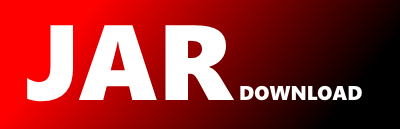
com.vii.brillien.plugin.DefinitionDownloader Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2012 Imre Fazekas.
* All rights reserved.
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* Neither the name of the Brillien nor the names of its
* terms and concepts may be used to endorse or promote products derived from this
* software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.vii.brillien.plugin;
import com.vii.brillien.core.component.server.discover.generator.Definition;
import com.vii.brillien.ignition.transport.BrillienCommunication;
import com.vii.brillien.ignition.transport.Configuration;
import com.vii.brillien.ignition.transport.pure.PureMediator;
import com.vii.brillien.kernel.axiom.transport.Communication;
import com.vii.streamline.services.IOServices;
import com.vii.streamline.services.ThreadServices;
import com.vii.streamline.services.json.JsonServices;
import com.vii.streamline.structures.collections.InnerMap;
import com.vii.streamline.web.HttpServices;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import java.io.File;
import java.io.FileInputStream;
import java.util.Map;
/**
* Generates client Java classes responding to the given JSON structures
* @goal downloadDefinition
* @requiresProject false
*/
public class DefinitionDownloader extends AbstractMojo {
/**
* Location of the file.
* @parameter expression="configFile" default-value="${project.basedir}/config.json"
* @required
*/
private File configFile;
/**
* Name of the presence
* @parameter expression="presenceName"
* @required
*/
private String presenceName;
/**
* Version of the presence
* @parameter expression="presenceVersion"
* @required
*/
private String presenceVersion;
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
Configuration configuration = null;
try {
configuration = JsonServices.parseJSON(IOServices.getStreamAsString(new FileInputStream(configFile)), Configuration.class);
} catch (Exception e) {
throw new MojoFailureException( "Cannot read config file.", e );
}
PureMediator mediator = configuration.createPureMediator();
try {
getLog().info("Config read:" + configuration );
Map parameters = new InnerMap("presence", presenceName, "apiVersion", presenceVersion );
BrillienCommunication comm = mediator.prepareNewCommunication( "Discover", null, "getDefinition", Communication.TYPE_GET, parameters );
getLog().info("Getting definition..." );
BrillienCommunication response = mediator.sendCommunication( comm );
Definition definition = response.acquireResponse( Definition.class );
getLog().info("Retrieved definition:" + definition );
IOServices.writeToFile(
JsonServices.toJSON(definition),
"./" + presenceName + "Definition.json"
);
getLog().info("Done." );
} catch (Exception e) {
throw new MojoFailureException( "Cannot get the definition of the services of the presence:" + presenceName, e );
} finally {
HttpServices.shutdownWebClient();
ThreadServices.stop();
}
}
public static void main(String[] args) throws MojoExecutionException, MojoFailureException {
DefinitionDownloader generator = new DefinitionDownloader();
generator.configFile = new File( "clientConfiguration.json" );
generator.presenceName = "Discover";
generator.execute();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy