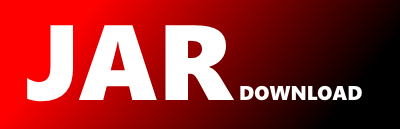
org.broadleafcommerce.common.config.RuntimeEnvironmentPropertiesConfigurer Maven / Gradle / Ivy
Show all versions of broadleaf-common Show documentation
/*
* Copyright 2008-2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.broadleafcommerce.common.config;
import org.apache.commons.collections.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.broadleafcommerce.common.logging.SupportLogManager;
import org.broadleafcommerce.common.logging.SupportLogger;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.config.ConfigurableListableBeanFactory;
import org.springframework.beans.factory.config.PropertyPlaceholderConfigurer;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.FileSystemResource;
import org.springframework.core.io.Resource;
import org.springframework.util.PropertyPlaceholderHelper;
import org.springframework.util.StringValueResolver;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.Set;
/**
* A property resource configurer that chooses the property file at runtime
* based on the runtime environment.
*
* Used for choosing properties files based on the current runtime environment,
* allowing for movement of the same application between multiple runtime
* environments without rebuilding.
*
* The property replacement semantics of this implementation are identical to
* PropertyPlaceholderConfigurer, from which this class inherits.
*
* <bean id="propertyConfigurator" class="frilista.framework.RuntimeEnvironmentPropertiesConfigurer">
* <property name="propertyLocation" value="/WEB-INF/runtime-properties/" />
* <property name="environments">
* <set>
* <value>production</value>
* <value>staging</value>
* <value>integration</value>
* <value>development</value>
* </set>
* </property>
* <property name="defaultEnvironment" value="development"/>
* </bean>
*
The keys of the environment specific properties files are
* compared to ensure that each property file defines the complete set of keys,
* in order to avoid environment-specific failures.
*
* An optional RuntimeEnvironmentKeyResolver implementation can be provided,
* allowing for customization of how the runtime environment is determined. If
* no implementation is provided, a default of
* SystemPropertyRuntimeEnvironmentKeyResolver is used (which uses the system
* property 'runtime.environment')
* @author Chris Lee
*/
public class RuntimeEnvironmentPropertiesConfigurer extends PropertyPlaceholderConfigurer implements InitializingBean {
private static final Log LOG = LogFactory.getLog(RuntimeEnvironmentPropertiesConfigurer.class);
protected SupportLogger logger = SupportLogManager.getLogger("UserOverride", this.getClass());
protected static final String SHARED_PROPERTY_OVERRIDE = "property-shared-override";
protected static final String PROPERTY_OVERRIDE = "property-override";
protected static Set