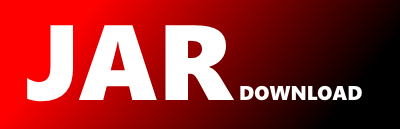
org.broadleafcommerce.common.persistence.EntityConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of broadleaf-common Show documentation
Show all versions of broadleaf-common Show documentation
A collection of classes shared by broadleaf profile, cms, admin, and core.
/*
* Copyright 2008-2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.broadleafcommerce.common.persistence;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.context.support.GenericXmlApplicationContext;
import org.springframework.core.io.Resource;
import org.springframework.stereotype.Component;
import javax.annotation.PostConstruct;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.Set;
@Component("blEntityConfiguration")
public class EntityConfiguration implements ApplicationContextAware {
private static final Log LOG = LogFactory.getLog(EntityConfiguration.class);
private ApplicationContext webApplicationContext;
private final HashMap> entityMap = new HashMap>(50);
private ApplicationContext applicationcontext;
private Resource[] entityContexts;
@javax.annotation.Resource(name="blMergedEntityContexts")
protected Set mergedEntityContexts;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
this.webApplicationContext = applicationContext;
}
@PostConstruct
public void configureMergedItems() {
Set temp = new LinkedHashSet();
if (mergedEntityContexts != null && !mergedEntityContexts.isEmpty()) {
for (String location : mergedEntityContexts) {
temp.add(webApplicationContext.getResource(location));
}
}
if (entityContexts != null) {
for (Resource resource : entityContexts) {
temp.add(resource);
}
}
entityContexts = temp.toArray(new Resource[temp.size()]);
applicationcontext = new GenericXmlApplicationContext(entityContexts);
}
public Class> lookupEntityClass(String beanId) {
Class> clazz;
if (entityMap.containsKey(beanId)) {
clazz = entityMap.get(beanId);
} else {
Object object = applicationcontext.getBean(beanId);
clazz = object.getClass();
entityMap.put(beanId, clazz);
}
if (LOG.isDebugEnabled()) {
LOG.debug("Returning class (" + clazz.getName() + ") configured with bean id (" + beanId + ')');
}
return clazz;
}
public String[] getEntityBeanNames() {
return applicationcontext.getBeanDefinitionNames();
}
public Class lookupEntityClass(String beanId, Class resultClass) {
Class clazz;
if (entityMap.containsKey(beanId)) {
clazz = (Class) entityMap.get(beanId);
} else {
Object object = applicationcontext.getBean(beanId);
clazz = (Class) object.getClass();
entityMap.put(beanId, clazz);
}
if (LOG.isDebugEnabled()) {
LOG.debug("Returning class (" + clazz.getName() + ") configured with bean id (" + beanId + ')');
}
return clazz;
}
public Object createEntityInstance(String beanId) {
Object bean = applicationcontext.getBean(beanId);
if (LOG.isDebugEnabled()) {
LOG.debug("Returning instance of class (" + bean.getClass().getName() + ") configured with bean id (" + beanId + ')');
}
return bean;
}
public T createEntityInstance(String beanId, Class resultClass) {
T bean = (T) applicationcontext.getBean(beanId);
if (LOG.isDebugEnabled()) {
LOG.debug("Returning instance of class (" + bean.getClass().getName() + ") configured with bean id (" + beanId + ')');
}
return bean;
}
public Resource[] getEntityContexts() {
return entityContexts;
}
public void setEntityContexts(Resource[] entityContexts) {
this.entityContexts = entityContexts;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy