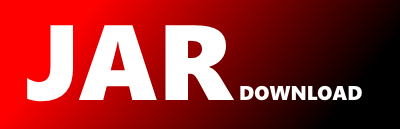
org.bridje.jfx.srcgen.model.ObjectInf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bridje-jfx-srcgen Show documentation
Show all versions of bridje-jfx-srcgen Show documentation
Source Generation for Bridje JavaFX tools and components.
The newest version!
package org.bridje.jfx.srcgen.model;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.Unmarshaller;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlElements;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlTransient;
/**
* Data object information for a JavaFX model.
*/
@XmlAccessorType(XmlAccessType.FIELD)
public class ObjectInf
{
@XmlID
@XmlAttribute
private String name;
private String description;
@XmlAttribute
private String key;
@XmlAttribute
private String toString;
@XmlTransient
private PropertyInf keyProperty;
@XmlTransient
private PropertyInf toStringProperty;
@XmlElementWrapper(name = "properties")
@XmlElements(
{
@XmlElement(name = "observableList", type = ObListPropertyInf.class)
,
@XmlElement(name = "property", type = PropertyInf.class)
})
private List properties;
@XmlElementWrapper(name = "includes")
@XmlElements(
{
@XmlElement(name = "include", type = IncludeInf.class)
})
private List includes;
@XmlTransient
private ModelInf model;
@XmlTransient
private List allProperties;
@XmlElementWrapper(name = "components")
@XmlElements(
{
@XmlElement(name = "table", type = TableComponent.class)
,
@XmlElement(name = "treeTable", type = TreeTableComponent.class)
})
private List components;
@XmlAttribute
private String base;
@XmlElementWrapper(name = "mappings")
@XmlElements(
{
@XmlElement(name = "mapping", type = ObjectInfMapping.class)
})
private List mappings;
/**
* The java class name of the object.
*
* @return The java class name of the object.
*/
public String getName()
{
return name;
}
/**
* The java class name of the object.
*
* @param name The java class name of the object.
*/
public void setName(String name)
{
this.name = name;
}
/**
* A description of this object.
*
* @return A description of this object.
*/
public String getDescription()
{
return description;
}
/**
* A description of this object.
*
* @param description A description of this object.
*/
public void setDescription(String description)
{
this.description = description;
}
/**
* The properties of this object.
*
* @return The properties of this object.
*/
public List getProperties()
{
if (properties == null)
{
properties = new ArrayList<>();
}
return properties;
}
/**
* The properties of this object.
*
* @param properties The properties of this object.
*/
public void setProperties(List properties)
{
this.properties = properties;
}
/**
* The parent JavaFx model of this object.
*
* @return The parent JavaFx model of this object.
*/
public ModelInf getModel()
{
return model;
}
/**
* The java package of this object.
*
* @return The java package of this object.
*/
public String getPackage()
{
return model.getPackage();
}
/**
* The full java class name for this object.
*
* @return The full java class name for this object.
*/
public String getFullName()
{
return getPackage() + "." + getName();
}
/**
* Called by JAXB.
*
* @param u The unmarshaller.
* @param parent The parent.
*/
public void afterUnmarshal(Unmarshaller u, Object parent)
{
model = (ModelInf) parent;
}
/**
* The key property for this object.
*
* @return The key property for this object.
*/
public PropertyInf getKeyProperty()
{
return keyProperty;
}
/**
* The key property for this object.
*
* @param keyProperty The key property for this object.
*/
public void setKeyProperty(PropertyInf keyProperty)
{
if (keyProperty == null && key != null)
{
keyProperty = findProperty(key);
}
this.keyProperty = keyProperty;
}
/**
* The list of java classes to include.
*
* @return The list of java classes to include.
*/
public List getIncludes()
{
return includes;
}
/**
* The list of java classes to include.
*
* @param includes The list of java classes to include.
*/
public void setIncludes(List includes)
{
this.includes = includes;
}
/**
* The toString property.
*
* @return The toString property.
*/
public PropertyInf getToStringProperty()
{
if (toString == null && key != null)
{
toString = key;
}
if (toStringProperty == null && toString != null)
{
toStringProperty = findProperty(toString);
}
return toStringProperty;
}
/**
* The toString property.
*
* @param toStringProperty The toString property.
*/
public void setToStringProperty(PropertyInf toStringProperty)
{
this.toStringProperty = toStringProperty;
}
/**
* The components that must be generated for this object.
*
* @return The components that must be generated for this object.
*/
public List getComponents()
{
return components;
}
/**
* The components that must be generated for this object.
*
* @param components The components that must be generated for this object.
*/
public void setComponents(List components)
{
this.components = components;
}
/**
* The base object.
*
* @return The base object.
*/
public String getBase()
{
return base;
}
/**
* The base object.
*
* @param base The base object.
*/
public void setBase(String base)
{
this.base = base;
}
/**
* The list of mappings for this object.
*
* @return The list of mappings for this object.
*/
public List getMappings()
{
return mappings;
}
/**
* The list of mappings for this object.
*
* @param mappings The list of mappings for this object.
*/
public void setMappings(List mappings)
{
this.mappings = mappings;
}
/**
* Finds the given property in this object.
*
* @param propName The name of the property.
*
* @return The property found, or null if it does not exsits.
*/
public PropertyInf findProperty(String propName)
{
for (PropertyInf property : getProperties())
{
if (property.getName().equals(propName))
{
return property;
}
}
return null;
}
/**
* Determines if the given property is a list.
*
* @param propName The property name.
*
* @return true the property is a list, false otherwise.
*/
public boolean isList(String propName)
{
PropertyInf prop = findProperty(propName);
if (prop != null)
{
return prop.getIsList();
}
return false;
}
/**
* Determines if the given property is an object.
*
* @param propName The property name.
*
* @return true the property is a list, false otherwise.
*/
public boolean isObject(String propName)
{
PropertyInf prop = findProperty(propName);
if (prop != null)
{
return model.isObject(prop.getType());
}
return false;
}
/**
* Finds the object information of a property by the property name.
*
* @param propName The property name.
*
* @return The object information of the property, or null if the property is not found.
*/
public ObjectInf findObject(String propName)
{
PropertyInf prop = findProperty(propName);
if (prop != null)
{
return model.findObject(prop.getType());
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy