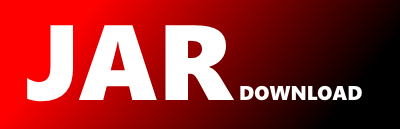
org.bouncycastle.tls.DTLSProtocol Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bctls-fips Show documentation
Show all versions of bctls-fips Show documentation
The Bouncy Castle Java APIs for the TLS, including a JSSE provider. The APIs are designed primarily to be used in conjunction with the BC FIPS provider. The APIs may also be used with other providers although if being used in a FIPS context it is the responsibility of the user to ensure that any other providers used are FIPS certified and used appropriately.
package org.bouncycastle.tls;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.util.Hashtable;
import java.util.Vector;
import org.bouncycastle.util.Arrays;
public abstract class DTLSProtocol
{
protected DTLSProtocol()
{
}
protected void processFinished(byte[] body, byte[] expected_verify_data)
throws IOException
{
ByteArrayInputStream buf = new ByteArrayInputStream(body);
byte[] verify_data = TlsUtils.readFully(expected_verify_data.length, buf);
TlsProtocol.assertEmpty(buf);
if (!Arrays.constantTimeAreEqual(expected_verify_data, verify_data))
{
throw new TlsFatalAlert(AlertDescription.handshake_failure);
}
}
protected static void applyMaxFragmentLengthExtension(DTLSRecordLayer recordLayer, short maxFragmentLength)
throws IOException
{
if (maxFragmentLength >= 0)
{
if (!MaxFragmentLength.isValid(maxFragmentLength))
{
throw new TlsFatalAlert(AlertDescription.internal_error);
}
int plainTextLimit = 1 << (8 + maxFragmentLength);
recordLayer.setPlaintextLimit(plainTextLimit);
}
}
protected static short evaluateMaxFragmentLengthExtension(boolean resumedSession, Hashtable clientExtensions,
Hashtable serverExtensions, short alertDescription) throws IOException
{
short maxFragmentLength = TlsExtensionsUtils.getMaxFragmentLengthExtension(serverExtensions);
if (maxFragmentLength >= 0)
{
if (!MaxFragmentLength.isValid(maxFragmentLength)
|| (!resumedSession && maxFragmentLength != TlsExtensionsUtils
.getMaxFragmentLengthExtension(clientExtensions)))
{
throw new TlsFatalAlert(alertDescription);
}
}
return maxFragmentLength;
}
protected static byte[] generateCertificate(TlsContext context, Certificate certificate, OutputStream endPointHash)
throws IOException
{
ByteArrayOutputStream buf = new ByteArrayOutputStream();
certificate.encode(context, buf, endPointHash);
return buf.toByteArray();
}
protected static byte[] generateSupplementalData(Vector supplementalData)
throws IOException
{
ByteArrayOutputStream buf = new ByteArrayOutputStream();
TlsProtocol.writeSupplementalData(buf, supplementalData);
return buf.toByteArray();
}
protected static byte[] createVerifyData(TlsContext context, DTLSReliableHandshake handshake, boolean isServer)
{
return TlsUtils.calculateTLSVerifyData(context, handshake.getHandshakeHash(), isServer);
}
protected static void sendCertificateMessage(TlsContext context, DTLSReliableHandshake handshake,
Certificate certificate, OutputStream endPointHash) throws IOException
{
SecurityParameters securityParameters = context.getSecurityParametersHandshake();
if (null != securityParameters.getLocalCertificate())
{
throw new TlsFatalAlert(AlertDescription.internal_error);
}
if (null == certificate)
{
certificate = Certificate.EMPTY_CHAIN;
}
byte[] certificateBody = generateCertificate(context, certificate, endPointHash);
handshake.sendMessage(HandshakeType.certificate, certificateBody);
securityParameters.localCertificate = certificate;
}
protected static int validateSelectedCipherSuite(int selectedCipherSuite, short alertDescription)
throws IOException
{
switch (TlsUtils.getEncryptionAlgorithm(selectedCipherSuite))
{
case EncryptionAlgorithm.RC4_40:
case EncryptionAlgorithm.RC4_128:
case -1:
throw new TlsFatalAlert(alertDescription);
default:
return selectedCipherSuite;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy