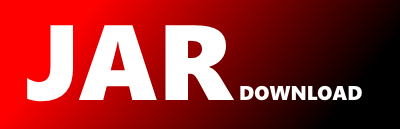
org.bridgedb.bio.EnsemblCompatibilityMapper Maven / Gradle / Ivy
// BridgeDb,
// An abstraction layer for identifier mapping services, both local and online.
// Copyright 2006-2012 BridgeDb developers
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
package org.bridgedb.bio;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.bridgedb.DataSource;
import org.bridgedb.IDMapper;
import org.bridgedb.IDMapperCapabilities;
import org.bridgedb.IDMapperException;
import org.bridgedb.Xref;
import org.bridgedb.impl.InternalUtils;
/**
* @deprecated this class is no longer needed for recent Ensembl id mapping databases
*/
public class EnsemblCompatibilityMapper implements IDMapper
{
@Override
public Map> mapID(Collection srcXrefs, DataSource... tgtDataSources)
throws IDMapperException
{
return InternalUtils.mapMultiFromSingle(this, srcXrefs, tgtDataSources);
}
@Override
public Set mapID(Xref ref, DataSource... tgtDataSources) throws IDMapperException
{
Set result = new HashSet();
if (!allDataSources.contains(ref.getDataSource())) return result;
Set dest = new HashSet();
// either from old to new or new to old, but not both at the same time...
if (ref.getDataSource() == BioDataSource.ENSEMBL)
dest.addAll (oldDataSources);
else
dest.add(BioDataSource.ENSEMBL);
// keep only tgtDataSources that were asked for, if defined
if (tgtDataSources != null && tgtDataSources.length > 0)
dest.retainAll(Arrays.asList(tgtDataSources));
for (DataSource ds : dest)
{
if (ds == ref.getDataSource()) continue;
result.add(new Xref(ref.getId(), ds));
}
return result;
}
@Override
public boolean xrefExists(Xref xref) throws IDMapperException
{
return true;
}
@Override
public Set freeSearch(String text, int limit) throws IDMapperException
{
return Collections.emptySet();
}
private final Set oldDataSources;
private final Set allDataSources;
public EnsemblCompatibilityMapper()
{
oldDataSources = new HashSet();
oldDataSources.add(BioDataSource.ENSEMBL_BSUBTILIS);
oldDataSources.add(BioDataSource.ENSEMBL_CELEGANS);
oldDataSources.add(BioDataSource.ENSEMBL_CHICKEN);
oldDataSources.add(BioDataSource.ENSEMBL_CHIMP);
oldDataSources.add(BioDataSource.ENSEMBL_COW);
oldDataSources.add(BioDataSource.ENSEMBL_DOG);
oldDataSources.add(BioDataSource.ENSEMBL_ECOLI);
oldDataSources.add(BioDataSource.ENSEMBL_FRUITFLY);
oldDataSources.add(BioDataSource.ENSEMBL_HORSE);
oldDataSources.add(BioDataSource.ENSEMBL_HUMAN);
oldDataSources.add(BioDataSource.ENSEMBL_MOSQUITO);
oldDataSources.add(BioDataSource.ENSEMBL_MOUSE);
oldDataSources.add(BioDataSource.ENSEMBL_MTUBERCULOSIS);
oldDataSources.add(BioDataSource.ENSEMBL_RAT);
oldDataSources.add(BioDataSource.ENSEMBL_SCEREVISIAE);
oldDataSources.add(BioDataSource.ENSEMBL_XENOPUS);
oldDataSources.add(BioDataSource.ENSEMBL_ZEBRAFISH);
allDataSources = new HashSet(oldDataSources);
allDataSources.add(BioDataSource.ENSEMBL);
}
@Override
public IDMapperCapabilities getCapabilities()
{
return new IDMapperCapabilities()
{
@Override
public boolean isMappingSupported(DataSource src, DataSource tgt) throws IDMapperException
{
return (oldDataSources.contains(src) && tgt == BioDataSource.ENSEMBL)
||
(oldDataSources.contains(tgt) && src == BioDataSource.ENSEMBL);
}
@Override
public boolean isFreeSearchSupported()
{
return false;
}
@Override
public Set getSupportedTgtDataSources() throws IDMapperException
{
return allDataSources;
}
@Override
public Set getSupportedSrcDataSources() throws IDMapperException
{
return allDataSources;
}
@Override
public String getProperty(String key)
{
return null;
}
@Override
public Set getKeys()
{
return Collections.emptySet();
}
};
}
private boolean closed = false;
@Override
public void close() throws IDMapperException
{
closed = true;
}
@Override
public boolean isConnected()
{
return !closed;
}
@Override
public String toString()
{
return "old-ensembl-systemcode-compatbility-mapper";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy