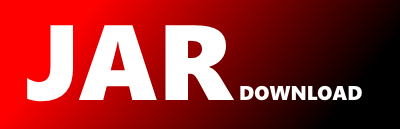
org.brutusin.rpc.actions.DynamicSchemaProviderActionHelper Maven / Gradle / Ivy
/*
* Copyright 2015 Ignacio del Valle Alles [email protected].
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.brutusin.rpc.actions;
import java.util.HashMap;
import java.util.Map;
import org.brutusin.rpc.RpcContext;
import org.brutusin.json.spi.Expression;
import org.brutusin.json.spi.JsonCodec;
import org.brutusin.json.spi.JsonSchema;
import org.brutusin.json.DynamicSchemaProvider;
import org.brutusin.rpc.RpcAction;
import org.brutusin.rpc.RpcService;
import org.brutusin.rpc.http.HttpAction;
/**
*
* @author Ignacio del Valle Alles [email protected]
*/
public class DynamicSchemaProviderActionHelper {
public static Map execute(DynamicSchemaProviderInput input, Map> services) throws Exception {
if (input.getId() == null) {
throw new IllegalArgumentException("Service id is required");
}
RpcService service = services.get(input.getId());
if (service == null) {
throw new IllegalArgumentException("Invalid service id '" + input.getId() + "'");
}
DynamicSchemaProvider schemaProvider;
if (DynamicSchemaProvider.class.isAssignableFrom(service.getInputClass())) {
schemaProvider = (DynamicSchemaProvider) service.getInputClass().newInstance();
} else {
schemaProvider = null;
}
return getVariableSchemas(schemaProvider, service.getInputClass(), input);
}
private static Map getVariableSchemas(DynamicSchemaProvider schemaProvider, Class inputClass, DynamicSchemaProviderInput input) {
Map ret = new HashMap();
for (String fieldName : input.getFieldNames()) {
if (!ret.containsKey(fieldName)) {
if (schemaProvider == null) {
Expression exp = JsonCodec.getInstance().compile(fieldName);
JsonSchema projectedSchema = exp.projectSchema(JsonCodec.getInstance().getSchema(inputClass));
ret.put(fieldName, projectedSchema);
} else {
if (fieldName.equals("$")) {
ret.put(fieldName, JsonCodec.getInstance().getSchema(inputClass));
} else {
ret.put(fieldName, schemaProvider.getDynamicSchema(fieldName, input.getInput()));
}
}
}
}
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy