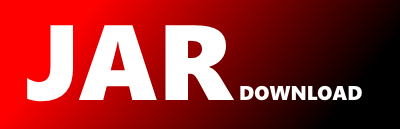
commonMain.org.brightify.hyperdrive.utils.Optional.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlin-utils-jvm Show documentation
Show all versions of kotlin-utils-jvm Show documentation
Module containing various utils used throughout Hyperdrive.
package org.brightify.hyperdrive.utils
import kotlinx.coroutines.FlowPreview
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.emptyFlow
import kotlinx.coroutines.flow.flatMapConcat
import kotlinx.coroutines.flow.flowOf
public sealed interface Optional {
public data class Some(public val value: T): Optional
public object None: Optional
public fun withValue(block: (T) -> Unit) {
if (this is Some) {
block(value)
}
}
}
public inline fun Optional.toKotlin(): T? {
return when (this) {
is Optional.Some -> value
is Optional.None -> null
}
}
public inline fun T?.toOptional(): Optional {
return this?.let { Optional.Some(it) } ?: Optional.None
}
public inline fun Optional.map(transform: (T) -> U): Optional {
return flatMap { Optional.Some(transform(it)) }
}
public inline fun Optional.mapToKotlin(transform: (T) -> U): U? {
return when (this) {
is Optional.Some -> transform(value)
is Optional.None -> null
}
}
public inline fun Optional.flatMap(transform: (T) -> Optional): Optional {
return when (this) {
is Optional.Some -> transform(value)
is Optional.None -> Optional.None
}
}
@OptIn(FlowPreview::class)
public fun Flow>.filterSome(): Flow {
return flatMapConcat {
when (it) {
is Optional.Some -> flowOf(it.value)
Optional.None -> emptyFlow()
}
}
}
public inline fun Optional.someOrDefault(block: () -> T): T {
return when (this) {
is Optional.Some -> value
Optional.None -> block()
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy