected static boolean isCorrectType(short nameType, Object name)
{
switch (nameType)
{
case NameType.host_name:
return name instanceof String;
default:
throw new IllegalArgumentException("'nameType' is an unsupported NameType");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy