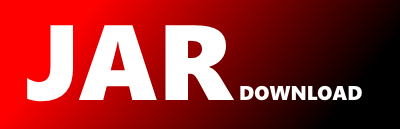
com.vii.brillien.plugin.JsonMappingGenerator Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2012 Imre Fazekas.
* All rights reserved.
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* Neither the name of the Brillien nor the names of its
* terms and concepts may be used to endorse or promote products derived from this
* software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.vii.brillien.plugin;
import com.vii.brillien.core.component.server.discover.generator.*;
import com.vii.brillien.ignition.BrillienStatics;
import com.vii.brillien.ignition.transport.BrillienCommunication;
import com.vii.brillien.ignition.transport.Configuration;
import com.vii.brillien.kernel.BrillienException;
import com.vii.brillien.kernel.axiom.transport.Communication;
import com.vii.streamline.services.IOServices;
import com.vii.streamline.services.RegularServices;
import com.vii.streamline.services.StringServices;
import com.vii.streamline.services.error.StreamLineException;
import com.vii.streamline.services.json.JsonServices;
import com.vii.streamline.services.json.converters.BooleanConverter;
import com.vii.streamline.services.json.converters.DateConverter;
import com.vii.streamline.services.json.converters.DoubleConverter;
import com.vii.streamline.services.json.converters.LongConverter;
import com.vii.streamline.services.json.matchers.PatternMatcher;
import com.vii.streamline.structures.collections.InnerList;
import com.vii.streamline.structures.collections.InnerMap;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.svenson.JSONProperty;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Date;
import java.util.List;
import java.util.Map;
/**
* Generates Java classes responding to the given JSON structures
* @goal generateJsonTypes
* @requiresProject false
*/
public class JsonMappingGenerator extends AbstractMojo {
/**
* Location of the file.
* @parameter expression="jsonFile" default-value="${project.basedir}/gen/definitions.json"
* @required
*/
private File jsonFile;
/**
* Location of the file.
* @parameter expression="projectDirectory" default-value="${project.basedir}"
*/
private File projectDirectory;
/**
* Location of the file.
* @parameter expression="srcFolder" default-value="${project.basedir}/src"
*/
private File srcDirectory;
public File getJsonFile() {
return jsonFile;
}
public void setJsonFile(File jsonFile) {
this.jsonFile = jsonFile;
}
public File getSrcDirectory() {
return srcDirectory;
}
public void setSrcDirectory(File srcDirectory) {
this.srcDirectory = srcDirectory;
}
private TypeInfo comformiseType(TypeInfo typeInfo) throws BrillienException {
String lowReference = typeInfo.getTypeName().toLowerCase();
if( lowReference.equals("int") || lowReference.equals("integer") || lowReference.equals("long") || lowReference.equals("number") || lowReference.equals("numeric") ){
typeInfo.setTypeName("Long");
typeInfo.setConverter(LongConverter.class);
}
else if( lowReference.equals("boolean") || lowReference.equals("bool") ){
typeInfo.setTypeName("Boolean");
typeInfo.setConverter(BooleanConverter.class);
}
else if( lowReference.equals("double") || lowReference.equals("float") ){
typeInfo.setTypeName( "Double" );
typeInfo.setConverter( DoubleConverter.class );
}
else if( lowReference.equals("date") || lowReference.equals("time") ){
typeInfo.setTypeName("Date");
typeInfo.setConverter( DateConverter.class );
typeInfo.addImportType( Date.class );
} else{
typeInfo.setJsonType(false);
}
return typeInfo;
}
private TypeInfo getType(String reference) throws BrillienException {
if( reference.contains(".") ){
TypeInfo typeInfo = new TypeInfo();
typeInfo.setTypeName( reference );
try{
Class c = Class.forName( reference );
typeInfo.addImportType( c );
} catch (ClassNotFoundException e) { }
return typeInfo;
}
reference = StringServices.capitalize(reference.replaceAll("\\s", ""));
TypeInfo typeInfo = new TypeInfo();
if( !RegularServices.matches( reference, "\\p{L}{1,}[\\{\\[\\]\\}]*" ) )
throw new BrillienException( StreamLineException.ERROR_DATA_ERROR, "Not a proper type:" + reference );
int index = -1;
if( (index = reference.indexOf("[")) > -1 || (index = reference.indexOf("{")) > -1 ){
typeInfo.setTypeName( reference.substring(0, index) );
String containerPart = reference.substring( index );
for( int i=0; i");
typeInfo.addContainer(List.class);
typeInfo.concatContainerPattern( "[]" );
}
else if( containerTypeDef.startsWith("{") ){
typeInfo.setPrintName("Map");
typeInfo.addContainer(Map.class);
typeInfo.concatContainerPattern("{}");
}
else throw new BrillienException(StreamLineException.ERROR_DATA_ERROR, "Cannot interpret this type definition:" + reference );
}
} else
typeInfo.setTypeName( reference );
return comformiseType(typeInfo);
}
private void manageImport(TypeInfo info, List imports, StringBuilder sb){
for (Class container : info.getContainers()) {
if( !imports.contains( container.getName() ) ){
sb.append( "import " + container.getName() + ";" + "\n" );
imports.add( container.getName() );
}
}
if( info.getConverter() != null )
if( !imports.contains( info.getConverter().getName() ) ){
sb.append( "import " + info.getConverter().getName() + ";" + "\n" );
imports.add( info.getConverter().getName() );
}
for (Class toImport : info.getToImport()) {
if( !imports.contains( toImport.getName() ) ){
sb.append( "import " + toImport.getName() + ";" + "\n" );
imports.add( toImport.getName() );
}
}
}
private void generateTests( String transport, String tpkg, String ppkg, String spkg, Entity entity, Definition def) throws IOException, BrillienException {
String apiVersion = def.getMetaInfo().getApiVersion();
String brillienVersion = apiVersion.endsWith("-SNAPSHOT") ? apiVersion.substring(0, apiVersion.indexOf("-SNAPSHOT")) : apiVersion;
String className = StringServices.capitalize( entity.getName() + StringServices.capitalize(transport) + "Tester" );
Class mediatorType = MetaInfo.getMediator(transport);
StringBuilder sb = new StringBuilder();
sb.append( "package " + tpkg + ";" + "\n\n" );
sb.append( "import " + spkg + ".*;" + "\n" );
sb.append( "import " + ppkg + ".*;" + "\n" );
sb.append( "import " + mediatorType.getName() + ";" + "\n" );
sb.append( "import " + JsonServices.class.getName() + ";" + "\n" );
sb.append( "import " + Configuration.class.getName() + ";" + "\n" );
sb.append( "import " + FileInputStream.class.getName() + ";" + "\n" );
sb.append( "import " + IOServices.class.getName() + ";" + "\n" );
sb.append( "import " + InnerMap.class.getName() + ";" + "\n" );
sb.append( "import " + BrillienCommunication.class.getName() + ";" + "\n" );
sb.append( "import " + Communication.class.getName() + ";" + "\n" );
sb.append( "import " + StreamLineException.class.getName() + ";" + "\n" );
sb.append( "import " + BrillienException.class.getName() + ";" + "\n" );
sb.append( "import " + PatternMatcher.class.getName() + ";" + "\n" );
sb.append( "import " + List.class.getName() + ";" + "\n" );
sb.append( "import " + Map.class.getName() + ";" + "\n" );
sb.append( "import org.junit.BeforeClass;\n" );
sb.append( "import org.junit.Test;\n" );
sb.append( "import org.junit.Ignore;\n" );
sb.append( "import org.junit.AfterClass;\n" );
sb.append( "\npublic class " + className + " {" + "\n" );
sb.append( "\n\n\tprotected static String configPath = \"To be filled...\";" + "\n" );
sb.append( "\n\n\tprotected static " + mediatorType.getSimpleName() + " mediator;" + "\n" );
sb.append( "\n\n\t@BeforeClass" );
sb.append( "\n\tpublic static void initTests () throws BrillienException{" );
sb.append( "\n\t\ttry {" );
sb.append( "\n\t\t\tConfiguration configuration = JsonServices.parseJSON(IOServices.getStreamAsString(new FileInputStream( configPath )), Configuration.class);" );
sb.append( "\n\t\t\tmediator = configuration.create"+ mediatorType.getSimpleName() +"();" );
sb.append( "\n\t\t} catch (Exception e) {" );
sb.append( "\n\t\t\tthrow new BrillienException( StreamLineException.ERROR_INTERNAL_ERROR, \"Cannot read config file.\", e );" );
sb.append( "\n\t\t}" );
sb.append( "\n\t}" );
sb.append( "\n\n\t@AfterClass" );
sb.append( "\n\tpublic static void destructTests ( ) throws BrillienException{" );
sb.append( "\n\t\tmediator.disconnect();" );
sb.append( "\n\t}" );
for (Service service : entity.getServices()) {
TypeInfo sinfo = getType( service.getReturnType() );
sb.append( "\n\n\t@Test" );
sb.append( "\n\t@Ignore" );
sb.append( "\n\tpublic void test" + StringServices.capitalize(service.getName()) + " () throws StreamLineException, BrillienException{" + "" );
for (int i=0; i( " );
for (int i=0; i 0 )
sb.delete( sb.length()-2, sb.length() );
sb.append("\n\t\t) );");
sb.append( "\n\t\t"+ "BrillienCommunication responseComm = mediator.sendCommunication( comm );" );
sb.append( "\n\t\t"+ sinfo.getPrintName() +" response = responseComm.acquireResponse( " +
(sinfo.getContainers().size() == 0
? sinfo.getTypeName()+".class"
: sinfo.getContainers().get( sinfo.getContainers().size()-1 ).getSimpleName()+".class, PatternMatcher.createEncapsulationMatcher(\""+ sinfo.getContainerPattern() +"\"), " + sinfo.getTypeName() + ".class")
+ ");"
);
sb.append( "\n\t\tSystem.out.println( \"Response::\"+ response );" );
sb.append( "\n\t}" + "\n" );
}
sb.append( "\n}" + "\n" );
IOServices.writeToFile(sb.toString(),
srcDirectory.getAbsolutePath() + File.separator + "test" + File.separator + "java" + File.separator +
tpkg.replaceAll("\\.", File.separator) + File.separator + className + ".java"
);
}
private void setVersion( String appName, String apiVersion ) throws IOException, BrillienException {
String brillienVersion = apiVersion.endsWith("-SNAPSHOT") ? apiVersion.substring(0, apiVersion.indexOf("-SNAPSHOT")) : apiVersion;
String configFile = IOServices.getStreamAsString( IOServices.getInputStream(
srcDirectory.getAbsolutePath() + File.separator + "main" + File.separator + BrillienStatics.PRESENCE_CONFIG_FILE
) );
getLog().info("Refining config file:" + srcDirectory.getAbsolutePath() + File.separator + "main" + File.separator + BrillienStatics.PRESENCE_CONFIG_FILE);
configFile = configFile.replaceAll( "\"applicationVersion.*\\,", "\"applicationVersion\": \"" + brillienVersion + BrillienStatics.VERSION_SEPARATOR + "\\$SYSTEM_TIME\",");
IOServices.writeToFile(configFile,
srcDirectory.getAbsolutePath() + File.separator + "main" + File.separator + BrillienStatics.PRESENCE_CONFIG_FILE
);
String mavenVersion = apiVersion.contains("SNAPSHOT") ? apiVersion : apiVersion + "-SNAPSHOT";
String pomFile = IOServices.getStreamAsString( IOServices.getInputStream(
projectDirectory.getAbsolutePath() + File.separator + "pom.xml"
) );
getLog().info("Refining pom file:" + projectDirectory.getAbsolutePath() + File.separator + "pom.xml" );
pomFile = pomFile.replaceAll( "\\[\\p{Alnum}\\-\\_]+\\<\\/version\\>", "" + mavenVersion +" ");
pomFile = pomFile.replaceAll( "\\[\\p{Alnum}\\-\\_]+\\<\\/finalName\\>", "" + appName + "-" + mavenVersion +" ");
IOServices.writeToFile( pomFile, projectDirectory.getAbsolutePath() + File.separator + "pom.xml" );
}
private void generateServiceClient( String transport, String cpkg, String spkg, Entity entity, Definition def) throws IOException, BrillienException {
String apiVersion = def.getMetaInfo().getApiVersion();
String brillienVersion = apiVersion.endsWith("-SNAPSHOT") ? apiVersion.substring(0, apiVersion.indexOf("-SNAPSHOT")) : apiVersion;
String className = StringServices.capitalize( entity.getName() + StringServices.capitalize(transport) + "Client" );
Class mediatorType = MetaInfo.getMediator(transport);
StringBuilder sb = new StringBuilder();
sb.append( "package " + cpkg + ";" + "\n\n" );
sb.append( "import " + spkg + ".*;" + "\n" );
sb.append( "import " + mediatorType.getName() + ";" + "\n" );
sb.append( "import " + JsonServices.class.getName() + ";" + "\n" );
sb.append( "import " + Configuration.class.getName() + ";" + "\n" );
sb.append( "import " + FileInputStream.class.getName() + ";" + "\n" );
sb.append( "import " + IOServices.class.getName() + ";" + "\n" );
sb.append( "import " + InnerMap.class.getName() + ";" + "\n" );
sb.append( "import " + BrillienCommunication.class.getName() + ";" + "\n" );
sb.append( "import " + Communication.class.getName() + ";" + "\n" );
sb.append( "import " + StreamLineException.class.getName() + ";" + "\n" );
sb.append( "import " + BrillienException.class.getName() + ";" + "\n" );
sb.append( "import " + PatternMatcher.class.getName() + ";" + "\n" );
sb.append( "import " + List.class.getName() + ";" + "\n" );
sb.append( "import " + Map.class.getName() + ";" + "\n" );
sb.append( "\npublic class " + className + " {" + "\n" );
sb.append( "\n\n\tprotected " + mediatorType.getSimpleName() + " mediator;" + "\n" );
sb.append( "\n\n\tpublic " + className + "(String configPath) throws BrillienException{" );
sb.append( "\n\t\ttry {" );
sb.append( "\n\t\t\tConfiguration configuration = JsonServices.parseJSON(IOServices.getStreamAsString(new FileInputStream( configPath )), Configuration.class);" );
sb.append( "\n\t\t\tmediator = configuration.create"+ mediatorType.getSimpleName() +"();" );
sb.append( "\n\t\t} catch (Exception e) {" );
sb.append( "\n\t\t\tthrow new BrillienException( StreamLineException.ERROR_INTERNAL_ERROR, \"Cannot read config file.\", e );" );
sb.append( "\n\t\t}" );
sb.append( "\n\t}" );
for (Service service : entity.getServices()) {
TypeInfo sinfo = getType( service.getReturnType() );
sb.append( "\n\tpublic void call" + StringServices.capitalize(service.getName()) + " ( " );
for (int i=0; i 0 )
sb.delete( sb.length()-2, sb.length() );
sb.append( " ) throws StreamLineException, BrillienException{" + "" );
sb.append( "\n\t\tBrillienCommunication comm = mediator.prepareNewCommunication( \"" + StringServices.capitalize( entity.getName() ) + "\", \"" + brillienVersion + "\", \"" + service.getName() +"\", Communication.TYPE_GET, new InnerMap( " );
for (int i=0; i 0 )
sb.delete( sb.length()-2, sb.length() );
sb.append("\n\t\t) );");
sb.append( "\n\t\t"+ "BrillienCommunication responseComm = mediator.sendCommunication( comm );" );
sb.append( "\n\t\t"+ sinfo.getPrintName() +" response = responseComm.acquireResponse( " +
(sinfo.getContainers().size() == 0
? sinfo.getTypeName()+".class"
: sinfo.getContainers().get( sinfo.getContainers().size()-1 ).getSimpleName()+".class, PatternMatcher.createEncapsulationMatcher(\""+ sinfo.getContainerPattern() +"\"), " + sinfo.getTypeName() + ".class")
+ ");"
);
sb.append( "\n\t\tSystem.out.println( \"Response::\"+ response );" );
sb.append( "\n\t}" + "\n" );
}
sb.append( "\n}" + "\n" );
IOServices.delete( new File( srcDirectory.getAbsolutePath() + File.separator + "main" + File.separator + "java" + File.separator +
cpkg.replaceAll("\\.", File.separator) + File.separator + className + ".java" )
);
IOServices.writeToFile(sb.toString(),
srcDirectory.getAbsolutePath() + File.separator + "main" + File.separator + "java" + File.separator +
cpkg.replaceAll("\\.", File.separator) + File.separator + className + ".java"
);
}
private void generateServiceType(String ppkg, String spkg, Entity entity, Definition def) throws IOException, BrillienException {
String apiVersion = def.getMetaInfo().getApiVersion();
String brillienVersion = apiVersion.endsWith("-SNAPSHOT") ? apiVersion.substring(0, apiVersion.indexOf("-SNAPSHOT")) : apiVersion;
String className = StringServices.capitalize( entity.getName() );
StringBuilder sb = new StringBuilder();
sb.append( "package " + ppkg + ";" + "\n\n" );
sb.append( "import " + spkg + ".*;" + "\n" );
sb.append( "import com.vii.brillien.core.component.SuperPresence;" + "\n" );
sb.append( "import com.vii.brillien.kernel.BrillienException;" + "\n" );
sb.append( "import com.vii.brillien.kernel.annotations.PresenceService;" + "\n" );
sb.append( "import com.vii.brillien.kernel.annotations.lifecycle.Resident;" + "\n" );
sb.append( "import com.vii.brillien.kernel.annotations.sso.Authenticator;" + "\n" );
sb.append( "import com.vii.brillien.kernel.annotations.apikey.Revealed;" + "\n" );
sb.append( "import com.vii.brillien.kernel.annotations.NullResponseAllowed;" + "\n" );
sb.append( "import com.vii.streamline.annotation.P;" + "\n" );
List imports = new InnerList();
for (Service service : entity.getServices() ) {
TypeInfo sinfo = getType( service.getReturnType() );
manageImport( sinfo, imports, sb );
for (Parameter parameter : service.getParameters()) {
TypeInfo tinfo = getType( parameter.getType() );
manageImport( tinfo, imports, sb );
}
}
sb.append( "\n@PresenceService( logLevel = PresenceService." + (entity.getLogLevel()==null?"INFO":entity.getLogLevel()) + " )" );
sb.append( "\n@Resident" );
if(entity.getAuthenticator() != null && entity.getAuthenticator())
sb.append( "\n@Authenticator" );
if(entity.getRevealed() != null && entity.getRevealed())
sb.append( "\n@Revealed" );
sb.append( "\npublic class " + className + " extends SuperPresence{" + "\n" );
sb.append( "\n\t{");
if( entity.getNeedToSystemLog() != null && entity.getNeedToSystemLog() )
sb.append( "\n\tneedToSystemLog = true;");
sb.append( "\n\t}\n" );
for (Service service : entity.getServices()) {
if(service.getAuthenticator() != null && service.getAuthenticator())
sb.append( "\n\t@Authenticator" );
if(service.getRevealed() != null && service.getRevealed())
sb.append( "\n\t@Revealed" );
if(service.getNullResponseAllowed() != null && service.getNullResponseAllowed())
sb.append( "\n\t@NullResponseAllowed" );
TypeInfo returnType = getType( service.getReturnType() );
sb.append( "\n\tpublic " + returnType.getPrintName() + " " + service.getName() + "( ");
boolean first = true;
for (Parameter parameter : service.getParameters()) {
TypeInfo paramType = getType(parameter.getType());
sb.append( (first?"":", ") + "@P(name = \"" + parameter.getName() + "\")" + paramType.getPrintName() + " " + parameter.getName());
first = false;
}
sb.append( " ) throws BrillienException {" );
sb.append( "\n\t\treturn null;" );
sb.append( "\n\t}" );
}
sb.append( "\n}" + "\n" );
IOServices.delete( new File( srcDirectory.getAbsolutePath() + File.separator + "main" + File.separator + "java" + File.separator +
ppkg.replaceAll("\\.", File.separator) + File.separator + className + ".java" )
);
IOServices.writeToFile(sb.toString(),
srcDirectory.getAbsolutePath() + File.separator + "main" + File.separator + "java" + File.separator +
ppkg.replaceAll("\\.", File.separator) + File.separator + className + ".java"
);
}
private void generateJavaType(String packageName, Structure structure) throws IOException, BrillienException {
String className = StringServices.capitalize( structure.getName() );
StringBuilder sb = new StringBuilder();
StringBuilder fsb = new StringBuilder();
StringBuilder msb = new StringBuilder();
sb.append( "package " + packageName + ";" + "\n\n" );
sb.append( "import " + JSONProperty.class.getName() + ";" + "\n" );
List imports = new InnerList();
for (String fieldName : structure.getAttributes().keySet()) {
TypeInfo info = getType( structure.getAttributes().get(fieldName) );
manageImport( info, imports, sb );
}
sb.append( "\npublic class " + className + "{" + "\n" );
for (String fieldName : structure.getAttributes().keySet()) {
TypeInfo info = getType( structure.getAttributes().get(fieldName) );
String f = StringServices.capitalize( fieldName );
fsb.append("\n\tprivate " + info.getPrintName() + " " + fieldName + ";");
msb.append("\n\t@JSONProperty( ignoreIfNull = true )" );
if( info.getConverter() != null )
msb.append("\n\t@JSONConverter( type="+info.getConverter().getSimpleName()+".class )");
msb.append("\n\tpublic "+ info.getPrintName() +" get" + f + "( ){" );
msb.append("\n\t\treturn "+ fieldName +";" );
msb.append("\n\t}\n" );
if( info.getContainers().size()>0 && !info.isJsonType() )
msb.append("\n\t@JSONTypeHint( "+info.getTypeName()+".class )");
msb.append("\n\tpublic void set" + f + "( "+ info.getPrintName() + " " + fieldName +" ){" );
msb.append("\n\t\tthis."+ fieldName + " = " + fieldName +";" );
msb.append("\n\t}\n");
}
sb.append( fsb + "\n" );
sb.append( msb );
sb.append( "\n}" + "\n" );
IOServices.writeToFile(sb.toString(),
srcDirectory.getAbsolutePath() + File.separator + "main" + File.separator + "java" + File.separator +
packageName.replaceAll("\\.", File.separator) + File.separator + className + ".java"
);
}
private void generateJsonFrom(File jsonFile) throws MojoExecutionException {
try {
Definition def = JsonServices.parseJSON(IOServices.getStreamAsString( new FileInputStream(jsonFile)), Definition.class );
getLog().info( "Definition mapped." );
if( def.getMetaInfo() == null || def.getMetaInfo().getPackageName() == null || def.getMetaInfo().getPackageName().length() == 0 || !RegularServices.matches(def.getMetaInfo().getPackageName(),"\\p{L}+(\\.\\p{L}+)*") )
throw new BrillienException(StreamLineException.ERROR_DATA_ERROR, "Proper Java-conform packagename need to be given.");
String packageName = def.getMetaInfo().getPackageName();
String apiVersion = def.getMetaInfo().getApiVersion();
if( apiVersion != null )
setVersion( def.getMetaInfo().getAppName(), apiVersion );
String tpkg = packageName + ".test";
String spkg = packageName + ".spec";
String ppkg = packageName + ".presence";
String cpkg = packageName + ".client";
for (Structure structure: def.getStructures()) {
getLog().info( "Generating Java structure: " + structure.getName() );
generateJavaType( spkg, structure );
}
for (Entity entity : def.getEntities()) {
if( def.getMetaInfo().getGeneratePresence() != null && def.getMetaInfo().getGeneratePresence() ){
getLog().info( "Generating Presence: " + entity.getName() );
generateServiceType( ppkg, spkg, entity, def );
}
if( def.getMetaInfo().getGenerateClient() != null && def.getMetaInfo().getGenerateClient() ){
for (String transport : def.getMetaInfo().getTransports()) {
getLog().info( "Generating client: " + entity.getName() );
generateServiceClient( transport, cpkg, spkg, entity, def);
}
}
if( def.getMetaInfo().getGenerateTests() != null && def.getMetaInfo().getGenerateTests() ){
for (String transport : def.getMetaInfo().getTransports()) {
getLog().info( "Generating Test for Presence: " + entity.getName() );
generateTests( transport, tpkg, ppkg, spkg, entity, def );
}
}
}
getLog().info( "Definition processed." );
} catch (BrillienException e) {
throw new MojoExecutionException( e.getMessage(), e );
} catch (Exception e) {
throw new MojoExecutionException( "Invalid JSON structure", e );
}
}
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
getLog().info("Directory where to start: " + jsonFile );
if( jsonFile!=null && jsonFile.exists() && jsonFile.getName().endsWith(".json") ){
getLog().info("Found file to process: " + jsonFile.getAbsolutePath() );
generateJsonFrom(jsonFile);
} else
throw new MojoFailureException("A proper json file need to be set to be parsed.");
getLog().info( "Done." );
}
/*
public static void main(String[] args) throws MojoExecutionException, MojoFailureException, IOException {
JsonMappingGenerator gen = new JsonMappingGenerator();
gen.setJsonFile(new File("./definitions.json"));
gen.setSrcDirectory( new File("/Ruse/JAges/patronus-services/provider/src") );
gen.execute();
}
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy