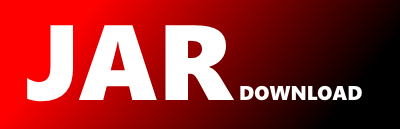
org.broadleafcommerce.common.email.service.info.EmailInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of broadleaf-common Show documentation
Show all versions of broadleaf-common Show documentation
A collection of classes shared by broadleaf profile, cms, admin, and core.
/*
* Copyright 2008-2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.broadleafcommerce.common.email.service.info;
import org.broadleafcommerce.common.email.service.message.Attachment;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
/**
* @author jfischer
*
*/
public class EmailInfo implements Serializable {
private static final long serialVersionUID = 1L;
private String emailType;
private String emailTemplate;
private String subject;
private String fromAddress;
private String messageBody;
private List attachments = new ArrayList();
private String sendEmailReliableAsync;
private String sendAsyncPriority;
/**
* @return the emailType
*/
public String getEmailType() {
return emailType;
}
/**
* @param emailType the emailType to set
*/
public void setEmailType(String emailType) {
this.emailType = emailType;
}
/**
* @return the emailTemplate
*/
public String getEmailTemplate() {
return emailTemplate;
}
/**
* @param emailTemplate the emailTemplate to set
*/
public void setEmailTemplate(String emailTemplate) {
this.emailTemplate = emailTemplate;
}
/**
* @return the subject
*/
public String getSubject() {
return subject;
}
/**
* @param subject the subject to set
*/
public void setSubject(String subject) {
this.subject = subject;
}
/**
* @return the fromAddress
*/
public String getFromAddress() {
return fromAddress;
}
/**
* @param fromAddress the fromAddress to set
*/
public void setFromAddress(String fromAddress) {
this.fromAddress = fromAddress;
}
/**
* @return the sendEmailReliableAsync
*/
public String getSendEmailReliableAsync() {
return sendEmailReliableAsync;
}
/**
* @param sendEmailReliableAsync the sendEmailReliableAsync to set
*/
public void setSendEmailReliableAsync(String sendEmailReliableAsync) {
this.sendEmailReliableAsync = sendEmailReliableAsync;
}
/**
* @return the sendAsyncPriority
*/
public String getSendAsyncPriority() {
return sendAsyncPriority;
}
/**
* @param sendAsyncPriority the sendAsyncPriority to set
*/
public void setSendAsyncPriority(String sendAsyncPriority) {
this.sendAsyncPriority = sendAsyncPriority;
}
public String getMessageBody() {
return messageBody;
}
public void setMessageBody(String messageBody) {
this.messageBody = messageBody;
}
public List getAttachments() {
return attachments;
}
public void setAttachments(List attachments) {
this.attachments = attachments;
}
public synchronized EmailInfo clone() {
EmailInfo info = new EmailInfo();
info.setAttachments(attachments);
info.setEmailTemplate(emailTemplate);
info.setEmailType(emailType);
info.setFromAddress(fromAddress);
info.setMessageBody(messageBody);
info.setSendAsyncPriority(sendAsyncPriority);
info.setSendEmailReliableAsync(sendEmailReliableAsync);
info.setSubject(subject);
return info;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy