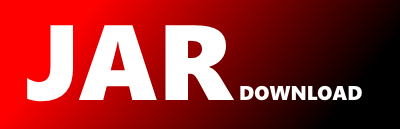
org.bridje.orm.impl.JoinQueryImpl Maven / Gradle / Ivy
/*
* Copyright 2016 Bridje Framework.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.bridje.orm.impl;
import java.sql.SQLException;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import org.bridje.orm.Column;
import org.bridje.orm.Condition;
import org.bridje.orm.OrderBy;
import org.bridje.orm.Query;
import org.bridje.orm.TableColumn;
import org.bridje.orm.impl.sql.SelectBuilder;
class JoinQueryImpl extends AbstractQuery implements Query
{
private TableRelationColumnImpl relation;
private TableImpl related;
private Condition condition;
private final AbstractQuery baseQuery;
private final JoinType type;
public JoinQueryImpl(JoinType type, AbstractQuery baseQuery,
TableRelationColumnImpl relation)
{
this.type = type;
this.baseQuery = baseQuery;
this.relation = relation;
}
public JoinQueryImpl(JoinType type, AbstractQuery baseQuery, TableImpl related, Condition condition)
{
this.related = related;
this.condition = condition;
this.baseQuery = baseQuery;
this.type = type;
}
@Override
public Query paging(int page, int size)
{
baseQuery.paging(page, size);
return this;
}
@Override
public Query where(Condition condition)
{
baseQuery.where(condition);
return this;
}
@Override
public Query orderBy(OrderBy... statements)
{
baseQuery.orderBy(statements);
return this;
}
@Override
protected int getPage()
{
return baseQuery.getPage();
}
@Override
protected int getPageSize()
{
return baseQuery.getPageSize();
}
@Override
protected TableImpl getTable()
{
if(relation == null) return related;
return (TableImpl)relation.getRelated();
}
@Override
protected TableImpl> getBaseTable()
{
return baseQuery.getBaseTable();
}
@Override
protected EntityContextImpl getCtx()
{
return baseQuery.getCtx();
}
@Override
protected SelectBuilder createQuery(String fields, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy