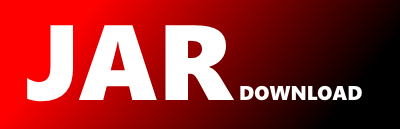
org.broadleafcommerce.common.email.service.EmailTrackingManagerImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of broadleaf-common Show documentation
Show all versions of broadleaf-common Show documentation
A collection of classes shared by broadleaf profile, cms, admin, and core.
/*
* Copyright 2008-2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.broadleafcommerce.common.email.service;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.broadleafcommerce.common.email.dao.EmailReportingDao;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Iterator;
import java.util.Map;
/**
* @author jfischer
*/
@Service("blEmailTrackingManager")
public class EmailTrackingManagerImpl implements EmailTrackingManager {
private static final Log LOG = LogFactory.getLog(EmailTrackingManagerImpl.class);
@Resource(name = "blEmailReportingDao")
protected EmailReportingDao emailReportingDao;
public Long createTrackedEmail(String emailAddress, String type, String extraValue) {
return emailReportingDao.createTracking(emailAddress, type, extraValue);
}
public void recordClick(Long emailId, Map parameterMap, String customerId, Map extraValues) {
if (LOG.isDebugEnabled()) {
LOG.debug("recordClick() => Click detected for Email[" + emailId + "]");
}
Iterator keys = parameterMap.keySet().iterator();
// clean up and normalize the query string
ArrayList queryParms = new ArrayList();
while (keys.hasNext()) {
String p = keys.next();
// exclude email_id from the parms list
if (!p.equals("email_id")) {
queryParms.add(p);
}
}
String newQuery = null;
if (!queryParms.isEmpty()) {
String[] p = queryParms.toArray(new String[queryParms.size()]);
Arrays.sort(p);
StringBuffer newQueryParms = new StringBuffer();
for (int cnt = 0; cnt < p.length; cnt++) {
newQueryParms.append(p[cnt]);
newQueryParms.append("=");
newQueryParms.append(parameterMap.get(p[cnt]));
if (cnt != p.length - 1) {
newQueryParms.append("&");
}
}
newQuery = newQueryParms.toString();
}
emailReportingDao.recordClick(emailId, customerId, extraValues.get("requestUri"), newQuery);
}
/*
* (non-Javadoc)
* @see
* com.containerstore.web.task.service.EmailTrackingManager#recordOpen(java
* .lang.String, javax.servlet.http.HttpServletRequest)
*/
public void recordOpen(Long emailId, Map extraValues) {
if (LOG.isDebugEnabled()) {
LOG.debug("Recording open for email id: " + emailId);
}
// extract necessary information from the request and record the open
emailReportingDao.recordOpen(emailId, extraValues.get("userAgent"));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy