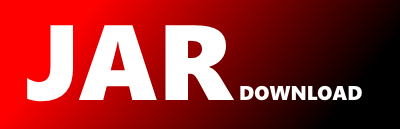
org.bsc.bean.BeanManagerUtils Maven / Gradle / Ivy
package org.bsc.bean;
import static org.bsc.bean.PropertyDescriptorField.DEFAULT_VALUE;
import java.beans.BeanDescriptor;
import java.beans.BeanInfo;
import java.beans.Beans;
import java.beans.EventSetDescriptor;
import java.beans.MethodDescriptor;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Method;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.sql.SQLException;
import java.sql.Types;
import java.util.Collection;
import java.util.Collections;
import java.util.Enumeration;
import org.bsc.bean.dyna.DynaPropertyDescriptor;
import org.bsc.bean.dyna.DynaPropertyDescriptorJoin;
import org.bsc.util.Configurator;
import org.bsc.util.Log;
/**
* Class utility
*
* Title: Bean Manager
* Description: ORM framework
* Copyright: Copyright (c) 2003
* Company:
* @author BARTOLOMEO Sorrentino
* @version 1.0
*/
public class BeanManagerUtils {
public static final String FORMATPATTERN = "formatPattern";
public static final EventSetDescriptor[] EMPTY_EVENTDESCRIPTOR = new EventSetDescriptor[0];
public static final MethodDescriptor[] EMPTY_METHODDESCRIPTOR = new MethodDescriptor[0];
public static final PropertyDescriptor[] EMPTY_PROPERTYDESCRIPTOR = new PropertyDescriptor[0];
public static final BeanInfo[] EMPTY_ADDITIONALBEANINFO = new BeanInfo[0];
public static final BeanManagerMessages messages = Configurator.getMessages();
/**
load a BeanInfo for URI
If uri string start with character '/' the bean info will be loaded using
XmlBeanInfo.loadFromUri factory method, otherwise the uri will be
loaded using Class.forName
@todo implement support of xml using digester
@param loader classloader used for load the resource ( null for default )
@param uri resource uri
*/
static public BeanInfo loadBeanInfo( ClassLoader loader, String uri ) {
if( uri==null ) return null;
BeanInfo result = null;
try {
if( loader==null ) loader = BeanManagerUtils.class.getClassLoader();
if( uri.startsWith("/") ) {
//java.io.InputStream is = loader.getResourceAsStream( uri.substring(1) );
//result = XmlBeanInfo.loadFromUri( new InputSource(is) );
throw new UnsupportedOperationException( "load beanInfo from external source (e.g. xml) is not allowed");
}
else {
Class> beanInfoClass = Class.forName(uri,true,loader);
result = (BeanInfo)beanInfoClass.newInstance();
}
return result;
}
catch( Exception ex ) {
Log.error( "BeanUtil.loadBeanInfo exception", ex );
}
return null;
}
/**
* return an unmodifiable Map that contains only PropertyDescriptorField
*
* @param pp property descriptor array
*
* @see bsc.bean.PropertyDescriptorField
*/
@SuppressWarnings("unchecked")
static public java.util.Map getPropertyFieldMap( PropertyDescriptor [] pp ) {
if(pp==null) return Collections.emptyMap();
try {
java.util.Map fields = new java.util.HashMap(pp.length);
for( int i=0; i beanClass ) {
if( beanClass==null ) return null;
try {
String className = beanClass.getName().concat("BeanInfo");
return loadBeanInfo( loader, className );
}
catch( Exception ex ) {
Log.error( "BeanUtil.getBeanInfo exception", ex );
}
return null;
}
/**
map into Hashtable all the PropertyDescriptor inside BeanInfo
@param beanInfo BeanInfo instance
@see BeanManagerUtils#getBeanInfo
@see java.beans.PropertyDescriptor
*/
static public java.util.Map getProperties( BeanInfo beanInfo ) {
if(beanInfo==null) return null;
try {
PropertyDescriptor [] pp = beanInfo.getPropertyDescriptors();
java.util.Map fields = new java.util.HashMap(pp.length);
for( int i=0; i
Example:
public BeanDescriptor getBeanDescriptor() {
return BeanManagerUtils.createBeanDescriptor( beanClass, "<entity>" );
}
@param beanClass bean Class
@param entityName name of db entity (table)
@return new BeanDescriptor
@see java.beans.BeanDescriptor
@see java.beans.BeanInfo#getBeanDescriptor
*/
public static java.beans.BeanDescriptor createBeanDescriptor( Class> beanClass, String entityName ) {
BeanDescriptor bs = new BeanDescriptor(beanClass);
bs.setValue( "entityName", entityName );
return bs;
}
/**
* return property value
*
*@param property
*@param bean bean instance
*@return property value
*/
public static Object getPropertyValue( PropertyDescriptor p, Object bean ) {
java.lang.reflect.Method get = p.getReadMethod();
try {
return get.invoke( bean );
}
catch( Exception ex ) {
Log.error("getPropertyValue", ex);
}
return null;
}
/**
* get all properties of bean info ( getAdditionalBeanInfo also )
*
* - if there is a name conflict the beanInfo properties have the precedence
*
* @todo implement the join condition(s) inheritance
* @param beanInfo BeanInfo
* @return PropertyDescriptor[] all properties as array
* @Deprecated use aggregateProperties
*/
@Deprecated
public static PropertyDescriptor[] getBeanProperties( BeanInfo beanInfo ) {
if (beanInfo == null) {
throw new java.lang.IllegalArgumentException(getMessage(
"ex.param_0_is_null", new Object[] {"beanInfo"}));
}
java.util.Mapfinal BeanInfo aggregate = new AggregateBeanInfo(); @Override public BeanInfo[] getAdditionalBeanInfo() { return new BeanInfo[] { aggregate }; } @Override public PropertyDescriptor[] getPropertyDescriptors() { return BeanManagerUtils.aggregateProperties( getBeanClass(), super.getPropertyDescriptors(), aggregate ); } ** * * @param beanClass class of the Owner Bean * @param properties properties of the Owner BeanInfo * @param aggregate BeanInfo array * @return */ public static PropertyDescriptor[] aggregateProperties( Class> beanClass, PropertyDescriptor[] properties, BeanInfo...aggregate ) { java.util.Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy