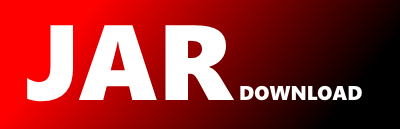
org.broadleafcommerce.cms.admin.web.controller.AdminAssetUploadController Maven / Gradle / Ivy
/*
* Copyright 2008-2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.broadleafcommerce.cms.admin.web.controller;
import org.broadleafcommerce.cms.file.domain.ImageStaticAssetImpl;
import org.broadleafcommerce.cms.file.domain.StaticAsset;
import org.broadleafcommerce.cms.file.service.StaticAssetService;
import org.broadleafcommerce.cms.file.service.StaticAssetStorageService;
import org.broadleafcommerce.common.persistence.EntityConfiguration;
import org.broadleafcommerce.openadmin.server.service.handler.CustomPersistenceHandler;
import org.broadleafcommerce.openadmin.web.controller.AdminAbstractController;
import org.broadleafcommerce.openadmin.web.form.component.ListGrid;
import org.broadleafcommerce.openadmin.web.form.component.ListGrid.Type;
import org.codehaus.jackson.map.ObjectMapper;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.util.MultiValueMap;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* AdminAssetUploadController handles uploading or selecting assets.
*
* Used with entities like {@link SkuImpl} and {@link CategoryImpl} that have {@link CustomPersistenceHandler}
* configurations that provide support for adding maps of Media objects.
*
* @author Brian Polster (bpolster)
*/
@Controller("blAdminAssetUploadController")
@RequestMapping("/{sectionKey}")
public class AdminAssetUploadController extends AdminAbstractController {
@Resource(name = "blEntityConfiguration")
protected EntityConfiguration entityConfiguration;
@Resource(name = "blStaticAssetStorageService")
protected StaticAssetStorageService staticAssetStorageService;
@Resource(name = "blStaticAssetService")
protected StaticAssetService staticAssetService;
@Resource(name = "blAdminAssetController")
protected AdminAssetController assetController;
@RequestMapping(value = "/{id}/chooseAsset", method = RequestMethod.GET)
public String chooseMediaForMapKey(HttpServletRequest request, HttpServletResponse response, Model model,
@PathVariable(value = "sectionKey") String sectionKey,
@PathVariable(value = "id") String id,
@RequestParam MultiValueMap requestParams) throws Exception {
Map pathVars = new HashMap();
pathVars.put("sectionKey", AdminAssetController.SECTION_KEY);
assetController.viewEntityList(request, response, model, pathVars, requestParams);
ListGrid listGrid = (ListGrid) model.asMap().get("listGrid");
listGrid.setPathOverride("/" + sectionKey + "/" + id + "/chooseAsset");
listGrid.setListGridType(Type.ASSET);
String userAgent = request.getHeader("User-Agent");
model.addAttribute("isIE", userAgent.contains("MSIE"));
model.addAttribute("viewType", "modal/selectAsset");
model.addAttribute("currentUrl", request.getRequestURL().toString());
model.addAttribute("modalHeaderType", "selectAsset");
model.addAttribute("currentParams", new ObjectMapper().writeValueAsString(requestParams));
// We need these attributes to be set appropriately here
model.addAttribute("entityId", id);
model.addAttribute("sectionKey", sectionKey);
return "modules/modalContainer";
}
@RequestMapping(value = "/{id}/uploadAsset", method = RequestMethod.POST)
public ResponseEntity
© 2015 - 2024 Weber Informatics LLC | Privacy Policy