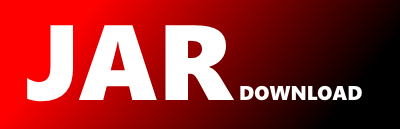
org.bremersee.utils.IoUtils Maven / Gradle / Ivy
/*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.bremersee.utils;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
/**
*
* Some IO utilities.
*
*
* @author Christian Bremer
*/
public abstract class IoUtils {
private IoUtils() {
// utility class, never constructed
}
/**
* Copies data.
*
* @param inputStream
* the input data
* @param outputStream
* the output destination
* @param closeStreams
* if {@code true} the streams will be closed otherwise they will stay open
* @return the length of copied data
* @throws RuntimeException
* if copying fails
*/
public static long copySilently(InputStream inputStream, OutputStream outputStream, boolean closeStreams) {
return copySilently(inputStream, outputStream, closeStreams, null);
}
/**
* Copies data.
*
* @param inputStream
* the input data
* @param outputStream
* the output destination
* @param closeStreams
* if {@code true} the streams will be closed otherwise they will stay open
* @param listener
* a listener (may be {@code null})
* @return the length of copied data
* @throws RuntimeException
* if copying fails
*/
public static long copySilently(InputStream inputStream, OutputStream outputStream, boolean closeStreams, InputStreamListener listener) {
long totalLen = 0L;
int len;
byte[] buf = new byte[4096];
try {
while ((len = inputStream.read(buf)) != -1) {
outputStream.write(buf, 0, len);
totalLen = totalLen + len;
if (listener != null) {
listener.onReadBytes(buf, 0, len);
}
}
outputStream.flush();
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
if (closeStreams) {
try {
inputStream.close();
} catch (IOException ignored) {
// ignored
}
try {
outputStream.close();
} catch (IOException ignored) {
// ignored
}
}
}
return totalLen;
}
/**
* Copies characters.
*
* @param reader
* the reader
* @param writer
* the writer
* @param closeReaderAndWriter
* if {@code true} the reader and writer will be closed otherwise they will stay open
* @return the size of the characters
* @throws RuntimeException
* if copying fails
*/
public static long copySilently(Reader reader, Writer writer, boolean closeReaderAndWriter) {
return copySilently(reader, writer, closeReaderAndWriter, null);
}
/**
* Copies characters.
*
* @param reader
* the reader
* @param writer
* the writer
* @param closeReaderAndWriter
* if {@code true} the reader and writer will be closed otherwise they will stay open
* @param listener
* a listener (may be {@code null})
* @return the size of the characters
* @throws RuntimeException
* if copying fails
*/
public static long copySilently(Reader reader, Writer writer, boolean closeReaderAndWriter, ReaderListener listener) {
long totalLen = 0L;
int len;
char[] buf = new char[4096];
try {
while ((len = reader.read(buf)) != -1) {
writer.write(buf, 0, len);
totalLen = totalLen + len;
if (listener != null) {
listener.onReadChars(buf, 0, len);
}
}
writer.flush();
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
if (closeReaderAndWriter) {
try {
reader.close();
} catch (IOException ignored) {
// ignored
}
try {
writer.close();
} catch (IOException ignored) {
// ignored
}
}
}
return totalLen;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy