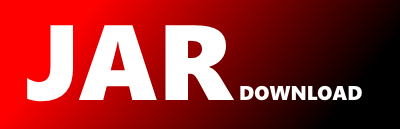
org.bouncycastle.jsse.provider.JsseUtils_8 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bctls-fips Show documentation
Show all versions of bctls-fips Show documentation
The Bouncy Castle Java APIs for the TLS, including a JSSE provider. The APIs are designed primarily to be used in conjunction with the BC FIPS provider. The APIs may also be used with other providers although if being used in a FIPS context it is the responsibility of the user to ensure that any other providers used are FIPS certified and used appropriately.
package org.bouncycastle.jsse.provider;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import javax.net.ssl.SNIHostName;
import javax.net.ssl.SNIMatcher;
import javax.net.ssl.SNIServerName;
import javax.net.ssl.StandardConstants;
import org.bouncycastle.jsse.BCSNIHostName;
import org.bouncycastle.jsse.BCSNIMatcher;
import org.bouncycastle.jsse.BCSNIServerName;
import org.bouncycastle.jsse.BCStandardConstants;
abstract class JsseUtils_8
extends JsseUtils_7
{
static class ExportSNIMatcher extends SNIMatcher
{
private final BCSNIMatcher matcher;
ExportSNIMatcher(BCSNIMatcher matcher)
{
super(matcher.getType());
this.matcher = matcher;
}
@Override
public boolean matches(SNIServerName serverName)
{
return matcher.matches(importSNIServerName(serverName));
}
BCSNIMatcher unwrap()
{
return matcher;
}
}
static class ImportSNIMatcher extends BCSNIMatcher
{
private final SNIMatcher matcher;
ImportSNIMatcher(SNIMatcher matcher)
{
super(matcher.getType());
this.matcher = matcher;
}
@Override
public boolean matches(BCSNIServerName serverName)
{
return matcher.matches(exportSNIServerName(serverName));
}
SNIMatcher unwrap()
{
return matcher;
}
}
static class UnknownServerName extends SNIServerName
{
UnknownServerName(int type, byte[] encoded)
{
super(type, encoded);
}
}
static SNIMatcher exportSNIMatcher(BCSNIMatcher matcher)
{
if (null == matcher)
{
return null;
}
if (matcher instanceof ImportSNIMatcher)
{
return ((ImportSNIMatcher)matcher).unwrap();
}
return new ExportSNIMatcher(matcher);
}
/*
* NOTE: Currently return type is Object to isolate callers from JDK8 type
*/
static Object exportSNIMatchers(Collection matchers)
{
if (matchers.isEmpty())
{
return Collections.emptyList();
}
ArrayList result = new ArrayList(matchers.size());
for (BCSNIMatcher matcher : matchers)
{
result.add(exportSNIMatcher(matcher));
}
return Collections.unmodifiableList(result);
}
static SNIServerName exportSNIServerName(BCSNIServerName serverName)
{
if (null == serverName)
{
return null;
}
int type = serverName.getType();
byte[] encoded = serverName.getEncoded();
switch (type)
{
case BCStandardConstants.SNI_HOST_NAME:
return new SNIHostName(encoded);
default:
return new UnknownServerName(type, encoded);
}
}
/*
* NOTE: Currently return type is Object to isolate callers from JDK8 type
*/
static Object exportSNIServerNames(Collection serverNames)
{
if (serverNames.isEmpty())
{
return Collections.emptyList();
}
ArrayList result = new ArrayList(serverNames.size());
for (BCSNIServerName serverName : serverNames)
{
result.add(exportSNIServerName(serverName));
}
return Collections.unmodifiableList(result);
}
static BCSNIMatcher importSNIMatcher(SNIMatcher matcher)
{
if (null == matcher)
{
return null;
}
if (matcher instanceof ExportSNIMatcher)
{
return ((ExportSNIMatcher)matcher).unwrap();
}
return new ImportSNIMatcher(matcher);
}
/*
* NOTE: Currently argument is Object type to isolate callers from JDK8 type
*/
static List importSNIMatchers(Object getSNIMatchersResult)
{
@SuppressWarnings("unchecked")
Collection matchers = (Collection)getSNIMatchersResult;
if (matchers.isEmpty())
{
return Collections.emptyList();
}
ArrayList result = new ArrayList(matchers.size());
for (SNIMatcher matcher : matchers)
{
result.add(importSNIMatcher(matcher));
}
return Collections.unmodifiableList(result);
}
static BCSNIServerName importSNIServerName(SNIServerName serverName)
{
if (null == serverName)
{
return null;
}
int type = serverName.getType();
byte[] encoded = serverName.getEncoded();
switch (type)
{
case StandardConstants.SNI_HOST_NAME:
return new BCSNIHostName(encoded);
default:
return new BCUnknownServerName(type, encoded);
}
}
/*
* NOTE: Currently argument is Object type to isolate callers from JDK8 type
*/
static List importSNIServerNames(Object getServerNamesResult)
{
@SuppressWarnings("unchecked")
Collection serverNames = (Collection)getServerNamesResult;
if (serverNames.isEmpty())
{
return Collections.emptyList();
}
ArrayList result = new ArrayList(serverNames.size());
for (SNIServerName serverName : serverNames)
{
result.add(importSNIServerName(serverName));
}
return Collections.unmodifiableList(result);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy