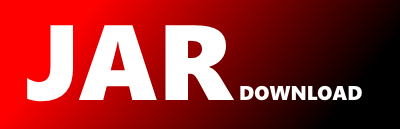
org.bytedeco.javacpp.openblas Maven / Gradle / Ivy
// Targeted by JavaCPP version 1.2.5: DO NOT EDIT THIS FILE
package org.bytedeco.javacpp;
import java.nio.*;
import org.bytedeco.javacpp.*;
import org.bytedeco.javacpp.annotation.*;
public class openblas extends org.bytedeco.javacpp.presets.openblas {
static { Loader.load(); }
// Parsed from openblas_config.h
// #ifndef OPENBLAS_CONFIG_H
// #define OPENBLAS_CONFIG_H
public static final int OPENBLAS_OS_LINUX = 1;
public static final int OPENBLAS_ARCH_X86_64 = 1;
public static final int OPENBLAS_C_GCC = 1;
public static final int OPENBLAS___64BIT__ = 1;
// #define OPENBLAS_PTHREAD_CREATE_FUNC pthread_create
// #define OPENBLAS_BUNDERSCORE _
public static final int OPENBLAS_NEEDBUNDERSCORE = 1;
// #define OPENBLAS_GENERIC
public static final int OPENBLAS_L1_DATA_SIZE = 32768;
public static final int OPENBLAS_L1_DATA_LINESIZE = 128;
public static final int OPENBLAS_L2_SIZE = 512488;
public static final int OPENBLAS_L2_LINESIZE = 128;
public static final int OPENBLAS_DTB_DEFAULT_ENTRIES = 128;
public static final int OPENBLAS_DTB_SIZE = 4096;
public static final int OPENBLAS_L2_ASSOCIATIVE = 8;
// #define OPENBLAS_CORE_generic
public static final String OPENBLAS_CHAR_CORENAME = "generic";
public static final int OPENBLAS_SLOCAL_BUFFER_SIZE = 4096;
public static final int OPENBLAS_DLOCAL_BUFFER_SIZE = 4096;
public static final int OPENBLAS_CLOCAL_BUFFER_SIZE = 8192;
public static final int OPENBLAS_ZLOCAL_BUFFER_SIZE = 8192;
public static final int OPENBLAS_GEMM_MULTITHREAD_THRESHOLD = 4;
public static final String OPENBLAS_VERSION = " OpenBLAS 0.2.19 ";
/*This is only for "make install" target.*/
// #if defined(OPENBLAS_OS_WINNT) || defined(OPENBLAS_OS_CYGWIN_NT) || defined(OPENBLAS_OS_INTERIX)
// #define OPENBLAS_WINDOWS_ABI
// #define OPENBLAS_OS_WINDOWS
// #ifdef DOUBLE
// #define DOUBLE_DEFINED DOUBLE
// #undef DOUBLE
// #endif
// #endif
// #ifdef OPENBLAS_NEEDBUNDERSCORE
// #define BLASFUNC(FUNC) FUNC##_
// #else
// #define BLASFUNC(FUNC) FUNC
// #endif
// #ifdef OPENBLAS_QUAD_PRECISION
// #elif defined OPENBLAS_EXPRECISION
// #else
// #define xdouble double
// #endif
// #if defined(OPENBLAS_OS_WINDOWS) && defined(OPENBLAS___64BIT__)
// #else
// #endif
// #ifdef OPENBLAS_USE64BITINT
// #else
// #endif
// #if defined(XDOUBLE) || defined(DOUBLE)
// #define FLOATRET FLOAT
// #else
// #ifdef NEED_F2CCONV
// #define FLOATRET double
// #else
// #define FLOATRET float
// #endif
// #endif
/* Inclusion of a standard header file is needed for definition of __STDC_*
predefined macros with some compilers (e.g. GCC 4.7 on Linux). This occurs
as a side effect of including either or . */
// #include
/* C99 supports complex floating numbers natively, which GCC also offers as an
extension since version 3.0. If neither are available, use a compatible
structure as fallback (see Clause 6.2.5.13 of the C99 standard). */
// #if ((defined(__STDC_IEC_559_COMPLEX__) || __STDC_VERSION__ >= 199901L ||
// (__GNUC__ >= 3 && !defined(__cplusplus))) && !(defined(FORCE_OPENBLAS_COMPLEX_STRUCT)))
// #define OPENBLAS_COMPLEX_C99
// #ifndef __cplusplus
// #include
// #endif
// #define openblas_make_complex_float(real, imag) ((real) + ((imag) * _Complex_I))
// #define openblas_make_complex_double(real, imag) ((real) + ((imag) * _Complex_I))
// #define openblas_make_complex_xdouble(real, imag) ((real) + ((imag) * _Complex_I))
// #define openblas_complex_float_real(z) (creal(z))
// #define openblas_complex_float_imag(z) (cimag(z))
// #define openblas_complex_double_real(z) (creal(z))
// #define openblas_complex_double_imag(z) (cimag(z))
// #define openblas_complex_xdouble_real(z) (creal(z))
// #define openblas_complex_xdouble_imag(z) (cimag(z))
// #else
// #endif
// #endif /* OPENBLAS_CONFIG_H */
// Parsed from cblas.h
// #ifndef CBLAS_H
// #define CBLAS_H
// #include
// #include "openblas_config.h"
// #ifdef __cplusplus
/* Assume C declarations for C++ */
// #endif /* __cplusplus */
/*Set the number of threads on runtime.*/
/*Get the number of threads on runtime.*/
/*Get the number of physical processors (cores).*/
/*Get the build configure on runtime.*/
/*Get the CPU corename on runtime.*/
/* Get the parallelization type which is used by OpenBLAS */
/* OpenBLAS is compiled for sequential use */
public static final int OPENBLAS_SEQUENTIAL = 0;
/* OpenBLAS is compiled using normal threading model */
public static final int OPENBLAS_THREAD = 1;
/* OpenBLAS is compiled using OpenMP threading model */
public static final int OPENBLAS_OPENMP = 2;
/*
* Since all of GotoBlas was written without const,
* we disable it at build time.
*/
// #ifndef OPENBLAS_CONST
// # define OPENBLAS_CONST const
// #endif
// #define CBLAS_INDEX size_t
/** enum CBLAS_ORDER */
public static final int CblasRowMajor= 101, CblasColMajor= 102;
/** enum CBLAS_TRANSPOSE */
public static final int CblasNoTrans= 111, CblasTrans= 112, CblasConjTrans= 113, CblasConjNoTrans= 114;
/** enum CBLAS_UPLO */
public static final int CblasUpper= 121, CblasLower= 122;
/** enum CBLAS_DIAG */
public static final int CblasNonUnit= 131, CblasUnit= 132;
/** enum CBLAS_SIDE */
public static final int CblasLeft= 141, CblasRight= 142;
public static native float cblas_sdsdot(@Cast("const blasint") long n, float alpha, @Const FloatPointer x, @Cast("const blasint") long incx, @Const FloatPointer y, @Cast("const blasint") long incy);
public static native float cblas_sdsdot(@Cast("const blasint") long n, float alpha, @Const FloatBuffer x, @Cast("const blasint") long incx, @Const FloatBuffer y, @Cast("const blasint") long incy);
public static native float cblas_sdsdot(@Cast("const blasint") long n, float alpha, @Const float[] x, @Cast("const blasint") long incx, @Const float[] y, @Cast("const blasint") long incy);
public static native double cblas_dsdot(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx, @Const FloatPointer y, @Cast("const blasint") long incy);
public static native double cblas_dsdot(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx, @Const FloatBuffer y, @Cast("const blasint") long incy);
public static native double cblas_dsdot(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx, @Const float[] y, @Cast("const blasint") long incy);
public static native float cblas_sdot(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx, @Const FloatPointer y, @Cast("const blasint") long incy);
public static native float cblas_sdot(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx, @Const FloatBuffer y, @Cast("const blasint") long incy);
public static native float cblas_sdot(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx, @Const float[] y, @Cast("const blasint") long incy);
public static native double cblas_ddot(@Cast("const blasint") long n, @Const DoublePointer x, @Cast("const blasint") long incx, @Const DoublePointer y, @Cast("const blasint") long incy);
public static native double cblas_ddot(@Cast("const blasint") long n, @Const DoubleBuffer x, @Cast("const blasint") long incx, @Const DoubleBuffer y, @Cast("const blasint") long incy);
public static native double cblas_ddot(@Cast("const blasint") long n, @Const double[] x, @Cast("const blasint") long incx, @Const double[] y, @Cast("const blasint") long incy);
public static native void cblas_cdotu_sub(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx, @Const FloatPointer y, @Cast("const blasint") long incy, @Cast("openblas_complex_float*") FloatPointer ret);
public static native void cblas_cdotu_sub(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx, @Const FloatBuffer y, @Cast("const blasint") long incy, @Cast("openblas_complex_float*") FloatBuffer ret);
public static native void cblas_cdotu_sub(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx, @Const float[] y, @Cast("const blasint") long incy, @Cast("openblas_complex_float*") float[] ret);
public static native void cblas_cdotc_sub(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx, @Const FloatPointer y, @Cast("const blasint") long incy, @Cast("openblas_complex_float*") FloatPointer ret);
public static native void cblas_cdotc_sub(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx, @Const FloatBuffer y, @Cast("const blasint") long incy, @Cast("openblas_complex_float*") FloatBuffer ret);
public static native void cblas_cdotc_sub(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx, @Const float[] y, @Cast("const blasint") long incy, @Cast("openblas_complex_float*") float[] ret);
public static native void cblas_zdotu_sub(@Cast("const blasint") long n, @Const DoublePointer x, @Cast("const blasint") long incx, @Const DoublePointer y, @Cast("const blasint") long incy, @Cast("openblas_complex_double*") DoublePointer ret);
public static native void cblas_zdotu_sub(@Cast("const blasint") long n, @Const DoubleBuffer x, @Cast("const blasint") long incx, @Const DoubleBuffer y, @Cast("const blasint") long incy, @Cast("openblas_complex_double*") DoubleBuffer ret);
public static native void cblas_zdotu_sub(@Cast("const blasint") long n, @Const double[] x, @Cast("const blasint") long incx, @Const double[] y, @Cast("const blasint") long incy, @Cast("openblas_complex_double*") double[] ret);
public static native void cblas_zdotc_sub(@Cast("const blasint") long n, @Const DoublePointer x, @Cast("const blasint") long incx, @Const DoublePointer y, @Cast("const blasint") long incy, @Cast("openblas_complex_double*") DoublePointer ret);
public static native void cblas_zdotc_sub(@Cast("const blasint") long n, @Const DoubleBuffer x, @Cast("const blasint") long incx, @Const DoubleBuffer y, @Cast("const blasint") long incy, @Cast("openblas_complex_double*") DoubleBuffer ret);
public static native void cblas_zdotc_sub(@Cast("const blasint") long n, @Const double[] x, @Cast("const blasint") long incx, @Const double[] y, @Cast("const blasint") long incy, @Cast("openblas_complex_double*") double[] ret);
public static native float cblas_sasum(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx);
public static native float cblas_sasum(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx);
public static native float cblas_sasum(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx);
public static native double cblas_dasum(@Cast("const blasint") long n, @Const DoublePointer x, @Cast("const blasint") long incx);
public static native double cblas_dasum(@Cast("const blasint") long n, @Const DoubleBuffer x, @Cast("const blasint") long incx);
public static native double cblas_dasum(@Cast("const blasint") long n, @Const double[] x, @Cast("const blasint") long incx);
public static native float cblas_scasum(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx);
public static native float cblas_scasum(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx);
public static native float cblas_scasum(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx);
public static native double cblas_dzasum(@Cast("const blasint") long n, @Const DoublePointer x, @Cast("const blasint") long incx);
public static native double cblas_dzasum(@Cast("const blasint") long n, @Const DoubleBuffer x, @Cast("const blasint") long incx);
public static native double cblas_dzasum(@Cast("const blasint") long n, @Const double[] x, @Cast("const blasint") long incx);
public static native float cblas_snrm2(@Cast("const blasint") long N, @Const FloatPointer X, @Cast("const blasint") long incX);
public static native float cblas_snrm2(@Cast("const blasint") long N, @Const FloatBuffer X, @Cast("const blasint") long incX);
public static native float cblas_snrm2(@Cast("const blasint") long N, @Const float[] X, @Cast("const blasint") long incX);
public static native double cblas_dnrm2(@Cast("const blasint") long N, @Const DoublePointer X, @Cast("const blasint") long incX);
public static native double cblas_dnrm2(@Cast("const blasint") long N, @Const DoubleBuffer X, @Cast("const blasint") long incX);
public static native double cblas_dnrm2(@Cast("const blasint") long N, @Const double[] X, @Cast("const blasint") long incX);
public static native float cblas_scnrm2(@Cast("const blasint") long N, @Const FloatPointer X, @Cast("const blasint") long incX);
public static native float cblas_scnrm2(@Cast("const blasint") long N, @Const FloatBuffer X, @Cast("const blasint") long incX);
public static native float cblas_scnrm2(@Cast("const blasint") long N, @Const float[] X, @Cast("const blasint") long incX);
public static native double cblas_dznrm2(@Cast("const blasint") long N, @Const DoublePointer X, @Cast("const blasint") long incX);
public static native double cblas_dznrm2(@Cast("const blasint") long N, @Const DoubleBuffer X, @Cast("const blasint") long incX);
public static native double cblas_dznrm2(@Cast("const blasint") long N, @Const double[] X, @Cast("const blasint") long incX);
public static native @Cast("size_t") long cblas_isamax(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_isamax(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_isamax(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_idamax(@Cast("const blasint") long n, @Const DoublePointer x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_idamax(@Cast("const blasint") long n, @Const DoubleBuffer x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_idamax(@Cast("const blasint") long n, @Const double[] x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_icamax(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_icamax(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_icamax(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_izamax(@Cast("const blasint") long n, @Const DoublePointer x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_izamax(@Cast("const blasint") long n, @Const DoubleBuffer x, @Cast("const blasint") long incx);
public static native @Cast("size_t") long cblas_izamax(@Cast("const blasint") long n, @Const double[] x, @Cast("const blasint") long incx);
public static native void cblas_saxpy(@Cast("const blasint") long n, float alpha, @Const FloatPointer x, @Cast("const blasint") long incx, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_saxpy(@Cast("const blasint") long n, float alpha, @Const FloatBuffer x, @Cast("const blasint") long incx, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_saxpy(@Cast("const blasint") long n, float alpha, @Const float[] x, @Cast("const blasint") long incx, float[] y, @Cast("const blasint") long incy);
public static native void cblas_daxpy(@Cast("const blasint") long n, double alpha, @Const DoublePointer x, @Cast("const blasint") long incx, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_daxpy(@Cast("const blasint") long n, double alpha, @Const DoubleBuffer x, @Cast("const blasint") long incx, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_daxpy(@Cast("const blasint") long n, double alpha, @Const double[] x, @Cast("const blasint") long incx, double[] y, @Cast("const blasint") long incy);
public static native void cblas_caxpy(@Cast("const blasint") long n, @Const FloatPointer alpha, @Const FloatPointer x, @Cast("const blasint") long incx, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_caxpy(@Cast("const blasint") long n, @Const FloatBuffer alpha, @Const FloatBuffer x, @Cast("const blasint") long incx, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_caxpy(@Cast("const blasint") long n, @Const float[] alpha, @Const float[] x, @Cast("const blasint") long incx, float[] y, @Cast("const blasint") long incy);
public static native void cblas_zaxpy(@Cast("const blasint") long n, @Const DoublePointer alpha, @Const DoublePointer x, @Cast("const blasint") long incx, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_zaxpy(@Cast("const blasint") long n, @Const DoubleBuffer alpha, @Const DoubleBuffer x, @Cast("const blasint") long incx, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_zaxpy(@Cast("const blasint") long n, @Const double[] alpha, @Const double[] x, @Cast("const blasint") long incx, double[] y, @Cast("const blasint") long incy);
public static native void cblas_scopy(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_scopy(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_scopy(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx, float[] y, @Cast("const blasint") long incy);
public static native void cblas_dcopy(@Cast("const blasint") long n, @Const DoublePointer x, @Cast("const blasint") long incx, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_dcopy(@Cast("const blasint") long n, @Const DoubleBuffer x, @Cast("const blasint") long incx, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_dcopy(@Cast("const blasint") long n, @Const double[] x, @Cast("const blasint") long incx, double[] y, @Cast("const blasint") long incy);
public static native void cblas_ccopy(@Cast("const blasint") long n, @Const FloatPointer x, @Cast("const blasint") long incx, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_ccopy(@Cast("const blasint") long n, @Const FloatBuffer x, @Cast("const blasint") long incx, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_ccopy(@Cast("const blasint") long n, @Const float[] x, @Cast("const blasint") long incx, float[] y, @Cast("const blasint") long incy);
public static native void cblas_zcopy(@Cast("const blasint") long n, @Const DoublePointer x, @Cast("const blasint") long incx, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_zcopy(@Cast("const blasint") long n, @Const DoubleBuffer x, @Cast("const blasint") long incx, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_zcopy(@Cast("const blasint") long n, @Const double[] x, @Cast("const blasint") long incx, double[] y, @Cast("const blasint") long incy);
public static native void cblas_sswap(@Cast("const blasint") long n, FloatPointer x, @Cast("const blasint") long incx, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_sswap(@Cast("const blasint") long n, FloatBuffer x, @Cast("const blasint") long incx, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_sswap(@Cast("const blasint") long n, float[] x, @Cast("const blasint") long incx, float[] y, @Cast("const blasint") long incy);
public static native void cblas_dswap(@Cast("const blasint") long n, DoublePointer x, @Cast("const blasint") long incx, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_dswap(@Cast("const blasint") long n, DoubleBuffer x, @Cast("const blasint") long incx, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_dswap(@Cast("const blasint") long n, double[] x, @Cast("const blasint") long incx, double[] y, @Cast("const blasint") long incy);
public static native void cblas_cswap(@Cast("const blasint") long n, FloatPointer x, @Cast("const blasint") long incx, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_cswap(@Cast("const blasint") long n, FloatBuffer x, @Cast("const blasint") long incx, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_cswap(@Cast("const blasint") long n, float[] x, @Cast("const blasint") long incx, float[] y, @Cast("const blasint") long incy);
public static native void cblas_zswap(@Cast("const blasint") long n, DoublePointer x, @Cast("const blasint") long incx, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_zswap(@Cast("const blasint") long n, DoubleBuffer x, @Cast("const blasint") long incx, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_zswap(@Cast("const blasint") long n, double[] x, @Cast("const blasint") long incx, double[] y, @Cast("const blasint") long incy);
public static native void cblas_srot(@Cast("const blasint") long N, FloatPointer X, @Cast("const blasint") long incX, FloatPointer Y, @Cast("const blasint") long incY, float c, float s);
public static native void cblas_srot(@Cast("const blasint") long N, FloatBuffer X, @Cast("const blasint") long incX, FloatBuffer Y, @Cast("const blasint") long incY, float c, float s);
public static native void cblas_srot(@Cast("const blasint") long N, float[] X, @Cast("const blasint") long incX, float[] Y, @Cast("const blasint") long incY, float c, float s);
public static native void cblas_drot(@Cast("const blasint") long N, DoublePointer X, @Cast("const blasint") long incX, DoublePointer Y, @Cast("const blasint") long incY, double c, double s);
public static native void cblas_drot(@Cast("const blasint") long N, DoubleBuffer X, @Cast("const blasint") long incX, DoubleBuffer Y, @Cast("const blasint") long incY, double c, double s);
public static native void cblas_drot(@Cast("const blasint") long N, double[] X, @Cast("const blasint") long incX, double[] Y, @Cast("const blasint") long incY, double c, double s);
public static native void cblas_srotg(FloatPointer a, FloatPointer b, FloatPointer c, FloatPointer s);
public static native void cblas_srotg(FloatBuffer a, FloatBuffer b, FloatBuffer c, FloatBuffer s);
public static native void cblas_srotg(float[] a, float[] b, float[] c, float[] s);
public static native void cblas_drotg(DoublePointer a, DoublePointer b, DoublePointer c, DoublePointer s);
public static native void cblas_drotg(DoubleBuffer a, DoubleBuffer b, DoubleBuffer c, DoubleBuffer s);
public static native void cblas_drotg(double[] a, double[] b, double[] c, double[] s);
public static native void cblas_srotm(@Cast("const blasint") long N, FloatPointer X, @Cast("const blasint") long incX, FloatPointer Y, @Cast("const blasint") long incY, @Const FloatPointer P);
public static native void cblas_srotm(@Cast("const blasint") long N, FloatBuffer X, @Cast("const blasint") long incX, FloatBuffer Y, @Cast("const blasint") long incY, @Const FloatBuffer P);
public static native void cblas_srotm(@Cast("const blasint") long N, float[] X, @Cast("const blasint") long incX, float[] Y, @Cast("const blasint") long incY, @Const float[] P);
public static native void cblas_drotm(@Cast("const blasint") long N, DoublePointer X, @Cast("const blasint") long incX, DoublePointer Y, @Cast("const blasint") long incY, @Const DoublePointer P);
public static native void cblas_drotm(@Cast("const blasint") long N, DoubleBuffer X, @Cast("const blasint") long incX, DoubleBuffer Y, @Cast("const blasint") long incY, @Const DoubleBuffer P);
public static native void cblas_drotm(@Cast("const blasint") long N, double[] X, @Cast("const blasint") long incX, double[] Y, @Cast("const blasint") long incY, @Const double[] P);
public static native void cblas_srotmg(FloatPointer d1, FloatPointer d2, FloatPointer b1, float b2, FloatPointer P);
public static native void cblas_srotmg(FloatBuffer d1, FloatBuffer d2, FloatBuffer b1, float b2, FloatBuffer P);
public static native void cblas_srotmg(float[] d1, float[] d2, float[] b1, float b2, float[] P);
public static native void cblas_drotmg(DoublePointer d1, DoublePointer d2, DoublePointer b1, double b2, DoublePointer P);
public static native void cblas_drotmg(DoubleBuffer d1, DoubleBuffer d2, DoubleBuffer b1, double b2, DoubleBuffer P);
public static native void cblas_drotmg(double[] d1, double[] d2, double[] b1, double b2, double[] P);
public static native void cblas_sscal(@Cast("const blasint") long N, float alpha, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_sscal(@Cast("const blasint") long N, float alpha, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_sscal(@Cast("const blasint") long N, float alpha, float[] X, @Cast("const blasint") long incX);
public static native void cblas_dscal(@Cast("const blasint") long N, double alpha, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_dscal(@Cast("const blasint") long N, double alpha, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_dscal(@Cast("const blasint") long N, double alpha, double[] X, @Cast("const blasint") long incX);
public static native void cblas_cscal(@Cast("const blasint") long N, @Const FloatPointer alpha, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_cscal(@Cast("const blasint") long N, @Const FloatBuffer alpha, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_cscal(@Cast("const blasint") long N, @Const float[] alpha, float[] X, @Cast("const blasint") long incX);
public static native void cblas_zscal(@Cast("const blasint") long N, @Const DoublePointer alpha, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_zscal(@Cast("const blasint") long N, @Const DoubleBuffer alpha, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_zscal(@Cast("const blasint") long N, @Const double[] alpha, double[] X, @Cast("const blasint") long incX);
public static native void cblas_csscal(@Cast("const blasint") long N, float alpha, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_csscal(@Cast("const blasint") long N, float alpha, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_csscal(@Cast("const blasint") long N, float alpha, float[] X, @Cast("const blasint") long incX);
public static native void cblas_zdscal(@Cast("const blasint") long N, double alpha, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_zdscal(@Cast("const blasint") long N, double alpha, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_zdscal(@Cast("const blasint") long N, double alpha, double[] X, @Cast("const blasint") long incX);
public static native void cblas_sgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
float alpha, @Const FloatPointer a, @Cast("const blasint") long lda, @Const FloatPointer x, @Cast("const blasint") long incx, float beta, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_sgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
float alpha, @Const FloatBuffer a, @Cast("const blasint") long lda, @Const FloatBuffer x, @Cast("const blasint") long incx, float beta, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_sgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
float alpha, @Const float[] a, @Cast("const blasint") long lda, @Const float[] x, @Cast("const blasint") long incx, float beta, float[] y, @Cast("const blasint") long incy);
public static native void cblas_dgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
double alpha, @Const DoublePointer a, @Cast("const blasint") long lda, @Const DoublePointer x, @Cast("const blasint") long incx, double beta, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_dgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
double alpha, @Const DoubleBuffer a, @Cast("const blasint") long lda, @Const DoubleBuffer x, @Cast("const blasint") long incx, double beta, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_dgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
double alpha, @Const double[] a, @Cast("const blasint") long lda, @Const double[] x, @Cast("const blasint") long incx, double beta, double[] y, @Cast("const blasint") long incy);
public static native void cblas_cgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
@Const FloatPointer alpha, @Const FloatPointer a, @Cast("const blasint") long lda, @Const FloatPointer x, @Cast("const blasint") long incx, @Const FloatPointer beta, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_cgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
@Const FloatBuffer alpha, @Const FloatBuffer a, @Cast("const blasint") long lda, @Const FloatBuffer x, @Cast("const blasint") long incx, @Const FloatBuffer beta, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_cgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
@Const float[] alpha, @Const float[] a, @Cast("const blasint") long lda, @Const float[] x, @Cast("const blasint") long incx, @Const float[] beta, float[] y, @Cast("const blasint") long incy);
public static native void cblas_zgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
@Const DoublePointer alpha, @Const DoublePointer a, @Cast("const blasint") long lda, @Const DoublePointer x, @Cast("const blasint") long incx, @Const DoublePointer beta, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_zgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
@Const DoubleBuffer alpha, @Const DoubleBuffer a, @Cast("const blasint") long lda, @Const DoubleBuffer x, @Cast("const blasint") long incx, @Const DoubleBuffer beta, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_zgemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int trans, @Cast("const blasint") long m, @Cast("const blasint") long n,
@Const double[] alpha, @Const double[] a, @Cast("const blasint") long lda, @Const double[] x, @Cast("const blasint") long incx, @Const double[] beta, double[] y, @Cast("const blasint") long incy);
public static native void cblas_sger(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, float alpha, @Const FloatPointer X, @Cast("const blasint") long incX, @Const FloatPointer Y, @Cast("const blasint") long incY, FloatPointer A, @Cast("const blasint") long lda);
public static native void cblas_sger(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, float alpha, @Const FloatBuffer X, @Cast("const blasint") long incX, @Const FloatBuffer Y, @Cast("const blasint") long incY, FloatBuffer A, @Cast("const blasint") long lda);
public static native void cblas_sger(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, float alpha, @Const float[] X, @Cast("const blasint") long incX, @Const float[] Y, @Cast("const blasint") long incY, float[] A, @Cast("const blasint") long lda);
public static native void cblas_dger(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, double alpha, @Const DoublePointer X, @Cast("const blasint") long incX, @Const DoublePointer Y, @Cast("const blasint") long incY, DoublePointer A, @Cast("const blasint") long lda);
public static native void cblas_dger(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer X, @Cast("const blasint") long incX, @Const DoubleBuffer Y, @Cast("const blasint") long incY, DoubleBuffer A, @Cast("const blasint") long lda);
public static native void cblas_dger(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, double alpha, @Const double[] X, @Cast("const blasint") long incX, @Const double[] Y, @Cast("const blasint") long incY, double[] A, @Cast("const blasint") long lda);
public static native void cblas_cgeru(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const FloatPointer alpha, @Const FloatPointer X, @Cast("const blasint") long incX, @Const FloatPointer Y, @Cast("const blasint") long incY, FloatPointer A, @Cast("const blasint") long lda);
public static native void cblas_cgeru(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const FloatBuffer alpha, @Const FloatBuffer X, @Cast("const blasint") long incX, @Const FloatBuffer Y, @Cast("const blasint") long incY, FloatBuffer A, @Cast("const blasint") long lda);
public static native void cblas_cgeru(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const float[] alpha, @Const float[] X, @Cast("const blasint") long incX, @Const float[] Y, @Cast("const blasint") long incY, float[] A, @Cast("const blasint") long lda);
public static native void cblas_cgerc(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const FloatPointer alpha, @Const FloatPointer X, @Cast("const blasint") long incX, @Const FloatPointer Y, @Cast("const blasint") long incY, FloatPointer A, @Cast("const blasint") long lda);
public static native void cblas_cgerc(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const FloatBuffer alpha, @Const FloatBuffer X, @Cast("const blasint") long incX, @Const FloatBuffer Y, @Cast("const blasint") long incY, FloatBuffer A, @Cast("const blasint") long lda);
public static native void cblas_cgerc(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const float[] alpha, @Const float[] X, @Cast("const blasint") long incX, @Const float[] Y, @Cast("const blasint") long incY, float[] A, @Cast("const blasint") long lda);
public static native void cblas_zgeru(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const DoublePointer alpha, @Const DoublePointer X, @Cast("const blasint") long incX, @Const DoublePointer Y, @Cast("const blasint") long incY, DoublePointer A, @Cast("const blasint") long lda);
public static native void cblas_zgeru(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const DoubleBuffer alpha, @Const DoubleBuffer X, @Cast("const blasint") long incX, @Const DoubleBuffer Y, @Cast("const blasint") long incY, DoubleBuffer A, @Cast("const blasint") long lda);
public static native void cblas_zgeru(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const double[] alpha, @Const double[] X, @Cast("const blasint") long incX, @Const double[] Y, @Cast("const blasint") long incY, double[] A, @Cast("const blasint") long lda);
public static native void cblas_zgerc(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const DoublePointer alpha, @Const DoublePointer X, @Cast("const blasint") long incX, @Const DoublePointer Y, @Cast("const blasint") long incY, DoublePointer A, @Cast("const blasint") long lda);
public static native void cblas_zgerc(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const DoubleBuffer alpha, @Const DoubleBuffer X, @Cast("const blasint") long incX, @Const DoubleBuffer Y, @Cast("const blasint") long incY, DoubleBuffer A, @Cast("const blasint") long lda);
public static native void cblas_zgerc(@Cast("const CBLAS_ORDER") int order, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const double[] alpha, @Const double[] X, @Cast("const blasint") long incX, @Const double[] Y, @Cast("const blasint") long incY, double[] A, @Cast("const blasint") long lda);
public static native void cblas_strsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_strsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_strsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const float[] A, @Cast("const blasint") long lda, float[] X, @Cast("const blasint") long incX);
public static native void cblas_dtrsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_dtrsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_dtrsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const double[] A, @Cast("const blasint") long lda, double[] X, @Cast("const blasint") long incX);
public static native void cblas_ctrsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_ctrsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ctrsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const float[] A, @Cast("const blasint") long lda, float[] X, @Cast("const blasint") long incX);
public static native void cblas_ztrsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_ztrsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ztrsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const double[] A, @Cast("const blasint") long lda, double[] X, @Cast("const blasint") long incX);
public static native void cblas_strmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_strmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_strmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const float[] A, @Cast("const blasint") long lda, float[] X, @Cast("const blasint") long incX);
public static native void cblas_dtrmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_dtrmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_dtrmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const double[] A, @Cast("const blasint") long lda, double[] X, @Cast("const blasint") long incX);
public static native void cblas_ctrmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_ctrmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ctrmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const float[] A, @Cast("const blasint") long lda, float[] X, @Cast("const blasint") long incX);
public static native void cblas_ztrmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_ztrmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ztrmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long N, @Const double[] A, @Cast("const blasint") long lda, double[] X, @Cast("const blasint") long incX);
public static native void cblas_ssyr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatPointer X, @Cast("const blasint") long incX, FloatPointer A, @Cast("const blasint") long lda);
public static native void cblas_ssyr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatBuffer X, @Cast("const blasint") long incX, FloatBuffer A, @Cast("const blasint") long lda);
public static native void cblas_ssyr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const float[] X, @Cast("const blasint") long incX, float[] A, @Cast("const blasint") long lda);
public static native void cblas_dsyr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoublePointer X, @Cast("const blasint") long incX, DoublePointer A, @Cast("const blasint") long lda);
public static native void cblas_dsyr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer X, @Cast("const blasint") long incX, DoubleBuffer A, @Cast("const blasint") long lda);
public static native void cblas_dsyr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const double[] X, @Cast("const blasint") long incX, double[] A, @Cast("const blasint") long lda);
public static native void cblas_cher(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatPointer X, @Cast("const blasint") long incX, FloatPointer A, @Cast("const blasint") long lda);
public static native void cblas_cher(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatBuffer X, @Cast("const blasint") long incX, FloatBuffer A, @Cast("const blasint") long lda);
public static native void cblas_cher(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const float[] X, @Cast("const blasint") long incX, float[] A, @Cast("const blasint") long lda);
public static native void cblas_zher(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoublePointer X, @Cast("const blasint") long incX, DoublePointer A, @Cast("const blasint") long lda);
public static native void cblas_zher(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer X, @Cast("const blasint") long incX, DoubleBuffer A, @Cast("const blasint") long lda);
public static native void cblas_zher(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const double[] X, @Cast("const blasint") long incX, double[] A, @Cast("const blasint") long lda);
public static native void cblas_ssyr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo,@Cast("const blasint") long N, float alpha, @Const FloatPointer X,
@Cast("const blasint") long incX, @Const FloatPointer Y, @Cast("const blasint") long incY, FloatPointer A, @Cast("const blasint") long lda);
public static native void cblas_ssyr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo,@Cast("const blasint") long N, float alpha, @Const FloatBuffer X,
@Cast("const blasint") long incX, @Const FloatBuffer Y, @Cast("const blasint") long incY, FloatBuffer A, @Cast("const blasint") long lda);
public static native void cblas_ssyr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo,@Cast("const blasint") long N, float alpha, @Const float[] X,
@Cast("const blasint") long incX, @Const float[] Y, @Cast("const blasint") long incY, float[] A, @Cast("const blasint") long lda);
public static native void cblas_dsyr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoublePointer X,
@Cast("const blasint") long incX, @Const DoublePointer Y, @Cast("const blasint") long incY, DoublePointer A, @Cast("const blasint") long lda);
public static native void cblas_dsyr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer X,
@Cast("const blasint") long incX, @Const DoubleBuffer Y, @Cast("const blasint") long incY, DoubleBuffer A, @Cast("const blasint") long lda);
public static native void cblas_dsyr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const double[] X,
@Cast("const blasint") long incX, @Const double[] Y, @Cast("const blasint") long incY, double[] A, @Cast("const blasint") long lda);
public static native void cblas_cher2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const FloatPointer alpha, @Const FloatPointer X, @Cast("const blasint") long incX,
@Const FloatPointer Y, @Cast("const blasint") long incY, FloatPointer A, @Cast("const blasint") long lda);
public static native void cblas_cher2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const FloatBuffer alpha, @Const FloatBuffer X, @Cast("const blasint") long incX,
@Const FloatBuffer Y, @Cast("const blasint") long incY, FloatBuffer A, @Cast("const blasint") long lda);
public static native void cblas_cher2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const float[] alpha, @Const float[] X, @Cast("const blasint") long incX,
@Const float[] Y, @Cast("const blasint") long incY, float[] A, @Cast("const blasint") long lda);
public static native void cblas_zher2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const DoublePointer alpha, @Const DoublePointer X, @Cast("const blasint") long incX,
@Const DoublePointer Y, @Cast("const blasint") long incY, DoublePointer A, @Cast("const blasint") long lda);
public static native void cblas_zher2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const DoubleBuffer alpha, @Const DoubleBuffer X, @Cast("const blasint") long incX,
@Const DoubleBuffer Y, @Cast("const blasint") long incY, DoubleBuffer A, @Cast("const blasint") long lda);
public static native void cblas_zher2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const double[] alpha, @Const double[] X, @Cast("const blasint") long incX,
@Const double[] Y, @Cast("const blasint") long incY, double[] A, @Cast("const blasint") long lda);
public static native void cblas_sgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, float alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer X, @Cast("const blasint") long incX, float beta, FloatPointer Y, @Cast("const blasint") long incY);
public static native void cblas_sgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, float alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer X, @Cast("const blasint") long incX, float beta, FloatBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_sgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, float alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] X, @Cast("const blasint") long incX, float beta, float[] Y, @Cast("const blasint") long incY);
public static native void cblas_dgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, double alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer X, @Cast("const blasint") long incX, double beta, DoublePointer Y, @Cast("const blasint") long incY);
public static native void cblas_dgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, double alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer X, @Cast("const blasint") long incX, double beta, DoubleBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_dgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, double alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] X, @Cast("const blasint") long incX, double beta, double[] Y, @Cast("const blasint") long incY);
public static native void cblas_cgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, @Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer X, @Cast("const blasint") long incX, @Const FloatPointer beta, FloatPointer Y, @Cast("const blasint") long incY);
public static native void cblas_cgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, @Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer X, @Cast("const blasint") long incX, @Const FloatBuffer beta, FloatBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_cgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, @Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] X, @Cast("const blasint") long incX, @Const float[] beta, float[] Y, @Cast("const blasint") long incY);
public static native void cblas_zgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, @Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer X, @Cast("const blasint") long incX, @Const DoublePointer beta, DoublePointer Y, @Cast("const blasint") long incY);
public static native void cblas_zgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, @Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer X, @Cast("const blasint") long incX, @Const DoubleBuffer beta, DoubleBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_zgbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Cast("const blasint") long KL, @Cast("const blasint") long KU, @Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] X, @Cast("const blasint") long incX, @Const double[] beta, double[] Y, @Cast("const blasint") long incY);
public static native void cblas_ssbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K, float alpha, @Const FloatPointer A,
@Cast("const blasint") long lda, @Const FloatPointer X, @Cast("const blasint") long incX, float beta, FloatPointer Y, @Cast("const blasint") long incY);
public static native void cblas_ssbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K, float alpha, @Const FloatBuffer A,
@Cast("const blasint") long lda, @Const FloatBuffer X, @Cast("const blasint") long incX, float beta, FloatBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_ssbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K, float alpha, @Const float[] A,
@Cast("const blasint") long lda, @Const float[] X, @Cast("const blasint") long incX, float beta, float[] Y, @Cast("const blasint") long incY);
public static native void cblas_dsbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K, double alpha, @Const DoublePointer A,
@Cast("const blasint") long lda, @Const DoublePointer X, @Cast("const blasint") long incX, double beta, DoublePointer Y, @Cast("const blasint") long incY);
public static native void cblas_dsbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K, double alpha, @Const DoubleBuffer A,
@Cast("const blasint") long lda, @Const DoubleBuffer X, @Cast("const blasint") long incX, double beta, DoubleBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_dsbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K, double alpha, @Const double[] A,
@Cast("const blasint") long lda, @Const double[] X, @Cast("const blasint") long incX, double beta, double[] Y, @Cast("const blasint") long incY);
public static native void cblas_stbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_stbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_stbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const float[] A, @Cast("const blasint") long lda, float[] X, @Cast("const blasint") long incX);
public static native void cblas_dtbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_dtbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_dtbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const double[] A, @Cast("const blasint") long lda, double[] X, @Cast("const blasint") long incX);
public static native void cblas_ctbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_ctbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ctbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const float[] A, @Cast("const blasint") long lda, float[] X, @Cast("const blasint") long incX);
public static native void cblas_ztbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_ztbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ztbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const double[] A, @Cast("const blasint") long lda, double[] X, @Cast("const blasint") long incX);
public static native void cblas_stbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_stbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_stbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const float[] A, @Cast("const blasint") long lda, float[] X, @Cast("const blasint") long incX);
public static native void cblas_dtbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_dtbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_dtbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const double[] A, @Cast("const blasint") long lda, double[] X, @Cast("const blasint") long incX);
public static native void cblas_ctbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_ctbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ctbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const float[] A, @Cast("const blasint") long lda, float[] X, @Cast("const blasint") long incX);
public static native void cblas_ztbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_ztbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ztbsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const double[] A, @Cast("const blasint") long lda, double[] X, @Cast("const blasint") long incX);
public static native void cblas_stpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const FloatPointer Ap, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_stpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const FloatBuffer Ap, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_stpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const float[] Ap, float[] X, @Cast("const blasint") long incX);
public static native void cblas_dtpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const DoublePointer Ap, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_dtpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const DoubleBuffer Ap, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_dtpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const double[] Ap, double[] X, @Cast("const blasint") long incX);
public static native void cblas_ctpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const FloatPointer Ap, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_ctpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const FloatBuffer Ap, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ctpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const float[] Ap, float[] X, @Cast("const blasint") long incX);
public static native void cblas_ztpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const DoublePointer Ap, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_ztpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const DoubleBuffer Ap, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ztpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const double[] Ap, double[] X, @Cast("const blasint") long incX);
public static native void cblas_stpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const FloatPointer Ap, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_stpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const FloatBuffer Ap, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_stpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const float[] Ap, float[] X, @Cast("const blasint") long incX);
public static native void cblas_dtpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const DoublePointer Ap, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_dtpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const DoubleBuffer Ap, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_dtpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const double[] Ap, double[] X, @Cast("const blasint") long incX);
public static native void cblas_ctpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const FloatPointer Ap, FloatPointer X, @Cast("const blasint") long incX);
public static native void cblas_ctpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const FloatBuffer Ap, FloatBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ctpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const float[] Ap, float[] X, @Cast("const blasint") long incX);
public static native void cblas_ztpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const DoublePointer Ap, DoublePointer X, @Cast("const blasint") long incX);
public static native void cblas_ztpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const DoubleBuffer Ap, DoubleBuffer X, @Cast("const blasint") long incX);
public static native void cblas_ztpsv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_DIAG") int Diag,
@Cast("const blasint") long N, @Const double[] Ap, double[] X, @Cast("const blasint") long incX);
public static native void cblas_ssymv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatPointer A,
@Cast("const blasint") long lda, @Const FloatPointer X, @Cast("const blasint") long incX, float beta, FloatPointer Y, @Cast("const blasint") long incY);
public static native void cblas_ssymv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatBuffer A,
@Cast("const blasint") long lda, @Const FloatBuffer X, @Cast("const blasint") long incX, float beta, FloatBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_ssymv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const float[] A,
@Cast("const blasint") long lda, @Const float[] X, @Cast("const blasint") long incX, float beta, float[] Y, @Cast("const blasint") long incY);
public static native void cblas_dsymv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoublePointer A,
@Cast("const blasint") long lda, @Const DoublePointer X, @Cast("const blasint") long incX, double beta, DoublePointer Y, @Cast("const blasint") long incY);
public static native void cblas_dsymv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer A,
@Cast("const blasint") long lda, @Const DoubleBuffer X, @Cast("const blasint") long incX, double beta, DoubleBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_dsymv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const double[] A,
@Cast("const blasint") long lda, @Const double[] X, @Cast("const blasint") long incX, double beta, double[] Y, @Cast("const blasint") long incY);
public static native void cblas_chemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const FloatPointer alpha, @Const FloatPointer A,
@Cast("const blasint") long lda, @Const FloatPointer X, @Cast("const blasint") long incX, @Const FloatPointer beta, FloatPointer Y, @Cast("const blasint") long incY);
public static native void cblas_chemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const FloatBuffer alpha, @Const FloatBuffer A,
@Cast("const blasint") long lda, @Const FloatBuffer X, @Cast("const blasint") long incX, @Const FloatBuffer beta, FloatBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_chemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const float[] alpha, @Const float[] A,
@Cast("const blasint") long lda, @Const float[] X, @Cast("const blasint") long incX, @Const float[] beta, float[] Y, @Cast("const blasint") long incY);
public static native void cblas_zhemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const DoublePointer alpha, @Const DoublePointer A,
@Cast("const blasint") long lda, @Const DoublePointer X, @Cast("const blasint") long incX, @Const DoublePointer beta, DoublePointer Y, @Cast("const blasint") long incY);
public static native void cblas_zhemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const DoubleBuffer alpha, @Const DoubleBuffer A,
@Cast("const blasint") long lda, @Const DoubleBuffer X, @Cast("const blasint") long incX, @Const DoubleBuffer beta, DoubleBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_zhemv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const double[] alpha, @Const double[] A,
@Cast("const blasint") long lda, @Const double[] X, @Cast("const blasint") long incX, @Const double[] beta, double[] Y, @Cast("const blasint") long incY);
public static native void cblas_sspmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatPointer Ap,
@Const FloatPointer X, @Cast("const blasint") long incX, float beta, FloatPointer Y, @Cast("const blasint") long incY);
public static native void cblas_sspmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatBuffer Ap,
@Const FloatBuffer X, @Cast("const blasint") long incX, float beta, FloatBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_sspmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const float[] Ap,
@Const float[] X, @Cast("const blasint") long incX, float beta, float[] Y, @Cast("const blasint") long incY);
public static native void cblas_dspmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoublePointer Ap,
@Const DoublePointer X, @Cast("const blasint") long incX, double beta, DoublePointer Y, @Cast("const blasint") long incY);
public static native void cblas_dspmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer Ap,
@Const DoubleBuffer X, @Cast("const blasint") long incX, double beta, DoubleBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_dspmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const double[] Ap,
@Const double[] X, @Cast("const blasint") long incX, double beta, double[] Y, @Cast("const blasint") long incY);
public static native void cblas_sspr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatPointer X, @Cast("const blasint") long incX, FloatPointer Ap);
public static native void cblas_sspr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatBuffer X, @Cast("const blasint") long incX, FloatBuffer Ap);
public static native void cblas_sspr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const float[] X, @Cast("const blasint") long incX, float[] Ap);
public static native void cblas_dspr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoublePointer X, @Cast("const blasint") long incX, DoublePointer Ap);
public static native void cblas_dspr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer X, @Cast("const blasint") long incX, DoubleBuffer Ap);
public static native void cblas_dspr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const double[] X, @Cast("const blasint") long incX, double[] Ap);
public static native void cblas_chpr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatPointer X, @Cast("const blasint") long incX, FloatPointer A);
public static native void cblas_chpr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatBuffer X, @Cast("const blasint") long incX, FloatBuffer A);
public static native void cblas_chpr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const float[] X, @Cast("const blasint") long incX, float[] A);
public static native void cblas_zhpr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoublePointer X,@Cast("const blasint") long incX, DoublePointer A);
public static native void cblas_zhpr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer X,@Cast("const blasint") long incX, DoubleBuffer A);
public static native void cblas_zhpr(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const double[] X,@Cast("const blasint") long incX, double[] A);
public static native void cblas_sspr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatPointer X, @Cast("const blasint") long incX, @Const FloatPointer Y, @Cast("const blasint") long incY, FloatPointer A);
public static native void cblas_sspr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const FloatBuffer X, @Cast("const blasint") long incX, @Const FloatBuffer Y, @Cast("const blasint") long incY, FloatBuffer A);
public static native void cblas_sspr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, float alpha, @Const float[] X, @Cast("const blasint") long incX, @Const float[] Y, @Cast("const blasint") long incY, float[] A);
public static native void cblas_dspr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoublePointer X, @Cast("const blasint") long incX, @Const DoublePointer Y, @Cast("const blasint") long incY, DoublePointer A);
public static native void cblas_dspr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer X, @Cast("const blasint") long incX, @Const DoubleBuffer Y, @Cast("const blasint") long incY, DoubleBuffer A);
public static native void cblas_dspr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, double alpha, @Const double[] X, @Cast("const blasint") long incX, @Const double[] Y, @Cast("const blasint") long incY, double[] A);
public static native void cblas_chpr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const FloatPointer alpha, @Const FloatPointer X, @Cast("const blasint") long incX, @Const FloatPointer Y, @Cast("const blasint") long incY, FloatPointer Ap);
public static native void cblas_chpr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const FloatBuffer alpha, @Const FloatBuffer X, @Cast("const blasint") long incX, @Const FloatBuffer Y, @Cast("const blasint") long incY, FloatBuffer Ap);
public static native void cblas_chpr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const float[] alpha, @Const float[] X, @Cast("const blasint") long incX, @Const float[] Y, @Cast("const blasint") long incY, float[] Ap);
public static native void cblas_zhpr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const DoublePointer alpha, @Const DoublePointer X, @Cast("const blasint") long incX, @Const DoublePointer Y, @Cast("const blasint") long incY, DoublePointer Ap);
public static native void cblas_zhpr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const DoubleBuffer alpha, @Const DoubleBuffer X, @Cast("const blasint") long incX, @Const DoubleBuffer Y, @Cast("const blasint") long incY, DoubleBuffer Ap);
public static native void cblas_zhpr2(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Const double[] alpha, @Const double[] X, @Cast("const blasint") long incX, @Const double[] Y, @Cast("const blasint") long incY, double[] Ap);
public static native void cblas_chbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer X, @Cast("const blasint") long incX, @Const FloatPointer beta, FloatPointer Y, @Cast("const blasint") long incY);
public static native void cblas_chbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer X, @Cast("const blasint") long incX, @Const FloatBuffer beta, FloatBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_chbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] X, @Cast("const blasint") long incX, @Const float[] beta, float[] Y, @Cast("const blasint") long incY);
public static native void cblas_zhbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer X, @Cast("const blasint") long incX, @Const DoublePointer beta, DoublePointer Y, @Cast("const blasint") long incY);
public static native void cblas_zhbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer X, @Cast("const blasint") long incX, @Const DoubleBuffer beta, DoubleBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_zhbmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] X, @Cast("const blasint") long incX, @Const double[] beta, double[] Y, @Cast("const blasint") long incY);
public static native void cblas_chpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N,
@Const FloatPointer alpha, @Const FloatPointer Ap, @Const FloatPointer X, @Cast("const blasint") long incX, @Const FloatPointer beta, FloatPointer Y, @Cast("const blasint") long incY);
public static native void cblas_chpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N,
@Const FloatBuffer alpha, @Const FloatBuffer Ap, @Const FloatBuffer X, @Cast("const blasint") long incX, @Const FloatBuffer beta, FloatBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_chpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N,
@Const float[] alpha, @Const float[] Ap, @Const float[] X, @Cast("const blasint") long incX, @Const float[] beta, float[] Y, @Cast("const blasint") long incY);
public static native void cblas_zhpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N,
@Const DoublePointer alpha, @Const DoublePointer Ap, @Const DoublePointer X, @Cast("const blasint") long incX, @Const DoublePointer beta, DoublePointer Y, @Cast("const blasint") long incY);
public static native void cblas_zhpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N,
@Const DoubleBuffer alpha, @Const DoubleBuffer Ap, @Const DoubleBuffer X, @Cast("const blasint") long incX, @Const DoubleBuffer beta, DoubleBuffer Y, @Cast("const blasint") long incY);
public static native void cblas_zhpmv(@Cast("const CBLAS_ORDER") int order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long N,
@Const double[] alpha, @Const double[] Ap, @Const double[] X, @Cast("const blasint") long incX, @Const double[] beta, double[] Y, @Cast("const blasint") long incY);
public static native void cblas_sgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
float alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer B, @Cast("const blasint") long ldb, float beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_sgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
float alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer B, @Cast("const blasint") long ldb, float beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_sgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
float alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] B, @Cast("const blasint") long ldb, float beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_dgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
double alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer B, @Cast("const blasint") long ldb, double beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_dgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
double alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer B, @Cast("const blasint") long ldb, double beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_dgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
double alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] B, @Cast("const blasint") long ldb, double beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_cgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer B, @Cast("const blasint") long ldb, @Const FloatPointer beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_cgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer B, @Cast("const blasint") long ldb, @Const FloatBuffer beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_cgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] B, @Cast("const blasint") long ldb, @Const float[] beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_zgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer B, @Cast("const blasint") long ldb, @Const DoublePointer beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_zgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer B, @Cast("const blasint") long ldb, @Const DoubleBuffer beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_zgemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_TRANSPOSE") int TransA, @Cast("const CBLAS_TRANSPOSE") int TransB, @Cast("const blasint") long M, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] B, @Cast("const blasint") long ldb, @Const double[] beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_ssymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
float alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer B, @Cast("const blasint") long ldb, float beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_ssymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
float alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer B, @Cast("const blasint") long ldb, float beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_ssymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
float alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] B, @Cast("const blasint") long ldb, float beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_dsymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
double alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer B, @Cast("const blasint") long ldb, double beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_dsymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
double alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer B, @Cast("const blasint") long ldb, double beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_dsymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
double alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] B, @Cast("const blasint") long ldb, double beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_csymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer B, @Cast("const blasint") long ldb, @Const FloatPointer beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_csymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer B, @Cast("const blasint") long ldb, @Const FloatBuffer beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_csymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] B, @Cast("const blasint") long ldb, @Const float[] beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_zsymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer B, @Cast("const blasint") long ldb, @Const DoublePointer beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_zsymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer B, @Cast("const blasint") long ldb, @Const DoubleBuffer beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_zsymm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] B, @Cast("const blasint") long ldb, @Const double[] beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_ssyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, float alpha, @Const FloatPointer A, @Cast("const blasint") long lda, float beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_ssyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, float alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, float beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_ssyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, float alpha, @Const float[] A, @Cast("const blasint") long lda, float beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_dsyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, double alpha, @Const DoublePointer A, @Cast("const blasint") long lda, double beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_dsyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, double alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, double beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_dsyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, double alpha, @Const double[] A, @Cast("const blasint") long lda, double beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_csyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_csyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_csyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_zsyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_zsyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_zsyrk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_ssyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, float alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer B, @Cast("const blasint") long ldb, float beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_ssyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, float alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer B, @Cast("const blasint") long ldb, float beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_ssyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, float alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] B, @Cast("const blasint") long ldb, float beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_dsyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, double alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer B, @Cast("const blasint") long ldb, double beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_dsyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, double alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer B, @Cast("const blasint") long ldb, double beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_dsyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, double alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] B, @Cast("const blasint") long ldb, double beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_csyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer B, @Cast("const blasint") long ldb, @Const FloatPointer beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_csyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer B, @Cast("const blasint") long ldb, @Const FloatBuffer beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_csyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] B, @Cast("const blasint") long ldb, @Const float[] beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_zsyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer B, @Cast("const blasint") long ldb, @Const DoublePointer beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_zsyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer B, @Cast("const blasint") long ldb, @Const DoubleBuffer beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_zsyr2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans,
@Cast("const blasint") long N, @Cast("const blasint") long K, @Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] B, @Cast("const blasint") long ldb, @Const double[] beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_strmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, float alpha, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer B, @Cast("const blasint") long ldb);
public static native void cblas_strmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, float alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer B, @Cast("const blasint") long ldb);
public static native void cblas_strmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, float alpha, @Const float[] A, @Cast("const blasint") long lda, float[] B, @Cast("const blasint") long ldb);
public static native void cblas_dtrmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, double alpha, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer B, @Cast("const blasint") long ldb);
public static native void cblas_dtrmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer B, @Cast("const blasint") long ldb);
public static native void cblas_dtrmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, double alpha, @Const double[] A, @Cast("const blasint") long lda, double[] B, @Cast("const blasint") long ldb);
public static native void cblas_ctrmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer B, @Cast("const blasint") long ldb);
public static native void cblas_ctrmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer B, @Cast("const blasint") long ldb);
public static native void cblas_ctrmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, float[] B, @Cast("const blasint") long ldb);
public static native void cblas_ztrmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer B, @Cast("const blasint") long ldb);
public static native void cblas_ztrmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer B, @Cast("const blasint") long ldb);
public static native void cblas_ztrmm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, double[] B, @Cast("const blasint") long ldb);
public static native void cblas_strsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, float alpha, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer B, @Cast("const blasint") long ldb);
public static native void cblas_strsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, float alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer B, @Cast("const blasint") long ldb);
public static native void cblas_strsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, float alpha, @Const float[] A, @Cast("const blasint") long lda, float[] B, @Cast("const blasint") long ldb);
public static native void cblas_dtrsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, double alpha, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer B, @Cast("const blasint") long ldb);
public static native void cblas_dtrsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, double alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer B, @Cast("const blasint") long ldb);
public static native void cblas_dtrsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, double alpha, @Const double[] A, @Cast("const blasint") long lda, double[] B, @Cast("const blasint") long ldb);
public static native void cblas_ctrsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, FloatPointer B, @Cast("const blasint") long ldb);
public static native void cblas_ctrsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, FloatBuffer B, @Cast("const blasint") long ldb);
public static native void cblas_ctrsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, float[] B, @Cast("const blasint") long ldb);
public static native void cblas_ztrsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, DoublePointer B, @Cast("const blasint") long ldb);
public static native void cblas_ztrsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, DoubleBuffer B, @Cast("const blasint") long ldb);
public static native void cblas_ztrsm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int TransA,
@Cast("const CBLAS_DIAG") int Diag, @Cast("const blasint") long M, @Cast("const blasint") long N, @Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, double[] B, @Cast("const blasint") long ldb);
public static native void cblas_chemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer B, @Cast("const blasint") long ldb, @Const FloatPointer beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_chemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer B, @Cast("const blasint") long ldb, @Const FloatBuffer beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_chemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] B, @Cast("const blasint") long ldb, @Const float[] beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_zhemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer B, @Cast("const blasint") long ldb, @Const DoublePointer beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_zhemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer B, @Cast("const blasint") long ldb, @Const DoubleBuffer beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_zhemm(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_SIDE") int Side, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const blasint") long M, @Cast("const blasint") long N,
@Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] B, @Cast("const blasint") long ldb, @Const double[] beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_cherk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
float alpha, @Const FloatPointer A, @Cast("const blasint") long lda, float beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_cherk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
float alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, float beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_cherk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
float alpha, @Const float[] A, @Cast("const blasint") long lda, float beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_zherk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
double alpha, @Const DoublePointer A, @Cast("const blasint") long lda, double beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_zherk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
double alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, double beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_zherk(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
double alpha, @Const double[] A, @Cast("const blasint") long lda, double beta, double[] C, @Cast("const blasint") long ldc);
public static native void cblas_cher2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const FloatPointer alpha, @Const FloatPointer A, @Cast("const blasint") long lda, @Const FloatPointer B, @Cast("const blasint") long ldb, float beta, FloatPointer C, @Cast("const blasint") long ldc);
public static native void cblas_cher2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const FloatBuffer alpha, @Const FloatBuffer A, @Cast("const blasint") long lda, @Const FloatBuffer B, @Cast("const blasint") long ldb, float beta, FloatBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_cher2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const float[] alpha, @Const float[] A, @Cast("const blasint") long lda, @Const float[] B, @Cast("const blasint") long ldb, float beta, float[] C, @Cast("const blasint") long ldc);
public static native void cblas_zher2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const DoublePointer alpha, @Const DoublePointer A, @Cast("const blasint") long lda, @Const DoublePointer B, @Cast("const blasint") long ldb, double beta, DoublePointer C, @Cast("const blasint") long ldc);
public static native void cblas_zher2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const DoubleBuffer alpha, @Const DoubleBuffer A, @Cast("const blasint") long lda, @Const DoubleBuffer B, @Cast("const blasint") long ldb, double beta, DoubleBuffer C, @Cast("const blasint") long ldc);
public static native void cblas_zher2k(@Cast("const CBLAS_ORDER") int Order, @Cast("const CBLAS_UPLO") int Uplo, @Cast("const CBLAS_TRANSPOSE") int Trans, @Cast("const blasint") long N, @Cast("const blasint") long K,
@Const double[] alpha, @Const double[] A, @Cast("const blasint") long lda, @Const double[] B, @Cast("const blasint") long ldb, double beta, double[] C, @Cast("const blasint") long ldc);
/*** BLAS extensions ***/
public static native void cblas_saxpby(@Cast("const blasint") long n, float alpha, @Const FloatPointer x, @Cast("const blasint") long incx,float beta, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_saxpby(@Cast("const blasint") long n, float alpha, @Const FloatBuffer x, @Cast("const blasint") long incx,float beta, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_saxpby(@Cast("const blasint") long n, float alpha, @Const float[] x, @Cast("const blasint") long incx,float beta, float[] y, @Cast("const blasint") long incy);
public static native void cblas_daxpby(@Cast("const blasint") long n, double alpha, @Const DoublePointer x, @Cast("const blasint") long incx,double beta, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_daxpby(@Cast("const blasint") long n, double alpha, @Const DoubleBuffer x, @Cast("const blasint") long incx,double beta, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_daxpby(@Cast("const blasint") long n, double alpha, @Const double[] x, @Cast("const blasint") long incx,double beta, double[] y, @Cast("const blasint") long incy);
public static native void cblas_caxpby(@Cast("const blasint") long n, @Const FloatPointer alpha, @Const FloatPointer x, @Cast("const blasint") long incx,@Const FloatPointer beta, FloatPointer y, @Cast("const blasint") long incy);
public static native void cblas_caxpby(@Cast("const blasint") long n, @Const FloatBuffer alpha, @Const FloatBuffer x, @Cast("const blasint") long incx,@Const FloatBuffer beta, FloatBuffer y, @Cast("const blasint") long incy);
public static native void cblas_caxpby(@Cast("const blasint") long n, @Const float[] alpha, @Const float[] x, @Cast("const blasint") long incx,@Const float[] beta, float[] y, @Cast("const blasint") long incy);
public static native void cblas_zaxpby(@Cast("const blasint") long n, @Const DoublePointer alpha, @Const DoublePointer x, @Cast("const blasint") long incx,@Const DoublePointer beta, DoublePointer y, @Cast("const blasint") long incy);
public static native void cblas_zaxpby(@Cast("const blasint") long n, @Const DoubleBuffer alpha, @Const DoubleBuffer x, @Cast("const blasint") long incx,@Const DoubleBuffer beta, DoubleBuffer y, @Cast("const blasint") long incy);
public static native void cblas_zaxpby(@Cast("const blasint") long n, @Const double[] alpha, @Const double[] x, @Cast("const blasint") long incx,@Const double[] beta, double[] y, @Cast("const blasint") long incy);
// #ifdef __cplusplus
// #endif /* __cplusplus */
// #endif
// Parsed from blas_extra.h
// Based on code found in https://github.com/deeplearning4j/libnd4j/blob/master/blas/cpu/NativeBlas.cpp
// #include
// #ifdef _WIN32
// #include
// #else
// #include
// #endif
public static native int maxThreads(); public static native void maxThreads(int maxThreads);
public static native int vendor(); public static native void vendor(int vendor);
public static native void blas_set_num_threads(int num);
public static native int blas_get_num_threads();
/**
* 0 - Unknown
* 1 - cuBLAS
* 2 - OpenBLAS
* 3 - MKL
*/
public static native int blas_get_vendor();
// Parsed from lapacke_config.h
/*****************************************************************************
Copyright (c) 2010, Intel Corp.
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice,
this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
* Neither the name of Intel Corporation nor the names of its contributors
may be used to endorse or promote products derived from this software
without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF
THE POSSIBILITY OF SUCH DAMAGE.
******************************************************************************
* Contents: Native C interface to LAPACK
* Author: Intel Corporation
* Generated May, 2011
*****************************************************************************/
// #ifndef _LAPACKE_CONFIG_H_
// #define _LAPACKE_CONFIG_H_
// For Android prior to API 21 (no include)
// #if defined(__ANDROID__)
// #if __ANDROID_API__ < 21
// #define LAPACK_COMPLEX_STRUCTURE
// #endif
// #endif
// #ifdef __cplusplus
// #if defined(LAPACK_COMPLEX_CPP)
// #include
// #endif
// #endif /* __cplusplus */
// #include
// #ifndef lapack_int
// #if defined(LAPACK_ILP64)
// #define lapack_int long
// #else
// #define lapack_int int
// #endif
// #endif
// #ifndef lapack_logical
// #define lapack_logical lapack_int
// #endif
// #ifndef LAPACK_COMPLEX_CUSTOM
// #endif
// #ifndef LAPACK_malloc
// #define LAPACK_malloc( size ) malloc( size )
// #endif
// #ifndef LAPACK_free
// #define LAPACK_free( p ) free( p )
// #endif
// #ifdef __cplusplus
// #endif /* __cplusplus */
// #endif /* _LAPACKE_CONFIG_H_ */
// Parsed from lapacke_mangling.h
// #ifndef LAPACK_HEADER_INCLUDED
// #define LAPACK_HEADER_INCLUDED
// #ifndef LAPACK_GLOBAL
// #if defined(LAPACK_GLOBAL_PATTERN_LC) || defined(ADD_)
// #define LAPACK_GLOBAL(lcname,UCNAME) lcname##_
// #elif defined(LAPACK_GLOBAL_PATTERN_UC) || defined(UPPER)
// #define LAPACK_GLOBAL(lcname,UCNAME) UCNAME
// #elif defined(LAPACK_GLOBAL_PATTERN_MC) || defined(NOCHANGE)
// #define LAPACK_GLOBAL(lcname,UCNAME) lcname
// #else
// #define LAPACK_GLOBAL(lcname,UCNAME) lcname##_
// #endif
// #endif
// #endif
// Parsed from lapacke.h
/*****************************************************************************
Copyright (c) 2014, Intel Corp.
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice,
this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
* Neither the name of Intel Corporation nor the names of its contributors
may be used to endorse or promote products derived from this software
without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF
THE POSSIBILITY OF SUCH DAMAGE.
******************************************************************************
* Contents: Native C interface to LAPACK
* Author: Intel Corporation
* Generated August, 2015
*****************************************************************************/
// #ifndef _LAPACKE_H_
// #define _LAPACKE_H_
/*
* Turn on HAVE_LAPACK_CONFIG_H to redefine C-LAPACK datatypes
*/
// #ifdef HAVE_LAPACK_CONFIG_H
// #include "lapacke_config.h"
// #endif
// #include
// #ifndef lapack_int
// #define lapack_int int
// #endif
// #ifndef lapack_logical
// #define lapack_logical lapack_int
// #endif
/* Complex types are structures equivalent to the
* Fortran complex types COMPLEX(4) and COMPLEX(8).
*
* One can also redefine the types with his own types
* for example by including in the code definitions like
*
* #define lapack_complex_float std::complex
* #define lapack_complex_double std::complex
*
* or define these types in the command line:
*
* -Dlapack_complex_float="std::complex"
* -Dlapack_complex_double="std::complex"
*/
// #ifndef LAPACK_COMPLEX_CUSTOM
// #endif
// #ifdef __cplusplus
// #endif /* __cplusplus */
// #ifndef LAPACKE_malloc
// #define LAPACKE_malloc( size ) malloc( size )
// #endif
// #ifndef LAPACKE_free
// #define LAPACKE_free( p ) free( p )
// #endif
// #define LAPACK_C2INT( x ) (lapack_int)(*((float*)&x ))
// #define LAPACK_Z2INT( x ) (lapack_int)(*((double*)&x ))
public static final int LAPACK_ROW_MAJOR = 101;
public static final int LAPACK_COL_MAJOR = 102;
public static final int LAPACK_WORK_MEMORY_ERROR = -1010;
public static final int LAPACK_TRANSPOSE_MEMORY_ERROR = -1011;
/* Callback logical functions of one, two, or three arguments are used
* to select eigenvalues to sort to the top left of the Schur form.
* The value is selected if function returns TRUE (non-zero). */
public static class LAPACK_S_SELECT2 extends FunctionPointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public LAPACK_S_SELECT2(Pointer p) { super(p); }
protected LAPACK_S_SELECT2() { allocate(); }
private native void allocate();
public native int call( @Const FloatPointer arg0, @Const FloatPointer arg1 );
}
public static class LAPACK_S_SELECT3 extends FunctionPointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public LAPACK_S_SELECT3(Pointer p) { super(p); }
protected LAPACK_S_SELECT3() { allocate(); }
private native void allocate();
public native int call( @Const FloatPointer arg0, @Const FloatPointer arg1, @Const FloatPointer arg2 );
}
public static class LAPACK_D_SELECT2 extends FunctionPointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public LAPACK_D_SELECT2(Pointer p) { super(p); }
protected LAPACK_D_SELECT2() { allocate(); }
private native void allocate();
public native int call( @Const DoublePointer arg0, @Const DoublePointer arg1 );
}
public static class LAPACK_D_SELECT3 extends FunctionPointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public LAPACK_D_SELECT3(Pointer p) { super(p); }
protected LAPACK_D_SELECT3() { allocate(); }
private native void allocate();
public native int call( @Const DoublePointer arg0, @Const DoublePointer arg1, @Const DoublePointer arg2 );
}
public static class LAPACK_C_SELECT1 extends FunctionPointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public LAPACK_C_SELECT1(Pointer p) { super(p); }
protected LAPACK_C_SELECT1() { allocate(); }
private native void allocate();
public native int call( @Cast("const lapack_complex_float*") FloatPointer arg0 );
}
public static class LAPACK_C_SELECT2 extends FunctionPointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public LAPACK_C_SELECT2(Pointer p) { super(p); }
protected LAPACK_C_SELECT2() { allocate(); }
private native void allocate();
public native int call( @Cast("const lapack_complex_float*") FloatPointer arg0, @Cast("const lapack_complex_float*") FloatPointer arg1 );
}
public static class LAPACK_Z_SELECT1 extends FunctionPointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public LAPACK_Z_SELECT1(Pointer p) { super(p); }
protected LAPACK_Z_SELECT1() { allocate(); }
private native void allocate();
public native int call( @Cast("const lapack_complex_double*") DoublePointer arg0 );
}
public static class LAPACK_Z_SELECT2 extends FunctionPointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public LAPACK_Z_SELECT2(Pointer p) { super(p); }
protected LAPACK_Z_SELECT2() { allocate(); }
private native void allocate();
public native int call( @Cast("const lapack_complex_double*") DoublePointer arg0, @Cast("const lapack_complex_double*") DoublePointer arg1 );
}
// #include "lapacke_mangling.h"
public static native int LAPACK_lsame( @Cast("char*") BytePointer ca, @Cast("char*") BytePointer cb,
int lca, int lcb );
public static native int LAPACK_lsame( @Cast("char*") ByteBuffer ca, @Cast("char*") ByteBuffer cb,
int lca, int lcb );
public static native int LAPACK_lsame( @Cast("char*") byte[] ca, @Cast("char*") byte[] cb,
int lca, int lcb );
/* C-LAPACK function prototypes */
public static native int LAPACKE_sbdsdc( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, FloatPointer d, FloatPointer e, FloatPointer u,
int ldu, FloatPointer vt, int ldvt, FloatPointer q,
IntPointer iq );
public static native int LAPACKE_sbdsdc( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, FloatBuffer d, FloatBuffer e, FloatBuffer u,
int ldu, FloatBuffer vt, int ldvt, FloatBuffer q,
IntBuffer iq );
public static native int LAPACKE_sbdsdc( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, float[] d, float[] e, float[] u,
int ldu, float[] vt, int ldvt, float[] q,
int[] iq );
public static native int LAPACKE_dbdsdc( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, DoublePointer d, DoublePointer e, DoublePointer u,
int ldu, DoublePointer vt, int ldvt,
DoublePointer q, IntPointer iq );
public static native int LAPACKE_dbdsdc( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, DoubleBuffer d, DoubleBuffer e, DoubleBuffer u,
int ldu, DoubleBuffer vt, int ldvt,
DoubleBuffer q, IntBuffer iq );
public static native int LAPACKE_dbdsdc( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, double[] d, double[] e, double[] u,
int ldu, double[] vt, int ldvt,
double[] q, int[] iq );
public static native int LAPACKE_sbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
FloatPointer d, FloatPointer e, FloatPointer vt, int ldvt,
FloatPointer u, int ldu, FloatPointer c, int ldc );
public static native int LAPACKE_sbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
FloatBuffer d, FloatBuffer e, FloatBuffer vt, int ldvt,
FloatBuffer u, int ldu, FloatBuffer c, int ldc );
public static native int LAPACKE_sbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
float[] d, float[] e, float[] vt, int ldvt,
float[] u, int ldu, float[] c, int ldc );
public static native int LAPACKE_dbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
DoublePointer d, DoublePointer e, DoublePointer vt, int ldvt,
DoublePointer u, int ldu, DoublePointer c,
int ldc );
public static native int LAPACKE_dbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer vt, int ldvt,
DoubleBuffer u, int ldu, DoubleBuffer c,
int ldc );
public static native int LAPACKE_dbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
double[] d, double[] e, double[] vt, int ldvt,
double[] u, int ldu, double[] c,
int ldc );
public static native int LAPACKE_cbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
FloatPointer d, FloatPointer e, @Cast("lapack_complex_float*") FloatPointer vt,
int ldvt, @Cast("lapack_complex_float*") FloatPointer u,
int ldu, @Cast("lapack_complex_float*") FloatPointer c,
int ldc );
public static native int LAPACKE_cbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
FloatBuffer d, FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer vt,
int ldvt, @Cast("lapack_complex_float*") FloatBuffer u,
int ldu, @Cast("lapack_complex_float*") FloatBuffer c,
int ldc );
public static native int LAPACKE_cbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
float[] d, float[] e, @Cast("lapack_complex_float*") float[] vt,
int ldvt, @Cast("lapack_complex_float*") float[] u,
int ldu, @Cast("lapack_complex_float*") float[] c,
int ldc );
public static native int LAPACKE_zbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer vt,
int ldvt, @Cast("lapack_complex_double*") DoublePointer u,
int ldu, @Cast("lapack_complex_double*") DoublePointer c,
int ldc );
public static native int LAPACKE_zbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer vt,
int ldvt, @Cast("lapack_complex_double*") DoubleBuffer u,
int ldu, @Cast("lapack_complex_double*") DoubleBuffer c,
int ldc );
public static native int LAPACKE_zbdsqr( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] vt,
int ldvt, @Cast("lapack_complex_double*") double[] u,
int ldu, @Cast("lapack_complex_double*") double[] c,
int ldc );
public static native int LAPACKE_sbdsvdx( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e,
int vl, int vu,
int il, int iu, int ns,
FloatPointer s, FloatPointer z, int ldz,
IntPointer superb );
public static native int LAPACKE_sbdsvdx( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e,
int vl, int vu,
int il, int iu, int ns,
FloatBuffer s, FloatBuffer z, int ldz,
IntBuffer superb );
public static native int LAPACKE_sbdsvdx( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e,
int vl, int vu,
int il, int iu, int ns,
float[] s, float[] z, int ldz,
int[] superb );
public static native int LAPACKE_dbdsvdx( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e,
int vl, int vu,
int il, int iu, int ns,
DoublePointer s, DoublePointer z, int ldz,
IntPointer superb );
public static native int LAPACKE_dbdsvdx( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e,
int vl, int vu,
int il, int iu, int ns,
DoubleBuffer s, DoubleBuffer z, int ldz,
IntBuffer superb );
public static native int LAPACKE_dbdsvdx( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e,
int vl, int vu,
int il, int iu, int ns,
double[] s, double[] z, int ldz,
int[] superb );
public static native int LAPACKE_sdisna( @Cast("char") byte job, int m, int n, @Const FloatPointer d,
FloatPointer sep );
public static native int LAPACKE_sdisna( @Cast("char") byte job, int m, int n, @Const FloatBuffer d,
FloatBuffer sep );
public static native int LAPACKE_sdisna( @Cast("char") byte job, int m, int n, @Const float[] d,
float[] sep );
public static native int LAPACKE_ddisna( @Cast("char") byte job, int m, int n,
@Const DoublePointer d, DoublePointer sep );
public static native int LAPACKE_ddisna( @Cast("char") byte job, int m, int n,
@Const DoubleBuffer d, DoubleBuffer sep );
public static native int LAPACKE_ddisna( @Cast("char") byte job, int m, int n,
@Const double[] d, double[] sep );
public static native int LAPACKE_sgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, FloatPointer ab, int ldab, FloatPointer d,
FloatPointer e, FloatPointer q, int ldq, FloatPointer pt,
int ldpt, FloatPointer c, int ldc );
public static native int LAPACKE_sgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, FloatBuffer ab, int ldab, FloatBuffer d,
FloatBuffer e, FloatBuffer q, int ldq, FloatBuffer pt,
int ldpt, FloatBuffer c, int ldc );
public static native int LAPACKE_sgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, float[] ab, int ldab, float[] d,
float[] e, float[] q, int ldq, float[] pt,
int ldpt, float[] c, int ldc );
public static native int LAPACKE_dgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, DoublePointer ab, int ldab,
DoublePointer d, DoublePointer e, DoublePointer q, int ldq,
DoublePointer pt, int ldpt, DoublePointer c,
int ldc );
public static native int LAPACKE_dgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, DoubleBuffer ab, int ldab,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer q, int ldq,
DoubleBuffer pt, int ldpt, DoubleBuffer c,
int ldc );
public static native int LAPACKE_dgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, double[] ab, int ldab,
double[] d, double[] e, double[] q, int ldq,
double[] pt, int ldpt, double[] c,
int ldc );
public static native int LAPACKE_cgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer pt, int ldpt,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer pt, int ldpt,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_float*") float[] ab,
int ldab, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] pt, int ldpt,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer pt, int ldpt,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer pt, int ldpt,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zgbbrd( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_double*") double[] ab,
int ldab, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] pt, int ldpt,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_sgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const FloatPointer ab,
int ldab, @Const IntPointer ipiv, float anorm,
FloatPointer rcond );
public static native int LAPACKE_sgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const FloatBuffer ab,
int ldab, @Const IntBuffer ipiv, float anorm,
FloatBuffer rcond );
public static native int LAPACKE_sgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const float[] ab,
int ldab, @Const int[] ipiv, float anorm,
float[] rcond );
public static native int LAPACKE_dgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const DoublePointer ab,
int ldab, @Const IntPointer ipiv,
double anorm, DoublePointer rcond );
public static native int LAPACKE_dgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const DoubleBuffer ab,
int ldab, @Const IntBuffer ipiv,
double anorm, DoubleBuffer rcond );
public static native int LAPACKE_dgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const double[] ab,
int ldab, @Const int[] ipiv,
double anorm, double[] rcond );
public static native int LAPACKE_cgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Const IntPointer ipiv, float anorm, FloatPointer rcond );
public static native int LAPACKE_cgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Const IntBuffer ipiv, float anorm, FloatBuffer rcond );
public static native int LAPACKE_cgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Const int[] ipiv, float anorm, float[] rcond );
public static native int LAPACKE_zgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond );
public static native int LAPACKE_zgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_zgbcon( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
@Const int[] ipiv, double anorm,
double[] rcond );
public static native int LAPACKE_sgbequ( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatPointer ab,
int ldab, FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_sgbequ( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatBuffer ab,
int ldab, FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_sgbequ( int matrix_layout, int m, int n,
int kl, int ku, @Const float[] ab,
int ldab, float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax );
public static native int LAPACKE_dgbequ( int matrix_layout, int m, int n,
int kl, int ku, @Const DoublePointer ab,
int ldab, DoublePointer r, DoublePointer c,
DoublePointer rowcnd, DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_dgbequ( int matrix_layout, int m, int n,
int kl, int ku, @Const DoubleBuffer ab,
int ldab, DoubleBuffer r, DoubleBuffer c,
DoubleBuffer rowcnd, DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_dgbequ( int matrix_layout, int m, int n,
int kl, int ku, @Const double[] ab,
int ldab, double[] r, double[] c,
double[] rowcnd, double[] colcnd, double[] amax );
public static native int LAPACKE_cgbequ( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
FloatPointer r, FloatPointer c, FloatPointer rowcnd, FloatPointer colcnd,
FloatPointer amax );
public static native int LAPACKE_cgbequ( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd, FloatBuffer colcnd,
FloatBuffer amax );
public static native int LAPACKE_cgbequ( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
float[] r, float[] c, float[] rowcnd, float[] colcnd,
float[] amax );
public static native int LAPACKE_zgbequ( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
DoublePointer r, DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd,
DoublePointer amax );
public static native int LAPACKE_zgbequ( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd,
DoubleBuffer amax );
public static native int LAPACKE_zgbequ( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
double[] r, double[] c, double[] rowcnd, double[] colcnd,
double[] amax );
public static native int LAPACKE_sgbequb( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatPointer ab,
int ldab, FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_sgbequb( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatBuffer ab,
int ldab, FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_sgbequb( int matrix_layout, int m, int n,
int kl, int ku, @Const float[] ab,
int ldab, float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax );
public static native int LAPACKE_dgbequb( int matrix_layout, int m, int n,
int kl, int ku, @Const DoublePointer ab,
int ldab, DoublePointer r, DoublePointer c,
DoublePointer rowcnd, DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_dgbequb( int matrix_layout, int m, int n,
int kl, int ku, @Const DoubleBuffer ab,
int ldab, DoubleBuffer r, DoubleBuffer c,
DoubleBuffer rowcnd, DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_dgbequb( int matrix_layout, int m, int n,
int kl, int ku, @Const double[] ab,
int ldab, double[] r, double[] c,
double[] rowcnd, double[] colcnd, double[] amax );
public static native int LAPACKE_cgbequb( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
FloatPointer r, FloatPointer c, FloatPointer rowcnd, FloatPointer colcnd,
FloatPointer amax );
public static native int LAPACKE_cgbequb( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd, FloatBuffer colcnd,
FloatBuffer amax );
public static native int LAPACKE_cgbequb( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
float[] r, float[] c, float[] rowcnd, float[] colcnd,
float[] amax );
public static native int LAPACKE_zgbequb( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
DoublePointer r, DoublePointer c, DoublePointer rowcnd,
DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_zgbequb( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd,
DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_zgbequb( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
double[] r, double[] c, double[] rowcnd,
double[] colcnd, double[] amax );
public static native int LAPACKE_sgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const FloatPointer ab, int ldab, @Const FloatPointer afb,
int ldafb, @Const IntPointer ipiv,
@Const FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_sgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const FloatBuffer ab, int ldab, @Const FloatBuffer afb,
int ldafb, @Const IntBuffer ipiv,
@Const FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_sgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const float[] ab, int ldab, @Const float[] afb,
int ldafb, @Const int[] ipiv,
@Const float[] b, int ldb, float[] x,
int ldx, float[] ferr, float[] berr );
public static native int LAPACKE_dgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const DoublePointer ab, int ldab, @Const DoublePointer afb,
int ldafb, @Const IntPointer ipiv,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const DoubleBuffer ab, int ldab, @Const DoubleBuffer afb,
int ldafb, @Const IntBuffer ipiv,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const double[] ab, int ldab, @Const double[] afb,
int ldafb, @Const int[] ipiv,
@Const double[] b, int ldb, double[] x,
int ldx, double[] ferr, double[] berr );
public static native int LAPACKE_cgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("const lapack_complex_float*") FloatPointer afb, int ldafb,
@Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_cgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("const lapack_complex_float*") FloatBuffer afb, int ldafb,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_cgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Cast("const lapack_complex_float*") float[] afb, int ldafb,
@Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("const lapack_complex_double*") DoublePointer afb, int ldafb,
@Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("const lapack_complex_double*") DoubleBuffer afb, int ldafb,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zgbrfs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
@Cast("const lapack_complex_double*") double[] afb, int ldafb,
@Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_sgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs, FloatPointer ab,
int ldab, IntPointer ipiv, FloatPointer b,
int ldb );
public static native int LAPACKE_sgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs, FloatBuffer ab,
int ldab, IntBuffer ipiv, FloatBuffer b,
int ldb );
public static native int LAPACKE_sgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs, float[] ab,
int ldab, int[] ipiv, float[] b,
int ldb );
public static native int LAPACKE_dgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs, DoublePointer ab,
int ldab, IntPointer ipiv, DoublePointer b,
int ldb );
public static native int LAPACKE_dgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs, DoubleBuffer ab,
int ldab, IntBuffer ipiv, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs, double[] ab,
int ldab, int[] ipiv, double[] b,
int ldb );
public static native int LAPACKE_cgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_float*") float[] ab, int ldab,
int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zgbsv( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_double*") double[] ab, int ldab,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_sgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, FloatPointer ab, int ldab,
FloatPointer afb, int ldafb, IntPointer ipiv,
@Cast("char*") BytePointer equed, FloatPointer r, FloatPointer c, FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer rpivot );
public static native int LAPACKE_sgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, FloatBuffer ab, int ldab,
FloatBuffer afb, int ldafb, IntBuffer ipiv,
@Cast("char*") ByteBuffer equed, FloatBuffer r, FloatBuffer c, FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer rpivot );
public static native int LAPACKE_sgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, float[] ab, int ldab,
float[] afb, int ldafb, int[] ipiv,
@Cast("char*") byte[] equed, float[] r, float[] c, float[] b,
int ldb, float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
float[] rpivot );
public static native int LAPACKE_dgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, DoublePointer ab, int ldab,
DoublePointer afb, int ldafb, IntPointer ipiv,
@Cast("char*") BytePointer equed, DoublePointer r, DoublePointer c, DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer rpivot );
public static native int LAPACKE_dgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, DoubleBuffer ab, int ldab,
DoubleBuffer afb, int ldafb, IntBuffer ipiv,
@Cast("char*") ByteBuffer equed, DoubleBuffer r, DoubleBuffer c, DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer rpivot );
public static native int LAPACKE_dgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, double[] ab, int ldab,
double[] afb, int ldafb, int[] ipiv,
@Cast("char*") byte[] equed, double[] r, double[] c, double[] b,
int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
double[] rpivot );
public static native int LAPACKE_cgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab, @Cast("lapack_complex_float*") FloatPointer afb,
int ldafb, IntPointer ipiv, @Cast("char*") BytePointer equed,
FloatPointer r, FloatPointer c, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, FloatPointer rpivot );
public static native int LAPACKE_cgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab, @Cast("lapack_complex_float*") FloatBuffer afb,
int ldafb, IntBuffer ipiv, @Cast("char*") ByteBuffer equed,
FloatBuffer r, FloatBuffer c, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, FloatBuffer rpivot );
public static native int LAPACKE_cgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_float*") float[] ab,
int ldab, @Cast("lapack_complex_float*") float[] afb,
int ldafb, int[] ipiv, @Cast("char*") byte[] equed,
float[] r, float[] c, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, float[] rpivot );
public static native int LAPACKE_zgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab, @Cast("lapack_complex_double*") DoublePointer afb,
int ldafb, IntPointer ipiv, @Cast("char*") BytePointer equed,
DoublePointer r, DoublePointer c, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr, DoublePointer rpivot );
public static native int LAPACKE_zgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab, @Cast("lapack_complex_double*") DoubleBuffer afb,
int ldafb, IntBuffer ipiv, @Cast("char*") ByteBuffer equed,
DoubleBuffer r, DoubleBuffer c, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer rpivot );
public static native int LAPACKE_zgbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_double*") double[] ab,
int ldab, @Cast("lapack_complex_double*") double[] afb,
int ldafb, int[] ipiv, @Cast("char*") byte[] equed,
double[] r, double[] c, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, double[] rcond, double[] ferr,
double[] berr, double[] rpivot );
public static native int LAPACKE_sgbtrf( int matrix_layout, int m, int n,
int kl, int ku, FloatPointer ab,
int ldab, IntPointer ipiv );
public static native int LAPACKE_sgbtrf( int matrix_layout, int m, int n,
int kl, int ku, FloatBuffer ab,
int ldab, IntBuffer ipiv );
public static native int LAPACKE_sgbtrf( int matrix_layout, int m, int n,
int kl, int ku, float[] ab,
int ldab, int[] ipiv );
public static native int LAPACKE_dgbtrf( int matrix_layout, int m, int n,
int kl, int ku, DoublePointer ab,
int ldab, IntPointer ipiv );
public static native int LAPACKE_dgbtrf( int matrix_layout, int m, int n,
int kl, int ku, DoubleBuffer ab,
int ldab, IntBuffer ipiv );
public static native int LAPACKE_dgbtrf( int matrix_layout, int m, int n,
int kl, int ku, double[] ab,
int ldab, int[] ipiv );
public static native int LAPACKE_cgbtrf( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
IntPointer ipiv );
public static native int LAPACKE_cgbtrf( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
IntBuffer ipiv );
public static native int LAPACKE_cgbtrf( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_float*") float[] ab, int ldab,
int[] ipiv );
public static native int LAPACKE_zgbtrf( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
IntPointer ipiv );
public static native int LAPACKE_zgbtrf( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
IntBuffer ipiv );
public static native int LAPACKE_zgbtrf( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_double*") double[] ab, int ldab,
int[] ipiv );
public static native int LAPACKE_sgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const FloatPointer ab, int ldab,
@Const IntPointer ipiv, FloatPointer b, int ldb );
public static native int LAPACKE_sgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const FloatBuffer ab, int ldab,
@Const IntBuffer ipiv, FloatBuffer b, int ldb );
public static native int LAPACKE_sgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const float[] ab, int ldab,
@Const int[] ipiv, float[] b, int ldb );
public static native int LAPACKE_dgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const DoublePointer ab, int ldab,
@Const IntPointer ipiv, DoublePointer b, int ldb );
public static native int LAPACKE_dgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const DoubleBuffer ab, int ldab,
@Const IntBuffer ipiv, DoubleBuffer b, int ldb );
public static native int LAPACKE_dgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const double[] ab, int ldab,
@Const int[] ipiv, double[] b, int ldb );
public static native int LAPACKE_cgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zgbtrs( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_sgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const FloatPointer scale,
int m, FloatPointer v, int ldv );
public static native int LAPACKE_sgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const FloatBuffer scale,
int m, FloatBuffer v, int ldv );
public static native int LAPACKE_sgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const float[] scale,
int m, float[] v, int ldv );
public static native int LAPACKE_dgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const DoublePointer scale,
int m, DoublePointer v, int ldv );
public static native int LAPACKE_dgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const DoubleBuffer scale,
int m, DoubleBuffer v, int ldv );
public static native int LAPACKE_dgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const double[] scale,
int m, double[] v, int ldv );
public static native int LAPACKE_cgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const FloatPointer scale,
int m, @Cast("lapack_complex_float*") FloatPointer v,
int ldv );
public static native int LAPACKE_cgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const FloatBuffer scale,
int m, @Cast("lapack_complex_float*") FloatBuffer v,
int ldv );
public static native int LAPACKE_cgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const float[] scale,
int m, @Cast("lapack_complex_float*") float[] v,
int ldv );
public static native int LAPACKE_zgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const DoublePointer scale,
int m, @Cast("lapack_complex_double*") DoublePointer v,
int ldv );
public static native int LAPACKE_zgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const DoubleBuffer scale,
int m, @Cast("lapack_complex_double*") DoubleBuffer v,
int ldv );
public static native int LAPACKE_zgebak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const double[] scale,
int m, @Cast("lapack_complex_double*") double[] v,
int ldv );
public static native int LAPACKE_sgebal( int matrix_layout, @Cast("char") byte job, int n, FloatPointer a,
int lda, IntPointer ilo, IntPointer ihi,
FloatPointer scale );
public static native int LAPACKE_sgebal( int matrix_layout, @Cast("char") byte job, int n, FloatBuffer a,
int lda, IntBuffer ilo, IntBuffer ihi,
FloatBuffer scale );
public static native int LAPACKE_sgebal( int matrix_layout, @Cast("char") byte job, int n, float[] a,
int lda, int[] ilo, int[] ihi,
float[] scale );
public static native int LAPACKE_dgebal( int matrix_layout, @Cast("char") byte job, int n, DoublePointer a,
int lda, IntPointer ilo, IntPointer ihi,
DoublePointer scale );
public static native int LAPACKE_dgebal( int matrix_layout, @Cast("char") byte job, int n, DoubleBuffer a,
int lda, IntBuffer ilo, IntBuffer ihi,
DoubleBuffer scale );
public static native int LAPACKE_dgebal( int matrix_layout, @Cast("char") byte job, int n, double[] a,
int lda, int[] ilo, int[] ihi,
double[] scale );
public static native int LAPACKE_cgebal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ilo, IntPointer ihi, FloatPointer scale );
public static native int LAPACKE_cgebal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ilo, IntBuffer ihi, FloatBuffer scale );
public static native int LAPACKE_cgebal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ilo, int[] ihi, float[] scale );
public static native int LAPACKE_zgebal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ilo, IntPointer ihi, DoublePointer scale );
public static native int LAPACKE_zgebal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer scale );
public static native int LAPACKE_zgebal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ilo, int[] ihi, double[] scale );
public static native int LAPACKE_sgebrd( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer d, FloatPointer e,
FloatPointer tauq, FloatPointer taup );
public static native int LAPACKE_sgebrd( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer d, FloatBuffer e,
FloatBuffer tauq, FloatBuffer taup );
public static native int LAPACKE_sgebrd( int matrix_layout, int m, int n,
float[] a, int lda, float[] d, float[] e,
float[] tauq, float[] taup );
public static native int LAPACKE_dgebrd( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer d, DoublePointer e,
DoublePointer tauq, DoublePointer taup );
public static native int LAPACKE_dgebrd( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer d, DoubleBuffer e,
DoubleBuffer tauq, DoubleBuffer taup );
public static native int LAPACKE_dgebrd( int matrix_layout, int m, int n,
double[] a, int lda, double[] d, double[] e,
double[] tauq, double[] taup );
public static native int LAPACKE_cgebrd( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda, FloatPointer d,
FloatPointer e, @Cast("lapack_complex_float*") FloatPointer tauq,
@Cast("lapack_complex_float*") FloatPointer taup );
public static native int LAPACKE_cgebrd( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda, FloatBuffer d,
FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer tauq,
@Cast("lapack_complex_float*") FloatBuffer taup );
public static native int LAPACKE_cgebrd( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda, float[] d,
float[] e, @Cast("lapack_complex_float*") float[] tauq,
@Cast("lapack_complex_float*") float[] taup );
public static native int LAPACKE_zgebrd( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda, DoublePointer d,
DoublePointer e, @Cast("lapack_complex_double*") DoublePointer tauq,
@Cast("lapack_complex_double*") DoublePointer taup );
public static native int LAPACKE_zgebrd( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda, DoubleBuffer d,
DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer tauq,
@Cast("lapack_complex_double*") DoubleBuffer taup );
public static native int LAPACKE_zgebrd( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda, double[] d,
double[] e, @Cast("lapack_complex_double*") double[] tauq,
@Cast("lapack_complex_double*") double[] taup );
public static native int LAPACKE_sgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Const FloatPointer a, int lda, float anorm,
FloatPointer rcond );
public static native int LAPACKE_sgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Const FloatBuffer a, int lda, float anorm,
FloatBuffer rcond );
public static native int LAPACKE_sgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Const float[] a, int lda, float anorm,
float[] rcond );
public static native int LAPACKE_dgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Const DoublePointer a, int lda, double anorm,
DoublePointer rcond );
public static native int LAPACKE_dgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Const DoubleBuffer a, int lda, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_dgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Const double[] a, int lda, double anorm,
double[] rcond );
public static native int LAPACKE_cgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
float anorm, FloatPointer rcond );
public static native int LAPACKE_cgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
float anorm, FloatBuffer rcond );
public static native int LAPACKE_cgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float anorm, float[] rcond );
public static native int LAPACKE_zgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
double anorm, DoublePointer rcond );
public static native int LAPACKE_zgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
double anorm, DoubleBuffer rcond );
public static native int LAPACKE_zgecon( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double anorm, double[] rcond );
public static native int LAPACKE_sgeequ( int matrix_layout, int m, int n,
@Const FloatPointer a, int lda, FloatPointer r, FloatPointer c,
FloatPointer rowcnd, FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_sgeequ( int matrix_layout, int m, int n,
@Const FloatBuffer a, int lda, FloatBuffer r, FloatBuffer c,
FloatBuffer rowcnd, FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_sgeequ( int matrix_layout, int m, int n,
@Const float[] a, int lda, float[] r, float[] c,
float[] rowcnd, float[] colcnd, float[] amax );
public static native int LAPACKE_dgeequ( int matrix_layout, int m, int n,
@Const DoublePointer a, int lda, DoublePointer r,
DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd,
DoublePointer amax );
public static native int LAPACKE_dgeequ( int matrix_layout, int m, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer r,
DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd,
DoubleBuffer amax );
public static native int LAPACKE_dgeequ( int matrix_layout, int m, int n,
@Const double[] a, int lda, double[] r,
double[] c, double[] rowcnd, double[] colcnd,
double[] amax );
public static native int LAPACKE_cgeequ( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer r, FloatPointer c, FloatPointer rowcnd, FloatPointer colcnd,
FloatPointer amax );
public static native int LAPACKE_cgeequ( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd, FloatBuffer colcnd,
FloatBuffer amax );
public static native int LAPACKE_cgeequ( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] r, float[] c, float[] rowcnd, float[] colcnd,
float[] amax );
public static native int LAPACKE_zgeequ( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer r, DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd,
DoublePointer amax );
public static native int LAPACKE_zgeequ( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd,
DoubleBuffer amax );
public static native int LAPACKE_zgeequ( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] r, double[] c, double[] rowcnd, double[] colcnd,
double[] amax );
public static native int LAPACKE_sgeequb( int matrix_layout, int m, int n,
@Const FloatPointer a, int lda, FloatPointer r, FloatPointer c,
FloatPointer rowcnd, FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_sgeequb( int matrix_layout, int m, int n,
@Const FloatBuffer a, int lda, FloatBuffer r, FloatBuffer c,
FloatBuffer rowcnd, FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_sgeequb( int matrix_layout, int m, int n,
@Const float[] a, int lda, float[] r, float[] c,
float[] rowcnd, float[] colcnd, float[] amax );
public static native int LAPACKE_dgeequb( int matrix_layout, int m, int n,
@Const DoublePointer a, int lda, DoublePointer r,
DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd,
DoublePointer amax );
public static native int LAPACKE_dgeequb( int matrix_layout, int m, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer r,
DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd,
DoubleBuffer amax );
public static native int LAPACKE_dgeequb( int matrix_layout, int m, int n,
@Const double[] a, int lda, double[] r,
double[] c, double[] rowcnd, double[] colcnd,
double[] amax );
public static native int LAPACKE_cgeequb( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer r, FloatPointer c, FloatPointer rowcnd, FloatPointer colcnd,
FloatPointer amax );
public static native int LAPACKE_cgeequb( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd, FloatBuffer colcnd,
FloatBuffer amax );
public static native int LAPACKE_cgeequb( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] r, float[] c, float[] rowcnd, float[] colcnd,
float[] amax );
public static native int LAPACKE_zgeequb( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer r, DoublePointer c, DoublePointer rowcnd,
DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_zgeequb( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd,
DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_zgeequb( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] r, double[] c, double[] rowcnd,
double[] colcnd, double[] amax );
public static native int LAPACKE_sgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, int n, FloatPointer a,
int lda, IntPointer sdim, FloatPointer wr,
FloatPointer wi, FloatPointer vs, int ldvs );
public static native int LAPACKE_sgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, int n, FloatBuffer a,
int lda, IntBuffer sdim, FloatBuffer wr,
FloatBuffer wi, FloatBuffer vs, int ldvs );
public static native int LAPACKE_sgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, int n, float[] a,
int lda, int[] sdim, float[] wr,
float[] wi, float[] vs, int ldvs );
public static native int LAPACKE_dgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, int n, DoublePointer a,
int lda, IntPointer sdim, DoublePointer wr,
DoublePointer wi, DoublePointer vs, int ldvs );
public static native int LAPACKE_dgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, int n, DoubleBuffer a,
int lda, IntBuffer sdim, DoubleBuffer wr,
DoubleBuffer wi, DoubleBuffer vs, int ldvs );
public static native int LAPACKE_dgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, int n, double[] a,
int lda, int[] sdim, double[] wr,
double[] wi, double[] vs, int ldvs );
public static native int LAPACKE_cgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer sdim, @Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer vs, int ldvs );
public static native int LAPACKE_cgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer sdim, @Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer vs, int ldvs );
public static native int LAPACKE_cgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] sdim, @Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] vs, int ldvs );
public static native int LAPACKE_zgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer sdim, @Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vs, int ldvs );
public static native int LAPACKE_zgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer sdim, @Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vs, int ldvs );
public static native int LAPACKE_zgees( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] sdim, @Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vs, int ldvs );
public static native int LAPACKE_sgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, @Cast("char") byte sense, int n,
FloatPointer a, int lda, IntPointer sdim,
FloatPointer wr, FloatPointer wi, FloatPointer vs, int ldvs,
FloatPointer rconde, FloatPointer rcondv );
public static native int LAPACKE_sgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, @Cast("char") byte sense, int n,
FloatBuffer a, int lda, IntBuffer sdim,
FloatBuffer wr, FloatBuffer wi, FloatBuffer vs, int ldvs,
FloatBuffer rconde, FloatBuffer rcondv );
public static native int LAPACKE_sgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, @Cast("char") byte sense, int n,
float[] a, int lda, int[] sdim,
float[] wr, float[] wi, float[] vs, int ldvs,
float[] rconde, float[] rcondv );
public static native int LAPACKE_dgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, @Cast("char") byte sense, int n,
DoublePointer a, int lda, IntPointer sdim,
DoublePointer wr, DoublePointer wi, DoublePointer vs, int ldvs,
DoublePointer rconde, DoublePointer rcondv );
public static native int LAPACKE_dgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, @Cast("char") byte sense, int n,
DoubleBuffer a, int lda, IntBuffer sdim,
DoubleBuffer wr, DoubleBuffer wi, DoubleBuffer vs, int ldvs,
DoubleBuffer rconde, DoubleBuffer rcondv );
public static native int LAPACKE_dgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, @Cast("char") byte sense, int n,
double[] a, int lda, int[] sdim,
double[] wr, double[] wi, double[] vs, int ldvs,
double[] rconde, double[] rcondv );
public static native int LAPACKE_cgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer sdim, @Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer vs, int ldvs,
FloatPointer rconde, FloatPointer rcondv );
public static native int LAPACKE_cgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer sdim, @Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer vs, int ldvs,
FloatBuffer rconde, FloatBuffer rcondv );
public static native int LAPACKE_cgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] sdim, @Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] vs, int ldvs,
float[] rconde, float[] rcondv );
public static native int LAPACKE_zgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer sdim, @Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vs, int ldvs,
DoublePointer rconde, DoublePointer rcondv );
public static native int LAPACKE_zgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer sdim, @Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vs, int ldvs,
DoubleBuffer rconde, DoubleBuffer rcondv );
public static native int LAPACKE_zgeesx( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] sdim, @Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vs, int ldvs,
double[] rconde, double[] rcondv );
public static native int LAPACKE_sgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatPointer a, int lda, FloatPointer wr,
FloatPointer wi, FloatPointer vl, int ldvl, FloatPointer vr,
int ldvr );
public static native int LAPACKE_sgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatBuffer a, int lda, FloatBuffer wr,
FloatBuffer wi, FloatBuffer vl, int ldvl, FloatBuffer vr,
int ldvr );
public static native int LAPACKE_sgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, float[] a, int lda, float[] wr,
float[] wi, float[] vl, int ldvl, float[] vr,
int ldvr );
public static native int LAPACKE_dgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoublePointer a, int lda, DoublePointer wr,
DoublePointer wi, DoublePointer vl, int ldvl, DoublePointer vr,
int ldvr );
public static native int LAPACKE_dgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoubleBuffer a, int lda, DoubleBuffer wr,
DoubleBuffer wi, DoubleBuffer vl, int ldvl, DoubleBuffer vr,
int ldvr );
public static native int LAPACKE_dgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, double[] a, int lda, double[] wr,
double[] wi, double[] vl, int ldvl, double[] vr,
int ldvr );
public static native int LAPACKE_cgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer w, @Cast("lapack_complex_float*") FloatPointer vl,
int ldvl, @Cast("lapack_complex_float*") FloatPointer vr,
int ldvr );
public static native int LAPACKE_cgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer vl,
int ldvl, @Cast("lapack_complex_float*") FloatBuffer vr,
int ldvr );
public static native int LAPACKE_cgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] w, @Cast("lapack_complex_float*") float[] vl,
int ldvl, @Cast("lapack_complex_float*") float[] vr,
int ldvr );
public static native int LAPACKE_zgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr );
public static native int LAPACKE_zgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr );
public static native int LAPACKE_zgeev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr );
public static native int LAPACKE_sgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, FloatPointer a,
int lda, FloatPointer wr, FloatPointer wi, FloatPointer vl,
int ldvl, FloatPointer vr, int ldvr,
IntPointer ilo, IntPointer ihi, FloatPointer scale,
FloatPointer abnrm, FloatPointer rconde, FloatPointer rcondv );
public static native int LAPACKE_sgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, FloatBuffer a,
int lda, FloatBuffer wr, FloatBuffer wi, FloatBuffer vl,
int ldvl, FloatBuffer vr, int ldvr,
IntBuffer ilo, IntBuffer ihi, FloatBuffer scale,
FloatBuffer abnrm, FloatBuffer rconde, FloatBuffer rcondv );
public static native int LAPACKE_sgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, float[] a,
int lda, float[] wr, float[] wi, float[] vl,
int ldvl, float[] vr, int ldvr,
int[] ilo, int[] ihi, float[] scale,
float[] abnrm, float[] rconde, float[] rcondv );
public static native int LAPACKE_dgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, DoublePointer a,
int lda, DoublePointer wr, DoublePointer wi, DoublePointer vl,
int ldvl, DoublePointer vr, int ldvr,
IntPointer ilo, IntPointer ihi, DoublePointer scale,
DoublePointer abnrm, DoublePointer rconde, DoublePointer rcondv );
public static native int LAPACKE_dgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, DoubleBuffer a,
int lda, DoubleBuffer wr, DoubleBuffer wi, DoubleBuffer vl,
int ldvl, DoubleBuffer vr, int ldvr,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer scale,
DoubleBuffer abnrm, DoubleBuffer rconde, DoubleBuffer rcondv );
public static native int LAPACKE_dgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, double[] a,
int lda, double[] wr, double[] wi, double[] vl,
int ldvl, double[] vr, int ldvr,
int[] ilo, int[] ihi, double[] scale,
double[] abnrm, double[] rconde, double[] rcondv );
public static native int LAPACKE_cgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer w, @Cast("lapack_complex_float*") FloatPointer vl,
int ldvl, @Cast("lapack_complex_float*") FloatPointer vr,
int ldvr, IntPointer ilo, IntPointer ihi,
FloatPointer scale, FloatPointer abnrm, FloatPointer rconde,
FloatPointer rcondv );
public static native int LAPACKE_cgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer vl,
int ldvl, @Cast("lapack_complex_float*") FloatBuffer vr,
int ldvr, IntBuffer ilo, IntBuffer ihi,
FloatBuffer scale, FloatBuffer abnrm, FloatBuffer rconde,
FloatBuffer rcondv );
public static native int LAPACKE_cgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] w, @Cast("lapack_complex_float*") float[] vl,
int ldvl, @Cast("lapack_complex_float*") float[] vr,
int ldvr, int[] ilo, int[] ihi,
float[] scale, float[] abnrm, float[] rconde,
float[] rcondv );
public static native int LAPACKE_zgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer w, @Cast("lapack_complex_double*") DoublePointer vl,
int ldvl, @Cast("lapack_complex_double*") DoublePointer vr,
int ldvr, IntPointer ilo, IntPointer ihi,
DoublePointer scale, DoublePointer abnrm, DoublePointer rconde,
DoublePointer rcondv );
public static native int LAPACKE_zgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer vl,
int ldvl, @Cast("lapack_complex_double*") DoubleBuffer vr,
int ldvr, IntBuffer ilo, IntBuffer ihi,
DoubleBuffer scale, DoubleBuffer abnrm, DoubleBuffer rconde,
DoubleBuffer rcondv );
public static native int LAPACKE_zgeevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] w, @Cast("lapack_complex_double*") double[] vl,
int ldvl, @Cast("lapack_complex_double*") double[] vr,
int ldvr, int[] ilo, int[] ihi,
double[] scale, double[] abnrm, double[] rconde,
double[] rcondv );
public static native int LAPACKE_sgehrd( int matrix_layout, int n, int ilo,
int ihi, FloatPointer a, int lda,
FloatPointer tau );
public static native int LAPACKE_sgehrd( int matrix_layout, int n, int ilo,
int ihi, FloatBuffer a, int lda,
FloatBuffer tau );
public static native int LAPACKE_sgehrd( int matrix_layout, int n, int ilo,
int ihi, float[] a, int lda,
float[] tau );
public static native int LAPACKE_dgehrd( int matrix_layout, int n, int ilo,
int ihi, DoublePointer a, int lda,
DoublePointer tau );
public static native int LAPACKE_dgehrd( int matrix_layout, int n, int ilo,
int ihi, DoubleBuffer a, int lda,
DoubleBuffer tau );
public static native int LAPACKE_dgehrd( int matrix_layout, int n, int ilo,
int ihi, double[] a, int lda,
double[] tau );
public static native int LAPACKE_cgehrd( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cgehrd( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cgehrd( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zgehrd( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zgehrd( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zgehrd( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_sgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, FloatPointer a, int lda, FloatPointer sva,
FloatPointer u, int ldu, FloatPointer v, int ldv,
FloatPointer stat, IntPointer istat );
public static native int LAPACKE_sgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, FloatBuffer a, int lda, FloatBuffer sva,
FloatBuffer u, int ldu, FloatBuffer v, int ldv,
FloatBuffer stat, IntBuffer istat );
public static native int LAPACKE_sgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, float[] a, int lda, float[] sva,
float[] u, int ldu, float[] v, int ldv,
float[] stat, int[] istat );
public static native int LAPACKE_dgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, DoublePointer a, int lda, DoublePointer sva,
DoublePointer u, int ldu, DoublePointer v, int ldv,
DoublePointer stat, IntPointer istat );
public static native int LAPACKE_dgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, DoubleBuffer a, int lda, DoubleBuffer sva,
DoubleBuffer u, int ldu, DoubleBuffer v, int ldv,
DoubleBuffer stat, IntBuffer istat );
public static native int LAPACKE_dgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, double[] a, int lda, double[] sva,
double[] u, int ldu, double[] v, int ldv,
double[] stat, int[] istat );
public static native int LAPACKE_cgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, @Cast("lapack_complex_float*") FloatPointer a, int lda, FloatPointer sva,
@Cast("lapack_complex_float*") FloatPointer u, int ldu, @Cast("lapack_complex_float*") FloatPointer v, int ldv,
FloatPointer stat, IntPointer istat );
public static native int LAPACKE_cgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, @Cast("lapack_complex_float*") FloatBuffer a, int lda, FloatBuffer sva,
@Cast("lapack_complex_float*") FloatBuffer u, int ldu, @Cast("lapack_complex_float*") FloatBuffer v, int ldv,
FloatBuffer stat, IntBuffer istat );
public static native int LAPACKE_cgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, @Cast("lapack_complex_float*") float[] a, int lda, float[] sva,
@Cast("lapack_complex_float*") float[] u, int ldu, @Cast("lapack_complex_float*") float[] v, int ldv,
float[] stat, int[] istat );
public static native int LAPACKE_zgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, @Cast("lapack_complex_double*") DoublePointer a, int lda, DoublePointer sva,
@Cast("lapack_complex_double*") DoublePointer u, int ldu, @Cast("lapack_complex_double*") DoublePointer v, int ldv,
DoublePointer stat, IntPointer istat );
public static native int LAPACKE_zgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, @Cast("lapack_complex_double*") DoubleBuffer a, int lda, DoubleBuffer sva,
@Cast("lapack_complex_double*") DoubleBuffer u, int ldu, @Cast("lapack_complex_double*") DoubleBuffer v, int ldv,
DoubleBuffer stat, IntBuffer istat );
public static native int LAPACKE_zgejsv( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp, int m,
int n, @Cast("lapack_complex_double*") double[] a, int lda, double[] sva,
@Cast("lapack_complex_double*") double[] u, int ldu, @Cast("lapack_complex_double*") double[] v, int ldv,
double[] stat, int[] istat );
public static native int LAPACKE_sgelq2( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau );
public static native int LAPACKE_sgelq2( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau );
public static native int LAPACKE_sgelq2( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau );
public static native int LAPACKE_dgelq2( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau );
public static native int LAPACKE_dgelq2( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau );
public static native int LAPACKE_dgelq2( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau );
public static native int LAPACKE_cgelq2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cgelq2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cgelq2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zgelq2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zgelq2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zgelq2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_sgelqf( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau );
public static native int LAPACKE_sgelqf( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau );
public static native int LAPACKE_sgelqf( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau );
public static native int LAPACKE_dgelqf( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau );
public static native int LAPACKE_dgelqf( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau );
public static native int LAPACKE_dgelqf( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau );
public static native int LAPACKE_cgelqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cgelqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cgelqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zgelqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zgelqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zgelqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_sgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, FloatPointer a,
int lda, FloatPointer b, int ldb );
public static native int LAPACKE_sgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, FloatBuffer a,
int lda, FloatBuffer b, int ldb );
public static native int LAPACKE_sgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, float[] a,
int lda, float[] b, int ldb );
public static native int LAPACKE_dgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, DoublePointer a,
int lda, DoublePointer b, int ldb );
public static native int LAPACKE_dgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb );
public static native int LAPACKE_dgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, double[] a,
int lda, double[] b, int ldb );
public static native int LAPACKE_cgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zgels( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sgelsd( int matrix_layout, int m, int n,
int nrhs, FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer s, float rcond,
IntPointer rank );
public static native int LAPACKE_sgelsd( int matrix_layout, int m, int n,
int nrhs, FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer s, float rcond,
IntBuffer rank );
public static native int LAPACKE_sgelsd( int matrix_layout, int m, int n,
int nrhs, float[] a, int lda, float[] b,
int ldb, float[] s, float rcond,
int[] rank );
public static native int LAPACKE_dgelsd( int matrix_layout, int m, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer s, double rcond,
IntPointer rank );
public static native int LAPACKE_dgelsd( int matrix_layout, int m, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer s, double rcond,
IntBuffer rank );
public static native int LAPACKE_dgelsd( int matrix_layout, int m, int n,
int nrhs, double[] a, int lda,
double[] b, int ldb, double[] s, double rcond,
int[] rank );
public static native int LAPACKE_cgelsd( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, FloatPointer s, float rcond,
IntPointer rank );
public static native int LAPACKE_cgelsd( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, FloatBuffer s, float rcond,
IntBuffer rank );
public static native int LAPACKE_cgelsd( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, float[] s, float rcond,
int[] rank );
public static native int LAPACKE_zgelsd( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, DoublePointer s, double rcond,
IntPointer rank );
public static native int LAPACKE_zgelsd( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, DoubleBuffer s, double rcond,
IntBuffer rank );
public static native int LAPACKE_zgelsd( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, double[] s, double rcond,
int[] rank );
public static native int LAPACKE_sgelss( int matrix_layout, int m, int n,
int nrhs, FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer s, float rcond,
IntPointer rank );
public static native int LAPACKE_sgelss( int matrix_layout, int m, int n,
int nrhs, FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer s, float rcond,
IntBuffer rank );
public static native int LAPACKE_sgelss( int matrix_layout, int m, int n,
int nrhs, float[] a, int lda, float[] b,
int ldb, float[] s, float rcond,
int[] rank );
public static native int LAPACKE_dgelss( int matrix_layout, int m, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer s, double rcond,
IntPointer rank );
public static native int LAPACKE_dgelss( int matrix_layout, int m, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer s, double rcond,
IntBuffer rank );
public static native int LAPACKE_dgelss( int matrix_layout, int m, int n,
int nrhs, double[] a, int lda,
double[] b, int ldb, double[] s, double rcond,
int[] rank );
public static native int LAPACKE_cgelss( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, FloatPointer s, float rcond,
IntPointer rank );
public static native int LAPACKE_cgelss( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, FloatBuffer s, float rcond,
IntBuffer rank );
public static native int LAPACKE_cgelss( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, float[] s, float rcond,
int[] rank );
public static native int LAPACKE_zgelss( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, DoublePointer s, double rcond,
IntPointer rank );
public static native int LAPACKE_zgelss( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, DoubleBuffer s, double rcond,
IntBuffer rank );
public static native int LAPACKE_zgelss( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, double[] s, double rcond,
int[] rank );
public static native int LAPACKE_sgelsy( int matrix_layout, int m, int n,
int nrhs, FloatPointer a, int lda, FloatPointer b,
int ldb, IntPointer jpvt, float rcond,
IntPointer rank );
public static native int LAPACKE_sgelsy( int matrix_layout, int m, int n,
int nrhs, FloatBuffer a, int lda, FloatBuffer b,
int ldb, IntBuffer jpvt, float rcond,
IntBuffer rank );
public static native int LAPACKE_sgelsy( int matrix_layout, int m, int n,
int nrhs, float[] a, int lda, float[] b,
int ldb, int[] jpvt, float rcond,
int[] rank );
public static native int LAPACKE_dgelsy( int matrix_layout, int m, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer b, int ldb, IntPointer jpvt,
double rcond, IntPointer rank );
public static native int LAPACKE_dgelsy( int matrix_layout, int m, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, IntBuffer jpvt,
double rcond, IntBuffer rank );
public static native int LAPACKE_dgelsy( int matrix_layout, int m, int n,
int nrhs, double[] a, int lda,
double[] b, int ldb, int[] jpvt,
double rcond, int[] rank );
public static native int LAPACKE_cgelsy( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, IntPointer jpvt, float rcond,
IntPointer rank );
public static native int LAPACKE_cgelsy( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, IntBuffer jpvt, float rcond,
IntBuffer rank );
public static native int LAPACKE_cgelsy( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, int[] jpvt, float rcond,
int[] rank );
public static native int LAPACKE_zgelsy( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, IntPointer jpvt, double rcond,
IntPointer rank );
public static native int LAPACKE_zgelsy( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, IntBuffer jpvt, double rcond,
IntBuffer rank );
public static native int LAPACKE_zgelsy( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, int[] jpvt, double rcond,
int[] rank );
public static native int LAPACKE_sgeqlf( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau );
public static native int LAPACKE_sgeqlf( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau );
public static native int LAPACKE_sgeqlf( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau );
public static native int LAPACKE_dgeqlf( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau );
public static native int LAPACKE_dgeqlf( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau );
public static native int LAPACKE_dgeqlf( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau );
public static native int LAPACKE_cgeqlf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cgeqlf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cgeqlf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zgeqlf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zgeqlf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zgeqlf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_sgeqp3( int matrix_layout, int m, int n,
FloatPointer a, int lda, IntPointer jpvt,
FloatPointer tau );
public static native int LAPACKE_sgeqp3( int matrix_layout, int m, int n,
FloatBuffer a, int lda, IntBuffer jpvt,
FloatBuffer tau );
public static native int LAPACKE_sgeqp3( int matrix_layout, int m, int n,
float[] a, int lda, int[] jpvt,
float[] tau );
public static native int LAPACKE_dgeqp3( int matrix_layout, int m, int n,
DoublePointer a, int lda, IntPointer jpvt,
DoublePointer tau );
public static native int LAPACKE_dgeqp3( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, IntBuffer jpvt,
DoubleBuffer tau );
public static native int LAPACKE_dgeqp3( int matrix_layout, int m, int n,
double[] a, int lda, int[] jpvt,
double[] tau );
public static native int LAPACKE_cgeqp3( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer jpvt, @Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cgeqp3( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer jpvt, @Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cgeqp3( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] jpvt, @Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zgeqp3( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer jpvt, @Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zgeqp3( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer jpvt, @Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zgeqp3( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] jpvt, @Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_sgeqr2( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau );
public static native int LAPACKE_sgeqr2( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau );
public static native int LAPACKE_sgeqr2( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau );
public static native int LAPACKE_dgeqr2( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau );
public static native int LAPACKE_dgeqr2( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau );
public static native int LAPACKE_dgeqr2( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau );
public static native int LAPACKE_cgeqr2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cgeqr2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cgeqr2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zgeqr2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zgeqr2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zgeqr2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_sgeqrf( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau );
public static native int LAPACKE_sgeqrf( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau );
public static native int LAPACKE_sgeqrf( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau );
public static native int LAPACKE_dgeqrf( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau );
public static native int LAPACKE_dgeqrf( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau );
public static native int LAPACKE_dgeqrf( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau );
public static native int LAPACKE_cgeqrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cgeqrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cgeqrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zgeqrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zgeqrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zgeqrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_sgeqrfp( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau );
public static native int LAPACKE_sgeqrfp( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau );
public static native int LAPACKE_sgeqrfp( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau );
public static native int LAPACKE_dgeqrfp( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau );
public static native int LAPACKE_dgeqrfp( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau );
public static native int LAPACKE_dgeqrfp( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau );
public static native int LAPACKE_cgeqrfp( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cgeqrfp( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cgeqrfp( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zgeqrfp( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zgeqrfp( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zgeqrfp( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_sgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const FloatPointer af, int ldaf,
@Const IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_sgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const FloatBuffer af, int ldaf,
@Const IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_sgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const float[] a, int lda,
@Const float[] af, int ldaf,
@Const int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr );
public static native int LAPACKE_dgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoublePointer a, int lda,
@Const DoublePointer af, int ldaf,
@Const IntPointer ipiv, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoubleBuffer a, int lda,
@Const DoubleBuffer af, int ldaf,
@Const IntBuffer ipiv, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const double[] a, int lda,
@Const double[] af, int ldaf,
@Const int[] ipiv, @Const double[] b,
int ldb, double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_cgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_cgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_cgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zgerfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_sgerqf( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau );
public static native int LAPACKE_sgerqf( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau );
public static native int LAPACKE_sgerqf( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau );
public static native int LAPACKE_dgerqf( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau );
public static native int LAPACKE_dgerqf( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau );
public static native int LAPACKE_dgerqf( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau );
public static native int LAPACKE_cgerqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cgerqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cgerqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zgerqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zgerqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zgerqf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_sgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, FloatPointer a, int lda, FloatPointer s,
FloatPointer u, int ldu, FloatPointer vt,
int ldvt );
public static native int LAPACKE_sgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, FloatBuffer a, int lda, FloatBuffer s,
FloatBuffer u, int ldu, FloatBuffer vt,
int ldvt );
public static native int LAPACKE_sgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, float[] a, int lda, float[] s,
float[] u, int ldu, float[] vt,
int ldvt );
public static native int LAPACKE_dgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, DoublePointer a, int lda, DoublePointer s,
DoublePointer u, int ldu, DoublePointer vt,
int ldvt );
public static native int LAPACKE_dgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, DoubleBuffer a, int lda, DoubleBuffer s,
DoubleBuffer u, int ldu, DoubleBuffer vt,
int ldvt );
public static native int LAPACKE_dgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, double[] a, int lda, double[] s,
double[] u, int ldu, double[] vt,
int ldvt );
public static native int LAPACKE_cgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, FloatPointer s, @Cast("lapack_complex_float*") FloatPointer u,
int ldu, @Cast("lapack_complex_float*") FloatPointer vt,
int ldvt );
public static native int LAPACKE_cgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer u,
int ldu, @Cast("lapack_complex_float*") FloatBuffer vt,
int ldvt );
public static native int LAPACKE_cgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, float[] s, @Cast("lapack_complex_float*") float[] u,
int ldu, @Cast("lapack_complex_float*") float[] vt,
int ldvt );
public static native int LAPACKE_zgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, DoublePointer s, @Cast("lapack_complex_double*") DoublePointer u,
int ldu, @Cast("lapack_complex_double*") DoublePointer vt,
int ldvt );
public static native int LAPACKE_zgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer s, @Cast("lapack_complex_double*") DoubleBuffer u,
int ldu, @Cast("lapack_complex_double*") DoubleBuffer vt,
int ldvt );
public static native int LAPACKE_zgesdd( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, double[] s, @Cast("lapack_complex_double*") double[] u,
int ldu, @Cast("lapack_complex_double*") double[] vt,
int ldvt );
public static native int LAPACKE_sgesv( int matrix_layout, int n, int nrhs,
FloatPointer a, int lda, IntPointer ipiv, FloatPointer b,
int ldb );
public static native int LAPACKE_sgesv( int matrix_layout, int n, int nrhs,
FloatBuffer a, int lda, IntBuffer ipiv, FloatBuffer b,
int ldb );
public static native int LAPACKE_sgesv( int matrix_layout, int n, int nrhs,
float[] a, int lda, int[] ipiv, float[] b,
int ldb );
public static native int LAPACKE_dgesv( int matrix_layout, int n, int nrhs,
DoublePointer a, int lda, IntPointer ipiv,
DoublePointer b, int ldb );
public static native int LAPACKE_dgesv( int matrix_layout, int n, int nrhs,
DoubleBuffer a, int lda, IntBuffer ipiv,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dgesv( int matrix_layout, int n, int nrhs,
double[] a, int lda, int[] ipiv,
double[] b, int ldb );
public static native int LAPACKE_cgesv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cgesv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cgesv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zgesv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zgesv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zgesv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_dsgesv( int matrix_layout, int n, int nrhs,
DoublePointer a, int lda, IntPointer ipiv,
DoublePointer b, int ldb, DoublePointer x, int ldx,
IntPointer iter );
public static native int LAPACKE_dsgesv( int matrix_layout, int n, int nrhs,
DoubleBuffer a, int lda, IntBuffer ipiv,
DoubleBuffer b, int ldb, DoubleBuffer x, int ldx,
IntBuffer iter );
public static native int LAPACKE_dsgesv( int matrix_layout, int n, int nrhs,
double[] a, int lda, int[] ipiv,
double[] b, int ldb, double[] x, int ldx,
int[] iter );
public static native int LAPACKE_zcgesv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, IntPointer iter );
public static native int LAPACKE_zcgesv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, IntBuffer iter );
public static native int LAPACKE_zcgesv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, int[] iter );
public static native int LAPACKE_sgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, FloatPointer a, int lda,
FloatPointer s, FloatPointer u, int ldu, FloatPointer vt,
int ldvt, FloatPointer superb );
public static native int LAPACKE_sgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer u, int ldu, FloatBuffer vt,
int ldvt, FloatBuffer superb );
public static native int LAPACKE_sgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, float[] a, int lda,
float[] s, float[] u, int ldu, float[] vt,
int ldvt, float[] superb );
public static native int LAPACKE_dgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, DoublePointer a,
int lda, DoublePointer s, DoublePointer u, int ldu,
DoublePointer vt, int ldvt, DoublePointer superb );
public static native int LAPACKE_dgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, DoubleBuffer a,
int lda, DoubleBuffer s, DoubleBuffer u, int ldu,
DoubleBuffer vt, int ldvt, DoubleBuffer superb );
public static native int LAPACKE_dgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, double[] a,
int lda, double[] s, double[] u, int ldu,
double[] vt, int ldvt, double[] superb );
public static native int LAPACKE_cgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, FloatPointer s, @Cast("lapack_complex_float*") FloatPointer u,
int ldu, @Cast("lapack_complex_float*") FloatPointer vt,
int ldvt, FloatPointer superb );
public static native int LAPACKE_cgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer u,
int ldu, @Cast("lapack_complex_float*") FloatBuffer vt,
int ldvt, FloatBuffer superb );
public static native int LAPACKE_cgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, @Cast("lapack_complex_float*") float[] a,
int lda, float[] s, @Cast("lapack_complex_float*") float[] u,
int ldu, @Cast("lapack_complex_float*") float[] vt,
int ldvt, float[] superb );
public static native int LAPACKE_zgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, DoublePointer s, @Cast("lapack_complex_double*") DoublePointer u,
int ldu, @Cast("lapack_complex_double*") DoublePointer vt,
int ldvt, DoublePointer superb );
public static native int LAPACKE_zgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer s, @Cast("lapack_complex_double*") DoubleBuffer u,
int ldu, @Cast("lapack_complex_double*") DoubleBuffer vt,
int ldvt, DoubleBuffer superb );
public static native int LAPACKE_zgesvd( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, @Cast("lapack_complex_double*") double[] a,
int lda, double[] s, @Cast("lapack_complex_double*") double[] u,
int ldu, @Cast("lapack_complex_double*") double[] vt,
int ldvt, double[] superb );
public static native int LAPACKE_sgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, FloatPointer a,
int lda, int vl, int vu,
int il, int iu, int ns,
FloatPointer s, FloatPointer u, int ldu,
FloatPointer vt, int ldvt,
IntPointer superb );
public static native int LAPACKE_sgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, FloatBuffer a,
int lda, int vl, int vu,
int il, int iu, int ns,
FloatBuffer s, FloatBuffer u, int ldu,
FloatBuffer vt, int ldvt,
IntBuffer superb );
public static native int LAPACKE_sgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, float[] a,
int lda, int vl, int vu,
int il, int iu, int ns,
float[] s, float[] u, int ldu,
float[] vt, int ldvt,
int[] superb );
public static native int LAPACKE_dgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, DoublePointer a,
int lda, int vl, int vu,
int il, int iu, int ns,
DoublePointer s, DoublePointer u, int ldu,
DoublePointer vt, int ldvt,
IntPointer superb );
public static native int LAPACKE_dgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, DoubleBuffer a,
int lda, int vl, int vu,
int il, int iu, int ns,
DoubleBuffer s, DoubleBuffer u, int ldu,
DoubleBuffer vt, int ldvt,
IntBuffer superb );
public static native int LAPACKE_dgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, double[] a,
int lda, int vl, int vu,
int il, int iu, int ns,
double[] s, double[] u, int ldu,
double[] vt, int ldvt,
int[] superb );
public static native int LAPACKE_cgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, int vl, int vu,
int il, int iu, int ns,
FloatPointer s, @Cast("lapack_complex_float*") FloatPointer u, int ldu,
@Cast("lapack_complex_float*") FloatPointer vt, int ldvt,
IntPointer superb );
public static native int LAPACKE_cgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, int vl, int vu,
int il, int iu, int ns,
FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer u, int ldu,
@Cast("lapack_complex_float*") FloatBuffer vt, int ldvt,
IntBuffer superb );
public static native int LAPACKE_cgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_float*") float[] a,
int lda, int vl, int vu,
int il, int iu, int ns,
float[] s, @Cast("lapack_complex_float*") float[] u, int ldu,
@Cast("lapack_complex_float*") float[] vt, int ldvt,
int[] superb );
public static native int LAPACKE_zgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, int vl, int vu,
int il, int iu, int ns,
DoublePointer s, @Cast("lapack_complex_double*") DoublePointer u, int ldu,
@Cast("lapack_complex_double*") DoublePointer vt, int ldvt,
IntPointer superb );
public static native int LAPACKE_zgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, int vl, int vu,
int il, int iu, int ns,
DoubleBuffer s, @Cast("lapack_complex_double*") DoubleBuffer u, int ldu,
@Cast("lapack_complex_double*") DoubleBuffer vt, int ldvt,
IntBuffer superb );
public static native int LAPACKE_zgesvdx( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_double*") double[] a,
int lda, int vl, int vu,
int il, int iu, int ns,
double[] s, @Cast("lapack_complex_double*") double[] u, int ldu,
@Cast("lapack_complex_double*") double[] vt, int ldvt,
int[] superb );
public static native int LAPACKE_sgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, FloatPointer a, int lda,
FloatPointer sva, int mv, FloatPointer v, int ldv,
FloatPointer stat );
public static native int LAPACKE_sgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, FloatBuffer a, int lda,
FloatBuffer sva, int mv, FloatBuffer v, int ldv,
FloatBuffer stat );
public static native int LAPACKE_sgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, float[] a, int lda,
float[] sva, int mv, float[] v, int ldv,
float[] stat );
public static native int LAPACKE_dgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, DoublePointer a,
int lda, DoublePointer sva, int mv,
DoublePointer v, int ldv, DoublePointer stat );
public static native int LAPACKE_dgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, DoubleBuffer a,
int lda, DoubleBuffer sva, int mv,
DoubleBuffer v, int ldv, DoubleBuffer stat );
public static native int LAPACKE_dgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, double[] a,
int lda, double[] sva, int mv,
double[] v, int ldv, double[] stat );
public static native int LAPACKE_cgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, FloatPointer sva, int mv,
@Cast("lapack_complex_float*") FloatPointer v, int ldv, FloatPointer stat );
public static native int LAPACKE_cgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer sva, int mv,
@Cast("lapack_complex_float*") FloatBuffer v, int ldv, FloatBuffer stat );
public static native int LAPACKE_cgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, @Cast("lapack_complex_float*") float[] a,
int lda, float[] sva, int mv,
@Cast("lapack_complex_float*") float[] v, int ldv, float[] stat );
public static native int LAPACKE_zgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, DoublePointer sva, int mv,
@Cast("lapack_complex_double*") DoublePointer v, int ldv, DoublePointer stat );
public static native int LAPACKE_zgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer sva, int mv,
@Cast("lapack_complex_double*") DoubleBuffer v, int ldv, DoubleBuffer stat );
public static native int LAPACKE_zgesvj( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu, @Cast("char") byte jobv,
int m, int n, @Cast("lapack_complex_double*") double[] a,
int lda, double[] sva, int mv,
@Cast("lapack_complex_double*") double[] v, int ldv, double[] stat );
public static native int LAPACKE_sgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, FloatPointer a,
int lda, FloatPointer af, int ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, FloatPointer r, FloatPointer c,
FloatPointer b, int ldb, FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer rpivot );
public static native int LAPACKE_sgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, FloatBuffer a,
int lda, FloatBuffer af, int ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, FloatBuffer r, FloatBuffer c,
FloatBuffer b, int ldb, FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer rpivot );
public static native int LAPACKE_sgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, float[] a,
int lda, float[] af, int ldaf,
int[] ipiv, @Cast("char*") byte[] equed, float[] r, float[] c,
float[] b, int ldb, float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
float[] rpivot );
public static native int LAPACKE_dgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, DoublePointer a,
int lda, DoublePointer af, int ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, DoublePointer r, DoublePointer c,
DoublePointer b, int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer rpivot );
public static native int LAPACKE_dgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, DoubleBuffer a,
int lda, DoubleBuffer af, int ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, DoubleBuffer r, DoubleBuffer c,
DoubleBuffer b, int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer rpivot );
public static native int LAPACKE_dgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, double[] a,
int lda, double[] af, int ldaf,
int[] ipiv, @Cast("char*") byte[] equed, double[] r, double[] c,
double[] b, int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
double[] rpivot );
public static native int LAPACKE_cgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer af, int ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, FloatPointer r, FloatPointer c,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer rpivot );
public static native int LAPACKE_cgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer af, int ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, FloatBuffer r, FloatBuffer c,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer rpivot );
public static native int LAPACKE_cgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] af, int ldaf,
int[] ipiv, @Cast("char*") byte[] equed, float[] r, float[] c,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
float[] rpivot );
public static native int LAPACKE_zgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer af, int ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, DoublePointer r, DoublePointer c,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer rpivot );
public static native int LAPACKE_zgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer af, int ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, DoubleBuffer r, DoubleBuffer c,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer rpivot );
public static native int LAPACKE_zgesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] af, int ldaf,
int[] ipiv, @Cast("char*") byte[] equed, double[] r, double[] c,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
double[] rpivot );
public static native int LAPACKE_sgetf2( int matrix_layout, int m, int n,
FloatPointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_sgetf2( int matrix_layout, int m, int n,
FloatBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_sgetf2( int matrix_layout, int m, int n,
float[] a, int lda, int[] ipiv );
public static native int LAPACKE_dgetf2( int matrix_layout, int m, int n,
DoublePointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_dgetf2( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_dgetf2( int matrix_layout, int m, int n,
double[] a, int lda, int[] ipiv );
public static native int LAPACKE_cgetf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_cgetf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_cgetf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zgetf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zgetf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zgetf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_sgetrf( int matrix_layout, int m, int n,
FloatPointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_sgetrf( int matrix_layout, int m, int n,
FloatBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_sgetrf( int matrix_layout, int m, int n,
float[] a, int lda, int[] ipiv );
public static native int LAPACKE_dgetrf( int matrix_layout, int m, int n,
DoublePointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_dgetrf( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_dgetrf( int matrix_layout, int m, int n,
double[] a, int lda, int[] ipiv );
public static native int LAPACKE_cgetrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_cgetrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_cgetrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zgetrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zgetrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zgetrf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_sgetrf2( int matrix_layout, int m, int n,
FloatPointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_sgetrf2( int matrix_layout, int m, int n,
FloatBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_sgetrf2( int matrix_layout, int m, int n,
float[] a, int lda, int[] ipiv );
public static native int LAPACKE_dgetrf2( int matrix_layout, int m, int n,
DoublePointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_dgetrf2( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_dgetrf2( int matrix_layout, int m, int n,
double[] a, int lda, int[] ipiv );
public static native int LAPACKE_cgetrf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_cgetrf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_cgetrf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zgetrf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zgetrf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zgetrf2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_sgetri( int matrix_layout, int n, FloatPointer a,
int lda, @Const IntPointer ipiv );
public static native int LAPACKE_sgetri( int matrix_layout, int n, FloatBuffer a,
int lda, @Const IntBuffer ipiv );
public static native int LAPACKE_sgetri( int matrix_layout, int n, float[] a,
int lda, @Const int[] ipiv );
public static native int LAPACKE_dgetri( int matrix_layout, int n, DoublePointer a,
int lda, @Const IntPointer ipiv );
public static native int LAPACKE_dgetri( int matrix_layout, int n, DoubleBuffer a,
int lda, @Const IntBuffer ipiv );
public static native int LAPACKE_dgetri( int matrix_layout, int n, double[] a,
int lda, @Const int[] ipiv );
public static native int LAPACKE_cgetri( int matrix_layout, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_cgetri( int matrix_layout, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_cgetri( int matrix_layout, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_zgetri( int matrix_layout, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_zgetri( int matrix_layout, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_zgetri( int matrix_layout, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_sgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer b, int ldb );
public static native int LAPACKE_sgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer b, int ldb );
public static native int LAPACKE_sgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const float[] a, int lda,
@Const int[] ipiv, float[] b, int ldb );
public static native int LAPACKE_dgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoublePointer a, int lda,
@Const IntPointer ipiv, DoublePointer b, int ldb );
public static native int LAPACKE_dgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoubleBuffer a, int lda,
@Const IntBuffer ipiv, DoubleBuffer b, int ldb );
public static native int LAPACKE_dgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const double[] a, int lda,
@Const int[] ipiv, double[] b, int ldb );
public static native int LAPACKE_cgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zgetrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const FloatPointer lscale,
@Const FloatPointer rscale, int m, FloatPointer v,
int ldv );
public static native int LAPACKE_sggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const FloatBuffer lscale,
@Const FloatBuffer rscale, int m, FloatBuffer v,
int ldv );
public static native int LAPACKE_sggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const float[] lscale,
@Const float[] rscale, int m, float[] v,
int ldv );
public static native int LAPACKE_dggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const DoublePointer lscale,
@Const DoublePointer rscale, int m, DoublePointer v,
int ldv );
public static native int LAPACKE_dggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const DoubleBuffer lscale,
@Const DoubleBuffer rscale, int m, DoubleBuffer v,
int ldv );
public static native int LAPACKE_dggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const double[] lscale,
@Const double[] rscale, int m, double[] v,
int ldv );
public static native int LAPACKE_cggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const FloatPointer lscale,
@Const FloatPointer rscale, int m,
@Cast("lapack_complex_float*") FloatPointer v, int ldv );
public static native int LAPACKE_cggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const FloatBuffer lscale,
@Const FloatBuffer rscale, int m,
@Cast("lapack_complex_float*") FloatBuffer v, int ldv );
public static native int LAPACKE_cggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const float[] lscale,
@Const float[] rscale, int m,
@Cast("lapack_complex_float*") float[] v, int ldv );
public static native int LAPACKE_zggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const DoublePointer lscale,
@Const DoublePointer rscale, int m,
@Cast("lapack_complex_double*") DoublePointer v, int ldv );
public static native int LAPACKE_zggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const DoubleBuffer lscale,
@Const DoubleBuffer rscale, int m,
@Cast("lapack_complex_double*") DoubleBuffer v, int ldv );
public static native int LAPACKE_zggbak( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side, int n,
int ilo, int ihi, @Const double[] lscale,
@Const double[] rscale, int m,
@Cast("lapack_complex_double*") double[] v, int ldv );
public static native int LAPACKE_sggbal( int matrix_layout, @Cast("char") byte job, int n, FloatPointer a,
int lda, FloatPointer b, int ldb,
IntPointer ilo, IntPointer ihi, FloatPointer lscale,
FloatPointer rscale );
public static native int LAPACKE_sggbal( int matrix_layout, @Cast("char") byte job, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
IntBuffer ilo, IntBuffer ihi, FloatBuffer lscale,
FloatBuffer rscale );
public static native int LAPACKE_sggbal( int matrix_layout, @Cast("char") byte job, int n, float[] a,
int lda, float[] b, int ldb,
int[] ilo, int[] ihi, float[] lscale,
float[] rscale );
public static native int LAPACKE_dggbal( int matrix_layout, @Cast("char") byte job, int n, DoublePointer a,
int lda, DoublePointer b, int ldb,
IntPointer ilo, IntPointer ihi, DoublePointer lscale,
DoublePointer rscale );
public static native int LAPACKE_dggbal( int matrix_layout, @Cast("char") byte job, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer lscale,
DoubleBuffer rscale );
public static native int LAPACKE_dggbal( int matrix_layout, @Cast("char") byte job, int n, double[] a,
int lda, double[] b, int ldb,
int[] ilo, int[] ihi, double[] lscale,
double[] rscale );
public static native int LAPACKE_cggbal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
IntPointer ilo, IntPointer ihi, FloatPointer lscale,
FloatPointer rscale );
public static native int LAPACKE_cggbal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
IntBuffer ilo, IntBuffer ihi, FloatBuffer lscale,
FloatBuffer rscale );
public static native int LAPACKE_cggbal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
int[] ilo, int[] ihi, float[] lscale,
float[] rscale );
public static native int LAPACKE_zggbal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
IntPointer ilo, IntPointer ihi, DoublePointer lscale,
DoublePointer rscale );
public static native int LAPACKE_zggbal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer lscale,
DoubleBuffer rscale );
public static native int LAPACKE_zggbal( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
int[] ilo, int[] ihi, double[] lscale,
double[] rscale );
public static native int LAPACKE_sgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_S_SELECT3 selctg, int n, FloatPointer a,
int lda, FloatPointer b, int ldb,
IntPointer sdim, FloatPointer alphar, FloatPointer alphai,
FloatPointer beta, FloatPointer vsl, int ldvsl, FloatPointer vsr,
int ldvsr );
public static native int LAPACKE_sgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_S_SELECT3 selctg, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
IntBuffer sdim, FloatBuffer alphar, FloatBuffer alphai,
FloatBuffer beta, FloatBuffer vsl, int ldvsl, FloatBuffer vsr,
int ldvsr );
public static native int LAPACKE_sgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_S_SELECT3 selctg, int n, float[] a,
int lda, float[] b, int ldb,
int[] sdim, float[] alphar, float[] alphai,
float[] beta, float[] vsl, int ldvsl, float[] vsr,
int ldvsr );
public static native int LAPACKE_dgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_D_SELECT3 selctg, int n, DoublePointer a,
int lda, DoublePointer b, int ldb,
IntPointer sdim, DoublePointer alphar, DoublePointer alphai,
DoublePointer beta, DoublePointer vsl, int ldvsl,
DoublePointer vsr, int ldvsr );
public static native int LAPACKE_dgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_D_SELECT3 selctg, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
IntBuffer sdim, DoubleBuffer alphar, DoubleBuffer alphai,
DoubleBuffer beta, DoubleBuffer vsl, int ldvsl,
DoubleBuffer vsr, int ldvsr );
public static native int LAPACKE_dgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_D_SELECT3 selctg, int n, double[] a,
int lda, double[] b, int ldb,
int[] sdim, double[] alphar, double[] alphai,
double[] beta, double[] vsl, int ldvsl,
double[] vsr, int ldvsr );
public static native int LAPACKE_cgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_C_SELECT2 selctg, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
IntPointer sdim, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer vsl,
int ldvsl, @Cast("lapack_complex_float*") FloatPointer vsr,
int ldvsr );
public static native int LAPACKE_cgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_C_SELECT2 selctg, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
IntBuffer sdim, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer vsl,
int ldvsl, @Cast("lapack_complex_float*") FloatBuffer vsr,
int ldvsr );
public static native int LAPACKE_cgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_C_SELECT2 selctg, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
int[] sdim, @Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta, @Cast("lapack_complex_float*") float[] vsl,
int ldvsl, @Cast("lapack_complex_float*") float[] vsr,
int ldvsr );
public static native int LAPACKE_zgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_Z_SELECT2 selctg, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
IntPointer sdim, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoublePointer vsr, int ldvsr );
public static native int LAPACKE_zgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_Z_SELECT2 selctg, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
IntBuffer sdim, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoubleBuffer vsr, int ldvsr );
public static native int LAPACKE_zgges( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr, @Cast("char") byte sort,
LAPACK_Z_SELECT2 selctg, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
int[] sdim, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vsl, int ldvsl,
@Cast("lapack_complex_double*") double[] vsr, int ldvsr );
public static native int LAPACKE_sgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, int n,
FloatPointer a, int lda, FloatPointer b, int ldb,
IntPointer sdim, FloatPointer alphar, FloatPointer alphai,
FloatPointer beta, FloatPointer vsl, int ldvsl,
FloatPointer vsr, int ldvsr );
public static native int LAPACKE_sgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, int n,
FloatBuffer a, int lda, FloatBuffer b, int ldb,
IntBuffer sdim, FloatBuffer alphar, FloatBuffer alphai,
FloatBuffer beta, FloatBuffer vsl, int ldvsl,
FloatBuffer vsr, int ldvsr );
public static native int LAPACKE_sgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, int n,
float[] a, int lda, float[] b, int ldb,
int[] sdim, float[] alphar, float[] alphai,
float[] beta, float[] vsl, int ldvsl,
float[] vsr, int ldvsr );
public static native int LAPACKE_dgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, int n,
DoublePointer a, int lda, DoublePointer b, int ldb,
IntPointer sdim, DoublePointer alphar, DoublePointer alphai,
DoublePointer beta, DoublePointer vsl, int ldvsl,
DoublePointer vsr, int ldvsr );
public static native int LAPACKE_dgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, int n,
DoubleBuffer a, int lda, DoubleBuffer b, int ldb,
IntBuffer sdim, DoubleBuffer alphar, DoubleBuffer alphai,
DoubleBuffer beta, DoubleBuffer vsl, int ldvsl,
DoubleBuffer vsr, int ldvsr );
public static native int LAPACKE_dgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, int n,
double[] a, int lda, double[] b, int ldb,
int[] sdim, double[] alphar, double[] alphai,
double[] beta, double[] vsl, int ldvsl,
double[] vsr, int ldvsr );
public static native int LAPACKE_cgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
IntPointer sdim, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatPointer vsr, int ldvsr );
public static native int LAPACKE_cgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
IntBuffer sdim, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatBuffer vsr, int ldvsr );
public static native int LAPACKE_cgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
int[] sdim, @Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vsl, int ldvsl,
@Cast("lapack_complex_float*") float[] vsr, int ldvsr );
public static native int LAPACKE_zgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
IntPointer sdim, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoublePointer vsr, int ldvsr );
public static native int LAPACKE_zgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
IntBuffer sdim, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoubleBuffer vsr, int ldvsr );
public static native int LAPACKE_zgges3( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
int[] sdim, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vsl, int ldvsl,
@Cast("lapack_complex_double*") double[] vsr, int ldvsr );
public static native int LAPACKE_sggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, @Cast("char") byte sense,
int n, FloatPointer a, int lda, FloatPointer b,
int ldb, IntPointer sdim, FloatPointer alphar,
FloatPointer alphai, FloatPointer beta, FloatPointer vsl,
int ldvsl, FloatPointer vsr, int ldvsr,
FloatPointer rconde, FloatPointer rcondv );
public static native int LAPACKE_sggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, @Cast("char") byte sense,
int n, FloatBuffer a, int lda, FloatBuffer b,
int ldb, IntBuffer sdim, FloatBuffer alphar,
FloatBuffer alphai, FloatBuffer beta, FloatBuffer vsl,
int ldvsl, FloatBuffer vsr, int ldvsr,
FloatBuffer rconde, FloatBuffer rcondv );
public static native int LAPACKE_sggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, @Cast("char") byte sense,
int n, float[] a, int lda, float[] b,
int ldb, int[] sdim, float[] alphar,
float[] alphai, float[] beta, float[] vsl,
int ldvsl, float[] vsr, int ldvsr,
float[] rconde, float[] rcondv );
public static native int LAPACKE_dggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, @Cast("char") byte sense,
int n, DoublePointer a, int lda, DoublePointer b,
int ldb, IntPointer sdim, DoublePointer alphar,
DoublePointer alphai, DoublePointer beta, DoublePointer vsl,
int ldvsl, DoublePointer vsr, int ldvsr,
DoublePointer rconde, DoublePointer rcondv );
public static native int LAPACKE_dggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, @Cast("char") byte sense,
int n, DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, IntBuffer sdim, DoubleBuffer alphar,
DoubleBuffer alphai, DoubleBuffer beta, DoubleBuffer vsl,
int ldvsl, DoubleBuffer vsr, int ldvsr,
DoubleBuffer rconde, DoubleBuffer rcondv );
public static native int LAPACKE_dggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, @Cast("char") byte sense,
int n, double[] a, int lda, double[] b,
int ldb, int[] sdim, double[] alphar,
double[] alphai, double[] beta, double[] vsl,
int ldvsl, double[] vsr, int ldvsr,
double[] rconde, double[] rcondv );
public static native int LAPACKE_cggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, IntPointer sdim,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatPointer vsr, int ldvsr,
FloatPointer rconde, FloatPointer rcondv );
public static native int LAPACKE_cggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, IntBuffer sdim,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatBuffer vsr, int ldvsr,
FloatBuffer rconde, FloatBuffer rcondv );
public static native int LAPACKE_cggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, int[] sdim,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vsl, int ldvsl,
@Cast("lapack_complex_float*") float[] vsr, int ldvsr,
float[] rconde, float[] rcondv );
public static native int LAPACKE_zggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, IntPointer sdim,
@Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoublePointer vsr, int ldvsr,
DoublePointer rconde, DoublePointer rcondv );
public static native int LAPACKE_zggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, IntBuffer sdim,
@Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoubleBuffer vsr, int ldvsr,
DoubleBuffer rconde, DoubleBuffer rcondv );
public static native int LAPACKE_zggesx( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, int[] sdim,
@Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vsl, int ldvsl,
@Cast("lapack_complex_double*") double[] vsr, int ldvsr,
double[] rconde, double[] rcondv );
public static native int LAPACKE_sggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer alphar, FloatPointer alphai,
FloatPointer beta, FloatPointer vl, int ldvl, FloatPointer vr,
int ldvr );
public static native int LAPACKE_sggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer alphar, FloatBuffer alphai,
FloatBuffer beta, FloatBuffer vl, int ldvl, FloatBuffer vr,
int ldvr );
public static native int LAPACKE_sggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, float[] a, int lda, float[] b,
int ldb, float[] alphar, float[] alphai,
float[] beta, float[] vl, int ldvl, float[] vr,
int ldvr );
public static native int LAPACKE_dggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoublePointer a, int lda, DoublePointer b,
int ldb, DoublePointer alphar, DoublePointer alphai,
DoublePointer beta, DoublePointer vl, int ldvl, DoublePointer vr,
int ldvr );
public static native int LAPACKE_dggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, DoubleBuffer alphar, DoubleBuffer alphai,
DoubleBuffer beta, DoubleBuffer vl, int ldvl, DoubleBuffer vr,
int ldvr );
public static native int LAPACKE_dggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, double[] a, int lda, double[] b,
int ldb, double[] alphar, double[] alphai,
double[] beta, double[] vl, int ldvl, double[] vr,
int ldvr );
public static native int LAPACKE_cggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer vl,
int ldvl, @Cast("lapack_complex_float*") FloatPointer vr,
int ldvr );
public static native int LAPACKE_cggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer vl,
int ldvl, @Cast("lapack_complex_float*") FloatBuffer vr,
int ldvr );
public static native int LAPACKE_cggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta, @Cast("lapack_complex_float*") float[] vl,
int ldvl, @Cast("lapack_complex_float*") float[] vr,
int ldvr );
public static native int LAPACKE_zggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr );
public static native int LAPACKE_zggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr );
public static native int LAPACKE_zggev( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr );
public static native int LAPACKE_sggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatPointer a, int lda,
FloatPointer b, int ldb,
FloatPointer alphar, FloatPointer alphai, FloatPointer beta,
FloatPointer vl, int ldvl,
FloatPointer vr, int ldvr );
public static native int LAPACKE_sggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatBuffer a, int lda,
FloatBuffer b, int ldb,
FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta,
FloatBuffer vl, int ldvl,
FloatBuffer vr, int ldvr );
public static native int LAPACKE_sggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, float[] a, int lda,
float[] b, int ldb,
float[] alphar, float[] alphai, float[] beta,
float[] vl, int ldvl,
float[] vr, int ldvr );
public static native int LAPACKE_dggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoublePointer a, int lda,
DoublePointer b, int ldb,
DoublePointer alphar, DoublePointer alphai, DoublePointer beta,
DoublePointer vl, int ldvl,
DoublePointer vr, int ldvr );
public static native int LAPACKE_dggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb,
DoubleBuffer alphar, DoubleBuffer alphai, DoubleBuffer beta,
DoubleBuffer vl, int ldvl,
DoubleBuffer vr, int ldvr );
public static native int LAPACKE_dggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, double[] a, int lda,
double[] b, int ldb,
double[] alphar, double[] alphai, double[] beta,
double[] vl, int ldvl,
double[] vr, int ldvr );
public static native int LAPACKE_cggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr );
public static native int LAPACKE_cggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr );
public static native int LAPACKE_cggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr );
public static native int LAPACKE_zggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr );
public static native int LAPACKE_zggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr );
public static native int LAPACKE_zggev3( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr );
public static native int LAPACKE_sggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, FloatPointer a,
int lda, FloatPointer b, int ldb,
FloatPointer alphar, FloatPointer alphai, FloatPointer beta, FloatPointer vl,
int ldvl, FloatPointer vr, int ldvr,
IntPointer ilo, IntPointer ihi, FloatPointer lscale,
FloatPointer rscale, FloatPointer abnrm, FloatPointer bbnrm,
FloatPointer rconde, FloatPointer rcondv );
public static native int LAPACKE_sggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta, FloatBuffer vl,
int ldvl, FloatBuffer vr, int ldvr,
IntBuffer ilo, IntBuffer ihi, FloatBuffer lscale,
FloatBuffer rscale, FloatBuffer abnrm, FloatBuffer bbnrm,
FloatBuffer rconde, FloatBuffer rcondv );
public static native int LAPACKE_sggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, float[] a,
int lda, float[] b, int ldb,
float[] alphar, float[] alphai, float[] beta, float[] vl,
int ldvl, float[] vr, int ldvr,
int[] ilo, int[] ihi, float[] lscale,
float[] rscale, float[] abnrm, float[] bbnrm,
float[] rconde, float[] rcondv );
public static native int LAPACKE_dggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, DoublePointer a,
int lda, DoublePointer b, int ldb,
DoublePointer alphar, DoublePointer alphai, DoublePointer beta,
DoublePointer vl, int ldvl, DoublePointer vr,
int ldvr, IntPointer ilo, IntPointer ihi,
DoublePointer lscale, DoublePointer rscale, DoublePointer abnrm,
DoublePointer bbnrm, DoublePointer rconde, DoublePointer rcondv );
public static native int LAPACKE_dggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
DoubleBuffer alphar, DoubleBuffer alphai, DoubleBuffer beta,
DoubleBuffer vl, int ldvl, DoubleBuffer vr,
int ldvr, IntBuffer ilo, IntBuffer ihi,
DoubleBuffer lscale, DoubleBuffer rscale, DoubleBuffer abnrm,
DoubleBuffer bbnrm, DoubleBuffer rconde, DoubleBuffer rcondv );
public static native int LAPACKE_dggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, double[] a,
int lda, double[] b, int ldb,
double[] alphar, double[] alphai, double[] beta,
double[] vl, int ldvl, double[] vr,
int ldvr, int[] ilo, int[] ihi,
double[] lscale, double[] rscale, double[] abnrm,
double[] bbnrm, double[] rconde, double[] rcondv );
public static native int LAPACKE_cggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer vl,
int ldvl, @Cast("lapack_complex_float*") FloatPointer vr,
int ldvr, IntPointer ilo, IntPointer ihi,
FloatPointer lscale, FloatPointer rscale, FloatPointer abnrm,
FloatPointer bbnrm, FloatPointer rconde, FloatPointer rcondv );
public static native int LAPACKE_cggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer vl,
int ldvl, @Cast("lapack_complex_float*") FloatBuffer vr,
int ldvr, IntBuffer ilo, IntBuffer ihi,
FloatBuffer lscale, FloatBuffer rscale, FloatBuffer abnrm,
FloatBuffer bbnrm, FloatBuffer rconde, FloatBuffer rcondv );
public static native int LAPACKE_cggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta, @Cast("lapack_complex_float*") float[] vl,
int ldvl, @Cast("lapack_complex_float*") float[] vr,
int ldvr, int[] ilo, int[] ihi,
float[] lscale, float[] rscale, float[] abnrm,
float[] bbnrm, float[] rconde, float[] rcondv );
public static native int LAPACKE_zggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
IntPointer ilo, IntPointer ihi, DoublePointer lscale,
DoublePointer rscale, DoublePointer abnrm, DoublePointer bbnrm,
DoublePointer rconde, DoublePointer rcondv );
public static native int LAPACKE_zggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer lscale,
DoubleBuffer rscale, DoubleBuffer abnrm, DoubleBuffer bbnrm,
DoubleBuffer rconde, DoubleBuffer rcondv );
public static native int LAPACKE_zggevx( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
int[] ilo, int[] ihi, double[] lscale,
double[] rscale, double[] abnrm, double[] bbnrm,
double[] rconde, double[] rcondv );
public static native int LAPACKE_sggglm( int matrix_layout, int n, int m,
int p, FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer d, FloatPointer x, FloatPointer y );
public static native int LAPACKE_sggglm( int matrix_layout, int n, int m,
int p, FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer d, FloatBuffer x, FloatBuffer y );
public static native int LAPACKE_sggglm( int matrix_layout, int n, int m,
int p, float[] a, int lda, float[] b,
int ldb, float[] d, float[] x, float[] y );
public static native int LAPACKE_dggglm( int matrix_layout, int n, int m,
int p, DoublePointer a, int lda, DoublePointer b,
int ldb, DoublePointer d, DoublePointer x, DoublePointer y );
public static native int LAPACKE_dggglm( int matrix_layout, int n, int m,
int p, DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, DoubleBuffer d, DoubleBuffer x, DoubleBuffer y );
public static native int LAPACKE_dggglm( int matrix_layout, int n, int m,
int p, double[] a, int lda, double[] b,
int ldb, double[] d, double[] x, double[] y );
public static native int LAPACKE_cggglm( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer x, @Cast("lapack_complex_float*") FloatPointer y );
public static native int LAPACKE_cggglm( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer x, @Cast("lapack_complex_float*") FloatBuffer y );
public static native int LAPACKE_cggglm( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] d,
@Cast("lapack_complex_float*") float[] x, @Cast("lapack_complex_float*") float[] y );
public static native int LAPACKE_zggglm( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer x, @Cast("lapack_complex_double*") DoublePointer y );
public static native int LAPACKE_zggglm( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer x, @Cast("lapack_complex_double*") DoubleBuffer y );
public static native int LAPACKE_zggglm( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] d,
@Cast("lapack_complex_double*") double[] x, @Cast("lapack_complex_double*") double[] y );
public static native int LAPACKE_sgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatPointer a, int lda, FloatPointer b, int ldb,
FloatPointer q, int ldq, FloatPointer z, int ldz );
public static native int LAPACKE_sgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatBuffer a, int lda, FloatBuffer b, int ldb,
FloatBuffer q, int ldq, FloatBuffer z, int ldz );
public static native int LAPACKE_sgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
float[] a, int lda, float[] b, int ldb,
float[] q, int ldq, float[] z, int ldz );
public static native int LAPACKE_dgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoublePointer a, int lda, DoublePointer b, int ldb,
DoublePointer q, int ldq, DoublePointer z,
int ldz );
public static native int LAPACKE_dgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoubleBuffer a, int lda, DoubleBuffer b, int ldb,
DoubleBuffer q, int ldq, DoubleBuffer z,
int ldz );
public static native int LAPACKE_dgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
double[] a, int lda, double[] b, int ldb,
double[] q, int ldq, double[] z,
int ldz );
public static native int LAPACKE_cgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_cgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_cgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz );
public static native int LAPACKE_zgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz );
public static native int LAPACKE_zgghrd( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz );
public static native int LAPACKE_sgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatPointer a, int lda, FloatPointer b, int ldb,
FloatPointer q, int ldq, FloatPointer z, int ldz );
public static native int LAPACKE_sgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatBuffer a, int lda, FloatBuffer b, int ldb,
FloatBuffer q, int ldq, FloatBuffer z, int ldz );
public static native int LAPACKE_sgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
float[] a, int lda, float[] b, int ldb,
float[] q, int ldq, float[] z, int ldz );
public static native int LAPACKE_dgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoublePointer a, int lda, DoublePointer b, int ldb,
DoublePointer q, int ldq, DoublePointer z,
int ldz );
public static native int LAPACKE_dgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoubleBuffer a, int lda, DoubleBuffer b, int ldb,
DoubleBuffer q, int ldq, DoubleBuffer z,
int ldz );
public static native int LAPACKE_dgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
double[] a, int lda, double[] b, int ldb,
double[] q, int ldq, double[] z,
int ldz );
public static native int LAPACKE_cgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_cgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_cgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz );
public static native int LAPACKE_zgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz );
public static native int LAPACKE_zgghd3( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz );
public static native int LAPACKE_sgglse( int matrix_layout, int m, int n,
int p, FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer c, FloatPointer d, FloatPointer x );
public static native int LAPACKE_sgglse( int matrix_layout, int m, int n,
int p, FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer c, FloatBuffer d, FloatBuffer x );
public static native int LAPACKE_sgglse( int matrix_layout, int m, int n,
int p, float[] a, int lda, float[] b,
int ldb, float[] c, float[] d, float[] x );
public static native int LAPACKE_dgglse( int matrix_layout, int m, int n,
int p, DoublePointer a, int lda, DoublePointer b,
int ldb, DoublePointer c, DoublePointer d, DoublePointer x );
public static native int LAPACKE_dgglse( int matrix_layout, int m, int n,
int p, DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, DoubleBuffer c, DoubleBuffer d, DoubleBuffer x );
public static native int LAPACKE_dgglse( int matrix_layout, int m, int n,
int p, double[] a, int lda, double[] b,
int ldb, double[] c, double[] d, double[] x );
public static native int LAPACKE_cgglse( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer c,
@Cast("lapack_complex_float*") FloatPointer d, @Cast("lapack_complex_float*") FloatPointer x );
public static native int LAPACKE_cgglse( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer c,
@Cast("lapack_complex_float*") FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer x );
public static native int LAPACKE_cgglse( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] c,
@Cast("lapack_complex_float*") float[] d, @Cast("lapack_complex_float*") float[] x );
public static native int LAPACKE_zgglse( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer c,
@Cast("lapack_complex_double*") DoublePointer d, @Cast("lapack_complex_double*") DoublePointer x );
public static native int LAPACKE_zgglse( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer c,
@Cast("lapack_complex_double*") DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer x );
public static native int LAPACKE_zgglse( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] c,
@Cast("lapack_complex_double*") double[] d, @Cast("lapack_complex_double*") double[] x );
public static native int LAPACKE_sggqrf( int matrix_layout, int n, int m,
int p, FloatPointer a, int lda, FloatPointer taua,
FloatPointer b, int ldb, FloatPointer taub );
public static native int LAPACKE_sggqrf( int matrix_layout, int n, int m,
int p, FloatBuffer a, int lda, FloatBuffer taua,
FloatBuffer b, int ldb, FloatBuffer taub );
public static native int LAPACKE_sggqrf( int matrix_layout, int n, int m,
int p, float[] a, int lda, float[] taua,
float[] b, int ldb, float[] taub );
public static native int LAPACKE_dggqrf( int matrix_layout, int n, int m,
int p, DoublePointer a, int lda,
DoublePointer taua, DoublePointer b, int ldb,
DoublePointer taub );
public static native int LAPACKE_dggqrf( int matrix_layout, int n, int m,
int p, DoubleBuffer a, int lda,
DoubleBuffer taua, DoubleBuffer b, int ldb,
DoubleBuffer taub );
public static native int LAPACKE_dggqrf( int matrix_layout, int n, int m,
int p, double[] a, int lda,
double[] taua, double[] b, int ldb,
double[] taub );
public static native int LAPACKE_cggqrf( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer taua,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer taub );
public static native int LAPACKE_cggqrf( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer taua,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer taub );
public static native int LAPACKE_cggqrf( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] taua,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] taub );
public static native int LAPACKE_zggqrf( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer taua,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer taub );
public static native int LAPACKE_zggqrf( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer taua,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer taub );
public static native int LAPACKE_zggqrf( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] taua,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] taub );
public static native int LAPACKE_sggrqf( int matrix_layout, int m, int p,
int n, FloatPointer a, int lda, FloatPointer taua,
FloatPointer b, int ldb, FloatPointer taub );
public static native int LAPACKE_sggrqf( int matrix_layout, int m, int p,
int n, FloatBuffer a, int lda, FloatBuffer taua,
FloatBuffer b, int ldb, FloatBuffer taub );
public static native int LAPACKE_sggrqf( int matrix_layout, int m, int p,
int n, float[] a, int lda, float[] taua,
float[] b, int ldb, float[] taub );
public static native int LAPACKE_dggrqf( int matrix_layout, int m, int p,
int n, DoublePointer a, int lda,
DoublePointer taua, DoublePointer b, int ldb,
DoublePointer taub );
public static native int LAPACKE_dggrqf( int matrix_layout, int m, int p,
int n, DoubleBuffer a, int lda,
DoubleBuffer taua, DoubleBuffer b, int ldb,
DoubleBuffer taub );
public static native int LAPACKE_dggrqf( int matrix_layout, int m, int p,
int n, double[] a, int lda,
double[] taua, double[] b, int ldb,
double[] taub );
public static native int LAPACKE_cggrqf( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer taua,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer taub );
public static native int LAPACKE_cggrqf( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer taua,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer taub );
public static native int LAPACKE_cggrqf( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] taua,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] taub );
public static native int LAPACKE_zggrqf( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer taua,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer taub );
public static native int LAPACKE_zggrqf( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer taua,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer taub );
public static native int LAPACKE_zggrqf( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] taua,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] taub );
public static native int LAPACKE_sggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
IntPointer k, IntPointer l, FloatPointer a,
int lda, FloatPointer b, int ldb,
FloatPointer alpha, FloatPointer beta, FloatPointer u, int ldu,
FloatPointer v, int ldv, FloatPointer q, int ldq,
IntPointer iwork );
public static native int LAPACKE_sggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
IntBuffer k, IntBuffer l, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
FloatBuffer alpha, FloatBuffer beta, FloatBuffer u, int ldu,
FloatBuffer v, int ldv, FloatBuffer q, int ldq,
IntBuffer iwork );
public static native int LAPACKE_sggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
int[] k, int[] l, float[] a,
int lda, float[] b, int ldb,
float[] alpha, float[] beta, float[] u, int ldu,
float[] v, int ldv, float[] q, int ldq,
int[] iwork );
public static native int LAPACKE_dggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
IntPointer k, IntPointer l, DoublePointer a,
int lda, DoublePointer b, int ldb,
DoublePointer alpha, DoublePointer beta, DoublePointer u,
int ldu, DoublePointer v, int ldv, DoublePointer q,
int ldq, IntPointer iwork );
public static native int LAPACKE_dggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
IntBuffer k, IntBuffer l, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
DoubleBuffer alpha, DoubleBuffer beta, DoubleBuffer u,
int ldu, DoubleBuffer v, int ldv, DoubleBuffer q,
int ldq, IntBuffer iwork );
public static native int LAPACKE_dggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
int[] k, int[] l, double[] a,
int lda, double[] b, int ldb,
double[] alpha, double[] beta, double[] u,
int ldu, double[] v, int ldv, double[] q,
int ldq, int[] iwork );
public static native int LAPACKE_cggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
IntPointer k, IntPointer l,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
FloatPointer alpha, FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer u,
int ldu, @Cast("lapack_complex_float*") FloatPointer v,
int ldv, @Cast("lapack_complex_float*") FloatPointer q,
int ldq, IntPointer iwork );
public static native int LAPACKE_cggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
IntBuffer k, IntBuffer l,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
FloatBuffer alpha, FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer u,
int ldu, @Cast("lapack_complex_float*") FloatBuffer v,
int ldv, @Cast("lapack_complex_float*") FloatBuffer q,
int ldq, IntBuffer iwork );
public static native int LAPACKE_cggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
int[] k, int[] l,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
float[] alpha, float[] beta, @Cast("lapack_complex_float*") float[] u,
int ldu, @Cast("lapack_complex_float*") float[] v,
int ldv, @Cast("lapack_complex_float*") float[] q,
int ldq, int[] iwork );
public static native int LAPACKE_zggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
IntPointer k, IntPointer l,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
DoublePointer alpha, DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer u, int ldu,
@Cast("lapack_complex_double*") DoublePointer v, int ldv,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
IntPointer iwork );
public static native int LAPACKE_zggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
IntBuffer k, IntBuffer l,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
DoubleBuffer alpha, DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer u, int ldu,
@Cast("lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
IntBuffer iwork );
public static native int LAPACKE_zggsvd3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int n, int p,
int[] k, int[] l,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
double[] alpha, double[] beta,
@Cast("lapack_complex_double*") double[] u, int ldu,
@Cast("lapack_complex_double*") double[] v, int ldv,
@Cast("lapack_complex_double*") double[] q, int ldq,
int[] iwork );
public static native int LAPACKE_sggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n, FloatPointer a,
int lda, FloatPointer b, int ldb, float tola,
float tolb, IntPointer k, IntPointer l, FloatPointer u,
int ldu, FloatPointer v, int ldv, FloatPointer q,
int ldq );
public static native int LAPACKE_sggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb, float tola,
float tolb, IntBuffer k, IntBuffer l, FloatBuffer u,
int ldu, FloatBuffer v, int ldv, FloatBuffer q,
int ldq );
public static native int LAPACKE_sggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n, float[] a,
int lda, float[] b, int ldb, float tola,
float tolb, int[] k, int[] l, float[] u,
int ldu, float[] v, int ldv, float[] q,
int ldq );
public static native int LAPACKE_dggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n, DoublePointer a,
int lda, DoublePointer b, int ldb,
double tola, double tolb, IntPointer k,
IntPointer l, DoublePointer u, int ldu, DoublePointer v,
int ldv, DoublePointer q, int ldq );
public static native int LAPACKE_dggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
double tola, double tolb, IntBuffer k,
IntBuffer l, DoubleBuffer u, int ldu, DoubleBuffer v,
int ldv, DoubleBuffer q, int ldq );
public static native int LAPACKE_dggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n, double[] a,
int lda, double[] b, int ldb,
double tola, double tolb, int[] k,
int[] l, double[] u, int ldu, double[] v,
int ldv, double[] q, int ldq );
public static native int LAPACKE_cggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb, float tola,
float tolb, IntPointer k, IntPointer l,
@Cast("lapack_complex_float*") FloatPointer u, int ldu,
@Cast("lapack_complex_float*") FloatPointer v, int ldv,
@Cast("lapack_complex_float*") FloatPointer q, int ldq );
public static native int LAPACKE_cggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb, float tola,
float tolb, IntBuffer k, IntBuffer l,
@Cast("lapack_complex_float*") FloatBuffer u, int ldu,
@Cast("lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq );
public static native int LAPACKE_cggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb, float tola,
float tolb, int[] k, int[] l,
@Cast("lapack_complex_float*") float[] u, int ldu,
@Cast("lapack_complex_float*") float[] v, int ldv,
@Cast("lapack_complex_float*") float[] q, int ldq );
public static native int LAPACKE_zggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
double tola, double tolb, IntPointer k,
IntPointer l, @Cast("lapack_complex_double*") DoublePointer u,
int ldu, @Cast("lapack_complex_double*") DoublePointer v,
int ldv, @Cast("lapack_complex_double*") DoublePointer q,
int ldq );
public static native int LAPACKE_zggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
double tola, double tolb, IntBuffer k,
IntBuffer l, @Cast("lapack_complex_double*") DoubleBuffer u,
int ldu, @Cast("lapack_complex_double*") DoubleBuffer v,
int ldv, @Cast("lapack_complex_double*") DoubleBuffer q,
int ldq );
public static native int LAPACKE_zggsvp3( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
double tola, double tolb, int[] k,
int[] l, @Cast("lapack_complex_double*") double[] u,
int ldu, @Cast("lapack_complex_double*") double[] v,
int ldv, @Cast("lapack_complex_double*") double[] q,
int ldq );
public static native int LAPACKE_sgtcon( @Cast("char") byte norm, int n, @Const FloatPointer dl,
@Const FloatPointer d, @Const FloatPointer du, @Const FloatPointer du2,
@Const IntPointer ipiv, float anorm, FloatPointer rcond );
public static native int LAPACKE_sgtcon( @Cast("char") byte norm, int n, @Const FloatBuffer dl,
@Const FloatBuffer d, @Const FloatBuffer du, @Const FloatBuffer du2,
@Const IntBuffer ipiv, float anorm, FloatBuffer rcond );
public static native int LAPACKE_sgtcon( @Cast("char") byte norm, int n, @Const float[] dl,
@Const float[] d, @Const float[] du, @Const float[] du2,
@Const int[] ipiv, float anorm, float[] rcond );
public static native int LAPACKE_dgtcon( @Cast("char") byte norm, int n, @Const DoublePointer dl,
@Const DoublePointer d, @Const DoublePointer du, @Const DoublePointer du2,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond );
public static native int LAPACKE_dgtcon( @Cast("char") byte norm, int n, @Const DoubleBuffer dl,
@Const DoubleBuffer d, @Const DoubleBuffer du, @Const DoubleBuffer du2,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_dgtcon( @Cast("char") byte norm, int n, @Const double[] dl,
@Const double[] d, @Const double[] du, @Const double[] du2,
@Const int[] ipiv, double anorm,
double[] rcond );
public static native int LAPACKE_cgtcon( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("const lapack_complex_float*") FloatPointer du2,
@Const IntPointer ipiv, float anorm, FloatPointer rcond );
public static native int LAPACKE_cgtcon( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("const lapack_complex_float*") FloatBuffer du2,
@Const IntBuffer ipiv, float anorm, FloatBuffer rcond );
public static native int LAPACKE_cgtcon( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("const lapack_complex_float*") float[] du2,
@Const int[] ipiv, float anorm, float[] rcond );
public static native int LAPACKE_zgtcon( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("const lapack_complex_double*") DoublePointer du2,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond );
public static native int LAPACKE_zgtcon( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("const lapack_complex_double*") DoubleBuffer du2,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_zgtcon( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("const lapack_complex_double*") double[] du2,
@Const int[] ipiv, double anorm,
double[] rcond );
public static native int LAPACKE_sgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatPointer dl, @Const FloatPointer d,
@Const FloatPointer du, @Const FloatPointer dlf, @Const FloatPointer df,
@Const FloatPointer duf, @Const FloatPointer du2,
@Const IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_sgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatBuffer dl, @Const FloatBuffer d,
@Const FloatBuffer du, @Const FloatBuffer dlf, @Const FloatBuffer df,
@Const FloatBuffer duf, @Const FloatBuffer du2,
@Const IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_sgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const float[] dl, @Const float[] d,
@Const float[] du, @Const float[] dlf, @Const float[] df,
@Const float[] duf, @Const float[] du2,
@Const int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr );
public static native int LAPACKE_dgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoublePointer dl, @Const DoublePointer d,
@Const DoublePointer du, @Const DoublePointer dlf,
@Const DoublePointer df, @Const DoublePointer duf,
@Const DoublePointer du2, @Const IntPointer ipiv,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoubleBuffer dl, @Const DoubleBuffer d,
@Const DoubleBuffer du, @Const DoubleBuffer dlf,
@Const DoubleBuffer df, @Const DoubleBuffer duf,
@Const DoubleBuffer du2, @Const IntBuffer ipiv,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const double[] dl, @Const double[] d,
@Const double[] du, @Const double[] dlf,
@Const double[] df, @Const double[] duf,
@Const double[] du2, @Const int[] ipiv,
@Const double[] b, int ldb, double[] x,
int ldx, double[] ferr, double[] berr );
public static native int LAPACKE_cgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("const lapack_complex_float*") FloatPointer dlf,
@Cast("const lapack_complex_float*") FloatPointer df,
@Cast("const lapack_complex_float*") FloatPointer duf,
@Cast("const lapack_complex_float*") FloatPointer du2,
@Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_cgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("const lapack_complex_float*") FloatBuffer dlf,
@Cast("const lapack_complex_float*") FloatBuffer df,
@Cast("const lapack_complex_float*") FloatBuffer duf,
@Cast("const lapack_complex_float*") FloatBuffer du2,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_cgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("const lapack_complex_float*") float[] dlf,
@Cast("const lapack_complex_float*") float[] df,
@Cast("const lapack_complex_float*") float[] duf,
@Cast("const lapack_complex_float*") float[] du2,
@Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("const lapack_complex_double*") DoublePointer dlf,
@Cast("const lapack_complex_double*") DoublePointer df,
@Cast("const lapack_complex_double*") DoublePointer duf,
@Cast("const lapack_complex_double*") DoublePointer du2,
@Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("const lapack_complex_double*") DoubleBuffer dlf,
@Cast("const lapack_complex_double*") DoubleBuffer df,
@Cast("const lapack_complex_double*") DoubleBuffer duf,
@Cast("const lapack_complex_double*") DoubleBuffer du2,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zgtrfs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("const lapack_complex_double*") double[] dlf,
@Cast("const lapack_complex_double*") double[] df,
@Cast("const lapack_complex_double*") double[] duf,
@Cast("const lapack_complex_double*") double[] du2,
@Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_sgtsv( int matrix_layout, int n, int nrhs,
FloatPointer dl, FloatPointer d, FloatPointer du, FloatPointer b,
int ldb );
public static native int LAPACKE_sgtsv( int matrix_layout, int n, int nrhs,
FloatBuffer dl, FloatBuffer d, FloatBuffer du, FloatBuffer b,
int ldb );
public static native int LAPACKE_sgtsv( int matrix_layout, int n, int nrhs,
float[] dl, float[] d, float[] du, float[] b,
int ldb );
public static native int LAPACKE_dgtsv( int matrix_layout, int n, int nrhs,
DoublePointer dl, DoublePointer d, DoublePointer du, DoublePointer b,
int ldb );
public static native int LAPACKE_dgtsv( int matrix_layout, int n, int nrhs,
DoubleBuffer dl, DoubleBuffer d, DoubleBuffer du, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dgtsv( int matrix_layout, int n, int nrhs,
double[] dl, double[] d, double[] du, double[] b,
int ldb );
public static native int LAPACKE_cgtsv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer dl, @Cast("lapack_complex_float*") FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer du, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cgtsv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer dl, @Cast("lapack_complex_float*") FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer du, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cgtsv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") float[] dl, @Cast("lapack_complex_float*") float[] d,
@Cast("lapack_complex_float*") float[] du, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zgtsv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer dl, @Cast("lapack_complex_double*") DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer du, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zgtsv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer dl, @Cast("lapack_complex_double*") DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer du, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zgtsv( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") double[] dl, @Cast("lapack_complex_double*") double[] d,
@Cast("lapack_complex_double*") double[] du, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_sgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const FloatPointer dl,
@Const FloatPointer d, @Const FloatPointer du, FloatPointer dlf,
FloatPointer df, FloatPointer duf, FloatPointer du2, IntPointer ipiv,
@Const FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_sgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const FloatBuffer dl,
@Const FloatBuffer d, @Const FloatBuffer du, FloatBuffer dlf,
FloatBuffer df, FloatBuffer duf, FloatBuffer du2, IntBuffer ipiv,
@Const FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_sgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const float[] dl,
@Const float[] d, @Const float[] du, float[] dlf,
float[] df, float[] duf, float[] du2, int[] ipiv,
@Const float[] b, int ldb, float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr );
public static native int LAPACKE_dgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const DoublePointer dl,
@Const DoublePointer d, @Const DoublePointer du, DoublePointer dlf,
DoublePointer df, DoublePointer duf, DoublePointer du2,
IntPointer ipiv, @Const DoublePointer b, int ldb,
DoublePointer x, int ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const DoubleBuffer dl,
@Const DoubleBuffer d, @Const DoubleBuffer du, DoubleBuffer dlf,
DoubleBuffer df, DoubleBuffer duf, DoubleBuffer du2,
IntBuffer ipiv, @Const DoubleBuffer b, int ldb,
DoubleBuffer x, int ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const double[] dl,
@Const double[] d, @Const double[] du, double[] dlf,
double[] df, double[] duf, double[] du2,
int[] ipiv, @Const double[] b, int ldb,
double[] x, int ldx, double[] rcond,
double[] ferr, double[] berr );
public static native int LAPACKE_cgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("lapack_complex_float*") FloatPointer dlf, @Cast("lapack_complex_float*") FloatPointer df,
@Cast("lapack_complex_float*") FloatPointer duf, @Cast("lapack_complex_float*") FloatPointer du2,
IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_cgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("lapack_complex_float*") FloatBuffer dlf, @Cast("lapack_complex_float*") FloatBuffer df,
@Cast("lapack_complex_float*") FloatBuffer duf, @Cast("lapack_complex_float*") FloatBuffer du2,
IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_cgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("lapack_complex_float*") float[] dlf, @Cast("lapack_complex_float*") float[] df,
@Cast("lapack_complex_float*") float[] duf, @Cast("lapack_complex_float*") float[] du2,
int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr );
public static native int LAPACKE_zgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("lapack_complex_double*") DoublePointer dlf,
@Cast("lapack_complex_double*") DoublePointer df,
@Cast("lapack_complex_double*") DoublePointer duf,
@Cast("lapack_complex_double*") DoublePointer du2, IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("lapack_complex_double*") DoubleBuffer dlf,
@Cast("lapack_complex_double*") DoubleBuffer df,
@Cast("lapack_complex_double*") DoubleBuffer duf,
@Cast("lapack_complex_double*") DoubleBuffer du2, IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zgtsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("lapack_complex_double*") double[] dlf,
@Cast("lapack_complex_double*") double[] df,
@Cast("lapack_complex_double*") double[] duf,
@Cast("lapack_complex_double*") double[] du2, int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_sgttrf( int n, FloatPointer dl, FloatPointer d, FloatPointer du,
FloatPointer du2, IntPointer ipiv );
public static native int LAPACKE_sgttrf( int n, FloatBuffer dl, FloatBuffer d, FloatBuffer du,
FloatBuffer du2, IntBuffer ipiv );
public static native int LAPACKE_sgttrf( int n, float[] dl, float[] d, float[] du,
float[] du2, int[] ipiv );
public static native int LAPACKE_dgttrf( int n, DoublePointer dl, DoublePointer d, DoublePointer du,
DoublePointer du2, IntPointer ipiv );
public static native int LAPACKE_dgttrf( int n, DoubleBuffer dl, DoubleBuffer d, DoubleBuffer du,
DoubleBuffer du2, IntBuffer ipiv );
public static native int LAPACKE_dgttrf( int n, double[] dl, double[] d, double[] du,
double[] du2, int[] ipiv );
public static native int LAPACKE_cgttrf( int n, @Cast("lapack_complex_float*") FloatPointer dl,
@Cast("lapack_complex_float*") FloatPointer d, @Cast("lapack_complex_float*") FloatPointer du,
@Cast("lapack_complex_float*") FloatPointer du2, IntPointer ipiv );
public static native int LAPACKE_cgttrf( int n, @Cast("lapack_complex_float*") FloatBuffer dl,
@Cast("lapack_complex_float*") FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer du,
@Cast("lapack_complex_float*") FloatBuffer du2, IntBuffer ipiv );
public static native int LAPACKE_cgttrf( int n, @Cast("lapack_complex_float*") float[] dl,
@Cast("lapack_complex_float*") float[] d, @Cast("lapack_complex_float*") float[] du,
@Cast("lapack_complex_float*") float[] du2, int[] ipiv );
public static native int LAPACKE_zgttrf( int n, @Cast("lapack_complex_double*") DoublePointer dl,
@Cast("lapack_complex_double*") DoublePointer d, @Cast("lapack_complex_double*") DoublePointer du,
@Cast("lapack_complex_double*") DoublePointer du2, IntPointer ipiv );
public static native int LAPACKE_zgttrf( int n, @Cast("lapack_complex_double*") DoubleBuffer dl,
@Cast("lapack_complex_double*") DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer du,
@Cast("lapack_complex_double*") DoubleBuffer du2, IntBuffer ipiv );
public static native int LAPACKE_zgttrf( int n, @Cast("lapack_complex_double*") double[] dl,
@Cast("lapack_complex_double*") double[] d, @Cast("lapack_complex_double*") double[] du,
@Cast("lapack_complex_double*") double[] du2, int[] ipiv );
public static native int LAPACKE_sgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatPointer dl, @Const FloatPointer d,
@Const FloatPointer du, @Const FloatPointer du2,
@Const IntPointer ipiv, FloatPointer b, int ldb );
public static native int LAPACKE_sgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatBuffer dl, @Const FloatBuffer d,
@Const FloatBuffer du, @Const FloatBuffer du2,
@Const IntBuffer ipiv, FloatBuffer b, int ldb );
public static native int LAPACKE_sgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const float[] dl, @Const float[] d,
@Const float[] du, @Const float[] du2,
@Const int[] ipiv, float[] b, int ldb );
public static native int LAPACKE_dgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoublePointer dl, @Const DoublePointer d,
@Const DoublePointer du, @Const DoublePointer du2,
@Const IntPointer ipiv, DoublePointer b, int ldb );
public static native int LAPACKE_dgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoubleBuffer dl, @Const DoubleBuffer d,
@Const DoubleBuffer du, @Const DoubleBuffer du2,
@Const IntBuffer ipiv, DoubleBuffer b, int ldb );
public static native int LAPACKE_dgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const double[] dl, @Const double[] d,
@Const double[] du, @Const double[] du2,
@Const int[] ipiv, double[] b, int ldb );
public static native int LAPACKE_cgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("const lapack_complex_float*") FloatPointer du2,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("const lapack_complex_float*") FloatBuffer du2,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("const lapack_complex_float*") float[] du2,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("const lapack_complex_double*") DoublePointer du2,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("const lapack_complex_double*") DoubleBuffer du2,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zgttrs( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("const lapack_complex_double*") double[] du2,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_chbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz );
public static native int LAPACKE_chbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz );
public static native int LAPACKE_chbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") float[] ab,
int ldab, float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz );
public static native int LAPACKE_zhbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz );
public static native int LAPACKE_zhbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz );
public static native int LAPACKE_zhbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") double[] ab,
int ldab, double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz );
public static native int LAPACKE_chbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz );
public static native int LAPACKE_chbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz );
public static native int LAPACKE_chbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") float[] ab,
int ldab, float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz );
public static native int LAPACKE_zhbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz );
public static native int LAPACKE_zhbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz );
public static native int LAPACKE_zhbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") double[] ab,
int ldab, double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz );
public static native int LAPACKE_chbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer q, int ldq, float vl,
float vu, int il, int iu, float abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, IntPointer ifail );
public static native int LAPACKE_chbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq, float vl,
float vu, int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, IntBuffer ifail );
public static native int LAPACKE_chbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] q, int ldq, float vl,
float vu, int il, int iu, float abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, int[] ifail );
public static native int LAPACKE_zhbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer q, int ldq, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_zhbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_zhbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] q, int ldq, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] ifail );
public static native int LAPACKE_chbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("const lapack_complex_float*") FloatPointer bb, int ldbb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx );
public static native int LAPACKE_chbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("const lapack_complex_float*") FloatBuffer bb, int ldbb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx );
public static native int LAPACKE_chbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("const lapack_complex_float*") float[] bb, int ldbb,
@Cast("lapack_complex_float*") float[] x, int ldx );
public static native int LAPACKE_zhbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("const lapack_complex_double*") DoublePointer bb, int ldbb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx );
public static native int LAPACKE_zhbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("const lapack_complex_double*") DoubleBuffer bb, int ldbb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx );
public static native int LAPACKE_zhbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("const lapack_complex_double*") double[] bb, int ldbb,
@Cast("lapack_complex_double*") double[] x, int ldx );
public static native int LAPACKE_chbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer bb, int ldbb, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_chbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer bb, int ldbb, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_chbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] bb, int ldbb, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zhbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer bb, int ldbb, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz );
public static native int LAPACKE_zhbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer bb, int ldbb, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz );
public static native int LAPACKE_zhbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] bb, int ldbb, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz );
public static native int LAPACKE_chbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer bb, int ldbb, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_chbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer bb, int ldbb, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_chbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] bb, int ldbb, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zhbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer bb, int ldbb,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz );
public static native int LAPACKE_zhbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer bb, int ldbb,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz );
public static native int LAPACKE_zhbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] bb, int ldbb,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz );
public static native int LAPACKE_chbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer bb, int ldbb,
@Cast("lapack_complex_float*") FloatPointer q, int ldq, float vl,
float vu, int il, int iu, float abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, IntPointer ifail );
public static native int LAPACKE_chbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer bb, int ldbb,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq, float vl,
float vu, int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, IntBuffer ifail );
public static native int LAPACKE_chbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] bb, int ldbb,
@Cast("lapack_complex_float*") float[] q, int ldq, float vl,
float vu, int il, int iu, float abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, int[] ifail );
public static native int LAPACKE_zhbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer bb, int ldbb,
@Cast("lapack_complex_double*") DoublePointer q, int ldq, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_zhbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer bb, int ldbb,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_zhbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] bb, int ldbb,
@Cast("lapack_complex_double*") double[] q, int ldq, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] ifail );
public static native int LAPACKE_chbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer q, int ldq );
public static native int LAPACKE_chbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq );
public static native int LAPACKE_chbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") float[] ab,
int ldab, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] q, int ldq );
public static native int LAPACKE_zhbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer q, int ldq );
public static native int LAPACKE_zhbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq );
public static native int LAPACKE_zhbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") double[] ab,
int ldab, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] q, int ldq );
public static native int LAPACKE_checon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv, float anorm, FloatPointer rcond );
public static native int LAPACKE_checon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv, float anorm, FloatBuffer rcond );
public static native int LAPACKE_checon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv, float anorm, float[] rcond );
public static native int LAPACKE_zhecon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond );
public static native int LAPACKE_zhecon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_zhecon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv, double anorm,
double[] rcond );
public static native int LAPACKE_cheequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer s, FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_cheequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_cheequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] s, float[] scond, float[] amax );
public static native int LAPACKE_zheequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer s, DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_zheequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_zheequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] s, double[] scond, double[] amax );
public static native int LAPACKE_cheev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda, FloatPointer w );
public static native int LAPACKE_cheev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda, FloatBuffer w );
public static native int LAPACKE_cheev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda, float[] w );
public static native int LAPACKE_zheev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda, DoublePointer w );
public static native int LAPACKE_zheev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda, DoubleBuffer w );
public static native int LAPACKE_zheev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda, double[] w );
public static native int LAPACKE_cheevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda, FloatPointer w );
public static native int LAPACKE_cheevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda, FloatBuffer w );
public static native int LAPACKE_cheevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda, float[] w );
public static native int LAPACKE_zheevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
DoublePointer w );
public static native int LAPACKE_zheevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer w );
public static native int LAPACKE_zheevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
double[] w );
public static native int LAPACKE_cheevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, float vl, float vu, int il,
int iu, float abstol, IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
IntPointer isuppz );
public static native int LAPACKE_cheevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, float vl, float vu, int il,
int iu, float abstol, IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
IntBuffer isuppz );
public static native int LAPACKE_cheevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, float vl, float vu, int il,
int iu, float abstol, int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
int[] isuppz );
public static native int LAPACKE_zheevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, double vl, double vu, int il,
int iu, double abstol, IntPointer m,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer isuppz );
public static native int LAPACKE_zheevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, double vl, double vu, int il,
int iu, double abstol, IntBuffer m,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer isuppz );
public static native int LAPACKE_zheevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, double vl, double vu, int il,
int iu, double abstol, int[] m,
double[] w, @Cast("lapack_complex_double*") double[] z, int ldz,
int[] isuppz );
public static native int LAPACKE_cheevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, float vl, float vu, int il,
int iu, float abstol, IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_cheevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, float vl, float vu, int il,
int iu, float abstol, IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_cheevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, float vl, float vu, int il,
int iu, float abstol, int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
int[] ifail );
public static native int LAPACKE_zheevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, double vl, double vu, int il,
int iu, double abstol, IntPointer m,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_zheevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, double vl, double vu, int il,
int iu, double abstol, IntBuffer m,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_zheevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, double vl, double vu, int il,
int iu, double abstol, int[] m,
double[] w, @Cast("lapack_complex_double*") double[] z, int ldz,
int[] ifail );
public static native int LAPACKE_chegst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_chegst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_chegst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zhegst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zhegst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zhegst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_chegv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, FloatPointer w );
public static native int LAPACKE_chegv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, FloatBuffer w );
public static native int LAPACKE_chegv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, float[] w );
public static native int LAPACKE_zhegv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, DoublePointer w );
public static native int LAPACKE_zhegv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, DoubleBuffer w );
public static native int LAPACKE_zhegv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, double[] w );
public static native int LAPACKE_chegvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, FloatPointer w );
public static native int LAPACKE_chegvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, FloatBuffer w );
public static native int LAPACKE_chegvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, float[] w );
public static native int LAPACKE_zhegvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, DoublePointer w );
public static native int LAPACKE_zhegvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, DoubleBuffer w );
public static native int LAPACKE_zhegvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, double[] w );
public static native int LAPACKE_chegvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb, float vl,
float vu, int il, int iu, float abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, IntPointer ifail );
public static native int LAPACKE_chegvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb, float vl,
float vu, int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, IntBuffer ifail );
public static native int LAPACKE_chegvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb, float vl,
float vu, int il, int iu, float abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, int[] ifail );
public static native int LAPACKE_zhegvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_zhegvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_zhegvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] ifail );
public static native int LAPACKE_cherfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_cherfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_cherfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zherfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zherfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zherfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_chesv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_chesv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_chesv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zhesv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zhesv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zhesv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_chesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer af,
int ldaf, IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_chesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer af,
int ldaf, IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_chesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] af,
int ldaf, int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr );
public static native int LAPACKE_zhesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer af,
int ldaf, IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zhesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer af,
int ldaf, IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zhesvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] af,
int ldaf, int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_chetrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda, FloatPointer d,
FloatPointer e, @Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_chetrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda, FloatBuffer d,
FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_chetrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda, float[] d,
float[] e, @Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zhetrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda, DoublePointer d,
DoublePointer e, @Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zhetrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda, DoubleBuffer d,
DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zhetrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda, double[] d,
double[] e, @Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_chetrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_chetrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_chetrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zhetrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zhetrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zhetrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_chetri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_chetri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_chetri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_zhetri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_zhetri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_zhetri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_chetrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_chetrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_chetrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zhetrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zhetrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zhetrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_chfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, float alpha,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
float beta, @Cast("lapack_complex_float*") FloatPointer c );
public static native int LAPACKE_chfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, float alpha,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
float beta, @Cast("lapack_complex_float*") FloatBuffer c );
public static native int LAPACKE_chfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, float alpha,
@Cast("const lapack_complex_float*") float[] a, int lda,
float beta, @Cast("lapack_complex_float*") float[] c );
public static native int LAPACKE_zhfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, double alpha,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
double beta, @Cast("lapack_complex_double*") DoublePointer c );
public static native int LAPACKE_zhfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, double alpha,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
double beta, @Cast("lapack_complex_double*") DoubleBuffer c );
public static native int LAPACKE_zhfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, double alpha,
@Cast("const lapack_complex_double*") double[] a, int lda,
double beta, @Cast("lapack_complex_double*") double[] c );
public static native int LAPACKE_shgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatPointer h, int ldh, FloatPointer t, int ldt,
FloatPointer alphar, FloatPointer alphai, FloatPointer beta, FloatPointer q,
int ldq, FloatPointer z, int ldz );
public static native int LAPACKE_shgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatBuffer h, int ldh, FloatBuffer t, int ldt,
FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta, FloatBuffer q,
int ldq, FloatBuffer z, int ldz );
public static native int LAPACKE_shgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
float[] h, int ldh, float[] t, int ldt,
float[] alphar, float[] alphai, float[] beta, float[] q,
int ldq, float[] z, int ldz );
public static native int LAPACKE_dhgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoublePointer h, int ldh, DoublePointer t, int ldt,
DoublePointer alphar, DoublePointer alphai, DoublePointer beta,
DoublePointer q, int ldq, DoublePointer z,
int ldz );
public static native int LAPACKE_dhgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoubleBuffer h, int ldh, DoubleBuffer t, int ldt,
DoubleBuffer alphar, DoubleBuffer alphai, DoubleBuffer beta,
DoubleBuffer q, int ldq, DoubleBuffer z,
int ldz );
public static native int LAPACKE_dhgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
double[] h, int ldh, double[] t, int ldt,
double[] alphar, double[] alphai, double[] beta,
double[] q, int ldq, double[] z,
int ldz );
public static native int LAPACKE_chgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatPointer h, int ldh,
@Cast("lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer q,
int ldq, @Cast("lapack_complex_float*") FloatPointer z,
int ldz );
public static native int LAPACKE_chgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatBuffer h, int ldh,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer q,
int ldq, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz );
public static native int LAPACKE_chgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") float[] h, int ldh,
@Cast("lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta, @Cast("lapack_complex_float*") float[] q,
int ldq, @Cast("lapack_complex_float*") float[] z,
int ldz );
public static native int LAPACKE_zhgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoublePointer h, int ldh,
@Cast("lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz );
public static native int LAPACKE_zhgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoubleBuffer h, int ldh,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz );
public static native int LAPACKE_zhgeqz( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") double[] h, int ldh,
@Cast("lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz );
public static native int LAPACKE_chpcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, float anorm, FloatPointer rcond );
public static native int LAPACKE_chpcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, float anorm, FloatBuffer rcond );
public static native int LAPACKE_chpcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, float anorm, float[] rcond );
public static native int LAPACKE_zhpcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond );
public static native int LAPACKE_zhpcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_zhpcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv, double anorm,
double[] rcond );
public static native int LAPACKE_chpev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_chpev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_chpev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zhpev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz );
public static native int LAPACKE_zhpev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz );
public static native int LAPACKE_zhpev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz );
public static native int LAPACKE_chpevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_chpevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_chpevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zhpevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz );
public static native int LAPACKE_zhpevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz );
public static native int LAPACKE_zhpevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz );
public static native int LAPACKE_chpevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer ap, float vl,
float vu, int il, int iu, float abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, IntPointer ifail );
public static native int LAPACKE_chpevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer ap, float vl,
float vu, int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, IntBuffer ifail );
public static native int LAPACKE_chpevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] ap, float vl,
float vu, int il, int iu, float abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, int[] ifail );
public static native int LAPACKE_zhpevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer ap, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_zhpevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer ap, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_zhpevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] ap, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] ifail );
public static native int LAPACKE_chpgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer bp );
public static native int LAPACKE_chpgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer bp );
public static native int LAPACKE_chpgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] bp );
public static native int LAPACKE_zhpgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer bp );
public static native int LAPACKE_zhpgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer bp );
public static native int LAPACKE_zhpgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] bp );
public static native int LAPACKE_chpgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer bp, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_chpgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer bp, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_chpgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] bp, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zhpgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer bp, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz );
public static native int LAPACKE_zhpgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer bp, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz );
public static native int LAPACKE_zhpgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] bp, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz );
public static native int LAPACKE_chpgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer bp, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_chpgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer bp, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_chpgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] bp, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zhpgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer bp, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz );
public static native int LAPACKE_zhpgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer bp, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz );
public static native int LAPACKE_zhpgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] bp, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz );
public static native int LAPACKE_chpgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, @Cast("lapack_complex_float*") FloatPointer bp,
float vl, float vu, int il, int iu,
float abstol, IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_chpgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, @Cast("lapack_complex_float*") FloatBuffer bp,
float vl, float vu, int il, int iu,
float abstol, IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_chpgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, @Cast("lapack_complex_float*") float[] bp,
float vl, float vu, int il, int iu,
float abstol, int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
int[] ifail );
public static native int LAPACKE_zhpgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, @Cast("lapack_complex_double*") DoublePointer bp,
double vl, double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_zhpgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, @Cast("lapack_complex_double*") DoubleBuffer bp,
double vl, double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_zhpgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, @Cast("lapack_complex_double*") double[] bp,
double vl, double vu, int il, int iu,
double abstol, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] ifail );
public static native int LAPACKE_chprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer afp,
@Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_chprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer afp,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_chprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] afp,
@Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zhprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer afp,
@Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zhprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer afp,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zhprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] afp,
@Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_chpsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_chpsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_chpsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] ap,
int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zhpsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zhpsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zhpsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] ap,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_chpsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer afp, IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_chpsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer afp, IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_chpsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] afp, int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr );
public static native int LAPACKE_zhpsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer afp, IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zhpsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer afp, IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zhpsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] afp, int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_chptrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_chptrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_chptrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zhptrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zhptrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zhptrd( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_chptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, IntPointer ipiv );
public static native int LAPACKE_chptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_chptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, int[] ipiv );
public static native int LAPACKE_zhptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, IntPointer ipiv );
public static native int LAPACKE_zhptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_zhptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, int[] ipiv );
public static native int LAPACKE_chptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, @Const IntPointer ipiv );
public static native int LAPACKE_chptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, @Const IntBuffer ipiv );
public static native int LAPACKE_chptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, @Const int[] ipiv );
public static native int LAPACKE_zhptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, @Const IntPointer ipiv );
public static native int LAPACKE_zhptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, @Const IntBuffer ipiv );
public static native int LAPACKE_zhptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, @Const int[] ipiv );
public static native int LAPACKE_chptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_chptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_chptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zhptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zhptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zhptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_shsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
IntPointer select, int n, @Const FloatPointer h,
int ldh, FloatPointer wr, @Const FloatPointer wi,
FloatPointer vl, int ldvl, FloatPointer vr,
int ldvr, int mm, IntPointer m,
IntPointer ifaill, IntPointer ifailr );
public static native int LAPACKE_shsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
IntBuffer select, int n, @Const FloatBuffer h,
int ldh, FloatBuffer wr, @Const FloatBuffer wi,
FloatBuffer vl, int ldvl, FloatBuffer vr,
int ldvr, int mm, IntBuffer m,
IntBuffer ifaill, IntBuffer ifailr );
public static native int LAPACKE_shsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
int[] select, int n, @Const float[] h,
int ldh, float[] wr, @Const float[] wi,
float[] vl, int ldvl, float[] vr,
int ldvr, int mm, int[] m,
int[] ifaill, int[] ifailr );
public static native int LAPACKE_dhsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
IntPointer select, int n,
@Const DoublePointer h, int ldh, DoublePointer wr,
@Const DoublePointer wi, DoublePointer vl, int ldvl,
DoublePointer vr, int ldvr, int mm,
IntPointer m, IntPointer ifaill,
IntPointer ifailr );
public static native int LAPACKE_dhsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
IntBuffer select, int n,
@Const DoubleBuffer h, int ldh, DoubleBuffer wr,
@Const DoubleBuffer wi, DoubleBuffer vl, int ldvl,
DoubleBuffer vr, int ldvr, int mm,
IntBuffer m, IntBuffer ifaill,
IntBuffer ifailr );
public static native int LAPACKE_dhsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
int[] select, int n,
@Const double[] h, int ldh, double[] wr,
@Const double[] wi, double[] vl, int ldvl,
double[] vr, int ldvr, int mm,
int[] m, int[] ifaill,
int[] ifailr );
public static native int LAPACKE_chsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
@Const IntPointer select, int n,
@Cast("const lapack_complex_float*") FloatPointer h, int ldh,
@Cast("lapack_complex_float*") FloatPointer w, @Cast("lapack_complex_float*") FloatPointer vl,
int ldvl, @Cast("lapack_complex_float*") FloatPointer vr,
int ldvr, int mm, IntPointer m,
IntPointer ifaill, IntPointer ifailr );
public static native int LAPACKE_chsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_float*") FloatBuffer h, int ldh,
@Cast("lapack_complex_float*") FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer vl,
int ldvl, @Cast("lapack_complex_float*") FloatBuffer vr,
int ldvr, int mm, IntBuffer m,
IntBuffer ifaill, IntBuffer ifailr );
public static native int LAPACKE_chsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
@Const int[] select, int n,
@Cast("const lapack_complex_float*") float[] h, int ldh,
@Cast("lapack_complex_float*") float[] w, @Cast("lapack_complex_float*") float[] vl,
int ldvl, @Cast("lapack_complex_float*") float[] vr,
int ldvr, int mm, int[] m,
int[] ifaill, int[] ifailr );
public static native int LAPACKE_zhsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
@Const IntPointer select, int n,
@Cast("const lapack_complex_double*") DoublePointer h, int ldh,
@Cast("lapack_complex_double*") DoublePointer w, @Cast("lapack_complex_double*") DoublePointer vl,
int ldvl, @Cast("lapack_complex_double*") DoublePointer vr,
int ldvr, int mm, IntPointer m,
IntPointer ifaill, IntPointer ifailr );
public static native int LAPACKE_zhsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_double*") DoubleBuffer h, int ldh,
@Cast("lapack_complex_double*") DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer vl,
int ldvl, @Cast("lapack_complex_double*") DoubleBuffer vr,
int ldvr, int mm, IntBuffer m,
IntBuffer ifaill, IntBuffer ifailr );
public static native int LAPACKE_zhsein( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc, @Cast("char") byte initv,
@Const int[] select, int n,
@Cast("const lapack_complex_double*") double[] h, int ldh,
@Cast("lapack_complex_double*") double[] w, @Cast("lapack_complex_double*") double[] vl,
int ldvl, @Cast("lapack_complex_double*") double[] vr,
int ldvr, int mm, int[] m,
int[] ifaill, int[] ifailr );
public static native int LAPACKE_shseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi, FloatPointer h,
int ldh, FloatPointer wr, FloatPointer wi, FloatPointer z,
int ldz );
public static native int LAPACKE_shseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi, FloatBuffer h,
int ldh, FloatBuffer wr, FloatBuffer wi, FloatBuffer z,
int ldz );
public static native int LAPACKE_shseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi, float[] h,
int ldh, float[] wr, float[] wi, float[] z,
int ldz );
public static native int LAPACKE_dhseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi, DoublePointer h,
int ldh, DoublePointer wr, DoublePointer wi, DoublePointer z,
int ldz );
public static native int LAPACKE_dhseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi, DoubleBuffer h,
int ldh, DoubleBuffer wr, DoubleBuffer wi, DoubleBuffer z,
int ldz );
public static native int LAPACKE_dhseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi, double[] h,
int ldh, double[] wr, double[] wi, double[] z,
int ldz );
public static native int LAPACKE_chseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi,
@Cast("lapack_complex_float*") FloatPointer h, int ldh,
@Cast("lapack_complex_float*") FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz );
public static native int LAPACKE_chseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi,
@Cast("lapack_complex_float*") FloatBuffer h, int ldh,
@Cast("lapack_complex_float*") FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz );
public static native int LAPACKE_chseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi,
@Cast("lapack_complex_float*") float[] h, int ldh,
@Cast("lapack_complex_float*") float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz );
public static native int LAPACKE_zhseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi,
@Cast("lapack_complex_double*") DoublePointer h, int ldh,
@Cast("lapack_complex_double*") DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz );
public static native int LAPACKE_zhseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi,
@Cast("lapack_complex_double*") DoubleBuffer h, int ldh,
@Cast("lapack_complex_double*") DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz );
public static native int LAPACKE_zhseqr( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz, int n,
int ilo, int ihi,
@Cast("lapack_complex_double*") double[] h, int ldh,
@Cast("lapack_complex_double*") double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz );
public static native int LAPACKE_clacgv( int n, @Cast("lapack_complex_float*") FloatPointer x,
int incx );
public static native int LAPACKE_clacgv( int n, @Cast("lapack_complex_float*") FloatBuffer x,
int incx );
public static native int LAPACKE_clacgv( int n, @Cast("lapack_complex_float*") float[] x,
int incx );
public static native int LAPACKE_zlacgv( int n, @Cast("lapack_complex_double*") DoublePointer x,
int incx );
public static native int LAPACKE_zlacgv( int n, @Cast("lapack_complex_double*") DoubleBuffer x,
int incx );
public static native int LAPACKE_zlacgv( int n, @Cast("lapack_complex_double*") double[] x,
int incx );
public static native int LAPACKE_slacn2( int n, FloatPointer v, FloatPointer x, IntPointer isgn,
FloatPointer est, IntPointer kase, IntPointer isave );
public static native int LAPACKE_slacn2( int n, FloatBuffer v, FloatBuffer x, IntBuffer isgn,
FloatBuffer est, IntBuffer kase, IntBuffer isave );
public static native int LAPACKE_slacn2( int n, float[] v, float[] x, int[] isgn,
float[] est, int[] kase, int[] isave );
public static native int LAPACKE_dlacn2( int n, DoublePointer v, DoublePointer x, IntPointer isgn,
DoublePointer est, IntPointer kase, IntPointer isave );
public static native int LAPACKE_dlacn2( int n, DoubleBuffer v, DoubleBuffer x, IntBuffer isgn,
DoubleBuffer est, IntBuffer kase, IntBuffer isave );
public static native int LAPACKE_dlacn2( int n, double[] v, double[] x, int[] isgn,
double[] est, int[] kase, int[] isave );
public static native int LAPACKE_clacn2( int n, @Cast("lapack_complex_float*") FloatPointer v,
@Cast("lapack_complex_float*") FloatPointer x,
FloatPointer est, IntPointer kase, IntPointer isave );
public static native int LAPACKE_clacn2( int n, @Cast("lapack_complex_float*") FloatBuffer v,
@Cast("lapack_complex_float*") FloatBuffer x,
FloatBuffer est, IntBuffer kase, IntBuffer isave );
public static native int LAPACKE_clacn2( int n, @Cast("lapack_complex_float*") float[] v,
@Cast("lapack_complex_float*") float[] x,
float[] est, int[] kase, int[] isave );
public static native int LAPACKE_zlacn2( int n, @Cast("lapack_complex_double*") DoublePointer v,
@Cast("lapack_complex_double*") DoublePointer x,
DoublePointer est, IntPointer kase, IntPointer isave );
public static native int LAPACKE_zlacn2( int n, @Cast("lapack_complex_double*") DoubleBuffer v,
@Cast("lapack_complex_double*") DoubleBuffer x,
DoubleBuffer est, IntBuffer kase, IntBuffer isave );
public static native int LAPACKE_zlacn2( int n, @Cast("lapack_complex_double*") double[] v,
@Cast("lapack_complex_double*") double[] x,
double[] est, int[] kase, int[] isave );
public static native int LAPACKE_slacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const FloatPointer a, int lda, FloatPointer b,
int ldb );
public static native int LAPACKE_slacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const FloatBuffer a, int lda, FloatBuffer b,
int ldb );
public static native int LAPACKE_slacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const float[] a, int lda, float[] b,
int ldb );
public static native int LAPACKE_dlacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const DoublePointer a, int lda, DoublePointer b,
int ldb );
public static native int LAPACKE_dlacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const DoubleBuffer a, int lda, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dlacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const double[] a, int lda, double[] b,
int ldb );
public static native int LAPACKE_clacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_clacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_clacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zlacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zlacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zlacpy( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_clacp2( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_clacp2( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_clacp2( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zlacp2( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zlacp2( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zlacp2( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_zlag2c( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer sa, int ldsa );
public static native int LAPACKE_zlag2c( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer sa, int ldsa );
public static native int LAPACKE_zlag2c( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_float*") float[] sa, int ldsa );
public static native int LAPACKE_slag2d( int matrix_layout, int m, int n,
@Const FloatPointer sa, int ldsa, DoublePointer a,
int lda );
public static native int LAPACKE_slag2d( int matrix_layout, int m, int n,
@Const FloatBuffer sa, int ldsa, DoubleBuffer a,
int lda );
public static native int LAPACKE_slag2d( int matrix_layout, int m, int n,
@Const float[] sa, int ldsa, double[] a,
int lda );
public static native int LAPACKE_dlag2s( int matrix_layout, int m, int n,
@Const DoublePointer a, int lda, FloatPointer sa,
int ldsa );
public static native int LAPACKE_dlag2s( int matrix_layout, int m, int n,
@Const DoubleBuffer a, int lda, FloatBuffer sa,
int ldsa );
public static native int LAPACKE_dlag2s( int matrix_layout, int m, int n,
@Const double[] a, int lda, float[] sa,
int ldsa );
public static native int LAPACKE_clag2z( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer sa, int ldsa,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_clag2z( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer sa, int ldsa,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_clag2z( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") float[] sa, int ldsa,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_slagge( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatPointer d,
FloatPointer a, int lda, IntPointer iseed );
public static native int LAPACKE_slagge( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatBuffer d,
FloatBuffer a, int lda, IntBuffer iseed );
public static native int LAPACKE_slagge( int matrix_layout, int m, int n,
int kl, int ku, @Const float[] d,
float[] a, int lda, int[] iseed );
public static native int LAPACKE_dlagge( int matrix_layout, int m, int n,
int kl, int ku, @Const DoublePointer d,
DoublePointer a, int lda, IntPointer iseed );
public static native int LAPACKE_dlagge( int matrix_layout, int m, int n,
int kl, int ku, @Const DoubleBuffer d,
DoubleBuffer a, int lda, IntBuffer iseed );
public static native int LAPACKE_dlagge( int matrix_layout, int m, int n,
int kl, int ku, @Const double[] d,
double[] a, int lda, int[] iseed );
public static native int LAPACKE_clagge( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer iseed );
public static native int LAPACKE_clagge( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer iseed );
public static native int LAPACKE_clagge( int matrix_layout, int m, int n,
int kl, int ku, @Const float[] d,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] iseed );
public static native int LAPACKE_zlagge( int matrix_layout, int m, int n,
int kl, int ku, @Const DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer iseed );
public static native int LAPACKE_zlagge( int matrix_layout, int m, int n,
int kl, int ku, @Const DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer iseed );
public static native int LAPACKE_zlagge( int matrix_layout, int m, int n,
int kl, int ku, @Const double[] d,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] iseed );
public static native float LAPACKE_slamch( @Cast("char") byte cmach );
public static native double LAPACKE_dlamch( @Cast("char") byte cmach );
public static native float LAPACKE_slange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const FloatPointer a, int lda );
public static native float LAPACKE_slange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const FloatBuffer a, int lda );
public static native float LAPACKE_slange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const float[] a, int lda );
public static native double LAPACKE_dlange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const DoublePointer a, int lda );
public static native double LAPACKE_dlange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const DoubleBuffer a, int lda );
public static native double LAPACKE_dlange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const double[] a, int lda );
public static native float LAPACKE_clange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda );
public static native float LAPACKE_clange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda );
public static native float LAPACKE_clange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_float*") float[] a,
int lda );
public static native double LAPACKE_zlange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda );
public static native double LAPACKE_zlange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda );
public static native double LAPACKE_zlange( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_double*") double[] a,
int lda );
public static native float LAPACKE_clanhe( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda );
public static native float LAPACKE_clanhe( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda );
public static native float LAPACKE_clanhe( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda );
public static native double LAPACKE_zlanhe( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda );
public static native double LAPACKE_zlanhe( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda );
public static native double LAPACKE_zlanhe( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda );
public static native float LAPACKE_slansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Const FloatPointer a, int lda );
public static native float LAPACKE_slansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Const FloatBuffer a, int lda );
public static native float LAPACKE_slansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Const float[] a, int lda );
public static native double LAPACKE_dlansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Const DoublePointer a, int lda );
public static native double LAPACKE_dlansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Const DoubleBuffer a, int lda );
public static native double LAPACKE_dlansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Const double[] a, int lda );
public static native float LAPACKE_clansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda );
public static native float LAPACKE_clansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda );
public static native float LAPACKE_clansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda );
public static native double LAPACKE_zlansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda );
public static native double LAPACKE_zlansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda );
public static native double LAPACKE_zlansy( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda );
public static native float LAPACKE_slantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Const FloatPointer a,
int lda );
public static native float LAPACKE_slantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Const FloatBuffer a,
int lda );
public static native float LAPACKE_slantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Const float[] a,
int lda );
public static native double LAPACKE_dlantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Const DoublePointer a,
int lda );
public static native double LAPACKE_dlantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Const DoubleBuffer a,
int lda );
public static native double LAPACKE_dlantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Const double[] a,
int lda );
public static native float LAPACKE_clantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda );
public static native float LAPACKE_clantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda );
public static native float LAPACKE_clantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Cast("const lapack_complex_float*") float[] a,
int lda );
public static native double LAPACKE_zlantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda );
public static native double LAPACKE_zlantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda );
public static native double LAPACKE_zlantr( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int m, int n, @Cast("const lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_slarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Const FloatPointer v, int ldv,
@Const FloatPointer t, int ldt, FloatPointer c,
int ldc );
public static native int LAPACKE_slarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Const FloatBuffer v, int ldv,
@Const FloatBuffer t, int ldt, FloatBuffer c,
int ldc );
public static native int LAPACKE_slarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Const float[] v, int ldv,
@Const float[] t, int ldt, float[] c,
int ldc );
public static native int LAPACKE_dlarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Const DoublePointer v, int ldv,
@Const DoublePointer t, int ldt, DoublePointer c,
int ldc );
public static native int LAPACKE_dlarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Const DoubleBuffer v, int ldv,
@Const DoubleBuffer t, int ldt, DoubleBuffer c,
int ldc );
public static native int LAPACKE_dlarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Const double[] v, int ldv,
@Const double[] t, int ldt, double[] c,
int ldc );
public static native int LAPACKE_clarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Cast("const lapack_complex_float*") FloatPointer v,
int ldv, @Cast("const lapack_complex_float*") FloatPointer t,
int ldt, @Cast("lapack_complex_float*") FloatPointer c,
int ldc );
public static native int LAPACKE_clarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Cast("const lapack_complex_float*") FloatBuffer v,
int ldv, @Cast("const lapack_complex_float*") FloatBuffer t,
int ldt, @Cast("lapack_complex_float*") FloatBuffer c,
int ldc );
public static native int LAPACKE_clarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Cast("const lapack_complex_float*") float[] v,
int ldv, @Cast("const lapack_complex_float*") float[] t,
int ldt, @Cast("lapack_complex_float*") float[] c,
int ldc );
public static native int LAPACKE_zlarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Cast("const lapack_complex_double*") DoublePointer v,
int ldv, @Cast("const lapack_complex_double*") DoublePointer t,
int ldt, @Cast("lapack_complex_double*") DoublePointer c,
int ldc );
public static native int LAPACKE_zlarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Cast("const lapack_complex_double*") DoubleBuffer v,
int ldv, @Cast("const lapack_complex_double*") DoubleBuffer t,
int ldt, @Cast("lapack_complex_double*") DoubleBuffer c,
int ldc );
public static native int LAPACKE_zlarfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, @Cast("const lapack_complex_double*") double[] v,
int ldv, @Cast("const lapack_complex_double*") double[] t,
int ldt, @Cast("lapack_complex_double*") double[] c,
int ldc );
public static native int LAPACKE_slarfg( int n, FloatPointer alpha, FloatPointer x,
int incx, FloatPointer tau );
public static native int LAPACKE_slarfg( int n, FloatBuffer alpha, FloatBuffer x,
int incx, FloatBuffer tau );
public static native int LAPACKE_slarfg( int n, float[] alpha, float[] x,
int incx, float[] tau );
public static native int LAPACKE_dlarfg( int n, DoublePointer alpha, DoublePointer x,
int incx, DoublePointer tau );
public static native int LAPACKE_dlarfg( int n, DoubleBuffer alpha, DoubleBuffer x,
int incx, DoubleBuffer tau );
public static native int LAPACKE_dlarfg( int n, double[] alpha, double[] x,
int incx, double[] tau );
public static native int LAPACKE_clarfg( int n, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer x, int incx,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_clarfg( int n, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer x, int incx,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_clarfg( int n, @Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] x, int incx,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zlarfg( int n, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer x, int incx,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zlarfg( int n, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer x, int incx,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zlarfg( int n, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] x, int incx,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_slarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const FloatPointer v,
int ldv, @Const FloatPointer tau, FloatPointer t,
int ldt );
public static native int LAPACKE_slarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const FloatBuffer v,
int ldv, @Const FloatBuffer tau, FloatBuffer t,
int ldt );
public static native int LAPACKE_slarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const float[] v,
int ldv, @Const float[] tau, float[] t,
int ldt );
public static native int LAPACKE_dlarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const DoublePointer v,
int ldv, @Const DoublePointer tau, DoublePointer t,
int ldt );
public static native int LAPACKE_dlarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const DoubleBuffer v,
int ldv, @Const DoubleBuffer tau, DoubleBuffer t,
int ldt );
public static native int LAPACKE_dlarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const double[] v,
int ldv, @Const double[] tau, double[] t,
int ldt );
public static native int LAPACKE_clarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_float*") FloatPointer v, int ldv,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer t, int ldt );
public static native int LAPACKE_clarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt );
public static native int LAPACKE_clarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_float*") float[] v, int ldv,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] t, int ldt );
public static native int LAPACKE_zlarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_double*") DoublePointer v, int ldv,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer t, int ldt );
public static native int LAPACKE_zlarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt );
public static native int LAPACKE_zlarft( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_double*") double[] v, int ldv,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] t, int ldt );
public static native int LAPACKE_slarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const FloatPointer v, float tau, FloatPointer c,
int ldc, FloatPointer work );
public static native int LAPACKE_slarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const FloatBuffer v, float tau, FloatBuffer c,
int ldc, FloatBuffer work );
public static native int LAPACKE_slarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const float[] v, float tau, float[] c,
int ldc, float[] work );
public static native int LAPACKE_dlarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const DoublePointer v, double tau, DoublePointer c,
int ldc, DoublePointer work );
public static native int LAPACKE_dlarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const DoubleBuffer v, double tau, DoubleBuffer c,
int ldc, DoubleBuffer work );
public static native int LAPACKE_dlarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const double[] v, double tau, double[] c,
int ldc, double[] work );
public static native int LAPACKE_clarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_float*") FloatPointer v,
@ByVal @Cast("lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer c,
int ldc, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_clarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_float*") FloatBuffer v,
@ByVal @Cast("lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer c,
int ldc, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_clarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_float*") float[] v,
@ByVal @Cast("lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] c,
int ldc, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zlarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_double*") DoublePointer v,
@ByVal @Cast("lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer c,
int ldc, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zlarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_double*") DoubleBuffer v,
@ByVal @Cast("lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer c,
int ldc, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zlarfx( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_double*") double[] v,
@ByVal @Cast("lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] c,
int ldc, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_slarnv( int idist, IntPointer iseed, int n,
FloatPointer x );
public static native int LAPACKE_slarnv( int idist, IntBuffer iseed, int n,
FloatBuffer x );
public static native int LAPACKE_slarnv( int idist, int[] iseed, int n,
float[] x );
public static native int LAPACKE_dlarnv( int idist, IntPointer iseed, int n,
DoublePointer x );
public static native int LAPACKE_dlarnv( int idist, IntBuffer iseed, int n,
DoubleBuffer x );
public static native int LAPACKE_dlarnv( int idist, int[] iseed, int n,
double[] x );
public static native int LAPACKE_clarnv( int idist, IntPointer iseed, int n,
@Cast("lapack_complex_float*") FloatPointer x );
public static native int LAPACKE_clarnv( int idist, IntBuffer iseed, int n,
@Cast("lapack_complex_float*") FloatBuffer x );
public static native int LAPACKE_clarnv( int idist, int[] iseed, int n,
@Cast("lapack_complex_float*") float[] x );
public static native int LAPACKE_zlarnv( int idist, IntPointer iseed, int n,
@Cast("lapack_complex_double*") DoublePointer x );
public static native int LAPACKE_zlarnv( int idist, IntBuffer iseed, int n,
@Cast("lapack_complex_double*") DoubleBuffer x );
public static native int LAPACKE_zlarnv( int idist, int[] iseed, int n,
@Cast("lapack_complex_double*") double[] x );
public static native int LAPACKE_slascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, FloatPointer a,
int lda );
public static native int LAPACKE_slascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, FloatBuffer a,
int lda );
public static native int LAPACKE_slascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, float[] a,
int lda );
public static native int LAPACKE_dlascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, DoublePointer a,
int lda );
public static native int LAPACKE_dlascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, DoubleBuffer a,
int lda );
public static native int LAPACKE_dlascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, double[] a,
int lda );
public static native int LAPACKE_clascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_clascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_clascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, @Cast("lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_zlascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zlascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zlascl( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, @Cast("lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_slaset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, float alpha, float beta, FloatPointer a,
int lda );
public static native int LAPACKE_slaset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, float alpha, float beta, FloatBuffer a,
int lda );
public static native int LAPACKE_slaset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, float alpha, float beta, float[] a,
int lda );
public static native int LAPACKE_dlaset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, double alpha, double beta, DoublePointer a,
int lda );
public static native int LAPACKE_dlaset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, double alpha, double beta, DoubleBuffer a,
int lda );
public static native int LAPACKE_dlaset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, double alpha, double beta, double[] a,
int lda );
public static native int LAPACKE_claset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_float*") FloatPointer alpha,
@ByVal @Cast("lapack_complex_float*") FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_claset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_float*") FloatBuffer alpha,
@ByVal @Cast("lapack_complex_float*") FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_claset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_float*") float[] alpha,
@ByVal @Cast("lapack_complex_float*") float[] beta, @Cast("lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_zlaset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_double*") DoublePointer alpha,
@ByVal @Cast("lapack_complex_double*") DoublePointer beta, @Cast("lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zlaset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_double*") DoubleBuffer alpha,
@ByVal @Cast("lapack_complex_double*") DoubleBuffer beta, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zlaset( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_double*") double[] alpha,
@ByVal @Cast("lapack_complex_double*") double[] beta, @Cast("lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_slasrt( @Cast("char") byte id, int n, FloatPointer d );
public static native int LAPACKE_slasrt( @Cast("char") byte id, int n, FloatBuffer d );
public static native int LAPACKE_slasrt( @Cast("char") byte id, int n, float[] d );
public static native int LAPACKE_dlasrt( @Cast("char") byte id, int n, DoublePointer d );
public static native int LAPACKE_dlasrt( @Cast("char") byte id, int n, DoubleBuffer d );
public static native int LAPACKE_dlasrt( @Cast("char") byte id, int n, double[] d );
public static native int LAPACKE_slaswp( int matrix_layout, int n, FloatPointer a,
int lda, int k1, int k2,
@Const IntPointer ipiv, int incx );
public static native int LAPACKE_slaswp( int matrix_layout, int n, FloatBuffer a,
int lda, int k1, int k2,
@Const IntBuffer ipiv, int incx );
public static native int LAPACKE_slaswp( int matrix_layout, int n, float[] a,
int lda, int k1, int k2,
@Const int[] ipiv, int incx );
public static native int LAPACKE_dlaswp( int matrix_layout, int n, DoublePointer a,
int lda, int k1, int k2,
@Const IntPointer ipiv, int incx );
public static native int LAPACKE_dlaswp( int matrix_layout, int n, DoubleBuffer a,
int lda, int k1, int k2,
@Const IntBuffer ipiv, int incx );
public static native int LAPACKE_dlaswp( int matrix_layout, int n, double[] a,
int lda, int k1, int k2,
@Const int[] ipiv, int incx );
public static native int LAPACKE_claswp( int matrix_layout, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
int k1, int k2, @Const IntPointer ipiv,
int incx );
public static native int LAPACKE_claswp( int matrix_layout, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
int k1, int k2, @Const IntBuffer ipiv,
int incx );
public static native int LAPACKE_claswp( int matrix_layout, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int k1, int k2, @Const int[] ipiv,
int incx );
public static native int LAPACKE_zlaswp( int matrix_layout, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
int k1, int k2, @Const IntPointer ipiv,
int incx );
public static native int LAPACKE_zlaswp( int matrix_layout, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
int k1, int k2, @Const IntBuffer ipiv,
int incx );
public static native int LAPACKE_zlaswp( int matrix_layout, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int k1, int k2, @Const int[] ipiv,
int incx );
public static native int LAPACKE_slatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntPointer iseed, @Cast("char") byte sym, FloatPointer d,
int mode, float cond, float dmax,
int kl, int ku, @Cast("char") byte pack, FloatPointer a,
int lda );
public static native int LAPACKE_slatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntBuffer iseed, @Cast("char") byte sym, FloatBuffer d,
int mode, float cond, float dmax,
int kl, int ku, @Cast("char") byte pack, FloatBuffer a,
int lda );
public static native int LAPACKE_slatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, int[] iseed, @Cast("char") byte sym, float[] d,
int mode, float cond, float dmax,
int kl, int ku, @Cast("char") byte pack, float[] a,
int lda );
public static native int LAPACKE_dlatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntPointer iseed, @Cast("char") byte sym, DoublePointer d,
int mode, double cond, double dmax,
int kl, int ku, @Cast("char") byte pack, DoublePointer a,
int lda );
public static native int LAPACKE_dlatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntBuffer iseed, @Cast("char") byte sym, DoubleBuffer d,
int mode, double cond, double dmax,
int kl, int ku, @Cast("char") byte pack, DoubleBuffer a,
int lda );
public static native int LAPACKE_dlatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, int[] iseed, @Cast("char") byte sym, double[] d,
int mode, double cond, double dmax,
int kl, int ku, @Cast("char") byte pack, double[] a,
int lda );
public static native int LAPACKE_clatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntPointer iseed, @Cast("char") byte sym, FloatPointer d,
int mode, float cond, float dmax,
int kl, int ku, @Cast("char") byte pack,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_clatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntBuffer iseed, @Cast("char") byte sym, FloatBuffer d,
int mode, float cond, float dmax,
int kl, int ku, @Cast("char") byte pack,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_clatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, int[] iseed, @Cast("char") byte sym, float[] d,
int mode, float cond, float dmax,
int kl, int ku, @Cast("char") byte pack,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zlatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntPointer iseed, @Cast("char") byte sym, DoublePointer d,
int mode, double cond, double dmax,
int kl, int ku, @Cast("char") byte pack,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zlatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntBuffer iseed, @Cast("char") byte sym, DoubleBuffer d,
int mode, double cond, double dmax,
int kl, int ku, @Cast("char") byte pack,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zlatms( int matrix_layout, int m, int n,
@Cast("char") byte dist, int[] iseed, @Cast("char") byte sym, double[] d,
int mode, double cond, double dmax,
int kl, int ku, @Cast("char") byte pack,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_slauum( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda );
public static native int LAPACKE_slauum( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda );
public static native int LAPACKE_slauum( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda );
public static native int LAPACKE_dlauum( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda );
public static native int LAPACKE_dlauum( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda );
public static native int LAPACKE_dlauum( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda );
public static native int LAPACKE_clauum( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_clauum( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_clauum( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zlauum( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zlauum( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zlauum( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_sopgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, @Const FloatPointer tau, FloatPointer q,
int ldq );
public static native int LAPACKE_sopgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, @Const FloatBuffer tau, FloatBuffer q,
int ldq );
public static native int LAPACKE_sopgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, @Const float[] tau, float[] q,
int ldq );
public static native int LAPACKE_dopgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, @Const DoublePointer tau, DoublePointer q,
int ldq );
public static native int LAPACKE_dopgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, @Const DoubleBuffer tau, DoubleBuffer q,
int ldq );
public static native int LAPACKE_dopgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, @Const double[] tau, double[] q,
int ldq );
public static native int LAPACKE_sopmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const FloatPointer ap,
@Const FloatPointer tau, FloatPointer c, int ldc );
public static native int LAPACKE_sopmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const FloatBuffer ap,
@Const FloatBuffer tau, FloatBuffer c, int ldc );
public static native int LAPACKE_sopmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const float[] ap,
@Const float[] tau, float[] c, int ldc );
public static native int LAPACKE_dopmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const DoublePointer ap,
@Const DoublePointer tau, DoublePointer c, int ldc );
public static native int LAPACKE_dopmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const DoubleBuffer ap,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc );
public static native int LAPACKE_dopmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const double[] ap,
@Const double[] tau, double[] c, int ldc );
public static native int LAPACKE_sorgbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, FloatPointer a, int lda,
@Const FloatPointer tau );
public static native int LAPACKE_sorgbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, FloatBuffer a, int lda,
@Const FloatBuffer tau );
public static native int LAPACKE_sorgbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, float[] a, int lda,
@Const float[] tau );
public static native int LAPACKE_dorgbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, DoublePointer a,
int lda, @Const DoublePointer tau );
public static native int LAPACKE_dorgbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, DoubleBuffer a,
int lda, @Const DoubleBuffer tau );
public static native int LAPACKE_dorgbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, double[] a,
int lda, @Const double[] tau );
public static native int LAPACKE_sorghr( int matrix_layout, int n, int ilo,
int ihi, FloatPointer a, int lda,
@Const FloatPointer tau );
public static native int LAPACKE_sorghr( int matrix_layout, int n, int ilo,
int ihi, FloatBuffer a, int lda,
@Const FloatBuffer tau );
public static native int LAPACKE_sorghr( int matrix_layout, int n, int ilo,
int ihi, float[] a, int lda,
@Const float[] tau );
public static native int LAPACKE_dorghr( int matrix_layout, int n, int ilo,
int ihi, DoublePointer a, int lda,
@Const DoublePointer tau );
public static native int LAPACKE_dorghr( int matrix_layout, int n, int ilo,
int ihi, DoubleBuffer a, int lda,
@Const DoubleBuffer tau );
public static native int LAPACKE_dorghr( int matrix_layout, int n, int ilo,
int ihi, double[] a, int lda,
@Const double[] tau );
public static native int LAPACKE_sorglq( int matrix_layout, int m, int n,
int k, FloatPointer a, int lda,
@Const FloatPointer tau );
public static native int LAPACKE_sorglq( int matrix_layout, int m, int n,
int k, FloatBuffer a, int lda,
@Const FloatBuffer tau );
public static native int LAPACKE_sorglq( int matrix_layout, int m, int n,
int k, float[] a, int lda,
@Const float[] tau );
public static native int LAPACKE_dorglq( int matrix_layout, int m, int n,
int k, DoublePointer a, int lda,
@Const DoublePointer tau );
public static native int LAPACKE_dorglq( int matrix_layout, int m, int n,
int k, DoubleBuffer a, int lda,
@Const DoubleBuffer tau );
public static native int LAPACKE_dorglq( int matrix_layout, int m, int n,
int k, double[] a, int lda,
@Const double[] tau );
public static native int LAPACKE_sorgql( int matrix_layout, int m, int n,
int k, FloatPointer a, int lda,
@Const FloatPointer tau );
public static native int LAPACKE_sorgql( int matrix_layout, int m, int n,
int k, FloatBuffer a, int lda,
@Const FloatBuffer tau );
public static native int LAPACKE_sorgql( int matrix_layout, int m, int n,
int k, float[] a, int lda,
@Const float[] tau );
public static native int LAPACKE_dorgql( int matrix_layout, int m, int n,
int k, DoublePointer a, int lda,
@Const DoublePointer tau );
public static native int LAPACKE_dorgql( int matrix_layout, int m, int n,
int k, DoubleBuffer a, int lda,
@Const DoubleBuffer tau );
public static native int LAPACKE_dorgql( int matrix_layout, int m, int n,
int k, double[] a, int lda,
@Const double[] tau );
public static native int LAPACKE_sorgqr( int matrix_layout, int m, int n,
int k, FloatPointer a, int lda,
@Const FloatPointer tau );
public static native int LAPACKE_sorgqr( int matrix_layout, int m, int n,
int k, FloatBuffer a, int lda,
@Const FloatBuffer tau );
public static native int LAPACKE_sorgqr( int matrix_layout, int m, int n,
int k, float[] a, int lda,
@Const float[] tau );
public static native int LAPACKE_dorgqr( int matrix_layout, int m, int n,
int k, DoublePointer a, int lda,
@Const DoublePointer tau );
public static native int LAPACKE_dorgqr( int matrix_layout, int m, int n,
int k, DoubleBuffer a, int lda,
@Const DoubleBuffer tau );
public static native int LAPACKE_dorgqr( int matrix_layout, int m, int n,
int k, double[] a, int lda,
@Const double[] tau );
public static native int LAPACKE_sorgrq( int matrix_layout, int m, int n,
int k, FloatPointer a, int lda,
@Const FloatPointer tau );
public static native int LAPACKE_sorgrq( int matrix_layout, int m, int n,
int k, FloatBuffer a, int lda,
@Const FloatBuffer tau );
public static native int LAPACKE_sorgrq( int matrix_layout, int m, int n,
int k, float[] a, int lda,
@Const float[] tau );
public static native int LAPACKE_dorgrq( int matrix_layout, int m, int n,
int k, DoublePointer a, int lda,
@Const DoublePointer tau );
public static native int LAPACKE_dorgrq( int matrix_layout, int m, int n,
int k, DoubleBuffer a, int lda,
@Const DoubleBuffer tau );
public static native int LAPACKE_dorgrq( int matrix_layout, int m, int n,
int k, double[] a, int lda,
@Const double[] tau );
public static native int LAPACKE_sorgtr( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda, @Const FloatPointer tau );
public static native int LAPACKE_sorgtr( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda, @Const FloatBuffer tau );
public static native int LAPACKE_sorgtr( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda, @Const float[] tau );
public static native int LAPACKE_dorgtr( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda, @Const DoublePointer tau );
public static native int LAPACKE_dorgtr( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, @Const DoubleBuffer tau );
public static native int LAPACKE_dorgtr( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda, @Const double[] tau );
public static native int LAPACKE_sormbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatPointer a, int lda, @Const FloatPointer tau,
FloatPointer c, int ldc );
public static native int LAPACKE_sormbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatBuffer a, int lda, @Const FloatBuffer tau,
FloatBuffer c, int ldc );
public static native int LAPACKE_sormbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const float[] a, int lda, @Const float[] tau,
float[] c, int ldc );
public static native int LAPACKE_dormbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoublePointer a, int lda, @Const DoublePointer tau,
DoublePointer c, int ldc );
public static native int LAPACKE_dormbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoubleBuffer a, int lda, @Const DoubleBuffer tau,
DoubleBuffer c, int ldc );
public static native int LAPACKE_dormbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const double[] a, int lda, @Const double[] tau,
double[] c, int ldc );
public static native int LAPACKE_sormhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc );
public static native int LAPACKE_sormhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc );
public static native int LAPACKE_sormhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc );
public static native int LAPACKE_dormhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc );
public static native int LAPACKE_dormhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc );
public static native int LAPACKE_dormhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc );
public static native int LAPACKE_sormlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatPointer a, int lda, @Const FloatPointer tau,
FloatPointer c, int ldc );
public static native int LAPACKE_sormlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatBuffer a, int lda, @Const FloatBuffer tau,
FloatBuffer c, int ldc );
public static native int LAPACKE_sormlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const float[] a, int lda, @Const float[] tau,
float[] c, int ldc );
public static native int LAPACKE_dormlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoublePointer a, int lda, @Const DoublePointer tau,
DoublePointer c, int ldc );
public static native int LAPACKE_dormlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoubleBuffer a, int lda, @Const DoubleBuffer tau,
DoubleBuffer c, int ldc );
public static native int LAPACKE_dormlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const double[] a, int lda, @Const double[] tau,
double[] c, int ldc );
public static native int LAPACKE_sormql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatPointer a, int lda, @Const FloatPointer tau,
FloatPointer c, int ldc );
public static native int LAPACKE_sormql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatBuffer a, int lda, @Const FloatBuffer tau,
FloatBuffer c, int ldc );
public static native int LAPACKE_sormql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const float[] a, int lda, @Const float[] tau,
float[] c, int ldc );
public static native int LAPACKE_dormql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoublePointer a, int lda, @Const DoublePointer tau,
DoublePointer c, int ldc );
public static native int LAPACKE_dormql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoubleBuffer a, int lda, @Const DoubleBuffer tau,
DoubleBuffer c, int ldc );
public static native int LAPACKE_dormql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const double[] a, int lda, @Const double[] tau,
double[] c, int ldc );
public static native int LAPACKE_sormqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatPointer a, int lda, @Const FloatPointer tau,
FloatPointer c, int ldc );
public static native int LAPACKE_sormqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatBuffer a, int lda, @Const FloatBuffer tau,
FloatBuffer c, int ldc );
public static native int LAPACKE_sormqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const float[] a, int lda, @Const float[] tau,
float[] c, int ldc );
public static native int LAPACKE_dormqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoublePointer a, int lda, @Const DoublePointer tau,
DoublePointer c, int ldc );
public static native int LAPACKE_dormqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoubleBuffer a, int lda, @Const DoubleBuffer tau,
DoubleBuffer c, int ldc );
public static native int LAPACKE_dormqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const double[] a, int lda, @Const double[] tau,
double[] c, int ldc );
public static native int LAPACKE_sormrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatPointer a, int lda, @Const FloatPointer tau,
FloatPointer c, int ldc );
public static native int LAPACKE_sormrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatBuffer a, int lda, @Const FloatBuffer tau,
FloatBuffer c, int ldc );
public static native int LAPACKE_sormrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const float[] a, int lda, @Const float[] tau,
float[] c, int ldc );
public static native int LAPACKE_dormrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoublePointer a, int lda, @Const DoublePointer tau,
DoublePointer c, int ldc );
public static native int LAPACKE_dormrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoubleBuffer a, int lda, @Const DoubleBuffer tau,
DoubleBuffer c, int ldc );
public static native int LAPACKE_dormrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const double[] a, int lda, @Const double[] tau,
double[] c, int ldc );
public static native int LAPACKE_sormrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc );
public static native int LAPACKE_sormrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc );
public static native int LAPACKE_sormrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc );
public static native int LAPACKE_dormrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc );
public static native int LAPACKE_dormrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc );
public static native int LAPACKE_dormrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc );
public static native int LAPACKE_sormtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const FloatPointer a,
int lda, @Const FloatPointer tau, FloatPointer c,
int ldc );
public static native int LAPACKE_sormtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const FloatBuffer a,
int lda, @Const FloatBuffer tau, FloatBuffer c,
int ldc );
public static native int LAPACKE_sormtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const float[] a,
int lda, @Const float[] tau, float[] c,
int ldc );
public static native int LAPACKE_dormtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const DoublePointer a,
int lda, @Const DoublePointer tau, DoublePointer c,
int ldc );
public static native int LAPACKE_dormtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const DoubleBuffer a,
int lda, @Const DoubleBuffer tau, DoubleBuffer c,
int ldc );
public static native int LAPACKE_dormtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n, @Const double[] a,
int lda, @Const double[] tau, double[] c,
int ldc );
public static native int LAPACKE_spbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const FloatPointer ab, int ldab,
float anorm, FloatPointer rcond );
public static native int LAPACKE_spbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const FloatBuffer ab, int ldab,
float anorm, FloatBuffer rcond );
public static native int LAPACKE_spbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const float[] ab, int ldab,
float anorm, float[] rcond );
public static native int LAPACKE_dpbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const DoublePointer ab, int ldab,
double anorm, DoublePointer rcond );
public static native int LAPACKE_dpbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const DoubleBuffer ab, int ldab,
double anorm, DoubleBuffer rcond );
public static native int LAPACKE_dpbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const double[] ab, int ldab,
double anorm, double[] rcond );
public static native int LAPACKE_cpbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") FloatPointer ab,
int ldab, float anorm, FloatPointer rcond );
public static native int LAPACKE_cpbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab, float anorm, FloatBuffer rcond );
public static native int LAPACKE_cpbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") float[] ab,
int ldab, float anorm, float[] rcond );
public static native int LAPACKE_zpbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, double anorm, DoublePointer rcond );
public static native int LAPACKE_zpbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, double anorm, DoubleBuffer rcond );
public static native int LAPACKE_zpbcon( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") double[] ab,
int ldab, double anorm, double[] rcond );
public static native int LAPACKE_spbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const FloatPointer ab, int ldab,
FloatPointer s, FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_spbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const FloatBuffer ab, int ldab,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_spbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const float[] ab, int ldab,
float[] s, float[] scond, float[] amax );
public static native int LAPACKE_dpbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const DoublePointer ab, int ldab,
DoublePointer s, DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_dpbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const DoubleBuffer ab, int ldab,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_dpbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const double[] ab, int ldab,
double[] s, double[] scond, double[] amax );
public static native int LAPACKE_cpbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") FloatPointer ab,
int ldab, FloatPointer s, FloatPointer scond,
FloatPointer amax );
public static native int LAPACKE_cpbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab, FloatBuffer s, FloatBuffer scond,
FloatBuffer amax );
public static native int LAPACKE_cpbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") float[] ab,
int ldab, float[] s, float[] scond,
float[] amax );
public static native int LAPACKE_zpbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer s, DoublePointer scond,
DoublePointer amax );
public static native int LAPACKE_zpbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer s, DoubleBuffer scond,
DoubleBuffer amax );
public static native int LAPACKE_zpbequ( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") double[] ab,
int ldab, double[] s, double[] scond,
double[] amax );
public static native int LAPACKE_spbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const FloatPointer ab,
int ldab, @Const FloatPointer afb, int ldafb,
@Const FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_spbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const FloatBuffer ab,
int ldab, @Const FloatBuffer afb, int ldafb,
@Const FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_spbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const float[] ab,
int ldab, @Const float[] afb, int ldafb,
@Const float[] b, int ldb, float[] x,
int ldx, float[] ferr, float[] berr );
public static native int LAPACKE_dpbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const DoublePointer ab,
int ldab, @Const DoublePointer afb, int ldafb,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dpbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const DoubleBuffer ab,
int ldab, @Const DoubleBuffer afb, int ldafb,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dpbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const double[] ab,
int ldab, @Const double[] afb, int ldafb,
@Const double[] b, int ldb, double[] x,
int ldx, double[] ferr, double[] berr );
public static native int LAPACKE_cpbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("const lapack_complex_float*") FloatPointer afb, int ldafb,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_cpbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("const lapack_complex_float*") FloatBuffer afb, int ldafb,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_cpbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Cast("const lapack_complex_float*") float[] afb, int ldafb,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zpbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("const lapack_complex_double*") DoublePointer afb, int ldafb,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zpbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("const lapack_complex_double*") DoubleBuffer afb, int ldafb,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zpbrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
@Cast("const lapack_complex_double*") double[] afb, int ldafb,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_spbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, FloatPointer bb, int ldbb );
public static native int LAPACKE_spbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, FloatBuffer bb, int ldbb );
public static native int LAPACKE_spbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, float[] bb, int ldbb );
public static native int LAPACKE_dpbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, DoublePointer bb, int ldbb );
public static native int LAPACKE_dpbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, DoubleBuffer bb, int ldbb );
public static native int LAPACKE_dpbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, double[] bb, int ldbb );
public static native int LAPACKE_cpbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_float*") FloatPointer bb,
int ldbb );
public static native int LAPACKE_cpbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_float*") FloatBuffer bb,
int ldbb );
public static native int LAPACKE_cpbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_float*") float[] bb,
int ldbb );
public static native int LAPACKE_zpbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_double*") DoublePointer bb,
int ldbb );
public static native int LAPACKE_zpbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_double*") DoubleBuffer bb,
int ldbb );
public static native int LAPACKE_zpbstf( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_double*") double[] bb,
int ldbb );
public static native int LAPACKE_spbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, FloatPointer ab,
int ldab, FloatPointer b, int ldb );
public static native int LAPACKE_spbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, FloatBuffer ab,
int ldab, FloatBuffer b, int ldb );
public static native int LAPACKE_spbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, float[] ab,
int ldab, float[] b, int ldb );
public static native int LAPACKE_dpbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, DoublePointer ab,
int ldab, DoublePointer b, int ldb );
public static native int LAPACKE_dpbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, DoubleBuffer ab,
int ldab, DoubleBuffer b, int ldb );
public static native int LAPACKE_dpbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, double[] ab,
int ldab, double[] b, int ldb );
public static native int LAPACKE_cpbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zpbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zpbsv( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_spbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs, FloatPointer ab,
int ldab, FloatPointer afb, int ldafb,
@Cast("char*") BytePointer equed, FloatPointer s, FloatPointer b, int ldb,
FloatPointer x, int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_spbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs, FloatBuffer ab,
int ldab, FloatBuffer afb, int ldafb,
@Cast("char*") ByteBuffer equed, FloatBuffer s, FloatBuffer b, int ldb,
FloatBuffer x, int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_spbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs, float[] ab,
int ldab, float[] afb, int ldafb,
@Cast("char*") byte[] equed, float[] s, float[] b, int ldb,
float[] x, int ldx, float[] rcond, float[] ferr,
float[] berr );
public static native int LAPACKE_dpbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs, DoublePointer ab,
int ldab, DoublePointer afb, int ldafb,
@Cast("char*") BytePointer equed, DoublePointer s, DoublePointer b, int ldb,
DoublePointer x, int ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dpbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs, DoubleBuffer ab,
int ldab, DoubleBuffer afb, int ldafb,
@Cast("char*") ByteBuffer equed, DoubleBuffer s, DoubleBuffer b, int ldb,
DoubleBuffer x, int ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dpbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs, double[] ab,
int ldab, double[] afb, int ldafb,
@Cast("char*") byte[] equed, double[] s, double[] b, int ldb,
double[] x, int ldx, double[] rcond,
double[] ferr, double[] berr );
public static native int LAPACKE_cpbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer afb, int ldafb,
@Cast("char*") BytePointer equed, FloatPointer s, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_cpbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer afb, int ldafb,
@Cast("char*") ByteBuffer equed, FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_cpbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] afb, int ldafb,
@Cast("char*") byte[] equed, float[] s, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr );
public static native int LAPACKE_zpbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer afb, int ldafb,
@Cast("char*") BytePointer equed, DoublePointer s, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr );
public static native int LAPACKE_zpbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer afb, int ldafb,
@Cast("char*") ByteBuffer equed, DoubleBuffer s, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr );
public static native int LAPACKE_zpbsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] afb, int ldafb,
@Cast("char*") byte[] equed, double[] s, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, double[] rcond, double[] ferr,
double[] berr );
public static native int LAPACKE_spbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, FloatPointer ab, int ldab );
public static native int LAPACKE_spbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, FloatBuffer ab, int ldab );
public static native int LAPACKE_spbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, float[] ab, int ldab );
public static native int LAPACKE_dpbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, DoublePointer ab, int ldab );
public static native int LAPACKE_dpbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, DoubleBuffer ab, int ldab );
public static native int LAPACKE_dpbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, double[] ab, int ldab );
public static native int LAPACKE_cpbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab );
public static native int LAPACKE_cpbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab );
public static native int LAPACKE_cpbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") float[] ab,
int ldab );
public static native int LAPACKE_zpbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab );
public static native int LAPACKE_zpbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab );
public static native int LAPACKE_zpbtrf( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") double[] ab,
int ldab );
public static native int LAPACKE_spbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const FloatPointer ab,
int ldab, FloatPointer b, int ldb );
public static native int LAPACKE_spbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const FloatBuffer ab,
int ldab, FloatBuffer b, int ldb );
public static native int LAPACKE_spbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const float[] ab,
int ldab, float[] b, int ldb );
public static native int LAPACKE_dpbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const DoublePointer ab,
int ldab, DoublePointer b, int ldb );
public static native int LAPACKE_dpbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const DoubleBuffer ab,
int ldab, DoubleBuffer b, int ldb );
public static native int LAPACKE_dpbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const double[] ab,
int ldab, double[] b, int ldb );
public static native int LAPACKE_cpbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zpbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zpbtrs( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_spftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, FloatPointer a );
public static native int LAPACKE_spftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, FloatBuffer a );
public static native int LAPACKE_spftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, float[] a );
public static native int LAPACKE_dpftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, DoublePointer a );
public static native int LAPACKE_dpftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, DoubleBuffer a );
public static native int LAPACKE_dpftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, double[] a );
public static native int LAPACKE_cpftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a );
public static native int LAPACKE_cpftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a );
public static native int LAPACKE_cpftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a );
public static native int LAPACKE_zpftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a );
public static native int LAPACKE_zpftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a );
public static native int LAPACKE_zpftrf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a );
public static native int LAPACKE_spftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, FloatPointer a );
public static native int LAPACKE_spftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, FloatBuffer a );
public static native int LAPACKE_spftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, float[] a );
public static native int LAPACKE_dpftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, DoublePointer a );
public static native int LAPACKE_dpftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, DoubleBuffer a );
public static native int LAPACKE_dpftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, double[] a );
public static native int LAPACKE_cpftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a );
public static native int LAPACKE_cpftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a );
public static native int LAPACKE_cpftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a );
public static native int LAPACKE_zpftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a );
public static native int LAPACKE_zpftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a );
public static native int LAPACKE_zpftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a );
public static native int LAPACKE_spftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const FloatPointer a,
FloatPointer b, int ldb );
public static native int LAPACKE_spftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const FloatBuffer a,
FloatBuffer b, int ldb );
public static native int LAPACKE_spftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const float[] a,
float[] b, int ldb );
public static native int LAPACKE_dpftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const DoublePointer a,
DoublePointer b, int ldb );
public static native int LAPACKE_dpftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const DoubleBuffer a,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dpftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const double[] a,
double[] b, int ldb );
public static native int LAPACKE_cpftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer a,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] a,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer a,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zpftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zpftrs( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] a,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_spocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer a, int lda, float anorm,
FloatPointer rcond );
public static native int LAPACKE_spocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer a, int lda, float anorm,
FloatBuffer rcond );
public static native int LAPACKE_spocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] a, int lda, float anorm,
float[] rcond );
public static native int LAPACKE_dpocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer a, int lda, double anorm,
DoublePointer rcond );
public static native int LAPACKE_dpocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer a, int lda, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_dpocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] a, int lda, double anorm,
double[] rcond );
public static native int LAPACKE_cpocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
float anorm, FloatPointer rcond );
public static native int LAPACKE_cpocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
float anorm, FloatBuffer rcond );
public static native int LAPACKE_cpocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float anorm, float[] rcond );
public static native int LAPACKE_zpocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
double anorm, DoublePointer rcond );
public static native int LAPACKE_zpocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
double anorm, DoubleBuffer rcond );
public static native int LAPACKE_zpocon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double anorm, double[] rcond );
public static native int LAPACKE_spoequ( int matrix_layout, int n, @Const FloatPointer a,
int lda, FloatPointer s, FloatPointer scond,
FloatPointer amax );
public static native int LAPACKE_spoequ( int matrix_layout, int n, @Const FloatBuffer a,
int lda, FloatBuffer s, FloatBuffer scond,
FloatBuffer amax );
public static native int LAPACKE_spoequ( int matrix_layout, int n, @Const float[] a,
int lda, float[] s, float[] scond,
float[] amax );
public static native int LAPACKE_dpoequ( int matrix_layout, int n, @Const DoublePointer a,
int lda, DoublePointer s, DoublePointer scond,
DoublePointer amax );
public static native int LAPACKE_dpoequ( int matrix_layout, int n, @Const DoubleBuffer a,
int lda, DoubleBuffer s, DoubleBuffer scond,
DoubleBuffer amax );
public static native int LAPACKE_dpoequ( int matrix_layout, int n, @Const double[] a,
int lda, double[] s, double[] scond,
double[] amax );
public static native int LAPACKE_cpoequ( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer s, FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_cpoequ( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_cpoequ( int matrix_layout, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] s, float[] scond, float[] amax );
public static native int LAPACKE_zpoequ( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer s, DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_zpoequ( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_zpoequ( int matrix_layout, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] s, double[] scond, double[] amax );
public static native int LAPACKE_spoequb( int matrix_layout, int n, @Const FloatPointer a,
int lda, FloatPointer s, FloatPointer scond,
FloatPointer amax );
public static native int LAPACKE_spoequb( int matrix_layout, int n, @Const FloatBuffer a,
int lda, FloatBuffer s, FloatBuffer scond,
FloatBuffer amax );
public static native int LAPACKE_spoequb( int matrix_layout, int n, @Const float[] a,
int lda, float[] s, float[] scond,
float[] amax );
public static native int LAPACKE_dpoequb( int matrix_layout, int n, @Const DoublePointer a,
int lda, DoublePointer s, DoublePointer scond,
DoublePointer amax );
public static native int LAPACKE_dpoequb( int matrix_layout, int n, @Const DoubleBuffer a,
int lda, DoubleBuffer s, DoubleBuffer scond,
DoubleBuffer amax );
public static native int LAPACKE_dpoequb( int matrix_layout, int n, @Const double[] a,
int lda, double[] s, double[] scond,
double[] amax );
public static native int LAPACKE_cpoequb( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer s, FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_cpoequb( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_cpoequb( int matrix_layout, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] s, float[] scond, float[] amax );
public static native int LAPACKE_zpoequb( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer s, DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_zpoequb( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_zpoequb( int matrix_layout, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] s, double[] scond, double[] amax );
public static native int LAPACKE_sporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const FloatPointer af, int ldaf, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_sporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const FloatBuffer af, int ldaf, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_sporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
@Const float[] af, int ldaf, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr );
public static native int LAPACKE_dporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a, int lda,
@Const DoublePointer af, int ldaf, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a, int lda,
@Const DoubleBuffer af, int ldaf, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a, int lda,
@Const double[] af, int ldaf, @Const double[] b,
int ldb, double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_cporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer af,
int ldaf, @Cast("const lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_cporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer af,
int ldaf, @Cast("const lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_cporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] af,
int ldaf, @Cast("const lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] ferr, float[] berr );
public static native int LAPACKE_zporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer af,
int ldaf, @Cast("const lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer af,
int ldaf, @Cast("const lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zporfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] af,
int ldaf, @Cast("const lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, double[] ferr, double[] berr );
public static native int LAPACKE_sposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer a, int lda, FloatPointer b,
int ldb );
public static native int LAPACKE_sposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer a, int lda, FloatBuffer b,
int ldb );
public static native int LAPACKE_sposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] a, int lda, float[] b,
int ldb );
public static native int LAPACKE_dposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer a, int lda, DoublePointer b,
int ldb );
public static native int LAPACKE_dposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer a, int lda, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] a, int lda, double[] b,
int ldb );
public static native int LAPACKE_cposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_dsposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer x, int ldx,
IntPointer iter );
public static native int LAPACKE_dsposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer x, int ldx,
IntBuffer iter );
public static native int LAPACKE_dsposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] a, int lda,
double[] b, int ldb, double[] x, int ldx,
int[] iter );
public static native int LAPACKE_zcposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, IntPointer iter );
public static native int LAPACKE_zcposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, IntBuffer iter );
public static native int LAPACKE_zcposv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, int[] iter );
public static native int LAPACKE_sposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer a, int lda, FloatPointer af,
int ldaf, @Cast("char*") BytePointer equed, FloatPointer s, FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_sposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer a, int lda, FloatBuffer af,
int ldaf, @Cast("char*") ByteBuffer equed, FloatBuffer s, FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_sposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, float[] a, int lda, float[] af,
int ldaf, @Cast("char*") byte[] equed, float[] s, float[] b,
int ldb, float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr );
public static native int LAPACKE_dposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer af, int ldaf, @Cast("char*") BytePointer equed, DoublePointer s,
DoublePointer b, int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer af, int ldaf, @Cast("char*") ByteBuffer equed, DoubleBuffer s,
DoubleBuffer b, int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, double[] a, int lda,
double[] af, int ldaf, @Cast("char*") byte[] equed, double[] s,
double[] b, int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_cposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer af,
int ldaf, @Cast("char*") BytePointer equed, FloatPointer s,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_cposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer af,
int ldaf, @Cast("char*") ByteBuffer equed, FloatBuffer s,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_cposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] af,
int ldaf, @Cast("char*") byte[] equed, float[] s,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr );
public static native int LAPACKE_zposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer af,
int ldaf, @Cast("char*") BytePointer equed, DoublePointer s,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer af,
int ldaf, @Cast("char*") ByteBuffer equed, DoubleBuffer s,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zposvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] af,
int ldaf, @Cast("char*") byte[] equed, double[] s,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_spotrf2( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda );
public static native int LAPACKE_spotrf2( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda );
public static native int LAPACKE_spotrf2( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda );
public static native int LAPACKE_dpotrf2( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda );
public static native int LAPACKE_dpotrf2( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda );
public static native int LAPACKE_dpotrf2( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda );
public static native int LAPACKE_cpotrf2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_cpotrf2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_cpotrf2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zpotrf2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zpotrf2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zpotrf2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_spotrf( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda );
public static native int LAPACKE_spotrf( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda );
public static native int LAPACKE_spotrf( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda );
public static native int LAPACKE_dpotrf( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda );
public static native int LAPACKE_dpotrf( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda );
public static native int LAPACKE_dpotrf( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda );
public static native int LAPACKE_cpotrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_cpotrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_cpotrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zpotrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zpotrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zpotrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_spotri( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda );
public static native int LAPACKE_spotri( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda );
public static native int LAPACKE_spotri( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda );
public static native int LAPACKE_dpotri( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda );
public static native int LAPACKE_dpotri( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda );
public static native int LAPACKE_dpotri( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda );
public static native int LAPACKE_cpotri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_cpotri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_cpotri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zpotri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zpotri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zpotri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_spotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
FloatPointer b, int ldb );
public static native int LAPACKE_spotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
FloatBuffer b, int ldb );
public static native int LAPACKE_spotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
float[] b, int ldb );
public static native int LAPACKE_dpotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a, int lda,
DoublePointer b, int ldb );
public static native int LAPACKE_dpotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a, int lda,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dpotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a, int lda,
double[] b, int ldb );
public static native int LAPACKE_cpotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cpotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cpotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zpotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zpotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zpotrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_sppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, float anorm, FloatPointer rcond );
public static native int LAPACKE_sppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, float anorm, FloatBuffer rcond );
public static native int LAPACKE_sppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, float anorm, float[] rcond );
public static native int LAPACKE_dppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, double anorm, DoublePointer rcond );
public static native int LAPACKE_dppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, double anorm, DoubleBuffer rcond );
public static native int LAPACKE_dppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, double anorm, double[] rcond );
public static native int LAPACKE_cppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap, float anorm,
FloatPointer rcond );
public static native int LAPACKE_cppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap, float anorm,
FloatBuffer rcond );
public static native int LAPACKE_cppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap, float anorm,
float[] rcond );
public static native int LAPACKE_zppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap, double anorm,
DoublePointer rcond );
public static native int LAPACKE_zppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_zppcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap, double anorm,
double[] rcond );
public static native int LAPACKE_sppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, FloatPointer s, FloatPointer scond,
FloatPointer amax );
public static native int LAPACKE_sppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, FloatBuffer s, FloatBuffer scond,
FloatBuffer amax );
public static native int LAPACKE_sppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, float[] s, float[] scond,
float[] amax );
public static native int LAPACKE_dppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, DoublePointer s, DoublePointer scond,
DoublePointer amax );
public static native int LAPACKE_dppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, DoubleBuffer s, DoubleBuffer scond,
DoubleBuffer amax );
public static native int LAPACKE_dppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, double[] s, double[] scond,
double[] amax );
public static native int LAPACKE_cppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap, FloatPointer s,
FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_cppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap, FloatBuffer s,
FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_cppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap, float[] s,
float[] scond, float[] amax );
public static native int LAPACKE_zppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap, DoublePointer s,
DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_zppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap, DoubleBuffer s,
DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_zppequ( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap, double[] s,
double[] scond, double[] amax );
public static native int LAPACKE_spprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer ap, @Const FloatPointer afp,
@Const FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_spprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer ap, @Const FloatBuffer afp,
@Const FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_spprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] ap, @Const float[] afp,
@Const float[] b, int ldb, float[] x,
int ldx, float[] ferr, float[] berr );
public static native int LAPACKE_dpprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer ap, @Const DoublePointer afp,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dpprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer ap, @Const DoubleBuffer afp,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dpprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] ap, @Const double[] afp,
@Const double[] b, int ldb, double[] x,
int ldx, double[] ferr, double[] berr );
public static native int LAPACKE_cpprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer afp,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_cpprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer afp,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_cpprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] afp,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zpprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer afp,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zpprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer afp,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zpprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] afp,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_sppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer ap, FloatPointer b,
int ldb );
public static native int LAPACKE_sppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer ap, FloatBuffer b,
int ldb );
public static native int LAPACKE_sppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] ap, float[] b,
int ldb );
public static native int LAPACKE_dppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer ap, DoublePointer b,
int ldb );
public static native int LAPACKE_dppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer ap, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] ap, double[] b,
int ldb );
public static native int LAPACKE_cppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zppsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer ap, FloatPointer afp, @Cast("char*") BytePointer equed,
FloatPointer s, FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_sppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer ap, FloatBuffer afp, @Cast("char*") ByteBuffer equed,
FloatBuffer s, FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_sppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, float[] ap, float[] afp, @Cast("char*") byte[] equed,
float[] s, float[] b, int ldb, float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr );
public static native int LAPACKE_dppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer ap, DoublePointer afp,
@Cast("char*") BytePointer equed, DoublePointer s, DoublePointer b, int ldb,
DoublePointer x, int ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer ap, DoubleBuffer afp,
@Cast("char*") ByteBuffer equed, DoubleBuffer s, DoubleBuffer b, int ldb,
DoubleBuffer x, int ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, double[] ap, double[] afp,
@Cast("char*") byte[] equed, double[] s, double[] b, int ldb,
double[] x, int ldx, double[] rcond,
double[] ferr, double[] berr );
public static native int LAPACKE_cppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer afp, @Cast("char*") BytePointer equed, FloatPointer s,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_cppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer afp, @Cast("char*") ByteBuffer equed, FloatBuffer s,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_cppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] afp, @Cast("char*") byte[] equed, float[] s,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr );
public static native int LAPACKE_zppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer afp, @Cast("char*") BytePointer equed, DoublePointer s,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer afp, @Cast("char*") ByteBuffer equed, DoubleBuffer s,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zppsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] afp, @Cast("char*") byte[] equed, double[] s,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_spptrf( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer ap );
public static native int LAPACKE_spptrf( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer ap );
public static native int LAPACKE_spptrf( int matrix_layout, @Cast("char") byte uplo, int n,
float[] ap );
public static native int LAPACKE_dpptrf( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap );
public static native int LAPACKE_dpptrf( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap );
public static native int LAPACKE_dpptrf( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap );
public static native int LAPACKE_cpptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_cpptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_cpptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_zpptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_zpptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_zpptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_spptri( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer ap );
public static native int LAPACKE_spptri( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer ap );
public static native int LAPACKE_spptri( int matrix_layout, @Cast("char") byte uplo, int n,
float[] ap );
public static native int LAPACKE_dpptri( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap );
public static native int LAPACKE_dpptri( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap );
public static native int LAPACKE_dpptri( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap );
public static native int LAPACKE_cpptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_cpptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_cpptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_zpptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_zpptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_zpptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_spptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer ap, FloatPointer b,
int ldb );
public static native int LAPACKE_spptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer ap, FloatBuffer b,
int ldb );
public static native int LAPACKE_spptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] ap, float[] b,
int ldb );
public static native int LAPACKE_dpptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer ap, DoublePointer b,
int ldb );
public static native int LAPACKE_dpptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer ap, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dpptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] ap, double[] b,
int ldb );
public static native int LAPACKE_cpptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zpptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zpptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_spstrf( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda, IntPointer piv, IntPointer rank,
float tol );
public static native int LAPACKE_spstrf( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda, IntBuffer piv, IntBuffer rank,
float tol );
public static native int LAPACKE_spstrf( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda, int[] piv, int[] rank,
float tol );
public static native int LAPACKE_dpstrf( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda, IntPointer piv, IntPointer rank,
double tol );
public static native int LAPACKE_dpstrf( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, IntBuffer piv, IntBuffer rank,
double tol );
public static native int LAPACKE_dpstrf( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda, int[] piv, int[] rank,
double tol );
public static native int LAPACKE_cpstrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer piv, IntPointer rank, float tol );
public static native int LAPACKE_cpstrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer piv, IntBuffer rank, float tol );
public static native int LAPACKE_cpstrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] piv, int[] rank, float tol );
public static native int LAPACKE_zpstrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer piv, IntPointer rank, double tol );
public static native int LAPACKE_zpstrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer piv, IntBuffer rank, double tol );
public static native int LAPACKE_zpstrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] piv, int[] rank, double tol );
public static native int LAPACKE_sptcon( int n, @Const FloatPointer d, @Const FloatPointer e,
float anorm, FloatPointer rcond );
public static native int LAPACKE_sptcon( int n, @Const FloatBuffer d, @Const FloatBuffer e,
float anorm, FloatBuffer rcond );
public static native int LAPACKE_sptcon( int n, @Const float[] d, @Const float[] e,
float anorm, float[] rcond );
public static native int LAPACKE_dptcon( int n, @Const DoublePointer d, @Const DoublePointer e,
double anorm, DoublePointer rcond );
public static native int LAPACKE_dptcon( int n, @Const DoubleBuffer d, @Const DoubleBuffer e,
double anorm, DoubleBuffer rcond );
public static native int LAPACKE_dptcon( int n, @Const double[] d, @Const double[] e,
double anorm, double[] rcond );
public static native int LAPACKE_cptcon( int n, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, float anorm,
FloatPointer rcond );
public static native int LAPACKE_cptcon( int n, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, float anorm,
FloatBuffer rcond );
public static native int LAPACKE_cptcon( int n, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, float anorm,
float[] rcond );
public static native int LAPACKE_zptcon( int n, @Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e, double anorm,
DoublePointer rcond );
public static native int LAPACKE_zptcon( int n, @Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_zptcon( int n, @Const double[] d,
@Cast("const lapack_complex_double*") double[] e, double anorm,
double[] rcond );
public static native int LAPACKE_spteqr( int matrix_layout, @Cast("char") byte compz, int n, FloatPointer d,
FloatPointer e, FloatPointer z, int ldz );
public static native int LAPACKE_spteqr( int matrix_layout, @Cast("char") byte compz, int n, FloatBuffer d,
FloatBuffer e, FloatBuffer z, int ldz );
public static native int LAPACKE_spteqr( int matrix_layout, @Cast("char") byte compz, int n, float[] d,
float[] e, float[] z, int ldz );
public static native int LAPACKE_dpteqr( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, DoublePointer z, int ldz );
public static native int LAPACKE_dpteqr( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer z, int ldz );
public static native int LAPACKE_dpteqr( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, double[] z, int ldz );
public static native int LAPACKE_cpteqr( int matrix_layout, @Cast("char") byte compz, int n, FloatPointer d,
FloatPointer e, @Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_cpteqr( int matrix_layout, @Cast("char") byte compz, int n, FloatBuffer d,
FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_cpteqr( int matrix_layout, @Cast("char") byte compz, int n, float[] d,
float[] e, @Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zpteqr( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer z,
int ldz );
public static native int LAPACKE_zpteqr( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz );
public static native int LAPACKE_zpteqr( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] z,
int ldz );
public static native int LAPACKE_sptrfs( int matrix_layout, int n, int nrhs,
@Const FloatPointer d, @Const FloatPointer e, @Const FloatPointer df,
@Const FloatPointer ef, @Const FloatPointer b, int ldb,
FloatPointer x, int ldx, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_sptrfs( int matrix_layout, int n, int nrhs,
@Const FloatBuffer d, @Const FloatBuffer e, @Const FloatBuffer df,
@Const FloatBuffer ef, @Const FloatBuffer b, int ldb,
FloatBuffer x, int ldx, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_sptrfs( int matrix_layout, int n, int nrhs,
@Const float[] d, @Const float[] e, @Const float[] df,
@Const float[] ef, @Const float[] b, int ldb,
float[] x, int ldx, float[] ferr, float[] berr );
public static native int LAPACKE_dptrfs( int matrix_layout, int n, int nrhs,
@Const DoublePointer d, @Const DoublePointer e, @Const DoublePointer df,
@Const DoublePointer ef, @Const DoublePointer b, int ldb,
DoublePointer x, int ldx, DoublePointer ferr,
DoublePointer berr );
public static native int LAPACKE_dptrfs( int matrix_layout, int n, int nrhs,
@Const DoubleBuffer d, @Const DoubleBuffer e, @Const DoubleBuffer df,
@Const DoubleBuffer ef, @Const DoubleBuffer b, int ldb,
DoubleBuffer x, int ldx, DoubleBuffer ferr,
DoubleBuffer berr );
public static native int LAPACKE_dptrfs( int matrix_layout, int n, int nrhs,
@Const double[] d, @Const double[] e, @Const double[] df,
@Const double[] ef, @Const double[] b, int ldb,
double[] x, int ldx, double[] ferr,
double[] berr );
public static native int LAPACKE_cptrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, @Const FloatPointer df,
@Cast("const lapack_complex_float*") FloatPointer ef,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_cptrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, @Const FloatBuffer df,
@Cast("const lapack_complex_float*") FloatBuffer ef,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_cptrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, @Const float[] df,
@Cast("const lapack_complex_float*") float[] ef,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zptrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e, @Const DoublePointer df,
@Cast("const lapack_complex_double*") DoublePointer ef,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zptrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e, @Const DoubleBuffer df,
@Cast("const lapack_complex_double*") DoubleBuffer ef,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zptrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] d,
@Cast("const lapack_complex_double*") double[] e, @Const double[] df,
@Cast("const lapack_complex_double*") double[] ef,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_sptsv( int matrix_layout, int n, int nrhs,
FloatPointer d, FloatPointer e, FloatPointer b, int ldb );
public static native int LAPACKE_sptsv( int matrix_layout, int n, int nrhs,
FloatBuffer d, FloatBuffer e, FloatBuffer b, int ldb );
public static native int LAPACKE_sptsv( int matrix_layout, int n, int nrhs,
float[] d, float[] e, float[] b, int ldb );
public static native int LAPACKE_dptsv( int matrix_layout, int n, int nrhs,
DoublePointer d, DoublePointer e, DoublePointer b, int ldb );
public static native int LAPACKE_dptsv( int matrix_layout, int n, int nrhs,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer b, int ldb );
public static native int LAPACKE_dptsv( int matrix_layout, int n, int nrhs,
double[] d, double[] e, double[] b, int ldb );
public static native int LAPACKE_cptsv( int matrix_layout, int n, int nrhs,
FloatPointer d, @Cast("lapack_complex_float*") FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cptsv( int matrix_layout, int n, int nrhs,
FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cptsv( int matrix_layout, int n, int nrhs,
float[] d, @Cast("lapack_complex_float*") float[] e,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zptsv( int matrix_layout, int n, int nrhs,
DoublePointer d, @Cast("lapack_complex_double*") DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zptsv( int matrix_layout, int n, int nrhs,
DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zptsv( int matrix_layout, int n, int nrhs,
double[] d, @Cast("lapack_complex_double*") double[] e,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const FloatPointer d, @Const FloatPointer e,
FloatPointer df, FloatPointer ef, @Const FloatPointer b, int ldb,
FloatPointer x, int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_sptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const FloatBuffer d, @Const FloatBuffer e,
FloatBuffer df, FloatBuffer ef, @Const FloatBuffer b, int ldb,
FloatBuffer x, int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_sptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const float[] d, @Const float[] e,
float[] df, float[] ef, @Const float[] b, int ldb,
float[] x, int ldx, float[] rcond, float[] ferr,
float[] berr );
public static native int LAPACKE_dptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const DoublePointer d, @Const DoublePointer e,
DoublePointer df, DoublePointer ef, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const DoubleBuffer d, @Const DoubleBuffer e,
DoubleBuffer df, DoubleBuffer ef, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const double[] d, @Const double[] e,
double[] df, double[] ef, @Const double[] b,
int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_cptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, FloatPointer df,
@Cast("lapack_complex_float*") FloatPointer ef,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_cptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, FloatBuffer df,
@Cast("lapack_complex_float*") FloatBuffer ef,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_cptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, float[] df,
@Cast("lapack_complex_float*") float[] ef,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr );
public static native int LAPACKE_zptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e, DoublePointer df,
@Cast("lapack_complex_double*") DoublePointer ef,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e, DoubleBuffer df,
@Cast("lapack_complex_double*") DoubleBuffer ef,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zptsvx( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const double[] d,
@Cast("const lapack_complex_double*") double[] e, double[] df,
@Cast("lapack_complex_double*") double[] ef,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_spttrf( int n, FloatPointer d, FloatPointer e );
public static native int LAPACKE_spttrf( int n, FloatBuffer d, FloatBuffer e );
public static native int LAPACKE_spttrf( int n, float[] d, float[] e );
public static native int LAPACKE_dpttrf( int n, DoublePointer d, DoublePointer e );
public static native int LAPACKE_dpttrf( int n, DoubleBuffer d, DoubleBuffer e );
public static native int LAPACKE_dpttrf( int n, double[] d, double[] e );
public static native int LAPACKE_cpttrf( int n, FloatPointer d, @Cast("lapack_complex_float*") FloatPointer e );
public static native int LAPACKE_cpttrf( int n, FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer e );
public static native int LAPACKE_cpttrf( int n, float[] d, @Cast("lapack_complex_float*") float[] e );
public static native int LAPACKE_zpttrf( int n, DoublePointer d, @Cast("lapack_complex_double*") DoublePointer e );
public static native int LAPACKE_zpttrf( int n, DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer e );
public static native int LAPACKE_zpttrf( int n, double[] d, @Cast("lapack_complex_double*") double[] e );
public static native int LAPACKE_spttrs( int matrix_layout, int n, int nrhs,
@Const FloatPointer d, @Const FloatPointer e, FloatPointer b,
int ldb );
public static native int LAPACKE_spttrs( int matrix_layout, int n, int nrhs,
@Const FloatBuffer d, @Const FloatBuffer e, FloatBuffer b,
int ldb );
public static native int LAPACKE_spttrs( int matrix_layout, int n, int nrhs,
@Const float[] d, @Const float[] e, float[] b,
int ldb );
public static native int LAPACKE_dpttrs( int matrix_layout, int n, int nrhs,
@Const DoublePointer d, @Const DoublePointer e, DoublePointer b,
int ldb );
public static native int LAPACKE_dpttrs( int matrix_layout, int n, int nrhs,
@Const DoubleBuffer d, @Const DoubleBuffer e, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dpttrs( int matrix_layout, int n, int nrhs,
@Const double[] d, @Const double[] e, double[] b,
int ldb );
public static native int LAPACKE_cpttrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpttrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpttrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpttrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zpttrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zpttrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] d,
@Cast("const lapack_complex_double*") double[] e,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_ssbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, FloatPointer ab, int ldab, FloatPointer w,
FloatPointer z, int ldz );
public static native int LAPACKE_ssbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, FloatBuffer ab, int ldab, FloatBuffer w,
FloatBuffer z, int ldz );
public static native int LAPACKE_ssbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, float[] ab, int ldab, float[] w,
float[] z, int ldz );
public static native int LAPACKE_dsbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, DoublePointer ab, int ldab, DoublePointer w,
DoublePointer z, int ldz );
public static native int LAPACKE_dsbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, DoubleBuffer ab, int ldab, DoubleBuffer w,
DoubleBuffer z, int ldz );
public static native int LAPACKE_dsbev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, double[] ab, int ldab, double[] w,
double[] z, int ldz );
public static native int LAPACKE_ssbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, FloatPointer ab, int ldab, FloatPointer w,
FloatPointer z, int ldz );
public static native int LAPACKE_ssbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, FloatBuffer ab, int ldab, FloatBuffer w,
FloatBuffer z, int ldz );
public static native int LAPACKE_ssbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, float[] ab, int ldab, float[] w,
float[] z, int ldz );
public static native int LAPACKE_dsbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, DoublePointer ab, int ldab,
DoublePointer w, DoublePointer z, int ldz );
public static native int LAPACKE_dsbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, DoubleBuffer ab, int ldab,
DoubleBuffer w, DoubleBuffer z, int ldz );
public static native int LAPACKE_dsbevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int kd, double[] ab, int ldab,
double[] w, double[] z, int ldz );
public static native int LAPACKE_ssbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd, FloatPointer ab,
int ldab, FloatPointer q, int ldq, float vl,
float vu, int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_ssbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd, FloatBuffer ab,
int ldab, FloatBuffer q, int ldq, float vl,
float vu, int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_ssbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd, float[] ab,
int ldab, float[] q, int ldq, float vl,
float vu, int il, int iu, float abstol,
int[] m, float[] w, float[] z, int ldz,
int[] ifail );
public static native int LAPACKE_dsbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd, DoublePointer ab,
int ldab, DoublePointer q, int ldq,
double vl, double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w, DoublePointer z,
int ldz, IntPointer ifail );
public static native int LAPACKE_dsbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd, DoubleBuffer ab,
int ldab, DoubleBuffer q, int ldq,
double vl, double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, IntBuffer ifail );
public static native int LAPACKE_dsbevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int kd, double[] ab,
int ldab, double[] q, int ldq,
double vl, double vu, int il, int iu,
double abstol, int[] m, double[] w, double[] z,
int ldz, int[] ifail );
public static native int LAPACKE_ssbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb, FloatPointer ab,
int ldab, @Const FloatPointer bb, int ldbb,
FloatPointer x, int ldx );
public static native int LAPACKE_ssbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb, FloatBuffer ab,
int ldab, @Const FloatBuffer bb, int ldbb,
FloatBuffer x, int ldx );
public static native int LAPACKE_ssbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb, float[] ab,
int ldab, @Const float[] bb, int ldbb,
float[] x, int ldx );
public static native int LAPACKE_dsbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb, DoublePointer ab,
int ldab, @Const DoublePointer bb, int ldbb,
DoublePointer x, int ldx );
public static native int LAPACKE_dsbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb, DoubleBuffer ab,
int ldab, @Const DoubleBuffer bb, int ldbb,
DoubleBuffer x, int ldx );
public static native int LAPACKE_dsbgst( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int ka, int kb, double[] ab,
int ldab, @Const double[] bb, int ldbb,
double[] x, int ldx );
public static native int LAPACKE_ssbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, FloatPointer ab,
int ldab, FloatPointer bb, int ldbb, FloatPointer w,
FloatPointer z, int ldz );
public static native int LAPACKE_ssbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, FloatBuffer ab,
int ldab, FloatBuffer bb, int ldbb, FloatBuffer w,
FloatBuffer z, int ldz );
public static native int LAPACKE_ssbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, float[] ab,
int ldab, float[] bb, int ldbb, float[] w,
float[] z, int ldz );
public static native int LAPACKE_dsbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, DoublePointer ab,
int ldab, DoublePointer bb, int ldbb,
DoublePointer w, DoublePointer z, int ldz );
public static native int LAPACKE_dsbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, DoubleBuffer ab,
int ldab, DoubleBuffer bb, int ldbb,
DoubleBuffer w, DoubleBuffer z, int ldz );
public static native int LAPACKE_dsbgv( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, double[] ab,
int ldab, double[] bb, int ldbb,
double[] w, double[] z, int ldz );
public static native int LAPACKE_ssbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, FloatPointer ab,
int ldab, FloatPointer bb, int ldbb,
FloatPointer w, FloatPointer z, int ldz );
public static native int LAPACKE_ssbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, FloatBuffer ab,
int ldab, FloatBuffer bb, int ldbb,
FloatBuffer w, FloatBuffer z, int ldz );
public static native int LAPACKE_ssbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, float[] ab,
int ldab, float[] bb, int ldbb,
float[] w, float[] z, int ldz );
public static native int LAPACKE_dsbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, DoublePointer ab,
int ldab, DoublePointer bb, int ldbb,
DoublePointer w, DoublePointer z, int ldz );
public static native int LAPACKE_dsbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, DoubleBuffer ab,
int ldab, DoubleBuffer bb, int ldbb,
DoubleBuffer w, DoubleBuffer z, int ldz );
public static native int LAPACKE_dsbgvd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
int ka, int kb, double[] ab,
int ldab, double[] bb, int ldbb,
double[] w, double[] z, int ldz );
public static native int LAPACKE_ssbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
FloatPointer ab, int ldab, FloatPointer bb,
int ldbb, FloatPointer q, int ldq, float vl,
float vu, int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_ssbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
FloatBuffer ab, int ldab, FloatBuffer bb,
int ldbb, FloatBuffer q, int ldq, float vl,
float vu, int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_ssbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
float[] ab, int ldab, float[] bb,
int ldbb, float[] q, int ldq, float vl,
float vu, int il, int iu, float abstol,
int[] m, float[] w, float[] z, int ldz,
int[] ifail );
public static native int LAPACKE_dsbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
DoublePointer ab, int ldab, DoublePointer bb,
int ldbb, DoublePointer q, int ldq,
double vl, double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w, DoublePointer z,
int ldz, IntPointer ifail );
public static native int LAPACKE_dsbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
DoubleBuffer ab, int ldab, DoubleBuffer bb,
int ldbb, DoubleBuffer q, int ldq,
double vl, double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, IntBuffer ifail );
public static native int LAPACKE_dsbgvx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, int ka, int kb,
double[] ab, int ldab, double[] bb,
int ldbb, double[] q, int ldq,
double vl, double vu, int il, int iu,
double abstol, int[] m, double[] w, double[] z,
int ldz, int[] ifail );
public static native int LAPACKE_ssbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, FloatPointer ab, int ldab, FloatPointer d,
FloatPointer e, FloatPointer q, int ldq );
public static native int LAPACKE_ssbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, FloatBuffer ab, int ldab, FloatBuffer d,
FloatBuffer e, FloatBuffer q, int ldq );
public static native int LAPACKE_ssbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, float[] ab, int ldab, float[] d,
float[] e, float[] q, int ldq );
public static native int LAPACKE_dsbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, DoublePointer ab, int ldab,
DoublePointer d, DoublePointer e, DoublePointer q, int ldq );
public static native int LAPACKE_dsbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, DoubleBuffer ab, int ldab,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer q, int ldq );
public static native int LAPACKE_dsbtrd( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo, int n,
int kd, double[] ab, int ldab,
double[] d, double[] e, double[] q, int ldq );
public static native int LAPACKE_ssfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, float alpha,
@Const FloatPointer a, int lda, float beta,
FloatPointer c );
public static native int LAPACKE_ssfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, float alpha,
@Const FloatBuffer a, int lda, float beta,
FloatBuffer c );
public static native int LAPACKE_ssfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, float alpha,
@Const float[] a, int lda, float beta,
float[] c );
public static native int LAPACKE_dsfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, double alpha,
@Const DoublePointer a, int lda, double beta,
DoublePointer c );
public static native int LAPACKE_dsfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, double alpha,
@Const DoubleBuffer a, int lda, double beta,
DoubleBuffer c );
public static native int LAPACKE_dsfrk( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte trans,
int n, int k, double alpha,
@Const double[] a, int lda, double beta,
double[] c );
public static native int LAPACKE_sspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, @Const IntPointer ipiv, float anorm,
FloatPointer rcond );
public static native int LAPACKE_sspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, @Const IntBuffer ipiv, float anorm,
FloatBuffer rcond );
public static native int LAPACKE_sspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, @Const int[] ipiv, float anorm,
float[] rcond );
public static native int LAPACKE_dspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, @Const IntPointer ipiv,
double anorm, DoublePointer rcond );
public static native int LAPACKE_dspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, @Const IntBuffer ipiv,
double anorm, DoubleBuffer rcond );
public static native int LAPACKE_dspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, @Const int[] ipiv,
double anorm, double[] rcond );
public static native int LAPACKE_cspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, float anorm, FloatPointer rcond );
public static native int LAPACKE_cspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, float anorm, FloatBuffer rcond );
public static native int LAPACKE_cspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, float anorm, float[] rcond );
public static native int LAPACKE_zspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond );
public static native int LAPACKE_zspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_zspcon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv, double anorm,
double[] rcond );
public static native int LAPACKE_sspev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
FloatPointer ap, FloatPointer w, FloatPointer z, int ldz );
public static native int LAPACKE_sspev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
FloatBuffer ap, FloatBuffer w, FloatBuffer z, int ldz );
public static native int LAPACKE_sspev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
float[] ap, float[] w, float[] z, int ldz );
public static native int LAPACKE_dspev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
DoublePointer ap, DoublePointer w, DoublePointer z, int ldz );
public static native int LAPACKE_dspev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
DoubleBuffer ap, DoubleBuffer w, DoubleBuffer z, int ldz );
public static native int LAPACKE_dspev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
double[] ap, double[] w, double[] z, int ldz );
public static native int LAPACKE_sspevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
FloatPointer ap, FloatPointer w, FloatPointer z, int ldz );
public static native int LAPACKE_sspevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
FloatBuffer ap, FloatBuffer w, FloatBuffer z, int ldz );
public static native int LAPACKE_sspevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
float[] ap, float[] w, float[] z, int ldz );
public static native int LAPACKE_dspevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
DoublePointer ap, DoublePointer w, DoublePointer z, int ldz );
public static native int LAPACKE_dspevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
DoubleBuffer ap, DoubleBuffer w, DoubleBuffer z, int ldz );
public static native int LAPACKE_dspevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
double[] ap, double[] w, double[] z, int ldz );
public static native int LAPACKE_sspevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, FloatPointer ap, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_sspevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, FloatBuffer ap, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_sspevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, float[] ap, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w, float[] z, int ldz,
int[] ifail );
public static native int LAPACKE_dspevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, DoublePointer ap, double vl, double vu,
int il, int iu, double abstol,
IntPointer m, DoublePointer w, DoublePointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_dspevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, DoubleBuffer ap, double vl, double vu,
int il, int iu, double abstol,
IntBuffer m, DoubleBuffer w, DoubleBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_dspevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, double[] ap, double vl, double vu,
int il, int iu, double abstol,
int[] m, double[] w, double[] z, int ldz,
int[] ifail );
public static native int LAPACKE_sspgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, FloatPointer ap, @Const FloatPointer bp );
public static native int LAPACKE_sspgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, FloatBuffer ap, @Const FloatBuffer bp );
public static native int LAPACKE_sspgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, float[] ap, @Const float[] bp );
public static native int LAPACKE_dspgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, DoublePointer ap, @Const DoublePointer bp );
public static native int LAPACKE_dspgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, DoubleBuffer ap, @Const DoubleBuffer bp );
public static native int LAPACKE_dspgst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, double[] ap, @Const double[] bp );
public static native int LAPACKE_sspgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatPointer ap, FloatPointer bp,
FloatPointer w, FloatPointer z, int ldz );
public static native int LAPACKE_sspgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatBuffer ap, FloatBuffer bp,
FloatBuffer w, FloatBuffer z, int ldz );
public static native int LAPACKE_sspgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, float[] ap, float[] bp,
float[] w, float[] z, int ldz );
public static native int LAPACKE_dspgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoublePointer ap, DoublePointer bp,
DoublePointer w, DoublePointer z, int ldz );
public static native int LAPACKE_dspgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoubleBuffer ap, DoubleBuffer bp,
DoubleBuffer w, DoubleBuffer z, int ldz );
public static native int LAPACKE_dspgv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, double[] ap, double[] bp,
double[] w, double[] z, int ldz );
public static native int LAPACKE_sspgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatPointer ap, FloatPointer bp,
FloatPointer w, FloatPointer z, int ldz );
public static native int LAPACKE_sspgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatBuffer ap, FloatBuffer bp,
FloatBuffer w, FloatBuffer z, int ldz );
public static native int LAPACKE_sspgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, float[] ap, float[] bp,
float[] w, float[] z, int ldz );
public static native int LAPACKE_dspgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoublePointer ap, DoublePointer bp,
DoublePointer w, DoublePointer z, int ldz );
public static native int LAPACKE_dspgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoubleBuffer ap, DoubleBuffer bp,
DoubleBuffer w, DoubleBuffer z, int ldz );
public static native int LAPACKE_dspgvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, double[] ap, double[] bp,
double[] w, double[] z, int ldz );
public static native int LAPACKE_sspgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, FloatPointer ap,
FloatPointer bp, float vl, float vu, int il,
int iu, float abstol, IntPointer m, FloatPointer w,
FloatPointer z, int ldz, IntPointer ifail );
public static native int LAPACKE_sspgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, FloatBuffer ap,
FloatBuffer bp, float vl, float vu, int il,
int iu, float abstol, IntBuffer m, FloatBuffer w,
FloatBuffer z, int ldz, IntBuffer ifail );
public static native int LAPACKE_sspgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, float[] ap,
float[] bp, float vl, float vu, int il,
int iu, float abstol, int[] m, float[] w,
float[] z, int ldz, int[] ifail );
public static native int LAPACKE_dspgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, DoublePointer ap,
DoublePointer bp, double vl, double vu, int il,
int iu, double abstol, IntPointer m,
DoublePointer w, DoublePointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_dspgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, DoubleBuffer ap,
DoubleBuffer bp, double vl, double vu, int il,
int iu, double abstol, IntBuffer m,
DoubleBuffer w, DoubleBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_dspgvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, double[] ap,
double[] bp, double vl, double vu, int il,
int iu, double abstol, int[] m,
double[] w, double[] z, int ldz,
int[] ifail );
public static native int LAPACKE_ssprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer ap, @Const FloatPointer afp,
@Const IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_ssprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer ap, @Const FloatBuffer afp,
@Const IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_ssprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] ap, @Const float[] afp,
@Const int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr );
public static native int LAPACKE_dsprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer ap, @Const DoublePointer afp,
@Const IntPointer ipiv, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dsprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer ap, @Const DoubleBuffer afp,
@Const IntBuffer ipiv, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dsprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] ap, @Const double[] afp,
@Const int[] ipiv, @Const double[] b,
int ldb, double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_csprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer afp,
@Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_csprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer afp,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_csprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] afp,
@Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zsprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer afp,
@Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zsprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer afp,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zsprfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] afp,
@Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_sspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer ap, IntPointer ipiv,
FloatPointer b, int ldb );
public static native int LAPACKE_sspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer ap, IntBuffer ipiv,
FloatBuffer b, int ldb );
public static native int LAPACKE_sspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] ap, int[] ipiv,
float[] b, int ldb );
public static native int LAPACKE_dspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer ap, IntPointer ipiv,
DoublePointer b, int ldb );
public static native int LAPACKE_dspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer ap, IntBuffer ipiv,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] ap, int[] ipiv,
double[] b, int ldb );
public static native int LAPACKE_cspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] ap,
int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zspsv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] ap,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_sspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer ap, FloatPointer afp,
IntPointer ipiv, @Const FloatPointer b, int ldb,
FloatPointer x, int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_sspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer ap, FloatBuffer afp,
IntBuffer ipiv, @Const FloatBuffer b, int ldb,
FloatBuffer x, int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_sspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] ap, float[] afp,
int[] ipiv, @Const float[] b, int ldb,
float[] x, int ldx, float[] rcond, float[] ferr,
float[] berr );
public static native int LAPACKE_dspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer ap, DoublePointer afp,
IntPointer ipiv, @Const DoublePointer b, int ldb,
DoublePointer x, int ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer ap, DoubleBuffer afp,
IntBuffer ipiv, @Const DoubleBuffer b, int ldb,
DoubleBuffer x, int ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] ap, double[] afp,
int[] ipiv, @Const double[] b, int ldb,
double[] x, int ldx, double[] rcond,
double[] ferr, double[] berr );
public static native int LAPACKE_cspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer afp, IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_cspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer afp, IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_cspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] afp, int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr );
public static native int LAPACKE_zspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer afp, IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer afp, IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zspsvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] afp, int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_ssptrd( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer ap,
FloatPointer d, FloatPointer e, FloatPointer tau );
public static native int LAPACKE_ssptrd( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer ap,
FloatBuffer d, FloatBuffer e, FloatBuffer tau );
public static native int LAPACKE_ssptrd( int matrix_layout, @Cast("char") byte uplo, int n, float[] ap,
float[] d, float[] e, float[] tau );
public static native int LAPACKE_dsptrd( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap, DoublePointer d, DoublePointer e, DoublePointer tau );
public static native int LAPACKE_dsptrd( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap, DoubleBuffer d, DoubleBuffer e, DoubleBuffer tau );
public static native int LAPACKE_dsptrd( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap, double[] d, double[] e, double[] tau );
public static native int LAPACKE_ssptrf( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer ap,
IntPointer ipiv );
public static native int LAPACKE_ssptrf( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer ap,
IntBuffer ipiv );
public static native int LAPACKE_ssptrf( int matrix_layout, @Cast("char") byte uplo, int n, float[] ap,
int[] ipiv );
public static native int LAPACKE_dsptrf( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap, IntPointer ipiv );
public static native int LAPACKE_dsptrf( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_dsptrf( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap, int[] ipiv );
public static native int LAPACKE_csptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, IntPointer ipiv );
public static native int LAPACKE_csptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_csptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, int[] ipiv );
public static native int LAPACKE_zsptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, IntPointer ipiv );
public static native int LAPACKE_zsptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_zsptrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, int[] ipiv );
public static native int LAPACKE_ssptri( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer ap,
@Const IntPointer ipiv );
public static native int LAPACKE_ssptri( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer ap,
@Const IntBuffer ipiv );
public static native int LAPACKE_ssptri( int matrix_layout, @Cast("char") byte uplo, int n, float[] ap,
@Const int[] ipiv );
public static native int LAPACKE_dsptri( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap, @Const IntPointer ipiv );
public static native int LAPACKE_dsptri( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap, @Const IntBuffer ipiv );
public static native int LAPACKE_dsptri( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap, @Const int[] ipiv );
public static native int LAPACKE_csptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, @Const IntPointer ipiv );
public static native int LAPACKE_csptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, @Const IntBuffer ipiv );
public static native int LAPACKE_csptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, @Const int[] ipiv );
public static native int LAPACKE_zsptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, @Const IntPointer ipiv );
public static native int LAPACKE_zsptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, @Const IntBuffer ipiv );
public static native int LAPACKE_zsptri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, @Const int[] ipiv );
public static native int LAPACKE_ssptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer ap,
@Const IntPointer ipiv, FloatPointer b, int ldb );
public static native int LAPACKE_ssptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer ap,
@Const IntBuffer ipiv, FloatBuffer b, int ldb );
public static native int LAPACKE_ssptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] ap,
@Const int[] ipiv, float[] b, int ldb );
public static native int LAPACKE_dsptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer ap,
@Const IntPointer ipiv, DoublePointer b, int ldb );
public static native int LAPACKE_dsptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer ap,
@Const IntBuffer ipiv, DoubleBuffer b, int ldb );
public static native int LAPACKE_dsptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] ap,
@Const int[] ipiv, double[] b, int ldb );
public static native int LAPACKE_csptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_csptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_csptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zsptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zsptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zsptrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_sstebz( @Cast("char") byte range, @Cast("char") byte order, int n, float vl,
float vu, int il, int iu, float abstol,
@Const FloatPointer d, @Const FloatPointer e, IntPointer m,
IntPointer nsplit, FloatPointer w, IntPointer iblock,
IntPointer isplit );
public static native int LAPACKE_sstebz( @Cast("char") byte range, @Cast("char") byte order, int n, float vl,
float vu, int il, int iu, float abstol,
@Const FloatBuffer d, @Const FloatBuffer e, IntBuffer m,
IntBuffer nsplit, FloatBuffer w, IntBuffer iblock,
IntBuffer isplit );
public static native int LAPACKE_sstebz( @Cast("char") byte range, @Cast("char") byte order, int n, float vl,
float vu, int il, int iu, float abstol,
@Const float[] d, @Const float[] e, int[] m,
int[] nsplit, float[] w, int[] iblock,
int[] isplit );
public static native int LAPACKE_dstebz( @Cast("char") byte range, @Cast("char") byte order, int n, double vl,
double vu, int il, int iu,
double abstol, @Const DoublePointer d, @Const DoublePointer e,
IntPointer m, IntPointer nsplit, DoublePointer w,
IntPointer iblock, IntPointer isplit );
public static native int LAPACKE_dstebz( @Cast("char") byte range, @Cast("char") byte order, int n, double vl,
double vu, int il, int iu,
double abstol, @Const DoubleBuffer d, @Const DoubleBuffer e,
IntBuffer m, IntBuffer nsplit, DoubleBuffer w,
IntBuffer iblock, IntBuffer isplit );
public static native int LAPACKE_dstebz( @Cast("char") byte range, @Cast("char") byte order, int n, double vl,
double vu, int il, int iu,
double abstol, @Const double[] d, @Const double[] e,
int[] m, int[] nsplit, double[] w,
int[] iblock, int[] isplit );
public static native int LAPACKE_sstedc( int matrix_layout, @Cast("char") byte compz, int n, FloatPointer d,
FloatPointer e, FloatPointer z, int ldz );
public static native int LAPACKE_sstedc( int matrix_layout, @Cast("char") byte compz, int n, FloatBuffer d,
FloatBuffer e, FloatBuffer z, int ldz );
public static native int LAPACKE_sstedc( int matrix_layout, @Cast("char") byte compz, int n, float[] d,
float[] e, float[] z, int ldz );
public static native int LAPACKE_dstedc( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, DoublePointer z, int ldz );
public static native int LAPACKE_dstedc( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer z, int ldz );
public static native int LAPACKE_dstedc( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, double[] z, int ldz );
public static native int LAPACKE_cstedc( int matrix_layout, @Cast("char") byte compz, int n, FloatPointer d,
FloatPointer e, @Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_cstedc( int matrix_layout, @Cast("char") byte compz, int n, FloatBuffer d,
FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_cstedc( int matrix_layout, @Cast("char") byte compz, int n, float[] d,
float[] e, @Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zstedc( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer z,
int ldz );
public static native int LAPACKE_zstedc( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz );
public static native int LAPACKE_zstedc( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] z,
int ldz );
public static native int LAPACKE_sstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z, int ldz,
IntPointer isuppz );
public static native int LAPACKE_sstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, int ldz,
IntBuffer isuppz );
public static native int LAPACKE_sstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w, float[] z, int ldz,
int[] isuppz );
public static native int LAPACKE_dstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w, DoublePointer z,
int ldz, IntPointer isuppz );
public static native int LAPACKE_dstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, IntBuffer isuppz );
public static native int LAPACKE_dstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w, double[] z,
int ldz, int[] isuppz );
public static native int LAPACKE_cstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, IntPointer isuppz );
public static native int LAPACKE_cstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, IntBuffer isuppz );
public static native int LAPACKE_cstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, int[] isuppz );
public static native int LAPACKE_zstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer isuppz );
public static native int LAPACKE_zstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer isuppz );
public static native int LAPACKE_zstegr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] isuppz );
public static native int LAPACKE_sstein( int matrix_layout, int n, @Const FloatPointer d,
@Const FloatPointer e, int m, @Const FloatPointer w,
@Const IntPointer iblock, @Const IntPointer isplit,
FloatPointer z, int ldz, IntPointer ifailv );
public static native int LAPACKE_sstein( int matrix_layout, int n, @Const FloatBuffer d,
@Const FloatBuffer e, int m, @Const FloatBuffer w,
@Const IntBuffer iblock, @Const IntBuffer isplit,
FloatBuffer z, int ldz, IntBuffer ifailv );
public static native int LAPACKE_sstein( int matrix_layout, int n, @Const float[] d,
@Const float[] e, int m, @Const float[] w,
@Const int[] iblock, @Const int[] isplit,
float[] z, int ldz, int[] ifailv );
public static native int LAPACKE_dstein( int matrix_layout, int n, @Const DoublePointer d,
@Const DoublePointer e, int m, @Const DoublePointer w,
@Const IntPointer iblock, @Const IntPointer isplit,
DoublePointer z, int ldz, IntPointer ifailv );
public static native int LAPACKE_dstein( int matrix_layout, int n, @Const DoubleBuffer d,
@Const DoubleBuffer e, int m, @Const DoubleBuffer w,
@Const IntBuffer iblock, @Const IntBuffer isplit,
DoubleBuffer z, int ldz, IntBuffer ifailv );
public static native int LAPACKE_dstein( int matrix_layout, int n, @Const double[] d,
@Const double[] e, int m, @Const double[] w,
@Const int[] iblock, @Const int[] isplit,
double[] z, int ldz, int[] ifailv );
public static native int LAPACKE_cstein( int matrix_layout, int n, @Const FloatPointer d,
@Const FloatPointer e, int m, @Const FloatPointer w,
@Const IntPointer iblock, @Const IntPointer isplit,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
IntPointer ifailv );
public static native int LAPACKE_cstein( int matrix_layout, int n, @Const FloatBuffer d,
@Const FloatBuffer e, int m, @Const FloatBuffer w,
@Const IntBuffer iblock, @Const IntBuffer isplit,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
IntBuffer ifailv );
public static native int LAPACKE_cstein( int matrix_layout, int n, @Const float[] d,
@Const float[] e, int m, @Const float[] w,
@Const int[] iblock, @Const int[] isplit,
@Cast("lapack_complex_float*") float[] z, int ldz,
int[] ifailv );
public static native int LAPACKE_zstein( int matrix_layout, int n, @Const DoublePointer d,
@Const DoublePointer e, int m, @Const DoublePointer w,
@Const IntPointer iblock, @Const IntPointer isplit,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer ifailv );
public static native int LAPACKE_zstein( int matrix_layout, int n, @Const DoubleBuffer d,
@Const DoubleBuffer e, int m, @Const DoubleBuffer w,
@Const IntBuffer iblock, @Const IntBuffer isplit,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer ifailv );
public static native int LAPACKE_zstein( int matrix_layout, int n, @Const double[] d,
@Const double[] e, int m, @Const double[] w,
@Const int[] iblock, @Const int[] isplit,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] ifailv );
public static native int LAPACKE_sstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl, float vu,
int il, int iu, IntPointer m,
FloatPointer w, FloatPointer z, int ldz, int nzc,
IntPointer isuppz, IntPointer tryrac );
public static native int LAPACKE_sstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl, float vu,
int il, int iu, IntBuffer m,
FloatBuffer w, FloatBuffer z, int ldz, int nzc,
IntBuffer isuppz, IntBuffer tryrac );
public static native int LAPACKE_sstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl, float vu,
int il, int iu, int[] m,
float[] w, float[] z, int ldz, int nzc,
int[] isuppz, int[] tryrac );
public static native int LAPACKE_dstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
IntPointer m, DoublePointer w, DoublePointer z, int ldz,
int nzc, IntPointer isuppz,
IntPointer tryrac );
public static native int LAPACKE_dstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
IntBuffer m, DoubleBuffer w, DoubleBuffer z, int ldz,
int nzc, IntBuffer isuppz,
IntBuffer tryrac );
public static native int LAPACKE_dstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
int[] m, double[] w, double[] z, int ldz,
int nzc, int[] isuppz,
int[] tryrac );
public static native int LAPACKE_cstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl, float vu,
int il, int iu, IntPointer m,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z, int ldz,
int nzc, IntPointer isuppz,
IntPointer tryrac );
public static native int LAPACKE_cstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl, float vu,
int il, int iu, IntBuffer m,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z, int ldz,
int nzc, IntBuffer isuppz,
IntBuffer tryrac );
public static native int LAPACKE_cstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl, float vu,
int il, int iu, int[] m,
float[] w, @Cast("lapack_complex_float*") float[] z, int ldz,
int nzc, int[] isuppz,
int[] tryrac );
public static native int LAPACKE_zstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
IntPointer m, DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, int nzc, IntPointer isuppz,
IntPointer tryrac );
public static native int LAPACKE_zstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
IntBuffer m, DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, int nzc, IntBuffer isuppz,
IntBuffer tryrac );
public static native int LAPACKE_zstemr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
int[] m, double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, int nzc, int[] isuppz,
int[] tryrac );
public static native int LAPACKE_ssteqr( int matrix_layout, @Cast("char") byte compz, int n, FloatPointer d,
FloatPointer e, FloatPointer z, int ldz );
public static native int LAPACKE_ssteqr( int matrix_layout, @Cast("char") byte compz, int n, FloatBuffer d,
FloatBuffer e, FloatBuffer z, int ldz );
public static native int LAPACKE_ssteqr( int matrix_layout, @Cast("char") byte compz, int n, float[] d,
float[] e, float[] z, int ldz );
public static native int LAPACKE_dsteqr( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, DoublePointer z, int ldz );
public static native int LAPACKE_dsteqr( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer z, int ldz );
public static native int LAPACKE_dsteqr( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, double[] z, int ldz );
public static native int LAPACKE_csteqr( int matrix_layout, @Cast("char") byte compz, int n, FloatPointer d,
FloatPointer e, @Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_csteqr( int matrix_layout, @Cast("char") byte compz, int n, FloatBuffer d,
FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_csteqr( int matrix_layout, @Cast("char") byte compz, int n, float[] d,
float[] e, @Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zsteqr( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer z,
int ldz );
public static native int LAPACKE_zsteqr( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz );
public static native int LAPACKE_zsteqr( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] z,
int ldz );
public static native int LAPACKE_ssterf( int n, FloatPointer d, FloatPointer e );
public static native int LAPACKE_ssterf( int n, FloatBuffer d, FloatBuffer e );
public static native int LAPACKE_ssterf( int n, float[] d, float[] e );
public static native int LAPACKE_dsterf( int n, DoublePointer d, DoublePointer e );
public static native int LAPACKE_dsterf( int n, DoubleBuffer d, DoubleBuffer e );
public static native int LAPACKE_dsterf( int n, double[] d, double[] e );
public static native int LAPACKE_sstev( int matrix_layout, @Cast("char") byte jobz, int n, FloatPointer d,
FloatPointer e, FloatPointer z, int ldz );
public static native int LAPACKE_sstev( int matrix_layout, @Cast("char") byte jobz, int n, FloatBuffer d,
FloatBuffer e, FloatBuffer z, int ldz );
public static native int LAPACKE_sstev( int matrix_layout, @Cast("char") byte jobz, int n, float[] d,
float[] e, float[] z, int ldz );
public static native int LAPACKE_dstev( int matrix_layout, @Cast("char") byte jobz, int n, DoublePointer d,
DoublePointer e, DoublePointer z, int ldz );
public static native int LAPACKE_dstev( int matrix_layout, @Cast("char") byte jobz, int n, DoubleBuffer d,
DoubleBuffer e, DoubleBuffer z, int ldz );
public static native int LAPACKE_dstev( int matrix_layout, @Cast("char") byte jobz, int n, double[] d,
double[] e, double[] z, int ldz );
public static native int LAPACKE_sstevd( int matrix_layout, @Cast("char") byte jobz, int n, FloatPointer d,
FloatPointer e, FloatPointer z, int ldz );
public static native int LAPACKE_sstevd( int matrix_layout, @Cast("char") byte jobz, int n, FloatBuffer d,
FloatBuffer e, FloatBuffer z, int ldz );
public static native int LAPACKE_sstevd( int matrix_layout, @Cast("char") byte jobz, int n, float[] d,
float[] e, float[] z, int ldz );
public static native int LAPACKE_dstevd( int matrix_layout, @Cast("char") byte jobz, int n, DoublePointer d,
DoublePointer e, DoublePointer z, int ldz );
public static native int LAPACKE_dstevd( int matrix_layout, @Cast("char") byte jobz, int n, DoubleBuffer d,
DoubleBuffer e, DoubleBuffer z, int ldz );
public static native int LAPACKE_dstevd( int matrix_layout, @Cast("char") byte jobz, int n, double[] d,
double[] e, double[] z, int ldz );
public static native int LAPACKE_sstevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z, int ldz,
IntPointer isuppz );
public static native int LAPACKE_sstevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, int ldz,
IntBuffer isuppz );
public static native int LAPACKE_sstevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w, float[] z, int ldz,
int[] isuppz );
public static native int LAPACKE_dstevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w, DoublePointer z,
int ldz, IntPointer isuppz );
public static native int LAPACKE_dstevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, IntBuffer isuppz );
public static native int LAPACKE_dstevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w, double[] z,
int ldz, int[] isuppz );
public static native int LAPACKE_sstevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_sstevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_sstevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w, float[] z, int ldz,
int[] ifail );
public static native int LAPACKE_dstevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w, DoublePointer z,
int ldz, IntPointer ifail );
public static native int LAPACKE_dstevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, IntBuffer ifail );
public static native int LAPACKE_dstevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w, double[] z,
int ldz, int[] ifail );
public static native int LAPACKE_ssycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer a, int lda,
@Const IntPointer ipiv, float anorm, FloatPointer rcond );
public static native int LAPACKE_ssycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer a, int lda,
@Const IntBuffer ipiv, float anorm, FloatBuffer rcond );
public static native int LAPACKE_ssycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] a, int lda,
@Const int[] ipiv, float anorm, float[] rcond );
public static native int LAPACKE_dsycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer a, int lda,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond );
public static native int LAPACKE_dsycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer a, int lda,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_dsycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] a, int lda,
@Const int[] ipiv, double anorm,
double[] rcond );
public static native int LAPACKE_csycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv, float anorm, FloatPointer rcond );
public static native int LAPACKE_csycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv, float anorm, FloatBuffer rcond );
public static native int LAPACKE_csycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv, float anorm, float[] rcond );
public static native int LAPACKE_zsycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond );
public static native int LAPACKE_zsycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond );
public static native int LAPACKE_zsycon( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv, double anorm,
double[] rcond );
public static native int LAPACKE_ssyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer a, int lda, FloatPointer s,
FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_ssyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer a, int lda, FloatBuffer s,
FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_ssyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] a, int lda, float[] s,
float[] scond, float[] amax );
public static native int LAPACKE_dsyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer a, int lda, DoublePointer s,
DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_dsyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer s,
DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_dsyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] a, int lda, double[] s,
double[] scond, double[] amax );
public static native int LAPACKE_csyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer s, FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_csyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_csyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] s, float[] scond, float[] amax );
public static native int LAPACKE_zsyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer s, DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_zsyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_zsyequb( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] s, double[] scond, double[] amax );
public static native int LAPACKE_ssyev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
FloatPointer a, int lda, FloatPointer w );
public static native int LAPACKE_ssyev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda, FloatBuffer w );
public static native int LAPACKE_ssyev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
float[] a, int lda, float[] w );
public static native int LAPACKE_dsyev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
DoublePointer a, int lda, DoublePointer w );
public static native int LAPACKE_dsyev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda, DoubleBuffer w );
public static native int LAPACKE_dsyev( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
double[] a, int lda, double[] w );
public static native int LAPACKE_ssyevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
FloatPointer a, int lda, FloatPointer w );
public static native int LAPACKE_ssyevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda, FloatBuffer w );
public static native int LAPACKE_ssyevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
float[] a, int lda, float[] w );
public static native int LAPACKE_dsyevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
DoublePointer a, int lda, DoublePointer w );
public static native int LAPACKE_dsyevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda, DoubleBuffer w );
public static native int LAPACKE_dsyevd( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo, int n,
double[] a, int lda, double[] w );
public static native int LAPACKE_ssyevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, FloatPointer a, int lda, float vl,
float vu, int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z, int ldz,
IntPointer isuppz );
public static native int LAPACKE_ssyevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, FloatBuffer a, int lda, float vl,
float vu, int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, int ldz,
IntBuffer isuppz );
public static native int LAPACKE_ssyevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, float[] a, int lda, float vl,
float vu, int il, int iu, float abstol,
int[] m, float[] w, float[] z, int ldz,
int[] isuppz );
public static native int LAPACKE_dsyevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, DoublePointer a, int lda, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w, DoublePointer z,
int ldz, IntPointer isuppz );
public static native int LAPACKE_dsyevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, DoubleBuffer a, int lda, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, IntBuffer isuppz );
public static native int LAPACKE_dsyevr( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, double[] a, int lda, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w, double[] z,
int ldz, int[] isuppz );
public static native int LAPACKE_ssyevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, FloatPointer a, int lda, float vl,
float vu, int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_ssyevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, FloatBuffer a, int lda, float vl,
float vu, int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_ssyevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, float[] a, int lda, float vl,
float vu, int il, int iu, float abstol,
int[] m, float[] w, float[] z, int ldz,
int[] ifail );
public static native int LAPACKE_dsyevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, DoublePointer a, int lda, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w, DoublePointer z,
int ldz, IntPointer ifail );
public static native int LAPACKE_dsyevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, DoubleBuffer a, int lda, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, IntBuffer ifail );
public static native int LAPACKE_dsyevx( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range, @Cast("char") byte uplo,
int n, double[] a, int lda, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w, double[] z,
int ldz, int[] ifail );
public static native int LAPACKE_ssygst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, FloatPointer a, int lda,
@Const FloatPointer b, int ldb );
public static native int LAPACKE_ssygst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, FloatBuffer a, int lda,
@Const FloatBuffer b, int ldb );
public static native int LAPACKE_ssygst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, float[] a, int lda,
@Const float[] b, int ldb );
public static native int LAPACKE_dsygst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, DoublePointer a, int lda,
@Const DoublePointer b, int ldb );
public static native int LAPACKE_dsygst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, DoubleBuffer a, int lda,
@Const DoubleBuffer b, int ldb );
public static native int LAPACKE_dsygst( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, double[] a, int lda,
@Const double[] b, int ldb );
public static native int LAPACKE_ssygv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatPointer a, int lda,
FloatPointer b, int ldb, FloatPointer w );
public static native int LAPACKE_ssygv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatBuffer a, int lda,
FloatBuffer b, int ldb, FloatBuffer w );
public static native int LAPACKE_ssygv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, float[] a, int lda,
float[] b, int ldb, float[] w );
public static native int LAPACKE_dsygv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer w );
public static native int LAPACKE_dsygv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer w );
public static native int LAPACKE_dsygv( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, double[] a, int lda,
double[] b, int ldb, double[] w );
public static native int LAPACKE_ssygvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatPointer a, int lda,
FloatPointer b, int ldb, FloatPointer w );
public static native int LAPACKE_ssygvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatBuffer a, int lda,
FloatBuffer b, int ldb, FloatBuffer w );
public static native int LAPACKE_ssygvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, float[] a, int lda,
float[] b, int ldb, float[] w );
public static native int LAPACKE_dsygvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer w );
public static native int LAPACKE_dsygvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer w );
public static native int LAPACKE_dsygvd( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, double[] a, int lda,
double[] b, int ldb, double[] w );
public static native int LAPACKE_ssygvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, FloatPointer a,
int lda, FloatPointer b, int ldb, float vl,
float vu, int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z, int ldz,
IntPointer ifail );
public static native int LAPACKE_ssygvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb, float vl,
float vu, int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, int ldz,
IntBuffer ifail );
public static native int LAPACKE_ssygvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, float[] a,
int lda, float[] b, int ldb, float vl,
float vu, int il, int iu, float abstol,
int[] m, float[] w, float[] z, int ldz,
int[] ifail );
public static native int LAPACKE_dsygvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, DoublePointer a,
int lda, DoublePointer b, int ldb, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w, DoublePointer z,
int ldz, IntPointer ifail );
public static native int LAPACKE_dsygvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, IntBuffer ifail );
public static native int LAPACKE_dsygvx( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, double[] a,
int lda, double[] b, int ldb, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w, double[] z,
int ldz, int[] ifail );
public static native int LAPACKE_ssyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const FloatPointer af, int ldaf,
@Const IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_ssyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const FloatBuffer af, int ldaf,
@Const IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_ssyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
@Const float[] af, int ldaf,
@Const int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr );
public static native int LAPACKE_dsyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a, int lda,
@Const DoublePointer af, int ldaf,
@Const IntPointer ipiv, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dsyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a, int lda,
@Const DoubleBuffer af, int ldaf,
@Const IntBuffer ipiv, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dsyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a, int lda,
@Const double[] af, int ldaf,
@Const int[] ipiv, @Const double[] b,
int ldb, double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_csyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_csyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_csyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_zsyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zsyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zsyrfs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_ssysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer a, int lda,
IntPointer ipiv, FloatPointer b, int ldb );
public static native int LAPACKE_ssysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer a, int lda,
IntBuffer ipiv, FloatBuffer b, int ldb );
public static native int LAPACKE_ssysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] a, int lda,
int[] ipiv, float[] b, int ldb );
public static native int LAPACKE_dsysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer a, int lda,
IntPointer ipiv, DoublePointer b, int ldb );
public static native int LAPACKE_dsysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer a, int lda,
IntBuffer ipiv, DoubleBuffer b, int ldb );
public static native int LAPACKE_dsysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] a, int lda,
int[] ipiv, double[] b, int ldb );
public static native int LAPACKE_csysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_csysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_csysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zsysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zsysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zsysv( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_ssysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
FloatPointer af, int ldaf, IntPointer ipiv,
@Const FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_ssysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
FloatBuffer af, int ldaf, IntBuffer ipiv,
@Const FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_ssysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
float[] af, int ldaf, int[] ipiv,
@Const float[] b, int ldb, float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr );
public static native int LAPACKE_dsysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a, int lda,
DoublePointer af, int ldaf, IntPointer ipiv,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr );
public static native int LAPACKE_dsysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a, int lda,
DoubleBuffer af, int ldaf, IntBuffer ipiv,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr );
public static native int LAPACKE_dsysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a, int lda,
double[] af, int ldaf, int[] ipiv,
@Const double[] b, int ldb, double[] x,
int ldx, double[] rcond, double[] ferr,
double[] berr );
public static native int LAPACKE_csysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer af,
int ldaf, IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_csysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer af,
int ldaf, IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_csysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] af,
int ldaf, int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr );
public static native int LAPACKE_zsysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer af,
int ldaf, IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_zsysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer af,
int ldaf, IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_zsysvx( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] af,
int ldaf, int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr );
public static native int LAPACKE_ssytrd( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda, FloatPointer d, FloatPointer e, FloatPointer tau );
public static native int LAPACKE_ssytrd( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda, FloatBuffer d, FloatBuffer e, FloatBuffer tau );
public static native int LAPACKE_ssytrd( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda, float[] d, float[] e, float[] tau );
public static native int LAPACKE_dsytrd( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda, DoublePointer d, DoublePointer e, DoublePointer tau );
public static native int LAPACKE_dsytrd( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, DoubleBuffer d, DoubleBuffer e, DoubleBuffer tau );
public static native int LAPACKE_dsytrd( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda, double[] d, double[] e, double[] tau );
public static native int LAPACKE_ssytrf( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda, IntPointer ipiv );
public static native int LAPACKE_ssytrf( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda, IntBuffer ipiv );
public static native int LAPACKE_ssytrf( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda, int[] ipiv );
public static native int LAPACKE_dsytrf( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda, IntPointer ipiv );
public static native int LAPACKE_dsytrf( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, IntBuffer ipiv );
public static native int LAPACKE_dsytrf( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda, int[] ipiv );
public static native int LAPACKE_csytrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_csytrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_csytrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zsytrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zsytrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zsytrf( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_ssytri( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda, @Const IntPointer ipiv );
public static native int LAPACKE_ssytri( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda, @Const IntBuffer ipiv );
public static native int LAPACKE_ssytri( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda, @Const int[] ipiv );
public static native int LAPACKE_dsytri( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda, @Const IntPointer ipiv );
public static native int LAPACKE_dsytri( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, @Const IntBuffer ipiv );
public static native int LAPACKE_dsytri( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda, @Const int[] ipiv );
public static native int LAPACKE_csytri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_csytri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_csytri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_zsytri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_zsytri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_zsytri( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_ssytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer b, int ldb );
public static native int LAPACKE_ssytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer b, int ldb );
public static native int LAPACKE_ssytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
@Const int[] ipiv, float[] b, int ldb );
public static native int LAPACKE_dsytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a, int lda,
@Const IntPointer ipiv, DoublePointer b, int ldb );
public static native int LAPACKE_dsytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a, int lda,
@Const IntBuffer ipiv, DoubleBuffer b, int ldb );
public static native int LAPACKE_dsytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a, int lda,
@Const int[] ipiv, double[] b, int ldb );
public static native int LAPACKE_csytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_csytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_csytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zsytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zsytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zsytrs( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_stbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd, @Const FloatPointer ab,
int ldab, FloatPointer rcond );
public static native int LAPACKE_stbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd, @Const FloatBuffer ab,
int ldab, FloatBuffer rcond );
public static native int LAPACKE_stbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd, @Const float[] ab,
int ldab, float[] rcond );
public static native int LAPACKE_dtbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd, @Const DoublePointer ab,
int ldab, DoublePointer rcond );
public static native int LAPACKE_dtbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd, @Const DoubleBuffer ab,
int ldab, DoubleBuffer rcond );
public static native int LAPACKE_dtbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd, @Const double[] ab,
int ldab, double[] rcond );
public static native int LAPACKE_ctbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
FloatPointer rcond );
public static native int LAPACKE_ctbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
FloatBuffer rcond );
public static native int LAPACKE_ctbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
float[] rcond );
public static native int LAPACKE_ztbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
DoublePointer rcond );
public static native int LAPACKE_ztbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
DoubleBuffer rcond );
public static native int LAPACKE_ztbcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
double[] rcond );
public static native int LAPACKE_stbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const FloatPointer ab, int ldab, @Const FloatPointer b,
int ldb, @Const FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_stbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const FloatBuffer ab, int ldab, @Const FloatBuffer b,
int ldb, @Const FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_stbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const float[] ab, int ldab, @Const float[] b,
int ldb, @Const float[] x, int ldx,
float[] ferr, float[] berr );
public static native int LAPACKE_dtbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const DoublePointer ab, int ldab, @Const DoublePointer b,
int ldb, @Const DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dtbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const DoubleBuffer ab, int ldab, @Const DoubleBuffer b,
int ldb, @Const DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dtbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const double[] ab, int ldab, @Const double[] b,
int ldb, @Const double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_ctbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("const lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_ctbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("const lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_ctbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("const lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr );
public static native int LAPACKE_ztbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("const lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_ztbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("const lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_ztbrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("const lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_stbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const FloatPointer ab, int ldab, FloatPointer b,
int ldb );
public static native int LAPACKE_stbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const FloatBuffer ab, int ldab, FloatBuffer b,
int ldb );
public static native int LAPACKE_stbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const float[] ab, int ldab, float[] b,
int ldb );
public static native int LAPACKE_dtbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const DoublePointer ab, int ldab, DoublePointer b,
int ldb );
public static native int LAPACKE_dtbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const DoubleBuffer ab, int ldab, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dtbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Const double[] ab, int ldab, double[] b,
int ldb );
public static native int LAPACKE_ctbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_ctbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_ctbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_ztbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_ztbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_ztbtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int kd, int nrhs,
@Cast("const lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_stfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
float alpha, @Const FloatPointer a, FloatPointer b,
int ldb );
public static native int LAPACKE_stfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
float alpha, @Const FloatBuffer a, FloatBuffer b,
int ldb );
public static native int LAPACKE_stfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
float alpha, @Const float[] a, float[] b,
int ldb );
public static native int LAPACKE_dtfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
double alpha, @Const DoublePointer a, DoublePointer b,
int ldb );
public static native int LAPACKE_dtfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
double alpha, @Const DoubleBuffer a, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dtfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
double alpha, @Const double[] a, double[] b,
int ldb );
public static native int LAPACKE_ctfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
@ByVal @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("const lapack_complex_float*") FloatPointer a,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_ctfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
@ByVal @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("const lapack_complex_float*") FloatBuffer a,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_ctfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
@ByVal @Cast("lapack_complex_float*") float[] alpha,
@Cast("const lapack_complex_float*") float[] a,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_ztfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
@ByVal @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("const lapack_complex_double*") DoublePointer a,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_ztfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
@ByVal @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("const lapack_complex_double*") DoubleBuffer a,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_ztfsm( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, @Cast("char") byte diag, int m, int n,
@ByVal @Cast("lapack_complex_double*") double[] alpha,
@Cast("const lapack_complex_double*") double[] a,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_stftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, FloatPointer a );
public static native int LAPACKE_stftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, FloatBuffer a );
public static native int LAPACKE_stftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, float[] a );
public static native int LAPACKE_dtftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, DoublePointer a );
public static native int LAPACKE_dtftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, DoubleBuffer a );
public static native int LAPACKE_dtftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, double[] a );
public static native int LAPACKE_ctftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_float*") FloatPointer a );
public static native int LAPACKE_ctftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_float*") FloatBuffer a );
public static native int LAPACKE_ctftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_float*") float[] a );
public static native int LAPACKE_ztftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_double*") DoublePointer a );
public static native int LAPACKE_ztftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_double*") DoubleBuffer a );
public static native int LAPACKE_ztftri( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_double*") double[] a );
public static native int LAPACKE_stfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatPointer arf, FloatPointer ap );
public static native int LAPACKE_stfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatBuffer arf, FloatBuffer ap );
public static native int LAPACKE_stfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const float[] arf, float[] ap );
public static native int LAPACKE_dtfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoublePointer arf, DoublePointer ap );
public static native int LAPACKE_dtfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoubleBuffer arf, DoubleBuffer ap );
public static native int LAPACKE_dtfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const double[] arf, double[] ap );
public static native int LAPACKE_ctfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer arf,
@Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_ctfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer arf,
@Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_ctfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] arf,
@Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_ztfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer arf,
@Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_ztfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer arf,
@Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_ztfttp( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] arf,
@Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_stfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatPointer arf, FloatPointer a,
int lda );
public static native int LAPACKE_stfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatBuffer arf, FloatBuffer a,
int lda );
public static native int LAPACKE_stfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const float[] arf, float[] a,
int lda );
public static native int LAPACKE_dtfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoublePointer arf, DoublePointer a,
int lda );
public static native int LAPACKE_dtfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoubleBuffer arf, DoubleBuffer a,
int lda );
public static native int LAPACKE_dtfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const double[] arf, double[] a,
int lda );
public static native int LAPACKE_ctfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer arf,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_ctfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer arf,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_ctfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] arf,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_ztfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer arf,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_ztfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer arf,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_ztfttr( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] arf,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_stgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const FloatPointer s, int lds, @Const FloatPointer p,
int ldp, FloatPointer vl, int ldvl,
FloatPointer vr, int ldvr, int mm,
IntPointer m );
public static native int LAPACKE_stgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const FloatBuffer s, int lds, @Const FloatBuffer p,
int ldp, FloatBuffer vl, int ldvl,
FloatBuffer vr, int ldvr, int mm,
IntBuffer m );
public static native int LAPACKE_stgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const float[] s, int lds, @Const float[] p,
int ldp, float[] vl, int ldvl,
float[] vr, int ldvr, int mm,
int[] m );
public static native int LAPACKE_dtgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const DoublePointer s, int lds, @Const DoublePointer p,
int ldp, DoublePointer vl, int ldvl,
DoublePointer vr, int ldvr, int mm,
IntPointer m );
public static native int LAPACKE_dtgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const DoubleBuffer s, int lds, @Const DoubleBuffer p,
int ldp, DoubleBuffer vl, int ldvl,
DoubleBuffer vr, int ldvr, int mm,
IntBuffer m );
public static native int LAPACKE_dtgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const double[] s, int lds, @Const double[] p,
int ldp, double[] vl, int ldvl,
double[] vr, int ldvr, int mm,
int[] m );
public static native int LAPACKE_ctgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_float*") FloatPointer s, int lds,
@Cast("const lapack_complex_float*") FloatPointer p, int ldp,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
int mm, IntPointer m );
public static native int LAPACKE_ctgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_float*") FloatBuffer s, int lds,
@Cast("const lapack_complex_float*") FloatBuffer p, int ldp,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
int mm, IntBuffer m );
public static native int LAPACKE_ctgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_float*") float[] s, int lds,
@Cast("const lapack_complex_float*") float[] p, int ldp,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
int mm, int[] m );
public static native int LAPACKE_ztgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_double*") DoublePointer s, int lds,
@Cast("const lapack_complex_double*") DoublePointer p, int ldp,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
int mm, IntPointer m );
public static native int LAPACKE_ztgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_double*") DoubleBuffer s, int lds,
@Cast("const lapack_complex_double*") DoubleBuffer p, int ldp,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
int mm, IntBuffer m );
public static native int LAPACKE_ztgevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_double*") double[] s, int lds,
@Cast("const lapack_complex_double*") double[] p, int ldp,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
int mm, int[] m );
public static native int LAPACKE_stgexc( int matrix_layout, int wantq,
int wantz, int n, FloatPointer a,
int lda, FloatPointer b, int ldb, FloatPointer q,
int ldq, FloatPointer z, int ldz,
IntPointer ifst, IntPointer ilst );
public static native int LAPACKE_stgexc( int matrix_layout, int wantq,
int wantz, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb, FloatBuffer q,
int ldq, FloatBuffer z, int ldz,
IntBuffer ifst, IntBuffer ilst );
public static native int LAPACKE_stgexc( int matrix_layout, int wantq,
int wantz, int n, float[] a,
int lda, float[] b, int ldb, float[] q,
int ldq, float[] z, int ldz,
int[] ifst, int[] ilst );
public static native int LAPACKE_dtgexc( int matrix_layout, int wantq,
int wantz, int n, DoublePointer a,
int lda, DoublePointer b, int ldb, DoublePointer q,
int ldq, DoublePointer z, int ldz,
IntPointer ifst, IntPointer ilst );
public static native int LAPACKE_dtgexc( int matrix_layout, int wantq,
int wantz, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb, DoubleBuffer q,
int ldq, DoubleBuffer z, int ldz,
IntBuffer ifst, IntBuffer ilst );
public static native int LAPACKE_dtgexc( int matrix_layout, int wantq,
int wantz, int n, double[] a,
int lda, double[] b, int ldb, double[] q,
int ldq, double[] z, int ldz,
int[] ifst, int[] ilst );
public static native int LAPACKE_ctgexc( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ctgexc( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ctgexc( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ztgexc( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ztgexc( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ztgexc( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_stgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntPointer select, int n, FloatPointer a,
int lda, FloatPointer b, int ldb,
FloatPointer alphar, FloatPointer alphai, FloatPointer beta, FloatPointer q,
int ldq, FloatPointer z, int ldz,
IntPointer m, FloatPointer pl, FloatPointer pr, FloatPointer dif );
public static native int LAPACKE_stgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntBuffer select, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta, FloatBuffer q,
int ldq, FloatBuffer z, int ldz,
IntBuffer m, FloatBuffer pl, FloatBuffer pr, FloatBuffer dif );
public static native int LAPACKE_stgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const int[] select, int n, float[] a,
int lda, float[] b, int ldb,
float[] alphar, float[] alphai, float[] beta, float[] q,
int ldq, float[] z, int ldz,
int[] m, float[] pl, float[] pr, float[] dif );
public static native int LAPACKE_dtgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntPointer select, int n,
DoublePointer a, int lda, DoublePointer b, int ldb,
DoublePointer alphar, DoublePointer alphai, DoublePointer beta,
DoublePointer q, int ldq, DoublePointer z, int ldz,
IntPointer m, DoublePointer pl, DoublePointer pr, DoublePointer dif );
public static native int LAPACKE_dtgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntBuffer select, int n,
DoubleBuffer a, int lda, DoubleBuffer b, int ldb,
DoubleBuffer alphar, DoubleBuffer alphai, DoubleBuffer beta,
DoubleBuffer q, int ldq, DoubleBuffer z, int ldz,
IntBuffer m, DoubleBuffer pl, DoubleBuffer pr, DoubleBuffer dif );
public static native int LAPACKE_dtgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const int[] select, int n,
double[] a, int lda, double[] b, int ldb,
double[] alphar, double[] alphai, double[] beta,
double[] q, int ldq, double[] z, int ldz,
int[] m, double[] pl, double[] pr, double[] dif );
public static native int LAPACKE_ctgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntPointer select, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer q,
int ldq, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, IntPointer m, FloatPointer pl, FloatPointer pr,
FloatPointer dif );
public static native int LAPACKE_ctgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntBuffer select, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer q,
int ldq, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, IntBuffer m, FloatBuffer pl, FloatBuffer pr,
FloatBuffer dif );
public static native int LAPACKE_ctgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const int[] select, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta, @Cast("lapack_complex_float*") float[] q,
int ldq, @Cast("lapack_complex_float*") float[] z,
int ldz, int[] m, float[] pl, float[] pr,
float[] dif );
public static native int LAPACKE_ztgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntPointer select, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer m, DoublePointer pl, DoublePointer pr, DoublePointer dif );
public static native int LAPACKE_ztgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntBuffer select, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer m, DoubleBuffer pl, DoubleBuffer pr, DoubleBuffer dif );
public static native int LAPACKE_ztgsen( int matrix_layout, int ijob,
int wantq, int wantz,
@Const int[] select, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] m, double[] pl, double[] pr, double[] dif );
public static native int LAPACKE_stgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, FloatPointer a, int lda,
FloatPointer b, int ldb, float tola, float tolb,
FloatPointer alpha, FloatPointer beta, FloatPointer u, int ldu,
FloatPointer v, int ldv, FloatPointer q, int ldq,
IntPointer ncycle );
public static native int LAPACKE_stgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, FloatBuffer a, int lda,
FloatBuffer b, int ldb, float tola, float tolb,
FloatBuffer alpha, FloatBuffer beta, FloatBuffer u, int ldu,
FloatBuffer v, int ldv, FloatBuffer q, int ldq,
IntBuffer ncycle );
public static native int LAPACKE_stgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, float[] a, int lda,
float[] b, int ldb, float tola, float tolb,
float[] alpha, float[] beta, float[] u, int ldu,
float[] v, int ldv, float[] q, int ldq,
int[] ncycle );
public static native int LAPACKE_dtgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, DoublePointer a,
int lda, DoublePointer b, int ldb,
double tola, double tolb, DoublePointer alpha,
DoublePointer beta, DoublePointer u, int ldu, DoublePointer v,
int ldv, DoublePointer q, int ldq,
IntPointer ncycle );
public static native int LAPACKE_dtgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
double tola, double tolb, DoubleBuffer alpha,
DoubleBuffer beta, DoubleBuffer u, int ldu, DoubleBuffer v,
int ldv, DoubleBuffer q, int ldq,
IntBuffer ncycle );
public static native int LAPACKE_dtgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, double[] a,
int lda, double[] b, int ldb,
double tola, double tolb, double[] alpha,
double[] beta, double[] u, int ldu, double[] v,
int ldv, double[] q, int ldq,
int[] ncycle );
public static native int LAPACKE_ctgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, float tola, float tolb, FloatPointer alpha,
FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer u, int ldu,
@Cast("lapack_complex_float*") FloatPointer v, int ldv,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
IntPointer ncycle );
public static native int LAPACKE_ctgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, float tola, float tolb, FloatBuffer alpha,
FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer u, int ldu,
@Cast("lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
IntBuffer ncycle );
public static native int LAPACKE_ctgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, float tola, float tolb, float[] alpha,
float[] beta, @Cast("lapack_complex_float*") float[] u, int ldu,
@Cast("lapack_complex_float*") float[] v, int ldv,
@Cast("lapack_complex_float*") float[] q, int ldq,
int[] ncycle );
public static native int LAPACKE_ztgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, double tola, double tolb,
DoublePointer alpha, DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer u, int ldu,
@Cast("lapack_complex_double*") DoublePointer v, int ldv,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
IntPointer ncycle );
public static native int LAPACKE_ztgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, double tola, double tolb,
DoubleBuffer alpha, DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer u, int ldu,
@Cast("lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
IntBuffer ncycle );
public static native int LAPACKE_ztgsja( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv, @Cast("char") byte jobq,
int m, int p, int n,
int k, int l, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, double tola, double tolb,
double[] alpha, double[] beta,
@Cast("lapack_complex_double*") double[] u, int ldu,
@Cast("lapack_complex_double*") double[] v, int ldv,
@Cast("lapack_complex_double*") double[] q, int ldq,
int[] ncycle );
public static native int LAPACKE_stgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const FloatPointer a, int lda, @Const FloatPointer b,
int ldb, @Const FloatPointer vl, int ldvl,
@Const FloatPointer vr, int ldvr, FloatPointer s,
FloatPointer dif, int mm, IntPointer m );
public static native int LAPACKE_stgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const FloatBuffer a, int lda, @Const FloatBuffer b,
int ldb, @Const FloatBuffer vl, int ldvl,
@Const FloatBuffer vr, int ldvr, FloatBuffer s,
FloatBuffer dif, int mm, IntBuffer m );
public static native int LAPACKE_stgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const float[] a, int lda, @Const float[] b,
int ldb, @Const float[] vl, int ldvl,
@Const float[] vr, int ldvr, float[] s,
float[] dif, int mm, int[] m );
public static native int LAPACKE_dtgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const DoublePointer a, int lda, @Const DoublePointer b,
int ldb, @Const DoublePointer vl, int ldvl,
@Const DoublePointer vr, int ldvr, DoublePointer s,
DoublePointer dif, int mm, IntPointer m );
public static native int LAPACKE_dtgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const DoubleBuffer a, int lda, @Const DoubleBuffer b,
int ldb, @Const DoubleBuffer vl, int ldvl,
@Const DoubleBuffer vr, int ldvr, DoubleBuffer s,
DoubleBuffer dif, int mm, IntBuffer m );
public static native int LAPACKE_dtgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const double[] a, int lda, @Const double[] b,
int ldb, @Const double[] vl, int ldvl,
@Const double[] vr, int ldvr, double[] s,
double[] dif, int mm, int[] m );
public static native int LAPACKE_ctgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("const lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("const lapack_complex_float*") FloatPointer vr, int ldvr,
FloatPointer s, FloatPointer dif, int mm, IntPointer m );
public static native int LAPACKE_ctgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("const lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("const lapack_complex_float*") FloatBuffer vr, int ldvr,
FloatBuffer s, FloatBuffer dif, int mm, IntBuffer m );
public static native int LAPACKE_ctgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("const lapack_complex_float*") float[] vl, int ldvl,
@Cast("const lapack_complex_float*") float[] vr, int ldvr,
float[] s, float[] dif, int mm, int[] m );
public static native int LAPACKE_ztgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("const lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("const lapack_complex_double*") DoublePointer vr, int ldvr,
DoublePointer s, DoublePointer dif, int mm,
IntPointer m );
public static native int LAPACKE_ztgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("const lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("const lapack_complex_double*") DoubleBuffer vr, int ldvr,
DoubleBuffer s, DoubleBuffer dif, int mm,
IntBuffer m );
public static native int LAPACKE_ztgsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("const lapack_complex_double*") double[] vl, int ldvl,
@Cast("const lapack_complex_double*") double[] vr, int ldvr,
double[] s, double[] dif, int mm,
int[] m );
public static native int LAPACKE_stgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const FloatPointer a,
int lda, @Const FloatPointer b, int ldb,
FloatPointer c, int ldc, @Const FloatPointer d,
int ldd, @Const FloatPointer e, int lde,
FloatPointer f, int ldf, FloatPointer scale, FloatPointer dif );
public static native int LAPACKE_stgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const FloatBuffer a,
int lda, @Const FloatBuffer b, int ldb,
FloatBuffer c, int ldc, @Const FloatBuffer d,
int ldd, @Const FloatBuffer e, int lde,
FloatBuffer f, int ldf, FloatBuffer scale, FloatBuffer dif );
public static native int LAPACKE_stgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const float[] a,
int lda, @Const float[] b, int ldb,
float[] c, int ldc, @Const float[] d,
int ldd, @Const float[] e, int lde,
float[] f, int ldf, float[] scale, float[] dif );
public static native int LAPACKE_dtgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const DoublePointer a,
int lda, @Const DoublePointer b, int ldb,
DoublePointer c, int ldc, @Const DoublePointer d,
int ldd, @Const DoublePointer e, int lde,
DoublePointer f, int ldf, DoublePointer scale,
DoublePointer dif );
public static native int LAPACKE_dtgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const DoubleBuffer a,
int lda, @Const DoubleBuffer b, int ldb,
DoubleBuffer c, int ldc, @Const DoubleBuffer d,
int ldd, @Const DoubleBuffer e, int lde,
DoubleBuffer f, int ldf, DoubleBuffer scale,
DoubleBuffer dif );
public static native int LAPACKE_dtgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const double[] a,
int lda, @Const double[] b, int ldb,
double[] c, int ldc, @Const double[] d,
int ldd, @Const double[] e, int lde,
double[] f, int ldf, double[] scale,
double[] dif );
public static native int LAPACKE_ctgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("const lapack_complex_float*") FloatPointer d, int ldd,
@Cast("const lapack_complex_float*") FloatPointer e, int lde,
@Cast("lapack_complex_float*") FloatPointer f, int ldf,
FloatPointer scale, FloatPointer dif );
public static native int LAPACKE_ctgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("const lapack_complex_float*") FloatBuffer d, int ldd,
@Cast("const lapack_complex_float*") FloatBuffer e, int lde,
@Cast("lapack_complex_float*") FloatBuffer f, int ldf,
FloatBuffer scale, FloatBuffer dif );
public static native int LAPACKE_ctgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("const lapack_complex_float*") float[] d, int ldd,
@Cast("const lapack_complex_float*") float[] e, int lde,
@Cast("lapack_complex_float*") float[] f, int ldf,
float[] scale, float[] dif );
public static native int LAPACKE_ztgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("const lapack_complex_double*") DoublePointer d, int ldd,
@Cast("const lapack_complex_double*") DoublePointer e, int lde,
@Cast("lapack_complex_double*") DoublePointer f, int ldf,
DoublePointer scale, DoublePointer dif );
public static native int LAPACKE_ztgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("const lapack_complex_double*") DoubleBuffer d, int ldd,
@Cast("const lapack_complex_double*") DoubleBuffer e, int lde,
@Cast("lapack_complex_double*") DoubleBuffer f, int ldf,
DoubleBuffer scale, DoubleBuffer dif );
public static native int LAPACKE_ztgsyl( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("const lapack_complex_double*") double[] d, int ldd,
@Cast("const lapack_complex_double*") double[] e, int lde,
@Cast("lapack_complex_double*") double[] f, int ldf,
double[] scale, double[] dif );
public static native int LAPACKE_stpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const FloatPointer ap, FloatPointer rcond );
public static native int LAPACKE_stpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const FloatBuffer ap, FloatBuffer rcond );
public static native int LAPACKE_stpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const float[] ap, float[] rcond );
public static native int LAPACKE_dtpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const DoublePointer ap, DoublePointer rcond );
public static native int LAPACKE_dtpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const DoubleBuffer ap, DoubleBuffer rcond );
public static native int LAPACKE_dtpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const double[] ap, double[] rcond );
public static native int LAPACKE_ctpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") FloatPointer ap,
FloatPointer rcond );
public static native int LAPACKE_ctpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") FloatBuffer ap,
FloatBuffer rcond );
public static native int LAPACKE_ctpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") float[] ap,
float[] rcond );
public static native int LAPACKE_ztpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") DoublePointer ap,
DoublePointer rcond );
public static native int LAPACKE_ztpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
DoubleBuffer rcond );
public static native int LAPACKE_ztpcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") double[] ap,
double[] rcond );
public static native int LAPACKE_stprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const FloatPointer ap,
@Const FloatPointer b, int ldb, @Const FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_stprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const FloatBuffer ap,
@Const FloatBuffer b, int ldb, @Const FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_stprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const float[] ap,
@Const float[] b, int ldb, @Const float[] x,
int ldx, float[] ferr, float[] berr );
public static native int LAPACKE_dtprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const DoublePointer ap,
@Const DoublePointer b, int ldb, @Const DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_dtprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const DoubleBuffer ap,
@Const DoubleBuffer b, int ldb, @Const DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_dtprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const double[] ap,
@Const double[] b, int ldb, @Const double[] x,
int ldx, double[] ferr, double[] berr );
public static native int LAPACKE_ctprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("const lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_ctprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("const lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_ctprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("const lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr );
public static native int LAPACKE_ztprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("const lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_ztprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("const lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_ztprfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("const lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_stptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
FloatPointer ap );
public static native int LAPACKE_stptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
FloatBuffer ap );
public static native int LAPACKE_stptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
float[] ap );
public static native int LAPACKE_dtptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
DoublePointer ap );
public static native int LAPACKE_dtptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
DoubleBuffer ap );
public static native int LAPACKE_dtptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
double[] ap );
public static native int LAPACKE_ctptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_ctptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_ctptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_ztptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_ztptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_ztptri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_stptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const FloatPointer ap,
FloatPointer b, int ldb );
public static native int LAPACKE_stptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const FloatBuffer ap,
FloatBuffer b, int ldb );
public static native int LAPACKE_stptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const float[] ap,
float[] b, int ldb );
public static native int LAPACKE_dtptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const DoublePointer ap,
DoublePointer b, int ldb );
public static native int LAPACKE_dtptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const DoubleBuffer ap,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dtptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const double[] ap,
double[] b, int ldb );
public static native int LAPACKE_ctptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_ctptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_ctptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_ztptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_ztptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_ztptrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_stpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatPointer ap, FloatPointer arf );
public static native int LAPACKE_stpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatBuffer ap, FloatBuffer arf );
public static native int LAPACKE_stpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const float[] ap, float[] arf );
public static native int LAPACKE_dtpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoublePointer ap, DoublePointer arf );
public static native int LAPACKE_dtpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoubleBuffer ap, DoubleBuffer arf );
public static native int LAPACKE_dtpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const double[] ap, double[] arf );
public static native int LAPACKE_ctpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer arf );
public static native int LAPACKE_ctpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer arf );
public static native int LAPACKE_ctpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] arf );
public static native int LAPACKE_ztpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer arf );
public static native int LAPACKE_ztpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer arf );
public static native int LAPACKE_ztpttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] arf );
public static native int LAPACKE_stpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, FloatPointer a, int lda );
public static native int LAPACKE_stpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, FloatBuffer a, int lda );
public static native int LAPACKE_stpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, float[] a, int lda );
public static native int LAPACKE_dtpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, DoublePointer a, int lda );
public static native int LAPACKE_dtpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, DoubleBuffer a, int lda );
public static native int LAPACKE_dtpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, double[] a, int lda );
public static native int LAPACKE_ctpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_ctpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_ctpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_ztpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_ztpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_ztpttr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_strcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const FloatPointer a, int lda,
FloatPointer rcond );
public static native int LAPACKE_strcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const FloatBuffer a, int lda,
FloatBuffer rcond );
public static native int LAPACKE_strcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const float[] a, int lda,
float[] rcond );
public static native int LAPACKE_dtrcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const DoublePointer a, int lda,
DoublePointer rcond );
public static native int LAPACKE_dtrcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const DoubleBuffer a, int lda,
DoubleBuffer rcond );
public static native int LAPACKE_dtrcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const double[] a, int lda,
double[] rcond );
public static native int LAPACKE_ctrcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, FloatPointer rcond );
public static native int LAPACKE_ctrcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer rcond );
public static native int LAPACKE_ctrcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") float[] a,
int lda, float[] rcond );
public static native int LAPACKE_ztrcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, DoublePointer rcond );
public static native int LAPACKE_ztrcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer rcond );
public static native int LAPACKE_ztrcon( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") double[] a,
int lda, double[] rcond );
public static native int LAPACKE_strevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
IntPointer select, int n, @Const FloatPointer t,
int ldt, FloatPointer vl, int ldvl,
FloatPointer vr, int ldvr, int mm,
IntPointer m );
public static native int LAPACKE_strevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
IntBuffer select, int n, @Const FloatBuffer t,
int ldt, FloatBuffer vl, int ldvl,
FloatBuffer vr, int ldvr, int mm,
IntBuffer m );
public static native int LAPACKE_strevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
int[] select, int n, @Const float[] t,
int ldt, float[] vl, int ldvl,
float[] vr, int ldvr, int mm,
int[] m );
public static native int LAPACKE_dtrevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
IntPointer select, int n,
@Const DoublePointer t, int ldt, DoublePointer vl,
int ldvl, DoublePointer vr, int ldvr,
int mm, IntPointer m );
public static native int LAPACKE_dtrevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
IntBuffer select, int n,
@Const DoubleBuffer t, int ldt, DoubleBuffer vl,
int ldvl, DoubleBuffer vr, int ldvr,
int mm, IntBuffer m );
public static native int LAPACKE_dtrevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
int[] select, int n,
@Const double[] t, int ldt, double[] vl,
int ldvl, double[] vr, int ldvr,
int mm, int[] m );
public static native int LAPACKE_ctrevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
int mm, IntPointer m );
public static native int LAPACKE_ctrevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
int mm, IntBuffer m );
public static native int LAPACKE_ctrevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
int mm, int[] m );
public static native int LAPACKE_ztrevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
int mm, IntPointer m );
public static native int LAPACKE_ztrevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
int mm, IntBuffer m );
public static native int LAPACKE_ztrevc( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
int mm, int[] m );
public static native int LAPACKE_strexc( int matrix_layout, @Cast("char") byte compq, int n, FloatPointer t,
int ldt, FloatPointer q, int ldq,
IntPointer ifst, IntPointer ilst );
public static native int LAPACKE_strexc( int matrix_layout, @Cast("char") byte compq, int n, FloatBuffer t,
int ldt, FloatBuffer q, int ldq,
IntBuffer ifst, IntBuffer ilst );
public static native int LAPACKE_strexc( int matrix_layout, @Cast("char") byte compq, int n, float[] t,
int ldt, float[] q, int ldq,
int[] ifst, int[] ilst );
public static native int LAPACKE_dtrexc( int matrix_layout, @Cast("char") byte compq, int n,
DoublePointer t, int ldt, DoublePointer q, int ldq,
IntPointer ifst, IntPointer ilst );
public static native int LAPACKE_dtrexc( int matrix_layout, @Cast("char") byte compq, int n,
DoubleBuffer t, int ldt, DoubleBuffer q, int ldq,
IntBuffer ifst, IntBuffer ilst );
public static native int LAPACKE_dtrexc( int matrix_layout, @Cast("char") byte compq, int n,
double[] t, int ldt, double[] q, int ldq,
int[] ifst, int[] ilst );
public static native int LAPACKE_ctrexc( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ctrexc( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ctrexc( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ztrexc( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ztrexc( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ztrexc( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_strrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const FloatPointer a,
int lda, @Const FloatPointer b, int ldb,
@Const FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr );
public static native int LAPACKE_strrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const FloatBuffer a,
int lda, @Const FloatBuffer b, int ldb,
@Const FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr );
public static native int LAPACKE_strrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const float[] a,
int lda, @Const float[] b, int ldb,
@Const float[] x, int ldx, float[] ferr,
float[] berr );
public static native int LAPACKE_dtrrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const DoublePointer a,
int lda, @Const DoublePointer b, int ldb,
@Const DoublePointer x, int ldx, DoublePointer ferr,
DoublePointer berr );
public static native int LAPACKE_dtrrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const DoubleBuffer a,
int lda, @Const DoubleBuffer b, int ldb,
@Const DoubleBuffer x, int ldx, DoubleBuffer ferr,
DoubleBuffer berr );
public static native int LAPACKE_dtrrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const double[] a,
int lda, @Const double[] b, int ldb,
@Const double[] x, int ldx, double[] ferr,
double[] berr );
public static native int LAPACKE_ctrrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("const lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr );
public static native int LAPACKE_ctrrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("const lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr );
public static native int LAPACKE_ctrrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("const lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr );
public static native int LAPACKE_ztrrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("const lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr );
public static native int LAPACKE_ztrrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("const lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr );
public static native int LAPACKE_ztrrfs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("const lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr );
public static native int LAPACKE_strsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntPointer select, int n, FloatPointer t,
int ldt, FloatPointer q, int ldq, FloatPointer wr,
FloatPointer wi, IntPointer m, FloatPointer s, FloatPointer sep );
public static native int LAPACKE_strsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntBuffer select, int n, FloatBuffer t,
int ldt, FloatBuffer q, int ldq, FloatBuffer wr,
FloatBuffer wi, IntBuffer m, FloatBuffer s, FloatBuffer sep );
public static native int LAPACKE_strsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const int[] select, int n, float[] t,
int ldt, float[] q, int ldq, float[] wr,
float[] wi, int[] m, float[] s, float[] sep );
public static native int LAPACKE_dtrsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntPointer select, int n,
DoublePointer t, int ldt, DoublePointer q, int ldq,
DoublePointer wr, DoublePointer wi, IntPointer m, DoublePointer s,
DoublePointer sep );
public static native int LAPACKE_dtrsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntBuffer select, int n,
DoubleBuffer t, int ldt, DoubleBuffer q, int ldq,
DoubleBuffer wr, DoubleBuffer wi, IntBuffer m, DoubleBuffer s,
DoubleBuffer sep );
public static native int LAPACKE_dtrsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const int[] select, int n,
double[] t, int ldt, double[] q, int ldq,
double[] wr, double[] wi, int[] m, double[] s,
double[] sep );
public static native int LAPACKE_ctrsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntPointer select, int n,
@Cast("lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer w, IntPointer m, FloatPointer s,
FloatPointer sep );
public static native int LAPACKE_ctrsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntBuffer select, int n,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer w, IntBuffer m, FloatBuffer s,
FloatBuffer sep );
public static native int LAPACKE_ctrsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const int[] select, int n,
@Cast("lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] w, int[] m, float[] s,
float[] sep );
public static native int LAPACKE_ztrsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntPointer select, int n,
@Cast("lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer w, IntPointer m, DoublePointer s,
DoublePointer sep );
public static native int LAPACKE_ztrsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntBuffer select, int n,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer w, IntBuffer m, DoubleBuffer s,
DoubleBuffer sep );
public static native int LAPACKE_ztrsen( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const int[] select, int n,
@Cast("lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] w, int[] m, double[] s,
double[] sep );
public static native int LAPACKE_strsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const FloatPointer t, int ldt, @Const FloatPointer vl,
int ldvl, @Const FloatPointer vr, int ldvr,
FloatPointer s, FloatPointer sep, int mm, IntPointer m );
public static native int LAPACKE_strsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const FloatBuffer t, int ldt, @Const FloatBuffer vl,
int ldvl, @Const FloatBuffer vr, int ldvr,
FloatBuffer s, FloatBuffer sep, int mm, IntBuffer m );
public static native int LAPACKE_strsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const float[] t, int ldt, @Const float[] vl,
int ldvl, @Const float[] vr, int ldvr,
float[] s, float[] sep, int mm, int[] m );
public static native int LAPACKE_dtrsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const DoublePointer t, int ldt, @Const DoublePointer vl,
int ldvl, @Const DoublePointer vr, int ldvr,
DoublePointer s, DoublePointer sep, int mm,
IntPointer m );
public static native int LAPACKE_dtrsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const DoubleBuffer t, int ldt, @Const DoubleBuffer vl,
int ldvl, @Const DoubleBuffer vr, int ldvr,
DoubleBuffer s, DoubleBuffer sep, int mm,
IntBuffer m );
public static native int LAPACKE_dtrsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const double[] t, int ldt, @Const double[] vl,
int ldvl, @Const double[] vr, int ldvr,
double[] s, double[] sep, int mm,
int[] m );
public static native int LAPACKE_ctrsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_float*") FloatPointer t, int ldt,
@Cast("const lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("const lapack_complex_float*") FloatPointer vr, int ldvr,
FloatPointer s, FloatPointer sep, int mm, IntPointer m );
public static native int LAPACKE_ctrsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("const lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("const lapack_complex_float*") FloatBuffer vr, int ldvr,
FloatBuffer s, FloatBuffer sep, int mm, IntBuffer m );
public static native int LAPACKE_ctrsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_float*") float[] t, int ldt,
@Cast("const lapack_complex_float*") float[] vl, int ldvl,
@Cast("const lapack_complex_float*") float[] vr, int ldvr,
float[] s, float[] sep, int mm, int[] m );
public static native int LAPACKE_ztrsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_double*") DoublePointer t, int ldt,
@Cast("const lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("const lapack_complex_double*") DoublePointer vr, int ldvr,
DoublePointer s, DoublePointer sep, int mm,
IntPointer m );
public static native int LAPACKE_ztrsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("const lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("const lapack_complex_double*") DoubleBuffer vr, int ldvr,
DoubleBuffer s, DoubleBuffer sep, int mm,
IntBuffer m );
public static native int LAPACKE_ztrsna( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_double*") double[] t, int ldt,
@Cast("const lapack_complex_double*") double[] vl, int ldvl,
@Cast("const lapack_complex_double*") double[] vr, int ldvr,
double[] s, double[] sep, int mm,
int[] m );
public static native int LAPACKE_strsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const FloatPointer a, int lda, @Const FloatPointer b,
int ldb, FloatPointer c, int ldc,
FloatPointer scale );
public static native int LAPACKE_strsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const FloatBuffer a, int lda, @Const FloatBuffer b,
int ldb, FloatBuffer c, int ldc,
FloatBuffer scale );
public static native int LAPACKE_strsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const float[] a, int lda, @Const float[] b,
int ldb, float[] c, int ldc,
float[] scale );
public static native int LAPACKE_dtrsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const DoublePointer a, int lda, @Const DoublePointer b,
int ldb, DoublePointer c, int ldc,
DoublePointer scale );
public static native int LAPACKE_dtrsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const DoubleBuffer a, int lda, @Const DoubleBuffer b,
int ldb, DoubleBuffer c, int ldc,
DoubleBuffer scale );
public static native int LAPACKE_dtrsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const double[] a, int lda, @Const double[] b,
int ldb, double[] c, int ldc,
double[] scale );
public static native int LAPACKE_ctrsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
FloatPointer scale );
public static native int LAPACKE_ctrsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
FloatBuffer scale );
public static native int LAPACKE_ctrsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] c, int ldc,
float[] scale );
public static native int LAPACKE_ztrsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
DoublePointer scale );
public static native int LAPACKE_ztrsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
DoubleBuffer scale );
public static native int LAPACKE_ztrsyl( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] c, int ldc,
double[] scale );
public static native int LAPACKE_strtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
FloatPointer a, int lda );
public static native int LAPACKE_strtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
FloatBuffer a, int lda );
public static native int LAPACKE_strtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
float[] a, int lda );
public static native int LAPACKE_dtrtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
DoublePointer a, int lda );
public static native int LAPACKE_dtrtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
DoubleBuffer a, int lda );
public static native int LAPACKE_dtrtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
double[] a, int lda );
public static native int LAPACKE_ctrtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_ctrtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_ctrtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_ztrtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_ztrtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_ztrtri( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_strtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const FloatPointer a,
int lda, FloatPointer b, int ldb );
public static native int LAPACKE_strtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const FloatBuffer a,
int lda, FloatBuffer b, int ldb );
public static native int LAPACKE_strtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const float[] a,
int lda, float[] b, int ldb );
public static native int LAPACKE_dtrtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const DoublePointer a,
int lda, DoublePointer b, int ldb );
public static native int LAPACKE_dtrtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const DoubleBuffer a,
int lda, DoubleBuffer b, int ldb );
public static native int LAPACKE_dtrtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs, @Const double[] a,
int lda, double[] b, int ldb );
public static native int LAPACKE_ctrtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_ctrtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_ctrtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_ztrtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_ztrtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_ztrtrs( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_strttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatPointer a, int lda,
FloatPointer arf );
public static native int LAPACKE_strttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatBuffer a, int lda,
FloatBuffer arf );
public static native int LAPACKE_strttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const float[] a, int lda,
float[] arf );
public static native int LAPACKE_dtrttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoublePointer a, int lda,
DoublePointer arf );
public static native int LAPACKE_dtrttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoubleBuffer a, int lda,
DoubleBuffer arf );
public static native int LAPACKE_dtrttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const double[] a, int lda,
double[] arf );
public static native int LAPACKE_ctrttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer arf );
public static native int LAPACKE_ctrttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer arf );
public static native int LAPACKE_ctrttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] arf );
public static native int LAPACKE_ztrttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer arf );
public static native int LAPACKE_ztrttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer arf );
public static native int LAPACKE_ztrttf( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] arf );
public static native int LAPACKE_strttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer a, int lda, FloatPointer ap );
public static native int LAPACKE_strttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer a, int lda, FloatBuffer ap );
public static native int LAPACKE_strttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] a, int lda, float[] ap );
public static native int LAPACKE_dtrttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer a, int lda, DoublePointer ap );
public static native int LAPACKE_dtrttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer ap );
public static native int LAPACKE_dtrttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] a, int lda, double[] ap );
public static native int LAPACKE_ctrttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_ctrttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_ctrttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_ztrttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_ztrttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_ztrttp( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_stzrzf( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau );
public static native int LAPACKE_stzrzf( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau );
public static native int LAPACKE_stzrzf( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau );
public static native int LAPACKE_dtzrzf( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau );
public static native int LAPACKE_dtzrzf( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau );
public static native int LAPACKE_dtzrzf( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau );
public static native int LAPACKE_ctzrzf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_ctzrzf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_ctzrzf( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_ztzrzf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_ztzrzf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_ztzrzf( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_cungbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cungbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cungbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau );
public static native int LAPACKE_zungbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zungbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zungbr( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] tau );
public static native int LAPACKE_cunghr( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cunghr( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cunghr( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau );
public static native int LAPACKE_zunghr( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zunghr( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zunghr( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] tau );
public static native int LAPACKE_cunglq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cunglq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cunglq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau );
public static native int LAPACKE_zunglq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zunglq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zunglq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] tau );
public static native int LAPACKE_cungql( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cungql( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cungql( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau );
public static native int LAPACKE_zungql( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zungql( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zungql( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] tau );
public static native int LAPACKE_cungqr( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cungqr( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cungqr( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau );
public static native int LAPACKE_zungqr( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zungqr( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zungqr( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] tau );
public static native int LAPACKE_cungrq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cungrq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cungrq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau );
public static native int LAPACKE_zungrq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zungrq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zungrq( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] tau );
public static native int LAPACKE_cungtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_cungtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_cungtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau );
public static native int LAPACKE_zungtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zungtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zungtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau );
public static native int LAPACKE_cunmbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cunmbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cunmbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zunmbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zunmbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zunmbr( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_cunmhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cunmhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cunmhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zunmhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zunmhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zunmhr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_cunmlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cunmlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cunmlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zunmlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zunmlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zunmlq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_cunmql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cunmql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cunmql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zunmql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zunmql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zunmql( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_cunmqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cunmqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cunmqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zunmqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zunmqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zunmqr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_cunmrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cunmrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cunmrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zunmrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zunmrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zunmrq( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_cunmrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cunmrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cunmrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zunmrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zunmrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zunmrz( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_cunmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cunmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cunmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zunmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zunmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zunmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_cupgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer q, int ldq );
public static native int LAPACKE_cupgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq );
public static native int LAPACKE_cupgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] q, int ldq );
public static native int LAPACKE_zupgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer q, int ldq );
public static native int LAPACKE_zupgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq );
public static native int LAPACKE_zupgtr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] q, int ldq );
public static native int LAPACKE_cupmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc );
public static native int LAPACKE_cupmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc );
public static native int LAPACKE_cupmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc );
public static native int LAPACKE_zupmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc );
public static native int LAPACKE_zupmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc );
public static native int LAPACKE_zupmtr( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo, @Cast("char") byte trans,
int m, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc );
public static native int LAPACKE_sbdsdc_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, FloatPointer d, FloatPointer e, FloatPointer u,
int ldu, FloatPointer vt, int ldvt,
FloatPointer q, IntPointer iq, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_sbdsdc_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, FloatBuffer d, FloatBuffer e, FloatBuffer u,
int ldu, FloatBuffer vt, int ldvt,
FloatBuffer q, IntBuffer iq, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_sbdsdc_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, float[] d, float[] e, float[] u,
int ldu, float[] vt, int ldvt,
float[] q, int[] iq, float[] work,
int[] iwork );
public static native int LAPACKE_dbdsdc_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, DoublePointer d, DoublePointer e, DoublePointer u,
int ldu, DoublePointer vt, int ldvt,
DoublePointer q, IntPointer iq, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dbdsdc_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, DoubleBuffer d, DoubleBuffer e, DoubleBuffer u,
int ldu, DoubleBuffer vt, int ldvt,
DoubleBuffer q, IntBuffer iq, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dbdsdc_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte compq,
int n, double[] d, double[] e, double[] u,
int ldu, double[] vt, int ldvt,
double[] q, int[] iq, double[] work,
int[] iwork );
public static native int LAPACKE_sbdsvdx_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e,
int vl, int vu,
int il, int iu, int ns,
FloatPointer s, FloatPointer z, int ldz,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sbdsvdx_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e,
int vl, int vu,
int il, int iu, int ns,
FloatBuffer s, FloatBuffer z, int ldz,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sbdsvdx_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e,
int vl, int vu,
int il, int iu, int ns,
float[] s, float[] z, int ldz,
float[] work, int[] iwork );
public static native int LAPACKE_dbdsvdx_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e,
int vl, int vu,
int il, int iu, int ns,
DoublePointer s, DoublePointer z, int ldz,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dbdsvdx_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e,
int vl, int vu,
int il, int iu, int ns,
DoubleBuffer s, DoubleBuffer z, int ldz,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dbdsvdx_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e,
int vl, int vu,
int il, int iu, int ns,
double[] s, double[] z, int ldz,
double[] work, int[] iwork );
public static native int LAPACKE_sbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
FloatPointer d, FloatPointer e, FloatPointer vt, int ldvt,
FloatPointer u, int ldu, FloatPointer c,
int ldc, FloatPointer work );
public static native int LAPACKE_sbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
FloatBuffer d, FloatBuffer e, FloatBuffer vt, int ldvt,
FloatBuffer u, int ldu, FloatBuffer c,
int ldc, FloatBuffer work );
public static native int LAPACKE_sbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
float[] d, float[] e, float[] vt, int ldvt,
float[] u, int ldu, float[] c,
int ldc, float[] work );
public static native int LAPACKE_dbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
DoublePointer d, DoublePointer e, DoublePointer vt,
int ldvt, DoublePointer u, int ldu,
DoublePointer c, int ldc, DoublePointer work );
public static native int LAPACKE_dbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer vt,
int ldvt, DoubleBuffer u, int ldu,
DoubleBuffer c, int ldc, DoubleBuffer work );
public static native int LAPACKE_dbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
double[] d, double[] e, double[] vt,
int ldvt, double[] u, int ldu,
double[] c, int ldc, double[] work );
public static native int LAPACKE_cbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
FloatPointer d, FloatPointer e, @Cast("lapack_complex_float*") FloatPointer vt,
int ldvt, @Cast("lapack_complex_float*") FloatPointer u,
int ldu, @Cast("lapack_complex_float*") FloatPointer c,
int ldc, FloatPointer work );
public static native int LAPACKE_cbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
FloatBuffer d, FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer vt,
int ldvt, @Cast("lapack_complex_float*") FloatBuffer u,
int ldu, @Cast("lapack_complex_float*") FloatBuffer c,
int ldc, FloatBuffer work );
public static native int LAPACKE_cbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
float[] d, float[] e, @Cast("lapack_complex_float*") float[] vt,
int ldvt, @Cast("lapack_complex_float*") float[] u,
int ldu, @Cast("lapack_complex_float*") float[] c,
int ldc, float[] work );
public static native int LAPACKE_zbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer vt,
int ldvt, @Cast("lapack_complex_double*") DoublePointer u,
int ldu, @Cast("lapack_complex_double*") DoublePointer c,
int ldc, DoublePointer work );
public static native int LAPACKE_zbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer vt,
int ldvt, @Cast("lapack_complex_double*") DoubleBuffer u,
int ldu, @Cast("lapack_complex_double*") DoubleBuffer c,
int ldc, DoubleBuffer work );
public static native int LAPACKE_zbdsqr_work( int matrix_layout, @Cast("char") byte uplo, int n,
int ncvt, int nru, int ncc,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] vt,
int ldvt, @Cast("lapack_complex_double*") double[] u,
int ldu, @Cast("lapack_complex_double*") double[] c,
int ldc, double[] work );
public static native int LAPACKE_sdisna_work( @Cast("char") byte job, int m, int n,
@Const FloatPointer d, FloatPointer sep );
public static native int LAPACKE_sdisna_work( @Cast("char") byte job, int m, int n,
@Const FloatBuffer d, FloatBuffer sep );
public static native int LAPACKE_sdisna_work( @Cast("char") byte job, int m, int n,
@Const float[] d, float[] sep );
public static native int LAPACKE_ddisna_work( @Cast("char") byte job, int m, int n,
@Const DoublePointer d, DoublePointer sep );
public static native int LAPACKE_ddisna_work( @Cast("char") byte job, int m, int n,
@Const DoubleBuffer d, DoubleBuffer sep );
public static native int LAPACKE_ddisna_work( @Cast("char") byte job, int m, int n,
@Const double[] d, double[] sep );
public static native int LAPACKE_sgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, FloatPointer ab, int ldab,
FloatPointer d, FloatPointer e, FloatPointer q, int ldq,
FloatPointer pt, int ldpt, FloatPointer c,
int ldc, FloatPointer work );
public static native int LAPACKE_sgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, FloatBuffer ab, int ldab,
FloatBuffer d, FloatBuffer e, FloatBuffer q, int ldq,
FloatBuffer pt, int ldpt, FloatBuffer c,
int ldc, FloatBuffer work );
public static native int LAPACKE_sgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, float[] ab, int ldab,
float[] d, float[] e, float[] q, int ldq,
float[] pt, int ldpt, float[] c,
int ldc, float[] work );
public static native int LAPACKE_dgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, DoublePointer ab, int ldab,
DoublePointer d, DoublePointer e, DoublePointer q, int ldq,
DoublePointer pt, int ldpt, DoublePointer c,
int ldc, DoublePointer work );
public static native int LAPACKE_dgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, DoubleBuffer ab, int ldab,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer q, int ldq,
DoubleBuffer pt, int ldpt, DoubleBuffer c,
int ldc, DoubleBuffer work );
public static native int LAPACKE_dgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, double[] ab, int ldab,
double[] d, double[] e, double[] q, int ldq,
double[] pt, int ldpt, double[] c,
int ldc, double[] work );
public static native int LAPACKE_cgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer pt, int ldpt,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer pt, int ldpt,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_float*") float[] ab,
int ldab, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] pt, int ldpt,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer pt, int ldpt,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer pt, int ldpt,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zgbbrd_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int ncc, int kl,
int ku, @Cast("lapack_complex_double*") double[] ab,
int ldab, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] pt, int ldpt,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const FloatPointer ab,
int ldab, @Const IntPointer ipiv,
float anorm, FloatPointer rcond, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_sgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const FloatBuffer ab,
int ldab, @Const IntBuffer ipiv,
float anorm, FloatBuffer rcond, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_sgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const float[] ab,
int ldab, @Const int[] ipiv,
float anorm, float[] rcond, float[] work,
int[] iwork );
public static native int LAPACKE_dgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const DoublePointer ab,
int ldab, @Const IntPointer ipiv,
double anorm, DoublePointer rcond, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const DoubleBuffer ab,
int ldab, @Const IntBuffer ipiv,
double anorm, DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku, @Const double[] ab,
int ldab, @Const int[] ipiv,
double anorm, double[] rcond, double[] work,
int[] iwork );
public static native int LAPACKE_cgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Const IntPointer ipiv, float anorm,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_cgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Const IntBuffer ipiv, float anorm,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_cgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Const int[] ipiv, float anorm,
float[] rcond, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_zgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, @Const IntPointer ipiv,
double anorm, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, @Const IntBuffer ipiv,
double anorm, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zgbcon_work( int matrix_layout, @Cast("char") byte norm, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") double[] ab,
int ldab, @Const int[] ipiv,
double anorm, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sgbequ_work( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatPointer ab,
int ldab, FloatPointer r, FloatPointer c,
FloatPointer rowcnd, FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_sgbequ_work( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatBuffer ab,
int ldab, FloatBuffer r, FloatBuffer c,
FloatBuffer rowcnd, FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_sgbequ_work( int matrix_layout, int m, int n,
int kl, int ku, @Const float[] ab,
int ldab, float[] r, float[] c,
float[] rowcnd, float[] colcnd, float[] amax );
public static native int LAPACKE_dgbequ_work( int matrix_layout, int m, int n,
int kl, int ku, @Const DoublePointer ab,
int ldab, DoublePointer r, DoublePointer c,
DoublePointer rowcnd, DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_dgbequ_work( int matrix_layout, int m, int n,
int kl, int ku, @Const DoubleBuffer ab,
int ldab, DoubleBuffer r, DoubleBuffer c,
DoubleBuffer rowcnd, DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_dgbequ_work( int matrix_layout, int m, int n,
int kl, int ku, @Const double[] ab,
int ldab, double[] r, double[] c,
double[] rowcnd, double[] colcnd, double[] amax );
public static native int LAPACKE_cgbequ_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_cgbequ_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_cgbequ_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax );
public static native int LAPACKE_zgbequ_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer r, DoublePointer c,
DoublePointer rowcnd, DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_zgbequ_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer r, DoubleBuffer c,
DoubleBuffer rowcnd, DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_zgbequ_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") double[] ab,
int ldab, double[] r, double[] c,
double[] rowcnd, double[] colcnd, double[] amax );
public static native int LAPACKE_sgbequb_work( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatPointer ab,
int ldab, FloatPointer r, FloatPointer c,
FloatPointer rowcnd, FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_sgbequb_work( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatBuffer ab,
int ldab, FloatBuffer r, FloatBuffer c,
FloatBuffer rowcnd, FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_sgbequb_work( int matrix_layout, int m, int n,
int kl, int ku, @Const float[] ab,
int ldab, float[] r, float[] c,
float[] rowcnd, float[] colcnd, float[] amax );
public static native int LAPACKE_dgbequb_work( int matrix_layout, int m, int n,
int kl, int ku, @Const DoublePointer ab,
int ldab, DoublePointer r, DoublePointer c,
DoublePointer rowcnd, DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_dgbequb_work( int matrix_layout, int m, int n,
int kl, int ku, @Const DoubleBuffer ab,
int ldab, DoubleBuffer r, DoubleBuffer c,
DoubleBuffer rowcnd, DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_dgbequb_work( int matrix_layout, int m, int n,
int kl, int ku, @Const double[] ab,
int ldab, double[] r, double[] c,
double[] rowcnd, double[] colcnd, double[] amax );
public static native int LAPACKE_cgbequb_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatPointer ab,
int ldab, FloatPointer r, FloatPointer c,
FloatPointer rowcnd, FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_cgbequb_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab, FloatBuffer r, FloatBuffer c,
FloatBuffer rowcnd, FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_cgbequb_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") float[] ab,
int ldab, float[] r, float[] c,
float[] rowcnd, float[] colcnd, float[] amax );
public static native int LAPACKE_zgbequb_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer r, DoublePointer c,
DoublePointer rowcnd, DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_zgbequb_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer r, DoubleBuffer c,
DoubleBuffer rowcnd, DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_zgbequb_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") double[] ab,
int ldab, double[] r, double[] c,
double[] rowcnd, double[] colcnd, double[] amax );
public static native int LAPACKE_sgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const FloatPointer ab, int ldab,
@Const FloatPointer afb, int ldafb,
@Const IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_sgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const FloatBuffer ab, int ldab,
@Const FloatBuffer afb, int ldafb,
@Const IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_sgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const float[] ab, int ldab,
@Const float[] afb, int ldafb,
@Const int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr, float[] work,
int[] iwork );
public static native int LAPACKE_dgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const DoublePointer ab, int ldab,
@Const DoublePointer afb, int ldafb,
@Const IntPointer ipiv, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const DoubleBuffer ab, int ldab,
@Const DoubleBuffer afb, int ldafb,
@Const IntBuffer ipiv, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const double[] ab, int ldab,
@Const double[] afb, int ldafb,
@Const int[] ipiv, @Const double[] b,
int ldb, double[] x, int ldx,
double[] ferr, double[] berr, double[] work,
int[] iwork );
public static native int LAPACKE_cgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("const lapack_complex_float*") FloatPointer afb,
int ldafb, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("const lapack_complex_float*") FloatBuffer afb,
int ldafb, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Cast("const lapack_complex_float*") float[] afb,
int ldafb, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab,
@Cast("const lapack_complex_double*") DoublePointer afb,
int ldafb, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab,
@Cast("const lapack_complex_double*") DoubleBuffer afb,
int ldafb, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zgbrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") double[] ab,
int ldab,
@Cast("const lapack_complex_double*") double[] afb,
int ldafb, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs, FloatPointer ab,
int ldab, IntPointer ipiv, FloatPointer b,
int ldb );
public static native int LAPACKE_sgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs, FloatBuffer ab,
int ldab, IntBuffer ipiv, FloatBuffer b,
int ldb );
public static native int LAPACKE_sgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs, float[] ab,
int ldab, int[] ipiv, float[] b,
int ldb );
public static native int LAPACKE_dgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs, DoublePointer ab,
int ldab, IntPointer ipiv, DoublePointer b,
int ldb );
public static native int LAPACKE_dgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs, DoubleBuffer ab,
int ldab, IntBuffer ipiv, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs, double[] ab,
int ldab, int[] ipiv, double[] b,
int ldb );
public static native int LAPACKE_cgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_float*") float[] ab, int ldab,
int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zgbsv_work( int matrix_layout, int n, int kl,
int ku, int nrhs,
@Cast("lapack_complex_double*") double[] ab, int ldab,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_sgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, FloatPointer ab, int ldab,
FloatPointer afb, int ldafb, IntPointer ipiv,
@Cast("char*") BytePointer equed, FloatPointer r, FloatPointer c, FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, FloatBuffer ab, int ldab,
FloatBuffer afb, int ldafb, IntBuffer ipiv,
@Cast("char*") ByteBuffer equed, FloatBuffer r, FloatBuffer c, FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, float[] ab, int ldab,
float[] afb, int ldafb, int[] ipiv,
@Cast("char*") byte[] equed, float[] r, float[] c, float[] b,
int ldb, float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
float[] work, int[] iwork );
public static native int LAPACKE_dgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, DoublePointer ab, int ldab,
DoublePointer afb, int ldafb, IntPointer ipiv,
@Cast("char*") BytePointer equed, DoublePointer r, DoublePointer c, DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, DoubleBuffer ab, int ldab,
DoubleBuffer afb, int ldafb, IntBuffer ipiv,
@Cast("char*") ByteBuffer equed, DoubleBuffer r, DoubleBuffer c, DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, double[] ab, int ldab,
double[] afb, int ldafb, int[] ipiv,
@Cast("char*") byte[] equed, double[] r, double[] c, double[] b,
int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
double[] work, int[] iwork );
public static native int LAPACKE_cgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab, @Cast("lapack_complex_float*") FloatPointer afb,
int ldafb, IntPointer ipiv, @Cast("char*") BytePointer equed,
FloatPointer r, FloatPointer c, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_cgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab, @Cast("lapack_complex_float*") FloatBuffer afb,
int ldafb, IntBuffer ipiv, @Cast("char*") ByteBuffer equed,
FloatBuffer r, FloatBuffer c, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_cgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_float*") float[] ab,
int ldab, @Cast("lapack_complex_float*") float[] afb,
int ldafb, int[] ipiv, @Cast("char*") byte[] equed,
float[] r, float[] c, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_zgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab, @Cast("lapack_complex_double*") DoublePointer afb,
int ldafb, IntPointer ipiv, @Cast("char*") BytePointer equed,
DoublePointer r, DoublePointer c, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork );
public static native int LAPACKE_zgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab, @Cast("lapack_complex_double*") DoubleBuffer afb,
int ldafb, IntBuffer ipiv, @Cast("char*") ByteBuffer equed,
DoubleBuffer r, DoubleBuffer c, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork );
public static native int LAPACKE_zgbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int kl, int ku,
int nrhs, @Cast("lapack_complex_double*") double[] ab,
int ldab, @Cast("lapack_complex_double*") double[] afb,
int ldafb, int[] ipiv, @Cast("char*") byte[] equed,
double[] r, double[] c, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, double[] rcond, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork );
public static native int LAPACKE_sgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku, FloatPointer ab,
int ldab, IntPointer ipiv );
public static native int LAPACKE_sgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku, FloatBuffer ab,
int ldab, IntBuffer ipiv );
public static native int LAPACKE_sgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku, float[] ab,
int ldab, int[] ipiv );
public static native int LAPACKE_dgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku, DoublePointer ab,
int ldab, IntPointer ipiv );
public static native int LAPACKE_dgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku, DoubleBuffer ab,
int ldab, IntBuffer ipiv );
public static native int LAPACKE_dgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku, double[] ab,
int ldab, int[] ipiv );
public static native int LAPACKE_cgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
IntPointer ipiv );
public static native int LAPACKE_cgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
IntBuffer ipiv );
public static native int LAPACKE_cgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_float*") float[] ab, int ldab,
int[] ipiv );
public static native int LAPACKE_zgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
IntPointer ipiv );
public static native int LAPACKE_zgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
IntBuffer ipiv );
public static native int LAPACKE_zgbtrf_work( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("lapack_complex_double*") double[] ab, int ldab,
int[] ipiv );
public static native int LAPACKE_sgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const FloatPointer ab, int ldab,
@Const IntPointer ipiv, FloatPointer b,
int ldb );
public static native int LAPACKE_sgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const FloatBuffer ab, int ldab,
@Const IntBuffer ipiv, FloatBuffer b,
int ldb );
public static native int LAPACKE_sgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const float[] ab, int ldab,
@Const int[] ipiv, float[] b,
int ldb );
public static native int LAPACKE_dgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const DoublePointer ab, int ldab,
@Const IntPointer ipiv, DoublePointer b,
int ldb );
public static native int LAPACKE_dgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const DoubleBuffer ab, int ldab,
@Const IntBuffer ipiv, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Const double[] ab, int ldab,
@Const int[] ipiv, double[] b,
int ldb );
public static native int LAPACKE_cgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zgbtrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int kl, int ku, int nrhs,
@Cast("const lapack_complex_double*") double[] ab,
int ldab, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const FloatPointer scale, int m, FloatPointer v,
int ldv );
public static native int LAPACKE_sgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const FloatBuffer scale, int m, FloatBuffer v,
int ldv );
public static native int LAPACKE_sgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const float[] scale, int m, float[] v,
int ldv );
public static native int LAPACKE_dgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const DoublePointer scale, int m, DoublePointer v,
int ldv );
public static native int LAPACKE_dgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const DoubleBuffer scale, int m, DoubleBuffer v,
int ldv );
public static native int LAPACKE_dgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const double[] scale, int m, double[] v,
int ldv );
public static native int LAPACKE_cgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const FloatPointer scale, int m,
@Cast("lapack_complex_float*") FloatPointer v, int ldv );
public static native int LAPACKE_cgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const FloatBuffer scale, int m,
@Cast("lapack_complex_float*") FloatBuffer v, int ldv );
public static native int LAPACKE_cgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const float[] scale, int m,
@Cast("lapack_complex_float*") float[] v, int ldv );
public static native int LAPACKE_zgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const DoublePointer scale, int m,
@Cast("lapack_complex_double*") DoublePointer v, int ldv );
public static native int LAPACKE_zgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const DoubleBuffer scale, int m,
@Cast("lapack_complex_double*") DoubleBuffer v, int ldv );
public static native int LAPACKE_zgebak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const double[] scale, int m,
@Cast("lapack_complex_double*") double[] v, int ldv );
public static native int LAPACKE_sgebal_work( int matrix_layout, @Cast("char") byte job, int n,
FloatPointer a, int lda, IntPointer ilo,
IntPointer ihi, FloatPointer scale );
public static native int LAPACKE_sgebal_work( int matrix_layout, @Cast("char") byte job, int n,
FloatBuffer a, int lda, IntBuffer ilo,
IntBuffer ihi, FloatBuffer scale );
public static native int LAPACKE_sgebal_work( int matrix_layout, @Cast("char") byte job, int n,
float[] a, int lda, int[] ilo,
int[] ihi, float[] scale );
public static native int LAPACKE_dgebal_work( int matrix_layout, @Cast("char") byte job, int n,
DoublePointer a, int lda, IntPointer ilo,
IntPointer ihi, DoublePointer scale );
public static native int LAPACKE_dgebal_work( int matrix_layout, @Cast("char") byte job, int n,
DoubleBuffer a, int lda, IntBuffer ilo,
IntBuffer ihi, DoubleBuffer scale );
public static native int LAPACKE_dgebal_work( int matrix_layout, @Cast("char") byte job, int n,
double[] a, int lda, int[] ilo,
int[] ihi, double[] scale );
public static native int LAPACKE_cgebal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ilo, IntPointer ihi,
FloatPointer scale );
public static native int LAPACKE_cgebal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ilo, IntBuffer ihi,
FloatBuffer scale );
public static native int LAPACKE_cgebal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ilo, int[] ihi,
float[] scale );
public static native int LAPACKE_zgebal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ilo, IntPointer ihi,
DoublePointer scale );
public static native int LAPACKE_zgebal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ilo, IntBuffer ihi,
DoubleBuffer scale );
public static native int LAPACKE_zgebal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ilo, int[] ihi,
double[] scale );
public static native int LAPACKE_sgebrd_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer d, FloatPointer e,
FloatPointer tauq, FloatPointer taup, FloatPointer work,
int lwork );
public static native int LAPACKE_sgebrd_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer d, FloatBuffer e,
FloatBuffer tauq, FloatBuffer taup, FloatBuffer work,
int lwork );
public static native int LAPACKE_sgebrd_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] d, float[] e,
float[] tauq, float[] taup, float[] work,
int lwork );
public static native int LAPACKE_dgebrd_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer d, DoublePointer e,
DoublePointer tauq, DoublePointer taup, DoublePointer work,
int lwork );
public static native int LAPACKE_dgebrd_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer d, DoubleBuffer e,
DoubleBuffer tauq, DoubleBuffer taup, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dgebrd_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] d, double[] e,
double[] tauq, double[] taup, double[] work,
int lwork );
public static native int LAPACKE_cgebrd_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
FloatPointer d, FloatPointer e, @Cast("lapack_complex_float*") FloatPointer tauq,
@Cast("lapack_complex_float*") FloatPointer taup,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgebrd_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer d, FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer tauq,
@Cast("lapack_complex_float*") FloatBuffer taup,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgebrd_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
float[] d, float[] e, @Cast("lapack_complex_float*") float[] tauq,
@Cast("lapack_complex_float*") float[] taup,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgebrd_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer tauq,
@Cast("lapack_complex_double*") DoublePointer taup,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zgebrd_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer tauq,
@Cast("lapack_complex_double*") DoubleBuffer taup,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zgebrd_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
double[] d, double[] e,
@Cast("lapack_complex_double*") double[] tauq,
@Cast("lapack_complex_double*") double[] taup,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Const FloatPointer a, int lda, float anorm,
FloatPointer rcond, FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Const FloatBuffer a, int lda, float anorm,
FloatBuffer rcond, FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Const float[] a, int lda, float anorm,
float[] rcond, float[] work, int[] iwork );
public static native int LAPACKE_dgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Const DoublePointer a, int lda, double anorm,
DoublePointer rcond, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Const DoubleBuffer a, int lda, double anorm,
DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Const double[] a, int lda, double anorm,
double[] rcond, double[] work,
int[] iwork );
public static native int LAPACKE_cgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
float anorm, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
float anorm, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float anorm, float[] rcond,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
double anorm, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
double anorm, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zgecon_work( int matrix_layout, @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double anorm, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sgeequ_work( int matrix_layout, int m, int n,
@Const FloatPointer a, int lda, FloatPointer r,
FloatPointer c, FloatPointer rowcnd, FloatPointer colcnd,
FloatPointer amax );
public static native int LAPACKE_sgeequ_work( int matrix_layout, int m, int n,
@Const FloatBuffer a, int lda, FloatBuffer r,
FloatBuffer c, FloatBuffer rowcnd, FloatBuffer colcnd,
FloatBuffer amax );
public static native int LAPACKE_sgeequ_work( int matrix_layout, int m, int n,
@Const float[] a, int lda, float[] r,
float[] c, float[] rowcnd, float[] colcnd,
float[] amax );
public static native int LAPACKE_dgeequ_work( int matrix_layout, int m, int n,
@Const DoublePointer a, int lda, DoublePointer r,
DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd,
DoublePointer amax );
public static native int LAPACKE_dgeequ_work( int matrix_layout, int m, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer r,
DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd,
DoubleBuffer amax );
public static native int LAPACKE_dgeequ_work( int matrix_layout, int m, int n,
@Const double[] a, int lda, double[] r,
double[] c, double[] rowcnd, double[] colcnd,
double[] amax );
public static native int LAPACKE_cgeequ_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_cgeequ_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_cgeequ_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax );
public static native int LAPACKE_zgeequ_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer r, DoublePointer c, DoublePointer rowcnd,
DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_zgeequ_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd,
DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_zgeequ_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] r, double[] c, double[] rowcnd,
double[] colcnd, double[] amax );
public static native int LAPACKE_sgeequb_work( int matrix_layout, int m, int n,
@Const FloatPointer a, int lda, FloatPointer r,
FloatPointer c, FloatPointer rowcnd, FloatPointer colcnd,
FloatPointer amax );
public static native int LAPACKE_sgeequb_work( int matrix_layout, int m, int n,
@Const FloatBuffer a, int lda, FloatBuffer r,
FloatBuffer c, FloatBuffer rowcnd, FloatBuffer colcnd,
FloatBuffer amax );
public static native int LAPACKE_sgeequb_work( int matrix_layout, int m, int n,
@Const float[] a, int lda, float[] r,
float[] c, float[] rowcnd, float[] colcnd,
float[] amax );
public static native int LAPACKE_dgeequb_work( int matrix_layout, int m, int n,
@Const DoublePointer a, int lda, DoublePointer r,
DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd,
DoublePointer amax );
public static native int LAPACKE_dgeequb_work( int matrix_layout, int m, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer r,
DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd,
DoubleBuffer amax );
public static native int LAPACKE_dgeequb_work( int matrix_layout, int m, int n,
@Const double[] a, int lda, double[] r,
double[] c, double[] rowcnd, double[] colcnd,
double[] amax );
public static native int LAPACKE_cgeequb_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax );
public static native int LAPACKE_cgeequb_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax );
public static native int LAPACKE_cgeequb_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax );
public static native int LAPACKE_zgeequb_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer r, DoublePointer c, DoublePointer rowcnd,
DoublePointer colcnd, DoublePointer amax );
public static native int LAPACKE_zgeequb_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd,
DoubleBuffer colcnd, DoubleBuffer amax );
public static native int LAPACKE_zgeequb_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] r, double[] c, double[] rowcnd,
double[] colcnd, double[] amax );
public static native int LAPACKE_sgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, int n, FloatPointer a,
int lda, IntPointer sdim, FloatPointer wr,
FloatPointer wi, FloatPointer vs, int ldvs,
FloatPointer work, int lwork,
IntPointer bwork );
public static native int LAPACKE_sgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, int n, FloatBuffer a,
int lda, IntBuffer sdim, FloatBuffer wr,
FloatBuffer wi, FloatBuffer vs, int ldvs,
FloatBuffer work, int lwork,
IntBuffer bwork );
public static native int LAPACKE_sgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, int n, float[] a,
int lda, int[] sdim, float[] wr,
float[] wi, float[] vs, int ldvs,
float[] work, int lwork,
int[] bwork );
public static native int LAPACKE_dgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, int n, DoublePointer a,
int lda, IntPointer sdim, DoublePointer wr,
DoublePointer wi, DoublePointer vs, int ldvs,
DoublePointer work, int lwork,
IntPointer bwork );
public static native int LAPACKE_dgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, int n, DoubleBuffer a,
int lda, IntBuffer sdim, DoubleBuffer wr,
DoubleBuffer wi, DoubleBuffer vs, int ldvs,
DoubleBuffer work, int lwork,
IntBuffer bwork );
public static native int LAPACKE_dgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, int n, double[] a,
int lda, int[] sdim, double[] wr,
double[] wi, double[] vs, int ldvs,
double[] work, int lwork,
int[] bwork );
public static native int LAPACKE_cgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer sdim, @Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer vs, int ldvs,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, IntPointer bwork );
public static native int LAPACKE_cgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer sdim, @Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer vs, int ldvs,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, IntBuffer bwork );
public static native int LAPACKE_cgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] sdim, @Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] vs, int ldvs,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int[] bwork );
public static native int LAPACKE_zgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer sdim, @Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vs, int ldvs,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, IntPointer bwork );
public static native int LAPACKE_zgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer sdim, @Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vs, int ldvs,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, IntBuffer bwork );
public static native int LAPACKE_zgees_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] sdim, @Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vs, int ldvs,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int[] bwork );
public static native int LAPACKE_sgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, @Cast("char") byte sense,
int n, FloatPointer a, int lda,
IntPointer sdim, FloatPointer wr, FloatPointer wi,
FloatPointer vs, int ldvs, FloatPointer rconde,
FloatPointer rcondv, FloatPointer work, int lwork,
IntPointer iwork, int liwork,
IntPointer bwork );
public static native int LAPACKE_sgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, @Cast("char") byte sense,
int n, FloatBuffer a, int lda,
IntBuffer sdim, FloatBuffer wr, FloatBuffer wi,
FloatBuffer vs, int ldvs, FloatBuffer rconde,
FloatBuffer rcondv, FloatBuffer work, int lwork,
IntBuffer iwork, int liwork,
IntBuffer bwork );
public static native int LAPACKE_sgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_S_SELECT2 select, @Cast("char") byte sense,
int n, float[] a, int lda,
int[] sdim, float[] wr, float[] wi,
float[] vs, int ldvs, float[] rconde,
float[] rcondv, float[] work, int lwork,
int[] iwork, int liwork,
int[] bwork );
public static native int LAPACKE_dgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, @Cast("char") byte sense,
int n, DoublePointer a, int lda,
IntPointer sdim, DoublePointer wr, DoublePointer wi,
DoublePointer vs, int ldvs, DoublePointer rconde,
DoublePointer rcondv, DoublePointer work, int lwork,
IntPointer iwork, int liwork,
IntPointer bwork );
public static native int LAPACKE_dgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, @Cast("char") byte sense,
int n, DoubleBuffer a, int lda,
IntBuffer sdim, DoubleBuffer wr, DoubleBuffer wi,
DoubleBuffer vs, int ldvs, DoubleBuffer rconde,
DoubleBuffer rcondv, DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork,
IntBuffer bwork );
public static native int LAPACKE_dgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_D_SELECT2 select, @Cast("char") byte sense,
int n, double[] a, int lda,
int[] sdim, double[] wr, double[] wi,
double[] vs, int ldvs, double[] rconde,
double[] rcondv, double[] work, int lwork,
int[] iwork, int liwork,
int[] bwork );
public static native int LAPACKE_cgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, @Cast("char") byte sense,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer sdim,
@Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer vs, int ldvs,
FloatPointer rconde, FloatPointer rcondv,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, IntPointer bwork );
public static native int LAPACKE_cgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, @Cast("char") byte sense,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer sdim,
@Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer vs, int ldvs,
FloatBuffer rconde, FloatBuffer rcondv,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, IntBuffer bwork );
public static native int LAPACKE_cgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_C_SELECT1 select, @Cast("char") byte sense,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, int[] sdim,
@Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] vs, int ldvs,
float[] rconde, float[] rcondv,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int[] bwork );
public static native int LAPACKE_zgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, @Cast("char") byte sense,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer sdim,
@Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vs, int ldvs,
DoublePointer rconde, DoublePointer rcondv,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, IntPointer bwork );
public static native int LAPACKE_zgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, @Cast("char") byte sense,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer sdim,
@Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vs, int ldvs,
DoubleBuffer rconde, DoubleBuffer rcondv,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, IntBuffer bwork );
public static native int LAPACKE_zgeesx_work( int matrix_layout, @Cast("char") byte jobvs, @Cast("char") byte sort,
LAPACK_Z_SELECT1 select, @Cast("char") byte sense,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, int[] sdim,
@Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vs, int ldvs,
double[] rconde, double[] rcondv,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int[] bwork );
public static native int LAPACKE_sgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatPointer a, int lda,
FloatPointer wr, FloatPointer wi, FloatPointer vl, int ldvl,
FloatPointer vr, int ldvr, FloatPointer work,
int lwork );
public static native int LAPACKE_sgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatBuffer a, int lda,
FloatBuffer wr, FloatBuffer wi, FloatBuffer vl, int ldvl,
FloatBuffer vr, int ldvr, FloatBuffer work,
int lwork );
public static native int LAPACKE_sgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, float[] a, int lda,
float[] wr, float[] wi, float[] vl, int ldvl,
float[] vr, int ldvr, float[] work,
int lwork );
public static native int LAPACKE_dgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoublePointer a, int lda,
DoublePointer wr, DoublePointer wi, DoublePointer vl,
int ldvl, DoublePointer vr, int ldvr,
DoublePointer work, int lwork );
public static native int LAPACKE_dgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoubleBuffer a, int lda,
DoubleBuffer wr, DoubleBuffer wi, DoubleBuffer vl,
int ldvl, DoubleBuffer vr, int ldvr,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, double[] a, int lda,
double[] wr, double[] wi, double[] vl,
int ldvl, double[] vr, int ldvr,
double[] work, int lwork );
public static native int LAPACKE_cgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork );
public static native int LAPACKE_cgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork );
public static native int LAPACKE_cgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork );
public static native int LAPACKE_zgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork );
public static native int LAPACKE_zgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork );
public static native int LAPACKE_zgeev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork );
public static native int LAPACKE_sgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, FloatPointer a,
int lda, FloatPointer wr, FloatPointer wi, FloatPointer vl,
int ldvl, FloatPointer vr, int ldvr,
IntPointer ilo, IntPointer ihi, FloatPointer scale,
FloatPointer abnrm, FloatPointer rconde, FloatPointer rcondv,
FloatPointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_sgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, FloatBuffer a,
int lda, FloatBuffer wr, FloatBuffer wi, FloatBuffer vl,
int ldvl, FloatBuffer vr, int ldvr,
IntBuffer ilo, IntBuffer ihi, FloatBuffer scale,
FloatBuffer abnrm, FloatBuffer rconde, FloatBuffer rcondv,
FloatBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_sgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, float[] a,
int lda, float[] wr, float[] wi, float[] vl,
int ldvl, float[] vr, int ldvr,
int[] ilo, int[] ihi, float[] scale,
float[] abnrm, float[] rconde, float[] rcondv,
float[] work, int lwork,
int[] iwork );
public static native int LAPACKE_dgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, DoublePointer a,
int lda, DoublePointer wr, DoublePointer wi,
DoublePointer vl, int ldvl, DoublePointer vr,
int ldvr, IntPointer ilo,
IntPointer ihi, DoublePointer scale, DoublePointer abnrm,
DoublePointer rconde, DoublePointer rcondv, DoublePointer work,
int lwork, IntPointer iwork );
public static native int LAPACKE_dgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, DoubleBuffer a,
int lda, DoubleBuffer wr, DoubleBuffer wi,
DoubleBuffer vl, int ldvl, DoubleBuffer vr,
int ldvr, IntBuffer ilo,
IntBuffer ihi, DoubleBuffer scale, DoubleBuffer abnrm,
DoubleBuffer rconde, DoubleBuffer rcondv, DoubleBuffer work,
int lwork, IntBuffer iwork );
public static native int LAPACKE_dgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, double[] a,
int lda, double[] wr, double[] wi,
double[] vl, int ldvl, double[] vr,
int ldvr, int[] ilo,
int[] ihi, double[] scale, double[] abnrm,
double[] rconde, double[] rcondv, double[] work,
int lwork, int[] iwork );
public static native int LAPACKE_cgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
IntPointer ilo, IntPointer ihi, FloatPointer scale,
FloatPointer abnrm, FloatPointer rconde, FloatPointer rcondv,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork );
public static native int LAPACKE_cgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
IntBuffer ilo, IntBuffer ihi, FloatBuffer scale,
FloatBuffer abnrm, FloatBuffer rconde, FloatBuffer rcondv,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork );
public static native int LAPACKE_cgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
int[] ilo, int[] ihi, float[] scale,
float[] abnrm, float[] rconde, float[] rcondv,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork );
public static native int LAPACKE_zgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
IntPointer ilo, IntPointer ihi, DoublePointer scale,
DoublePointer abnrm, DoublePointer rconde, DoublePointer rcondv,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork );
public static native int LAPACKE_zgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer scale,
DoubleBuffer abnrm, DoubleBuffer rconde, DoubleBuffer rcondv,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork );
public static native int LAPACKE_zgeevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
int[] ilo, int[] ihi, double[] scale,
double[] abnrm, double[] rconde, double[] rcondv,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork );
public static native int LAPACKE_sgehrd_work( int matrix_layout, int n, int ilo,
int ihi, FloatPointer a, int lda,
FloatPointer tau, FloatPointer work, int lwork );
public static native int LAPACKE_sgehrd_work( int matrix_layout, int n, int ilo,
int ihi, FloatBuffer a, int lda,
FloatBuffer tau, FloatBuffer work, int lwork );
public static native int LAPACKE_sgehrd_work( int matrix_layout, int n, int ilo,
int ihi, float[] a, int lda,
float[] tau, float[] work, int lwork );
public static native int LAPACKE_dgehrd_work( int matrix_layout, int n, int ilo,
int ihi, DoublePointer a, int lda,
DoublePointer tau, DoublePointer work, int lwork );
public static native int LAPACKE_dgehrd_work( int matrix_layout, int n, int ilo,
int ihi, DoubleBuffer a, int lda,
DoubleBuffer tau, DoubleBuffer work, int lwork );
public static native int LAPACKE_dgehrd_work( int matrix_layout, int n, int ilo,
int ihi, double[] a, int lda,
double[] tau, double[] work, int lwork );
public static native int LAPACKE_cgehrd_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgehrd_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgehrd_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgehrd_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zgehrd_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zgehrd_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, FloatPointer a,
int lda, FloatPointer sva, FloatPointer u,
int ldu, FloatPointer v, int ldv,
FloatPointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_sgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, FloatBuffer a,
int lda, FloatBuffer sva, FloatBuffer u,
int ldu, FloatBuffer v, int ldv,
FloatBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_sgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, float[] a,
int lda, float[] sva, float[] u,
int ldu, float[] v, int ldv,
float[] work, int lwork,
int[] iwork );
public static native int LAPACKE_dgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, DoublePointer a,
int lda, DoublePointer sva, DoublePointer u,
int ldu, DoublePointer v, int ldv,
DoublePointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_dgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, DoubleBuffer a,
int lda, DoubleBuffer sva, DoubleBuffer u,
int ldu, DoubleBuffer v, int ldv,
DoubleBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_dgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, double[] a,
int lda, double[] sva, double[] u,
int ldu, double[] v, int ldv,
double[] work, int lwork,
int[] iwork );
public static native int LAPACKE_cgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, FloatPointer sva, @Cast("lapack_complex_float*") FloatPointer u,
int ldu, @Cast("lapack_complex_float*") FloatPointer v, int ldv,
@Cast("lapack_complex_float*") FloatPointer cwork, int lwork,
FloatPointer work, int lrwork,
IntPointer iwork );
public static native int LAPACKE_cgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer sva, @Cast("lapack_complex_float*") FloatBuffer u,
int ldu, @Cast("lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("lapack_complex_float*") FloatBuffer cwork, int lwork,
FloatBuffer work, int lrwork,
IntBuffer iwork );
public static native int LAPACKE_cgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, @Cast("lapack_complex_float*") float[] a,
int lda, float[] sva, @Cast("lapack_complex_float*") float[] u,
int ldu, @Cast("lapack_complex_float*") float[] v, int ldv,
@Cast("lapack_complex_float*") float[] cwork, int lwork,
float[] work, int lrwork,
int[] iwork );
public static native int LAPACKE_zgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, DoublePointer sva, @Cast("lapack_complex_double*") DoublePointer u,
int ldu, @Cast("lapack_complex_double*") DoublePointer v, int ldv,
@Cast("lapack_complex_double*") DoublePointer cwork, int lwork,
DoublePointer work, int lrwork,
IntPointer iwork );
public static native int LAPACKE_zgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer sva, @Cast("lapack_complex_double*") DoubleBuffer u,
int ldu, @Cast("lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("lapack_complex_double*") DoubleBuffer cwork, int lwork,
DoubleBuffer work, int lrwork,
IntBuffer iwork );
public static native int LAPACKE_zgejsv_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, @Cast("char") byte jobr, @Cast("char") byte jobt, @Cast("char") byte jobp,
int m, int n, @Cast("lapack_complex_double*") double[] a,
int lda, double[] sva, @Cast("lapack_complex_double*") double[] u,
int ldu, @Cast("lapack_complex_double*") double[] v, int ldv,
@Cast("lapack_complex_double*") double[] cwork, int lwork,
double[] work, int lrwork,
int[] iwork );
public static native int LAPACKE_sgelq2_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau,
FloatPointer work );
public static native int LAPACKE_sgelq2_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau,
FloatBuffer work );
public static native int LAPACKE_sgelq2_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau,
float[] work );
public static native int LAPACKE_dgelq2_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau,
DoublePointer work );
public static native int LAPACKE_dgelq2_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau,
DoubleBuffer work );
public static native int LAPACKE_dgelq2_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau,
double[] work );
public static native int LAPACKE_cgelq2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_cgelq2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_cgelq2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zgelq2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zgelq2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zgelq2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_sgelqf_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau,
FloatPointer work, int lwork );
public static native int LAPACKE_sgelqf_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau,
FloatBuffer work, int lwork );
public static native int LAPACKE_sgelqf_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau,
float[] work, int lwork );
public static native int LAPACKE_dgelqf_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau,
DoublePointer work, int lwork );
public static native int LAPACKE_dgelqf_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgelqf_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau,
double[] work, int lwork );
public static native int LAPACKE_cgelqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgelqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgelqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgelqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zgelqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zgelqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, FloatPointer a,
int lda, FloatPointer b, int ldb,
FloatPointer work, int lwork );
public static native int LAPACKE_sgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
FloatBuffer work, int lwork );
public static native int LAPACKE_sgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, float[] a,
int lda, float[] b, int ldb,
float[] work, int lwork );
public static native int LAPACKE_dgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, DoublePointer a,
int lda, DoublePointer b, int ldb,
DoublePointer work, int lwork );
public static native int LAPACKE_dgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs, double[] a,
int lda, double[] b, int ldb,
double[] work, int lwork );
public static native int LAPACKE_cgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zgels_work( int matrix_layout, @Cast("char") byte trans, int m,
int n, int nrhs,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgelsd_work( int matrix_layout, int m, int n,
int nrhs, FloatPointer a, int lda,
FloatPointer b, int ldb, FloatPointer s, float rcond,
IntPointer rank, FloatPointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_sgelsd_work( int matrix_layout, int m, int n,
int nrhs, FloatBuffer a, int lda,
FloatBuffer b, int ldb, FloatBuffer s, float rcond,
IntBuffer rank, FloatBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_sgelsd_work( int matrix_layout, int m, int n,
int nrhs, float[] a, int lda,
float[] b, int ldb, float[] s, float rcond,
int[] rank, float[] work, int lwork,
int[] iwork );
public static native int LAPACKE_dgelsd_work( int matrix_layout, int m, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer s,
double rcond, IntPointer rank, DoublePointer work,
int lwork, IntPointer iwork );
public static native int LAPACKE_dgelsd_work( int matrix_layout, int m, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer s,
double rcond, IntBuffer rank, DoubleBuffer work,
int lwork, IntBuffer iwork );
public static native int LAPACKE_dgelsd_work( int matrix_layout, int m, int n,
int nrhs, double[] a, int lda,
double[] b, int ldb, double[] s,
double rcond, int[] rank, double[] work,
int lwork, int[] iwork );
public static native int LAPACKE_cgelsd_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, FloatPointer s, float rcond,
IntPointer rank, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork,
IntPointer iwork );
public static native int LAPACKE_cgelsd_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, FloatBuffer s, float rcond,
IntBuffer rank, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork,
IntBuffer iwork );
public static native int LAPACKE_cgelsd_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, float[] s, float rcond,
int[] rank, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork,
int[] iwork );
public static native int LAPACKE_zgelsd_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, DoublePointer s, double rcond,
IntPointer rank, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork,
IntPointer iwork );
public static native int LAPACKE_zgelsd_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, DoubleBuffer s, double rcond,
IntBuffer rank, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork,
IntBuffer iwork );
public static native int LAPACKE_zgelsd_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, double[] s, double rcond,
int[] rank, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork,
int[] iwork );
public static native int LAPACKE_sgelss_work( int matrix_layout, int m, int n,
int nrhs, FloatPointer a, int lda,
FloatPointer b, int ldb, FloatPointer s, float rcond,
IntPointer rank, FloatPointer work,
int lwork );
public static native int LAPACKE_sgelss_work( int matrix_layout, int m, int n,
int nrhs, FloatBuffer a, int lda,
FloatBuffer b, int ldb, FloatBuffer s, float rcond,
IntBuffer rank, FloatBuffer work,
int lwork );
public static native int LAPACKE_sgelss_work( int matrix_layout, int m, int n,
int nrhs, float[] a, int lda,
float[] b, int ldb, float[] s, float rcond,
int[] rank, float[] work,
int lwork );
public static native int LAPACKE_dgelss_work( int matrix_layout, int m, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer s,
double rcond, IntPointer rank, DoublePointer work,
int lwork );
public static native int LAPACKE_dgelss_work( int matrix_layout, int m, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer s,
double rcond, IntBuffer rank, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dgelss_work( int matrix_layout, int m, int n,
int nrhs, double[] a, int lda,
double[] b, int ldb, double[] s,
double rcond, int[] rank, double[] work,
int lwork );
public static native int LAPACKE_cgelss_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, FloatPointer s, float rcond,
IntPointer rank, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork );
public static native int LAPACKE_cgelss_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, FloatBuffer s, float rcond,
IntBuffer rank, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork );
public static native int LAPACKE_cgelss_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, float[] s, float rcond,
int[] rank, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork );
public static native int LAPACKE_zgelss_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, DoublePointer s, double rcond,
IntPointer rank, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork );
public static native int LAPACKE_zgelss_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, DoubleBuffer s, double rcond,
IntBuffer rank, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork );
public static native int LAPACKE_zgelss_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, double[] s, double rcond,
int[] rank, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork );
public static native int LAPACKE_sgelsy_work( int matrix_layout, int m, int n,
int nrhs, FloatPointer a, int lda,
FloatPointer b, int ldb, IntPointer jpvt,
float rcond, IntPointer rank, FloatPointer work,
int lwork );
public static native int LAPACKE_sgelsy_work( int matrix_layout, int m, int n,
int nrhs, FloatBuffer a, int lda,
FloatBuffer b, int ldb, IntBuffer jpvt,
float rcond, IntBuffer rank, FloatBuffer work,
int lwork );
public static native int LAPACKE_sgelsy_work( int matrix_layout, int m, int n,
int nrhs, float[] a, int lda,
float[] b, int ldb, int[] jpvt,
float rcond, int[] rank, float[] work,
int lwork );
public static native int LAPACKE_dgelsy_work( int matrix_layout, int m, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer b, int ldb, IntPointer jpvt,
double rcond, IntPointer rank, DoublePointer work,
int lwork );
public static native int LAPACKE_dgelsy_work( int matrix_layout, int m, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, IntBuffer jpvt,
double rcond, IntBuffer rank, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dgelsy_work( int matrix_layout, int m, int n,
int nrhs, double[] a, int lda,
double[] b, int ldb, int[] jpvt,
double rcond, int[] rank, double[] work,
int lwork );
public static native int LAPACKE_cgelsy_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, IntPointer jpvt, float rcond,
IntPointer rank, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork );
public static native int LAPACKE_cgelsy_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, IntBuffer jpvt, float rcond,
IntBuffer rank, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork );
public static native int LAPACKE_cgelsy_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, int[] jpvt, float rcond,
int[] rank, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork );
public static native int LAPACKE_zgelsy_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, IntPointer jpvt, double rcond,
IntPointer rank, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork );
public static native int LAPACKE_zgelsy_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, IntBuffer jpvt, double rcond,
IntBuffer rank, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork );
public static native int LAPACKE_zgelsy_work( int matrix_layout, int m, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, int[] jpvt, double rcond,
int[] rank, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork );
public static native int LAPACKE_sgeqlf_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau,
FloatPointer work, int lwork );
public static native int LAPACKE_sgeqlf_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau,
FloatBuffer work, int lwork );
public static native int LAPACKE_sgeqlf_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau,
float[] work, int lwork );
public static native int LAPACKE_dgeqlf_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau,
DoublePointer work, int lwork );
public static native int LAPACKE_dgeqlf_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgeqlf_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau,
double[] work, int lwork );
public static native int LAPACKE_cgeqlf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgeqlf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgeqlf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgeqlf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zgeqlf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zgeqlf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgeqp3_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, IntPointer jpvt,
FloatPointer tau, FloatPointer work, int lwork );
public static native int LAPACKE_sgeqp3_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, IntBuffer jpvt,
FloatBuffer tau, FloatBuffer work, int lwork );
public static native int LAPACKE_sgeqp3_work( int matrix_layout, int m, int n,
float[] a, int lda, int[] jpvt,
float[] tau, float[] work, int lwork );
public static native int LAPACKE_dgeqp3_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, IntPointer jpvt,
DoublePointer tau, DoublePointer work, int lwork );
public static native int LAPACKE_dgeqp3_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, IntBuffer jpvt,
DoubleBuffer tau, DoubleBuffer work, int lwork );
public static native int LAPACKE_dgeqp3_work( int matrix_layout, int m, int n,
double[] a, int lda, int[] jpvt,
double[] tau, double[] work, int lwork );
public static native int LAPACKE_cgeqp3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer jpvt, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork );
public static native int LAPACKE_cgeqp3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer jpvt, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork );
public static native int LAPACKE_cgeqp3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] jpvt, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork );
public static native int LAPACKE_zgeqp3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer jpvt, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork );
public static native int LAPACKE_zgeqp3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer jpvt, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork );
public static native int LAPACKE_zgeqp3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] jpvt, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork );
public static native int LAPACKE_sgeqr2_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau,
FloatPointer work );
public static native int LAPACKE_sgeqr2_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau,
FloatBuffer work );
public static native int LAPACKE_sgeqr2_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau,
float[] work );
public static native int LAPACKE_dgeqr2_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau,
DoublePointer work );
public static native int LAPACKE_dgeqr2_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau,
DoubleBuffer work );
public static native int LAPACKE_dgeqr2_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau,
double[] work );
public static native int LAPACKE_cgeqr2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_cgeqr2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_cgeqr2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zgeqr2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zgeqr2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zgeqr2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_sgeqrf_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau,
FloatPointer work, int lwork );
public static native int LAPACKE_sgeqrf_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau,
FloatBuffer work, int lwork );
public static native int LAPACKE_sgeqrf_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau,
float[] work, int lwork );
public static native int LAPACKE_dgeqrf_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau,
DoublePointer work, int lwork );
public static native int LAPACKE_dgeqrf_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgeqrf_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau,
double[] work, int lwork );
public static native int LAPACKE_cgeqrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgeqrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgeqrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgeqrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zgeqrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zgeqrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgeqrfp_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau,
FloatPointer work, int lwork );
public static native int LAPACKE_sgeqrfp_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau,
FloatBuffer work, int lwork );
public static native int LAPACKE_sgeqrfp_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau,
float[] work, int lwork );
public static native int LAPACKE_dgeqrfp_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau,
DoublePointer work, int lwork );
public static native int LAPACKE_dgeqrfp_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgeqrfp_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau,
double[] work, int lwork );
public static native int LAPACKE_cgeqrfp_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgeqrfp_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgeqrfp_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgeqrfp_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work,
int lwork );
public static native int LAPACKE_zgeqrfp_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work,
int lwork );
public static native int LAPACKE_zgeqrfp_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work,
int lwork );
public static native int LAPACKE_sgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const FloatPointer af, int ldaf,
@Const IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_sgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const FloatBuffer af, int ldaf,
@Const IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_sgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const float[] a, int lda,
@Const float[] af, int ldaf,
@Const int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr, float[] work,
int[] iwork );
public static native int LAPACKE_dgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoublePointer a,
int lda, @Const DoublePointer af,
int ldaf, @Const IntPointer ipiv,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoubleBuffer a,
int lda, @Const DoubleBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const double[] a,
int lda, @Const double[] af,
int ldaf, @Const int[] ipiv,
@Const double[] b, int ldb, double[] x,
int ldx, double[] ferr, double[] berr,
double[] work, int[] iwork );
public static native int LAPACKE_cgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zgerfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sgerqf_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau,
FloatPointer work, int lwork );
public static native int LAPACKE_sgerqf_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau,
FloatBuffer work, int lwork );
public static native int LAPACKE_sgerqf_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau,
float[] work, int lwork );
public static native int LAPACKE_dgerqf_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau,
DoublePointer work, int lwork );
public static native int LAPACKE_dgerqf_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgerqf_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau,
double[] work, int lwork );
public static native int LAPACKE_cgerqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgerqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgerqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgerqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zgerqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zgerqf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, FloatPointer a, int lda,
FloatPointer s, FloatPointer u, int ldu, FloatPointer vt,
int ldvt, FloatPointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_sgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer u, int ldu, FloatBuffer vt,
int ldvt, FloatBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_sgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, float[] a, int lda,
float[] s, float[] u, int ldu, float[] vt,
int ldvt, float[] work, int lwork,
int[] iwork );
public static native int LAPACKE_dgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, DoublePointer a, int lda,
DoublePointer s, DoublePointer u, int ldu,
DoublePointer vt, int ldvt, DoublePointer work,
int lwork, IntPointer iwork );
public static native int LAPACKE_dgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, DoubleBuffer a, int lda,
DoubleBuffer s, DoubleBuffer u, int ldu,
DoubleBuffer vt, int ldvt, DoubleBuffer work,
int lwork, IntBuffer iwork );
public static native int LAPACKE_dgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, double[] a, int lda,
double[] s, double[] u, int ldu,
double[] vt, int ldvt, double[] work,
int lwork, int[] iwork );
public static native int LAPACKE_cgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, FloatPointer s,
@Cast("lapack_complex_float*") FloatPointer u, int ldu,
@Cast("lapack_complex_float*") FloatPointer vt, int ldvt,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, IntPointer iwork );
public static native int LAPACKE_cgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer s,
@Cast("lapack_complex_float*") FloatBuffer u, int ldu,
@Cast("lapack_complex_float*") FloatBuffer vt, int ldvt,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, IntBuffer iwork );
public static native int LAPACKE_cgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, float[] s,
@Cast("lapack_complex_float*") float[] u, int ldu,
@Cast("lapack_complex_float*") float[] vt, int ldvt,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int[] iwork );
public static native int LAPACKE_zgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, DoublePointer s,
@Cast("lapack_complex_double*") DoublePointer u, int ldu,
@Cast("lapack_complex_double*") DoublePointer vt, int ldvt,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, IntPointer iwork );
public static native int LAPACKE_zgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer s,
@Cast("lapack_complex_double*") DoubleBuffer u, int ldu,
@Cast("lapack_complex_double*") DoubleBuffer vt, int ldvt,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, IntBuffer iwork );
public static native int LAPACKE_zgesdd_work( int matrix_layout, @Cast("char") byte jobz, int m,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, double[] s,
@Cast("lapack_complex_double*") double[] u, int ldu,
@Cast("lapack_complex_double*") double[] vt, int ldvt,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int[] iwork );
public static native int LAPACKE_sgesv_work( int matrix_layout, int n, int nrhs,
FloatPointer a, int lda, IntPointer ipiv,
FloatPointer b, int ldb );
public static native int LAPACKE_sgesv_work( int matrix_layout, int n, int nrhs,
FloatBuffer a, int lda, IntBuffer ipiv,
FloatBuffer b, int ldb );
public static native int LAPACKE_sgesv_work( int matrix_layout, int n, int nrhs,
float[] a, int lda, int[] ipiv,
float[] b, int ldb );
public static native int LAPACKE_dgesv_work( int matrix_layout, int n, int nrhs,
DoublePointer a, int lda, IntPointer ipiv,
DoublePointer b, int ldb );
public static native int LAPACKE_dgesv_work( int matrix_layout, int n, int nrhs,
DoubleBuffer a, int lda, IntBuffer ipiv,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dgesv_work( int matrix_layout, int n, int nrhs,
double[] a, int lda, int[] ipiv,
double[] b, int ldb );
public static native int LAPACKE_cgesv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cgesv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cgesv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zgesv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zgesv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zgesv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_dsgesv_work( int matrix_layout, int n, int nrhs,
DoublePointer a, int lda, IntPointer ipiv,
DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer work, FloatPointer swork,
IntPointer iter );
public static native int LAPACKE_dsgesv_work( int matrix_layout, int n, int nrhs,
DoubleBuffer a, int lda, IntBuffer ipiv,
DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer work, FloatBuffer swork,
IntBuffer iter );
public static native int LAPACKE_dsgesv_work( int matrix_layout, int n, int nrhs,
double[] a, int lda, int[] ipiv,
double[] b, int ldb, double[] x,
int ldx, double[] work, float[] swork,
int[] iter );
public static native int LAPACKE_zcgesv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, @Cast("lapack_complex_double*") DoublePointer work,
@Cast("lapack_complex_float*") FloatPointer swork, DoublePointer rwork,
IntPointer iter );
public static native int LAPACKE_zcgesv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, @Cast("lapack_complex_double*") DoubleBuffer work,
@Cast("lapack_complex_float*") FloatBuffer swork, DoubleBuffer rwork,
IntBuffer iter );
public static native int LAPACKE_zcgesv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, @Cast("lapack_complex_double*") double[] work,
@Cast("lapack_complex_float*") float[] swork, double[] rwork,
int[] iter );
public static native int LAPACKE_sgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, FloatPointer a,
int lda, FloatPointer s, FloatPointer u,
int ldu, FloatPointer vt, int ldvt,
FloatPointer work, int lwork );
public static native int LAPACKE_sgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, FloatBuffer a,
int lda, FloatBuffer s, FloatBuffer u,
int ldu, FloatBuffer vt, int ldvt,
FloatBuffer work, int lwork );
public static native int LAPACKE_sgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, float[] a,
int lda, float[] s, float[] u,
int ldu, float[] vt, int ldvt,
float[] work, int lwork );
public static native int LAPACKE_dgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, DoublePointer a,
int lda, DoublePointer s, DoublePointer u,
int ldu, DoublePointer vt, int ldvt,
DoublePointer work, int lwork );
public static native int LAPACKE_dgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, DoubleBuffer a,
int lda, DoubleBuffer s, DoubleBuffer u,
int ldu, DoubleBuffer vt, int ldvt,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n, double[] a,
int lda, double[] s, double[] u,
int ldu, double[] vt, int ldvt,
double[] work, int lwork );
public static native int LAPACKE_cgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
FloatPointer s, @Cast("lapack_complex_float*") FloatPointer u,
int ldu, @Cast("lapack_complex_float*") FloatPointer vt,
int ldvt, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork );
public static native int LAPACKE_cgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer u,
int ldu, @Cast("lapack_complex_float*") FloatBuffer vt,
int ldvt, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork );
public static native int LAPACKE_cgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
float[] s, @Cast("lapack_complex_float*") float[] u,
int ldu, @Cast("lapack_complex_float*") float[] vt,
int ldvt, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork );
public static native int LAPACKE_zgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
DoublePointer s, @Cast("lapack_complex_double*") DoublePointer u,
int ldu, @Cast("lapack_complex_double*") DoublePointer vt,
int ldvt, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork );
public static native int LAPACKE_zgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer s, @Cast("lapack_complex_double*") DoubleBuffer u,
int ldu, @Cast("lapack_complex_double*") DoubleBuffer vt,
int ldvt, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork );
public static native int LAPACKE_zgesvd_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt,
int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
double[] s, @Cast("lapack_complex_double*") double[] u,
int ldu, @Cast("lapack_complex_double*") double[] vt,
int ldvt, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork );
public static native int LAPACKE_sgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, FloatPointer a,
int lda, int vl, int vu,
int il, int iu, int ns,
FloatPointer s, FloatPointer u, int ldu,
FloatPointer vt, int ldvt,
FloatPointer work, int lwork, IntPointer iwork );
public static native int LAPACKE_sgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, FloatBuffer a,
int lda, int vl, int vu,
int il, int iu, int ns,
FloatBuffer s, FloatBuffer u, int ldu,
FloatBuffer vt, int ldvt,
FloatBuffer work, int lwork, IntBuffer iwork );
public static native int LAPACKE_sgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, float[] a,
int lda, int vl, int vu,
int il, int iu, int ns,
float[] s, float[] u, int ldu,
float[] vt, int ldvt,
float[] work, int lwork, int[] iwork );
public static native int LAPACKE_dgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, DoublePointer a,
int lda, int vl, int vu,
int il, int iu, int ns,
DoublePointer s, DoublePointer u, int ldu,
DoublePointer vt, int ldvt,
DoublePointer work, int lwork, IntPointer iwork );
public static native int LAPACKE_dgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, DoubleBuffer a,
int lda, int vl, int vu,
int il, int iu, int ns,
DoubleBuffer s, DoubleBuffer u, int ldu,
DoubleBuffer vt, int ldvt,
DoubleBuffer work, int lwork, IntBuffer iwork );
public static native int LAPACKE_dgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, double[] a,
int lda, int vl, int vu,
int il, int iu, int ns,
double[] s, double[] u, int ldu,
double[] vt, int ldvt,
double[] work, int lwork, int[] iwork );
public static native int LAPACKE_cgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, int vl, int vu,
int il, int iu, int ns,
FloatPointer s, @Cast("lapack_complex_float*") FloatPointer u, int ldu,
@Cast("lapack_complex_float*") FloatPointer vt, int ldvt,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, IntPointer iwork );
public static native int LAPACKE_cgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, int vl, int vu,
int il, int iu, int ns,
FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer u, int ldu,
@Cast("lapack_complex_float*") FloatBuffer vt, int ldvt,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, IntBuffer iwork );
public static native int LAPACKE_cgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_float*") float[] a,
int lda, int vl, int vu,
int il, int iu, int ns,
float[] s, @Cast("lapack_complex_float*") float[] u, int ldu,
@Cast("lapack_complex_float*") float[] vt, int ldvt,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int[] iwork );
public static native int LAPACKE_zgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, int vl, int vu,
int il, int iu, int ns,
DoublePointer s, @Cast("lapack_complex_double*") DoublePointer u, int ldu,
@Cast("lapack_complex_double*") DoublePointer vt, int ldvt,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, IntPointer iwork );
public static native int LAPACKE_zgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, int vl, int vu,
int il, int iu, int ns,
DoubleBuffer s, @Cast("lapack_complex_double*") DoubleBuffer u, int ldu,
@Cast("lapack_complex_double*") DoubleBuffer vt, int ldvt,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, IntBuffer iwork );
public static native int LAPACKE_zgesvdx_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobvt, @Cast("char") byte range,
int m, int n, @Cast("lapack_complex_double*") double[] a,
int lda, int vl, int vu,
int il, int iu, int ns,
double[] s, @Cast("lapack_complex_double*") double[] u, int ldu,
@Cast("lapack_complex_double*") double[] vt, int ldvt,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int[] iwork );
public static native int LAPACKE_sgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n, FloatPointer a,
int lda, FloatPointer sva, int mv,
FloatPointer v, int ldv, FloatPointer work,
int lwork );
public static native int LAPACKE_sgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n, FloatBuffer a,
int lda, FloatBuffer sva, int mv,
FloatBuffer v, int ldv, FloatBuffer work,
int lwork );
public static native int LAPACKE_sgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n, float[] a,
int lda, float[] sva, int mv,
float[] v, int ldv, float[] work,
int lwork );
public static native int LAPACKE_dgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n,
DoublePointer a, int lda, DoublePointer sva,
int mv, DoublePointer v, int ldv,
DoublePointer work, int lwork );
public static native int LAPACKE_dgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer sva,
int mv, DoubleBuffer v, int ldv,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n,
double[] a, int lda, double[] sva,
int mv, double[] v, int ldv,
double[] work, int lwork );
public static native int LAPACKE_cgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, FloatPointer sva, int mv,
@Cast("lapack_complex_float*") FloatPointer v, int ldv,
@Cast("lapack_complex_float*") FloatPointer cwork, int lwork,
FloatPointer rwork,int lrwork );
public static native int LAPACKE_cgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer sva, int mv,
@Cast("lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("lapack_complex_float*") FloatBuffer cwork, int lwork,
FloatBuffer rwork,int lrwork );
public static native int LAPACKE_cgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n, @Cast("lapack_complex_float*") float[] a,
int lda, float[] sva, int mv,
@Cast("lapack_complex_float*") float[] v, int ldv,
@Cast("lapack_complex_float*") float[] cwork, int lwork,
float[] rwork,int lrwork );
public static native int LAPACKE_zgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda, DoublePointer sva,
int mv, @Cast("lapack_complex_double*") DoublePointer v, int ldv,
@Cast("lapack_complex_double*") DoublePointer cwork, int lwork,
DoublePointer rwork, int lrwork );
public static native int LAPACKE_zgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda, DoubleBuffer sva,
int mv, @Cast("lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("lapack_complex_double*") DoubleBuffer cwork, int lwork,
DoubleBuffer rwork, int lrwork );
public static native int LAPACKE_zgesvj_work( int matrix_layout, @Cast("char") byte joba, @Cast("char") byte jobu,
@Cast("char") byte jobv, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda, double[] sva,
int mv, @Cast("lapack_complex_double*") double[] v, int ldv,
@Cast("lapack_complex_double*") double[] cwork, int lwork,
double[] rwork, int lrwork );
public static native int LAPACKE_sgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, FloatPointer a,
int lda, FloatPointer af, int ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, FloatPointer r,
FloatPointer c, FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, FloatBuffer a,
int lda, FloatBuffer af, int ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, FloatBuffer r,
FloatBuffer c, FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, float[] a,
int lda, float[] af, int ldaf,
int[] ipiv, @Cast("char*") byte[] equed, float[] r,
float[] c, float[] b, int ldb, float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, float[] work, int[] iwork );
public static native int LAPACKE_dgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, DoublePointer a,
int lda, DoublePointer af, int ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, DoublePointer r,
DoublePointer c, DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, DoubleBuffer a,
int lda, DoubleBuffer af, int ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, DoubleBuffer r,
DoubleBuffer c, DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, double[] a,
int lda, double[] af, int ldaf,
int[] ipiv, @Cast("char*") byte[] equed, double[] r,
double[] c, double[] b, int ldb, double[] x,
int ldx, double[] rcond, double[] ferr,
double[] berr, double[] work, int[] iwork );
public static native int LAPACKE_cgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer af, int ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, FloatPointer r,
FloatPointer c, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_cgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer af, int ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, FloatBuffer r,
FloatBuffer c, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_cgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] af, int ldaf,
int[] ipiv, @Cast("char*") byte[] equed, float[] r,
float[] c, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_zgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer af, int ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, DoublePointer r,
DoublePointer c, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork );
public static native int LAPACKE_zgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer af, int ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, DoubleBuffer r,
DoubleBuffer c, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork );
public static native int LAPACKE_zgesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] af, int ldaf,
int[] ipiv, @Cast("char*") byte[] equed, double[] r,
double[] c, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, double[] rcond, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork );
public static native int LAPACKE_sgetf2_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_sgetf2_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_sgetf2_work( int matrix_layout, int m, int n,
float[] a, int lda, int[] ipiv );
public static native int LAPACKE_dgetf2_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_dgetf2_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_dgetf2_work( int matrix_layout, int m, int n,
double[] a, int lda, int[] ipiv );
public static native int LAPACKE_cgetf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_cgetf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_cgetf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zgetf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zgetf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zgetf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_sgetrf_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_sgetrf_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_sgetrf_work( int matrix_layout, int m, int n,
float[] a, int lda, int[] ipiv );
public static native int LAPACKE_dgetrf_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_dgetrf_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_dgetrf_work( int matrix_layout, int m, int n,
double[] a, int lda, int[] ipiv );
public static native int LAPACKE_cgetrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_cgetrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_cgetrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zgetrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zgetrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zgetrf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_sgetrf2_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_sgetrf2_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_sgetrf2_work( int matrix_layout, int m, int n,
float[] a, int lda, int[] ipiv );
public static native int LAPACKE_dgetrf2_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, IntPointer ipiv );
public static native int LAPACKE_dgetrf2_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, IntBuffer ipiv );
public static native int LAPACKE_dgetrf2_work( int matrix_layout, int m, int n,
double[] a, int lda, int[] ipiv );
public static native int LAPACKE_cgetrf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_cgetrf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_cgetrf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zgetrf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zgetrf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zgetrf2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_sgetri_work( int matrix_layout, int n, FloatPointer a,
int lda, @Const IntPointer ipiv,
FloatPointer work, int lwork );
public static native int LAPACKE_sgetri_work( int matrix_layout, int n, FloatBuffer a,
int lda, @Const IntBuffer ipiv,
FloatBuffer work, int lwork );
public static native int LAPACKE_sgetri_work( int matrix_layout, int n, float[] a,
int lda, @Const int[] ipiv,
float[] work, int lwork );
public static native int LAPACKE_dgetri_work( int matrix_layout, int n, DoublePointer a,
int lda, @Const IntPointer ipiv,
DoublePointer work, int lwork );
public static native int LAPACKE_dgetri_work( int matrix_layout, int n, DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgetri_work( int matrix_layout, int n, double[] a,
int lda, @Const int[] ipiv,
double[] work, int lwork );
public static native int LAPACKE_cgetri_work( int matrix_layout, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgetri_work( int matrix_layout, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgetri_work( int matrix_layout, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgetri_work( int matrix_layout, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zgetri_work( int matrix_layout, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zgetri_work( int matrix_layout, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer b,
int ldb );
public static native int LAPACKE_sgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer b,
int ldb );
public static native int LAPACKE_sgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const float[] a, int lda,
@Const int[] ipiv, float[] b,
int ldb );
public static native int LAPACKE_dgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoublePointer a,
int lda, @Const IntPointer ipiv,
DoublePointer b, int ldb );
public static native int LAPACKE_dgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const double[] a,
int lda, @Const int[] ipiv,
double[] b, int ldb );
public static native int LAPACKE_cgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zgetrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const FloatPointer lscale, @Const FloatPointer rscale,
int m, FloatPointer v, int ldv );
public static native int LAPACKE_sggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const FloatBuffer lscale, @Const FloatBuffer rscale,
int m, FloatBuffer v, int ldv );
public static native int LAPACKE_sggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const float[] lscale, @Const float[] rscale,
int m, float[] v, int ldv );
public static native int LAPACKE_dggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const DoublePointer lscale, @Const DoublePointer rscale,
int m, DoublePointer v, int ldv );
public static native int LAPACKE_dggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const DoubleBuffer lscale, @Const DoubleBuffer rscale,
int m, DoubleBuffer v, int ldv );
public static native int LAPACKE_dggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const double[] lscale, @Const double[] rscale,
int m, double[] v, int ldv );
public static native int LAPACKE_cggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const FloatPointer lscale, @Const FloatPointer rscale,
int m, @Cast("lapack_complex_float*") FloatPointer v,
int ldv );
public static native int LAPACKE_cggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const FloatBuffer lscale, @Const FloatBuffer rscale,
int m, @Cast("lapack_complex_float*") FloatBuffer v,
int ldv );
public static native int LAPACKE_cggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const float[] lscale, @Const float[] rscale,
int m, @Cast("lapack_complex_float*") float[] v,
int ldv );
public static native int LAPACKE_zggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const DoublePointer lscale, @Const DoublePointer rscale,
int m, @Cast("lapack_complex_double*") DoublePointer v,
int ldv );
public static native int LAPACKE_zggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const DoubleBuffer lscale, @Const DoubleBuffer rscale,
int m, @Cast("lapack_complex_double*") DoubleBuffer v,
int ldv );
public static native int LAPACKE_zggbak_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte side,
int n, int ilo, int ihi,
@Const double[] lscale, @Const double[] rscale,
int m, @Cast("lapack_complex_double*") double[] v,
int ldv );
public static native int LAPACKE_sggbal_work( int matrix_layout, @Cast("char") byte job, int n,
FloatPointer a, int lda, FloatPointer b,
int ldb, IntPointer ilo,
IntPointer ihi, FloatPointer lscale, FloatPointer rscale,
FloatPointer work );
public static native int LAPACKE_sggbal_work( int matrix_layout, @Cast("char") byte job, int n,
FloatBuffer a, int lda, FloatBuffer b,
int ldb, IntBuffer ilo,
IntBuffer ihi, FloatBuffer lscale, FloatBuffer rscale,
FloatBuffer work );
public static native int LAPACKE_sggbal_work( int matrix_layout, @Cast("char") byte job, int n,
float[] a, int lda, float[] b,
int ldb, int[] ilo,
int[] ihi, float[] lscale, float[] rscale,
float[] work );
public static native int LAPACKE_dggbal_work( int matrix_layout, @Cast("char") byte job, int n,
DoublePointer a, int lda, DoublePointer b,
int ldb, IntPointer ilo,
IntPointer ihi, DoublePointer lscale, DoublePointer rscale,
DoublePointer work );
public static native int LAPACKE_dggbal_work( int matrix_layout, @Cast("char") byte job, int n,
DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, IntBuffer ilo,
IntBuffer ihi, DoubleBuffer lscale, DoubleBuffer rscale,
DoubleBuffer work );
public static native int LAPACKE_dggbal_work( int matrix_layout, @Cast("char") byte job, int n,
double[] a, int lda, double[] b,
int ldb, int[] ilo,
int[] ihi, double[] lscale, double[] rscale,
double[] work );
public static native int LAPACKE_cggbal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
IntPointer ilo, IntPointer ihi, FloatPointer lscale,
FloatPointer rscale, FloatPointer work );
public static native int LAPACKE_cggbal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
IntBuffer ilo, IntBuffer ihi, FloatBuffer lscale,
FloatBuffer rscale, FloatBuffer work );
public static native int LAPACKE_cggbal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
int[] ilo, int[] ihi, float[] lscale,
float[] rscale, float[] work );
public static native int LAPACKE_zggbal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
IntPointer ilo, IntPointer ihi,
DoublePointer lscale, DoublePointer rscale, DoublePointer work );
public static native int LAPACKE_zggbal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
IntBuffer ilo, IntBuffer ihi,
DoubleBuffer lscale, DoubleBuffer rscale, DoubleBuffer work );
public static native int LAPACKE_zggbal_work( int matrix_layout, @Cast("char") byte job, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
int[] ilo, int[] ihi,
double[] lscale, double[] rscale, double[] work );
public static native int LAPACKE_sgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, int n,
FloatPointer a, int lda, FloatPointer b,
int ldb, IntPointer sdim, FloatPointer alphar,
FloatPointer alphai, FloatPointer beta, FloatPointer vsl,
int ldvsl, FloatPointer vsr, int ldvsr,
FloatPointer work, int lwork,
IntPointer bwork );
public static native int LAPACKE_sgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, int n,
FloatBuffer a, int lda, FloatBuffer b,
int ldb, IntBuffer sdim, FloatBuffer alphar,
FloatBuffer alphai, FloatBuffer beta, FloatBuffer vsl,
int ldvsl, FloatBuffer vsr, int ldvsr,
FloatBuffer work, int lwork,
IntBuffer bwork );
public static native int LAPACKE_sgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, int n,
float[] a, int lda, float[] b,
int ldb, int[] sdim, float[] alphar,
float[] alphai, float[] beta, float[] vsl,
int ldvsl, float[] vsr, int ldvsr,
float[] work, int lwork,
int[] bwork );
public static native int LAPACKE_dgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, int n,
DoublePointer a, int lda, DoublePointer b,
int ldb, IntPointer sdim, DoublePointer alphar,
DoublePointer alphai, DoublePointer beta, DoublePointer vsl,
int ldvsl, DoublePointer vsr, int ldvsr,
DoublePointer work, int lwork,
IntPointer bwork );
public static native int LAPACKE_dgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, int n,
DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, IntBuffer sdim, DoubleBuffer alphar,
DoubleBuffer alphai, DoubleBuffer beta, DoubleBuffer vsl,
int ldvsl, DoubleBuffer vsr, int ldvsr,
DoubleBuffer work, int lwork,
IntBuffer bwork );
public static native int LAPACKE_dgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, int n,
double[] a, int lda, double[] b,
int ldb, int[] sdim, double[] alphar,
double[] alphai, double[] beta, double[] vsl,
int ldvsl, double[] vsr, int ldvsr,
double[] work, int lwork,
int[] bwork );
public static native int LAPACKE_cgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
IntPointer sdim, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatPointer vsr, int ldvsr,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, IntPointer bwork );
public static native int LAPACKE_cgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
IntBuffer sdim, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatBuffer vsr, int ldvsr,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, IntBuffer bwork );
public static native int LAPACKE_cgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
int[] sdim, @Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vsl, int ldvsl,
@Cast("lapack_complex_float*") float[] vsr, int ldvsr,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int[] bwork );
public static native int LAPACKE_zgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
IntPointer sdim, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoublePointer vsr, int ldvsr,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, IntPointer bwork );
public static native int LAPACKE_zgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
IntBuffer sdim, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoubleBuffer vsr, int ldvsr,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, IntBuffer bwork );
public static native int LAPACKE_zgges_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
int[] sdim, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vsl, int ldvsl,
@Cast("lapack_complex_double*") double[] vsr, int ldvsr,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int[] bwork );
public static native int LAPACKE_sgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg,
int n,
FloatPointer a, int lda,
FloatPointer b, int ldb, IntPointer sdim,
FloatPointer alphar, FloatPointer alphai, FloatPointer beta,
FloatPointer vsl, int ldvsl,
FloatPointer vsr, int ldvsr,
FloatPointer work, int lwork,
IntPointer bwork );
public static native int LAPACKE_sgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg,
int n,
FloatBuffer a, int lda,
FloatBuffer b, int ldb, IntBuffer sdim,
FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta,
FloatBuffer vsl, int ldvsl,
FloatBuffer vsr, int ldvsr,
FloatBuffer work, int lwork,
IntBuffer bwork );
public static native int LAPACKE_sgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg,
int n,
float[] a, int lda,
float[] b, int ldb, int[] sdim,
float[] alphar, float[] alphai, float[] beta,
float[] vsl, int ldvsl,
float[] vsr, int ldvsr,
float[] work, int lwork,
int[] bwork );
public static native int LAPACKE_dgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg,
int n,
DoublePointer a, int lda,
DoublePointer b, int ldb, IntPointer sdim,
DoublePointer alphar, DoublePointer alphai, DoublePointer beta,
DoublePointer vsl, int ldvsl,
DoublePointer vsr, int ldvsr,
DoublePointer work, int lwork,
IntPointer bwork );
public static native int LAPACKE_dgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg,
int n,
DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, IntBuffer sdim,
DoubleBuffer alphar, DoubleBuffer alphai, DoubleBuffer beta,
DoubleBuffer vsl, int ldvsl,
DoubleBuffer vsr, int ldvsr,
DoubleBuffer work, int lwork,
IntBuffer bwork );
public static native int LAPACKE_dgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg,
int n,
double[] a, int lda,
double[] b, int ldb, int[] sdim,
double[] alphar, double[] alphai, double[] beta,
double[] vsl, int ldvsl,
double[] vsr, int ldvsr,
double[] work, int lwork,
int[] bwork );
public static native int LAPACKE_cgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg,
int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
IntPointer sdim, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatPointer vsr, int ldvsr,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, IntPointer bwork );
public static native int LAPACKE_cgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg,
int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
IntBuffer sdim, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatBuffer vsr, int ldvsr,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, IntBuffer bwork );
public static native int LAPACKE_cgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg,
int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
int[] sdim, @Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vsl, int ldvsl,
@Cast("lapack_complex_float*") float[] vsr, int ldvsr,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int[] bwork );
public static native int LAPACKE_zgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg,
int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
IntPointer sdim, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoublePointer vsr, int ldvsr,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, IntPointer bwork );
public static native int LAPACKE_zgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg,
int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
IntBuffer sdim, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoubleBuffer vsr, int ldvsr,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, IntBuffer bwork );
public static native int LAPACKE_zgges3_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg,
int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
int[] sdim, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vsl, int ldvsl,
@Cast("lapack_complex_double*") double[] vsr, int ldvsr,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int[] bwork );
public static native int LAPACKE_sggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, @Cast("char") byte sense,
int n, FloatPointer a, int lda,
FloatPointer b, int ldb, IntPointer sdim,
FloatPointer alphar, FloatPointer alphai, FloatPointer beta,
FloatPointer vsl, int ldvsl, FloatPointer vsr,
int ldvsr, FloatPointer rconde, FloatPointer rcondv,
FloatPointer work, int lwork,
IntPointer iwork, int liwork,
IntPointer bwork );
public static native int LAPACKE_sggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, @Cast("char") byte sense,
int n, FloatBuffer a, int lda,
FloatBuffer b, int ldb, IntBuffer sdim,
FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta,
FloatBuffer vsl, int ldvsl, FloatBuffer vsr,
int ldvsr, FloatBuffer rconde, FloatBuffer rcondv,
FloatBuffer work, int lwork,
IntBuffer iwork, int liwork,
IntBuffer bwork );
public static native int LAPACKE_sggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_S_SELECT3 selctg, @Cast("char") byte sense,
int n, float[] a, int lda,
float[] b, int ldb, int[] sdim,
float[] alphar, float[] alphai, float[] beta,
float[] vsl, int ldvsl, float[] vsr,
int ldvsr, float[] rconde, float[] rcondv,
float[] work, int lwork,
int[] iwork, int liwork,
int[] bwork );
public static native int LAPACKE_dggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, @Cast("char") byte sense,
int n, DoublePointer a, int lda,
DoublePointer b, int ldb, IntPointer sdim,
DoublePointer alphar, DoublePointer alphai, DoublePointer beta,
DoublePointer vsl, int ldvsl, DoublePointer vsr,
int ldvsr, DoublePointer rconde,
DoublePointer rcondv, DoublePointer work, int lwork,
IntPointer iwork, int liwork,
IntPointer bwork );
public static native int LAPACKE_dggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, @Cast("char") byte sense,
int n, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, IntBuffer sdim,
DoubleBuffer alphar, DoubleBuffer alphai, DoubleBuffer beta,
DoubleBuffer vsl, int ldvsl, DoubleBuffer vsr,
int ldvsr, DoubleBuffer rconde,
DoubleBuffer rcondv, DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork,
IntBuffer bwork );
public static native int LAPACKE_dggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_D_SELECT3 selctg, @Cast("char") byte sense,
int n, double[] a, int lda,
double[] b, int ldb, int[] sdim,
double[] alphar, double[] alphai, double[] beta,
double[] vsl, int ldvsl, double[] vsr,
int ldvsr, double[] rconde,
double[] rcondv, double[] work, int lwork,
int[] iwork, int liwork,
int[] bwork );
public static native int LAPACKE_cggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, IntPointer sdim,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatPointer vsr, int ldvsr,
FloatPointer rconde, FloatPointer rcondv,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, IntPointer iwork,
int liwork, IntPointer bwork );
public static native int LAPACKE_cggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, IntBuffer sdim,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vsl, int ldvsl,
@Cast("lapack_complex_float*") FloatBuffer vsr, int ldvsr,
FloatBuffer rconde, FloatBuffer rcondv,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, IntBuffer iwork,
int liwork, IntBuffer bwork );
public static native int LAPACKE_cggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_C_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, int[] sdim,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vsl, int ldvsl,
@Cast("lapack_complex_float*") float[] vsr, int ldvsr,
float[] rconde, float[] rcondv,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int[] iwork,
int liwork, int[] bwork );
public static native int LAPACKE_zggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, IntPointer sdim,
@Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoublePointer vsr, int ldvsr,
DoublePointer rconde, DoublePointer rcondv,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, IntPointer iwork,
int liwork, IntPointer bwork );
public static native int LAPACKE_zggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, IntBuffer sdim,
@Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vsl, int ldvsl,
@Cast("lapack_complex_double*") DoubleBuffer vsr, int ldvsr,
DoubleBuffer rconde, DoubleBuffer rcondv,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, IntBuffer iwork,
int liwork, IntBuffer bwork );
public static native int LAPACKE_zggesx_work( int matrix_layout, @Cast("char") byte jobvsl, @Cast("char") byte jobvsr,
@Cast("char") byte sort, LAPACK_Z_SELECT2 selctg, @Cast("char") byte sense,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, int[] sdim,
@Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vsl, int ldvsl,
@Cast("lapack_complex_double*") double[] vsr, int ldvsr,
double[] rconde, double[] rcondv,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int[] iwork,
int liwork, int[] bwork );
public static native int LAPACKE_sggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer alphar, FloatPointer alphai,
FloatPointer beta, FloatPointer vl, int ldvl,
FloatPointer vr, int ldvr, FloatPointer work,
int lwork );
public static native int LAPACKE_sggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer alphar, FloatBuffer alphai,
FloatBuffer beta, FloatBuffer vl, int ldvl,
FloatBuffer vr, int ldvr, FloatBuffer work,
int lwork );
public static native int LAPACKE_sggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, float[] a, int lda, float[] b,
int ldb, float[] alphar, float[] alphai,
float[] beta, float[] vl, int ldvl,
float[] vr, int ldvr, float[] work,
int lwork );
public static native int LAPACKE_dggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer alphar,
DoublePointer alphai, DoublePointer beta, DoublePointer vl,
int ldvl, DoublePointer vr, int ldvr,
DoublePointer work, int lwork );
public static native int LAPACKE_dggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer alphar,
DoubleBuffer alphai, DoubleBuffer beta, DoubleBuffer vl,
int ldvl, DoubleBuffer vr, int ldvr,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, double[] a, int lda,
double[] b, int ldb, double[] alphar,
double[] alphai, double[] beta, double[] vl,
int ldvl, double[] vr, int ldvr,
double[] work, int lwork );
public static native int LAPACKE_cggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork );
public static native int LAPACKE_cggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork );
public static native int LAPACKE_cggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork );
public static native int LAPACKE_zggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork );
public static native int LAPACKE_zggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork );
public static native int LAPACKE_zggev_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork );
public static native int LAPACKE_sggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
FloatPointer a, int lda,
FloatPointer b, int ldb,
FloatPointer alphar, FloatPointer alphai, FloatPointer beta,
FloatPointer vl, int ldvl,
FloatPointer vr, int ldvr,
FloatPointer work, int lwork );
public static native int LAPACKE_sggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
FloatBuffer a, int lda,
FloatBuffer b, int ldb,
FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta,
FloatBuffer vl, int ldvl,
FloatBuffer vr, int ldvr,
FloatBuffer work, int lwork );
public static native int LAPACKE_sggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
float[] a, int lda,
float[] b, int ldb,
float[] alphar, float[] alphai, float[] beta,
float[] vl, int ldvl,
float[] vr, int ldvr,
float[] work, int lwork );
public static native int LAPACKE_dggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
DoublePointer a, int lda,
DoublePointer b, int ldb,
DoublePointer alphar, DoublePointer alphai, DoublePointer beta,
DoublePointer vl, int ldvl,
DoublePointer vr, int ldvr,
DoublePointer work, int lwork );
public static native int LAPACKE_dggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
DoubleBuffer a, int lda,
DoubleBuffer b, int ldb,
DoubleBuffer alphar, DoubleBuffer alphai, DoubleBuffer beta,
DoubleBuffer vl, int ldvl,
DoubleBuffer vr, int ldvr,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
double[] a, int lda,
double[] b, int ldb,
double[] alphar, double[] alphai, double[] beta,
double[] vl, int ldvl,
double[] vr, int ldvr,
double[] work, int lwork );
public static native int LAPACKE_cggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork );
public static native int LAPACKE_cggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork );
public static native int LAPACKE_cggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork );
public static native int LAPACKE_zggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork );
public static native int LAPACKE_zggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork );
public static native int LAPACKE_zggev3_work( int matrix_layout, @Cast("char") byte jobvl, @Cast("char") byte jobvr,
int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork );
public static native int LAPACKE_sggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, FloatPointer a,
int lda, FloatPointer b, int ldb,
FloatPointer alphar, FloatPointer alphai, FloatPointer beta,
FloatPointer vl, int ldvl, FloatPointer vr,
int ldvr, IntPointer ilo,
IntPointer ihi, FloatPointer lscale, FloatPointer rscale,
FloatPointer abnrm, FloatPointer bbnrm, FloatPointer rconde,
FloatPointer rcondv, FloatPointer work, int lwork,
IntPointer iwork, IntPointer bwork );
public static native int LAPACKE_sggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta,
FloatBuffer vl, int ldvl, FloatBuffer vr,
int ldvr, IntBuffer ilo,
IntBuffer ihi, FloatBuffer lscale, FloatBuffer rscale,
FloatBuffer abnrm, FloatBuffer bbnrm, FloatBuffer rconde,
FloatBuffer rcondv, FloatBuffer work, int lwork,
IntBuffer iwork, IntBuffer bwork );
public static native int LAPACKE_sggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, float[] a,
int lda, float[] b, int ldb,
float[] alphar, float[] alphai, float[] beta,
float[] vl, int ldvl, float[] vr,
int ldvr, int[] ilo,
int[] ihi, float[] lscale, float[] rscale,
float[] abnrm, float[] bbnrm, float[] rconde,
float[] rcondv, float[] work, int lwork,
int[] iwork, int[] bwork );
public static native int LAPACKE_dggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, DoublePointer a,
int lda, DoublePointer b, int ldb,
DoublePointer alphar, DoublePointer alphai, DoublePointer beta,
DoublePointer vl, int ldvl, DoublePointer vr,
int ldvr, IntPointer ilo,
IntPointer ihi, DoublePointer lscale, DoublePointer rscale,
DoublePointer abnrm, DoublePointer bbnrm, DoublePointer rconde,
DoublePointer rcondv, DoublePointer work, int lwork,
IntPointer iwork, IntPointer bwork );
public static native int LAPACKE_dggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
DoubleBuffer alphar, DoubleBuffer alphai, DoubleBuffer beta,
DoubleBuffer vl, int ldvl, DoubleBuffer vr,
int ldvr, IntBuffer ilo,
IntBuffer ihi, DoubleBuffer lscale, DoubleBuffer rscale,
DoubleBuffer abnrm, DoubleBuffer bbnrm, DoubleBuffer rconde,
DoubleBuffer rcondv, DoubleBuffer work, int lwork,
IntBuffer iwork, IntBuffer bwork );
public static native int LAPACKE_dggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n, double[] a,
int lda, double[] b, int ldb,
double[] alphar, double[] alphai, double[] beta,
double[] vl, int ldvl, double[] vr,
int ldvr, int[] ilo,
int[] ihi, double[] lscale, double[] rscale,
double[] abnrm, double[] bbnrm, double[] rconde,
double[] rcondv, double[] work, int lwork,
int[] iwork, int[] bwork );
public static native int LAPACKE_cggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
IntPointer ilo, IntPointer ihi, FloatPointer lscale,
FloatPointer rscale, FloatPointer abnrm, FloatPointer bbnrm,
FloatPointer rconde, FloatPointer rcondv,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, IntPointer iwork,
IntPointer bwork );
public static native int LAPACKE_cggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
IntBuffer ilo, IntBuffer ihi, FloatBuffer lscale,
FloatBuffer rscale, FloatBuffer abnrm, FloatBuffer bbnrm,
FloatBuffer rconde, FloatBuffer rcondv,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, IntBuffer iwork,
IntBuffer bwork );
public static native int LAPACKE_cggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
int[] ilo, int[] ihi, float[] lscale,
float[] rscale, float[] abnrm, float[] bbnrm,
float[] rconde, float[] rcondv,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int[] iwork,
int[] bwork );
public static native int LAPACKE_zggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
IntPointer ilo, IntPointer ihi,
DoublePointer lscale, DoublePointer rscale, DoublePointer abnrm,
DoublePointer bbnrm, DoublePointer rconde, DoublePointer rcondv,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, IntPointer iwork,
IntPointer bwork );
public static native int LAPACKE_zggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
IntBuffer ilo, IntBuffer ihi,
DoubleBuffer lscale, DoubleBuffer rscale, DoubleBuffer abnrm,
DoubleBuffer bbnrm, DoubleBuffer rconde, DoubleBuffer rcondv,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, IntBuffer iwork,
IntBuffer bwork );
public static native int LAPACKE_zggevx_work( int matrix_layout, @Cast("char") byte balanc, @Cast("char") byte jobvl,
@Cast("char") byte jobvr, @Cast("char") byte sense, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
int[] ilo, int[] ihi,
double[] lscale, double[] rscale, double[] abnrm,
double[] bbnrm, double[] rconde, double[] rcondv,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int[] iwork,
int[] bwork );
public static native int LAPACKE_sggglm_work( int matrix_layout, int n, int m,
int p, FloatPointer a, int lda,
FloatPointer b, int ldb, FloatPointer d, FloatPointer x,
FloatPointer y, FloatPointer work, int lwork );
public static native int LAPACKE_sggglm_work( int matrix_layout, int n, int m,
int p, FloatBuffer a, int lda,
FloatBuffer b, int ldb, FloatBuffer d, FloatBuffer x,
FloatBuffer y, FloatBuffer work, int lwork );
public static native int LAPACKE_sggglm_work( int matrix_layout, int n, int m,
int p, float[] a, int lda,
float[] b, int ldb, float[] d, float[] x,
float[] y, float[] work, int lwork );
public static native int LAPACKE_dggglm_work( int matrix_layout, int n, int m,
int p, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer d, DoublePointer x,
DoublePointer y, DoublePointer work, int lwork );
public static native int LAPACKE_dggglm_work( int matrix_layout, int n, int m,
int p, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer d, DoubleBuffer x,
DoubleBuffer y, DoubleBuffer work, int lwork );
public static native int LAPACKE_dggglm_work( int matrix_layout, int n, int m,
int p, double[] a, int lda,
double[] b, int ldb, double[] d, double[] x,
double[] y, double[] work, int lwork );
public static native int LAPACKE_cggglm_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer x,
@Cast("lapack_complex_float*") FloatPointer y,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cggglm_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer x,
@Cast("lapack_complex_float*") FloatBuffer y,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cggglm_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] d,
@Cast("lapack_complex_float*") float[] x,
@Cast("lapack_complex_float*") float[] y,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zggglm_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer x,
@Cast("lapack_complex_double*") DoublePointer y,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zggglm_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer x,
@Cast("lapack_complex_double*") DoubleBuffer y,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zggglm_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] d,
@Cast("lapack_complex_double*") double[] x,
@Cast("lapack_complex_double*") double[] y,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer q, int ldq,
FloatPointer z, int ldz );
public static native int LAPACKE_sgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer q, int ldq,
FloatBuffer z, int ldz );
public static native int LAPACKE_sgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
float[] a, int lda, float[] b,
int ldb, float[] q, int ldq,
float[] z, int ldz );
public static native int LAPACKE_dgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoublePointer a, int lda, DoublePointer b,
int ldb, DoublePointer q, int ldq,
DoublePointer z, int ldz );
public static native int LAPACKE_dgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, DoubleBuffer q, int ldq,
DoubleBuffer z, int ldz );
public static native int LAPACKE_dgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
double[] a, int lda, double[] b,
int ldb, double[] q, int ldq,
double[] z, int ldz );
public static native int LAPACKE_cgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer z, int ldz );
public static native int LAPACKE_cgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz );
public static native int LAPACKE_cgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] z, int ldz );
public static native int LAPACKE_zgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz );
public static native int LAPACKE_zgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz );
public static native int LAPACKE_zgghrd_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz );
public static native int LAPACKE_sgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatPointer a, int lda,
FloatPointer b, int ldb,
FloatPointer q, int ldq,
FloatPointer z, int ldz,
FloatPointer work, int lwork );
public static native int LAPACKE_sgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatBuffer a, int lda,
FloatBuffer b, int ldb,
FloatBuffer q, int ldq,
FloatBuffer z, int ldz,
FloatBuffer work, int lwork );
public static native int LAPACKE_sgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
float[] a, int lda,
float[] b, int ldb,
float[] q, int ldq,
float[] z, int ldz,
float[] work, int lwork );
public static native int LAPACKE_dgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoublePointer a, int lda,
DoublePointer b, int ldb,
DoublePointer q, int ldq,
DoublePointer z, int ldz,
DoublePointer work, int lwork );
public static native int LAPACKE_dgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoubleBuffer a, int lda,
DoubleBuffer b, int ldb,
DoubleBuffer q, int ldq,
DoubleBuffer z, int ldz,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
double[] a, int lda,
double[] b, int ldb,
double[] q, int ldq,
double[] z, int ldz,
double[] work, int lwork );
public static native int LAPACKE_cgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] z, int ldz,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
@Cast("lapack_complex_double*") DoublePointer work,
int lwork );
public static native int LAPACKE_zgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
@Cast("lapack_complex_double*") DoubleBuffer work,
int lwork );
public static native int LAPACKE_zgghd3_work( int matrix_layout, @Cast("char") byte compq, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz,
@Cast("lapack_complex_double*") double[] work,
int lwork );
public static native int LAPACKE_sgglse_work( int matrix_layout, int m, int n,
int p, FloatPointer a, int lda,
FloatPointer b, int ldb, FloatPointer c, FloatPointer d,
FloatPointer x, FloatPointer work, int lwork );
public static native int LAPACKE_sgglse_work( int matrix_layout, int m, int n,
int p, FloatBuffer a, int lda,
FloatBuffer b, int ldb, FloatBuffer c, FloatBuffer d,
FloatBuffer x, FloatBuffer work, int lwork );
public static native int LAPACKE_sgglse_work( int matrix_layout, int m, int n,
int p, float[] a, int lda,
float[] b, int ldb, float[] c, float[] d,
float[] x, float[] work, int lwork );
public static native int LAPACKE_dgglse_work( int matrix_layout, int m, int n,
int p, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer c, DoublePointer d,
DoublePointer x, DoublePointer work, int lwork );
public static native int LAPACKE_dgglse_work( int matrix_layout, int m, int n,
int p, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer c, DoubleBuffer d,
DoubleBuffer x, DoubleBuffer work, int lwork );
public static native int LAPACKE_dgglse_work( int matrix_layout, int m, int n,
int p, double[] a, int lda,
double[] b, int ldb, double[] c, double[] d,
double[] x, double[] work, int lwork );
public static native int LAPACKE_cgglse_work( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer c,
@Cast("lapack_complex_float*") FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer x,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cgglse_work( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer c,
@Cast("lapack_complex_float*") FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer x,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cgglse_work( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] c,
@Cast("lapack_complex_float*") float[] d,
@Cast("lapack_complex_float*") float[] x,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zgglse_work( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer c,
@Cast("lapack_complex_double*") DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer x,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zgglse_work( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer c,
@Cast("lapack_complex_double*") DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer x,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zgglse_work( int matrix_layout, int m, int n,
int p, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] c,
@Cast("lapack_complex_double*") double[] d,
@Cast("lapack_complex_double*") double[] x,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sggqrf_work( int matrix_layout, int n, int m,
int p, FloatPointer a, int lda,
FloatPointer taua, FloatPointer b, int ldb,
FloatPointer taub, FloatPointer work, int lwork );
public static native int LAPACKE_sggqrf_work( int matrix_layout, int n, int m,
int p, FloatBuffer a, int lda,
FloatBuffer taua, FloatBuffer b, int ldb,
FloatBuffer taub, FloatBuffer work, int lwork );
public static native int LAPACKE_sggqrf_work( int matrix_layout, int n, int m,
int p, float[] a, int lda,
float[] taua, float[] b, int ldb,
float[] taub, float[] work, int lwork );
public static native int LAPACKE_dggqrf_work( int matrix_layout, int n, int m,
int p, DoublePointer a, int lda,
DoublePointer taua, DoublePointer b, int ldb,
DoublePointer taub, DoublePointer work, int lwork );
public static native int LAPACKE_dggqrf_work( int matrix_layout, int n, int m,
int p, DoubleBuffer a, int lda,
DoubleBuffer taua, DoubleBuffer b, int ldb,
DoubleBuffer taub, DoubleBuffer work, int lwork );
public static native int LAPACKE_dggqrf_work( int matrix_layout, int n, int m,
int p, double[] a, int lda,
double[] taua, double[] b, int ldb,
double[] taub, double[] work, int lwork );
public static native int LAPACKE_cggqrf_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer taua,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer taub,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cggqrf_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer taua,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer taub,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cggqrf_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] taua,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] taub,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zggqrf_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer taua,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer taub,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zggqrf_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer taua,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer taub,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zggqrf_work( int matrix_layout, int n, int m,
int p, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] taua,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] taub,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sggrqf_work( int matrix_layout, int m, int p,
int n, FloatPointer a, int lda,
FloatPointer taua, FloatPointer b, int ldb,
FloatPointer taub, FloatPointer work, int lwork );
public static native int LAPACKE_sggrqf_work( int matrix_layout, int m, int p,
int n, FloatBuffer a, int lda,
FloatBuffer taua, FloatBuffer b, int ldb,
FloatBuffer taub, FloatBuffer work, int lwork );
public static native int LAPACKE_sggrqf_work( int matrix_layout, int m, int p,
int n, float[] a, int lda,
float[] taua, float[] b, int ldb,
float[] taub, float[] work, int lwork );
public static native int LAPACKE_dggrqf_work( int matrix_layout, int m, int p,
int n, DoublePointer a, int lda,
DoublePointer taua, DoublePointer b, int ldb,
DoublePointer taub, DoublePointer work, int lwork );
public static native int LAPACKE_dggrqf_work( int matrix_layout, int m, int p,
int n, DoubleBuffer a, int lda,
DoubleBuffer taua, DoubleBuffer b, int ldb,
DoubleBuffer taub, DoubleBuffer work, int lwork );
public static native int LAPACKE_dggrqf_work( int matrix_layout, int m, int p,
int n, double[] a, int lda,
double[] taua, double[] b, int ldb,
double[] taub, double[] work, int lwork );
public static native int LAPACKE_cggrqf_work( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer taua,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer taub,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cggrqf_work( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer taua,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer taub,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cggrqf_work( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] taua,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] taub,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zggrqf_work( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer taua,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer taub,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zggrqf_work( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer taua,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer taub,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zggrqf_work( int matrix_layout, int m, int p,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] taua,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] taub,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, IntPointer k, IntPointer l,
FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer alpha, FloatPointer beta,
FloatPointer u, int ldu, FloatPointer v,
int ldv, FloatPointer q, int ldq,
FloatPointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_sggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, IntBuffer k, IntBuffer l,
FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer alpha, FloatBuffer beta,
FloatBuffer u, int ldu, FloatBuffer v,
int ldv, FloatBuffer q, int ldq,
FloatBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_sggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, int[] k, int[] l,
float[] a, int lda, float[] b,
int ldb, float[] alpha, float[] beta,
float[] u, int ldu, float[] v,
int ldv, float[] q, int ldq,
float[] work, int lwork,
int[] iwork );
public static native int LAPACKE_dggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, IntPointer k, IntPointer l,
DoublePointer a, int lda, DoublePointer b,
int ldb, DoublePointer alpha, DoublePointer beta,
DoublePointer u, int ldu, DoublePointer v,
int ldv, DoublePointer q, int ldq,
DoublePointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_dggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, IntBuffer k, IntBuffer l,
DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, DoubleBuffer alpha, DoubleBuffer beta,
DoubleBuffer u, int ldu, DoubleBuffer v,
int ldv, DoubleBuffer q, int ldq,
DoubleBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_dggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, int[] k, int[] l,
double[] a, int lda, double[] b,
int ldb, double[] alpha, double[] beta,
double[] u, int ldu, double[] v,
int ldv, double[] q, int ldq,
double[] work, int lwork,
int[] iwork );
public static native int LAPACKE_cggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, IntPointer k, IntPointer l,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
FloatPointer alpha, FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer u, int ldu,
@Cast("lapack_complex_float*") FloatPointer v, int ldv,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, IntPointer iwork );
public static native int LAPACKE_cggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, IntBuffer k, IntBuffer l,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
FloatBuffer alpha, FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer u, int ldu,
@Cast("lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, IntBuffer iwork );
public static native int LAPACKE_cggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, int[] k, int[] l,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
float[] alpha, float[] beta,
@Cast("lapack_complex_float*") float[] u, int ldu,
@Cast("lapack_complex_float*") float[] v, int ldv,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int[] iwork );
public static native int LAPACKE_zggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, IntPointer k, IntPointer l,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
DoublePointer alpha, DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer u, int ldu,
@Cast("lapack_complex_double*") DoublePointer v, int ldv,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, IntPointer iwork );
public static native int LAPACKE_zggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, IntBuffer k, IntBuffer l,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
DoubleBuffer alpha, DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer u, int ldu,
@Cast("lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, IntBuffer iwork );
public static native int LAPACKE_zggsvd3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int n,
int p, int[] k, int[] l,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
double[] alpha, double[] beta,
@Cast("lapack_complex_double*") double[] u, int ldu,
@Cast("lapack_complex_double*") double[] v, int ldv,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int[] iwork );
public static native int LAPACKE_sggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, FloatPointer a, int lda,
FloatPointer b, int ldb, float tola,
float tolb, IntPointer k, IntPointer l,
FloatPointer u, int ldu, FloatPointer v,
int ldv, FloatPointer q, int ldq,
IntPointer iwork, FloatPointer tau,
FloatPointer work, int lwork );
public static native int LAPACKE_sggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, FloatBuffer a, int lda,
FloatBuffer b, int ldb, float tola,
float tolb, IntBuffer k, IntBuffer l,
FloatBuffer u, int ldu, FloatBuffer v,
int ldv, FloatBuffer q, int ldq,
IntBuffer iwork, FloatBuffer tau,
FloatBuffer work, int lwork );
public static native int LAPACKE_sggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, float[] a, int lda,
float[] b, int ldb, float tola,
float tolb, int[] k, int[] l,
float[] u, int ldu, float[] v,
int ldv, float[] q, int ldq,
int[] iwork, float[] tau,
float[] work, int lwork );
public static native int LAPACKE_dggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, DoublePointer a, int lda,
DoublePointer b, int ldb, double tola,
double tolb, IntPointer k, IntPointer l,
DoublePointer u, int ldu, DoublePointer v,
int ldv, DoublePointer q, int ldq,
IntPointer iwork, DoublePointer tau, DoublePointer work,
int lwork );
public static native int LAPACKE_dggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, double tola,
double tolb, IntBuffer k, IntBuffer l,
DoubleBuffer u, int ldu, DoubleBuffer v,
int ldv, DoubleBuffer q, int ldq,
IntBuffer iwork, DoubleBuffer tau, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, double[] a, int lda,
double[] b, int ldb, double tola,
double tolb, int[] k, int[] l,
double[] u, int ldu, double[] v,
int ldv, double[] q, int ldq,
int[] iwork, double[] tau, double[] work,
int lwork );
public static native int LAPACKE_cggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, float tola, float tolb,
IntPointer k, IntPointer l,
@Cast("lapack_complex_float*") FloatPointer u, int ldu,
@Cast("lapack_complex_float*") FloatPointer v, int ldv,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
IntPointer iwork, FloatPointer rwork,
@Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, float tola, float tolb,
IntBuffer k, IntBuffer l,
@Cast("lapack_complex_float*") FloatBuffer u, int ldu,
@Cast("lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
IntBuffer iwork, FloatBuffer rwork,
@Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, float tola, float tolb,
int[] k, int[] l,
@Cast("lapack_complex_float*") float[] u, int ldu,
@Cast("lapack_complex_float*") float[] v, int ldv,
@Cast("lapack_complex_float*") float[] q, int ldq,
int[] iwork, float[] rwork,
@Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, double tola, double tolb,
IntPointer k, IntPointer l,
@Cast("lapack_complex_double*") DoublePointer u, int ldu,
@Cast("lapack_complex_double*") DoublePointer v, int ldv,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
IntPointer iwork, DoublePointer rwork,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, double tola, double tolb,
IntBuffer k, IntBuffer l,
@Cast("lapack_complex_double*") DoubleBuffer u, int ldu,
@Cast("lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
IntBuffer iwork, DoubleBuffer rwork,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zggsvp3_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, double tola, double tolb,
int[] k, int[] l,
@Cast("lapack_complex_double*") double[] u, int ldu,
@Cast("lapack_complex_double*") double[] v, int ldv,
@Cast("lapack_complex_double*") double[] q, int ldq,
int[] iwork, double[] rwork,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_sgtcon_work( @Cast("char") byte norm, int n, @Const FloatPointer dl,
@Const FloatPointer d, @Const FloatPointer du,
@Const FloatPointer du2, @Const IntPointer ipiv,
float anorm, FloatPointer rcond, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_sgtcon_work( @Cast("char") byte norm, int n, @Const FloatBuffer dl,
@Const FloatBuffer d, @Const FloatBuffer du,
@Const FloatBuffer du2, @Const IntBuffer ipiv,
float anorm, FloatBuffer rcond, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_sgtcon_work( @Cast("char") byte norm, int n, @Const float[] dl,
@Const float[] d, @Const float[] du,
@Const float[] du2, @Const int[] ipiv,
float anorm, float[] rcond, float[] work,
int[] iwork );
public static native int LAPACKE_dgtcon_work( @Cast("char") byte norm, int n, @Const DoublePointer dl,
@Const DoublePointer d, @Const DoublePointer du,
@Const DoublePointer du2, @Const IntPointer ipiv,
double anorm, DoublePointer rcond, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dgtcon_work( @Cast("char") byte norm, int n, @Const DoubleBuffer dl,
@Const DoubleBuffer d, @Const DoubleBuffer du,
@Const DoubleBuffer du2, @Const IntBuffer ipiv,
double anorm, DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dgtcon_work( @Cast("char") byte norm, int n, @Const double[] dl,
@Const double[] d, @Const double[] du,
@Const double[] du2, @Const int[] ipiv,
double anorm, double[] rcond, double[] work,
int[] iwork );
public static native int LAPACKE_cgtcon_work( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("const lapack_complex_float*") FloatPointer du2,
@Const IntPointer ipiv, float anorm,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_cgtcon_work( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("const lapack_complex_float*") FloatBuffer du2,
@Const IntBuffer ipiv, float anorm,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_cgtcon_work( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("const lapack_complex_float*") float[] du2,
@Const int[] ipiv, float anorm,
float[] rcond, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zgtcon_work( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("const lapack_complex_double*") DoublePointer du2,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zgtcon_work( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("const lapack_complex_double*") DoubleBuffer du2,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zgtcon_work( @Cast("char") byte norm, int n,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("const lapack_complex_double*") double[] du2,
@Const int[] ipiv, double anorm,
double[] rcond, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_sgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatPointer dl,
@Const FloatPointer d, @Const FloatPointer du,
@Const FloatPointer dlf, @Const FloatPointer df,
@Const FloatPointer duf, @Const FloatPointer du2,
@Const IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_sgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatBuffer dl,
@Const FloatBuffer d, @Const FloatBuffer du,
@Const FloatBuffer dlf, @Const FloatBuffer df,
@Const FloatBuffer duf, @Const FloatBuffer du2,
@Const IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_sgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const float[] dl,
@Const float[] d, @Const float[] du,
@Const float[] dlf, @Const float[] df,
@Const float[] duf, @Const float[] du2,
@Const int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr, float[] work,
int[] iwork );
public static native int LAPACKE_dgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoublePointer dl,
@Const DoublePointer d, @Const DoublePointer du,
@Const DoublePointer dlf, @Const DoublePointer df,
@Const DoublePointer duf, @Const DoublePointer du2,
@Const IntPointer ipiv, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoubleBuffer dl,
@Const DoubleBuffer d, @Const DoubleBuffer du,
@Const DoubleBuffer dlf, @Const DoubleBuffer df,
@Const DoubleBuffer duf, @Const DoubleBuffer du2,
@Const IntBuffer ipiv, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const double[] dl,
@Const double[] d, @Const double[] du,
@Const double[] dlf, @Const double[] df,
@Const double[] duf, @Const double[] du2,
@Const int[] ipiv, @Const double[] b,
int ldb, double[] x, int ldx,
double[] ferr, double[] berr, double[] work,
int[] iwork );
public static native int LAPACKE_cgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("const lapack_complex_float*") FloatPointer dlf,
@Cast("const lapack_complex_float*") FloatPointer df,
@Cast("const lapack_complex_float*") FloatPointer duf,
@Cast("const lapack_complex_float*") FloatPointer du2,
@Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("const lapack_complex_float*") FloatBuffer dlf,
@Cast("const lapack_complex_float*") FloatBuffer df,
@Cast("const lapack_complex_float*") FloatBuffer duf,
@Cast("const lapack_complex_float*") FloatBuffer du2,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("const lapack_complex_float*") float[] dlf,
@Cast("const lapack_complex_float*") float[] df,
@Cast("const lapack_complex_float*") float[] duf,
@Cast("const lapack_complex_float*") float[] du2,
@Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("const lapack_complex_double*") DoublePointer dlf,
@Cast("const lapack_complex_double*") DoublePointer df,
@Cast("const lapack_complex_double*") DoublePointer duf,
@Cast("const lapack_complex_double*") DoublePointer du2,
@Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("const lapack_complex_double*") DoubleBuffer dlf,
@Cast("const lapack_complex_double*") DoubleBuffer df,
@Cast("const lapack_complex_double*") DoubleBuffer duf,
@Cast("const lapack_complex_double*") DoubleBuffer du2,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zgtrfs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("const lapack_complex_double*") double[] dlf,
@Cast("const lapack_complex_double*") double[] df,
@Cast("const lapack_complex_double*") double[] duf,
@Cast("const lapack_complex_double*") double[] du2,
@Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sgtsv_work( int matrix_layout, int n, int nrhs,
FloatPointer dl, FloatPointer d, FloatPointer du, FloatPointer b,
int ldb );
public static native int LAPACKE_sgtsv_work( int matrix_layout, int n, int nrhs,
FloatBuffer dl, FloatBuffer d, FloatBuffer du, FloatBuffer b,
int ldb );
public static native int LAPACKE_sgtsv_work( int matrix_layout, int n, int nrhs,
float[] dl, float[] d, float[] du, float[] b,
int ldb );
public static native int LAPACKE_dgtsv_work( int matrix_layout, int n, int nrhs,
DoublePointer dl, DoublePointer d, DoublePointer du, DoublePointer b,
int ldb );
public static native int LAPACKE_dgtsv_work( int matrix_layout, int n, int nrhs,
DoubleBuffer dl, DoubleBuffer d, DoubleBuffer du, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dgtsv_work( int matrix_layout, int n, int nrhs,
double[] dl, double[] d, double[] du, double[] b,
int ldb );
public static native int LAPACKE_cgtsv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer dl,
@Cast("lapack_complex_float*") FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer du,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cgtsv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer dl,
@Cast("lapack_complex_float*") FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer du,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cgtsv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_float*") float[] dl,
@Cast("lapack_complex_float*") float[] d,
@Cast("lapack_complex_float*") float[] du,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zgtsv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer dl,
@Cast("lapack_complex_double*") DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer du,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zgtsv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer dl,
@Cast("lapack_complex_double*") DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer du,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zgtsv_work( int matrix_layout, int n, int nrhs,
@Cast("lapack_complex_double*") double[] dl,
@Cast("lapack_complex_double*") double[] d,
@Cast("lapack_complex_double*") double[] du,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const FloatPointer dl,
@Const FloatPointer d, @Const FloatPointer du, FloatPointer dlf,
FloatPointer df, FloatPointer duf, FloatPointer du2,
IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const FloatBuffer dl,
@Const FloatBuffer d, @Const FloatBuffer du, FloatBuffer dlf,
FloatBuffer df, FloatBuffer duf, FloatBuffer du2,
IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const float[] dl,
@Const float[] d, @Const float[] du, float[] dlf,
float[] df, float[] duf, float[] du2,
int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
float[] work, int[] iwork );
public static native int LAPACKE_dgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const DoublePointer dl,
@Const DoublePointer d, @Const DoublePointer du, DoublePointer dlf,
DoublePointer df, DoublePointer duf, DoublePointer du2,
IntPointer ipiv, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const DoubleBuffer dl,
@Const DoubleBuffer d, @Const DoubleBuffer du, DoubleBuffer dlf,
DoubleBuffer df, DoubleBuffer duf, DoubleBuffer du2,
IntBuffer ipiv, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs, @Const double[] dl,
@Const double[] d, @Const double[] du, double[] dlf,
double[] df, double[] duf, double[] du2,
int[] ipiv, @Const double[] b,
int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
double[] work, int[] iwork );
public static native int LAPACKE_cgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("lapack_complex_float*") FloatPointer dlf,
@Cast("lapack_complex_float*") FloatPointer df,
@Cast("lapack_complex_float*") FloatPointer duf,
@Cast("lapack_complex_float*") FloatPointer du2, IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("lapack_complex_float*") FloatBuffer dlf,
@Cast("lapack_complex_float*") FloatBuffer df,
@Cast("lapack_complex_float*") FloatBuffer duf,
@Cast("lapack_complex_float*") FloatBuffer du2, IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("lapack_complex_float*") float[] dlf,
@Cast("lapack_complex_float*") float[] df,
@Cast("lapack_complex_float*") float[] duf,
@Cast("lapack_complex_float*") float[] du2, int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("lapack_complex_double*") DoublePointer dlf,
@Cast("lapack_complex_double*") DoublePointer df,
@Cast("lapack_complex_double*") DoublePointer duf,
@Cast("lapack_complex_double*") DoublePointer du2, IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("lapack_complex_double*") DoubleBuffer dlf,
@Cast("lapack_complex_double*") DoubleBuffer df,
@Cast("lapack_complex_double*") DoubleBuffer duf,
@Cast("lapack_complex_double*") DoubleBuffer du2, IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zgtsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte trans,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("lapack_complex_double*") double[] dlf,
@Cast("lapack_complex_double*") double[] df,
@Cast("lapack_complex_double*") double[] duf,
@Cast("lapack_complex_double*") double[] du2, int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sgttrf_work( int n, FloatPointer dl, FloatPointer d, FloatPointer du,
FloatPointer du2, IntPointer ipiv );
public static native int LAPACKE_sgttrf_work( int n, FloatBuffer dl, FloatBuffer d, FloatBuffer du,
FloatBuffer du2, IntBuffer ipiv );
public static native int LAPACKE_sgttrf_work( int n, float[] dl, float[] d, float[] du,
float[] du2, int[] ipiv );
public static native int LAPACKE_dgttrf_work( int n, DoublePointer dl, DoublePointer d, DoublePointer du,
DoublePointer du2, IntPointer ipiv );
public static native int LAPACKE_dgttrf_work( int n, DoubleBuffer dl, DoubleBuffer d, DoubleBuffer du,
DoubleBuffer du2, IntBuffer ipiv );
public static native int LAPACKE_dgttrf_work( int n, double[] dl, double[] d, double[] du,
double[] du2, int[] ipiv );
public static native int LAPACKE_cgttrf_work( int n, @Cast("lapack_complex_float*") FloatPointer dl,
@Cast("lapack_complex_float*") FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer du,
@Cast("lapack_complex_float*") FloatPointer du2, IntPointer ipiv );
public static native int LAPACKE_cgttrf_work( int n, @Cast("lapack_complex_float*") FloatBuffer dl,
@Cast("lapack_complex_float*") FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer du,
@Cast("lapack_complex_float*") FloatBuffer du2, IntBuffer ipiv );
public static native int LAPACKE_cgttrf_work( int n, @Cast("lapack_complex_float*") float[] dl,
@Cast("lapack_complex_float*") float[] d,
@Cast("lapack_complex_float*") float[] du,
@Cast("lapack_complex_float*") float[] du2, int[] ipiv );
public static native int LAPACKE_zgttrf_work( int n, @Cast("lapack_complex_double*") DoublePointer dl,
@Cast("lapack_complex_double*") DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer du,
@Cast("lapack_complex_double*") DoublePointer du2, IntPointer ipiv );
public static native int LAPACKE_zgttrf_work( int n, @Cast("lapack_complex_double*") DoubleBuffer dl,
@Cast("lapack_complex_double*") DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer du,
@Cast("lapack_complex_double*") DoubleBuffer du2, IntBuffer ipiv );
public static native int LAPACKE_zgttrf_work( int n, @Cast("lapack_complex_double*") double[] dl,
@Cast("lapack_complex_double*") double[] d,
@Cast("lapack_complex_double*") double[] du,
@Cast("lapack_complex_double*") double[] du2, int[] ipiv );
public static native int LAPACKE_sgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatPointer dl,
@Const FloatPointer d, @Const FloatPointer du,
@Const FloatPointer du2, @Const IntPointer ipiv,
FloatPointer b, int ldb );
public static native int LAPACKE_sgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const FloatBuffer dl,
@Const FloatBuffer d, @Const FloatBuffer du,
@Const FloatBuffer du2, @Const IntBuffer ipiv,
FloatBuffer b, int ldb );
public static native int LAPACKE_sgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const float[] dl,
@Const float[] d, @Const float[] du,
@Const float[] du2, @Const int[] ipiv,
float[] b, int ldb );
public static native int LAPACKE_dgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoublePointer dl,
@Const DoublePointer d, @Const DoublePointer du,
@Const DoublePointer du2, @Const IntPointer ipiv,
DoublePointer b, int ldb );
public static native int LAPACKE_dgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const DoubleBuffer dl,
@Const DoubleBuffer d, @Const DoubleBuffer du,
@Const DoubleBuffer du2, @Const IntBuffer ipiv,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Const double[] dl,
@Const double[] d, @Const double[] du,
@Const double[] du2, @Const int[] ipiv,
double[] b, int ldb );
public static native int LAPACKE_cgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("const lapack_complex_float*") FloatPointer du2,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("const lapack_complex_float*") FloatBuffer du2,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("const lapack_complex_float*") float[] du2,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("const lapack_complex_double*") DoublePointer du2,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("const lapack_complex_double*") DoubleBuffer du2,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zgttrs_work( int matrix_layout, @Cast("char") byte trans, int n,
int nrhs,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("const lapack_complex_double*") double[] du2,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_chbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_chbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_chbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") float[] ab, int ldab,
float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_zhbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork );
public static native int LAPACKE_zhbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork );
public static native int LAPACKE_zhbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") double[] ab, int ldab,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
double[] rwork );
public static native int LAPACKE_chbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_chbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_chbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") float[] ab, int ldab,
float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_zhbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_zhbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_zhbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") double[] ab, int ldab,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_chbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
float vl, float vu, int il,
int iu, float abstol, IntPointer m,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_chbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
float vl, float vu, int il,
int iu, float abstol, IntBuffer m,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_chbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] q, int ldq,
float vl, float vu, int il,
int iu, float abstol, int[] m,
float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] iwork,
int[] ifail );
public static native int LAPACKE_zhbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
double vl, double vu, int il,
int iu, double abstol, IntPointer m,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_zhbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
double vl, double vu, int il,
int iu, double abstol, IntBuffer m,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_zhbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] q, int ldq,
double vl, double vu, int il,
int iu, double abstol, int[] m,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] iwork,
int[] ifail );
public static native int LAPACKE_chbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("const lapack_complex_float*") FloatPointer bb, int ldbb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_chbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("const lapack_complex_float*") FloatBuffer bb, int ldbb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_chbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("const lapack_complex_float*") float[] bb, int ldbb,
@Cast("lapack_complex_float*") float[] x, int ldx,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zhbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("const lapack_complex_double*") DoublePointer bb,
int ldbb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork );
public static native int LAPACKE_zhbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("const lapack_complex_double*") DoubleBuffer bb,
int ldbb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork );
public static native int LAPACKE_zhbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("const lapack_complex_double*") double[] bb,
int ldbb, @Cast("lapack_complex_double*") double[] x,
int ldx, @Cast("lapack_complex_double*") double[] work,
double[] rwork );
public static native int LAPACKE_chbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer bb, int ldbb,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_chbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer bb, int ldbb,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_chbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] bb, int ldbb,
float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_zhbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer bb, int ldbb,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork );
public static native int LAPACKE_zhbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer bb, int ldbb,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork );
public static native int LAPACKE_zhbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] bb, int ldbb,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
double[] rwork );
public static native int LAPACKE_chbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer bb, int ldbb,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_chbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer bb, int ldbb,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_chbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] bb, int ldbb,
float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_zhbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer bb, int ldbb,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_zhbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer bb, int ldbb,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_zhbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] bb, int ldbb,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_chbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab, @Cast("lapack_complex_float*") FloatPointer bb,
int ldbb, @Cast("lapack_complex_float*") FloatPointer q,
int ldq, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_chbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab, @Cast("lapack_complex_float*") FloatBuffer bb,
int ldbb, @Cast("lapack_complex_float*") FloatBuffer q,
int ldq, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_chbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, @Cast("lapack_complex_float*") float[] ab,
int ldab, @Cast("lapack_complex_float*") float[] bb,
int ldbb, @Cast("lapack_complex_float*") float[] q,
int ldq, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_zhbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab, @Cast("lapack_complex_double*") DoublePointer bb,
int ldbb, @Cast("lapack_complex_double*") DoublePointer q,
int ldq, double vl, double vu,
int il, int iu, double abstol,
IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_zhbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab, @Cast("lapack_complex_double*") DoubleBuffer bb,
int ldbb, @Cast("lapack_complex_double*") DoubleBuffer q,
int ldq, double vl, double vu,
int il, int iu, double abstol,
IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_zhbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, @Cast("lapack_complex_double*") double[] ab,
int ldab, @Cast("lapack_complex_double*") double[] bb,
int ldbb, @Cast("lapack_complex_double*") double[] q,
int ldq, double vl, double vu,
int il, int iu, double abstol,
int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_chbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
FloatPointer d, FloatPointer e, @Cast("lapack_complex_float*") FloatPointer q,
int ldq, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_chbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
FloatBuffer d, FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer q,
int ldq, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_chbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_float*") float[] ab, int ldab,
float[] d, float[] e, @Cast("lapack_complex_float*") float[] q,
int ldq, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zhbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer q,
int ldq, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zhbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer q,
int ldq, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zhbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd,
@Cast("lapack_complex_double*") double[] ab, int ldab,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] q,
int ldq, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_checon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv, float anorm,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_checon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv, float anorm,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_checon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv, float anorm,
float[] rcond, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zhecon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zhecon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zhecon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv, double anorm,
double[] rcond, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_cheequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer s, FloatPointer scond, FloatPointer amax,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_cheequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_cheequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] s, float[] scond, float[] amax,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zheequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer s, DoublePointer scond, DoublePointer amax,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zheequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zheequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] s, double[] scond, double[] amax,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_cheev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork );
public static native int LAPACKE_cheev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork );
public static native int LAPACKE_cheev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, float[] w,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork );
public static native int LAPACKE_zheev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork );
public static native int LAPACKE_zheev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork );
public static native int LAPACKE_zheev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, double[] w,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork );
public static native int LAPACKE_cheevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, int lrwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_cheevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, int lrwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_cheevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, float[] w,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int lrwork,
int[] iwork, int liwork );
public static native int LAPACKE_zheevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, int lrwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_zheevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, int lrwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_zheevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, double[] w,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int lrwork,
int[] iwork, int liwork );
public static native int LAPACKE_cheevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
float vl, float vu, int il,
int iu, float abstol, IntPointer m,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, IntPointer isuppz,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, int lrwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_cheevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
float vl, float vu, int il,
int iu, float abstol, IntBuffer m,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, IntBuffer isuppz,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, int lrwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_cheevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
float vl, float vu, int il,
int iu, float abstol, int[] m,
float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, int[] isuppz,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int lrwork,
int[] iwork, int liwork );
public static native int LAPACKE_zheevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
double vl, double vu, int il,
int iu, double abstol, IntPointer m,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, IntPointer isuppz,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, int lrwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_zheevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
double vl, double vu, int il,
int iu, double abstol, IntBuffer m,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, IntBuffer isuppz,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, int lrwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_zheevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
double vl, double vu, int il,
int iu, double abstol, int[] m,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, int[] isuppz,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int lrwork,
int[] iwork, int liwork );
public static native int LAPACKE_cheevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
float vl, float vu, int il,
int iu, float abstol, IntPointer m,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_cheevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
float vl, float vu, int il,
int iu, float abstol, IntBuffer m,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_cheevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
float vl, float vu, int il,
int iu, float abstol, int[] m,
float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_zheevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
double vl, double vu, int il,
int iu, double abstol, IntPointer m,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_zheevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
double vl, double vu, int il,
int iu, double abstol, IntBuffer m,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_zheevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
double vl, double vu, int il,
int iu, double abstol, int[] m,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_chegst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_chegst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_chegst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zhegst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zhegst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zhegst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_chegv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork );
public static native int LAPACKE_chegv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork );
public static native int LAPACKE_chegv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb, float[] w,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork );
public static native int LAPACKE_zhegv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork );
public static native int LAPACKE_zhegv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork );
public static native int LAPACKE_zhegv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
double[] w, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork );
public static native int LAPACKE_chegvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_chegvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_chegvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
float[] w, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_zhegvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_zhegvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_zhegvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
double[] w, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_chegvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
float vl, float vu, int il,
int iu, float abstol, IntPointer m,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_chegvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
float vl, float vu, int il,
int iu, float abstol, IntBuffer m,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_chegvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
float vl, float vu, int il,
int iu, float abstol, int[] m,
float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_zhegvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
double vl, double vu, int il,
int iu, double abstol, IntPointer m,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_zhegvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
double vl, double vu, int il,
int iu, double abstol, IntBuffer m,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_zhegvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
double vl, double vu, int il,
int iu, double abstol, int[] m,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_cherfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cherfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cherfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zherfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zherfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zherfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_chesv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_chesv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_chesv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zhesv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zhesv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zhesv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_chesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer af, int ldaf,
IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork );
public static native int LAPACKE_chesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer af, int ldaf,
IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork );
public static native int LAPACKE_chesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] af, int ldaf,
int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork );
public static native int LAPACKE_zhesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer af, int ldaf,
IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork );
public static native int LAPACKE_zhesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer af, int ldaf,
IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork );
public static native int LAPACKE_zhesvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] af, int ldaf,
int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork );
public static native int LAPACKE_chetrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
FloatPointer d, FloatPointer e, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_chetrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer d, FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_chetrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
float[] d, float[] e, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zhetrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zhetrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zhetrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
double[] d, double[] e,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_chetrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer work,
int lwork );
public static native int LAPACKE_chetrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork );
public static native int LAPACKE_chetrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv, @Cast("lapack_complex_float*") float[] work,
int lwork );
public static native int LAPACKE_zhetrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer work,
int lwork );
public static native int LAPACKE_zhetrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork );
public static native int LAPACKE_zhetrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv, @Cast("lapack_complex_double*") double[] work,
int lwork );
public static native int LAPACKE_chetri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_chetri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_chetri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zhetri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zhetri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zhetri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_chetrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_chetrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_chetrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zhetrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zhetrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zhetrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_chfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
float alpha, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, float beta,
@Cast("lapack_complex_float*") FloatPointer c );
public static native int LAPACKE_chfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
float alpha, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, float beta,
@Cast("lapack_complex_float*") FloatBuffer c );
public static native int LAPACKE_chfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
float alpha, @Cast("const lapack_complex_float*") float[] a,
int lda, float beta,
@Cast("lapack_complex_float*") float[] c );
public static native int LAPACKE_zhfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
double alpha, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, double beta,
@Cast("lapack_complex_double*") DoublePointer c );
public static native int LAPACKE_zhfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
double alpha, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, double beta,
@Cast("lapack_complex_double*") DoubleBuffer c );
public static native int LAPACKE_zhfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
double alpha, @Cast("const lapack_complex_double*") double[] a,
int lda, double beta,
@Cast("lapack_complex_double*") double[] c );
public static native int LAPACKE_shgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, FloatPointer h, int ldh,
FloatPointer t, int ldt, FloatPointer alphar,
FloatPointer alphai, FloatPointer beta, FloatPointer q,
int ldq, FloatPointer z, int ldz,
FloatPointer work, int lwork );
public static native int LAPACKE_shgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, FloatBuffer h, int ldh,
FloatBuffer t, int ldt, FloatBuffer alphar,
FloatBuffer alphai, FloatBuffer beta, FloatBuffer q,
int ldq, FloatBuffer z, int ldz,
FloatBuffer work, int lwork );
public static native int LAPACKE_shgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, float[] h, int ldh,
float[] t, int ldt, float[] alphar,
float[] alphai, float[] beta, float[] q,
int ldq, float[] z, int ldz,
float[] work, int lwork );
public static native int LAPACKE_dhgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, DoublePointer h, int ldh,
DoublePointer t, int ldt, DoublePointer alphar,
DoublePointer alphai, DoublePointer beta, DoublePointer q,
int ldq, DoublePointer z, int ldz,
DoublePointer work, int lwork );
public static native int LAPACKE_dhgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, DoubleBuffer h, int ldh,
DoubleBuffer t, int ldt, DoubleBuffer alphar,
DoubleBuffer alphai, DoubleBuffer beta, DoubleBuffer q,
int ldq, DoubleBuffer z, int ldz,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dhgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, double[] h, int ldh,
double[] t, int ldt, double[] alphar,
double[] alphai, double[] beta, double[] q,
int ldq, double[] z, int ldz,
double[] work, int lwork );
public static native int LAPACKE_chgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatPointer h,
int ldh, @Cast("lapack_complex_float*") FloatPointer t,
int ldt, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork );
public static native int LAPACKE_chgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatBuffer h,
int ldh, @Cast("lapack_complex_float*") FloatBuffer t,
int ldt, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork );
public static native int LAPACKE_chgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") float[] h,
int ldh, @Cast("lapack_complex_float*") float[] t,
int ldt, @Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] z, int ldz,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork );
public static native int LAPACKE_zhgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoublePointer h,
int ldh, @Cast("lapack_complex_double*") DoublePointer t,
int ldt, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork );
public static native int LAPACKE_zhgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoubleBuffer h,
int ldh, @Cast("lapack_complex_double*") DoubleBuffer t,
int ldt, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork );
public static native int LAPACKE_zhgeqz_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Cast("char") byte compz, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") double[] h,
int ldh, @Cast("lapack_complex_double*") double[] t,
int ldt, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork );
public static native int LAPACKE_chpcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, float anorm,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_chpcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, float anorm,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_chpcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, float anorm,
float[] rcond, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zhpcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zhpcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zhpcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv, double anorm,
double[] rcond, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_chpev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer ap, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_chpev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer ap, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_chpev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] ap, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zhpev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer ap,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork );
public static native int LAPACKE_zhpev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer ap,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork );
public static native int LAPACKE_zhpev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] ap,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
double[] rwork );
public static native int LAPACKE_chpevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer ap,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_chpevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer ap,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_chpevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] ap,
float[] w, @Cast("lapack_complex_float*") float[] z,
int ldz, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_zhpevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer ap,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_zhpevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer ap,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_zhpevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] ap,
double[] w, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_chpevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_chpevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_chpevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_zhpevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, double vl, double vu,
int il, int iu, double abstol,
IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_zhpevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, double vl, double vu,
int il, int iu, double abstol,
IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_zhpevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, double vl, double vu,
int il, int iu, double abstol,
int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_chpgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer bp );
public static native int LAPACKE_chpgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer bp );
public static native int LAPACKE_chpgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] bp );
public static native int LAPACKE_zhpgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer bp );
public static native int LAPACKE_zhpgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer bp );
public static native int LAPACKE_zhpgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] bp );
public static native int LAPACKE_chpgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer bp, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_chpgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer bp, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_chpgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] bp, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zhpgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer bp, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zhpgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer bp, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zhpgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] bp, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_chpgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer bp, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, int lrwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_chpgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer bp, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, int lrwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_chpgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] bp, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int lrwork,
int[] iwork, int liwork );
public static native int LAPACKE_zhpgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer bp, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, int lrwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_zhpgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer bp, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, int lrwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_zhpgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] bp, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int lrwork,
int[] iwork, int liwork );
public static native int LAPACKE_chpgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer bp, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_chpgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer bp, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_chpgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] bp, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_zhpgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer bp, double vl, double vu,
int il, int iu, double abstol,
IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_zhpgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer bp, double vl, double vu,
int il, int iu, double abstol,
IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_zhpgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] bp, double vl, double vu,
int il, int iu, double abstol,
int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_chprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer afp,
@Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_chprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer afp,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_chprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] afp,
@Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zhprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer afp,
@Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zhprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer afp,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zhprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] afp,
@Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_chpsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_chpsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_chpsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] ap,
int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zhpsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zhpsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zhpsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] ap,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_chpsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer afp, IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_chpsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer afp, IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_chpsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] afp, int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zhpsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer afp, IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zhpsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer afp, IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zhpsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] afp, int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_chptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_chptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_chptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zhptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zhptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zhptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_chptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, IntPointer ipiv );
public static native int LAPACKE_chptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_chptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, int[] ipiv );
public static native int LAPACKE_zhptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, IntPointer ipiv );
public static native int LAPACKE_zhptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_zhptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, int[] ipiv );
public static native int LAPACKE_chptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_chptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_chptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zhptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zhptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zhptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_chptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_chptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_chptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zhptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zhptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zhptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_shsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, IntPointer select,
int n, @Const FloatPointer h, int ldh,
FloatPointer wr, @Const FloatPointer wi, FloatPointer vl,
int ldvl, FloatPointer vr, int ldvr,
int mm, IntPointer m, FloatPointer work,
IntPointer ifaill, IntPointer ifailr );
public static native int LAPACKE_shsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, IntBuffer select,
int n, @Const FloatBuffer h, int ldh,
FloatBuffer wr, @Const FloatBuffer wi, FloatBuffer vl,
int ldvl, FloatBuffer vr, int ldvr,
int mm, IntBuffer m, FloatBuffer work,
IntBuffer ifaill, IntBuffer ifailr );
public static native int LAPACKE_shsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, int[] select,
int n, @Const float[] h, int ldh,
float[] wr, @Const float[] wi, float[] vl,
int ldvl, float[] vr, int ldvr,
int mm, int[] m, float[] work,
int[] ifaill, int[] ifailr );
public static native int LAPACKE_dhsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, IntPointer select,
int n, @Const DoublePointer h, int ldh,
DoublePointer wr, @Const DoublePointer wi, DoublePointer vl,
int ldvl, DoublePointer vr, int ldvr,
int mm, IntPointer m, DoublePointer work,
IntPointer ifaill, IntPointer ifailr );
public static native int LAPACKE_dhsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, IntBuffer select,
int n, @Const DoubleBuffer h, int ldh,
DoubleBuffer wr, @Const DoubleBuffer wi, DoubleBuffer vl,
int ldvl, DoubleBuffer vr, int ldvr,
int mm, IntBuffer m, DoubleBuffer work,
IntBuffer ifaill, IntBuffer ifailr );
public static native int LAPACKE_dhsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, int[] select,
int n, @Const double[] h, int ldh,
double[] wr, @Const double[] wi, double[] vl,
int ldvl, double[] vr, int ldvr,
int mm, int[] m, double[] work,
int[] ifaill, int[] ifailr );
public static native int LAPACKE_chsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, @Const IntPointer select,
int n, @Cast("const lapack_complex_float*") FloatPointer h,
int ldh, @Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
int mm, IntPointer m,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer ifaill, IntPointer ifailr );
public static native int LAPACKE_chsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, @Const IntBuffer select,
int n, @Cast("const lapack_complex_float*") FloatBuffer h,
int ldh, @Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
int mm, IntBuffer m,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer ifaill, IntBuffer ifailr );
public static native int LAPACKE_chsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, @Const int[] select,
int n, @Cast("const lapack_complex_float*") float[] h,
int ldh, @Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
int mm, int[] m,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] ifaill, int[] ifailr );
public static native int LAPACKE_zhsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, @Const IntPointer select,
int n, @Cast("const lapack_complex_double*") DoublePointer h,
int ldh, @Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
int mm, IntPointer m,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer ifaill, IntPointer ifailr );
public static native int LAPACKE_zhsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, @Const IntBuffer select,
int n, @Cast("const lapack_complex_double*") DoubleBuffer h,
int ldh, @Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
int mm, IntBuffer m,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer ifaill, IntBuffer ifailr );
public static native int LAPACKE_zhsein_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte eigsrc,
@Cast("char") byte initv, @Const int[] select,
int n, @Cast("const lapack_complex_double*") double[] h,
int ldh, @Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
int mm, int[] m,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] ifaill, int[] ifailr );
public static native int LAPACKE_shseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatPointer h, int ldh, FloatPointer wr, FloatPointer wi,
FloatPointer z, int ldz, FloatPointer work,
int lwork );
public static native int LAPACKE_shseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
FloatBuffer h, int ldh, FloatBuffer wr, FloatBuffer wi,
FloatBuffer z, int ldz, FloatBuffer work,
int lwork );
public static native int LAPACKE_shseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
float[] h, int ldh, float[] wr, float[] wi,
float[] z, int ldz, float[] work,
int lwork );
public static native int LAPACKE_dhseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoublePointer h, int ldh, DoublePointer wr,
DoublePointer wi, DoublePointer z, int ldz,
DoublePointer work, int lwork );
public static native int LAPACKE_dhseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
DoubleBuffer h, int ldh, DoubleBuffer wr,
DoubleBuffer wi, DoubleBuffer z, int ldz,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dhseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
double[] h, int ldh, double[] wr,
double[] wi, double[] z, int ldz,
double[] work, int lwork );
public static native int LAPACKE_chseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatPointer h, int ldh,
@Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_chseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") FloatBuffer h, int ldh,
@Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_chseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_float*") float[] h, int ldh,
@Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zhseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoublePointer h, int ldh,
@Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zhseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") DoubleBuffer h, int ldh,
@Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zhseqr_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compz,
int n, int ilo, int ihi,
@Cast("lapack_complex_double*") double[] h, int ldh,
@Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_clacgv_work( int n, @Cast("lapack_complex_float*") FloatPointer x,
int incx );
public static native int LAPACKE_clacgv_work( int n, @Cast("lapack_complex_float*") FloatBuffer x,
int incx );
public static native int LAPACKE_clacgv_work( int n, @Cast("lapack_complex_float*") float[] x,
int incx );
public static native int LAPACKE_zlacgv_work( int n, @Cast("lapack_complex_double*") DoublePointer x,
int incx );
public static native int LAPACKE_zlacgv_work( int n, @Cast("lapack_complex_double*") DoubleBuffer x,
int incx );
public static native int LAPACKE_zlacgv_work( int n, @Cast("lapack_complex_double*") double[] x,
int incx );
public static native int LAPACKE_slacn2_work( int n, FloatPointer v, FloatPointer x,
IntPointer isgn, FloatPointer est, IntPointer kase,
IntPointer isave );
public static native int LAPACKE_slacn2_work( int n, FloatBuffer v, FloatBuffer x,
IntBuffer isgn, FloatBuffer est, IntBuffer kase,
IntBuffer isave );
public static native int LAPACKE_slacn2_work( int n, float[] v, float[] x,
int[] isgn, float[] est, int[] kase,
int[] isave );
public static native int LAPACKE_dlacn2_work( int n, DoublePointer v, DoublePointer x,
IntPointer isgn, DoublePointer est, IntPointer kase,
IntPointer isave );
public static native int LAPACKE_dlacn2_work( int n, DoubleBuffer v, DoubleBuffer x,
IntBuffer isgn, DoubleBuffer est, IntBuffer kase,
IntBuffer isave );
public static native int LAPACKE_dlacn2_work( int n, double[] v, double[] x,
int[] isgn, double[] est, int[] kase,
int[] isave );
public static native int LAPACKE_clacn2_work( int n, @Cast("lapack_complex_float*") FloatPointer v,
@Cast("lapack_complex_float*") FloatPointer x,
FloatPointer est, IntPointer kase,
IntPointer isave );
public static native int LAPACKE_clacn2_work( int n, @Cast("lapack_complex_float*") FloatBuffer v,
@Cast("lapack_complex_float*") FloatBuffer x,
FloatBuffer est, IntBuffer kase,
IntBuffer isave );
public static native int LAPACKE_clacn2_work( int n, @Cast("lapack_complex_float*") float[] v,
@Cast("lapack_complex_float*") float[] x,
float[] est, int[] kase,
int[] isave );
public static native int LAPACKE_zlacn2_work( int n, @Cast("lapack_complex_double*") DoublePointer v,
@Cast("lapack_complex_double*") DoublePointer x,
DoublePointer est, IntPointer kase,
IntPointer isave );
public static native int LAPACKE_zlacn2_work( int n, @Cast("lapack_complex_double*") DoubleBuffer v,
@Cast("lapack_complex_double*") DoubleBuffer x,
DoubleBuffer est, IntBuffer kase,
IntBuffer isave );
public static native int LAPACKE_zlacn2_work( int n, @Cast("lapack_complex_double*") double[] v,
@Cast("lapack_complex_double*") double[] x,
double[] est, int[] kase,
int[] isave );
public static native int LAPACKE_slacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const FloatPointer a, int lda,
FloatPointer b, int ldb );
public static native int LAPACKE_slacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const FloatBuffer a, int lda,
FloatBuffer b, int ldb );
public static native int LAPACKE_slacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const float[] a, int lda,
float[] b, int ldb );
public static native int LAPACKE_dlacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const DoublePointer a, int lda,
DoublePointer b, int ldb );
public static native int LAPACKE_dlacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const DoubleBuffer a, int lda,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dlacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const double[] a, int lda,
double[] b, int ldb );
public static native int LAPACKE_clacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_clacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_clacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zlacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zlacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zlacpy_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_clacp2_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_clacp2_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_clacp2_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zlacp2_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zlacp2_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zlacp2_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @Const double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_zlag2c_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer sa, int ldsa );
public static native int LAPACKE_zlag2c_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer sa, int ldsa );
public static native int LAPACKE_zlag2c_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_float*") float[] sa, int ldsa );
public static native int LAPACKE_slag2d_work( int matrix_layout, int m, int n,
@Const FloatPointer sa, int ldsa, DoublePointer a,
int lda );
public static native int LAPACKE_slag2d_work( int matrix_layout, int m, int n,
@Const FloatBuffer sa, int ldsa, DoubleBuffer a,
int lda );
public static native int LAPACKE_slag2d_work( int matrix_layout, int m, int n,
@Const float[] sa, int ldsa, double[] a,
int lda );
public static native int LAPACKE_dlag2s_work( int matrix_layout, int m, int n,
@Const DoublePointer a, int lda, FloatPointer sa,
int ldsa );
public static native int LAPACKE_dlag2s_work( int matrix_layout, int m, int n,
@Const DoubleBuffer a, int lda, FloatBuffer sa,
int ldsa );
public static native int LAPACKE_dlag2s_work( int matrix_layout, int m, int n,
@Const double[] a, int lda, float[] sa,
int ldsa );
public static native int LAPACKE_clag2z_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer sa, int ldsa,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_clag2z_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer sa, int ldsa,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_clag2z_work( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") float[] sa, int ldsa,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_slagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatPointer d,
FloatPointer a, int lda, IntPointer iseed,
FloatPointer work );
public static native int LAPACKE_slagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatBuffer d,
FloatBuffer a, int lda, IntBuffer iseed,
FloatBuffer work );
public static native int LAPACKE_slagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const float[] d,
float[] a, int lda, int[] iseed,
float[] work );
public static native int LAPACKE_dlagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const DoublePointer d,
DoublePointer a, int lda, IntPointer iseed,
DoublePointer work );
public static native int LAPACKE_dlagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const DoubleBuffer d,
DoubleBuffer a, int lda, IntBuffer iseed,
DoubleBuffer work );
public static native int LAPACKE_dlagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const double[] d,
double[] a, int lda, int[] iseed,
double[] work );
public static native int LAPACKE_clagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer iseed, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_clagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer iseed, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_clagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const float[] d,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] iseed, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zlagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer iseed,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zlagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer iseed,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zlagge_work( int matrix_layout, int m, int n,
int kl, int ku, @Const double[] d,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] iseed,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_claghe_work( int matrix_layout, int n, int k,
@Const FloatPointer d, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer iseed,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_claghe_work( int matrix_layout, int n, int k,
@Const FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer iseed,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_claghe_work( int matrix_layout, int n, int k,
@Const float[] d, @Cast("lapack_complex_float*") float[] a,
int lda, int[] iseed,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zlaghe_work( int matrix_layout, int n, int k,
@Const DoublePointer d, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer iseed,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zlaghe_work( int matrix_layout, int n, int k,
@Const DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer iseed,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zlaghe_work( int matrix_layout, int n, int k,
@Const double[] d, @Cast("lapack_complex_double*") double[] a,
int lda, int[] iseed,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_slagsy_work( int matrix_layout, int n, int k,
@Const FloatPointer d, FloatPointer a, int lda,
IntPointer iseed, FloatPointer work );
public static native int LAPACKE_slagsy_work( int matrix_layout, int n, int k,
@Const FloatBuffer d, FloatBuffer a, int lda,
IntBuffer iseed, FloatBuffer work );
public static native int LAPACKE_slagsy_work( int matrix_layout, int n, int k,
@Const float[] d, float[] a, int lda,
int[] iseed, float[] work );
public static native int LAPACKE_dlagsy_work( int matrix_layout, int n, int k,
@Const DoublePointer d, DoublePointer a, int lda,
IntPointer iseed, DoublePointer work );
public static native int LAPACKE_dlagsy_work( int matrix_layout, int n, int k,
@Const DoubleBuffer d, DoubleBuffer a, int lda,
IntBuffer iseed, DoubleBuffer work );
public static native int LAPACKE_dlagsy_work( int matrix_layout, int n, int k,
@Const double[] d, double[] a, int lda,
int[] iseed, double[] work );
public static native int LAPACKE_clagsy_work( int matrix_layout, int n, int k,
@Const FloatPointer d, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer iseed,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_clagsy_work( int matrix_layout, int n, int k,
@Const FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer iseed,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_clagsy_work( int matrix_layout, int n, int k,
@Const float[] d, @Cast("lapack_complex_float*") float[] a,
int lda, int[] iseed,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zlagsy_work( int matrix_layout, int n, int k,
@Const DoublePointer d, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer iseed,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zlagsy_work( int matrix_layout, int n, int k,
@Const DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer iseed,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zlagsy_work( int matrix_layout, int n, int k,
@Const double[] d, @Cast("lapack_complex_double*") double[] a,
int lda, int[] iseed,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_slapmr_work( int matrix_layout, int forwrd,
int m, int n, FloatPointer x,
int ldx, IntPointer k );
public static native int LAPACKE_slapmr_work( int matrix_layout, int forwrd,
int m, int n, FloatBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_slapmr_work( int matrix_layout, int forwrd,
int m, int n, float[] x,
int ldx, int[] k );
public static native int LAPACKE_dlapmr_work( int matrix_layout, int forwrd,
int m, int n, DoublePointer x,
int ldx, IntPointer k );
public static native int LAPACKE_dlapmr_work( int matrix_layout, int forwrd,
int m, int n, DoubleBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_dlapmr_work( int matrix_layout, int forwrd,
int m, int n, double[] x,
int ldx, int[] k );
public static native int LAPACKE_clapmr_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
IntPointer k );
public static native int LAPACKE_clapmr_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
IntBuffer k );
public static native int LAPACKE_clapmr_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_float*") float[] x, int ldx,
int[] k );
public static native int LAPACKE_zlapmr_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
IntPointer k );
public static native int LAPACKE_zlapmr_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
IntBuffer k );
public static native int LAPACKE_zlapmr_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_double*") double[] x, int ldx,
int[] k );
public static native int LAPACKE_slapmt_work( int matrix_layout, int forwrd,
int m, int n, FloatPointer x,
int ldx, IntPointer k );
public static native int LAPACKE_slapmt_work( int matrix_layout, int forwrd,
int m, int n, FloatBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_slapmt_work( int matrix_layout, int forwrd,
int m, int n, float[] x,
int ldx, int[] k );
public static native int LAPACKE_dlapmt_work( int matrix_layout, int forwrd,
int m, int n, DoublePointer x,
int ldx, IntPointer k );
public static native int LAPACKE_dlapmt_work( int matrix_layout, int forwrd,
int m, int n, DoubleBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_dlapmt_work( int matrix_layout, int forwrd,
int m, int n, double[] x,
int ldx, int[] k );
public static native int LAPACKE_clapmt_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
IntPointer k );
public static native int LAPACKE_clapmt_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
IntBuffer k );
public static native int LAPACKE_clapmt_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_float*") float[] x, int ldx,
int[] k );
public static native int LAPACKE_zlapmt_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
IntPointer k );
public static native int LAPACKE_zlapmt_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
IntBuffer k );
public static native int LAPACKE_zlapmt_work( int matrix_layout, int forwrd,
int m, int n,
@Cast("lapack_complex_double*") double[] x, int ldx,
int[] k );
public static native int LAPACKE_slartgp_work( float f, float g, FloatPointer cs, FloatPointer sn,
FloatPointer r );
public static native int LAPACKE_slartgp_work( float f, float g, FloatBuffer cs, FloatBuffer sn,
FloatBuffer r );
public static native int LAPACKE_slartgp_work( float f, float g, float[] cs, float[] sn,
float[] r );
public static native int LAPACKE_dlartgp_work( double f, double g, DoublePointer cs, DoublePointer sn,
DoublePointer r );
public static native int LAPACKE_dlartgp_work( double f, double g, DoubleBuffer cs, DoubleBuffer sn,
DoubleBuffer r );
public static native int LAPACKE_dlartgp_work( double f, double g, double[] cs, double[] sn,
double[] r );
public static native int LAPACKE_slartgs_work( float x, float y, float sigma, FloatPointer cs,
FloatPointer sn );
public static native int LAPACKE_slartgs_work( float x, float y, float sigma, FloatBuffer cs,
FloatBuffer sn );
public static native int LAPACKE_slartgs_work( float x, float y, float sigma, float[] cs,
float[] sn );
public static native int LAPACKE_dlartgs_work( double x, double y, double sigma, DoublePointer cs,
DoublePointer sn );
public static native int LAPACKE_dlartgs_work( double x, double y, double sigma, DoubleBuffer cs,
DoubleBuffer sn );
public static native int LAPACKE_dlartgs_work( double x, double y, double sigma, double[] cs,
double[] sn );
public static native float LAPACKE_slapy2_work( float x, float y );
public static native double LAPACKE_dlapy2_work( double x, double y );
public static native float LAPACKE_slapy3_work( float x, float y, float z );
public static native double LAPACKE_dlapy3_work( double x, double y, double z );
public static native float LAPACKE_slamch_work( @Cast("char") byte cmach );
public static native double LAPACKE_dlamch_work( @Cast("char") byte cmach );
public static native float LAPACKE_slange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const FloatPointer a, int lda,
FloatPointer work );
public static native float LAPACKE_slange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const FloatBuffer a, int lda,
FloatBuffer work );
public static native float LAPACKE_slange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const float[] a, int lda,
float[] work );
public static native double LAPACKE_dlange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const DoublePointer a, int lda,
DoublePointer work );
public static native double LAPACKE_dlange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const DoubleBuffer a, int lda,
DoubleBuffer work );
public static native double LAPACKE_dlange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Const double[] a, int lda,
double[] work );
public static native float LAPACKE_clange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, FloatPointer work );
public static native float LAPACKE_clange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer work );
public static native float LAPACKE_clange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_float*") float[] a,
int lda, float[] work );
public static native double LAPACKE_zlange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, DoublePointer work );
public static native double LAPACKE_zlange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer work );
public static native double LAPACKE_zlange_work( int matrix_layout, @Cast("char") byte norm, int m,
int n, @Cast("const lapack_complex_double*") double[] a,
int lda, double[] work );
public static native float LAPACKE_clanhe_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, FloatPointer work );
public static native float LAPACKE_clanhe_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer work );
public static native float LAPACKE_clanhe_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] a,
int lda, float[] work );
public static native double LAPACKE_zlanhe_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, DoublePointer work );
public static native double LAPACKE_zlanhe_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer work );
public static native double LAPACKE_zlanhe_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] a,
int lda, double[] work );
public static native float LAPACKE_slansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Const FloatPointer a, int lda,
FloatPointer work );
public static native float LAPACKE_slansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Const FloatBuffer a, int lda,
FloatBuffer work );
public static native float LAPACKE_slansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Const float[] a, int lda,
float[] work );
public static native double LAPACKE_dlansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Const DoublePointer a, int lda,
DoublePointer work );
public static native double LAPACKE_dlansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Const DoubleBuffer a, int lda,
DoubleBuffer work );
public static native double LAPACKE_dlansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Const double[] a, int lda,
double[] work );
public static native float LAPACKE_clansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, FloatPointer work );
public static native float LAPACKE_clansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, FloatBuffer work );
public static native float LAPACKE_clansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] a,
int lda, float[] work );
public static native double LAPACKE_zlansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, DoublePointer work );
public static native double LAPACKE_zlansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, DoubleBuffer work );
public static native double LAPACKE_zlansy_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] a,
int lda, double[] work );
public static native float LAPACKE_slantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n, @Const FloatPointer a,
int lda, FloatPointer work );
public static native float LAPACKE_slantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n, @Const FloatBuffer a,
int lda, FloatBuffer work );
public static native float LAPACKE_slantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n, @Const float[] a,
int lda, float[] work );
public static native double LAPACKE_dlantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n,
@Const DoublePointer a, int lda, DoublePointer work );
public static native double LAPACKE_dlantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer work );
public static native double LAPACKE_dlantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n,
@Const double[] a, int lda, double[] work );
public static native float LAPACKE_clantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer work );
public static native float LAPACKE_clantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer work );
public static native float LAPACKE_clantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] work );
public static native double LAPACKE_zlantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer work );
public static native double LAPACKE_zlantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer work );
public static native double LAPACKE_zlantr_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] work );
public static native int LAPACKE_slarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, @Const FloatPointer v,
int ldv, @Const FloatPointer t, int ldt,
FloatPointer c, int ldc, FloatPointer work,
int ldwork );
public static native int LAPACKE_slarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, @Const FloatBuffer v,
int ldv, @Const FloatBuffer t, int ldt,
FloatBuffer c, int ldc, FloatBuffer work,
int ldwork );
public static native int LAPACKE_slarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, @Const float[] v,
int ldv, @Const float[] t, int ldt,
float[] c, int ldc, float[] work,
int ldwork );
public static native int LAPACKE_dlarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, @Const DoublePointer v,
int ldv, @Const DoublePointer t, int ldt,
DoublePointer c, int ldc, DoublePointer work,
int ldwork );
public static native int LAPACKE_dlarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, @Const DoubleBuffer v,
int ldv, @Const DoubleBuffer t, int ldt,
DoubleBuffer c, int ldc, DoubleBuffer work,
int ldwork );
public static native int LAPACKE_dlarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, @Const double[] v,
int ldv, @Const double[] t, int ldt,
double[] c, int ldc, double[] work,
int ldwork );
public static native int LAPACKE_clarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k,
@Cast("const lapack_complex_float*") FloatPointer v, int ldv,
@Cast("const lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, int ldwork );
public static native int LAPACKE_clarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("const lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, int ldwork );
public static native int LAPACKE_clarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k,
@Cast("const lapack_complex_float*") float[] v, int ldv,
@Cast("const lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, int ldwork );
public static native int LAPACKE_zlarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k,
@Cast("const lapack_complex_double*") DoublePointer v, int ldv,
@Cast("const lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work,
int ldwork );
public static native int LAPACKE_zlarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("const lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work,
int ldwork );
public static native int LAPACKE_zlarfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k,
@Cast("const lapack_complex_double*") double[] v, int ldv,
@Cast("const lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work,
int ldwork );
public static native int LAPACKE_slarfg_work( int n, FloatPointer alpha, FloatPointer x,
int incx, FloatPointer tau );
public static native int LAPACKE_slarfg_work( int n, FloatBuffer alpha, FloatBuffer x,
int incx, FloatBuffer tau );
public static native int LAPACKE_slarfg_work( int n, float[] alpha, float[] x,
int incx, float[] tau );
public static native int LAPACKE_dlarfg_work( int n, DoublePointer alpha, DoublePointer x,
int incx, DoublePointer tau );
public static native int LAPACKE_dlarfg_work( int n, DoubleBuffer alpha, DoubleBuffer x,
int incx, DoubleBuffer tau );
public static native int LAPACKE_dlarfg_work( int n, double[] alpha, double[] x,
int incx, double[] tau );
public static native int LAPACKE_clarfg_work( int n, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer x, int incx,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native int LAPACKE_clarfg_work( int n, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer x, int incx,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native int LAPACKE_clarfg_work( int n, @Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] x, int incx,
@Cast("lapack_complex_float*") float[] tau );
public static native int LAPACKE_zlarfg_work( int n, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer x, int incx,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native int LAPACKE_zlarfg_work( int n, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer x, int incx,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native int LAPACKE_zlarfg_work( int n, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] x, int incx,
@Cast("lapack_complex_double*") double[] tau );
public static native int LAPACKE_slarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const FloatPointer v,
int ldv, @Const FloatPointer tau, FloatPointer t,
int ldt );
public static native int LAPACKE_slarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const FloatBuffer v,
int ldv, @Const FloatBuffer tau, FloatBuffer t,
int ldt );
public static native int LAPACKE_slarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const float[] v,
int ldv, @Const float[] tau, float[] t,
int ldt );
public static native int LAPACKE_dlarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const DoublePointer v,
int ldv, @Const DoublePointer tau, DoublePointer t,
int ldt );
public static native int LAPACKE_dlarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const DoubleBuffer v,
int ldv, @Const DoubleBuffer tau, DoubleBuffer t,
int ldt );
public static native int LAPACKE_dlarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k, @Const double[] v,
int ldv, @Const double[] tau, double[] t,
int ldt );
public static native int LAPACKE_clarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_float*") FloatPointer v, int ldv,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer t, int ldt );
public static native int LAPACKE_clarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt );
public static native int LAPACKE_clarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_float*") float[] v, int ldv,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] t, int ldt );
public static native int LAPACKE_zlarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_double*") DoublePointer v, int ldv,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer t, int ldt );
public static native int LAPACKE_zlarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt );
public static native int LAPACKE_zlarft_work( int matrix_layout, @Cast("char") byte direct, @Cast("char") byte storev,
int n, int k,
@Cast("const lapack_complex_double*") double[] v, int ldv,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] t, int ldt );
public static native int LAPACKE_slarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const FloatPointer v, float tau,
FloatPointer c, int ldc, FloatPointer work );
public static native int LAPACKE_slarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const FloatBuffer v, float tau,
FloatBuffer c, int ldc, FloatBuffer work );
public static native int LAPACKE_slarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const float[] v, float tau,
float[] c, int ldc, float[] work );
public static native int LAPACKE_dlarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const DoublePointer v, double tau,
DoublePointer c, int ldc, DoublePointer work );
public static native int LAPACKE_dlarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const DoubleBuffer v, double tau,
DoubleBuffer c, int ldc, DoubleBuffer work );
public static native int LAPACKE_dlarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Const double[] v, double tau,
double[] c, int ldc, double[] work );
public static native int LAPACKE_clarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_float*") FloatPointer v,
@ByVal @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_clarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_float*") FloatBuffer v,
@ByVal @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_clarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_float*") float[] v,
@ByVal @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zlarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_double*") DoublePointer v,
@ByVal @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zlarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_double*") DoubleBuffer v,
@ByVal @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zlarfx_work( int matrix_layout, @Cast("char") byte side, int m,
int n, @Cast("const lapack_complex_double*") double[] v,
@ByVal @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_slarnv_work( int idist, IntPointer iseed,
int n, FloatPointer x );
public static native int LAPACKE_slarnv_work( int idist, IntBuffer iseed,
int n, FloatBuffer x );
public static native int LAPACKE_slarnv_work( int idist, int[] iseed,
int n, float[] x );
public static native int LAPACKE_dlarnv_work( int idist, IntPointer iseed,
int n, DoublePointer x );
public static native int LAPACKE_dlarnv_work( int idist, IntBuffer iseed,
int n, DoubleBuffer x );
public static native int LAPACKE_dlarnv_work( int idist, int[] iseed,
int n, double[] x );
public static native int LAPACKE_clarnv_work( int idist, IntPointer iseed,
int n, @Cast("lapack_complex_float*") FloatPointer x );
public static native int LAPACKE_clarnv_work( int idist, IntBuffer iseed,
int n, @Cast("lapack_complex_float*") FloatBuffer x );
public static native int LAPACKE_clarnv_work( int idist, int[] iseed,
int n, @Cast("lapack_complex_float*") float[] x );
public static native int LAPACKE_zlarnv_work( int idist, IntPointer iseed,
int n, @Cast("lapack_complex_double*") DoublePointer x );
public static native int LAPACKE_zlarnv_work( int idist, IntBuffer iseed,
int n, @Cast("lapack_complex_double*") DoubleBuffer x );
public static native int LAPACKE_zlarnv_work( int idist, int[] iseed,
int n, @Cast("lapack_complex_double*") double[] x );
public static native int LAPACKE_slascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, FloatPointer a,
int lda );
public static native int LAPACKE_slascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, FloatBuffer a,
int lda );
public static native int LAPACKE_slascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, float[] a,
int lda );
public static native int LAPACKE_dlascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, DoublePointer a,
int lda );
public static native int LAPACKE_dlascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, DoubleBuffer a,
int lda );
public static native int LAPACKE_dlascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, double[] a,
int lda );
public static native int LAPACKE_clascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_clascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_clascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, float cfrom, float cto,
int m, int n, @Cast("lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_zlascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zlascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zlascl_work( int matrix_layout, @Cast("char") byte type, int kl,
int ku, double cfrom, double cto,
int m, int n, @Cast("lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_slaset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, float alpha, float beta, FloatPointer a,
int lda );
public static native int LAPACKE_slaset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, float alpha, float beta, FloatBuffer a,
int lda );
public static native int LAPACKE_slaset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, float alpha, float beta, float[] a,
int lda );
public static native int LAPACKE_dlaset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, double alpha, double beta,
DoublePointer a, int lda );
public static native int LAPACKE_dlaset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, double alpha, double beta,
DoubleBuffer a, int lda );
public static native int LAPACKE_dlaset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, double alpha, double beta,
double[] a, int lda );
public static native int LAPACKE_claset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_float*") FloatPointer alpha,
@ByVal @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_claset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_float*") FloatBuffer alpha,
@ByVal @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_claset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_float*") float[] alpha,
@ByVal @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zlaset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_double*") DoublePointer alpha,
@ByVal @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zlaset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_double*") DoubleBuffer alpha,
@ByVal @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zlaset_work( int matrix_layout, @Cast("char") byte uplo, int m,
int n, @ByVal @Cast("lapack_complex_double*") double[] alpha,
@ByVal @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_slasrt_work( @Cast("char") byte id, int n, FloatPointer d );
public static native int LAPACKE_slasrt_work( @Cast("char") byte id, int n, FloatBuffer d );
public static native int LAPACKE_slasrt_work( @Cast("char") byte id, int n, float[] d );
public static native int LAPACKE_dlasrt_work( @Cast("char") byte id, int n, DoublePointer d );
public static native int LAPACKE_dlasrt_work( @Cast("char") byte id, int n, DoubleBuffer d );
public static native int LAPACKE_dlasrt_work( @Cast("char") byte id, int n, double[] d );
public static native int LAPACKE_slaswp_work( int matrix_layout, int n, FloatPointer a,
int lda, int k1, int k2,
@Const IntPointer ipiv, int incx );
public static native int LAPACKE_slaswp_work( int matrix_layout, int n, FloatBuffer a,
int lda, int k1, int k2,
@Const IntBuffer ipiv, int incx );
public static native int LAPACKE_slaswp_work( int matrix_layout, int n, float[] a,
int lda, int k1, int k2,
@Const int[] ipiv, int incx );
public static native int LAPACKE_dlaswp_work( int matrix_layout, int n, DoublePointer a,
int lda, int k1, int k2,
@Const IntPointer ipiv, int incx );
public static native int LAPACKE_dlaswp_work( int matrix_layout, int n, DoubleBuffer a,
int lda, int k1, int k2,
@Const IntBuffer ipiv, int incx );
public static native int LAPACKE_dlaswp_work( int matrix_layout, int n, double[] a,
int lda, int k1, int k2,
@Const int[] ipiv, int incx );
public static native int LAPACKE_claswp_work( int matrix_layout, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
int k1, int k2,
@Const IntPointer ipiv, int incx );
public static native int LAPACKE_claswp_work( int matrix_layout, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
int k1, int k2,
@Const IntBuffer ipiv, int incx );
public static native int LAPACKE_claswp_work( int matrix_layout, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int k1, int k2,
@Const int[] ipiv, int incx );
public static native int LAPACKE_zlaswp_work( int matrix_layout, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
int k1, int k2,
@Const IntPointer ipiv, int incx );
public static native int LAPACKE_zlaswp_work( int matrix_layout, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
int k1, int k2,
@Const IntBuffer ipiv, int incx );
public static native int LAPACKE_zlaswp_work( int matrix_layout, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int k1, int k2,
@Const int[] ipiv, int incx );
public static native int LAPACKE_slatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntPointer iseed, @Cast("char") byte sym,
FloatPointer d, int mode, float cond,
float dmax, int kl, int ku,
@Cast("char") byte pack, FloatPointer a, int lda,
FloatPointer work );
public static native int LAPACKE_slatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntBuffer iseed, @Cast("char") byte sym,
FloatBuffer d, int mode, float cond,
float dmax, int kl, int ku,
@Cast("char") byte pack, FloatBuffer a, int lda,
FloatBuffer work );
public static native int LAPACKE_slatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, int[] iseed, @Cast("char") byte sym,
float[] d, int mode, float cond,
float dmax, int kl, int ku,
@Cast("char") byte pack, float[] a, int lda,
float[] work );
public static native int LAPACKE_dlatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntPointer iseed, @Cast("char") byte sym,
DoublePointer d, int mode, double cond,
double dmax, int kl, int ku,
@Cast("char") byte pack, DoublePointer a, int lda,
DoublePointer work );
public static native int LAPACKE_dlatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntBuffer iseed, @Cast("char") byte sym,
DoubleBuffer d, int mode, double cond,
double dmax, int kl, int ku,
@Cast("char") byte pack, DoubleBuffer a, int lda,
DoubleBuffer work );
public static native int LAPACKE_dlatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, int[] iseed, @Cast("char") byte sym,
double[] d, int mode, double cond,
double dmax, int kl, int ku,
@Cast("char") byte pack, double[] a, int lda,
double[] work );
public static native int LAPACKE_clatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntPointer iseed, @Cast("char") byte sym,
FloatPointer d, int mode, float cond,
float dmax, int kl, int ku,
@Cast("char") byte pack, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_clatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntBuffer iseed, @Cast("char") byte sym,
FloatBuffer d, int mode, float cond,
float dmax, int kl, int ku,
@Cast("char") byte pack, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_clatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, int[] iseed, @Cast("char") byte sym,
float[] d, int mode, float cond,
float dmax, int kl, int ku,
@Cast("char") byte pack, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zlatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntPointer iseed, @Cast("char") byte sym,
DoublePointer d, int mode, double cond,
double dmax, int kl, int ku,
@Cast("char") byte pack, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zlatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, IntBuffer iseed, @Cast("char") byte sym,
DoubleBuffer d, int mode, double cond,
double dmax, int kl, int ku,
@Cast("char") byte pack, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zlatms_work( int matrix_layout, int m, int n,
@Cast("char") byte dist, int[] iseed, @Cast("char") byte sym,
double[] d, int mode, double cond,
double dmax, int kl, int ku,
@Cast("char") byte pack, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_slauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda );
public static native int LAPACKE_slauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda );
public static native int LAPACKE_slauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda );
public static native int LAPACKE_dlauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda );
public static native int LAPACKE_dlauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda );
public static native int LAPACKE_dlauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda );
public static native int LAPACKE_clauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_clauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_clauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zlauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zlauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zlauum_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_sopgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, @Const FloatPointer tau, FloatPointer q,
int ldq, FloatPointer work );
public static native int LAPACKE_sopgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, @Const FloatBuffer tau, FloatBuffer q,
int ldq, FloatBuffer work );
public static native int LAPACKE_sopgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, @Const float[] tau, float[] q,
int ldq, float[] work );
public static native int LAPACKE_dopgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, @Const DoublePointer tau, DoublePointer q,
int ldq, DoublePointer work );
public static native int LAPACKE_dopgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, @Const DoubleBuffer tau, DoubleBuffer q,
int ldq, DoubleBuffer work );
public static native int LAPACKE_dopgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, @Const double[] tau, double[] q,
int ldq, double[] work );
public static native int LAPACKE_sopmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const FloatPointer ap, @Const FloatPointer tau, FloatPointer c,
int ldc, FloatPointer work );
public static native int LAPACKE_sopmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const FloatBuffer ap, @Const FloatBuffer tau, FloatBuffer c,
int ldc, FloatBuffer work );
public static native int LAPACKE_sopmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const float[] ap, @Const float[] tau, float[] c,
int ldc, float[] work );
public static native int LAPACKE_dopmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const DoublePointer ap, @Const DoublePointer tau, DoublePointer c,
int ldc, DoublePointer work );
public static native int LAPACKE_dopmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const DoubleBuffer ap, @Const DoubleBuffer tau, DoubleBuffer c,
int ldc, DoubleBuffer work );
public static native int LAPACKE_dopmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const double[] ap, @Const double[] tau, double[] c,
int ldc, double[] work );
public static native int LAPACKE_sorgbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, FloatPointer a,
int lda, @Const FloatPointer tau, FloatPointer work,
int lwork );
public static native int LAPACKE_sorgbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, FloatBuffer a,
int lda, @Const FloatBuffer tau, FloatBuffer work,
int lwork );
public static native int LAPACKE_sorgbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, float[] a,
int lda, @Const float[] tau, float[] work,
int lwork );
public static native int LAPACKE_dorgbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, DoublePointer a,
int lda, @Const DoublePointer tau, DoublePointer work,
int lwork );
public static native int LAPACKE_dorgbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, DoubleBuffer a,
int lda, @Const DoubleBuffer tau, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dorgbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k, double[] a,
int lda, @Const double[] tau, double[] work,
int lwork );
public static native int LAPACKE_sorghr_work( int matrix_layout, int n, int ilo,
int ihi, FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer work,
int lwork );
public static native int LAPACKE_sorghr_work( int matrix_layout, int n, int ilo,
int ihi, FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer work,
int lwork );
public static native int LAPACKE_sorghr_work( int matrix_layout, int n, int ilo,
int ihi, float[] a, int lda,
@Const float[] tau, float[] work,
int lwork );
public static native int LAPACKE_dorghr_work( int matrix_layout, int n, int ilo,
int ihi, DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer work,
int lwork );
public static native int LAPACKE_dorghr_work( int matrix_layout, int n, int ilo,
int ihi, DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dorghr_work( int matrix_layout, int n, int ilo,
int ihi, double[] a, int lda,
@Const double[] tau, double[] work,
int lwork );
public static native int LAPACKE_sorglq_work( int matrix_layout, int m, int n,
int k, FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer work,
int lwork );
public static native int LAPACKE_sorglq_work( int matrix_layout, int m, int n,
int k, FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer work,
int lwork );
public static native int LAPACKE_sorglq_work( int matrix_layout, int m, int n,
int k, float[] a, int lda,
@Const float[] tau, float[] work,
int lwork );
public static native int LAPACKE_dorglq_work( int matrix_layout, int m, int n,
int k, DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer work,
int lwork );
public static native int LAPACKE_dorglq_work( int matrix_layout, int m, int n,
int k, DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dorglq_work( int matrix_layout, int m, int n,
int k, double[] a, int lda,
@Const double[] tau, double[] work,
int lwork );
public static native int LAPACKE_sorgql_work( int matrix_layout, int m, int n,
int k, FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer work,
int lwork );
public static native int LAPACKE_sorgql_work( int matrix_layout, int m, int n,
int k, FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer work,
int lwork );
public static native int LAPACKE_sorgql_work( int matrix_layout, int m, int n,
int k, float[] a, int lda,
@Const float[] tau, float[] work,
int lwork );
public static native int LAPACKE_dorgql_work( int matrix_layout, int m, int n,
int k, DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer work,
int lwork );
public static native int LAPACKE_dorgql_work( int matrix_layout, int m, int n,
int k, DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dorgql_work( int matrix_layout, int m, int n,
int k, double[] a, int lda,
@Const double[] tau, double[] work,
int lwork );
public static native int LAPACKE_sorgqr_work( int matrix_layout, int m, int n,
int k, FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer work,
int lwork );
public static native int LAPACKE_sorgqr_work( int matrix_layout, int m, int n,
int k, FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer work,
int lwork );
public static native int LAPACKE_sorgqr_work( int matrix_layout, int m, int n,
int k, float[] a, int lda,
@Const float[] tau, float[] work,
int lwork );
public static native int LAPACKE_dorgqr_work( int matrix_layout, int m, int n,
int k, DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer work,
int lwork );
public static native int LAPACKE_dorgqr_work( int matrix_layout, int m, int n,
int k, DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dorgqr_work( int matrix_layout, int m, int n,
int k, double[] a, int lda,
@Const double[] tau, double[] work,
int lwork );
public static native int LAPACKE_sorgrq_work( int matrix_layout, int m, int n,
int k, FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer work,
int lwork );
public static native int LAPACKE_sorgrq_work( int matrix_layout, int m, int n,
int k, FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer work,
int lwork );
public static native int LAPACKE_sorgrq_work( int matrix_layout, int m, int n,
int k, float[] a, int lda,
@Const float[] tau, float[] work,
int lwork );
public static native int LAPACKE_dorgrq_work( int matrix_layout, int m, int n,
int k, DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer work,
int lwork );
public static native int LAPACKE_dorgrq_work( int matrix_layout, int m, int n,
int k, DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dorgrq_work( int matrix_layout, int m, int n,
int k, double[] a, int lda,
@Const double[] tau, double[] work,
int lwork );
public static native int LAPACKE_sorgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda, @Const FloatPointer tau,
FloatPointer work, int lwork );
public static native int LAPACKE_sorgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda, @Const FloatBuffer tau,
FloatBuffer work, int lwork );
public static native int LAPACKE_sorgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda, @Const float[] tau,
float[] work, int lwork );
public static native int LAPACKE_dorgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda, @Const DoublePointer tau,
DoublePointer work, int lwork );
public static native int LAPACKE_dorgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda, @Const DoubleBuffer tau,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dorgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda, @Const double[] tau,
double[] work, int lwork );
public static native int LAPACKE_sormbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc,
FloatPointer work, int lwork );
public static native int LAPACKE_sormbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc,
FloatBuffer work, int lwork );
public static native int LAPACKE_sormbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc,
float[] work, int lwork );
public static native int LAPACKE_dormbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc,
DoublePointer work, int lwork );
public static native int LAPACKE_dormbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dormbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc,
double[] work, int lwork );
public static native int LAPACKE_sormhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc,
FloatPointer work, int lwork );
public static native int LAPACKE_sormhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc,
FloatBuffer work, int lwork );
public static native int LAPACKE_sormhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc,
float[] work, int lwork );
public static native int LAPACKE_dormhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc,
DoublePointer work, int lwork );
public static native int LAPACKE_dormhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dormhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc,
double[] work, int lwork );
public static native int LAPACKE_sormlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc,
FloatPointer work, int lwork );
public static native int LAPACKE_sormlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc,
FloatBuffer work, int lwork );
public static native int LAPACKE_sormlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc,
float[] work, int lwork );
public static native int LAPACKE_dormlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc,
DoublePointer work, int lwork );
public static native int LAPACKE_dormlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dormlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc,
double[] work, int lwork );
public static native int LAPACKE_sormql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc,
FloatPointer work, int lwork );
public static native int LAPACKE_sormql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc,
FloatBuffer work, int lwork );
public static native int LAPACKE_sormql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc,
float[] work, int lwork );
public static native int LAPACKE_dormql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc,
DoublePointer work, int lwork );
public static native int LAPACKE_dormql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dormql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc,
double[] work, int lwork );
public static native int LAPACKE_sormqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc,
FloatPointer work, int lwork );
public static native int LAPACKE_sormqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc,
FloatBuffer work, int lwork );
public static native int LAPACKE_sormqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc,
float[] work, int lwork );
public static native int LAPACKE_dormqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc,
DoublePointer work, int lwork );
public static native int LAPACKE_dormqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dormqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc,
double[] work, int lwork );
public static native int LAPACKE_sormrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc,
FloatPointer work, int lwork );
public static native int LAPACKE_sormrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc,
FloatBuffer work, int lwork );
public static native int LAPACKE_sormrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc,
float[] work, int lwork );
public static native int LAPACKE_dormrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc,
DoublePointer work, int lwork );
public static native int LAPACKE_dormrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dormrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc,
double[] work, int lwork );
public static native int LAPACKE_sormrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc,
FloatPointer work, int lwork );
public static native int LAPACKE_sormrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc,
FloatBuffer work, int lwork );
public static native int LAPACKE_sormrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc,
float[] work, int lwork );
public static native int LAPACKE_dormrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc,
DoublePointer work, int lwork );
public static native int LAPACKE_dormrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dormrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc,
double[] work, int lwork );
public static native int LAPACKE_sormtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const FloatPointer a, int lda,
@Const FloatPointer tau, FloatPointer c, int ldc,
FloatPointer work, int lwork );
public static native int LAPACKE_sormtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const FloatBuffer a, int lda,
@Const FloatBuffer tau, FloatBuffer c, int ldc,
FloatBuffer work, int lwork );
public static native int LAPACKE_sormtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const float[] a, int lda,
@Const float[] tau, float[] c, int ldc,
float[] work, int lwork );
public static native int LAPACKE_dormtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const DoublePointer a, int lda,
@Const DoublePointer tau, DoublePointer c, int ldc,
DoublePointer work, int lwork );
public static native int LAPACKE_dormtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const DoubleBuffer a, int lda,
@Const DoubleBuffer tau, DoubleBuffer c, int ldc,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dormtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Const double[] a, int lda,
@Const double[] tau, double[] c, int ldc,
double[] work, int lwork );
public static native int LAPACKE_spbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const FloatPointer ab, int ldab,
float anorm, FloatPointer rcond, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_spbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const FloatBuffer ab, int ldab,
float anorm, FloatBuffer rcond, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_spbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const float[] ab, int ldab,
float anorm, float[] rcond, float[] work,
int[] iwork );
public static native int LAPACKE_dpbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const DoublePointer ab,
int ldab, double anorm, DoublePointer rcond,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dpbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const DoubleBuffer ab,
int ldab, double anorm, DoubleBuffer rcond,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dpbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const double[] ab,
int ldab, double anorm, double[] rcond,
double[] work, int[] iwork );
public static native int LAPACKE_cpbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") FloatPointer ab,
int ldab, float anorm, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cpbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab, float anorm, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cpbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") float[] ab,
int ldab, float anorm, float[] rcond,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zpbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, double anorm, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zpbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, double anorm, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zpbcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") double[] ab,
int ldab, double anorm, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_spbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const FloatPointer ab, int ldab,
FloatPointer s, FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_spbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const FloatBuffer ab, int ldab,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_spbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const float[] ab, int ldab,
float[] s, float[] scond, float[] amax );
public static native int LAPACKE_dpbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const DoublePointer ab,
int ldab, DoublePointer s, DoublePointer scond,
DoublePointer amax );
public static native int LAPACKE_dpbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const DoubleBuffer ab,
int ldab, DoubleBuffer s, DoubleBuffer scond,
DoubleBuffer amax );
public static native int LAPACKE_dpbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Const double[] ab,
int ldab, double[] s, double[] scond,
double[] amax );
public static native int LAPACKE_cpbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") FloatPointer ab,
int ldab, FloatPointer s, FloatPointer scond,
FloatPointer amax );
public static native int LAPACKE_cpbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab, FloatBuffer s, FloatBuffer scond,
FloatBuffer amax );
public static native int LAPACKE_cpbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_float*") float[] ab,
int ldab, float[] s, float[] scond,
float[] amax );
public static native int LAPACKE_zpbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer s, DoublePointer scond,
DoublePointer amax );
public static native int LAPACKE_zpbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer s, DoubleBuffer scond,
DoubleBuffer amax );
public static native int LAPACKE_zpbequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("const lapack_complex_double*") double[] ab,
int ldab, double[] s, double[] scond,
double[] amax );
public static native int LAPACKE_spbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const FloatPointer ab,
int ldab, @Const FloatPointer afb,
int ldafb, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_spbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const FloatBuffer ab,
int ldab, @Const FloatBuffer afb,
int ldafb, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_spbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const float[] ab,
int ldab, @Const float[] afb,
int ldafb, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr, float[] work,
int[] iwork );
public static native int LAPACKE_dpbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Const DoublePointer ab, int ldab,
@Const DoublePointer afb, int ldafb,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dpbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Const DoubleBuffer ab, int ldab,
@Const DoubleBuffer afb, int ldafb,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dpbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Const double[] ab, int ldab,
@Const double[] afb, int ldafb,
@Const double[] b, int ldb, double[] x,
int ldx, double[] ferr, double[] berr,
double[] work, int[] iwork );
public static native int LAPACKE_cpbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("const lapack_complex_float*") FloatPointer afb,
int ldafb, @Cast("const lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cpbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("const lapack_complex_float*") FloatBuffer afb,
int ldafb, @Cast("const lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cpbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Cast("const lapack_complex_float*") float[] afb,
int ldafb, @Cast("const lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zpbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab,
@Cast("const lapack_complex_double*") DoublePointer afb,
int ldafb,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zpbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab,
@Cast("const lapack_complex_double*") DoubleBuffer afb,
int ldafb,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zpbrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") double[] ab,
int ldab,
@Cast("const lapack_complex_double*") double[] afb,
int ldafb,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_spbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, FloatPointer bb, int ldbb );
public static native int LAPACKE_spbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, FloatBuffer bb, int ldbb );
public static native int LAPACKE_spbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, float[] bb, int ldbb );
public static native int LAPACKE_dpbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, DoublePointer bb, int ldbb );
public static native int LAPACKE_dpbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, DoubleBuffer bb, int ldbb );
public static native int LAPACKE_dpbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, double[] bb, int ldbb );
public static native int LAPACKE_cpbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_float*") FloatPointer bb,
int ldbb );
public static native int LAPACKE_cpbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_float*") FloatBuffer bb,
int ldbb );
public static native int LAPACKE_cpbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_float*") float[] bb,
int ldbb );
public static native int LAPACKE_zpbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_double*") DoublePointer bb,
int ldbb );
public static native int LAPACKE_zpbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_double*") DoubleBuffer bb,
int ldbb );
public static native int LAPACKE_zpbstf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kb, @Cast("lapack_complex_double*") double[] bb,
int ldbb );
public static native int LAPACKE_spbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, FloatPointer ab,
int ldab, FloatPointer b, int ldb );
public static native int LAPACKE_spbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, FloatBuffer ab,
int ldab, FloatBuffer b, int ldb );
public static native int LAPACKE_spbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, float[] ab,
int ldab, float[] b, int ldb );
public static native int LAPACKE_dpbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, DoublePointer ab,
int ldab, DoublePointer b, int ldb );
public static native int LAPACKE_dpbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, DoubleBuffer ab,
int ldab, DoubleBuffer b, int ldb );
public static native int LAPACKE_dpbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, double[] ab,
int ldab, double[] b, int ldb );
public static native int LAPACKE_cpbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zpbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zpbsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_spbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
FloatPointer ab, int ldab, FloatPointer afb,
int ldafb, @Cast("char*") BytePointer equed, FloatPointer s,
FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, FloatPointer work, IntPointer iwork );
public static native int LAPACKE_spbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
FloatBuffer ab, int ldab, FloatBuffer afb,
int ldafb, @Cast("char*") ByteBuffer equed, FloatBuffer s,
FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_spbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
float[] ab, int ldab, float[] afb,
int ldafb, @Cast("char*") byte[] equed, float[] s,
float[] b, int ldb, float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, float[] work, int[] iwork );
public static native int LAPACKE_dpbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
DoublePointer ab, int ldab, DoublePointer afb,
int ldafb, @Cast("char*") BytePointer equed, DoublePointer s,
DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dpbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
DoubleBuffer ab, int ldab, DoubleBuffer afb,
int ldafb, @Cast("char*") ByteBuffer equed, DoubleBuffer s,
DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dpbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
double[] ab, int ldab, double[] afb,
int ldafb, @Cast("char*") byte[] equed, double[] s,
double[] b, int ldb, double[] x,
int ldx, double[] rcond, double[] ferr,
double[] berr, double[] work, int[] iwork );
public static native int LAPACKE_cpbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
@Cast("lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer afb, int ldafb,
@Cast("char*") BytePointer equed, FloatPointer s, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_cpbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer afb, int ldafb,
@Cast("char*") ByteBuffer equed, FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_cpbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
@Cast("lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] afb, int ldafb,
@Cast("char*") byte[] equed, float[] s, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_zpbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
@Cast("lapack_complex_double*") DoublePointer ab, int ldab,
@Cast("lapack_complex_double*") DoublePointer afb, int ldafb,
@Cast("char*") BytePointer equed, DoublePointer s,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zpbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ab, int ldab,
@Cast("lapack_complex_double*") DoubleBuffer afb, int ldafb,
@Cast("char*") ByteBuffer equed, DoubleBuffer s,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zpbsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int kd, int nrhs,
@Cast("lapack_complex_double*") double[] ab, int ldab,
@Cast("lapack_complex_double*") double[] afb, int ldafb,
@Cast("char*") byte[] equed, double[] s,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_spbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, FloatPointer ab, int ldab );
public static native int LAPACKE_spbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, FloatBuffer ab, int ldab );
public static native int LAPACKE_spbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, float[] ab, int ldab );
public static native int LAPACKE_dpbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, DoublePointer ab, int ldab );
public static native int LAPACKE_dpbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, DoubleBuffer ab, int ldab );
public static native int LAPACKE_dpbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, double[] ab, int ldab );
public static native int LAPACKE_cpbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatPointer ab,
int ldab );
public static native int LAPACKE_cpbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") FloatBuffer ab,
int ldab );
public static native int LAPACKE_cpbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_float*") float[] ab,
int ldab );
public static native int LAPACKE_zpbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoublePointer ab,
int ldab );
public static native int LAPACKE_zpbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") DoubleBuffer ab,
int ldab );
public static native int LAPACKE_zpbtrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, @Cast("lapack_complex_double*") double[] ab,
int ldab );
public static native int LAPACKE_spbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const FloatPointer ab,
int ldab, FloatPointer b, int ldb );
public static native int LAPACKE_spbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const FloatBuffer ab,
int ldab, FloatBuffer b, int ldb );
public static native int LAPACKE_spbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs, @Const float[] ab,
int ldab, float[] b, int ldb );
public static native int LAPACKE_dpbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Const DoublePointer ab, int ldab, DoublePointer b,
int ldb );
public static native int LAPACKE_dpbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Const DoubleBuffer ab, int ldab, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dpbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Const double[] ab, int ldab, double[] b,
int ldb );
public static native int LAPACKE_cpbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zpbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zpbtrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int kd, int nrhs,
@Cast("const lapack_complex_double*") double[] ab,
int ldab, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_spftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, FloatPointer a );
public static native int LAPACKE_spftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, FloatBuffer a );
public static native int LAPACKE_spftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, float[] a );
public static native int LAPACKE_dpftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, DoublePointer a );
public static native int LAPACKE_dpftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, DoubleBuffer a );
public static native int LAPACKE_dpftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, double[] a );
public static native int LAPACKE_cpftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a );
public static native int LAPACKE_cpftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a );
public static native int LAPACKE_cpftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a );
public static native int LAPACKE_zpftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a );
public static native int LAPACKE_zpftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a );
public static native int LAPACKE_zpftrf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a );
public static native int LAPACKE_spftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, FloatPointer a );
public static native int LAPACKE_spftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, FloatBuffer a );
public static native int LAPACKE_spftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, float[] a );
public static native int LAPACKE_dpftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, DoublePointer a );
public static native int LAPACKE_dpftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, DoubleBuffer a );
public static native int LAPACKE_dpftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, double[] a );
public static native int LAPACKE_cpftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatPointer a );
public static native int LAPACKE_cpftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") FloatBuffer a );
public static native int LAPACKE_cpftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_float*") float[] a );
public static native int LAPACKE_zpftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoublePointer a );
public static native int LAPACKE_zpftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") DoubleBuffer a );
public static native int LAPACKE_zpftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("lapack_complex_double*") double[] a );
public static native int LAPACKE_spftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const FloatPointer a,
FloatPointer b, int ldb );
public static native int LAPACKE_spftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const FloatBuffer a,
FloatBuffer b, int ldb );
public static native int LAPACKE_spftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const float[] a,
float[] b, int ldb );
public static native int LAPACKE_dpftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const DoublePointer a,
DoublePointer b, int ldb );
public static native int LAPACKE_dpftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const DoubleBuffer a,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dpftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs, @Const double[] a,
double[] b, int ldb );
public static native int LAPACKE_cpftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer a,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] a,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer a,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zpftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zpftrs_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] a,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_spocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer a, int lda, float anorm,
FloatPointer rcond, FloatPointer work, IntPointer iwork );
public static native int LAPACKE_spocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer a, int lda, float anorm,
FloatBuffer rcond, FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_spocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] a, int lda, float anorm,
float[] rcond, float[] work, int[] iwork );
public static native int LAPACKE_dpocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer a, int lda, double anorm,
DoublePointer rcond, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dpocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer a, int lda, double anorm,
DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dpocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] a, int lda, double anorm,
double[] rcond, double[] work,
int[] iwork );
public static native int LAPACKE_cpocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
float anorm, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cpocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
float anorm, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cpocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float anorm, float[] rcond,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zpocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
double anorm, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zpocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
double anorm, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zpocon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double anorm, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_spoequ_work( int matrix_layout, int n, @Const FloatPointer a,
int lda, FloatPointer s, FloatPointer scond,
FloatPointer amax );
public static native int LAPACKE_spoequ_work( int matrix_layout, int n, @Const FloatBuffer a,
int lda, FloatBuffer s, FloatBuffer scond,
FloatBuffer amax );
public static native int LAPACKE_spoequ_work( int matrix_layout, int n, @Const float[] a,
int lda, float[] s, float[] scond,
float[] amax );
public static native int LAPACKE_dpoequ_work( int matrix_layout, int n, @Const DoublePointer a,
int lda, DoublePointer s, DoublePointer scond,
DoublePointer amax );
public static native int LAPACKE_dpoequ_work( int matrix_layout, int n, @Const DoubleBuffer a,
int lda, DoubleBuffer s, DoubleBuffer scond,
DoubleBuffer amax );
public static native int LAPACKE_dpoequ_work( int matrix_layout, int n, @Const double[] a,
int lda, double[] s, double[] scond,
double[] amax );
public static native int LAPACKE_cpoequ_work( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer s, FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_cpoequ_work( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_cpoequ_work( int matrix_layout, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] s, float[] scond, float[] amax );
public static native int LAPACKE_zpoequ_work( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer s, DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_zpoequ_work( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_zpoequ_work( int matrix_layout, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] s, double[] scond, double[] amax );
public static native int LAPACKE_spoequb_work( int matrix_layout, int n, @Const FloatPointer a,
int lda, FloatPointer s, FloatPointer scond,
FloatPointer amax );
public static native int LAPACKE_spoequb_work( int matrix_layout, int n, @Const FloatBuffer a,
int lda, FloatBuffer s, FloatBuffer scond,
FloatBuffer amax );
public static native int LAPACKE_spoequb_work( int matrix_layout, int n, @Const float[] a,
int lda, float[] s, float[] scond,
float[] amax );
public static native int LAPACKE_dpoequb_work( int matrix_layout, int n,
@Const DoublePointer a, int lda, DoublePointer s,
DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_dpoequb_work( int matrix_layout, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer s,
DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_dpoequb_work( int matrix_layout, int n,
@Const double[] a, int lda, double[] s,
double[] scond, double[] amax );
public static native int LAPACKE_cpoequb_work( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer s, FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_cpoequb_work( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_cpoequb_work( int matrix_layout, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] s, float[] scond, float[] amax );
public static native int LAPACKE_zpoequb_work( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer s, DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_zpoequb_work( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_zpoequb_work( int matrix_layout, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] s, double[] scond, double[] amax );
public static native int LAPACKE_sporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const FloatPointer af, int ldaf,
@Const FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const FloatBuffer af, int ldaf,
@Const FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
@Const float[] af, int ldaf,
@Const float[] b, int ldb, float[] x,
int ldx, float[] ferr, float[] berr,
float[] work, int[] iwork );
public static native int LAPACKE_dporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a,
int lda, @Const DoublePointer af,
int ldaf, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a,
int lda, @Const DoubleBuffer af,
int ldaf, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a,
int lda, @Const double[] af,
int ldaf, @Const double[] b,
int ldb, double[] x, int ldx,
double[] ferr, double[] berr, double[] work,
int[] iwork );
public static native int LAPACKE_cporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer af,
int ldaf, @Cast("const lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer af,
int ldaf, @Cast("const lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] af,
int ldaf, @Cast("const lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer af,
int ldaf, @Cast("const lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer af,
int ldaf, @Cast("const lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zporfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] af,
int ldaf, @Cast("const lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer a, int lda,
FloatPointer b, int ldb );
public static native int LAPACKE_sposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer a, int lda,
FloatBuffer b, int ldb );
public static native int LAPACKE_sposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] a, int lda,
float[] b, int ldb );
public static native int LAPACKE_dposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer b, int ldb );
public static native int LAPACKE_dposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] a, int lda,
double[] b, int ldb );
public static native int LAPACKE_cposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_dsposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer work, FloatPointer swork,
IntPointer iter );
public static native int LAPACKE_dsposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer work, FloatBuffer swork,
IntBuffer iter );
public static native int LAPACKE_dsposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] a, int lda,
double[] b, int ldb, double[] x,
int ldx, double[] work, float[] swork,
int[] iter );
public static native int LAPACKE_zcposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, @Cast("lapack_complex_double*") DoublePointer work,
@Cast("lapack_complex_float*") FloatPointer swork, DoublePointer rwork,
IntPointer iter );
public static native int LAPACKE_zcposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, @Cast("lapack_complex_double*") DoubleBuffer work,
@Cast("lapack_complex_float*") FloatBuffer swork, DoubleBuffer rwork,
IntBuffer iter );
public static native int LAPACKE_zcposv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, @Cast("lapack_complex_double*") double[] work,
@Cast("lapack_complex_float*") float[] swork, double[] rwork,
int[] iter );
public static native int LAPACKE_sposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, FloatPointer a,
int lda, FloatPointer af, int ldaf,
@Cast("char*") BytePointer equed, FloatPointer s, FloatPointer b, int ldb,
FloatPointer x, int ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_sposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, FloatBuffer a,
int lda, FloatBuffer af, int ldaf,
@Cast("char*") ByteBuffer equed, FloatBuffer s, FloatBuffer b, int ldb,
FloatBuffer x, int ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_sposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, float[] a,
int lda, float[] af, int ldaf,
@Cast("char*") byte[] equed, float[] s, float[] b, int ldb,
float[] x, int ldx, float[] rcond,
float[] ferr, float[] berr, float[] work,
int[] iwork );
public static native int LAPACKE_dposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, DoublePointer a,
int lda, DoublePointer af, int ldaf,
@Cast("char*") BytePointer equed, DoublePointer s, DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, DoubleBuffer a,
int lda, DoubleBuffer af, int ldaf,
@Cast("char*") ByteBuffer equed, DoubleBuffer s, DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, double[] a,
int lda, double[] af, int ldaf,
@Cast("char*") byte[] equed, double[] s, double[] b,
int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
double[] work, int[] iwork );
public static native int LAPACKE_cposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer af, int ldaf,
@Cast("char*") BytePointer equed, FloatPointer s, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_cposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer af, int ldaf,
@Cast("char*") ByteBuffer equed, FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_cposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] af, int ldaf,
@Cast("char*") byte[] equed, float[] s, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_zposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer af, int ldaf,
@Cast("char*") BytePointer equed, DoublePointer s,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer af, int ldaf,
@Cast("char*") ByteBuffer equed, DoubleBuffer s,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zposvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] af, int ldaf,
@Cast("char*") byte[] equed, double[] s,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_spotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda );
public static native int LAPACKE_spotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda );
public static native int LAPACKE_spotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda );
public static native int LAPACKE_dpotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda );
public static native int LAPACKE_dpotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda );
public static native int LAPACKE_dpotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda );
public static native int LAPACKE_cpotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_cpotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_cpotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zpotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zpotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zpotrf2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_spotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda );
public static native int LAPACKE_spotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda );
public static native int LAPACKE_spotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda );
public static native int LAPACKE_dpotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda );
public static native int LAPACKE_dpotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda );
public static native int LAPACKE_dpotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda );
public static native int LAPACKE_cpotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_cpotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_cpotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zpotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zpotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zpotrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_spotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda );
public static native int LAPACKE_spotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda );
public static native int LAPACKE_spotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda );
public static native int LAPACKE_dpotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda );
public static native int LAPACKE_dpotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda );
public static native int LAPACKE_dpotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda );
public static native int LAPACKE_cpotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_cpotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_cpotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zpotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zpotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zpotri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_spotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
FloatPointer b, int ldb );
public static native int LAPACKE_spotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
FloatBuffer b, int ldb );
public static native int LAPACKE_spotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
float[] b, int ldb );
public static native int LAPACKE_dpotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a,
int lda, DoublePointer b, int ldb );
public static native int LAPACKE_dpotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a,
int lda, DoubleBuffer b, int ldb );
public static native int LAPACKE_dpotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a,
int lda, double[] b, int ldb );
public static native int LAPACKE_cpotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cpotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cpotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zpotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zpotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zpotrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_sppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, float anorm, FloatPointer rcond,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, float anorm, FloatBuffer rcond,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, float anorm, float[] rcond,
float[] work, int[] iwork );
public static native int LAPACKE_dppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, double anorm, DoublePointer rcond,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, double anorm, DoubleBuffer rcond,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, double anorm, double[] rcond,
double[] work, int[] iwork );
public static native int LAPACKE_cppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap, float anorm,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_cppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap, float anorm,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_cppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap, float anorm,
float[] rcond, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_zppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap, double anorm,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork );
public static native int LAPACKE_zppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap, double anorm,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork );
public static native int LAPACKE_zppcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap, double anorm,
double[] rcond, @Cast("lapack_complex_double*") double[] work,
double[] rwork );
public static native int LAPACKE_sppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, FloatPointer s, FloatPointer scond,
FloatPointer amax );
public static native int LAPACKE_sppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, FloatBuffer s, FloatBuffer scond,
FloatBuffer amax );
public static native int LAPACKE_sppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, float[] s, float[] scond,
float[] amax );
public static native int LAPACKE_dppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, DoublePointer s, DoublePointer scond,
DoublePointer amax );
public static native int LAPACKE_dppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, DoubleBuffer s, DoubleBuffer scond,
DoubleBuffer amax );
public static native int LAPACKE_dppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, double[] s, double[] scond,
double[] amax );
public static native int LAPACKE_cppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap, FloatPointer s,
FloatPointer scond, FloatPointer amax );
public static native int LAPACKE_cppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap, FloatBuffer s,
FloatBuffer scond, FloatBuffer amax );
public static native int LAPACKE_cppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap, float[] s,
float[] scond, float[] amax );
public static native int LAPACKE_zppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap, DoublePointer s,
DoublePointer scond, DoublePointer amax );
public static native int LAPACKE_zppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap, DoubleBuffer s,
DoubleBuffer scond, DoubleBuffer amax );
public static native int LAPACKE_zppequ_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap, double[] s,
double[] scond, double[] amax );
public static native int LAPACKE_spprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer ap,
@Const FloatPointer afp, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_spprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer ap,
@Const FloatBuffer afp, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_spprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] ap,
@Const float[] afp, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr, float[] work,
int[] iwork );
public static native int LAPACKE_dpprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer ap,
@Const DoublePointer afp, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dpprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer ap,
@Const DoubleBuffer afp, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dpprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] ap,
@Const double[] afp, @Const double[] b,
int ldb, double[] x, int ldx,
double[] ferr, double[] berr, double[] work,
int[] iwork );
public static native int LAPACKE_cpprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer afp,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cpprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer afp,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cpprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] afp,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zpprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer afp,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zpprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer afp,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zpprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] afp,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer ap, FloatPointer b,
int ldb );
public static native int LAPACKE_sppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer ap, FloatBuffer b,
int ldb );
public static native int LAPACKE_sppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] ap, float[] b,
int ldb );
public static native int LAPACKE_dppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer ap, DoublePointer b,
int ldb );
public static native int LAPACKE_dppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer ap, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] ap, double[] b,
int ldb );
public static native int LAPACKE_cppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zppsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, FloatPointer ap,
FloatPointer afp, @Cast("char*") BytePointer equed, FloatPointer s, FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, FloatBuffer ap,
FloatBuffer afp, @Cast("char*") ByteBuffer equed, FloatBuffer s, FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, float[] ap,
float[] afp, @Cast("char*") byte[] equed, float[] s, float[] b,
int ldb, float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
float[] work, int[] iwork );
public static native int LAPACKE_dppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, DoublePointer ap,
DoublePointer afp, @Cast("char*") BytePointer equed, DoublePointer s, DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, DoubleBuffer ap,
DoubleBuffer afp, @Cast("char*") ByteBuffer equed, DoubleBuffer s, DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, double[] ap,
double[] afp, @Cast("char*") byte[] equed, double[] s, double[] b,
int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
double[] work, int[] iwork );
public static native int LAPACKE_cppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer afp, @Cast("char*") BytePointer equed,
FloatPointer s, @Cast("lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_cppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer afp, @Cast("char*") ByteBuffer equed,
FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_cppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] afp, @Cast("char*") byte[] equed,
float[] s, @Cast("lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_zppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer afp, @Cast("char*") BytePointer equed,
DoublePointer s, @Cast("lapack_complex_double*") DoublePointer b,
int ldb, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork );
public static native int LAPACKE_zppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer afp, @Cast("char*") ByteBuffer equed,
DoubleBuffer s, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork );
public static native int LAPACKE_zppsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] afp, @Cast("char*") byte[] equed,
double[] s, @Cast("lapack_complex_double*") double[] b,
int ldb, @Cast("lapack_complex_double*") double[] x,
int ldx, double[] rcond, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork );
public static native int LAPACKE_spptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer ap );
public static native int LAPACKE_spptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer ap );
public static native int LAPACKE_spptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] ap );
public static native int LAPACKE_dpptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap );
public static native int LAPACKE_dpptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap );
public static native int LAPACKE_dpptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap );
public static native int LAPACKE_cpptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_cpptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_cpptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_zpptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_zpptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_zpptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_spptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer ap );
public static native int LAPACKE_spptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer ap );
public static native int LAPACKE_spptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] ap );
public static native int LAPACKE_dpptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap );
public static native int LAPACKE_dpptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap );
public static native int LAPACKE_dpptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap );
public static native int LAPACKE_cpptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_cpptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_cpptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_zpptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_zpptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_zpptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_spptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer ap, FloatPointer b,
int ldb );
public static native int LAPACKE_spptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer ap, FloatBuffer b,
int ldb );
public static native int LAPACKE_spptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] ap, float[] b,
int ldb );
public static native int LAPACKE_dpptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer ap, DoublePointer b,
int ldb );
public static native int LAPACKE_dpptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer ap, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dpptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] ap, double[] b,
int ldb );
public static native int LAPACKE_cpptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zpptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zpptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_spstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda, IntPointer piv,
IntPointer rank, float tol, FloatPointer work );
public static native int LAPACKE_spstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda, IntBuffer piv,
IntBuffer rank, float tol, FloatBuffer work );
public static native int LAPACKE_spstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda, int[] piv,
int[] rank, float tol, float[] work );
public static native int LAPACKE_dpstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda, IntPointer piv,
IntPointer rank, double tol, DoublePointer work );
public static native int LAPACKE_dpstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda, IntBuffer piv,
IntBuffer rank, double tol, DoubleBuffer work );
public static native int LAPACKE_dpstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda, int[] piv,
int[] rank, double tol, double[] work );
public static native int LAPACKE_cpstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer piv, IntPointer rank, float tol,
FloatPointer work );
public static native int LAPACKE_cpstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer piv, IntBuffer rank, float tol,
FloatBuffer work );
public static native int LAPACKE_cpstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] piv, int[] rank, float tol,
float[] work );
public static native int LAPACKE_zpstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer piv, IntPointer rank, double tol,
DoublePointer work );
public static native int LAPACKE_zpstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer piv, IntBuffer rank, double tol,
DoubleBuffer work );
public static native int LAPACKE_zpstrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] piv, int[] rank, double tol,
double[] work );
public static native int LAPACKE_sptcon_work( int n, @Const FloatPointer d, @Const FloatPointer e,
float anorm, FloatPointer rcond, FloatPointer work );
public static native int LAPACKE_sptcon_work( int n, @Const FloatBuffer d, @Const FloatBuffer e,
float anorm, FloatBuffer rcond, FloatBuffer work );
public static native int LAPACKE_sptcon_work( int n, @Const float[] d, @Const float[] e,
float anorm, float[] rcond, float[] work );
public static native int LAPACKE_dptcon_work( int n, @Const DoublePointer d, @Const DoublePointer e,
double anorm, DoublePointer rcond, DoublePointer work );
public static native int LAPACKE_dptcon_work( int n, @Const DoubleBuffer d, @Const DoubleBuffer e,
double anorm, DoubleBuffer rcond, DoubleBuffer work );
public static native int LAPACKE_dptcon_work( int n, @Const double[] d, @Const double[] e,
double anorm, double[] rcond, double[] work );
public static native int LAPACKE_cptcon_work( int n, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, float anorm,
FloatPointer rcond, FloatPointer work );
public static native int LAPACKE_cptcon_work( int n, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, float anorm,
FloatBuffer rcond, FloatBuffer work );
public static native int LAPACKE_cptcon_work( int n, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, float anorm,
float[] rcond, float[] work );
public static native int LAPACKE_zptcon_work( int n, @Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e, double anorm,
DoublePointer rcond, DoublePointer work );
public static native int LAPACKE_zptcon_work( int n, @Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e, double anorm,
DoubleBuffer rcond, DoubleBuffer work );
public static native int LAPACKE_zptcon_work( int n, @Const double[] d,
@Cast("const lapack_complex_double*") double[] e, double anorm,
double[] rcond, double[] work );
public static native int LAPACKE_spteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatPointer d, FloatPointer e, FloatPointer z, int ldz,
FloatPointer work );
public static native int LAPACKE_spteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatBuffer d, FloatBuffer e, FloatBuffer z, int ldz,
FloatBuffer work );
public static native int LAPACKE_spteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
float[] d, float[] e, float[] z, int ldz,
float[] work );
public static native int LAPACKE_dpteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, DoublePointer z, int ldz,
DoublePointer work );
public static native int LAPACKE_dpteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer z, int ldz,
DoubleBuffer work );
public static native int LAPACKE_dpteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, double[] z, int ldz,
double[] work );
public static native int LAPACKE_cpteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatPointer d, FloatPointer e, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, FloatPointer work );
public static native int LAPACKE_cpteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatBuffer d, FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, FloatBuffer work );
public static native int LAPACKE_cpteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
float[] d, float[] e, @Cast("lapack_complex_float*") float[] z,
int ldz, float[] work );
public static native int LAPACKE_zpteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, DoublePointer work );
public static native int LAPACKE_zpteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, DoubleBuffer work );
public static native int LAPACKE_zpteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] z,
int ldz, double[] work );
public static native int LAPACKE_sptrfs_work( int matrix_layout, int n, int nrhs,
@Const FloatPointer d, @Const FloatPointer e, @Const FloatPointer df,
@Const FloatPointer ef, @Const FloatPointer b, int ldb,
FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr, FloatPointer work );
public static native int LAPACKE_sptrfs_work( int matrix_layout, int n, int nrhs,
@Const FloatBuffer d, @Const FloatBuffer e, @Const FloatBuffer df,
@Const FloatBuffer ef, @Const FloatBuffer b, int ldb,
FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr, FloatBuffer work );
public static native int LAPACKE_sptrfs_work( int matrix_layout, int n, int nrhs,
@Const float[] d, @Const float[] e, @Const float[] df,
@Const float[] ef, @Const float[] b, int ldb,
float[] x, int ldx, float[] ferr,
float[] berr, float[] work );
public static native int LAPACKE_dptrfs_work( int matrix_layout, int n, int nrhs,
@Const DoublePointer d, @Const DoublePointer e,
@Const DoublePointer df, @Const DoublePointer ef,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr,
DoublePointer work );
public static native int LAPACKE_dptrfs_work( int matrix_layout, int n, int nrhs,
@Const DoubleBuffer d, @Const DoubleBuffer e,
@Const DoubleBuffer df, @Const DoubleBuffer ef,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work );
public static native int LAPACKE_dptrfs_work( int matrix_layout, int n, int nrhs,
@Const double[] d, @Const double[] e,
@Const double[] df, @Const double[] ef,
@Const double[] b, int ldb, double[] x,
int ldx, double[] ferr, double[] berr,
double[] work );
public static native int LAPACKE_cptrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, @Const FloatPointer df,
@Cast("const lapack_complex_float*") FloatPointer ef,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cptrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, @Const FloatBuffer df,
@Cast("const lapack_complex_float*") FloatBuffer ef,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cptrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, @Const float[] df,
@Cast("const lapack_complex_float*") float[] ef,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zptrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e,
@Const DoublePointer df,
@Cast("const lapack_complex_double*") DoublePointer ef,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zptrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e,
@Const DoubleBuffer df,
@Cast("const lapack_complex_double*") DoubleBuffer ef,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zptrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] d,
@Cast("const lapack_complex_double*") double[] e,
@Const double[] df,
@Cast("const lapack_complex_double*") double[] ef,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sptsv_work( int matrix_layout, int n, int nrhs,
FloatPointer d, FloatPointer e, FloatPointer b, int ldb );
public static native int LAPACKE_sptsv_work( int matrix_layout, int n, int nrhs,
FloatBuffer d, FloatBuffer e, FloatBuffer b, int ldb );
public static native int LAPACKE_sptsv_work( int matrix_layout, int n, int nrhs,
float[] d, float[] e, float[] b, int ldb );
public static native int LAPACKE_dptsv_work( int matrix_layout, int n, int nrhs,
DoublePointer d, DoublePointer e, DoublePointer b,
int ldb );
public static native int LAPACKE_dptsv_work( int matrix_layout, int n, int nrhs,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dptsv_work( int matrix_layout, int n, int nrhs,
double[] d, double[] e, double[] b,
int ldb );
public static native int LAPACKE_cptsv_work( int matrix_layout, int n, int nrhs,
FloatPointer d, @Cast("lapack_complex_float*") FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cptsv_work( int matrix_layout, int n, int nrhs,
FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cptsv_work( int matrix_layout, int n, int nrhs,
float[] d, @Cast("lapack_complex_float*") float[] e,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zptsv_work( int matrix_layout, int n, int nrhs,
DoublePointer d, @Cast("lapack_complex_double*") DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zptsv_work( int matrix_layout, int n, int nrhs,
DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zptsv_work( int matrix_layout, int n, int nrhs,
double[] d, @Cast("lapack_complex_double*") double[] e,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const FloatPointer d, @Const FloatPointer e,
FloatPointer df, FloatPointer ef, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer work );
public static native int LAPACKE_sptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const FloatBuffer d, @Const FloatBuffer e,
FloatBuffer df, FloatBuffer ef, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work );
public static native int LAPACKE_sptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const float[] d, @Const float[] e,
float[] df, float[] ef, @Const float[] b,
int ldb, float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
float[] work );
public static native int LAPACKE_dptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const DoublePointer d,
@Const DoublePointer e, DoublePointer df, DoublePointer ef,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr, DoublePointer work );
public static native int LAPACKE_dptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const DoubleBuffer d,
@Const DoubleBuffer e, DoubleBuffer df, DoubleBuffer ef,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work );
public static native int LAPACKE_dptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const double[] d,
@Const double[] e, double[] df, double[] ef,
@Const double[] b, int ldb, double[] x,
int ldx, double[] rcond, double[] ferr,
double[] berr, double[] work );
public static native int LAPACKE_cptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, FloatPointer df,
@Cast("lapack_complex_float*") FloatPointer ef,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, FloatBuffer df,
@Cast("lapack_complex_float*") FloatBuffer ef,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, float[] df,
@Cast("lapack_complex_float*") float[] ef,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e, DoublePointer df,
@Cast("lapack_complex_double*") DoublePointer ef,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e, DoubleBuffer df,
@Cast("lapack_complex_double*") DoubleBuffer ef,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zptsvx_work( int matrix_layout, @Cast("char") byte fact, int n,
int nrhs, @Const double[] d,
@Cast("const lapack_complex_double*") double[] e, double[] df,
@Cast("lapack_complex_double*") double[] ef,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_spttrf_work( int n, FloatPointer d, FloatPointer e );
public static native int LAPACKE_spttrf_work( int n, FloatBuffer d, FloatBuffer e );
public static native int LAPACKE_spttrf_work( int n, float[] d, float[] e );
public static native int LAPACKE_dpttrf_work( int n, DoublePointer d, DoublePointer e );
public static native int LAPACKE_dpttrf_work( int n, DoubleBuffer d, DoubleBuffer e );
public static native int LAPACKE_dpttrf_work( int n, double[] d, double[] e );
public static native int LAPACKE_cpttrf_work( int n, FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer e );
public static native int LAPACKE_cpttrf_work( int n, FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer e );
public static native int LAPACKE_cpttrf_work( int n, float[] d,
@Cast("lapack_complex_float*") float[] e );
public static native int LAPACKE_zpttrf_work( int n, DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer e );
public static native int LAPACKE_zpttrf_work( int n, DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer e );
public static native int LAPACKE_zpttrf_work( int n, double[] d,
@Cast("lapack_complex_double*") double[] e );
public static native int LAPACKE_spttrs_work( int matrix_layout, int n, int nrhs,
@Const FloatPointer d, @Const FloatPointer e, FloatPointer b,
int ldb );
public static native int LAPACKE_spttrs_work( int matrix_layout, int n, int nrhs,
@Const FloatBuffer d, @Const FloatBuffer e, FloatBuffer b,
int ldb );
public static native int LAPACKE_spttrs_work( int matrix_layout, int n, int nrhs,
@Const float[] d, @Const float[] e, float[] b,
int ldb );
public static native int LAPACKE_dpttrs_work( int matrix_layout, int n, int nrhs,
@Const DoublePointer d, @Const DoublePointer e, DoublePointer b,
int ldb );
public static native int LAPACKE_dpttrs_work( int matrix_layout, int n, int nrhs,
@Const DoubleBuffer d, @Const DoubleBuffer e, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dpttrs_work( int matrix_layout, int n, int nrhs,
@Const double[] d, @Const double[] e, double[] b,
int ldb );
public static native int LAPACKE_cpttrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_cpttrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_cpttrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zpttrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zpttrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zpttrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] d,
@Cast("const lapack_complex_double*") double[] e,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_ssbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, FloatPointer ab,
int ldab, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work );
public static native int LAPACKE_ssbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, FloatBuffer ab,
int ldab, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work );
public static native int LAPACKE_ssbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, float[] ab,
int ldab, float[] w, float[] z,
int ldz, float[] work );
public static native int LAPACKE_dsbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, DoublePointer ab,
int ldab, DoublePointer w, DoublePointer z,
int ldz, DoublePointer work );
public static native int LAPACKE_dsbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, DoubleBuffer ab,
int ldab, DoubleBuffer w, DoubleBuffer z,
int ldz, DoubleBuffer work );
public static native int LAPACKE_dsbev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, double[] ab,
int ldab, double[] w, double[] z,
int ldz, double[] work );
public static native int LAPACKE_ssbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, FloatPointer ab,
int ldab, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_ssbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, FloatBuffer ab,
int ldab, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_ssbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, float[] ab,
int ldab, float[] w, float[] z,
int ldz, float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_dsbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, DoublePointer ab,
int ldab, DoublePointer w, DoublePointer z,
int ldz, DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dsbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, DoubleBuffer ab,
int ldab, DoubleBuffer w, DoubleBuffer z,
int ldz, DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dsbevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int kd, double[] ab,
int ldab, double[] w, double[] z,
int ldz, double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_ssbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
FloatPointer ab, int ldab, FloatPointer q,
int ldq, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_ssbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
FloatBuffer ab, int ldab, FloatBuffer q,
int ldq, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_ssbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
float[] ab, int ldab, float[] q,
int ldq, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w, float[] z,
int ldz, float[] work, int[] iwork,
int[] ifail );
public static native int LAPACKE_dsbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
DoublePointer ab, int ldab, DoublePointer q,
int ldq, double vl, double vu,
int il, int iu, double abstol,
IntPointer m, DoublePointer w, DoublePointer z,
int ldz, DoublePointer work, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_dsbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
DoubleBuffer ab, int ldab, DoubleBuffer q,
int ldq, double vl, double vu,
int il, int iu, double abstol,
IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, DoubleBuffer work, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_dsbevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int kd,
double[] ab, int ldab, double[] q,
int ldq, double vl, double vu,
int il, int iu, double abstol,
int[] m, double[] w, double[] z,
int ldz, double[] work, int[] iwork,
int[] ifail );
public static native int LAPACKE_ssbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
FloatPointer ab, int ldab, @Const FloatPointer bb,
int ldbb, FloatPointer x, int ldx,
FloatPointer work );
public static native int LAPACKE_ssbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
FloatBuffer ab, int ldab, @Const FloatBuffer bb,
int ldbb, FloatBuffer x, int ldx,
FloatBuffer work );
public static native int LAPACKE_ssbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
float[] ab, int ldab, @Const float[] bb,
int ldbb, float[] x, int ldx,
float[] work );
public static native int LAPACKE_dsbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
DoublePointer ab, int ldab, @Const DoublePointer bb,
int ldbb, DoublePointer x, int ldx,
DoublePointer work );
public static native int LAPACKE_dsbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
DoubleBuffer ab, int ldab, @Const DoubleBuffer bb,
int ldbb, DoubleBuffer x, int ldx,
DoubleBuffer work );
public static native int LAPACKE_dsbgst_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int ka, int kb,
double[] ab, int ldab, @Const double[] bb,
int ldbb, double[] x, int ldx,
double[] work );
public static native int LAPACKE_ssbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
FloatPointer ab, int ldab, FloatPointer bb,
int ldbb, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work );
public static native int LAPACKE_ssbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
FloatBuffer ab, int ldab, FloatBuffer bb,
int ldbb, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work );
public static native int LAPACKE_ssbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
float[] ab, int ldab, float[] bb,
int ldbb, float[] w, float[] z,
int ldz, float[] work );
public static native int LAPACKE_dsbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
DoublePointer ab, int ldab, DoublePointer bb,
int ldbb, DoublePointer w, DoublePointer z,
int ldz, DoublePointer work );
public static native int LAPACKE_dsbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
DoubleBuffer ab, int ldab, DoubleBuffer bb,
int ldbb, DoubleBuffer w, DoubleBuffer z,
int ldz, DoubleBuffer work );
public static native int LAPACKE_dsbgv_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
double[] ab, int ldab, double[] bb,
int ldbb, double[] w, double[] z,
int ldz, double[] work );
public static native int LAPACKE_ssbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
FloatPointer ab, int ldab, FloatPointer bb,
int ldbb, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_ssbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
FloatBuffer ab, int ldab, FloatBuffer bb,
int ldbb, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_ssbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
float[] ab, int ldab, float[] bb,
int ldbb, float[] w, float[] z,
int ldz, float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_dsbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
DoublePointer ab, int ldab, DoublePointer bb,
int ldbb, DoublePointer w, DoublePointer z,
int ldz, DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dsbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
DoubleBuffer ab, int ldab, DoubleBuffer bb,
int ldbb, DoubleBuffer w, DoubleBuffer z,
int ldz, DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dsbgvd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, int ka, int kb,
double[] ab, int ldab, double[] bb,
int ldbb, double[] w, double[] z,
int ldz, double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_ssbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, FloatPointer ab, int ldab,
FloatPointer bb, int ldbb, FloatPointer q,
int ldq, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_ssbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, FloatBuffer ab, int ldab,
FloatBuffer bb, int ldbb, FloatBuffer q,
int ldq, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_ssbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, float[] ab, int ldab,
float[] bb, int ldbb, float[] q,
int ldq, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w, float[] z,
int ldz, float[] work, int[] iwork,
int[] ifail );
public static native int LAPACKE_dsbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, DoublePointer ab, int ldab,
DoublePointer bb, int ldbb, DoublePointer q,
int ldq, double vl, double vu,
int il, int iu, double abstol,
IntPointer m, DoublePointer w, DoublePointer z,
int ldz, DoublePointer work, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_dsbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, DoubleBuffer ab, int ldab,
DoubleBuffer bb, int ldbb, DoubleBuffer q,
int ldq, double vl, double vu,
int il, int iu, double abstol,
IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, DoubleBuffer work, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_dsbgvx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, int ka,
int kb, double[] ab, int ldab,
double[] bb, int ldbb, double[] q,
int ldq, double vl, double vu,
int il, int iu, double abstol,
int[] m, double[] w, double[] z,
int ldz, double[] work, int[] iwork,
int[] ifail );
public static native int LAPACKE_ssbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd, FloatPointer ab,
int ldab, FloatPointer d, FloatPointer e, FloatPointer q,
int ldq, FloatPointer work );
public static native int LAPACKE_ssbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd, FloatBuffer ab,
int ldab, FloatBuffer d, FloatBuffer e, FloatBuffer q,
int ldq, FloatBuffer work );
public static native int LAPACKE_ssbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd, float[] ab,
int ldab, float[] d, float[] e, float[] q,
int ldq, float[] work );
public static native int LAPACKE_dsbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd, DoublePointer ab,
int ldab, DoublePointer d, DoublePointer e,
DoublePointer q, int ldq, DoublePointer work );
public static native int LAPACKE_dsbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd, DoubleBuffer ab,
int ldab, DoubleBuffer d, DoubleBuffer e,
DoubleBuffer q, int ldq, DoubleBuffer work );
public static native int LAPACKE_dsbtrd_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte uplo,
int n, int kd, double[] ab,
int ldab, double[] d, double[] e,
double[] q, int ldq, double[] work );
public static native int LAPACKE_ssfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
float alpha, @Const FloatPointer a, int lda,
float beta, FloatPointer c );
public static native int LAPACKE_ssfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
float alpha, @Const FloatBuffer a, int lda,
float beta, FloatBuffer c );
public static native int LAPACKE_ssfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
float alpha, @Const float[] a, int lda,
float beta, float[] c );
public static native int LAPACKE_dsfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
double alpha, @Const DoublePointer a, int lda,
double beta, DoublePointer c );
public static native int LAPACKE_dsfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
double alpha, @Const DoubleBuffer a, int lda,
double beta, DoubleBuffer c );
public static native int LAPACKE_dsfrk_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte trans, int n, int k,
double alpha, @Const double[] a, int lda,
double beta, double[] c );
public static native int LAPACKE_sspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, @Const IntPointer ipiv,
float anorm, FloatPointer rcond, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_sspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, @Const IntBuffer ipiv,
float anorm, FloatBuffer rcond, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_sspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, @Const int[] ipiv,
float anorm, float[] rcond, float[] work,
int[] iwork );
public static native int LAPACKE_dspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, @Const IntPointer ipiv,
double anorm, DoublePointer rcond, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, @Const IntBuffer ipiv,
double anorm, DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, @Const int[] ipiv,
double anorm, double[] rcond, double[] work,
int[] iwork );
public static native int LAPACKE_cspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, float anorm,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_cspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, float anorm,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_cspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, float anorm,
float[] rcond, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zspcon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv, double anorm,
double[] rcond, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_sspev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, FloatPointer ap, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work );
public static native int LAPACKE_sspev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, FloatBuffer ap, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work );
public static native int LAPACKE_sspev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, float[] ap, float[] w, float[] z,
int ldz, float[] work );
public static native int LAPACKE_dspev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, DoublePointer ap, DoublePointer w, DoublePointer z,
int ldz, DoublePointer work );
public static native int LAPACKE_dspev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, DoubleBuffer ap, DoubleBuffer w, DoubleBuffer z,
int ldz, DoubleBuffer work );
public static native int LAPACKE_dspev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, double[] ap, double[] w, double[] z,
int ldz, double[] work );
public static native int LAPACKE_sspevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, FloatPointer ap, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_sspevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, FloatBuffer ap, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_sspevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, float[] ap, float[] w, float[] z,
int ldz, float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_dspevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, DoublePointer ap, DoublePointer w, DoublePointer z,
int ldz, DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dspevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, DoubleBuffer ap, DoubleBuffer w, DoubleBuffer z,
int ldz, DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dspevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, double[] ap, double[] w, double[] z,
int ldz, double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_sspevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, FloatPointer ap, float vl,
float vu, int il, int iu,
float abstol, IntPointer m, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_sspevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, FloatBuffer ap, float vl,
float vu, int il, int iu,
float abstol, IntBuffer m, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_sspevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, float[] ap, float vl,
float vu, int il, int iu,
float abstol, int[] m, float[] w, float[] z,
int ldz, float[] work, int[] iwork,
int[] ifail );
public static native int LAPACKE_dspevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, DoublePointer ap, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
DoublePointer z, int ldz, DoublePointer work,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_dspevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, DoubleBuffer ap, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, int ldz, DoubleBuffer work,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_dspevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, double[] ap, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
double[] z, int ldz, double[] work,
int[] iwork, int[] ifail );
public static native int LAPACKE_sspgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, FloatPointer ap, @Const FloatPointer bp );
public static native int LAPACKE_sspgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, FloatBuffer ap, @Const FloatBuffer bp );
public static native int LAPACKE_sspgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, float[] ap, @Const float[] bp );
public static native int LAPACKE_dspgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, DoublePointer ap, @Const DoublePointer bp );
public static native int LAPACKE_dspgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, DoubleBuffer ap, @Const DoubleBuffer bp );
public static native int LAPACKE_dspgst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, double[] ap, @Const double[] bp );
public static native int LAPACKE_sspgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatPointer ap, FloatPointer bp,
FloatPointer w, FloatPointer z, int ldz,
FloatPointer work );
public static native int LAPACKE_sspgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatBuffer ap, FloatBuffer bp,
FloatBuffer w, FloatBuffer z, int ldz,
FloatBuffer work );
public static native int LAPACKE_sspgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, float[] ap, float[] bp,
float[] w, float[] z, int ldz,
float[] work );
public static native int LAPACKE_dspgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoublePointer ap, DoublePointer bp,
DoublePointer w, DoublePointer z, int ldz,
DoublePointer work );
public static native int LAPACKE_dspgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoubleBuffer ap, DoubleBuffer bp,
DoubleBuffer w, DoubleBuffer z, int ldz,
DoubleBuffer work );
public static native int LAPACKE_dspgv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, double[] ap, double[] bp,
double[] w, double[] z, int ldz,
double[] work );
public static native int LAPACKE_sspgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatPointer ap, FloatPointer bp,
FloatPointer w, FloatPointer z, int ldz, FloatPointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_sspgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatBuffer ap, FloatBuffer bp,
FloatBuffer w, FloatBuffer z, int ldz, FloatBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_sspgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, float[] ap, float[] bp,
float[] w, float[] z, int ldz, float[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_dspgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoublePointer ap, DoublePointer bp,
DoublePointer w, DoublePointer z, int ldz,
DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dspgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoubleBuffer ap, DoubleBuffer bp,
DoubleBuffer w, DoubleBuffer z, int ldz,
DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dspgvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, double[] ap, double[] bp,
double[] w, double[] z, int ldz,
double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_sspgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, FloatPointer ap,
FloatPointer bp, float vl, float vu, int il,
int iu, float abstol, IntPointer m,
FloatPointer w, FloatPointer z, int ldz, FloatPointer work,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_sspgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, FloatBuffer ap,
FloatBuffer bp, float vl, float vu, int il,
int iu, float abstol, IntBuffer m,
FloatBuffer w, FloatBuffer z, int ldz, FloatBuffer work,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_sspgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, float[] ap,
float[] bp, float vl, float vu, int il,
int iu, float abstol, int[] m,
float[] w, float[] z, int ldz, float[] work,
int[] iwork, int[] ifail );
public static native int LAPACKE_dspgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, DoublePointer ap,
DoublePointer bp, double vl, double vu, int il,
int iu, double abstol, IntPointer m,
DoublePointer w, DoublePointer z, int ldz,
DoublePointer work, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_dspgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, DoubleBuffer ap,
DoubleBuffer bp, double vl, double vu, int il,
int iu, double abstol, IntBuffer m,
DoubleBuffer w, DoubleBuffer z, int ldz,
DoubleBuffer work, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_dspgvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, double[] ap,
double[] bp, double vl, double vu, int il,
int iu, double abstol, int[] m,
double[] w, double[] z, int ldz,
double[] work, int[] iwork,
int[] ifail );
public static native int LAPACKE_ssprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer ap,
@Const FloatPointer afp, @Const IntPointer ipiv,
@Const FloatPointer b, int ldb, FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_ssprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer ap,
@Const FloatBuffer afp, @Const IntBuffer ipiv,
@Const FloatBuffer b, int ldb, FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_ssprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] ap,
@Const float[] afp, @Const int[] ipiv,
@Const float[] b, int ldb, float[] x,
int ldx, float[] ferr, float[] berr,
float[] work, int[] iwork );
public static native int LAPACKE_dsprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer ap,
@Const DoublePointer afp, @Const IntPointer ipiv,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dsprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer ap,
@Const DoubleBuffer afp, @Const IntBuffer ipiv,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dsprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] ap,
@Const double[] afp, @Const int[] ipiv,
@Const double[] b, int ldb, double[] x,
int ldx, double[] ferr, double[] berr,
double[] work, int[] iwork );
public static native int LAPACKE_csprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer afp,
@Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_csprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer afp,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_csprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] afp,
@Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zsprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer afp,
@Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zsprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer afp,
@Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zsprfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] afp,
@Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_sspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer ap, IntPointer ipiv,
FloatPointer b, int ldb );
public static native int LAPACKE_sspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer ap, IntBuffer ipiv,
FloatBuffer b, int ldb );
public static native int LAPACKE_sspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] ap, int[] ipiv,
float[] b, int ldb );
public static native int LAPACKE_dspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer ap, IntPointer ipiv,
DoublePointer b, int ldb );
public static native int LAPACKE_dspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer ap, IntBuffer ipiv,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] ap, int[] ipiv,
double[] b, int ldb );
public static native int LAPACKE_cspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_cspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_cspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] ap,
int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_zspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_zspsv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] ap,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_sspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const FloatPointer ap,
FloatPointer afp, IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const FloatBuffer ap,
FloatBuffer afp, IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const float[] ap,
float[] afp, int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
float[] work, int[] iwork );
public static native int LAPACKE_dspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const DoublePointer ap,
DoublePointer afp, IntPointer ipiv, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const DoubleBuffer ap,
DoubleBuffer afp, IntBuffer ipiv, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const double[] ap,
double[] afp, int[] ipiv, @Const double[] b,
int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
double[] work, int[] iwork );
public static native int LAPACKE_cspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer afp, IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_cspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer afp, IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_cspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] afp, int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer afp, IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer afp, IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zspsvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] afp, int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_ssptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer ap, FloatPointer d, FloatPointer e, FloatPointer tau );
public static native int LAPACKE_ssptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer ap, FloatBuffer d, FloatBuffer e, FloatBuffer tau );
public static native int LAPACKE_ssptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] ap, float[] d, float[] e, float[] tau );
public static native int LAPACKE_dsptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap, DoublePointer d, DoublePointer e, DoublePointer tau );
public static native int LAPACKE_dsptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap, DoubleBuffer d, DoubleBuffer e, DoubleBuffer tau );
public static native int LAPACKE_dsptrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap, double[] d, double[] e, double[] tau );
public static native int LAPACKE_ssptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer ap, IntPointer ipiv );
public static native int LAPACKE_ssptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_ssptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] ap, int[] ipiv );
public static native int LAPACKE_dsptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap, IntPointer ipiv );
public static native int LAPACKE_dsptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_dsptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap, int[] ipiv );
public static native int LAPACKE_csptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap, IntPointer ipiv );
public static native int LAPACKE_csptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_csptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap, int[] ipiv );
public static native int LAPACKE_zsptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap, IntPointer ipiv );
public static native int LAPACKE_zsptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap, IntBuffer ipiv );
public static native int LAPACKE_zsptrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap, int[] ipiv );
public static native int LAPACKE_ssptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer ap, @Const IntPointer ipiv,
FloatPointer work );
public static native int LAPACKE_ssptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer ap, @Const IntBuffer ipiv,
FloatBuffer work );
public static native int LAPACKE_ssptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] ap, @Const int[] ipiv,
float[] work );
public static native int LAPACKE_dsptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer ap, @Const IntPointer ipiv,
DoublePointer work );
public static native int LAPACKE_dsptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer ap, @Const IntBuffer ipiv,
DoubleBuffer work );
public static native int LAPACKE_dsptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] ap, @Const int[] ipiv,
double[] work );
public static native int LAPACKE_csptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_csptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_csptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] ap,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zsptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zsptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zsptri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] ap,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_ssptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer ap,
@Const IntPointer ipiv, FloatPointer b,
int ldb );
public static native int LAPACKE_ssptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer ap,
@Const IntBuffer ipiv, FloatBuffer b,
int ldb );
public static native int LAPACKE_ssptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] ap,
@Const int[] ipiv, float[] b,
int ldb );
public static native int LAPACKE_dsptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer ap,
@Const IntPointer ipiv, DoublePointer b,
int ldb );
public static native int LAPACKE_dsptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer ap,
@Const IntBuffer ipiv, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dsptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] ap,
@Const int[] ipiv, double[] b,
int ldb );
public static native int LAPACKE_csptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_csptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_csptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_zsptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zsptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zsptrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sstebz_work( @Cast("char") byte range, @Cast("char") byte order, int n, float vl,
float vu, int il, int iu,
float abstol, @Const FloatPointer d, @Const FloatPointer e,
IntPointer m, IntPointer nsplit, FloatPointer w,
IntPointer iblock, IntPointer isplit,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_sstebz_work( @Cast("char") byte range, @Cast("char") byte order, int n, float vl,
float vu, int il, int iu,
float abstol, @Const FloatBuffer d, @Const FloatBuffer e,
IntBuffer m, IntBuffer nsplit, FloatBuffer w,
IntBuffer iblock, IntBuffer isplit,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_sstebz_work( @Cast("char") byte range, @Cast("char") byte order, int n, float vl,
float vu, int il, int iu,
float abstol, @Const float[] d, @Const float[] e,
int[] m, int[] nsplit, float[] w,
int[] iblock, int[] isplit,
float[] work, int[] iwork );
public static native int LAPACKE_dstebz_work( @Cast("char") byte range, @Cast("char") byte order, int n, double vl,
double vu, int il, int iu,
double abstol, @Const DoublePointer d, @Const DoublePointer e,
IntPointer m, IntPointer nsplit, DoublePointer w,
IntPointer iblock, IntPointer isplit,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dstebz_work( @Cast("char") byte range, @Cast("char") byte order, int n, double vl,
double vu, int il, int iu,
double abstol, @Const DoubleBuffer d, @Const DoubleBuffer e,
IntBuffer m, IntBuffer nsplit, DoubleBuffer w,
IntBuffer iblock, IntBuffer isplit,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dstebz_work( @Cast("char") byte range, @Cast("char") byte order, int n, double vl,
double vu, int il, int iu,
double abstol, @Const double[] d, @Const double[] e,
int[] m, int[] nsplit, double[] w,
int[] iblock, int[] isplit,
double[] work, int[] iwork );
public static native int LAPACKE_sstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatPointer d, FloatPointer e, FloatPointer z, int ldz,
FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_sstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatBuffer d, FloatBuffer e, FloatBuffer z, int ldz,
FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_sstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
float[] d, float[] e, float[] z, int ldz,
float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_dstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, DoublePointer z, int ldz,
DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer z, int ldz,
DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, double[] z, int ldz,
double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_cstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatPointer d, FloatPointer e, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_cstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatBuffer d, FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_cstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
float[] d, float[] e, @Cast("lapack_complex_float*") float[] z,
int ldz, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_zstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork,
int lrwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_zstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork,
int lrwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_zstedc_work( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] z,
int ldz, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork,
int lrwork, int[] iwork,
int liwork );
public static native int LAPACKE_sstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl,
float vu, int il, int iu,
float abstol, IntPointer m, FloatPointer w, FloatPointer z,
int ldz, IntPointer isuppz, FloatPointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_sstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl,
float vu, int il, int iu,
float abstol, IntBuffer m, FloatBuffer w, FloatBuffer z,
int ldz, IntBuffer isuppz, FloatBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_sstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl,
float vu, int il, int iu,
float abstol, int[] m, float[] w, float[] z,
int ldz, int[] isuppz, float[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_dstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
DoublePointer z, int ldz, IntPointer isuppz,
DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, int ldz, IntBuffer isuppz,
DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
double[] z, int ldz, int[] isuppz,
double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_cstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl,
float vu, int il, int iu,
float abstol, IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
IntPointer isuppz, FloatPointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_cstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl,
float vu, int il, int iu,
float abstol, IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
IntBuffer isuppz, FloatBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_cstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl,
float vu, int il, int iu,
float abstol, int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
int[] isuppz, float[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_zstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer isuppz, DoublePointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_zstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer isuppz, DoubleBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_zstegr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] isuppz, double[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_sstein_work( int matrix_layout, int n, @Const FloatPointer d,
@Const FloatPointer e, int m, @Const FloatPointer w,
@Const IntPointer iblock,
@Const IntPointer isplit, FloatPointer z,
int ldz, FloatPointer work, IntPointer iwork,
IntPointer ifailv );
public static native int LAPACKE_sstein_work( int matrix_layout, int n, @Const FloatBuffer d,
@Const FloatBuffer e, int m, @Const FloatBuffer w,
@Const IntBuffer iblock,
@Const IntBuffer isplit, FloatBuffer z,
int ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifailv );
public static native int LAPACKE_sstein_work( int matrix_layout, int n, @Const float[] d,
@Const float[] e, int m, @Const float[] w,
@Const int[] iblock,
@Const int[] isplit, float[] z,
int ldz, float[] work, int[] iwork,
int[] ifailv );
public static native int LAPACKE_dstein_work( int matrix_layout, int n, @Const DoublePointer d,
@Const DoublePointer e, int m, @Const DoublePointer w,
@Const IntPointer iblock,
@Const IntPointer isplit, DoublePointer z,
int ldz, DoublePointer work, IntPointer iwork,
IntPointer ifailv );
public static native int LAPACKE_dstein_work( int matrix_layout, int n, @Const DoubleBuffer d,
@Const DoubleBuffer e, int m, @Const DoubleBuffer w,
@Const IntBuffer iblock,
@Const IntBuffer isplit, DoubleBuffer z,
int ldz, DoubleBuffer work, IntBuffer iwork,
IntBuffer ifailv );
public static native int LAPACKE_dstein_work( int matrix_layout, int n, @Const double[] d,
@Const double[] e, int m, @Const double[] w,
@Const int[] iblock,
@Const int[] isplit, double[] z,
int ldz, double[] work, int[] iwork,
int[] ifailv );
public static native int LAPACKE_cstein_work( int matrix_layout, int n, @Const FloatPointer d,
@Const FloatPointer e, int m, @Const FloatPointer w,
@Const IntPointer iblock,
@Const IntPointer isplit,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
FloatPointer work, IntPointer iwork,
IntPointer ifailv );
public static native int LAPACKE_cstein_work( int matrix_layout, int n, @Const FloatBuffer d,
@Const FloatBuffer e, int m, @Const FloatBuffer w,
@Const IntBuffer iblock,
@Const IntBuffer isplit,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
FloatBuffer work, IntBuffer iwork,
IntBuffer ifailv );
public static native int LAPACKE_cstein_work( int matrix_layout, int n, @Const float[] d,
@Const float[] e, int m, @Const float[] w,
@Const int[] iblock,
@Const int[] isplit,
@Cast("lapack_complex_float*") float[] z, int ldz,
float[] work, int[] iwork,
int[] ifailv );
public static native int LAPACKE_zstein_work( int matrix_layout, int n, @Const DoublePointer d,
@Const DoublePointer e, int m, @Const DoublePointer w,
@Const IntPointer iblock,
@Const IntPointer isplit,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
DoublePointer work, IntPointer iwork,
IntPointer ifailv );
public static native int LAPACKE_zstein_work( int matrix_layout, int n, @Const DoubleBuffer d,
@Const DoubleBuffer e, int m, @Const DoubleBuffer w,
@Const IntBuffer iblock,
@Const IntBuffer isplit,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
DoubleBuffer work, IntBuffer iwork,
IntBuffer ifailv );
public static native int LAPACKE_zstein_work( int matrix_layout, int n, @Const double[] d,
@Const double[] e, int m, @Const double[] w,
@Const int[] iblock,
@Const int[] isplit,
@Cast("lapack_complex_double*") double[] z, int ldz,
double[] work, int[] iwork,
int[] ifailv );
public static native int LAPACKE_sstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl,
float vu, int il, int iu,
IntPointer m, FloatPointer w, FloatPointer z,
int ldz, int nzc,
IntPointer isuppz, IntPointer tryrac,
FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_sstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl,
float vu, int il, int iu,
IntBuffer m, FloatBuffer w, FloatBuffer z,
int ldz, int nzc,
IntBuffer isuppz, IntBuffer tryrac,
FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_sstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl,
float vu, int il, int iu,
int[] m, float[] w, float[] z,
int ldz, int nzc,
int[] isuppz, int[] tryrac,
float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_dstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
IntPointer m, DoublePointer w, DoublePointer z,
int ldz, int nzc,
IntPointer isuppz, IntPointer tryrac,
DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, int nzc,
IntBuffer isuppz, IntBuffer tryrac,
DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
int[] m, double[] w, double[] z,
int ldz, int nzc,
int[] isuppz, int[] tryrac,
double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_cstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl,
float vu, int il, int iu,
IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
int nzc, IntPointer isuppz,
IntPointer tryrac, FloatPointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_cstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl,
float vu, int il, int iu,
IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
int nzc, IntBuffer isuppz,
IntBuffer tryrac, FloatBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_cstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl,
float vu, int il, int iu,
int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int ldz,
int nzc, int[] isuppz,
int[] tryrac, float[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_zstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
int nzc, IntPointer isuppz,
IntPointer tryrac, DoublePointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_zstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
int nzc, IntBuffer isuppz,
IntBuffer tryrac, DoubleBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_zstemr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int ldz,
int nzc, int[] isuppz,
int[] tryrac, double[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_ssteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatPointer d, FloatPointer e, FloatPointer z, int ldz,
FloatPointer work );
public static native int LAPACKE_ssteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatBuffer d, FloatBuffer e, FloatBuffer z, int ldz,
FloatBuffer work );
public static native int LAPACKE_ssteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
float[] d, float[] e, float[] z, int ldz,
float[] work );
public static native int LAPACKE_dsteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, DoublePointer z, int ldz,
DoublePointer work );
public static native int LAPACKE_dsteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer z, int ldz,
DoubleBuffer work );
public static native int LAPACKE_dsteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, double[] z, int ldz,
double[] work );
public static native int LAPACKE_csteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatPointer d, FloatPointer e, @Cast("lapack_complex_float*") FloatPointer z,
int ldz, FloatPointer work );
public static native int LAPACKE_csteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
FloatBuffer d, FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer z,
int ldz, FloatBuffer work );
public static native int LAPACKE_csteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
float[] d, float[] e, @Cast("lapack_complex_float*") float[] z,
int ldz, float[] work );
public static native int LAPACKE_zsteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer z,
int ldz, DoublePointer work );
public static native int LAPACKE_zsteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer z,
int ldz, DoubleBuffer work );
public static native int LAPACKE_zsteqr_work( int matrix_layout, @Cast("char") byte compz, int n,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] z,
int ldz, double[] work );
public static native int LAPACKE_ssterf_work( int n, FloatPointer d, FloatPointer e );
public static native int LAPACKE_ssterf_work( int n, FloatBuffer d, FloatBuffer e );
public static native int LAPACKE_ssterf_work( int n, float[] d, float[] e );
public static native int LAPACKE_dsterf_work( int n, DoublePointer d, DoublePointer e );
public static native int LAPACKE_dsterf_work( int n, DoubleBuffer d, DoubleBuffer e );
public static native int LAPACKE_dsterf_work( int n, double[] d, double[] e );
public static native int LAPACKE_sstev_work( int matrix_layout, @Cast("char") byte jobz, int n,
FloatPointer d, FloatPointer e, FloatPointer z, int ldz,
FloatPointer work );
public static native int LAPACKE_sstev_work( int matrix_layout, @Cast("char") byte jobz, int n,
FloatBuffer d, FloatBuffer e, FloatBuffer z, int ldz,
FloatBuffer work );
public static native int LAPACKE_sstev_work( int matrix_layout, @Cast("char") byte jobz, int n,
float[] d, float[] e, float[] z, int ldz,
float[] work );
public static native int LAPACKE_dstev_work( int matrix_layout, @Cast("char") byte jobz, int n,
DoublePointer d, DoublePointer e, DoublePointer z, int ldz,
DoublePointer work );
public static native int LAPACKE_dstev_work( int matrix_layout, @Cast("char") byte jobz, int n,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer z, int ldz,
DoubleBuffer work );
public static native int LAPACKE_dstev_work( int matrix_layout, @Cast("char") byte jobz, int n,
double[] d, double[] e, double[] z, int ldz,
double[] work );
public static native int LAPACKE_sstevd_work( int matrix_layout, @Cast("char") byte jobz, int n,
FloatPointer d, FloatPointer e, FloatPointer z, int ldz,
FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_sstevd_work( int matrix_layout, @Cast("char") byte jobz, int n,
FloatBuffer d, FloatBuffer e, FloatBuffer z, int ldz,
FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_sstevd_work( int matrix_layout, @Cast("char") byte jobz, int n,
float[] d, float[] e, float[] z, int ldz,
float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_dstevd_work( int matrix_layout, @Cast("char") byte jobz, int n,
DoublePointer d, DoublePointer e, DoublePointer z, int ldz,
DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dstevd_work( int matrix_layout, @Cast("char") byte jobz, int n,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer z, int ldz,
DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dstevd_work( int matrix_layout, @Cast("char") byte jobz, int n,
double[] d, double[] e, double[] z, int ldz,
double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_sstevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl,
float vu, int il, int iu,
float abstol, IntPointer m, FloatPointer w, FloatPointer z,
int ldz, IntPointer isuppz, FloatPointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_sstevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl,
float vu, int il, int iu,
float abstol, IntBuffer m, FloatBuffer w, FloatBuffer z,
int ldz, IntBuffer isuppz, FloatBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_sstevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl,
float vu, int il, int iu,
float abstol, int[] m, float[] w, float[] z,
int ldz, int[] isuppz, float[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_dstevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
DoublePointer z, int ldz, IntPointer isuppz,
DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dstevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, int ldz, IntBuffer isuppz,
DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dstevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
double[] z, int ldz, int[] isuppz,
double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_sstevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatPointer d, FloatPointer e, float vl,
float vu, int il, int iu,
float abstol, IntPointer m, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_sstevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, FloatBuffer d, FloatBuffer e, float vl,
float vu, int il, int iu,
float abstol, IntBuffer m, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_sstevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, float[] d, float[] e, float vl,
float vu, int il, int iu,
float abstol, int[] m, float[] w, float[] z,
int ldz, float[] work, int[] iwork,
int[] ifail );
public static native int LAPACKE_dstevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoublePointer d, DoublePointer e, double vl,
double vu, int il, int iu,
double abstol, IntPointer m, DoublePointer w,
DoublePointer z, int ldz, DoublePointer work,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_dstevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, DoubleBuffer d, DoubleBuffer e, double vl,
double vu, int il, int iu,
double abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, int ldz, DoubleBuffer work,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_dstevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
int n, double[] d, double[] e, double vl,
double vu, int il, int iu,
double abstol, int[] m, double[] w,
double[] z, int ldz, double[] work,
int[] iwork, int[] ifail );
public static native int LAPACKE_ssycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer a, int lda,
@Const IntPointer ipiv, float anorm,
FloatPointer rcond, FloatPointer work, IntPointer iwork );
public static native int LAPACKE_ssycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer a, int lda,
@Const IntBuffer ipiv, float anorm,
FloatBuffer rcond, FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_ssycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] a, int lda,
@Const int[] ipiv, float anorm,
float[] rcond, float[] work, int[] iwork );
public static native int LAPACKE_dsycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer a, int lda,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dsycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer a, int lda,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dsycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] a, int lda,
@Const int[] ipiv, double anorm,
double[] rcond, double[] work,
int[] iwork );
public static native int LAPACKE_csycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv, float anorm,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_csycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv, float anorm,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_csycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv, float anorm,
float[] rcond, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zsycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv, double anorm,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zsycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv, double anorm,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zsycon_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv, double anorm,
double[] rcond, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_ssyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer a, int lda, FloatPointer s,
FloatPointer scond, FloatPointer amax, FloatPointer work );
public static native int LAPACKE_ssyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer a, int lda, FloatBuffer s,
FloatBuffer scond, FloatBuffer amax, FloatBuffer work );
public static native int LAPACKE_ssyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] a, int lda, float[] s,
float[] scond, float[] amax, float[] work );
public static native int LAPACKE_dsyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer a, int lda, DoublePointer s,
DoublePointer scond, DoublePointer amax, DoublePointer work );
public static native int LAPACKE_dsyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer s,
DoubleBuffer scond, DoubleBuffer amax, DoubleBuffer work );
public static native int LAPACKE_dsyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] a, int lda, double[] s,
double[] scond, double[] amax, double[] work );
public static native int LAPACKE_csyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer s, FloatPointer scond, FloatPointer amax,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_csyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_csyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] s, float[] scond, float[] amax,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zsyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer s, DoublePointer scond, DoublePointer amax,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zsyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zsyequb_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] s, double[] scond, double[] amax,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_ssyev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, FloatPointer a, int lda, FloatPointer w,
FloatPointer work, int lwork );
public static native int LAPACKE_ssyev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, FloatBuffer a, int lda, FloatBuffer w,
FloatBuffer work, int lwork );
public static native int LAPACKE_ssyev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, float[] a, int lda, float[] w,
float[] work, int lwork );
public static native int LAPACKE_dsyev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, DoublePointer a, int lda,
DoublePointer w, DoublePointer work, int lwork );
public static native int LAPACKE_dsyev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, DoubleBuffer a, int lda,
DoubleBuffer w, DoubleBuffer work, int lwork );
public static native int LAPACKE_dsyev_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, double[] a, int lda,
double[] w, double[] work, int lwork );
public static native int LAPACKE_ssyevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, FloatPointer a, int lda,
FloatPointer w, FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_ssyevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, FloatBuffer a, int lda,
FloatBuffer w, FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_ssyevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, float[] a, int lda,
float[] w, float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_dsyevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, DoublePointer a, int lda,
DoublePointer w, DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dsyevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, DoubleBuffer a, int lda,
DoubleBuffer w, DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dsyevd_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte uplo,
int n, double[] a, int lda,
double[] w, double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_ssyevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, FloatPointer a,
int lda, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z,
int ldz, IntPointer isuppz, FloatPointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_ssyevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, FloatBuffer a,
int lda, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z,
int ldz, IntBuffer isuppz, FloatBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_ssyevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, float[] a,
int lda, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w, float[] z,
int ldz, int[] isuppz, float[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_dsyevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, DoublePointer a,
int lda, double vl, double vu,
int il, int iu, double abstol,
IntPointer m, DoublePointer w, DoublePointer z,
int ldz, IntPointer isuppz,
DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dsyevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, double vl, double vu,
int il, int iu, double abstol,
IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, IntBuffer isuppz,
DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dsyevr_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, double[] a,
int lda, double vl, double vu,
int il, int iu, double abstol,
int[] m, double[] w, double[] z,
int ldz, int[] isuppz,
double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_ssyevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, FloatPointer a,
int lda, float vl, float vu,
int il, int iu, float abstol,
IntPointer m, FloatPointer w, FloatPointer z,
int ldz, FloatPointer work, int lwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_ssyevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, FloatBuffer a,
int lda, float vl, float vu,
int il, int iu, float abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z,
int ldz, FloatBuffer work, int lwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_ssyevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, float[] a,
int lda, float vl, float vu,
int il, int iu, float abstol,
int[] m, float[] w, float[] z,
int ldz, float[] work, int lwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_dsyevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, DoublePointer a,
int lda, double vl, double vu,
int il, int iu, double abstol,
IntPointer m, DoublePointer w, DoublePointer z,
int ldz, DoublePointer work, int lwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_dsyevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, double vl, double vu,
int il, int iu, double abstol,
IntBuffer m, DoubleBuffer w, DoubleBuffer z,
int ldz, DoubleBuffer work, int lwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_dsyevx_work( int matrix_layout, @Cast("char") byte jobz, @Cast("char") byte range,
@Cast("char") byte uplo, int n, double[] a,
int lda, double vl, double vu,
int il, int iu, double abstol,
int[] m, double[] w, double[] z,
int ldz, double[] work, int lwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_ssygst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, FloatPointer a, int lda,
@Const FloatPointer b, int ldb );
public static native int LAPACKE_ssygst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, FloatBuffer a, int lda,
@Const FloatBuffer b, int ldb );
public static native int LAPACKE_ssygst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, float[] a, int lda,
@Const float[] b, int ldb );
public static native int LAPACKE_dsygst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, DoublePointer a, int lda,
@Const DoublePointer b, int ldb );
public static native int LAPACKE_dsygst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, DoubleBuffer a, int lda,
@Const DoubleBuffer b, int ldb );
public static native int LAPACKE_dsygst_work( int matrix_layout, int itype, @Cast("char") byte uplo,
int n, double[] a, int lda,
@Const double[] b, int ldb );
public static native int LAPACKE_ssygv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatPointer a,
int lda, FloatPointer b, int ldb,
FloatPointer w, FloatPointer work, int lwork );
public static native int LAPACKE_ssygv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
FloatBuffer w, FloatBuffer work, int lwork );
public static native int LAPACKE_ssygv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, float[] a,
int lda, float[] b, int ldb,
float[] w, float[] work, int lwork );
public static native int LAPACKE_dsygv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoublePointer a,
int lda, DoublePointer b, int ldb,
DoublePointer w, DoublePointer work, int lwork );
public static native int LAPACKE_dsygv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
DoubleBuffer w, DoubleBuffer work, int lwork );
public static native int LAPACKE_dsygv_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, double[] a,
int lda, double[] b, int ldb,
double[] w, double[] work, int lwork );
public static native int LAPACKE_ssygvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatPointer a,
int lda, FloatPointer b, int ldb,
FloatPointer w, FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_ssygvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
FloatBuffer w, FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_ssygvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, float[] a,
int lda, float[] b, int ldb,
float[] w, float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_dsygvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoublePointer a,
int lda, DoublePointer b, int ldb,
DoublePointer w, DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dsygvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
DoubleBuffer w, DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dsygvd_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte uplo, int n, double[] a,
int lda, double[] b, int ldb,
double[] w, double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_ssygvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, FloatPointer a,
int lda, FloatPointer b, int ldb,
float vl, float vu, int il,
int iu, float abstol, IntPointer m,
FloatPointer w, FloatPointer z, int ldz, FloatPointer work,
int lwork, IntPointer iwork,
IntPointer ifail );
public static native int LAPACKE_ssygvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
float vl, float vu, int il,
int iu, float abstol, IntBuffer m,
FloatBuffer w, FloatBuffer z, int ldz, FloatBuffer work,
int lwork, IntBuffer iwork,
IntBuffer ifail );
public static native int LAPACKE_ssygvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, float[] a,
int lda, float[] b, int ldb,
float vl, float vu, int il,
int iu, float abstol, int[] m,
float[] w, float[] z, int ldz, float[] work,
int lwork, int[] iwork,
int[] ifail );
public static native int LAPACKE_dsygvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, DoublePointer a,
int lda, DoublePointer b, int ldb,
double vl, double vu, int il,
int iu, double abstol, IntPointer m,
DoublePointer w, DoublePointer z, int ldz,
DoublePointer work, int lwork,
IntPointer iwork, IntPointer ifail );
public static native int LAPACKE_dsygvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
double vl, double vu, int il,
int iu, double abstol, IntBuffer m,
DoubleBuffer w, DoubleBuffer z, int ldz,
DoubleBuffer work, int lwork,
IntBuffer iwork, IntBuffer ifail );
public static native int LAPACKE_dsygvx_work( int matrix_layout, int itype, @Cast("char") byte jobz,
@Cast("char") byte range, @Cast("char") byte uplo, int n, double[] a,
int lda, double[] b, int ldb,
double vl, double vu, int il,
int iu, double abstol, int[] m,
double[] w, double[] z, int ldz,
double[] work, int lwork,
int[] iwork, int[] ifail );
public static native int LAPACKE_ssyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const FloatPointer af, int ldaf,
@Const IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_ssyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const FloatBuffer af, int ldaf,
@Const IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_ssyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
@Const float[] af, int ldaf,
@Const int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] ferr, float[] berr, float[] work,
int[] iwork );
public static native int LAPACKE_dsyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a,
int lda, @Const DoublePointer af,
int ldaf, @Const IntPointer ipiv,
@Const DoublePointer b, int ldb, DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dsyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a,
int lda, @Const DoubleBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Const DoubleBuffer b, int ldb, DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dsyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a,
int lda, @Const double[] af,
int ldaf, @Const int[] ipiv,
@Const double[] b, int ldb, double[] x,
int ldx, double[] ferr, double[] berr,
double[] work, int[] iwork );
public static native int LAPACKE_csyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_csyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_csyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_zsyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("const lapack_complex_double*") DoublePointer af,
int ldaf, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_zsyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("const lapack_complex_double*") DoubleBuffer af,
int ldaf, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_zsyrfs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("const lapack_complex_double*") double[] af,
int ldaf, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_ssysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer a, int lda,
IntPointer ipiv, FloatPointer b, int ldb,
FloatPointer work, int lwork );
public static native int LAPACKE_ssysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer a, int lda,
IntBuffer ipiv, FloatBuffer b, int ldb,
FloatBuffer work, int lwork );
public static native int LAPACKE_ssysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] a, int lda,
int[] ipiv, float[] b, int ldb,
float[] work, int lwork );
public static native int LAPACKE_dsysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer a, int lda,
IntPointer ipiv, DoublePointer b, int ldb,
DoublePointer work, int lwork );
public static native int LAPACKE_dsysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer a, int lda,
IntBuffer ipiv, DoubleBuffer b, int ldb,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dsysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] a, int lda,
int[] ipiv, double[] b, int ldb,
double[] work, int lwork );
public static native int LAPACKE_csysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_csysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_csysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zsysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zsysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zsysv_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_ssysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const FloatPointer a,
int lda, FloatPointer af, int ldaf,
IntPointer ipiv, @Const FloatPointer b,
int ldb, FloatPointer x, int ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_ssysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const FloatBuffer a,
int lda, FloatBuffer af, int ldaf,
IntBuffer ipiv, @Const FloatBuffer b,
int ldb, FloatBuffer x, int ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_ssysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const float[] a,
int lda, float[] af, int ldaf,
int[] ipiv, @Const float[] b,
int ldb, float[] x, int ldx,
float[] rcond, float[] ferr, float[] berr,
float[] work, int lwork,
int[] iwork );
public static native int LAPACKE_dsysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const DoublePointer a,
int lda, DoublePointer af, int ldaf,
IntPointer ipiv, @Const DoublePointer b,
int ldb, DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_dsysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const DoubleBuffer a,
int lda, DoubleBuffer af, int ldaf,
IntBuffer ipiv, @Const DoubleBuffer b,
int ldb, DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_dsysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs, @Const double[] a,
int lda, double[] af, int ldaf,
int[] ipiv, @Const double[] b,
int ldb, double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
double[] work, int lwork,
int[] iwork );
public static native int LAPACKE_csysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer af, int ldaf,
IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
int ldb, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer rcond, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork );
public static native int LAPACKE_csysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer af, int ldaf,
IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer rcond, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork );
public static native int LAPACKE_csysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] af, int ldaf,
int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int ldb, @Cast("lapack_complex_float*") float[] x,
int ldx, float[] rcond, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork );
public static native int LAPACKE_zsysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer af, int ldaf,
IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork );
public static native int LAPACKE_zsysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer af, int ldaf,
IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork );
public static native int LAPACKE_zsysvx_work( int matrix_layout, @Cast("char") byte fact, @Cast("char") byte uplo,
int n, int nrhs,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] af, int ldaf,
int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] x, int ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork );
public static native int LAPACKE_ssytrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda, FloatPointer d, FloatPointer e,
FloatPointer tau, FloatPointer work, int lwork );
public static native int LAPACKE_ssytrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda, FloatBuffer d, FloatBuffer e,
FloatBuffer tau, FloatBuffer work, int lwork );
public static native int LAPACKE_ssytrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda, float[] d, float[] e,
float[] tau, float[] work, int lwork );
public static native int LAPACKE_dsytrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda, DoublePointer d, DoublePointer e,
DoublePointer tau, DoublePointer work, int lwork );
public static native int LAPACKE_dsytrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda, DoubleBuffer d, DoubleBuffer e,
DoubleBuffer tau, DoubleBuffer work, int lwork );
public static native int LAPACKE_dsytrd_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda, double[] d, double[] e,
double[] tau, double[] work, int lwork );
public static native int LAPACKE_ssytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda, IntPointer ipiv,
FloatPointer work, int lwork );
public static native int LAPACKE_ssytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda, IntBuffer ipiv,
FloatBuffer work, int lwork );
public static native int LAPACKE_ssytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda, int[] ipiv,
float[] work, int lwork );
public static native int LAPACKE_dsytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda, IntPointer ipiv,
DoublePointer work, int lwork );
public static native int LAPACKE_dsytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda, IntBuffer ipiv,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dsytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda, int[] ipiv,
double[] work, int lwork );
public static native int LAPACKE_csytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer work,
int lwork );
public static native int LAPACKE_csytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork );
public static native int LAPACKE_csytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv, @Cast("lapack_complex_float*") float[] work,
int lwork );
public static native int LAPACKE_zsytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer work,
int lwork );
public static native int LAPACKE_zsytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork );
public static native int LAPACKE_zsytrf_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv, @Cast("lapack_complex_double*") double[] work,
int lwork );
public static native int LAPACKE_ssytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer work );
public static native int LAPACKE_ssytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer work );
public static native int LAPACKE_ssytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda,
@Const int[] ipiv, float[] work );
public static native int LAPACKE_dsytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda,
@Const IntPointer ipiv, DoublePointer work );
public static native int LAPACKE_dsytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda,
@Const IntBuffer ipiv, DoubleBuffer work );
public static native int LAPACKE_dsytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda,
@Const int[] ipiv, double[] work );
public static native int LAPACKE_csytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_csytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_csytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zsytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zsytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zsytri_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_ssytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer b,
int ldb );
public static native int LAPACKE_ssytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer b,
int ldb );
public static native int LAPACKE_ssytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
@Const int[] ipiv, float[] b,
int ldb );
public static native int LAPACKE_dsytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a,
int lda, @Const IntPointer ipiv,
DoublePointer b, int ldb );
public static native int LAPACKE_dsytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dsytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a,
int lda, @Const int[] ipiv,
double[] b, int ldb );
public static native int LAPACKE_csytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_csytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_csytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zsytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zsytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zsytrs_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_stbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Const FloatPointer ab, int ldab, FloatPointer rcond,
FloatPointer work, IntPointer iwork );
public static native int LAPACKE_stbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Const FloatBuffer ab, int ldab, FloatBuffer rcond,
FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_stbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Const float[] ab, int ldab, float[] rcond,
float[] work, int[] iwork );
public static native int LAPACKE_dtbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Const DoublePointer ab, int ldab,
DoublePointer rcond, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dtbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Const DoubleBuffer ab, int ldab,
DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dtbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Const double[] ab, int ldab,
double[] rcond, double[] work,
int[] iwork );
public static native int LAPACKE_ctbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Cast("const lapack_complex_float*") FloatPointer ab, int ldab,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_ctbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Cast("const lapack_complex_float*") FloatBuffer ab, int ldab,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_ctbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Cast("const lapack_complex_float*") float[] ab, int ldab,
float[] rcond, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_ztbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_ztbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_ztbcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, int kd,
@Cast("const lapack_complex_double*") double[] ab,
int ldab, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_stbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const FloatPointer ab,
int ldab, @Const FloatPointer b, int ldb,
@Const FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr, FloatPointer work, IntPointer iwork );
public static native int LAPACKE_stbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const FloatBuffer ab,
int ldab, @Const FloatBuffer b, int ldb,
@Const FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr, FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_stbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const float[] ab,
int ldab, @Const float[] b, int ldb,
@Const float[] x, int ldx, float[] ferr,
float[] berr, float[] work, int[] iwork );
public static native int LAPACKE_dtbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const DoublePointer ab,
int ldab, @Const DoublePointer b,
int ldb, @Const DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dtbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const DoubleBuffer ab,
int ldab, @Const DoubleBuffer b,
int ldb, @Const DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dtbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const double[] ab,
int ldab, @Const double[] b,
int ldb, @Const double[] x, int ldx,
double[] ferr, double[] berr, double[] work,
int[] iwork );
public static native int LAPACKE_ctbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ab,
int ldab, @Cast("const lapack_complex_float*") FloatPointer b,
int ldb, @Cast("const lapack_complex_float*") FloatPointer x,
int ldx, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_ctbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab, @Cast("const lapack_complex_float*") FloatBuffer b,
int ldb, @Cast("const lapack_complex_float*") FloatBuffer x,
int ldx, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_ctbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Cast("const lapack_complex_float*") float[] ab,
int ldab, @Cast("const lapack_complex_float*") float[] b,
int ldb, @Cast("const lapack_complex_float*") float[] x,
int ldx, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_ztbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, @Cast("const lapack_complex_double*") DoublePointer b,
int ldb, @Cast("const lapack_complex_double*") DoublePointer x,
int ldx, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_ztbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, @Cast("const lapack_complex_double*") DoubleBuffer b,
int ldb, @Cast("const lapack_complex_double*") DoubleBuffer x,
int ldx, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_ztbrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs,
@Cast("const lapack_complex_double*") double[] ab,
int ldab, @Cast("const lapack_complex_double*") double[] b,
int ldb, @Cast("const lapack_complex_double*") double[] x,
int ldx, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_stbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const FloatPointer ab,
int ldab, FloatPointer b, int ldb );
public static native int LAPACKE_stbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const FloatBuffer ab,
int ldab, FloatBuffer b, int ldb );
public static native int LAPACKE_stbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const float[] ab,
int ldab, float[] b, int ldb );
public static native int LAPACKE_dtbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const DoublePointer ab,
int ldab, DoublePointer b, int ldb );
public static native int LAPACKE_dtbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const DoubleBuffer ab,
int ldab, DoubleBuffer b, int ldb );
public static native int LAPACKE_dtbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Const double[] ab,
int ldab, double[] b, int ldb );
public static native int LAPACKE_ctbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer ab,
int ldab, @Cast("lapack_complex_float*") FloatPointer b,
int ldb );
public static native int LAPACKE_ctbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab, @Cast("lapack_complex_float*") FloatBuffer b,
int ldb );
public static native int LAPACKE_ctbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs, @Cast("const lapack_complex_float*") float[] ab,
int ldab, @Cast("lapack_complex_float*") float[] b,
int ldb );
public static native int LAPACKE_ztbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab, @Cast("lapack_complex_double*") DoublePointer b,
int ldb );
public static native int LAPACKE_ztbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab, @Cast("lapack_complex_double*") DoubleBuffer b,
int ldb );
public static native int LAPACKE_ztbtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int kd,
int nrhs,
@Cast("const lapack_complex_double*") double[] ab,
int ldab, @Cast("lapack_complex_double*") double[] b,
int ldb );
public static native int LAPACKE_stfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, float alpha, @Const FloatPointer a,
FloatPointer b, int ldb );
public static native int LAPACKE_stfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, float alpha, @Const FloatBuffer a,
FloatBuffer b, int ldb );
public static native int LAPACKE_stfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, float alpha, @Const float[] a,
float[] b, int ldb );
public static native int LAPACKE_dtfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, double alpha, @Const DoublePointer a,
DoublePointer b, int ldb );
public static native int LAPACKE_dtfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, double alpha, @Const DoubleBuffer a,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dtfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, double alpha, @Const double[] a,
double[] b, int ldb );
public static native int LAPACKE_ctfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, @ByVal @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("const lapack_complex_float*") FloatPointer a,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_ctfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, @ByVal @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("const lapack_complex_float*") FloatBuffer a,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_ctfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, @ByVal @Cast("lapack_complex_float*") float[] alpha,
@Cast("const lapack_complex_float*") float[] a,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_ztfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, @ByVal @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("const lapack_complex_double*") DoublePointer a,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_ztfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, @ByVal @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("const lapack_complex_double*") DoubleBuffer a,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_ztfsm_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte side,
@Cast("char") byte uplo, @Cast("char") byte trans, @Cast("char") byte diag, int m,
int n, @ByVal @Cast("lapack_complex_double*") double[] alpha,
@Cast("const lapack_complex_double*") double[] a,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_stftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, FloatPointer a );
public static native int LAPACKE_stftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, FloatBuffer a );
public static native int LAPACKE_stftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, float[] a );
public static native int LAPACKE_dtftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, DoublePointer a );
public static native int LAPACKE_dtftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, DoubleBuffer a );
public static native int LAPACKE_dtftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, double[] a );
public static native int LAPACKE_ctftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("lapack_complex_float*") FloatPointer a );
public static native int LAPACKE_ctftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("lapack_complex_float*") FloatBuffer a );
public static native int LAPACKE_ctftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("lapack_complex_float*") float[] a );
public static native int LAPACKE_ztftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("lapack_complex_double*") DoublePointer a );
public static native int LAPACKE_ztftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("lapack_complex_double*") DoubleBuffer a );
public static native int LAPACKE_ztftri_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("lapack_complex_double*") double[] a );
public static native int LAPACKE_stfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatPointer arf, FloatPointer ap );
public static native int LAPACKE_stfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatBuffer arf, FloatBuffer ap );
public static native int LAPACKE_stfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const float[] arf, float[] ap );
public static native int LAPACKE_dtfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoublePointer arf, DoublePointer ap );
public static native int LAPACKE_dtfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoubleBuffer arf, DoubleBuffer ap );
public static native int LAPACKE_dtfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const double[] arf, double[] ap );
public static native int LAPACKE_ctfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer arf,
@Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_ctfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer arf,
@Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_ctfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] arf,
@Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_ztfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer arf,
@Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_ztfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer arf,
@Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_ztfttp_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] arf,
@Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_stfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatPointer arf, FloatPointer a,
int lda );
public static native int LAPACKE_stfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatBuffer arf, FloatBuffer a,
int lda );
public static native int LAPACKE_stfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const float[] arf, float[] a,
int lda );
public static native int LAPACKE_dtfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoublePointer arf, DoublePointer a,
int lda );
public static native int LAPACKE_dtfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoubleBuffer arf, DoubleBuffer a,
int lda );
public static native int LAPACKE_dtfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const double[] arf, double[] a,
int lda );
public static native int LAPACKE_ctfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer arf,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_ctfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer arf,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_ctfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] arf,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_ztfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer arf,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_ztfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer arf,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_ztfttr_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] arf,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_stgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const FloatPointer s, int lds, @Const FloatPointer p,
int ldp, FloatPointer vl, int ldvl,
FloatPointer vr, int ldvr, int mm,
IntPointer m, FloatPointer work );
public static native int LAPACKE_stgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const FloatBuffer s, int lds, @Const FloatBuffer p,
int ldp, FloatBuffer vl, int ldvl,
FloatBuffer vr, int ldvr, int mm,
IntBuffer m, FloatBuffer work );
public static native int LAPACKE_stgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const float[] s, int lds, @Const float[] p,
int ldp, float[] vl, int ldvl,
float[] vr, int ldvr, int mm,
int[] m, float[] work );
public static native int LAPACKE_dtgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const DoublePointer s, int lds,
@Const DoublePointer p, int ldp, DoublePointer vl,
int ldvl, DoublePointer vr, int ldvr,
int mm, IntPointer m, DoublePointer work );
public static native int LAPACKE_dtgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const DoubleBuffer s, int lds,
@Const DoubleBuffer p, int ldp, DoubleBuffer vl,
int ldvl, DoubleBuffer vr, int ldvr,
int mm, IntBuffer m, DoubleBuffer work );
public static native int LAPACKE_dtgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const double[] s, int lds,
@Const double[] p, int ldp, double[] vl,
int ldvl, double[] vr, int ldvr,
int mm, int[] m, double[] work );
public static native int LAPACKE_ctgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_float*") FloatPointer s, int lds,
@Cast("const lapack_complex_float*") FloatPointer p, int ldp,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
int mm, IntPointer m,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_ctgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_float*") FloatBuffer s, int lds,
@Cast("const lapack_complex_float*") FloatBuffer p, int ldp,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
int mm, IntBuffer m,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_ctgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_float*") float[] s, int lds,
@Cast("const lapack_complex_float*") float[] p, int ldp,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
int mm, int[] m,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_ztgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_double*") DoublePointer s, int lds,
@Cast("const lapack_complex_double*") DoublePointer p, int ldp,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
int mm, IntPointer m,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_ztgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_double*") DoubleBuffer s, int lds,
@Cast("const lapack_complex_double*") DoubleBuffer p, int ldp,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
int mm, IntBuffer m,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_ztgevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_double*") double[] s, int lds,
@Cast("const lapack_complex_double*") double[] p, int ldp,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
int mm, int[] m,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_stgexc_work( int matrix_layout, int wantq,
int wantz, int n, FloatPointer a,
int lda, FloatPointer b, int ldb,
FloatPointer q, int ldq, FloatPointer z,
int ldz, IntPointer ifst,
IntPointer ilst, FloatPointer work,
int lwork );
public static native int LAPACKE_stgexc_work( int matrix_layout, int wantq,
int wantz, int n, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
FloatBuffer q, int ldq, FloatBuffer z,
int ldz, IntBuffer ifst,
IntBuffer ilst, FloatBuffer work,
int lwork );
public static native int LAPACKE_stgexc_work( int matrix_layout, int wantq,
int wantz, int n, float[] a,
int lda, float[] b, int ldb,
float[] q, int ldq, float[] z,
int ldz, int[] ifst,
int[] ilst, float[] work,
int lwork );
public static native int LAPACKE_dtgexc_work( int matrix_layout, int wantq,
int wantz, int n, DoublePointer a,
int lda, DoublePointer b, int ldb,
DoublePointer q, int ldq, DoublePointer z,
int ldz, IntPointer ifst,
IntPointer ilst, DoublePointer work,
int lwork );
public static native int LAPACKE_dtgexc_work( int matrix_layout, int wantq,
int wantz, int n, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
DoubleBuffer q, int ldq, DoubleBuffer z,
int ldz, IntBuffer ifst,
IntBuffer ilst, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dtgexc_work( int matrix_layout, int wantq,
int wantz, int n, double[] a,
int lda, double[] b, int ldb,
double[] q, int ldq, double[] z,
int ldz, int[] ifst,
int[] ilst, double[] work,
int lwork );
public static native int LAPACKE_ctgexc_work( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ctgexc_work( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ctgexc_work( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ztgexc_work( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ztgexc_work( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_ztgexc_work( int matrix_layout, int wantq,
int wantz, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz,
int ifst, int ilst );
public static native int LAPACKE_stgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntPointer select, int n,
FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer alphar, FloatPointer alphai,
FloatPointer beta, FloatPointer q, int ldq, FloatPointer z,
int ldz, IntPointer m, FloatPointer pl,
FloatPointer pr, FloatPointer dif, FloatPointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_stgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntBuffer select, int n,
FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer alphar, FloatBuffer alphai,
FloatBuffer beta, FloatBuffer q, int ldq, FloatBuffer z,
int ldz, IntBuffer m, FloatBuffer pl,
FloatBuffer pr, FloatBuffer dif, FloatBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_stgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const int[] select, int n,
float[] a, int lda, float[] b,
int ldb, float[] alphar, float[] alphai,
float[] beta, float[] q, int ldq, float[] z,
int ldz, int[] m, float[] pl,
float[] pr, float[] dif, float[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_dtgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntPointer select, int n,
DoublePointer a, int lda, DoublePointer b,
int ldb, DoublePointer alphar, DoublePointer alphai,
DoublePointer beta, DoublePointer q, int ldq,
DoublePointer z, int ldz, IntPointer m,
DoublePointer pl, DoublePointer pr, DoublePointer dif,
DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dtgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntBuffer select, int n,
DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, DoubleBuffer alphar, DoubleBuffer alphai,
DoubleBuffer beta, DoubleBuffer q, int ldq,
DoubleBuffer z, int ldz, IntBuffer m,
DoubleBuffer pl, DoubleBuffer pr, DoubleBuffer dif,
DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dtgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const int[] select, int n,
double[] a, int lda, double[] b,
int ldb, double[] alphar, double[] alphai,
double[] beta, double[] q, int ldq,
double[] z, int ldz, int[] m,
double[] pl, double[] pr, double[] dif,
double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_ctgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntPointer select, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer z, int ldz,
IntPointer m, FloatPointer pl, FloatPointer pr, FloatPointer dif,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_ctgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntBuffer select, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer z, int ldz,
IntBuffer m, FloatBuffer pl, FloatBuffer pr, FloatBuffer dif,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_ctgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const int[] select, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] z, int ldz,
int[] m, float[] pl, float[] pr, float[] dif,
@Cast("lapack_complex_float*") float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_ztgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntPointer select, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer z, int ldz,
IntPointer m, DoublePointer pl, DoublePointer pr,
DoublePointer dif, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, IntPointer iwork,
int liwork );
public static native int LAPACKE_ztgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const IntBuffer select, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, int ldz,
IntBuffer m, DoubleBuffer pl, DoubleBuffer pr,
DoubleBuffer dif, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, IntBuffer iwork,
int liwork );
public static native int LAPACKE_ztgsen_work( int matrix_layout, int ijob,
int wantq, int wantz,
@Const int[] select, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] z, int ldz,
int[] m, double[] pl, double[] pr,
double[] dif, @Cast("lapack_complex_double*") double[] work,
int lwork, int[] iwork,
int liwork );
public static native int LAPACKE_stgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
FloatPointer a, int lda, FloatPointer b,
int ldb, float tola, float tolb,
FloatPointer alpha, FloatPointer beta, FloatPointer u,
int ldu, FloatPointer v, int ldv,
FloatPointer q, int ldq, FloatPointer work,
IntPointer ncycle );
public static native int LAPACKE_stgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
FloatBuffer a, int lda, FloatBuffer b,
int ldb, float tola, float tolb,
FloatBuffer alpha, FloatBuffer beta, FloatBuffer u,
int ldu, FloatBuffer v, int ldv,
FloatBuffer q, int ldq, FloatBuffer work,
IntBuffer ncycle );
public static native int LAPACKE_stgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
float[] a, int lda, float[] b,
int ldb, float tola, float tolb,
float[] alpha, float[] beta, float[] u,
int ldu, float[] v, int ldv,
float[] q, int ldq, float[] work,
int[] ncycle );
public static native int LAPACKE_dtgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
DoublePointer a, int lda, DoublePointer b,
int ldb, double tola, double tolb,
DoublePointer alpha, DoublePointer beta, DoublePointer u,
int ldu, DoublePointer v, int ldv,
DoublePointer q, int ldq, DoublePointer work,
IntPointer ncycle );
public static native int LAPACKE_dtgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
DoubleBuffer a, int lda, DoubleBuffer b,
int ldb, double tola, double tolb,
DoubleBuffer alpha, DoubleBuffer beta, DoubleBuffer u,
int ldu, DoubleBuffer v, int ldv,
DoubleBuffer q, int ldq, DoubleBuffer work,
IntBuffer ncycle );
public static native int LAPACKE_dtgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
double[] a, int lda, double[] b,
int ldb, double tola, double tolb,
double[] alpha, double[] beta, double[] u,
int ldu, double[] v, int ldv,
double[] q, int ldq, double[] work,
int[] ncycle );
public static native int LAPACKE_ctgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
float tola, float tolb, FloatPointer alpha,
FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer u,
int ldu, @Cast("lapack_complex_float*") FloatPointer v,
int ldv, @Cast("lapack_complex_float*") FloatPointer q,
int ldq, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer ncycle );
public static native int LAPACKE_ctgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
float tola, float tolb, FloatBuffer alpha,
FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer u,
int ldu, @Cast("lapack_complex_float*") FloatBuffer v,
int ldv, @Cast("lapack_complex_float*") FloatBuffer q,
int ldq, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer ncycle );
public static native int LAPACKE_ctgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
float tola, float tolb, float[] alpha,
float[] beta, @Cast("lapack_complex_float*") float[] u,
int ldu, @Cast("lapack_complex_float*") float[] v,
int ldv, @Cast("lapack_complex_float*") float[] q,
int ldq, @Cast("lapack_complex_float*") float[] work,
int[] ncycle );
public static native int LAPACKE_ztgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
double tola, double tolb, DoublePointer alpha,
DoublePointer beta, @Cast("lapack_complex_double*") DoublePointer u,
int ldu, @Cast("lapack_complex_double*") DoublePointer v,
int ldv, @Cast("lapack_complex_double*") DoublePointer q,
int ldq, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer ncycle );
public static native int LAPACKE_ztgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
double tola, double tolb, DoubleBuffer alpha,
DoubleBuffer beta, @Cast("lapack_complex_double*") DoubleBuffer u,
int ldu, @Cast("lapack_complex_double*") DoubleBuffer v,
int ldv, @Cast("lapack_complex_double*") DoubleBuffer q,
int ldq, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer ncycle );
public static native int LAPACKE_ztgsja_work( int matrix_layout, @Cast("char") byte jobu, @Cast("char") byte jobv,
@Cast("char") byte jobq, int m, int p,
int n, int k, int l,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
double tola, double tolb, double[] alpha,
double[] beta, @Cast("lapack_complex_double*") double[] u,
int ldu, @Cast("lapack_complex_double*") double[] v,
int ldv, @Cast("lapack_complex_double*") double[] q,
int ldq, @Cast("lapack_complex_double*") double[] work,
int[] ncycle );
public static native int LAPACKE_stgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const FloatPointer a, int lda, @Const FloatPointer b,
int ldb, @Const FloatPointer vl,
int ldvl, @Const FloatPointer vr,
int ldvr, FloatPointer s, FloatPointer dif,
int mm, IntPointer m, FloatPointer work,
int lwork, IntPointer iwork );
public static native int LAPACKE_stgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const FloatBuffer a, int lda, @Const FloatBuffer b,
int ldb, @Const FloatBuffer vl,
int ldvl, @Const FloatBuffer vr,
int ldvr, FloatBuffer s, FloatBuffer dif,
int mm, IntBuffer m, FloatBuffer work,
int lwork, IntBuffer iwork );
public static native int LAPACKE_stgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const float[] a, int lda, @Const float[] b,
int ldb, @Const float[] vl,
int ldvl, @Const float[] vr,
int ldvr, float[] s, float[] dif,
int mm, int[] m, float[] work,
int lwork, int[] iwork );
public static native int LAPACKE_dtgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const DoublePointer a, int lda,
@Const DoublePointer b, int ldb,
@Const DoublePointer vl, int ldvl,
@Const DoublePointer vr, int ldvr, DoublePointer s,
DoublePointer dif, int mm, IntPointer m,
DoublePointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_dtgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const DoubleBuffer a, int lda,
@Const DoubleBuffer b, int ldb,
@Const DoubleBuffer vl, int ldvl,
@Const DoubleBuffer vr, int ldvr, DoubleBuffer s,
DoubleBuffer dif, int mm, IntBuffer m,
DoubleBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_dtgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const double[] a, int lda,
@Const double[] b, int ldb,
@Const double[] vl, int ldvl,
@Const double[] vr, int ldvr, double[] s,
double[] dif, int mm, int[] m,
double[] work, int lwork,
int[] iwork );
public static native int LAPACKE_ctgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("const lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("const lapack_complex_float*") FloatPointer vr, int ldvr,
FloatPointer s, FloatPointer dif, int mm,
IntPointer m, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, IntPointer iwork );
public static native int LAPACKE_ctgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("const lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("const lapack_complex_float*") FloatBuffer vr, int ldvr,
FloatBuffer s, FloatBuffer dif, int mm,
IntBuffer m, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, IntBuffer iwork );
public static native int LAPACKE_ctgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("const lapack_complex_float*") float[] vl, int ldvl,
@Cast("const lapack_complex_float*") float[] vr, int ldvr,
float[] s, float[] dif, int mm,
int[] m, @Cast("lapack_complex_float*") float[] work,
int lwork, int[] iwork );
public static native int LAPACKE_ztgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("const lapack_complex_double*") DoublePointer vl,
int ldvl,
@Cast("const lapack_complex_double*") DoublePointer vr,
int ldvr, DoublePointer s, DoublePointer dif,
int mm, IntPointer m,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_ztgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("const lapack_complex_double*") DoubleBuffer vl,
int ldvl,
@Cast("const lapack_complex_double*") DoubleBuffer vr,
int ldvr, DoubleBuffer s, DoubleBuffer dif,
int mm, IntBuffer m,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_ztgsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("const lapack_complex_double*") double[] vl,
int ldvl,
@Cast("const lapack_complex_double*") double[] vr,
int ldvr, double[] s, double[] dif,
int mm, int[] m,
@Cast("lapack_complex_double*") double[] work, int lwork,
int[] iwork );
public static native int LAPACKE_stgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const FloatPointer a,
int lda, @Const FloatPointer b, int ldb,
FloatPointer c, int ldc, @Const FloatPointer d,
int ldd, @Const FloatPointer e, int lde,
FloatPointer f, int ldf, FloatPointer scale,
FloatPointer dif, FloatPointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_stgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const FloatBuffer a,
int lda, @Const FloatBuffer b, int ldb,
FloatBuffer c, int ldc, @Const FloatBuffer d,
int ldd, @Const FloatBuffer e, int lde,
FloatBuffer f, int ldf, FloatBuffer scale,
FloatBuffer dif, FloatBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_stgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const float[] a,
int lda, @Const float[] b, int ldb,
float[] c, int ldc, @Const float[] d,
int ldd, @Const float[] e, int lde,
float[] f, int ldf, float[] scale,
float[] dif, float[] work, int lwork,
int[] iwork );
public static native int LAPACKE_dtgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const DoublePointer a,
int lda, @Const DoublePointer b, int ldb,
DoublePointer c, int ldc, @Const DoublePointer d,
int ldd, @Const DoublePointer e, int lde,
DoublePointer f, int ldf, DoublePointer scale,
DoublePointer dif, DoublePointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_dtgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const DoubleBuffer a,
int lda, @Const DoubleBuffer b, int ldb,
DoubleBuffer c, int ldc, @Const DoubleBuffer d,
int ldd, @Const DoubleBuffer e, int lde,
DoubleBuffer f, int ldf, DoubleBuffer scale,
DoubleBuffer dif, DoubleBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_dtgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n, @Const double[] a,
int lda, @Const double[] b, int ldb,
double[] c, int ldc, @Const double[] d,
int ldd, @Const double[] e, int lde,
double[] f, int ldf, double[] scale,
double[] dif, double[] work, int lwork,
int[] iwork );
public static native int LAPACKE_ctgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("const lapack_complex_float*") FloatPointer d, int ldd,
@Cast("const lapack_complex_float*") FloatPointer e, int lde,
@Cast("lapack_complex_float*") FloatPointer f, int ldf,
FloatPointer scale, FloatPointer dif,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_ctgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("const lapack_complex_float*") FloatBuffer d, int ldd,
@Cast("const lapack_complex_float*") FloatBuffer e, int lde,
@Cast("lapack_complex_float*") FloatBuffer f, int ldf,
FloatBuffer scale, FloatBuffer dif,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_ctgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("const lapack_complex_float*") float[] d, int ldd,
@Cast("const lapack_complex_float*") float[] e, int lde,
@Cast("lapack_complex_float*") float[] f, int ldf,
float[] scale, float[] dif,
@Cast("lapack_complex_float*") float[] work, int lwork,
int[] iwork );
public static native int LAPACKE_ztgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("const lapack_complex_double*") DoublePointer d, int ldd,
@Cast("const lapack_complex_double*") DoublePointer e, int lde,
@Cast("lapack_complex_double*") DoublePointer f, int ldf,
DoublePointer scale, DoublePointer dif,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_ztgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("const lapack_complex_double*") DoubleBuffer d, int ldd,
@Cast("const lapack_complex_double*") DoubleBuffer e, int lde,
@Cast("lapack_complex_double*") DoubleBuffer f, int ldf,
DoubleBuffer scale, DoubleBuffer dif,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_ztgsyl_work( int matrix_layout, @Cast("char") byte trans, int ijob,
int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("const lapack_complex_double*") double[] d, int ldd,
@Cast("const lapack_complex_double*") double[] e, int lde,
@Cast("lapack_complex_double*") double[] f, int ldf,
double[] scale, double[] dif,
@Cast("lapack_complex_double*") double[] work, int lwork,
int[] iwork );
public static native int LAPACKE_stpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const FloatPointer ap,
FloatPointer rcond, FloatPointer work, IntPointer iwork );
public static native int LAPACKE_stpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const FloatBuffer ap,
FloatBuffer rcond, FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_stpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const float[] ap,
float[] rcond, float[] work, int[] iwork );
public static native int LAPACKE_dtpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const DoublePointer ap,
DoublePointer rcond, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dtpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const DoubleBuffer ap,
DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dtpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const double[] ap,
double[] rcond, double[] work,
int[] iwork );
public static native int LAPACKE_ctpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_float*") FloatPointer ap, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_ctpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_ctpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_float*") float[] ap, float[] rcond,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_ztpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_double*") DoublePointer ap, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_ztpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_ztpcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_double*") double[] ap, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_stprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const FloatPointer ap, @Const FloatPointer b, int ldb,
@Const FloatPointer x, int ldx, FloatPointer ferr,
FloatPointer berr, FloatPointer work, IntPointer iwork );
public static native int LAPACKE_stprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const FloatBuffer ap, @Const FloatBuffer b, int ldb,
@Const FloatBuffer x, int ldx, FloatBuffer ferr,
FloatBuffer berr, FloatBuffer work, IntBuffer iwork );
public static native int LAPACKE_stprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const float[] ap, @Const float[] b, int ldb,
@Const float[] x, int ldx, float[] ferr,
float[] berr, float[] work, int[] iwork );
public static native int LAPACKE_dtprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const DoublePointer ap, @Const DoublePointer b,
int ldb, @Const DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dtprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const DoubleBuffer ap, @Const DoubleBuffer b,
int ldb, @Const DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dtprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const double[] ap, @Const double[] b,
int ldb, @Const double[] x, int ldx,
double[] ferr, double[] berr, double[] work,
int[] iwork );
public static native int LAPACKE_ctprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("const lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_ctprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("const lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_ctprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("const lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_ztprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("const lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_ztprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("const lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_ztprfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("const lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_stptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, FloatPointer ap );
public static native int LAPACKE_stptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, FloatBuffer ap );
public static native int LAPACKE_stptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, float[] ap );
public static native int LAPACKE_dtptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, DoublePointer ap );
public static native int LAPACKE_dtptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, DoubleBuffer ap );
public static native int LAPACKE_dtptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, double[] ap );
public static native int LAPACKE_ctptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_ctptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_ctptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_ztptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_ztptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_ztptri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_stptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const FloatPointer ap, FloatPointer b, int ldb );
public static native int LAPACKE_stptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const FloatBuffer ap, FloatBuffer b, int ldb );
public static native int LAPACKE_stptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const float[] ap, float[] b, int ldb );
public static native int LAPACKE_dtptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const DoublePointer ap, DoublePointer b, int ldb );
public static native int LAPACKE_dtptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const DoubleBuffer ap, DoubleBuffer b, int ldb );
public static native int LAPACKE_dtptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const double[] ap, double[] b, int ldb );
public static native int LAPACKE_ctptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_ctptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_ctptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_ztptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_ztptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_ztptrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_stpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatPointer ap, FloatPointer arf );
public static native int LAPACKE_stpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatBuffer ap, FloatBuffer arf );
public static native int LAPACKE_stpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const float[] ap, float[] arf );
public static native int LAPACKE_dtpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoublePointer ap, DoublePointer arf );
public static native int LAPACKE_dtpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoubleBuffer ap, DoubleBuffer arf );
public static native int LAPACKE_dtpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const double[] ap, double[] arf );
public static native int LAPACKE_ctpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer arf );
public static native int LAPACKE_ctpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer arf );
public static native int LAPACKE_ctpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] arf );
public static native int LAPACKE_ztpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer arf );
public static native int LAPACKE_ztpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer arf );
public static native int LAPACKE_ztpttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] arf );
public static native int LAPACKE_stpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer ap, FloatPointer a, int lda );
public static native int LAPACKE_stpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer ap, FloatBuffer a, int lda );
public static native int LAPACKE_stpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] ap, float[] a, int lda );
public static native int LAPACKE_dtpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer ap, DoublePointer a, int lda );
public static native int LAPACKE_dtpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer ap, DoubleBuffer a, int lda );
public static native int LAPACKE_dtpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] ap, double[] a, int lda );
public static native int LAPACKE_ctpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_ctpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_ctpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_ztpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_ztpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_ztpttr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_strcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const FloatPointer a,
int lda, FloatPointer rcond, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_strcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const FloatBuffer a,
int lda, FloatBuffer rcond, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_strcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const float[] a,
int lda, float[] rcond, float[] work,
int[] iwork );
public static native int LAPACKE_dtrcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const DoublePointer a,
int lda, DoublePointer rcond, DoublePointer work,
IntPointer iwork );
public static native int LAPACKE_dtrcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const DoubleBuffer a,
int lda, DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork );
public static native int LAPACKE_dtrcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n, @Const double[] a,
int lda, double[] rcond, double[] work,
int[] iwork );
public static native int LAPACKE_ctrcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork );
public static native int LAPACKE_ctrcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork );
public static native int LAPACKE_ctrcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
float[] rcond, @Cast("lapack_complex_float*") float[] work,
float[] rwork );
public static native int LAPACKE_ztrcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork );
public static native int LAPACKE_ztrcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork );
public static native int LAPACKE_ztrcon_work( int matrix_layout, @Cast("char") byte norm, @Cast("char") byte uplo,
@Cast("char") byte diag, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
double[] rcond, @Cast("lapack_complex_double*") double[] work,
double[] rwork );
public static native int LAPACKE_strevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
IntPointer select, int n,
@Const FloatPointer t, int ldt, FloatPointer vl,
int ldvl, FloatPointer vr, int ldvr,
int mm, IntPointer m, FloatPointer work );
public static native int LAPACKE_strevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
IntBuffer select, int n,
@Const FloatBuffer t, int ldt, FloatBuffer vl,
int ldvl, FloatBuffer vr, int ldvr,
int mm, IntBuffer m, FloatBuffer work );
public static native int LAPACKE_strevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
int[] select, int n,
@Const float[] t, int ldt, float[] vl,
int ldvl, float[] vr, int ldvr,
int mm, int[] m, float[] work );
public static native int LAPACKE_dtrevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
IntPointer select, int n,
@Const DoublePointer t, int ldt, DoublePointer vl,
int ldvl, DoublePointer vr, int ldvr,
int mm, IntPointer m, DoublePointer work );
public static native int LAPACKE_dtrevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
IntBuffer select, int n,
@Const DoubleBuffer t, int ldt, DoubleBuffer vl,
int ldvl, DoubleBuffer vr, int ldvr,
int mm, IntBuffer m, DoubleBuffer work );
public static native int LAPACKE_dtrevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
int[] select, int n,
@Const double[] t, int ldt, double[] vl,
int ldvl, double[] vr, int ldvr,
int mm, int[] m, double[] work );
public static native int LAPACKE_ctrevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, int ldvr,
int mm, IntPointer m,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_ctrevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, int ldvr,
int mm, IntBuffer m,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_ctrevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] vl, int ldvl,
@Cast("lapack_complex_float*") float[] vr, int ldvr,
int mm, int[] m,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_ztrevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer vl, int ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, int ldvr,
int mm, IntPointer m,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_ztrevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer vl, int ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, int ldvr,
int mm, IntBuffer m,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_ztrevc_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] vl, int ldvl,
@Cast("lapack_complex_double*") double[] vr, int ldvr,
int mm, int[] m,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_strexc_work( int matrix_layout, @Cast("char") byte compq, int n,
FloatPointer t, int ldt, FloatPointer q,
int ldq, IntPointer ifst,
IntPointer ilst, FloatPointer work );
public static native int LAPACKE_strexc_work( int matrix_layout, @Cast("char") byte compq, int n,
FloatBuffer t, int ldt, FloatBuffer q,
int ldq, IntBuffer ifst,
IntBuffer ilst, FloatBuffer work );
public static native int LAPACKE_strexc_work( int matrix_layout, @Cast("char") byte compq, int n,
float[] t, int ldt, float[] q,
int ldq, int[] ifst,
int[] ilst, float[] work );
public static native int LAPACKE_dtrexc_work( int matrix_layout, @Cast("char") byte compq, int n,
DoublePointer t, int ldt, DoublePointer q,
int ldq, IntPointer ifst,
IntPointer ilst, DoublePointer work );
public static native int LAPACKE_dtrexc_work( int matrix_layout, @Cast("char") byte compq, int n,
DoubleBuffer t, int ldt, DoubleBuffer q,
int ldq, IntBuffer ifst,
IntBuffer ilst, DoubleBuffer work );
public static native int LAPACKE_dtrexc_work( int matrix_layout, @Cast("char") byte compq, int n,
double[] t, int ldt, double[] q,
int ldq, int[] ifst,
int[] ilst, double[] work );
public static native int LAPACKE_ctrexc_work( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ctrexc_work( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ctrexc_work( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ztrexc_work( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ztrexc_work( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_ztrexc_work( int matrix_layout, @Cast("char") byte compq, int n,
@Cast("lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] q, int ldq,
int ifst, int ilst );
public static native int LAPACKE_strrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const FloatPointer a, int lda, @Const FloatPointer b,
int ldb, @Const FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork );
public static native int LAPACKE_strrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const FloatBuffer a, int lda, @Const FloatBuffer b,
int ldb, @Const FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork );
public static native int LAPACKE_strrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const float[] a, int lda, @Const float[] b,
int ldb, @Const float[] x, int ldx,
float[] ferr, float[] berr, float[] work,
int[] iwork );
public static native int LAPACKE_dtrrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const DoublePointer a, int lda,
@Const DoublePointer b, int ldb,
@Const DoublePointer x, int ldx, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork );
public static native int LAPACKE_dtrrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const DoubleBuffer a, int lda,
@Const DoubleBuffer b, int ldb,
@Const DoubleBuffer x, int ldx, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork );
public static native int LAPACKE_dtrrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const double[] a, int lda,
@Const double[] b, int ldb,
@Const double[] x, int ldx, double[] ferr,
double[] berr, double[] work, int[] iwork );
public static native int LAPACKE_ctrrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("const lapack_complex_float*") FloatPointer x, int ldx,
FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork );
public static native int LAPACKE_ctrrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("const lapack_complex_float*") FloatBuffer x, int ldx,
FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork );
public static native int LAPACKE_ctrrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("const lapack_complex_float*") float[] x, int ldx,
float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork );
public static native int LAPACKE_ztrrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("const lapack_complex_double*") DoublePointer x, int ldx,
DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork );
public static native int LAPACKE_ztrrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("const lapack_complex_double*") DoubleBuffer x, int ldx,
DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork );
public static native int LAPACKE_ztrrfs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("const lapack_complex_double*") double[] x, int ldx,
double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork );
public static native int LAPACKE_strsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntPointer select, int n,
FloatPointer t, int ldt, FloatPointer q,
int ldq, FloatPointer wr, FloatPointer wi,
IntPointer m, FloatPointer s, FloatPointer sep,
FloatPointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_strsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntBuffer select, int n,
FloatBuffer t, int ldt, FloatBuffer q,
int ldq, FloatBuffer wr, FloatBuffer wi,
IntBuffer m, FloatBuffer s, FloatBuffer sep,
FloatBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_strsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const int[] select, int n,
float[] t, int ldt, float[] q,
int ldq, float[] wr, float[] wi,
int[] m, float[] s, float[] sep,
float[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_dtrsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntPointer select, int n,
DoublePointer t, int ldt, DoublePointer q,
int ldq, DoublePointer wr, DoublePointer wi,
IntPointer m, DoublePointer s, DoublePointer sep,
DoublePointer work, int lwork,
IntPointer iwork, int liwork );
public static native int LAPACKE_dtrsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntBuffer select, int n,
DoubleBuffer t, int ldt, DoubleBuffer q,
int ldq, DoubleBuffer wr, DoubleBuffer wi,
IntBuffer m, DoubleBuffer s, DoubleBuffer sep,
DoubleBuffer work, int lwork,
IntBuffer iwork, int liwork );
public static native int LAPACKE_dtrsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const int[] select, int n,
double[] t, int ldt, double[] q,
int ldq, double[] wr, double[] wi,
int[] m, double[] s, double[] sep,
double[] work, int lwork,
int[] iwork, int liwork );
public static native int LAPACKE_ctrsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntPointer select, int n,
@Cast("lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer w, IntPointer m,
FloatPointer s, FloatPointer sep,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_ctrsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntBuffer select, int n,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer w, IntBuffer m,
FloatBuffer s, FloatBuffer sep,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_ctrsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const int[] select, int n,
@Cast("lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] w, int[] m,
float[] s, float[] sep,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_ztrsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntPointer select, int n,
@Cast("lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer w, IntPointer m,
DoublePointer s, DoublePointer sep,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_ztrsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const IntBuffer select, int n,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer w, IntBuffer m,
DoubleBuffer s, DoubleBuffer sep,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_ztrsen_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte compq,
@Const int[] select, int n,
@Cast("lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] w, int[] m,
double[] s, double[] sep,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_strsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const FloatPointer t, int ldt, @Const FloatPointer vl,
int ldvl, @Const FloatPointer vr,
int ldvr, FloatPointer s, FloatPointer sep,
int mm, IntPointer m, FloatPointer work,
int ldwork, IntPointer iwork );
public static native int LAPACKE_strsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const FloatBuffer t, int ldt, @Const FloatBuffer vl,
int ldvl, @Const FloatBuffer vr,
int ldvr, FloatBuffer s, FloatBuffer sep,
int mm, IntBuffer m, FloatBuffer work,
int ldwork, IntBuffer iwork );
public static native int LAPACKE_strsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const float[] t, int ldt, @Const float[] vl,
int ldvl, @Const float[] vr,
int ldvr, float[] s, float[] sep,
int mm, int[] m, float[] work,
int ldwork, int[] iwork );
public static native int LAPACKE_dtrsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Const DoublePointer t, int ldt,
@Const DoublePointer vl, int ldvl,
@Const DoublePointer vr, int ldvr, DoublePointer s,
DoublePointer sep, int mm, IntPointer m,
DoublePointer work, int ldwork,
IntPointer iwork );
public static native int LAPACKE_dtrsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Const DoubleBuffer t, int ldt,
@Const DoubleBuffer vl, int ldvl,
@Const DoubleBuffer vr, int ldvr, DoubleBuffer s,
DoubleBuffer sep, int mm, IntBuffer m,
DoubleBuffer work, int ldwork,
IntBuffer iwork );
public static native int LAPACKE_dtrsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Const double[] t, int ldt,
@Const double[] vl, int ldvl,
@Const double[] vr, int ldvr, double[] s,
double[] sep, int mm, int[] m,
double[] work, int ldwork,
int[] iwork );
public static native int LAPACKE_ctrsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_float*") FloatPointer t, int ldt,
@Cast("const lapack_complex_float*") FloatPointer vl, int ldvl,
@Cast("const lapack_complex_float*") FloatPointer vr, int ldvr,
FloatPointer s, FloatPointer sep, int mm,
IntPointer m, @Cast("lapack_complex_float*") FloatPointer work,
int ldwork, FloatPointer rwork );
public static native int LAPACKE_ctrsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("const lapack_complex_float*") FloatBuffer vl, int ldvl,
@Cast("const lapack_complex_float*") FloatBuffer vr, int ldvr,
FloatBuffer s, FloatBuffer sep, int mm,
IntBuffer m, @Cast("lapack_complex_float*") FloatBuffer work,
int ldwork, FloatBuffer rwork );
public static native int LAPACKE_ctrsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_float*") float[] t, int ldt,
@Cast("const lapack_complex_float*") float[] vl, int ldvl,
@Cast("const lapack_complex_float*") float[] vr, int ldvr,
float[] s, float[] sep, int mm,
int[] m, @Cast("lapack_complex_float*") float[] work,
int ldwork, float[] rwork );
public static native int LAPACKE_ztrsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntPointer select, int n,
@Cast("const lapack_complex_double*") DoublePointer t, int ldt,
@Cast("const lapack_complex_double*") DoublePointer vl,
int ldvl,
@Cast("const lapack_complex_double*") DoublePointer vr,
int ldvr, DoublePointer s, DoublePointer sep,
int mm, IntPointer m,
@Cast("lapack_complex_double*") DoublePointer work, int ldwork,
DoublePointer rwork );
public static native int LAPACKE_ztrsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const IntBuffer select, int n,
@Cast("const lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("const lapack_complex_double*") DoubleBuffer vl,
int ldvl,
@Cast("const lapack_complex_double*") DoubleBuffer vr,
int ldvr, DoubleBuffer s, DoubleBuffer sep,
int mm, IntBuffer m,
@Cast("lapack_complex_double*") DoubleBuffer work, int ldwork,
DoubleBuffer rwork );
public static native int LAPACKE_ztrsna_work( int matrix_layout, @Cast("char") byte job, @Cast("char") byte howmny,
@Const int[] select, int n,
@Cast("const lapack_complex_double*") double[] t, int ldt,
@Cast("const lapack_complex_double*") double[] vl,
int ldvl,
@Cast("const lapack_complex_double*") double[] vr,
int ldvr, double[] s, double[] sep,
int mm, int[] m,
@Cast("lapack_complex_double*") double[] work, int ldwork,
double[] rwork );
public static native int LAPACKE_strsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const FloatPointer a, int lda, @Const FloatPointer b,
int ldb, FloatPointer c, int ldc,
FloatPointer scale );
public static native int LAPACKE_strsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const FloatBuffer a, int lda, @Const FloatBuffer b,
int ldb, FloatBuffer c, int ldc,
FloatBuffer scale );
public static native int LAPACKE_strsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const float[] a, int lda, @Const float[] b,
int ldb, float[] c, int ldc,
float[] scale );
public static native int LAPACKE_dtrsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const DoublePointer a, int lda,
@Const DoublePointer b, int ldb, DoublePointer c,
int ldc, DoublePointer scale );
public static native int LAPACKE_dtrsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const DoubleBuffer a, int lda,
@Const DoubleBuffer b, int ldb, DoubleBuffer c,
int ldc, DoubleBuffer scale );
public static native int LAPACKE_dtrsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Const double[] a, int lda,
@Const double[] b, int ldb, double[] c,
int ldc, double[] scale );
public static native int LAPACKE_ctrsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
FloatPointer scale );
public static native int LAPACKE_ctrsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
FloatBuffer scale );
public static native int LAPACKE_ctrsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] c, int ldc,
float[] scale );
public static native int LAPACKE_ztrsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
DoublePointer scale );
public static native int LAPACKE_ztrsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
DoubleBuffer scale );
public static native int LAPACKE_ztrsyl_work( int matrix_layout, @Cast("char") byte trana, @Cast("char") byte tranb,
int isgn, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] c, int ldc,
double[] scale );
public static native int LAPACKE_strtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, FloatPointer a, int lda );
public static native int LAPACKE_strtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, FloatBuffer a, int lda );
public static native int LAPACKE_strtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, float[] a, int lda );
public static native int LAPACKE_dtrtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, DoublePointer a, int lda );
public static native int LAPACKE_dtrtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, DoubleBuffer a, int lda );
public static native int LAPACKE_dtrtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, double[] a, int lda );
public static native int LAPACKE_ctrtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_ctrtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_ctrtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_ztrtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_ztrtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_ztrtri_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_strtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const FloatPointer a, int lda, FloatPointer b,
int ldb );
public static native int LAPACKE_strtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const FloatBuffer a, int lda, FloatBuffer b,
int ldb );
public static native int LAPACKE_strtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const float[] a, int lda, float[] b,
int ldb );
public static native int LAPACKE_dtrtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const DoublePointer a, int lda, DoublePointer b,
int ldb );
public static native int LAPACKE_dtrtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const DoubleBuffer a, int lda, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dtrtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Const double[] a, int lda, double[] b,
int ldb );
public static native int LAPACKE_ctrtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_ctrtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_ctrtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_ztrtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_ztrtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_ztrtrs_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte trans,
@Cast("char") byte diag, int n, int nrhs,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_strttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatPointer a, int lda,
FloatPointer arf );
public static native int LAPACKE_strttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatBuffer a, int lda,
FloatBuffer arf );
public static native int LAPACKE_strttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const float[] a, int lda,
float[] arf );
public static native int LAPACKE_dtrttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoublePointer a, int lda,
DoublePointer arf );
public static native int LAPACKE_dtrttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoubleBuffer a, int lda,
DoubleBuffer arf );
public static native int LAPACKE_dtrttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const double[] a, int lda,
double[] arf );
public static native int LAPACKE_ctrttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer arf );
public static native int LAPACKE_ctrttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer arf );
public static native int LAPACKE_ctrttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] arf );
public static native int LAPACKE_ztrttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer arf );
public static native int LAPACKE_ztrttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer arf );
public static native int LAPACKE_ztrttf_work( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] arf );
public static native int LAPACKE_strttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer a, int lda, FloatPointer ap );
public static native int LAPACKE_strttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer a, int lda, FloatBuffer ap );
public static native int LAPACKE_strttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] a, int lda, float[] ap );
public static native int LAPACKE_dtrttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer a, int lda, DoublePointer ap );
public static native int LAPACKE_dtrttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer a, int lda, DoubleBuffer ap );
public static native int LAPACKE_dtrttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] a, int lda, double[] ap );
public static native int LAPACKE_ctrttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_ctrttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_ctrttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] ap );
public static native int LAPACKE_ztrttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_ztrttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_ztrttp_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] ap );
public static native int LAPACKE_stzrzf_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer tau,
FloatPointer work, int lwork );
public static native int LAPACKE_stzrzf_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer tau,
FloatBuffer work, int lwork );
public static native int LAPACKE_stzrzf_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] tau,
float[] work, int lwork );
public static native int LAPACKE_dtzrzf_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer tau,
DoublePointer work, int lwork );
public static native int LAPACKE_dtzrzf_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer tau,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dtzrzf_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] tau,
double[] work, int lwork );
public static native int LAPACKE_ctzrzf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_ctzrzf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_ctzrzf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_ztzrzf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_ztzrzf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_ztzrzf_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cungbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cungbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cungbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zungbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zungbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zungbr_work( int matrix_layout, @Cast("char") byte vect, int m,
int n, int k,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunghr_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunghr_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunghr_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunghr_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoublePointer a,
int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunghr_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunghr_work( int matrix_layout, int n, int ilo,
int ihi, @Cast("lapack_complex_double*") double[] a,
int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunglq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunglq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunglq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunglq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoublePointer a,
int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunglq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunglq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") double[] a,
int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cungql_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cungql_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cungql_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zungql_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoublePointer a,
int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zungql_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zungql_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") double[] a,
int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cungqr_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cungqr_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cungqr_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zungqr_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoublePointer a,
int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zungqr_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zungqr_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") double[] a,
int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cungrq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cungrq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cungrq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zungrq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoublePointer a,
int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zungrq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zungrq_work( int matrix_layout, int m, int n,
int k, @Cast("lapack_complex_double*") double[] a,
int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cungtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cungtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cungtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zungtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zungtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zungtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunmbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunmbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunmbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunmbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Cast("const lapack_complex_double*") DoublePointer a,
int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunmbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunmbr_work( int matrix_layout, @Cast("char") byte vect, @Cast("char") byte side,
@Cast("char") byte trans, int m, int n,
int k, @Cast("const lapack_complex_double*") double[] a,
int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunmhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunmhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunmhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunmhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_double*") DoublePointer a,
int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunmhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunmhr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int ilo,
int ihi, @Cast("const lapack_complex_double*") double[] a,
int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunmlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunmlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunmlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunmlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunmlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunmlq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunmql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunmql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunmql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunmql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunmql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunmql_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunmqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunmqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunmqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunmqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunmqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunmqr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunmrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunmrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunmrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunmrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunmrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunmrq_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunmrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunmrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunmrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_float*") float[] a,
int lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunmrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_double*") DoublePointer a,
int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunmrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunmrz_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, @Cast("const lapack_complex_double*") double[] a,
int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cunmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer a, int lda,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer a, int lda,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_float*") float[] a, int lda,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_zunmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer a, int lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_double*") double[] a, int lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_cupgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer q, int ldq,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_cupgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer q, int ldq,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_cupgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] q, int ldq,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zupgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer q, int ldq,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zupgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer q, int ldq,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zupgtr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] q, int ldq,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_cupmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, int ldc,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_cupmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, int ldc,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_cupmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int ldc,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zupmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, int ldc,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zupmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, int ldc,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zupmtr_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte uplo,
@Cast("char") byte trans, int m, int n,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int ldc,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_claghe( int matrix_layout, int n, int k,
@Const FloatPointer d, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer iseed );
public static native int LAPACKE_claghe( int matrix_layout, int n, int k,
@Const FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer iseed );
public static native int LAPACKE_claghe( int matrix_layout, int n, int k,
@Const float[] d, @Cast("lapack_complex_float*") float[] a,
int lda, int[] iseed );
public static native int LAPACKE_zlaghe( int matrix_layout, int n, int k,
@Const DoublePointer d, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer iseed );
public static native int LAPACKE_zlaghe( int matrix_layout, int n, int k,
@Const DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer iseed );
public static native int LAPACKE_zlaghe( int matrix_layout, int n, int k,
@Const double[] d, @Cast("lapack_complex_double*") double[] a,
int lda, int[] iseed );
public static native int LAPACKE_slagsy( int matrix_layout, int n, int k,
@Const FloatPointer d, FloatPointer a, int lda,
IntPointer iseed );
public static native int LAPACKE_slagsy( int matrix_layout, int n, int k,
@Const FloatBuffer d, FloatBuffer a, int lda,
IntBuffer iseed );
public static native int LAPACKE_slagsy( int matrix_layout, int n, int k,
@Const float[] d, float[] a, int lda,
int[] iseed );
public static native int LAPACKE_dlagsy( int matrix_layout, int n, int k,
@Const DoublePointer d, DoublePointer a, int lda,
IntPointer iseed );
public static native int LAPACKE_dlagsy( int matrix_layout, int n, int k,
@Const DoubleBuffer d, DoubleBuffer a, int lda,
IntBuffer iseed );
public static native int LAPACKE_dlagsy( int matrix_layout, int n, int k,
@Const double[] d, double[] a, int lda,
int[] iseed );
public static native int LAPACKE_clagsy( int matrix_layout, int n, int k,
@Const FloatPointer d, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer iseed );
public static native int LAPACKE_clagsy( int matrix_layout, int n, int k,
@Const FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer iseed );
public static native int LAPACKE_clagsy( int matrix_layout, int n, int k,
@Const float[] d, @Cast("lapack_complex_float*") float[] a,
int lda, int[] iseed );
public static native int LAPACKE_zlagsy( int matrix_layout, int n, int k,
@Const DoublePointer d, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer iseed );
public static native int LAPACKE_zlagsy( int matrix_layout, int n, int k,
@Const DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer iseed );
public static native int LAPACKE_zlagsy( int matrix_layout, int n, int k,
@Const double[] d, @Cast("lapack_complex_double*") double[] a,
int lda, int[] iseed );
public static native int LAPACKE_slapmr( int matrix_layout, int forwrd,
int m, int n, FloatPointer x, int ldx,
IntPointer k );
public static native int LAPACKE_slapmr( int matrix_layout, int forwrd,
int m, int n, FloatBuffer x, int ldx,
IntBuffer k );
public static native int LAPACKE_slapmr( int matrix_layout, int forwrd,
int m, int n, float[] x, int ldx,
int[] k );
public static native int LAPACKE_dlapmr( int matrix_layout, int forwrd,
int m, int n, DoublePointer x,
int ldx, IntPointer k );
public static native int LAPACKE_dlapmr( int matrix_layout, int forwrd,
int m, int n, DoubleBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_dlapmr( int matrix_layout, int forwrd,
int m, int n, double[] x,
int ldx, int[] k );
public static native int LAPACKE_clapmr( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, IntPointer k );
public static native int LAPACKE_clapmr( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_clapmr( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_float*") float[] x,
int ldx, int[] k );
public static native int LAPACKE_zlapmr( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, IntPointer k );
public static native int LAPACKE_zlapmr( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_zlapmr( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_double*") double[] x,
int ldx, int[] k );
public static native int LAPACKE_slapmt( int matrix_layout, int forwrd,
int m, int n, FloatPointer x, int ldx,
IntPointer k );
public static native int LAPACKE_slapmt( int matrix_layout, int forwrd,
int m, int n, FloatBuffer x, int ldx,
IntBuffer k );
public static native int LAPACKE_slapmt( int matrix_layout, int forwrd,
int m, int n, float[] x, int ldx,
int[] k );
public static native int LAPACKE_dlapmt( int matrix_layout, int forwrd,
int m, int n, DoublePointer x,
int ldx, IntPointer k );
public static native int LAPACKE_dlapmt( int matrix_layout, int forwrd,
int m, int n, DoubleBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_dlapmt( int matrix_layout, int forwrd,
int m, int n, double[] x,
int ldx, int[] k );
public static native int LAPACKE_clapmt( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_float*") FloatPointer x,
int ldx, IntPointer k );
public static native int LAPACKE_clapmt( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_float*") FloatBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_clapmt( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_float*") float[] x,
int ldx, int[] k );
public static native int LAPACKE_zlapmt( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_double*") DoublePointer x,
int ldx, IntPointer k );
public static native int LAPACKE_zlapmt( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_double*") DoubleBuffer x,
int ldx, IntBuffer k );
public static native int LAPACKE_zlapmt( int matrix_layout, int forwrd,
int m, int n, @Cast("lapack_complex_double*") double[] x,
int ldx, int[] k );
public static native float LAPACKE_slapy2( float x, float y );
public static native double LAPACKE_dlapy2( double x, double y );
public static native float LAPACKE_slapy3( float x, float y, float z );
public static native double LAPACKE_dlapy3( double x, double y, double z );
public static native int LAPACKE_slartgp( float f, float g, FloatPointer cs, FloatPointer sn, FloatPointer r );
public static native int LAPACKE_slartgp( float f, float g, FloatBuffer cs, FloatBuffer sn, FloatBuffer r );
public static native int LAPACKE_slartgp( float f, float g, float[] cs, float[] sn, float[] r );
public static native int LAPACKE_dlartgp( double f, double g, DoublePointer cs, DoublePointer sn,
DoublePointer r );
public static native int LAPACKE_dlartgp( double f, double g, DoubleBuffer cs, DoubleBuffer sn,
DoubleBuffer r );
public static native int LAPACKE_dlartgp( double f, double g, double[] cs, double[] sn,
double[] r );
public static native int LAPACKE_slartgs( float x, float y, float sigma, FloatPointer cs,
FloatPointer sn );
public static native int LAPACKE_slartgs( float x, float y, float sigma, FloatBuffer cs,
FloatBuffer sn );
public static native int LAPACKE_slartgs( float x, float y, float sigma, float[] cs,
float[] sn );
public static native int LAPACKE_dlartgs( double x, double y, double sigma, DoublePointer cs,
DoublePointer sn );
public static native int LAPACKE_dlartgs( double x, double y, double sigma, DoubleBuffer cs,
DoubleBuffer sn );
public static native int LAPACKE_dlartgs( double x, double y, double sigma, double[] cs,
double[] sn );
//LAPACK 3.3.0
public static native int LAPACKE_cbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, FloatPointer theta, FloatPointer phi,
@Cast("lapack_complex_float*") FloatPointer u1, int ldu1,
@Cast("lapack_complex_float*") FloatPointer u2, int ldu2,
@Cast("lapack_complex_float*") FloatPointer v1t, int ldv1t,
@Cast("lapack_complex_float*") FloatPointer v2t, int ldv2t,
FloatPointer b11d, FloatPointer b11e, FloatPointer b12d, FloatPointer b12e,
FloatPointer b21d, FloatPointer b21e, FloatPointer b22d, FloatPointer b22e );
public static native int LAPACKE_cbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, FloatBuffer theta, FloatBuffer phi,
@Cast("lapack_complex_float*") FloatBuffer u1, int ldu1,
@Cast("lapack_complex_float*") FloatBuffer u2, int ldu2,
@Cast("lapack_complex_float*") FloatBuffer v1t, int ldv1t,
@Cast("lapack_complex_float*") FloatBuffer v2t, int ldv2t,
FloatBuffer b11d, FloatBuffer b11e, FloatBuffer b12d, FloatBuffer b12e,
FloatBuffer b21d, FloatBuffer b21e, FloatBuffer b22d, FloatBuffer b22e );
public static native int LAPACKE_cbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, float[] theta, float[] phi,
@Cast("lapack_complex_float*") float[] u1, int ldu1,
@Cast("lapack_complex_float*") float[] u2, int ldu2,
@Cast("lapack_complex_float*") float[] v1t, int ldv1t,
@Cast("lapack_complex_float*") float[] v2t, int ldv2t,
float[] b11d, float[] b11e, float[] b12d, float[] b12e,
float[] b21d, float[] b21e, float[] b22d, float[] b22e );
public static native int LAPACKE_cbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
FloatPointer theta, FloatPointer phi,
@Cast("lapack_complex_float*") FloatPointer u1, int ldu1,
@Cast("lapack_complex_float*") FloatPointer u2, int ldu2,
@Cast("lapack_complex_float*") FloatPointer v1t, int ldv1t,
@Cast("lapack_complex_float*") FloatPointer v2t, int ldv2t,
FloatPointer b11d, FloatPointer b11e, FloatPointer b12d,
FloatPointer b12e, FloatPointer b21d, FloatPointer b21e,
FloatPointer b22d, FloatPointer b22e, FloatPointer rwork,
int lrwork );
public static native int LAPACKE_cbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
FloatBuffer theta, FloatBuffer phi,
@Cast("lapack_complex_float*") FloatBuffer u1, int ldu1,
@Cast("lapack_complex_float*") FloatBuffer u2, int ldu2,
@Cast("lapack_complex_float*") FloatBuffer v1t, int ldv1t,
@Cast("lapack_complex_float*") FloatBuffer v2t, int ldv2t,
FloatBuffer b11d, FloatBuffer b11e, FloatBuffer b12d,
FloatBuffer b12e, FloatBuffer b21d, FloatBuffer b21e,
FloatBuffer b22d, FloatBuffer b22e, FloatBuffer rwork,
int lrwork );
public static native int LAPACKE_cbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
float[] theta, float[] phi,
@Cast("lapack_complex_float*") float[] u1, int ldu1,
@Cast("lapack_complex_float*") float[] u2, int ldu2,
@Cast("lapack_complex_float*") float[] v1t, int ldv1t,
@Cast("lapack_complex_float*") float[] v2t, int ldv2t,
float[] b11d, float[] b11e, float[] b12d,
float[] b12e, float[] b21d, float[] b21e,
float[] b22d, float[] b22e, float[] rwork,
int lrwork );
public static native int LAPACKE_cheswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int i1,
int i2 );
public static native int LAPACKE_cheswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int i1,
int i2 );
public static native int LAPACKE_cheswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int i1,
int i2 );
public static native int LAPACKE_cheswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int i1,
int i2 );
public static native int LAPACKE_cheswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int i1,
int i2 );
public static native int LAPACKE_cheswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int i1,
int i2 );
public static native int LAPACKE_chetri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_chetri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_chetri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_chetri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_chetri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_chetri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_chetri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv, int nb );
public static native int LAPACKE_chetri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv, int nb );
public static native int LAPACKE_chetri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv, int nb );
public static native int LAPACKE_chetri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, int nb );
public static native int LAPACKE_chetri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, int nb );
public static native int LAPACKE_chetri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int nb );
public static native int LAPACKE_chetrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_chetrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_chetrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_chetrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_chetrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_chetrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_csyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_csyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_csyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_csyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_csyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_csyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, @Cast("lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_csyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int i1,
int i2 );
public static native int LAPACKE_csyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int i1,
int i2 );
public static native int LAPACKE_csyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int i1,
int i2 );
public static native int LAPACKE_csyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int i1,
int i2 );
public static native int LAPACKE_csyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int i1,
int i2 );
public static native int LAPACKE_csyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int i1,
int i2 );
public static native int LAPACKE_csytri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_csytri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_csytri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_csytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_csytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_csytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_csytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv, int nb );
public static native int LAPACKE_csytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv, int nb );
public static native int LAPACKE_csytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv, int nb );
public static native int LAPACKE_csytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, int nb );
public static native int LAPACKE_csytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, int nb );
public static native int LAPACKE_csytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int nb );
public static native int LAPACKE_csytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_csytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_csytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_csytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_csytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_csytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_cunbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_float*") FloatPointer x11, int ldx11,
@Cast("lapack_complex_float*") FloatPointer x12, int ldx12,
@Cast("lapack_complex_float*") FloatPointer x21, int ldx21,
@Cast("lapack_complex_float*") FloatPointer x22, int ldx22,
FloatPointer theta, FloatPointer phi,
@Cast("lapack_complex_float*") FloatPointer taup1,
@Cast("lapack_complex_float*") FloatPointer taup2,
@Cast("lapack_complex_float*") FloatPointer tauq1,
@Cast("lapack_complex_float*") FloatPointer tauq2 );
public static native int LAPACKE_cunbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_float*") FloatBuffer x11, int ldx11,
@Cast("lapack_complex_float*") FloatBuffer x12, int ldx12,
@Cast("lapack_complex_float*") FloatBuffer x21, int ldx21,
@Cast("lapack_complex_float*") FloatBuffer x22, int ldx22,
FloatBuffer theta, FloatBuffer phi,
@Cast("lapack_complex_float*") FloatBuffer taup1,
@Cast("lapack_complex_float*") FloatBuffer taup2,
@Cast("lapack_complex_float*") FloatBuffer tauq1,
@Cast("lapack_complex_float*") FloatBuffer tauq2 );
public static native int LAPACKE_cunbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_float*") float[] x11, int ldx11,
@Cast("lapack_complex_float*") float[] x12, int ldx12,
@Cast("lapack_complex_float*") float[] x21, int ldx21,
@Cast("lapack_complex_float*") float[] x22, int ldx22,
float[] theta, float[] phi,
@Cast("lapack_complex_float*") float[] taup1,
@Cast("lapack_complex_float*") float[] taup2,
@Cast("lapack_complex_float*") float[] tauq1,
@Cast("lapack_complex_float*") float[] tauq2 );
public static native int LAPACKE_cunbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_float*") FloatPointer x11, int ldx11,
@Cast("lapack_complex_float*") FloatPointer x12, int ldx12,
@Cast("lapack_complex_float*") FloatPointer x21, int ldx21,
@Cast("lapack_complex_float*") FloatPointer x22, int ldx22,
FloatPointer theta, FloatPointer phi,
@Cast("lapack_complex_float*") FloatPointer taup1,
@Cast("lapack_complex_float*") FloatPointer taup2,
@Cast("lapack_complex_float*") FloatPointer tauq1,
@Cast("lapack_complex_float*") FloatPointer tauq2,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_cunbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_float*") FloatBuffer x11, int ldx11,
@Cast("lapack_complex_float*") FloatBuffer x12, int ldx12,
@Cast("lapack_complex_float*") FloatBuffer x21, int ldx21,
@Cast("lapack_complex_float*") FloatBuffer x22, int ldx22,
FloatBuffer theta, FloatBuffer phi,
@Cast("lapack_complex_float*") FloatBuffer taup1,
@Cast("lapack_complex_float*") FloatBuffer taup2,
@Cast("lapack_complex_float*") FloatBuffer tauq1,
@Cast("lapack_complex_float*") FloatBuffer tauq2,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_cunbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_float*") float[] x11, int ldx11,
@Cast("lapack_complex_float*") float[] x12, int ldx12,
@Cast("lapack_complex_float*") float[] x21, int ldx21,
@Cast("lapack_complex_float*") float[] x22, int ldx22,
float[] theta, float[] phi,
@Cast("lapack_complex_float*") float[] taup1,
@Cast("lapack_complex_float*") float[] taup2,
@Cast("lapack_complex_float*") float[] tauq1,
@Cast("lapack_complex_float*") float[] tauq2,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_cuncsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_float*") FloatPointer x11, int ldx11,
@Cast("lapack_complex_float*") FloatPointer x12, int ldx12,
@Cast("lapack_complex_float*") FloatPointer x21, int ldx21,
@Cast("lapack_complex_float*") FloatPointer x22, int ldx22,
FloatPointer theta, @Cast("lapack_complex_float*") FloatPointer u1,
int ldu1, @Cast("lapack_complex_float*") FloatPointer u2,
int ldu2, @Cast("lapack_complex_float*") FloatPointer v1t,
int ldv1t, @Cast("lapack_complex_float*") FloatPointer v2t,
int ldv2t );
public static native int LAPACKE_cuncsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_float*") FloatBuffer x11, int ldx11,
@Cast("lapack_complex_float*") FloatBuffer x12, int ldx12,
@Cast("lapack_complex_float*") FloatBuffer x21, int ldx21,
@Cast("lapack_complex_float*") FloatBuffer x22, int ldx22,
FloatBuffer theta, @Cast("lapack_complex_float*") FloatBuffer u1,
int ldu1, @Cast("lapack_complex_float*") FloatBuffer u2,
int ldu2, @Cast("lapack_complex_float*") FloatBuffer v1t,
int ldv1t, @Cast("lapack_complex_float*") FloatBuffer v2t,
int ldv2t );
public static native int LAPACKE_cuncsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_float*") float[] x11, int ldx11,
@Cast("lapack_complex_float*") float[] x12, int ldx12,
@Cast("lapack_complex_float*") float[] x21, int ldx21,
@Cast("lapack_complex_float*") float[] x22, int ldx22,
float[] theta, @Cast("lapack_complex_float*") float[] u1,
int ldu1, @Cast("lapack_complex_float*") float[] u2,
int ldu2, @Cast("lapack_complex_float*") float[] v1t,
int ldv1t, @Cast("lapack_complex_float*") float[] v2t,
int ldv2t );
public static native int LAPACKE_cuncsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, @Cast("lapack_complex_float*") FloatPointer x11,
int ldx11, @Cast("lapack_complex_float*") FloatPointer x12,
int ldx12, @Cast("lapack_complex_float*") FloatPointer x21,
int ldx21, @Cast("lapack_complex_float*") FloatPointer x22,
int ldx22, FloatPointer theta,
@Cast("lapack_complex_float*") FloatPointer u1, int ldu1,
@Cast("lapack_complex_float*") FloatPointer u2, int ldu2,
@Cast("lapack_complex_float*") FloatPointer v1t, int ldv1t,
@Cast("lapack_complex_float*") FloatPointer v2t, int ldv2t,
@Cast("lapack_complex_float*") FloatPointer work, int lwork,
FloatPointer rwork, int lrwork,
IntPointer iwork );
public static native int LAPACKE_cuncsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, @Cast("lapack_complex_float*") FloatBuffer x11,
int ldx11, @Cast("lapack_complex_float*") FloatBuffer x12,
int ldx12, @Cast("lapack_complex_float*") FloatBuffer x21,
int ldx21, @Cast("lapack_complex_float*") FloatBuffer x22,
int ldx22, FloatBuffer theta,
@Cast("lapack_complex_float*") FloatBuffer u1, int ldu1,
@Cast("lapack_complex_float*") FloatBuffer u2, int ldu2,
@Cast("lapack_complex_float*") FloatBuffer v1t, int ldv1t,
@Cast("lapack_complex_float*") FloatBuffer v2t, int ldv2t,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork,
FloatBuffer rwork, int lrwork,
IntBuffer iwork );
public static native int LAPACKE_cuncsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, @Cast("lapack_complex_float*") float[] x11,
int ldx11, @Cast("lapack_complex_float*") float[] x12,
int ldx12, @Cast("lapack_complex_float*") float[] x21,
int ldx21, @Cast("lapack_complex_float*") float[] x22,
int ldx22, float[] theta,
@Cast("lapack_complex_float*") float[] u1, int ldu1,
@Cast("lapack_complex_float*") float[] u2, int ldu2,
@Cast("lapack_complex_float*") float[] v1t, int ldv1t,
@Cast("lapack_complex_float*") float[] v2t, int ldv2t,
@Cast("lapack_complex_float*") float[] work, int lwork,
float[] rwork, int lrwork,
int[] iwork );
public static native int LAPACKE_cuncsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
@Cast("lapack_complex_float*") FloatPointer x11, int ldx11,
@Cast("lapack_complex_float*") FloatPointer x21, int ldx21,
@Cast("lapack_complex_float*") FloatPointer theta, @Cast("lapack_complex_float*") FloatPointer u1,
int ldu1, @Cast("lapack_complex_float*") FloatPointer u2,
int ldu2, @Cast("lapack_complex_float*") FloatPointer v1t, int ldv1t );
public static native int LAPACKE_cuncsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
@Cast("lapack_complex_float*") FloatBuffer x11, int ldx11,
@Cast("lapack_complex_float*") FloatBuffer x21, int ldx21,
@Cast("lapack_complex_float*") FloatBuffer theta, @Cast("lapack_complex_float*") FloatBuffer u1,
int ldu1, @Cast("lapack_complex_float*") FloatBuffer u2,
int ldu2, @Cast("lapack_complex_float*") FloatBuffer v1t, int ldv1t );
public static native int LAPACKE_cuncsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
@Cast("lapack_complex_float*") float[] x11, int ldx11,
@Cast("lapack_complex_float*") float[] x21, int ldx21,
@Cast("lapack_complex_float*") float[] theta, @Cast("lapack_complex_float*") float[] u1,
int ldu1, @Cast("lapack_complex_float*") float[] u2,
int ldu2, @Cast("lapack_complex_float*") float[] v1t, int ldv1t );
public static native int LAPACKE_cuncsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p,
int q, @Cast("lapack_complex_float*") FloatPointer x11, int ldx11,
@Cast("lapack_complex_float*") FloatPointer x21, int ldx21,
@Cast("lapack_complex_float*") FloatPointer theta, @Cast("lapack_complex_float*") FloatPointer u1,
int ldu1, @Cast("lapack_complex_float*") FloatPointer u2,
int ldu2, @Cast("lapack_complex_float*") FloatPointer v1t,
int ldv1t, @Cast("lapack_complex_float*") FloatPointer work,
int lwork, FloatPointer rwork, int lrwork,
IntPointer iwork );
public static native int LAPACKE_cuncsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p,
int q, @Cast("lapack_complex_float*") FloatBuffer x11, int ldx11,
@Cast("lapack_complex_float*") FloatBuffer x21, int ldx21,
@Cast("lapack_complex_float*") FloatBuffer theta, @Cast("lapack_complex_float*") FloatBuffer u1,
int ldu1, @Cast("lapack_complex_float*") FloatBuffer u2,
int ldu2, @Cast("lapack_complex_float*") FloatBuffer v1t,
int ldv1t, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork, FloatBuffer rwork, int lrwork,
IntBuffer iwork );
public static native int LAPACKE_cuncsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p,
int q, @Cast("lapack_complex_float*") float[] x11, int ldx11,
@Cast("lapack_complex_float*") float[] x21, int ldx21,
@Cast("lapack_complex_float*") float[] theta, @Cast("lapack_complex_float*") float[] u1,
int ldu1, @Cast("lapack_complex_float*") float[] u2,
int ldu2, @Cast("lapack_complex_float*") float[] v1t,
int ldv1t, @Cast("lapack_complex_float*") float[] work,
int lwork, float[] rwork, int lrwork,
int[] iwork );
public static native int LAPACKE_dbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, DoublePointer theta,
DoublePointer phi, DoublePointer u1, int ldu1, DoublePointer u2,
int ldu2, DoublePointer v1t, int ldv1t,
DoublePointer v2t, int ldv2t, DoublePointer b11d,
DoublePointer b11e, DoublePointer b12d, DoublePointer b12e,
DoublePointer b21d, DoublePointer b21e, DoublePointer b22d,
DoublePointer b22e );
public static native int LAPACKE_dbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, DoubleBuffer theta,
DoubleBuffer phi, DoubleBuffer u1, int ldu1, DoubleBuffer u2,
int ldu2, DoubleBuffer v1t, int ldv1t,
DoubleBuffer v2t, int ldv2t, DoubleBuffer b11d,
DoubleBuffer b11e, DoubleBuffer b12d, DoubleBuffer b12e,
DoubleBuffer b21d, DoubleBuffer b21e, DoubleBuffer b22d,
DoubleBuffer b22e );
public static native int LAPACKE_dbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, double[] theta,
double[] phi, double[] u1, int ldu1, double[] u2,
int ldu2, double[] v1t, int ldv1t,
double[] v2t, int ldv2t, double[] b11d,
double[] b11e, double[] b12d, double[] b12e,
double[] b21d, double[] b21e, double[] b22d,
double[] b22e );
public static native int LAPACKE_dbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
DoublePointer theta, DoublePointer phi, DoublePointer u1,
int ldu1, DoublePointer u2, int ldu2,
DoublePointer v1t, int ldv1t, DoublePointer v2t,
int ldv2t, DoublePointer b11d, DoublePointer b11e,
DoublePointer b12d, DoublePointer b12e, DoublePointer b21d,
DoublePointer b21e, DoublePointer b22d, DoublePointer b22e,
DoublePointer work, int lwork );
public static native int LAPACKE_dbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
DoubleBuffer theta, DoubleBuffer phi, DoubleBuffer u1,
int ldu1, DoubleBuffer u2, int ldu2,
DoubleBuffer v1t, int ldv1t, DoubleBuffer v2t,
int ldv2t, DoubleBuffer b11d, DoubleBuffer b11e,
DoubleBuffer b12d, DoubleBuffer b12e, DoubleBuffer b21d,
DoubleBuffer b21e, DoubleBuffer b22d, DoubleBuffer b22e,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
double[] theta, double[] phi, double[] u1,
int ldu1, double[] u2, int ldu2,
double[] v1t, int ldv1t, double[] v2t,
int ldv2t, double[] b11d, double[] b11e,
double[] b12d, double[] b12e, double[] b21d,
double[] b21e, double[] b22d, double[] b22e,
double[] work, int lwork );
public static native int LAPACKE_dorbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
DoublePointer x11, int ldx11, DoublePointer x12,
int ldx12, DoublePointer x21, int ldx21,
DoublePointer x22, int ldx22, DoublePointer theta,
DoublePointer phi, DoublePointer taup1, DoublePointer taup2,
DoublePointer tauq1, DoublePointer tauq2 );
public static native int LAPACKE_dorbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
DoubleBuffer x11, int ldx11, DoubleBuffer x12,
int ldx12, DoubleBuffer x21, int ldx21,
DoubleBuffer x22, int ldx22, DoubleBuffer theta,
DoubleBuffer phi, DoubleBuffer taup1, DoubleBuffer taup2,
DoubleBuffer tauq1, DoubleBuffer tauq2 );
public static native int LAPACKE_dorbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
double[] x11, int ldx11, double[] x12,
int ldx12, double[] x21, int ldx21,
double[] x22, int ldx22, double[] theta,
double[] phi, double[] taup1, double[] taup2,
double[] tauq1, double[] tauq2 );
public static native int LAPACKE_dorbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
DoublePointer x11, int ldx11, DoublePointer x12,
int ldx12, DoublePointer x21, int ldx21,
DoublePointer x22, int ldx22, DoublePointer theta,
DoublePointer phi, DoublePointer taup1, DoublePointer taup2,
DoublePointer tauq1, DoublePointer tauq2, DoublePointer work,
int lwork );
public static native int LAPACKE_dorbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
DoubleBuffer x11, int ldx11, DoubleBuffer x12,
int ldx12, DoubleBuffer x21, int ldx21,
DoubleBuffer x22, int ldx22, DoubleBuffer theta,
DoubleBuffer phi, DoubleBuffer taup1, DoubleBuffer taup2,
DoubleBuffer tauq1, DoubleBuffer tauq2, DoubleBuffer work,
int lwork );
public static native int LAPACKE_dorbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
double[] x11, int ldx11, double[] x12,
int ldx12, double[] x21, int ldx21,
double[] x22, int ldx22, double[] theta,
double[] phi, double[] taup1, double[] taup2,
double[] tauq1, double[] tauq2, double[] work,
int lwork );
public static native int LAPACKE_dorcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
DoublePointer x11, int ldx11, DoublePointer x12,
int ldx12, DoublePointer x21, int ldx21,
DoublePointer x22, int ldx22, DoublePointer theta,
DoublePointer u1, int ldu1, DoublePointer u2,
int ldu2, DoublePointer v1t, int ldv1t,
DoublePointer v2t, int ldv2t );
public static native int LAPACKE_dorcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
DoubleBuffer x11, int ldx11, DoubleBuffer x12,
int ldx12, DoubleBuffer x21, int ldx21,
DoubleBuffer x22, int ldx22, DoubleBuffer theta,
DoubleBuffer u1, int ldu1, DoubleBuffer u2,
int ldu2, DoubleBuffer v1t, int ldv1t,
DoubleBuffer v2t, int ldv2t );
public static native int LAPACKE_dorcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
double[] x11, int ldx11, double[] x12,
int ldx12, double[] x21, int ldx21,
double[] x22, int ldx22, double[] theta,
double[] u1, int ldu1, double[] u2,
int ldu2, double[] v1t, int ldv1t,
double[] v2t, int ldv2t );
public static native int LAPACKE_dorcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, DoublePointer x11, int ldx11,
DoublePointer x12, int ldx12, DoublePointer x21,
int ldx21, DoublePointer x22, int ldx22,
DoublePointer theta, DoublePointer u1, int ldu1,
DoublePointer u2, int ldu2, DoublePointer v1t,
int ldv1t, DoublePointer v2t, int ldv2t,
DoublePointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_dorcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, DoubleBuffer x11, int ldx11,
DoubleBuffer x12, int ldx12, DoubleBuffer x21,
int ldx21, DoubleBuffer x22, int ldx22,
DoubleBuffer theta, DoubleBuffer u1, int ldu1,
DoubleBuffer u2, int ldu2, DoubleBuffer v1t,
int ldv1t, DoubleBuffer v2t, int ldv2t,
DoubleBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_dorcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, double[] x11, int ldx11,
double[] x12, int ldx12, double[] x21,
int ldx21, double[] x22, int ldx22,
double[] theta, double[] u1, int ldu1,
double[] u2, int ldu2, double[] v1t,
int ldv1t, double[] v2t, int ldv2t,
double[] work, int lwork,
int[] iwork );
public static native int LAPACKE_dorcsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
DoublePointer x11, int ldx11, DoublePointer x21, int ldx21,
DoublePointer theta, DoublePointer u1, int ldu1, DoublePointer u2,
int ldu2, DoublePointer v1t, int ldv1t);
public static native int LAPACKE_dorcsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
DoubleBuffer x11, int ldx11, DoubleBuffer x21, int ldx21,
DoubleBuffer theta, DoubleBuffer u1, int ldu1, DoubleBuffer u2,
int ldu2, DoubleBuffer v1t, int ldv1t);
public static native int LAPACKE_dorcsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
double[] x11, int ldx11, double[] x21, int ldx21,
double[] theta, double[] u1, int ldu1, double[] u2,
int ldu2, double[] v1t, int ldv1t);
public static native int LAPACKE_dorcsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
DoublePointer x11, int ldx11, DoublePointer x21, int ldx21,
DoublePointer theta, DoublePointer u1, int ldu1, DoublePointer u2,
int ldu2, DoublePointer v1t, int ldv1t,
DoublePointer work, int lwork, IntPointer iwork );
public static native int LAPACKE_dorcsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
DoubleBuffer x11, int ldx11, DoubleBuffer x21, int ldx21,
DoubleBuffer theta, DoubleBuffer u1, int ldu1, DoubleBuffer u2,
int ldu2, DoubleBuffer v1t, int ldv1t,
DoubleBuffer work, int lwork, IntBuffer iwork );
public static native int LAPACKE_dorcsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
double[] x11, int ldx11, double[] x21, int ldx21,
double[] theta, double[] u1, int ldu1, double[] u2,
int ldu2, double[] v1t, int ldv1t,
double[] work, int lwork, int[] iwork );
public static native int LAPACKE_dsyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
DoublePointer a, int lda, @Const IntPointer ipiv, DoublePointer work);
public static native int LAPACKE_dsyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
DoubleBuffer a, int lda, @Const IntBuffer ipiv, DoubleBuffer work);
public static native int LAPACKE_dsyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
double[] a, int lda, @Const int[] ipiv, double[] work);
public static native int LAPACKE_dsyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, DoublePointer a, int lda,
@Const IntPointer ipiv, DoublePointer work );
public static native int LAPACKE_dsyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, DoubleBuffer a, int lda,
@Const IntBuffer ipiv, DoubleBuffer work );
public static native int LAPACKE_dsyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, double[] a, int lda,
@Const int[] ipiv, double[] work );
public static native int LAPACKE_dsyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int i1, int i2 );
public static native int LAPACKE_dsyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int i1, int i2 );
public static native int LAPACKE_dsyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int i1, int i2 );
public static native int LAPACKE_dsyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int i1, int i2 );
public static native int LAPACKE_dsyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int i1, int i2 );
public static native int LAPACKE_dsyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int i1, int i2 );
public static native int LAPACKE_dsytri2( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda, @Const IntPointer ipiv );
public static native int LAPACKE_dsytri2( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda, @Const IntBuffer ipiv );
public static native int LAPACKE_dsytri2( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda, @Const int[] ipiv );
public static native int LAPACKE_dsytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_dsytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_dsytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_dsytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda, @Const IntPointer ipiv,
int nb );
public static native int LAPACKE_dsytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda, @Const IntBuffer ipiv,
int nb );
public static native int LAPACKE_dsytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda, @Const int[] ipiv,
int nb );
public static native int LAPACKE_dsytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda,
@Const IntPointer ipiv, DoublePointer work,
int nb );
public static native int LAPACKE_dsytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda,
@Const IntBuffer ipiv, DoubleBuffer work,
int nb );
public static native int LAPACKE_dsytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda,
@Const int[] ipiv, double[] work,
int nb );
public static native int LAPACKE_dsytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a, int lda,
@Const IntPointer ipiv, DoublePointer b, int ldb );
public static native int LAPACKE_dsytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a, int lda,
@Const IntBuffer ipiv, DoubleBuffer b, int ldb );
public static native int LAPACKE_dsytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a, int lda,
@Const int[] ipiv, double[] b, int ldb );
public static native int LAPACKE_dsytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a,
int lda, @Const IntPointer ipiv,
DoublePointer b, int ldb, DoublePointer work );
public static native int LAPACKE_dsytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
DoubleBuffer b, int ldb, DoubleBuffer work );
public static native int LAPACKE_dsytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a,
int lda, @Const int[] ipiv,
double[] b, int ldb, double[] work );
public static native int LAPACKE_sbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, FloatPointer theta, FloatPointer phi,
FloatPointer u1, int ldu1, FloatPointer u2,
int ldu2, FloatPointer v1t, int ldv1t,
FloatPointer v2t, int ldv2t, FloatPointer b11d,
FloatPointer b11e, FloatPointer b12d, FloatPointer b12e, FloatPointer b21d,
FloatPointer b21e, FloatPointer b22d, FloatPointer b22e );
public static native int LAPACKE_sbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, FloatBuffer theta, FloatBuffer phi,
FloatBuffer u1, int ldu1, FloatBuffer u2,
int ldu2, FloatBuffer v1t, int ldv1t,
FloatBuffer v2t, int ldv2t, FloatBuffer b11d,
FloatBuffer b11e, FloatBuffer b12d, FloatBuffer b12e, FloatBuffer b21d,
FloatBuffer b21e, FloatBuffer b22d, FloatBuffer b22e );
public static native int LAPACKE_sbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, float[] theta, float[] phi,
float[] u1, int ldu1, float[] u2,
int ldu2, float[] v1t, int ldv1t,
float[] v2t, int ldv2t, float[] b11d,
float[] b11e, float[] b12d, float[] b12e, float[] b21d,
float[] b21e, float[] b22d, float[] b22e );
public static native int LAPACKE_sbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
FloatPointer theta, FloatPointer phi, FloatPointer u1,
int ldu1, FloatPointer u2, int ldu2,
FloatPointer v1t, int ldv1t, FloatPointer v2t,
int ldv2t, FloatPointer b11d, FloatPointer b11e,
FloatPointer b12d, FloatPointer b12e, FloatPointer b21d,
FloatPointer b21e, FloatPointer b22d, FloatPointer b22e,
FloatPointer work, int lwork );
public static native int LAPACKE_sbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
FloatBuffer theta, FloatBuffer phi, FloatBuffer u1,
int ldu1, FloatBuffer u2, int ldu2,
FloatBuffer v1t, int ldv1t, FloatBuffer v2t,
int ldv2t, FloatBuffer b11d, FloatBuffer b11e,
FloatBuffer b12d, FloatBuffer b12e, FloatBuffer b21d,
FloatBuffer b21e, FloatBuffer b22d, FloatBuffer b22e,
FloatBuffer work, int lwork );
public static native int LAPACKE_sbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
float[] theta, float[] phi, float[] u1,
int ldu1, float[] u2, int ldu2,
float[] v1t, int ldv1t, float[] v2t,
int ldv2t, float[] b11d, float[] b11e,
float[] b12d, float[] b12e, float[] b21d,
float[] b21e, float[] b22d, float[] b22e,
float[] work, int lwork );
public static native int LAPACKE_sorbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q, FloatPointer x11,
int ldx11, FloatPointer x12, int ldx12,
FloatPointer x21, int ldx21, FloatPointer x22,
int ldx22, FloatPointer theta, FloatPointer phi,
FloatPointer taup1, FloatPointer taup2, FloatPointer tauq1,
FloatPointer tauq2 );
public static native int LAPACKE_sorbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q, FloatBuffer x11,
int ldx11, FloatBuffer x12, int ldx12,
FloatBuffer x21, int ldx21, FloatBuffer x22,
int ldx22, FloatBuffer theta, FloatBuffer phi,
FloatBuffer taup1, FloatBuffer taup2, FloatBuffer tauq1,
FloatBuffer tauq2 );
public static native int LAPACKE_sorbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q, float[] x11,
int ldx11, float[] x12, int ldx12,
float[] x21, int ldx21, float[] x22,
int ldx22, float[] theta, float[] phi,
float[] taup1, float[] taup2, float[] tauq1,
float[] tauq2 );
public static native int LAPACKE_sorbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
FloatPointer x11, int ldx11, FloatPointer x12,
int ldx12, FloatPointer x21, int ldx21,
FloatPointer x22, int ldx22, FloatPointer theta,
FloatPointer phi, FloatPointer taup1, FloatPointer taup2,
FloatPointer tauq1, FloatPointer tauq2, FloatPointer work,
int lwork );
public static native int LAPACKE_sorbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
FloatBuffer x11, int ldx11, FloatBuffer x12,
int ldx12, FloatBuffer x21, int ldx21,
FloatBuffer x22, int ldx22, FloatBuffer theta,
FloatBuffer phi, FloatBuffer taup1, FloatBuffer taup2,
FloatBuffer tauq1, FloatBuffer tauq2, FloatBuffer work,
int lwork );
public static native int LAPACKE_sorbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
float[] x11, int ldx11, float[] x12,
int ldx12, float[] x21, int ldx21,
float[] x22, int ldx22, float[] theta,
float[] phi, float[] taup1, float[] taup2,
float[] tauq1, float[] tauq2, float[] work,
int lwork );
public static native int LAPACKE_sorcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q, FloatPointer x11,
int ldx11, FloatPointer x12, int ldx12,
FloatPointer x21, int ldx21, FloatPointer x22,
int ldx22, FloatPointer theta, FloatPointer u1,
int ldu1, FloatPointer u2, int ldu2,
FloatPointer v1t, int ldv1t, FloatPointer v2t,
int ldv2t );
public static native int LAPACKE_sorcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q, FloatBuffer x11,
int ldx11, FloatBuffer x12, int ldx12,
FloatBuffer x21, int ldx21, FloatBuffer x22,
int ldx22, FloatBuffer theta, FloatBuffer u1,
int ldu1, FloatBuffer u2, int ldu2,
FloatBuffer v1t, int ldv1t, FloatBuffer v2t,
int ldv2t );
public static native int LAPACKE_sorcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q, float[] x11,
int ldx11, float[] x12, int ldx12,
float[] x21, int ldx21, float[] x22,
int ldx22, float[] theta, float[] u1,
int ldu1, float[] u2, int ldu2,
float[] v1t, int ldv1t, float[] v2t,
int ldv2t );
public static native int LAPACKE_sorcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, FloatPointer x11, int ldx11,
FloatPointer x12, int ldx12, FloatPointer x21,
int ldx21, FloatPointer x22, int ldx22,
FloatPointer theta, FloatPointer u1, int ldu1,
FloatPointer u2, int ldu2, FloatPointer v1t,
int ldv1t, FloatPointer v2t, int ldv2t,
FloatPointer work, int lwork,
IntPointer iwork );
public static native int LAPACKE_sorcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, FloatBuffer x11, int ldx11,
FloatBuffer x12, int ldx12, FloatBuffer x21,
int ldx21, FloatBuffer x22, int ldx22,
FloatBuffer theta, FloatBuffer u1, int ldu1,
FloatBuffer u2, int ldu2, FloatBuffer v1t,
int ldv1t, FloatBuffer v2t, int ldv2t,
FloatBuffer work, int lwork,
IntBuffer iwork );
public static native int LAPACKE_sorcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, float[] x11, int ldx11,
float[] x12, int ldx12, float[] x21,
int ldx21, float[] x22, int ldx22,
float[] theta, float[] u1, int ldu1,
float[] u2, int ldu2, float[] v1t,
int ldv1t, float[] v2t, int ldv2t,
float[] work, int lwork,
int[] iwork );
public static native int LAPACKE_sorcsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
FloatPointer x11, int ldx11, FloatPointer x21, int ldx21,
FloatPointer theta, FloatPointer u1, int ldu1, FloatPointer u2,
int ldu2, FloatPointer v1t, int ldv1t);
public static native int LAPACKE_sorcsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
FloatBuffer x11, int ldx11, FloatBuffer x21, int ldx21,
FloatBuffer theta, FloatBuffer u1, int ldu1, FloatBuffer u2,
int ldu2, FloatBuffer v1t, int ldv1t);
public static native int LAPACKE_sorcsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
float[] x11, int ldx11, float[] x21, int ldx21,
float[] theta, float[] u1, int ldu1, float[] u2,
int ldu2, float[] v1t, int ldv1t);
public static native int LAPACKE_sorcsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
FloatPointer x11, int ldx11, FloatPointer x21, int ldx21,
FloatPointer theta, FloatPointer u1, int ldu1, FloatPointer u2,
int ldu2, FloatPointer v1t, int ldv1t,
FloatPointer work, int lwork, IntPointer iwork );
public static native int LAPACKE_sorcsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
FloatBuffer x11, int ldx11, FloatBuffer x21, int ldx21,
FloatBuffer theta, FloatBuffer u1, int ldu1, FloatBuffer u2,
int ldu2, FloatBuffer v1t, int ldv1t,
FloatBuffer work, int lwork, IntBuffer iwork );
public static native int LAPACKE_sorcsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
float[] x11, int ldx11, float[] x21, int ldx21,
float[] theta, float[] u1, int ldu1, float[] u2,
int ldu2, float[] v1t, int ldv1t,
float[] work, int lwork, int[] iwork );
public static native int LAPACKE_ssyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
FloatPointer a, int lda, @Const IntPointer ipiv, FloatPointer work );
public static native int LAPACKE_ssyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
FloatBuffer a, int lda, @Const IntBuffer ipiv, FloatBuffer work );
public static native int LAPACKE_ssyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
float[] a, int lda, @Const int[] ipiv, float[] work );
public static native int LAPACKE_ssyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer work );
public static native int LAPACKE_ssyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer work );
public static native int LAPACKE_ssyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, float[] a, int lda,
@Const int[] ipiv, float[] work );
public static native int LAPACKE_ssyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int i1, int i2 );
public static native int LAPACKE_ssyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int i1, int i2 );
public static native int LAPACKE_ssyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int i1, int i2 );
public static native int LAPACKE_ssyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int i1, int i2 );
public static native int LAPACKE_ssyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int i1, int i2 );
public static native int LAPACKE_ssyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int i1, int i2 );
public static native int LAPACKE_ssytri2( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda, @Const IntPointer ipiv );
public static native int LAPACKE_ssytri2( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda, @Const IntBuffer ipiv );
public static native int LAPACKE_ssytri2( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda, @Const int[] ipiv );
public static native int LAPACKE_ssytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, int lwork );
public static native int LAPACKE_ssytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, int lwork );
public static native int LAPACKE_ssytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int lwork );
public static native int LAPACKE_ssytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda, @Const IntPointer ipiv,
int nb );
public static native int LAPACKE_ssytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda, @Const IntBuffer ipiv,
int nb );
public static native int LAPACKE_ssytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda, @Const int[] ipiv,
int nb );
public static native int LAPACKE_ssytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer work,
int nb );
public static native int LAPACKE_ssytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer work,
int nb );
public static native int LAPACKE_ssytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda,
@Const int[] ipiv, float[] work,
int nb );
public static native int LAPACKE_ssytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer b, int ldb );
public static native int LAPACKE_ssytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer b, int ldb );
public static native int LAPACKE_ssytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
@Const int[] ipiv, float[] b, int ldb );
public static native int LAPACKE_ssytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a,
int lda, @Const IntPointer ipiv,
FloatPointer b, int ldb, FloatPointer work );
public static native int LAPACKE_ssytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a,
int lda, @Const IntBuffer ipiv,
FloatBuffer b, int ldb, FloatBuffer work );
public static native int LAPACKE_ssytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a,
int lda, @Const int[] ipiv,
float[] b, int ldb, float[] work );
public static native int LAPACKE_zbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, DoublePointer theta,
DoublePointer phi, @Cast("lapack_complex_double*") DoublePointer u1,
int ldu1, @Cast("lapack_complex_double*") DoublePointer u2,
int ldu2, @Cast("lapack_complex_double*") DoublePointer v1t,
int ldv1t, @Cast("lapack_complex_double*") DoublePointer v2t,
int ldv2t, DoublePointer b11d, DoublePointer b11e,
DoublePointer b12d, DoublePointer b12e, DoublePointer b21d,
DoublePointer b21e, DoublePointer b22d, DoublePointer b22e );
public static native int LAPACKE_zbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, DoubleBuffer theta,
DoubleBuffer phi, @Cast("lapack_complex_double*") DoubleBuffer u1,
int ldu1, @Cast("lapack_complex_double*") DoubleBuffer u2,
int ldu2, @Cast("lapack_complex_double*") DoubleBuffer v1t,
int ldv1t, @Cast("lapack_complex_double*") DoubleBuffer v2t,
int ldv2t, DoubleBuffer b11d, DoubleBuffer b11e,
DoubleBuffer b12d, DoubleBuffer b12e, DoubleBuffer b21d,
DoubleBuffer b21e, DoubleBuffer b22d, DoubleBuffer b22e );
public static native int LAPACKE_zbbcsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, int m,
int p, int q, double[] theta,
double[] phi, @Cast("lapack_complex_double*") double[] u1,
int ldu1, @Cast("lapack_complex_double*") double[] u2,
int ldu2, @Cast("lapack_complex_double*") double[] v1t,
int ldv1t, @Cast("lapack_complex_double*") double[] v2t,
int ldv2t, double[] b11d, double[] b11e,
double[] b12d, double[] b12e, double[] b21d,
double[] b21e, double[] b22d, double[] b22e );
public static native int LAPACKE_zbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
DoublePointer theta, DoublePointer phi,
@Cast("lapack_complex_double*") DoublePointer u1, int ldu1,
@Cast("lapack_complex_double*") DoublePointer u2, int ldu2,
@Cast("lapack_complex_double*") DoublePointer v1t, int ldv1t,
@Cast("lapack_complex_double*") DoublePointer v2t, int ldv2t,
DoublePointer b11d, DoublePointer b11e, DoublePointer b12d,
DoublePointer b12e, DoublePointer b21d, DoublePointer b21e,
DoublePointer b22d, DoublePointer b22e, DoublePointer rwork,
int lrwork );
public static native int LAPACKE_zbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
DoubleBuffer theta, DoubleBuffer phi,
@Cast("lapack_complex_double*") DoubleBuffer u1, int ldu1,
@Cast("lapack_complex_double*") DoubleBuffer u2, int ldu2,
@Cast("lapack_complex_double*") DoubleBuffer v1t, int ldv1t,
@Cast("lapack_complex_double*") DoubleBuffer v2t, int ldv2t,
DoubleBuffer b11d, DoubleBuffer b11e, DoubleBuffer b12d,
DoubleBuffer b12e, DoubleBuffer b21d, DoubleBuffer b21e,
DoubleBuffer b22d, DoubleBuffer b22e, DoubleBuffer rwork,
int lrwork );
public static native int LAPACKE_zbbcsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
int m, int p, int q,
double[] theta, double[] phi,
@Cast("lapack_complex_double*") double[] u1, int ldu1,
@Cast("lapack_complex_double*") double[] u2, int ldu2,
@Cast("lapack_complex_double*") double[] v1t, int ldv1t,
@Cast("lapack_complex_double*") double[] v2t, int ldv2t,
double[] b11d, double[] b11e, double[] b12d,
double[] b12e, double[] b21d, double[] b21e,
double[] b22d, double[] b22e, double[] rwork,
int lrwork );
public static native int LAPACKE_zheswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int i1,
int i2 );
public static native int LAPACKE_zheswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int i1,
int i2 );
public static native int LAPACKE_zheswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int i1,
int i2 );
public static native int LAPACKE_zheswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int i1,
int i2 );
public static native int LAPACKE_zheswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int i1,
int i2 );
public static native int LAPACKE_zheswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int i1,
int i2 );
public static native int LAPACKE_zhetri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_zhetri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_zhetri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_zhetri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zhetri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zhetri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_zhetri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv, int nb );
public static native int LAPACKE_zhetri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv, int nb );
public static native int LAPACKE_zhetri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv, int nb );
public static native int LAPACKE_zhetri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, int nb );
public static native int LAPACKE_zhetri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, int nb );
public static native int LAPACKE_zhetri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int nb );
public static native int LAPACKE_zhetrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zhetrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zhetrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_zhetrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zhetrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zhetrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_zsyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zsyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zsyconv( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_zsyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zsyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zsyconv_work( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte way,
int n, @Cast("lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_zsyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int i1,
int i2 );
public static native int LAPACKE_zsyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int i1,
int i2 );
public static native int LAPACKE_zsyswapr( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int i1,
int i2 );
public static native int LAPACKE_zsyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int i1,
int i2 );
public static native int LAPACKE_zsyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int i1,
int i2 );
public static native int LAPACKE_zsyswapr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int i1,
int i2 );
public static native int LAPACKE_zsytri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv );
public static native int LAPACKE_zsytri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv );
public static native int LAPACKE_zsytri2( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv );
public static native int LAPACKE_zsytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zsytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zsytri2_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_zsytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv, int nb );
public static native int LAPACKE_zsytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv, int nb );
public static native int LAPACKE_zsytri2x( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv, int nb );
public static native int LAPACKE_zsytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, int nb );
public static native int LAPACKE_zsytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, int nb );
public static native int LAPACKE_zsytri2x_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int nb );
public static native int LAPACKE_zsytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zsytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zsytrs2( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_zsytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zsytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zsytrs2_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_zunbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_double*") DoublePointer x11, int ldx11,
@Cast("lapack_complex_double*") DoublePointer x12, int ldx12,
@Cast("lapack_complex_double*") DoublePointer x21, int ldx21,
@Cast("lapack_complex_double*") DoublePointer x22, int ldx22,
DoublePointer theta, DoublePointer phi,
@Cast("lapack_complex_double*") DoublePointer taup1,
@Cast("lapack_complex_double*") DoublePointer taup2,
@Cast("lapack_complex_double*") DoublePointer tauq1,
@Cast("lapack_complex_double*") DoublePointer tauq2 );
public static native int LAPACKE_zunbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_double*") DoubleBuffer x11, int ldx11,
@Cast("lapack_complex_double*") DoubleBuffer x12, int ldx12,
@Cast("lapack_complex_double*") DoubleBuffer x21, int ldx21,
@Cast("lapack_complex_double*") DoubleBuffer x22, int ldx22,
DoubleBuffer theta, DoubleBuffer phi,
@Cast("lapack_complex_double*") DoubleBuffer taup1,
@Cast("lapack_complex_double*") DoubleBuffer taup2,
@Cast("lapack_complex_double*") DoubleBuffer tauq1,
@Cast("lapack_complex_double*") DoubleBuffer tauq2 );
public static native int LAPACKE_zunbdb( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_double*") double[] x11, int ldx11,
@Cast("lapack_complex_double*") double[] x12, int ldx12,
@Cast("lapack_complex_double*") double[] x21, int ldx21,
@Cast("lapack_complex_double*") double[] x22, int ldx22,
double[] theta, double[] phi,
@Cast("lapack_complex_double*") double[] taup1,
@Cast("lapack_complex_double*") double[] taup2,
@Cast("lapack_complex_double*") double[] tauq1,
@Cast("lapack_complex_double*") double[] tauq2 );
public static native int LAPACKE_zunbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_double*") DoublePointer x11, int ldx11,
@Cast("lapack_complex_double*") DoublePointer x12, int ldx12,
@Cast("lapack_complex_double*") DoublePointer x21, int ldx21,
@Cast("lapack_complex_double*") DoublePointer x22, int ldx22,
DoublePointer theta, DoublePointer phi,
@Cast("lapack_complex_double*") DoublePointer taup1,
@Cast("lapack_complex_double*") DoublePointer taup2,
@Cast("lapack_complex_double*") DoublePointer tauq1,
@Cast("lapack_complex_double*") DoublePointer tauq2,
@Cast("lapack_complex_double*") DoublePointer work, int lwork );
public static native int LAPACKE_zunbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_double*") DoubleBuffer x11, int ldx11,
@Cast("lapack_complex_double*") DoubleBuffer x12, int ldx12,
@Cast("lapack_complex_double*") DoubleBuffer x21, int ldx21,
@Cast("lapack_complex_double*") DoubleBuffer x22, int ldx22,
DoubleBuffer theta, DoubleBuffer phi,
@Cast("lapack_complex_double*") DoubleBuffer taup1,
@Cast("lapack_complex_double*") DoubleBuffer taup2,
@Cast("lapack_complex_double*") DoubleBuffer tauq1,
@Cast("lapack_complex_double*") DoubleBuffer tauq2,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork );
public static native int LAPACKE_zunbdb_work( int matrix_layout, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_double*") double[] x11, int ldx11,
@Cast("lapack_complex_double*") double[] x12, int ldx12,
@Cast("lapack_complex_double*") double[] x21, int ldx21,
@Cast("lapack_complex_double*") double[] x22, int ldx22,
double[] theta, double[] phi,
@Cast("lapack_complex_double*") double[] taup1,
@Cast("lapack_complex_double*") double[] taup2,
@Cast("lapack_complex_double*") double[] tauq1,
@Cast("lapack_complex_double*") double[] tauq2,
@Cast("lapack_complex_double*") double[] work, int lwork );
public static native int LAPACKE_zuncsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_double*") DoublePointer x11, int ldx11,
@Cast("lapack_complex_double*") DoublePointer x12, int ldx12,
@Cast("lapack_complex_double*") DoublePointer x21, int ldx21,
@Cast("lapack_complex_double*") DoublePointer x22, int ldx22,
DoublePointer theta, @Cast("lapack_complex_double*") DoublePointer u1,
int ldu1, @Cast("lapack_complex_double*") DoublePointer u2,
int ldu2, @Cast("lapack_complex_double*") DoublePointer v1t,
int ldv1t, @Cast("lapack_complex_double*") DoublePointer v2t,
int ldv2t );
public static native int LAPACKE_zuncsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_double*") DoubleBuffer x11, int ldx11,
@Cast("lapack_complex_double*") DoubleBuffer x12, int ldx12,
@Cast("lapack_complex_double*") DoubleBuffer x21, int ldx21,
@Cast("lapack_complex_double*") DoubleBuffer x22, int ldx22,
DoubleBuffer theta, @Cast("lapack_complex_double*") DoubleBuffer u1,
int ldu1, @Cast("lapack_complex_double*") DoubleBuffer u2,
int ldu2, @Cast("lapack_complex_double*") DoubleBuffer v1t,
int ldv1t, @Cast("lapack_complex_double*") DoubleBuffer v2t,
int ldv2t );
public static native int LAPACKE_zuncsd( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans, @Cast("char") byte signs,
int m, int p, int q,
@Cast("lapack_complex_double*") double[] x11, int ldx11,
@Cast("lapack_complex_double*") double[] x12, int ldx12,
@Cast("lapack_complex_double*") double[] x21, int ldx21,
@Cast("lapack_complex_double*") double[] x22, int ldx22,
double[] theta, @Cast("lapack_complex_double*") double[] u1,
int ldu1, @Cast("lapack_complex_double*") double[] u2,
int ldu2, @Cast("lapack_complex_double*") double[] v1t,
int ldv1t, @Cast("lapack_complex_double*") double[] v2t,
int ldv2t );
public static native int LAPACKE_zuncsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, @Cast("lapack_complex_double*") DoublePointer x11,
int ldx11, @Cast("lapack_complex_double*") DoublePointer x12,
int ldx12, @Cast("lapack_complex_double*") DoublePointer x21,
int ldx21, @Cast("lapack_complex_double*") DoublePointer x22,
int ldx22, DoublePointer theta,
@Cast("lapack_complex_double*") DoublePointer u1, int ldu1,
@Cast("lapack_complex_double*") DoublePointer u2, int ldu2,
@Cast("lapack_complex_double*") DoublePointer v1t, int ldv1t,
@Cast("lapack_complex_double*") DoublePointer v2t, int ldv2t,
@Cast("lapack_complex_double*") DoublePointer work, int lwork,
DoublePointer rwork, int lrwork,
IntPointer iwork );
public static native int LAPACKE_zuncsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, @Cast("lapack_complex_double*") DoubleBuffer x11,
int ldx11, @Cast("lapack_complex_double*") DoubleBuffer x12,
int ldx12, @Cast("lapack_complex_double*") DoubleBuffer x21,
int ldx21, @Cast("lapack_complex_double*") DoubleBuffer x22,
int ldx22, DoubleBuffer theta,
@Cast("lapack_complex_double*") DoubleBuffer u1, int ldu1,
@Cast("lapack_complex_double*") DoubleBuffer u2, int ldu2,
@Cast("lapack_complex_double*") DoubleBuffer v1t, int ldv1t,
@Cast("lapack_complex_double*") DoubleBuffer v2t, int ldv2t,
@Cast("lapack_complex_double*") DoubleBuffer work, int lwork,
DoubleBuffer rwork, int lrwork,
IntBuffer iwork );
public static native int LAPACKE_zuncsd_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, @Cast("char") byte jobv2t, @Cast("char") byte trans,
@Cast("char") byte signs, int m, int p,
int q, @Cast("lapack_complex_double*") double[] x11,
int ldx11, @Cast("lapack_complex_double*") double[] x12,
int ldx12, @Cast("lapack_complex_double*") double[] x21,
int ldx21, @Cast("lapack_complex_double*") double[] x22,
int ldx22, double[] theta,
@Cast("lapack_complex_double*") double[] u1, int ldu1,
@Cast("lapack_complex_double*") double[] u2, int ldu2,
@Cast("lapack_complex_double*") double[] v1t, int ldv1t,
@Cast("lapack_complex_double*") double[] v2t, int ldv2t,
@Cast("lapack_complex_double*") double[] work, int lwork,
double[] rwork, int lrwork,
int[] iwork );
public static native int LAPACKE_zuncsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
@Cast("lapack_complex_double*") DoublePointer x11, int ldx11,
@Cast("lapack_complex_double*") DoublePointer x21, int ldx21,
@Cast("lapack_complex_double*") DoublePointer theta, @Cast("lapack_complex_double*") DoublePointer u1,
int ldu1, @Cast("lapack_complex_double*") DoublePointer u2,
int ldu2, @Cast("lapack_complex_double*") DoublePointer v1t, int ldv1t );
public static native int LAPACKE_zuncsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
@Cast("lapack_complex_double*") DoubleBuffer x11, int ldx11,
@Cast("lapack_complex_double*") DoubleBuffer x21, int ldx21,
@Cast("lapack_complex_double*") DoubleBuffer theta, @Cast("lapack_complex_double*") DoubleBuffer u1,
int ldu1, @Cast("lapack_complex_double*") DoubleBuffer u2,
int ldu2, @Cast("lapack_complex_double*") DoubleBuffer v1t, int ldv1t );
public static native int LAPACKE_zuncsd2by1( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p, int q,
@Cast("lapack_complex_double*") double[] x11, int ldx11,
@Cast("lapack_complex_double*") double[] x21, int ldx21,
@Cast("lapack_complex_double*") double[] theta, @Cast("lapack_complex_double*") double[] u1,
int ldu1, @Cast("lapack_complex_double*") double[] u2,
int ldu2, @Cast("lapack_complex_double*") double[] v1t, int ldv1t );
public static native int LAPACKE_zuncsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p,
int q, @Cast("lapack_complex_double*") DoublePointer x11, int ldx11,
@Cast("lapack_complex_double*") DoublePointer x21, int ldx21,
@Cast("lapack_complex_double*") DoublePointer theta, @Cast("lapack_complex_double*") DoublePointer u1,
int ldu1, @Cast("lapack_complex_double*") DoublePointer u2,
int ldu2, @Cast("lapack_complex_double*") DoublePointer v1t,
int ldv1t, @Cast("lapack_complex_double*") DoublePointer work,
int lwork, DoublePointer rwork, int lrwork,
IntPointer iwork );
public static native int LAPACKE_zuncsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p,
int q, @Cast("lapack_complex_double*") DoubleBuffer x11, int ldx11,
@Cast("lapack_complex_double*") DoubleBuffer x21, int ldx21,
@Cast("lapack_complex_double*") DoubleBuffer theta, @Cast("lapack_complex_double*") DoubleBuffer u1,
int ldu1, @Cast("lapack_complex_double*") DoubleBuffer u2,
int ldu2, @Cast("lapack_complex_double*") DoubleBuffer v1t,
int ldv1t, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork, DoubleBuffer rwork, int lrwork,
IntBuffer iwork );
public static native int LAPACKE_zuncsd2by1_work( int matrix_layout, @Cast("char") byte jobu1, @Cast("char") byte jobu2,
@Cast("char") byte jobv1t, int m, int p,
int q, @Cast("lapack_complex_double*") double[] x11, int ldx11,
@Cast("lapack_complex_double*") double[] x21, int ldx21,
@Cast("lapack_complex_double*") double[] theta, @Cast("lapack_complex_double*") double[] u1,
int ldu1, @Cast("lapack_complex_double*") double[] u2,
int ldu2, @Cast("lapack_complex_double*") double[] v1t,
int ldv1t, @Cast("lapack_complex_double*") double[] work,
int lwork, double[] rwork, int lrwork,
int[] iwork );
//LAPACK 3.4.0
public static native int LAPACKE_sgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const FloatPointer v, int ldv,
@Const FloatPointer t, int ldt, FloatPointer c,
int ldc );
public static native int LAPACKE_sgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const FloatBuffer v, int ldv,
@Const FloatBuffer t, int ldt, FloatBuffer c,
int ldc );
public static native int LAPACKE_sgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const float[] v, int ldv,
@Const float[] t, int ldt, float[] c,
int ldc );
public static native int LAPACKE_dgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const DoublePointer v, int ldv,
@Const DoublePointer t, int ldt, DoublePointer c,
int ldc );
public static native int LAPACKE_dgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const DoubleBuffer v, int ldv,
@Const DoubleBuffer t, int ldt, DoubleBuffer c,
int ldc );
public static native int LAPACKE_dgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const double[] v, int ldv,
@Const double[] t, int ldt, double[] c,
int ldc );
public static native int LAPACKE_cgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_float*") FloatPointer v,
int ldv, @Cast("const lapack_complex_float*") FloatPointer t,
int ldt, @Cast("lapack_complex_float*") FloatPointer c,
int ldc );
public static native int LAPACKE_cgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_float*") FloatBuffer v,
int ldv, @Cast("const lapack_complex_float*") FloatBuffer t,
int ldt, @Cast("lapack_complex_float*") FloatBuffer c,
int ldc );
public static native int LAPACKE_cgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_float*") float[] v,
int ldv, @Cast("const lapack_complex_float*") float[] t,
int ldt, @Cast("lapack_complex_float*") float[] c,
int ldc );
public static native int LAPACKE_zgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_double*") DoublePointer v,
int ldv, @Cast("const lapack_complex_double*") DoublePointer t,
int ldt, @Cast("lapack_complex_double*") DoublePointer c,
int ldc );
public static native int LAPACKE_zgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_double*") DoubleBuffer v,
int ldv, @Cast("const lapack_complex_double*") DoubleBuffer t,
int ldt, @Cast("lapack_complex_double*") DoubleBuffer c,
int ldc );
public static native int LAPACKE_zgemqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_double*") double[] v,
int ldv, @Cast("const lapack_complex_double*") double[] t,
int ldt, @Cast("lapack_complex_double*") double[] c,
int ldc );
public static native int LAPACKE_sgeqrt( int matrix_layout, int m, int n,
int nb, FloatPointer a, int lda, FloatPointer t,
int ldt );
public static native int LAPACKE_sgeqrt( int matrix_layout, int m, int n,
int nb, FloatBuffer a, int lda, FloatBuffer t,
int ldt );
public static native int LAPACKE_sgeqrt( int matrix_layout, int m, int n,
int nb, float[] a, int lda, float[] t,
int ldt );
public static native int LAPACKE_dgeqrt( int matrix_layout, int m, int n,
int nb, DoublePointer a, int lda, DoublePointer t,
int ldt );
public static native int LAPACKE_dgeqrt( int matrix_layout, int m, int n,
int nb, DoubleBuffer a, int lda, DoubleBuffer t,
int ldt );
public static native int LAPACKE_dgeqrt( int matrix_layout, int m, int n,
int nb, double[] a, int lda, double[] t,
int ldt );
public static native int LAPACKE_cgeqrt( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer t,
int ldt );
public static native int LAPACKE_cgeqrt( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer t,
int ldt );
public static native int LAPACKE_cgeqrt( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] t,
int ldt );
public static native int LAPACKE_zgeqrt( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer t,
int ldt );
public static native int LAPACKE_zgeqrt( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer t,
int ldt );
public static native int LAPACKE_zgeqrt( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] t,
int ldt );
public static native int LAPACKE_sgeqrt2( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer t,
int ldt );
public static native int LAPACKE_sgeqrt2( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer t,
int ldt );
public static native int LAPACKE_sgeqrt2( int matrix_layout, int m, int n,
float[] a, int lda, float[] t,
int ldt );
public static native int LAPACKE_dgeqrt2( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer t,
int ldt );
public static native int LAPACKE_dgeqrt2( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer t,
int ldt );
public static native int LAPACKE_dgeqrt2( int matrix_layout, int m, int n,
double[] a, int lda, double[] t,
int ldt );
public static native int LAPACKE_cgeqrt2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer t, int ldt );
public static native int LAPACKE_cgeqrt2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt );
public static native int LAPACKE_cgeqrt2( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] t, int ldt );
public static native int LAPACKE_zgeqrt2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer t, int ldt );
public static native int LAPACKE_zgeqrt2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt );
public static native int LAPACKE_zgeqrt2( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] t, int ldt );
public static native int LAPACKE_sgeqrt3( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer t,
int ldt );
public static native int LAPACKE_sgeqrt3( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer t,
int ldt );
public static native int LAPACKE_sgeqrt3( int matrix_layout, int m, int n,
float[] a, int lda, float[] t,
int ldt );
public static native int LAPACKE_dgeqrt3( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer t,
int ldt );
public static native int LAPACKE_dgeqrt3( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer t,
int ldt );
public static native int LAPACKE_dgeqrt3( int matrix_layout, int m, int n,
double[] a, int lda, double[] t,
int ldt );
public static native int LAPACKE_cgeqrt3( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer t, int ldt );
public static native int LAPACKE_cgeqrt3( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt );
public static native int LAPACKE_cgeqrt3( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] t, int ldt );
public static native int LAPACKE_zgeqrt3( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer t, int ldt );
public static native int LAPACKE_zgeqrt3( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt );
public static native int LAPACKE_zgeqrt3( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] t, int ldt );
public static native int LAPACKE_stpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const FloatPointer v,
int ldv, @Const FloatPointer t, int ldt,
FloatPointer a, int lda, FloatPointer b,
int ldb );
public static native int LAPACKE_stpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const FloatBuffer v,
int ldv, @Const FloatBuffer t, int ldt,
FloatBuffer a, int lda, FloatBuffer b,
int ldb );
public static native int LAPACKE_stpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const float[] v,
int ldv, @Const float[] t, int ldt,
float[] a, int lda, float[] b,
int ldb );
public static native int LAPACKE_dtpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const DoublePointer v,
int ldv, @Const DoublePointer t, int ldt,
DoublePointer a, int lda, DoublePointer b,
int ldb );
public static native int LAPACKE_dtpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const DoubleBuffer v,
int ldv, @Const DoubleBuffer t, int ldt,
DoubleBuffer a, int lda, DoubleBuffer b,
int ldb );
public static native int LAPACKE_dtpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const double[] v,
int ldv, @Const double[] t, int ldt,
double[] a, int lda, double[] b,
int ldb );
public static native int LAPACKE_ctpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_float*") FloatPointer v, int ldv,
@Cast("const lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_ctpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("const lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_ctpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_float*") float[] v, int ldv,
@Cast("const lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_ztpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_double*") DoublePointer v, int ldv,
@Cast("const lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_ztpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("const lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_ztpmqrt( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_double*") double[] v, int ldv,
@Cast("const lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_stpqrt( int matrix_layout, int m, int n,
int l, int nb, FloatPointer a,
int lda, FloatPointer b, int ldb, FloatPointer t,
int ldt );
public static native int LAPACKE_stpqrt( int matrix_layout, int m, int n,
int l, int nb, FloatBuffer a,
int lda, FloatBuffer b, int ldb, FloatBuffer t,
int ldt );
public static native int LAPACKE_stpqrt( int matrix_layout, int m, int n,
int l, int nb, float[] a,
int lda, float[] b, int ldb, float[] t,
int ldt );
public static native int LAPACKE_dtpqrt( int matrix_layout, int m, int n,
int l, int nb, DoublePointer a,
int lda, DoublePointer b, int ldb, DoublePointer t,
int ldt );
public static native int LAPACKE_dtpqrt( int matrix_layout, int m, int n,
int l, int nb, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb, DoubleBuffer t,
int ldt );
public static native int LAPACKE_dtpqrt( int matrix_layout, int m, int n,
int l, int nb, double[] a,
int lda, double[] b, int ldb, double[] t,
int ldt );
public static native int LAPACKE_ctpqrt( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer t, int ldt );
public static native int LAPACKE_ctpqrt( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt );
public static native int LAPACKE_ctpqrt( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] t, int ldt );
public static native int LAPACKE_ztpqrt( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer t, int ldt );
public static native int LAPACKE_ztpqrt( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt );
public static native int LAPACKE_ztpqrt( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] t, int ldt );
public static native int LAPACKE_stpqrt2( int matrix_layout,
int m, int n, int l,
FloatPointer a, int lda,
FloatPointer b, int ldb,
FloatPointer t, int ldt );
public static native int LAPACKE_stpqrt2( int matrix_layout,
int m, int n, int l,
FloatBuffer a, int lda,
FloatBuffer b, int ldb,
FloatBuffer t, int ldt );
public static native int LAPACKE_stpqrt2( int matrix_layout,
int m, int n, int l,
float[] a, int lda,
float[] b, int ldb,
float[] t, int ldt );
public static native int LAPACKE_dtpqrt2( int matrix_layout,
int m, int n, int l,
DoublePointer a, int lda,
DoublePointer b, int ldb,
DoublePointer t, int ldt );
public static native int LAPACKE_dtpqrt2( int matrix_layout,
int m, int n, int l,
DoubleBuffer a, int lda,
DoubleBuffer b, int ldb,
DoubleBuffer t, int ldt );
public static native int LAPACKE_dtpqrt2( int matrix_layout,
int m, int n, int l,
double[] a, int lda,
double[] b, int ldb,
double[] t, int ldt );
public static native int LAPACKE_ctpqrt2( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer t, int ldt );
public static native int LAPACKE_ctpqrt2( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt );
public static native int LAPACKE_ctpqrt2( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] t, int ldt );
public static native int LAPACKE_ztpqrt2( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer t, int ldt );
public static native int LAPACKE_ztpqrt2( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt );
public static native int LAPACKE_ztpqrt2( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] t, int ldt );
public static native int LAPACKE_stprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l, @Const FloatPointer v,
int ldv, @Const FloatPointer t, int ldt,
FloatPointer a, int lda, FloatPointer b, int ldb );
public static native int LAPACKE_stprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l, @Const FloatBuffer v,
int ldv, @Const FloatBuffer t, int ldt,
FloatBuffer a, int lda, FloatBuffer b, int ldb );
public static native int LAPACKE_stprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l, @Const float[] v,
int ldv, @Const float[] t, int ldt,
float[] a, int lda, float[] b, int ldb );
public static native int LAPACKE_dtprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l, @Const DoublePointer v,
int ldv, @Const DoublePointer t, int ldt,
DoublePointer a, int lda, DoublePointer b, int ldb );
public static native int LAPACKE_dtprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l, @Const DoubleBuffer v,
int ldv, @Const DoubleBuffer t, int ldt,
DoubleBuffer a, int lda, DoubleBuffer b, int ldb );
public static native int LAPACKE_dtprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l, @Const double[] v,
int ldv, @Const double[] t, int ldt,
double[] a, int lda, double[] b, int ldb );
public static native int LAPACKE_ctprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l,
@Cast("const lapack_complex_float*") FloatPointer v, int ldv,
@Cast("const lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_ctprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l,
@Cast("const lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("const lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_ctprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l,
@Cast("const lapack_complex_float*") float[] v, int ldv,
@Cast("const lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_ztprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l,
@Cast("const lapack_complex_double*") DoublePointer v, int ldv,
@Cast("const lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_ztprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l,
@Cast("const lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("const lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_ztprfb( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans, @Cast("char") byte direct,
@Cast("char") byte storev, int m, int n,
int k, int l,
@Cast("const lapack_complex_double*") double[] v, int ldv,
@Cast("const lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_sgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const FloatPointer v, int ldv,
@Const FloatPointer t, int ldt, FloatPointer c,
int ldc, FloatPointer work );
public static native int LAPACKE_sgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const FloatBuffer v, int ldv,
@Const FloatBuffer t, int ldt, FloatBuffer c,
int ldc, FloatBuffer work );
public static native int LAPACKE_sgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const float[] v, int ldv,
@Const float[] t, int ldt, float[] c,
int ldc, float[] work );
public static native int LAPACKE_dgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const DoublePointer v, int ldv,
@Const DoublePointer t, int ldt, DoublePointer c,
int ldc, DoublePointer work );
public static native int LAPACKE_dgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const DoubleBuffer v, int ldv,
@Const DoubleBuffer t, int ldt, DoubleBuffer c,
int ldc, DoubleBuffer work );
public static native int LAPACKE_dgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Const double[] v, int ldv,
@Const double[] t, int ldt, double[] c,
int ldc, double[] work );
public static native int LAPACKE_cgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_float*") FloatPointer v,
int ldv, @Cast("const lapack_complex_float*") FloatPointer t,
int ldt, @Cast("lapack_complex_float*") FloatPointer c,
int ldc, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_cgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_float*") FloatBuffer v,
int ldv, @Cast("const lapack_complex_float*") FloatBuffer t,
int ldt, @Cast("lapack_complex_float*") FloatBuffer c,
int ldc, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_cgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_float*") float[] v,
int ldv, @Cast("const lapack_complex_float*") float[] t,
int ldt, @Cast("lapack_complex_float*") float[] c,
int ldc, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_double*") DoublePointer v,
int ldv, @Cast("const lapack_complex_double*") DoublePointer t,
int ldt, @Cast("lapack_complex_double*") DoublePointer c,
int ldc, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_double*") DoubleBuffer v,
int ldv, @Cast("const lapack_complex_double*") DoubleBuffer t,
int ldt, @Cast("lapack_complex_double*") DoubleBuffer c,
int ldc, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zgemqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int nb, @Cast("const lapack_complex_double*") double[] v,
int ldv, @Cast("const lapack_complex_double*") double[] t,
int ldt, @Cast("lapack_complex_double*") double[] c,
int ldc, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_sgeqrt_work( int matrix_layout, int m, int n,
int nb, FloatPointer a, int lda,
FloatPointer t, int ldt, FloatPointer work );
public static native int LAPACKE_sgeqrt_work( int matrix_layout, int m, int n,
int nb, FloatBuffer a, int lda,
FloatBuffer t, int ldt, FloatBuffer work );
public static native int LAPACKE_sgeqrt_work( int matrix_layout, int m, int n,
int nb, float[] a, int lda,
float[] t, int ldt, float[] work );
public static native int LAPACKE_dgeqrt_work( int matrix_layout, int m, int n,
int nb, DoublePointer a, int lda,
DoublePointer t, int ldt, DoublePointer work );
public static native int LAPACKE_dgeqrt_work( int matrix_layout, int m, int n,
int nb, DoubleBuffer a, int lda,
DoubleBuffer t, int ldt, DoubleBuffer work );
public static native int LAPACKE_dgeqrt_work( int matrix_layout, int m, int n,
int nb, double[] a, int lda,
double[] t, int ldt, double[] work );
public static native int LAPACKE_cgeqrt_work( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_float*") FloatPointer a,
int lda, @Cast("lapack_complex_float*") FloatPointer t,
int ldt, @Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_cgeqrt_work( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, @Cast("lapack_complex_float*") FloatBuffer t,
int ldt, @Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_cgeqrt_work( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_float*") float[] a,
int lda, @Cast("lapack_complex_float*") float[] t,
int ldt, @Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_zgeqrt_work( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_double*") DoublePointer a,
int lda, @Cast("lapack_complex_double*") DoublePointer t,
int ldt, @Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_zgeqrt_work( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, @Cast("lapack_complex_double*") DoubleBuffer t,
int ldt, @Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_zgeqrt_work( int matrix_layout, int m, int n,
int nb, @Cast("lapack_complex_double*") double[] a,
int lda, @Cast("lapack_complex_double*") double[] t,
int ldt, @Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_sgeqrt2_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer t,
int ldt );
public static native int LAPACKE_sgeqrt2_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer t,
int ldt );
public static native int LAPACKE_sgeqrt2_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] t,
int ldt );
public static native int LAPACKE_dgeqrt2_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer t,
int ldt );
public static native int LAPACKE_dgeqrt2_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer t,
int ldt );
public static native int LAPACKE_dgeqrt2_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] t,
int ldt );
public static native int LAPACKE_cgeqrt2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer t, int ldt );
public static native int LAPACKE_cgeqrt2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt );
public static native int LAPACKE_cgeqrt2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] t, int ldt );
public static native int LAPACKE_zgeqrt2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer t, int ldt );
public static native int LAPACKE_zgeqrt2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt );
public static native int LAPACKE_zgeqrt2_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] t, int ldt );
public static native int LAPACKE_sgeqrt3_work( int matrix_layout, int m, int n,
FloatPointer a, int lda, FloatPointer t,
int ldt );
public static native int LAPACKE_sgeqrt3_work( int matrix_layout, int m, int n,
FloatBuffer a, int lda, FloatBuffer t,
int ldt );
public static native int LAPACKE_sgeqrt3_work( int matrix_layout, int m, int n,
float[] a, int lda, float[] t,
int ldt );
public static native int LAPACKE_dgeqrt3_work( int matrix_layout, int m, int n,
DoublePointer a, int lda, DoublePointer t,
int ldt );
public static native int LAPACKE_dgeqrt3_work( int matrix_layout, int m, int n,
DoubleBuffer a, int lda, DoubleBuffer t,
int ldt );
public static native int LAPACKE_dgeqrt3_work( int matrix_layout, int m, int n,
double[] a, int lda, double[] t,
int ldt );
public static native int LAPACKE_cgeqrt3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer t, int ldt );
public static native int LAPACKE_cgeqrt3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt );
public static native int LAPACKE_cgeqrt3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] t, int ldt );
public static native int LAPACKE_zgeqrt3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer t, int ldt );
public static native int LAPACKE_zgeqrt3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt );
public static native int LAPACKE_zgeqrt3_work( int matrix_layout, int m, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] t, int ldt );
public static native int LAPACKE_stpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const FloatPointer v,
int ldv, @Const FloatPointer t, int ldt,
FloatPointer a, int lda, FloatPointer b,
int ldb, FloatPointer work );
public static native int LAPACKE_stpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const FloatBuffer v,
int ldv, @Const FloatBuffer t, int ldt,
FloatBuffer a, int lda, FloatBuffer b,
int ldb, FloatBuffer work );
public static native int LAPACKE_stpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const float[] v,
int ldv, @Const float[] t, int ldt,
float[] a, int lda, float[] b,
int ldb, float[] work );
public static native int LAPACKE_dtpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const DoublePointer v,
int ldv, @Const DoublePointer t,
int ldt, DoublePointer a, int lda,
DoublePointer b, int ldb, DoublePointer work );
public static native int LAPACKE_dtpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const DoubleBuffer v,
int ldv, @Const DoubleBuffer t,
int ldt, DoubleBuffer a, int lda,
DoubleBuffer b, int ldb, DoubleBuffer work );
public static native int LAPACKE_dtpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb, @Const double[] v,
int ldv, @Const double[] t,
int ldt, double[] a, int lda,
double[] b, int ldb, double[] work );
public static native int LAPACKE_ctpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_float*") FloatPointer v, int ldv,
@Cast("const lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_ctpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("const lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_ctpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_float*") float[] v, int ldv,
@Cast("const lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_ztpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_double*") DoublePointer v, int ldv,
@Cast("const lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_ztpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("const lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_ztpmqrt_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
int m, int n, int k,
int l, int nb,
@Cast("const lapack_complex_double*") double[] v, int ldv,
@Cast("const lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_stpqrt_work( int matrix_layout, int m, int n,
int l, int nb, FloatPointer a,
int lda, FloatPointer b, int ldb,
FloatPointer t, int ldt, FloatPointer work );
public static native int LAPACKE_stpqrt_work( int matrix_layout, int m, int n,
int l, int nb, FloatBuffer a,
int lda, FloatBuffer b, int ldb,
FloatBuffer t, int ldt, FloatBuffer work );
public static native int LAPACKE_stpqrt_work( int matrix_layout, int m, int n,
int l, int nb, float[] a,
int lda, float[] b, int ldb,
float[] t, int ldt, float[] work );
public static native int LAPACKE_dtpqrt_work( int matrix_layout, int m, int n,
int l, int nb, DoublePointer a,
int lda, DoublePointer b, int ldb,
DoublePointer t, int ldt, DoublePointer work );
public static native int LAPACKE_dtpqrt_work( int matrix_layout, int m, int n,
int l, int nb, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
DoubleBuffer t, int ldt, DoubleBuffer work );
public static native int LAPACKE_dtpqrt_work( int matrix_layout, int m, int n,
int l, int nb, double[] a,
int lda, double[] b, int ldb,
double[] t, int ldt, double[] work );
public static native int LAPACKE_ctpqrt_work( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer work );
public static native int LAPACKE_ctpqrt_work( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native int LAPACKE_ctpqrt_work( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] work );
public static native int LAPACKE_ztpqrt_work( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer work );
public static native int LAPACKE_ztpqrt_work( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native int LAPACKE_ztpqrt_work( int matrix_layout, int m, int n,
int l, int nb,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] work );
public static native int LAPACKE_stpqrt2_work( int matrix_layout,
int m, int n, int l,
FloatPointer a, int lda,
FloatPointer b, int ldb,
FloatPointer t, int ldt );
public static native int LAPACKE_stpqrt2_work( int matrix_layout,
int m, int n, int l,
FloatBuffer a, int lda,
FloatBuffer b, int ldb,
FloatBuffer t, int ldt );
public static native int LAPACKE_stpqrt2_work( int matrix_layout,
int m, int n, int l,
float[] a, int lda,
float[] b, int ldb,
float[] t, int ldt );
public static native int LAPACKE_dtpqrt2_work( int matrix_layout,
int m, int n, int l,
DoublePointer a, int lda,
DoublePointer b, int ldb,
DoublePointer t, int ldt );
public static native int LAPACKE_dtpqrt2_work( int matrix_layout,
int m, int n, int l,
DoubleBuffer a, int lda,
DoubleBuffer b, int ldb,
DoubleBuffer t, int ldt );
public static native int LAPACKE_dtpqrt2_work( int matrix_layout,
int m, int n, int l,
double[] a, int lda,
double[] b, int ldb,
double[] t, int ldt );
public static native int LAPACKE_ctpqrt2_work( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer t, int ldt );
public static native int LAPACKE_ctpqrt2_work( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer t, int ldt );
public static native int LAPACKE_ctpqrt2_work( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] t, int ldt );
public static native int LAPACKE_ztpqrt2_work( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer t, int ldt );
public static native int LAPACKE_ztpqrt2_work( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer t, int ldt );
public static native int LAPACKE_ztpqrt2_work( int matrix_layout,
int m, int n, int l,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] t, int ldt );
public static native int LAPACKE_stprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Const FloatPointer v, int ldv, @Const FloatPointer t,
int ldt, FloatPointer a, int lda,
FloatPointer b, int ldb, @Const FloatPointer work,
int ldwork );
public static native int LAPACKE_stprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Const FloatBuffer v, int ldv, @Const FloatBuffer t,
int ldt, FloatBuffer a, int lda,
FloatBuffer b, int ldb, @Const FloatBuffer work,
int ldwork );
public static native int LAPACKE_stprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Const float[] v, int ldv, @Const float[] t,
int ldt, float[] a, int lda,
float[] b, int ldb, @Const float[] work,
int ldwork );
public static native int LAPACKE_dtprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Const DoublePointer v, int ldv,
@Const DoublePointer t, int ldt, DoublePointer a,
int lda, DoublePointer b, int ldb,
@Const DoublePointer work, int ldwork );
public static native int LAPACKE_dtprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Const DoubleBuffer v, int ldv,
@Const DoubleBuffer t, int ldt, DoubleBuffer a,
int lda, DoubleBuffer b, int ldb,
@Const DoubleBuffer work, int ldwork );
public static native int LAPACKE_dtprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Const double[] v, int ldv,
@Const double[] t, int ldt, double[] a,
int lda, double[] b, int ldb,
@Const double[] work, int ldwork );
public static native int LAPACKE_ctprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Cast("const lapack_complex_float*") FloatPointer v, int ldv,
@Cast("const lapack_complex_float*") FloatPointer t, int ldt,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer work, int ldwork );
public static native int LAPACKE_ctprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Cast("const lapack_complex_float*") FloatBuffer v, int ldv,
@Cast("const lapack_complex_float*") FloatBuffer t, int ldt,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer work, int ldwork );
public static native int LAPACKE_ctprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Cast("const lapack_complex_float*") float[] v, int ldv,
@Cast("const lapack_complex_float*") float[] t, int ldt,
@Cast("lapack_complex_float*") float[] a, int lda,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] work, int ldwork );
public static native int LAPACKE_ztprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Cast("const lapack_complex_double*") DoublePointer v, int ldv,
@Cast("const lapack_complex_double*") DoublePointer t, int ldt,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer work, int ldwork );
public static native int LAPACKE_ztprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Cast("const lapack_complex_double*") DoubleBuffer v, int ldv,
@Cast("const lapack_complex_double*") DoubleBuffer t, int ldt,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, int ldwork );
public static native int LAPACKE_ztprfb_work( int matrix_layout, @Cast("char") byte side, @Cast("char") byte trans,
@Cast("char") byte direct, @Cast("char") byte storev, int m,
int n, int k, int l,
@Cast("const lapack_complex_double*") double[] v, int ldv,
@Cast("const lapack_complex_double*") double[] t, int ldt,
@Cast("lapack_complex_double*") double[] a, int lda,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] work, int ldwork );
//LAPACK 3.X.X
public static native int LAPACKE_ssysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer a, int lda,
IntPointer ipiv, FloatPointer b, int ldb );
public static native int LAPACKE_ssysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer a, int lda,
IntBuffer ipiv, FloatBuffer b, int ldb );
public static native int LAPACKE_ssysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] a, int lda,
int[] ipiv, float[] b, int ldb );
public static native int LAPACKE_dsysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer a, int lda,
IntPointer ipiv, DoublePointer b, int ldb );
public static native int LAPACKE_dsysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer a, int lda,
IntBuffer ipiv, DoubleBuffer b, int ldb );
public static native int LAPACKE_dsysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] a, int lda,
int[] ipiv, double[] b, int ldb );
public static native int LAPACKE_csysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_csysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_csysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zsysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zsysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zsysv_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_ssytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n, FloatPointer a,
int lda, IntPointer ipiv );
public static native int LAPACKE_ssytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n, FloatBuffer a,
int lda, IntBuffer ipiv );
public static native int LAPACKE_ssytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n, float[] a,
int lda, int[] ipiv );
public static native int LAPACKE_dsytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n, DoublePointer a,
int lda, IntPointer ipiv );
public static native int LAPACKE_dsytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n, DoubleBuffer a,
int lda, IntBuffer ipiv );
public static native int LAPACKE_dsytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n, double[] a,
int lda, int[] ipiv );
public static native int LAPACKE_csytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_csytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_csytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zsytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zsytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zsytrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_ssytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer b, int ldb );
public static native int LAPACKE_ssytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer b, int ldb );
public static native int LAPACKE_ssytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
@Const int[] ipiv, float[] b, int ldb );
public static native int LAPACKE_dsytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a, int lda,
@Const IntPointer ipiv, DoublePointer b, int ldb );
public static native int LAPACKE_dsytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a, int lda,
@Const IntBuffer ipiv, DoubleBuffer b, int ldb );
public static native int LAPACKE_dsytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a, int lda,
@Const int[] ipiv, double[] b, int ldb );
public static native int LAPACKE_csytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_csytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_csytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zsytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zsytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zsytrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_chetrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_chetrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_chetrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv );
public static native int LAPACKE_zhetrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv );
public static native int LAPACKE_zhetrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv );
public static native int LAPACKE_zhetrf_rook( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv );
public static native int LAPACKE_chetrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_chetrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_chetrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zhetrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zhetrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zhetrs_rook( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_csyr( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("const lapack_complex_float*") FloatPointer x, int incx,
@Cast("lapack_complex_float*") FloatPointer a, int lda );
public static native int LAPACKE_csyr( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("const lapack_complex_float*") FloatBuffer x, int incx,
@Cast("lapack_complex_float*") FloatBuffer a, int lda );
public static native int LAPACKE_csyr( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_float*") float[] alpha,
@Cast("const lapack_complex_float*") float[] x, int incx,
@Cast("lapack_complex_float*") float[] a, int lda );
public static native int LAPACKE_zsyr( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("const lapack_complex_double*") DoublePointer x, int incx,
@Cast("lapack_complex_double*") DoublePointer a, int lda );
public static native int LAPACKE_zsyr( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("const lapack_complex_double*") DoubleBuffer x, int incx,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda );
public static native int LAPACKE_zsyr( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_double*") double[] alpha,
@Cast("const lapack_complex_double*") double[] x, int incx,
@Cast("lapack_complex_double*") double[] a, int lda );
public static native int LAPACKE_ssysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatPointer a, int lda,
IntPointer ipiv, FloatPointer b, int ldb,
FloatPointer work, int lwork );
public static native int LAPACKE_ssysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, FloatBuffer a, int lda,
IntBuffer ipiv, FloatBuffer b, int ldb,
FloatBuffer work, int lwork );
public static native int LAPACKE_ssysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, float[] a, int lda,
int[] ipiv, float[] b, int ldb,
float[] work, int lwork );
public static native int LAPACKE_dsysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoublePointer a, int lda,
IntPointer ipiv, DoublePointer b, int ldb,
DoublePointer work, int lwork );
public static native int LAPACKE_dsysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, DoubleBuffer a, int lda,
IntBuffer ipiv, DoubleBuffer b, int ldb,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dsysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, double[] a, int lda,
int[] ipiv, double[] b, int ldb,
double[] work, int lwork );
public static native int LAPACKE_csysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatPointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb,
@Cast("lapack_complex_float*") FloatPointer work,
int lwork );
public static native int LAPACKE_csysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb,
@Cast("lapack_complex_float*") FloatBuffer work,
int lwork );
public static native int LAPACKE_csysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_float*") float[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb,
@Cast("lapack_complex_float*") float[] work,
int lwork );
public static native int LAPACKE_zsysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoublePointer a,
int lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb,
@Cast("lapack_complex_double*") DoublePointer work,
int lwork );
public static native int LAPACKE_zsysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb,
@Cast("lapack_complex_double*") DoubleBuffer work,
int lwork );
public static native int LAPACKE_zsysv_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("lapack_complex_double*") double[] a,
int lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb,
@Cast("lapack_complex_double*") double[] work,
int lwork );
public static native int LAPACKE_ssytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatPointer a, int lda, IntPointer ipiv,
FloatPointer work, int lwork );
public static native int LAPACKE_ssytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
FloatBuffer a, int lda, IntBuffer ipiv,
FloatBuffer work, int lwork );
public static native int LAPACKE_ssytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
float[] a, int lda, int[] ipiv,
float[] work, int lwork );
public static native int LAPACKE_dsytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoublePointer a, int lda, IntPointer ipiv,
DoublePointer work, int lwork );
public static native int LAPACKE_dsytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
DoubleBuffer a, int lda, IntBuffer ipiv,
DoubleBuffer work, int lwork );
public static native int LAPACKE_dsytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
double[] a, int lda, int[] ipiv,
double[] work, int lwork );
public static native int LAPACKE_csytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer work,
int lwork );
public static native int LAPACKE_csytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork );
public static native int LAPACKE_csytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv, @Cast("lapack_complex_float*") float[] work,
int lwork );
public static native int LAPACKE_zsytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer work,
int lwork );
public static native int LAPACKE_zsytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork );
public static native int LAPACKE_zsytrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv, @Cast("lapack_complex_double*") double[] work,
int lwork );
public static native int LAPACKE_ssytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatPointer a, int lda,
@Const IntPointer ipiv, FloatPointer b,
int ldb );
public static native int LAPACKE_ssytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const FloatBuffer a, int lda,
@Const IntBuffer ipiv, FloatBuffer b,
int ldb );
public static native int LAPACKE_ssytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const float[] a, int lda,
@Const int[] ipiv, float[] b,
int ldb );
public static native int LAPACKE_dsytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoublePointer a,
int lda, @Const IntPointer ipiv,
DoublePointer b, int ldb );
public static native int LAPACKE_dsytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
DoubleBuffer b, int ldb );
public static native int LAPACKE_dsytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Const double[] a,
int lda, @Const int[] ipiv,
double[] b, int ldb );
public static native int LAPACKE_csytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_csytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_csytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zsytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zsytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zsytrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_chetrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatPointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer work,
int lwork );
public static native int LAPACKE_chetrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") FloatBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer work,
int lwork );
public static native int LAPACKE_chetrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_float*") float[] a, int lda,
int[] ipiv, @Cast("lapack_complex_float*") float[] work,
int lwork );
public static native int LAPACKE_zhetrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoublePointer a, int lda,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer work,
int lwork );
public static native int LAPACKE_zhetrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") DoubleBuffer a, int lda,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer work,
int lwork );
public static native int LAPACKE_zhetrf_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("lapack_complex_double*") double[] a, int lda,
int[] ipiv, @Cast("lapack_complex_double*") double[] work,
int lwork );
public static native int LAPACKE_chetrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, int ldb );
public static native int LAPACKE_chetrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, int ldb );
public static native int LAPACKE_chetrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_float*") float[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int ldb );
public static native int LAPACKE_zhetrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
int lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, int ldb );
public static native int LAPACKE_zhetrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
int lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, int ldb );
public static native int LAPACKE_zhetrs_rook_work( int matrix_layout, @Cast("char") byte uplo, int n,
int nrhs, @Cast("const lapack_complex_double*") double[] a,
int lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int ldb );
public static native int LAPACKE_csyr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("const lapack_complex_float*") FloatPointer x,
int incx, @Cast("lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_csyr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("const lapack_complex_float*") FloatBuffer x,
int incx, @Cast("lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_csyr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_float*") float[] alpha,
@Cast("const lapack_complex_float*") float[] x,
int incx, @Cast("lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_zsyr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("const lapack_complex_double*") DoublePointer x,
int incx, @Cast("lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zsyr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("const lapack_complex_double*") DoubleBuffer x,
int incx, @Cast("lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zsyr_work( int matrix_layout, @Cast("char") byte uplo, int n,
@ByVal @Cast("lapack_complex_double*") double[] alpha,
@Cast("const lapack_complex_double*") double[] x,
int incx, @Cast("lapack_complex_double*") double[] a,
int lda );
public static native void LAPACKE_ilaver( @Const IntPointer vers_major,
@Const IntPointer vers_minor,
@Const IntPointer vers_patch );
public static native void LAPACKE_ilaver( @Const IntBuffer vers_major,
@Const IntBuffer vers_minor,
@Const IntBuffer vers_patch );
public static native void LAPACKE_ilaver( @Const int[] vers_major,
@Const int[] vers_minor,
@Const int[] vers_patch );
// LAPACK 3.3.0
// LAPACK 3.4.0
// LAPACK 3.5.0
// LAPACK 3.6.0
public static native void LAPACK_sgetrf( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_sgetrf( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_sgetrf( int[] m, int[] n, float[] a, int[] lda,
int[] ipiv, int[] info );
public static native void LAPACK_dgetrf( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_dgetrf( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_dgetrf( int[] m, int[] n, double[] a, int[] lda,
int[] ipiv, int[] info );
public static native void LAPACK_cgetrf( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer ipiv, IntPointer info );
public static native void LAPACK_cgetrf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_cgetrf( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] ipiv, int[] info );
public static native void LAPACK_zgetrf( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ipiv, IntPointer info );
public static native void LAPACK_zgetrf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_zgetrf( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ipiv, int[] info );
public static native void LAPACK_sgetrf2( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_sgetrf2( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_sgetrf2( int[] m, int[] n, float[] a, int[] lda,
int[] ipiv, int[] info );
public static native void LAPACK_dgetrf2( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_dgetrf2( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_dgetrf2( int[] m, int[] n, double[] a, int[] lda,
int[] ipiv, int[] info );
public static native void LAPACK_cgetrf2( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer ipiv, IntPointer info );
public static native void LAPACK_cgetrf2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_cgetrf2( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] ipiv, int[] info );
public static native void LAPACK_zgetrf2( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ipiv, IntPointer info );
public static native void LAPACK_zgetrf2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_zgetrf2( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ipiv, int[] info );
public static native void LAPACK_sgbtrf( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, FloatPointer ab, IntPointer ldab,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_sgbtrf( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, FloatBuffer ab, IntBuffer ldab,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_sgbtrf( int[] m, int[] n, int[] kl,
int[] ku, float[] ab, int[] ldab,
int[] ipiv, int[] info );
public static native void LAPACK_dgbtrf( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, DoublePointer ab, IntPointer ldab,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_dgbtrf( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, DoubleBuffer ab, IntBuffer ldab,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_dgbtrf( int[] m, int[] n, int[] kl,
int[] ku, double[] ab, int[] ldab,
int[] ipiv, int[] info );
public static native void LAPACK_cgbtrf( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_cgbtrf( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_cgbtrf( int[] m, int[] n, int[] kl,
int[] ku, @Cast("lapack_complex_float*") float[] ab, int[] ldab,
int[] ipiv, int[] info );
public static native void LAPACK_zgbtrf( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_zgbtrf( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_zgbtrf( int[] m, int[] n, int[] kl,
int[] ku, @Cast("lapack_complex_double*") double[] ab, int[] ldab,
int[] ipiv, int[] info );
public static native void LAPACK_sgttrf( IntPointer n, FloatPointer dl, FloatPointer d, FloatPointer du, FloatPointer du2,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_sgttrf( IntBuffer n, FloatBuffer dl, FloatBuffer d, FloatBuffer du, FloatBuffer du2,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_sgttrf( int[] n, float[] dl, float[] d, float[] du, float[] du2,
int[] ipiv, int[] info );
public static native void LAPACK_dgttrf( IntPointer n, DoublePointer dl, DoublePointer d, DoublePointer du,
DoublePointer du2, IntPointer ipiv, IntPointer info );
public static native void LAPACK_dgttrf( IntBuffer n, DoubleBuffer dl, DoubleBuffer d, DoubleBuffer du,
DoubleBuffer du2, IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_dgttrf( int[] n, double[] dl, double[] d, double[] du,
double[] du2, int[] ipiv, int[] info );
public static native void LAPACK_cgttrf( IntPointer n, @Cast("lapack_complex_float*") FloatPointer dl,
@Cast("lapack_complex_float*") FloatPointer d, @Cast("lapack_complex_float*") FloatPointer du,
@Cast("lapack_complex_float*") FloatPointer du2, IntPointer ipiv,
IntPointer info );
public static native void LAPACK_cgttrf( IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer dl,
@Cast("lapack_complex_float*") FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer du,
@Cast("lapack_complex_float*") FloatBuffer du2, IntBuffer ipiv,
IntBuffer info );
public static native void LAPACK_cgttrf( int[] n, @Cast("lapack_complex_float*") float[] dl,
@Cast("lapack_complex_float*") float[] d, @Cast("lapack_complex_float*") float[] du,
@Cast("lapack_complex_float*") float[] du2, int[] ipiv,
int[] info );
public static native void LAPACK_zgttrf( IntPointer n, @Cast("lapack_complex_double*") DoublePointer dl,
@Cast("lapack_complex_double*") DoublePointer d, @Cast("lapack_complex_double*") DoublePointer du,
@Cast("lapack_complex_double*") DoublePointer du2, IntPointer ipiv,
IntPointer info );
public static native void LAPACK_zgttrf( IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer dl,
@Cast("lapack_complex_double*") DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer du,
@Cast("lapack_complex_double*") DoubleBuffer du2, IntBuffer ipiv,
IntBuffer info );
public static native void LAPACK_zgttrf( int[] n, @Cast("lapack_complex_double*") double[] dl,
@Cast("lapack_complex_double*") double[] d, @Cast("lapack_complex_double*") double[] du,
@Cast("lapack_complex_double*") double[] du2, int[] ipiv,
int[] info );
public static native void LAPACK_spotrf2( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_spotrf2( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_spotrf2( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
int[] info );
public static native void LAPACK_dpotrf2( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_dpotrf2( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_dpotrf2( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
int[] info );
public static native void LAPACK_cpotrf2( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_cpotrf2( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_cpotrf2( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] info );
public static native void LAPACK_zpotrf2( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_zpotrf2( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_zpotrf2( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] info );
public static native void LAPACK_spotrf( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_spotrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_spotrf( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
int[] info );
public static native void LAPACK_dpotrf( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_dpotrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_dpotrf( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
int[] info );
public static native void LAPACK_cpotrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_cpotrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_cpotrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] info );
public static native void LAPACK_zpotrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_zpotrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_zpotrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] info );
public static native void LAPACK_dpstrf( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer piv, IntPointer rank, DoublePointer tol,
DoublePointer work, IntPointer info );
public static native void LAPACK_dpstrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer piv, IntBuffer rank, DoubleBuffer tol,
DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dpstrf( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
int[] piv, int[] rank, double[] tol,
double[] work, int[] info );
public static native void LAPACK_spstrf( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer piv, IntPointer rank, FloatPointer tol, FloatPointer work,
IntPointer info );
public static native void LAPACK_spstrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer piv, IntBuffer rank, FloatBuffer tol, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_spstrf( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
int[] piv, int[] rank, float[] tol, float[] work,
int[] info );
public static native void LAPACK_zpstrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer piv, IntPointer rank,
DoublePointer tol, DoublePointer work, IntPointer info );
public static native void LAPACK_zpstrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer piv, IntBuffer rank,
DoubleBuffer tol, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zpstrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] piv, int[] rank,
double[] tol, double[] work, int[] info );
public static native void LAPACK_cpstrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer piv, IntPointer rank,
FloatPointer tol, FloatPointer work, IntPointer info );
public static native void LAPACK_cpstrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer piv, IntBuffer rank,
FloatBuffer tol, FloatBuffer work, IntBuffer info );
public static native void LAPACK_cpstrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] piv, int[] rank,
float[] tol, float[] work, int[] info );
public static native void LAPACK_dpftrf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a,
IntPointer info );
public static native void LAPACK_dpftrf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a,
IntBuffer info );
public static native void LAPACK_dpftrf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, double[] a,
int[] info );
public static native void LAPACK_spftrf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a,
IntPointer info );
public static native void LAPACK_spftrf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a,
IntBuffer info );
public static native void LAPACK_spftrf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, float[] a,
int[] info );
public static native void LAPACK_zpftrf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer info );
public static native void LAPACK_zpftrf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer info );
public static native void LAPACK_zpftrf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] info );
public static native void LAPACK_cpftrf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer info );
public static native void LAPACK_cpftrf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer info );
public static native void LAPACK_cpftrf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] info );
public static native void LAPACK_spptrf( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer ap, IntPointer info );
public static native void LAPACK_spptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer ap, IntBuffer info );
public static native void LAPACK_spptrf( @Cast("char*") byte[] uplo, int[] n, float[] ap, int[] info );
public static native void LAPACK_dpptrf( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer ap, IntPointer info );
public static native void LAPACK_dpptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer ap, IntBuffer info );
public static native void LAPACK_dpptrf( @Cast("char*") byte[] uplo, int[] n, double[] ap, int[] info );
public static native void LAPACK_cpptrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer info );
public static native void LAPACK_cpptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer info );
public static native void LAPACK_cpptrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] ap,
int[] info );
public static native void LAPACK_zpptrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer info );
public static native void LAPACK_zpptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer info );
public static native void LAPACK_zpptrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] ap,
int[] info );
public static native void LAPACK_spbtrf( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, FloatPointer ab,
IntPointer ldab, IntPointer info );
public static native void LAPACK_spbtrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, FloatBuffer ab,
IntBuffer ldab, IntBuffer info );
public static native void LAPACK_spbtrf( @Cast("char*") byte[] uplo, int[] n, int[] kd, float[] ab,
int[] ldab, int[] info );
public static native void LAPACK_dpbtrf( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, DoublePointer ab,
IntPointer ldab, IntPointer info );
public static native void LAPACK_dpbtrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, DoubleBuffer ab,
IntBuffer ldab, IntBuffer info );
public static native void LAPACK_dpbtrf( @Cast("char*") byte[] uplo, int[] n, int[] kd, double[] ab,
int[] ldab, int[] info );
public static native void LAPACK_cpbtrf( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab,
IntPointer info );
public static native void LAPACK_cpbtrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
IntBuffer info );
public static native void LAPACK_cpbtrf( @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("lapack_complex_float*") float[] ab, int[] ldab,
int[] info );
public static native void LAPACK_zpbtrf( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab,
IntPointer info );
public static native void LAPACK_zpbtrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
IntBuffer info );
public static native void LAPACK_zpbtrf( @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("lapack_complex_double*") double[] ab, int[] ldab,
int[] info );
public static native void LAPACK_spttrf( IntPointer n, FloatPointer d, FloatPointer e, IntPointer info );
public static native void LAPACK_spttrf( IntBuffer n, FloatBuffer d, FloatBuffer e, IntBuffer info );
public static native void LAPACK_spttrf( int[] n, float[] d, float[] e, int[] info );
public static native void LAPACK_dpttrf( IntPointer n, DoublePointer d, DoublePointer e, IntPointer info );
public static native void LAPACK_dpttrf( IntBuffer n, DoubleBuffer d, DoubleBuffer e, IntBuffer info );
public static native void LAPACK_dpttrf( int[] n, double[] d, double[] e, int[] info );
public static native void LAPACK_cpttrf( IntPointer n, FloatPointer d, @Cast("lapack_complex_float*") FloatPointer e,
IntPointer info );
public static native void LAPACK_cpttrf( IntBuffer n, FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer e,
IntBuffer info );
public static native void LAPACK_cpttrf( int[] n, float[] d, @Cast("lapack_complex_float*") float[] e,
int[] info );
public static native void LAPACK_zpttrf( IntPointer n, DoublePointer d, @Cast("lapack_complex_double*") DoublePointer e,
IntPointer info );
public static native void LAPACK_zpttrf( IntBuffer n, DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer e,
IntBuffer info );
public static native void LAPACK_zpttrf( int[] n, double[] d, @Cast("lapack_complex_double*") double[] e,
int[] info );
public static native void LAPACK_ssytrf( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer ipiv, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_ssytrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer ipiv, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_ssytrf( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
int[] ipiv, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dsytrf( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer ipiv, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dsytrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer ipiv, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dsytrf( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
int[] ipiv, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_csytrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_csytrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_csytrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zsytrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zsytrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zsytrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_chetrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_chetrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_chetrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zhetrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zhetrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zhetrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_ssptrf( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer ap, IntPointer ipiv,
IntPointer info );
public static native void LAPACK_ssptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer ap, IntBuffer ipiv,
IntBuffer info );
public static native void LAPACK_ssptrf( @Cast("char*") byte[] uplo, int[] n, float[] ap, int[] ipiv,
int[] info );
public static native void LAPACK_dsptrf( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer ap, IntPointer ipiv,
IntPointer info );
public static native void LAPACK_dsptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer ap, IntBuffer ipiv,
IntBuffer info );
public static native void LAPACK_dsptrf( @Cast("char*") byte[] uplo, int[] n, double[] ap, int[] ipiv,
int[] info );
public static native void LAPACK_csptrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_csptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_csptrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] ap,
int[] ipiv, int[] info );
public static native void LAPACK_zsptrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_zsptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_zsptrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] ap,
int[] ipiv, int[] info );
public static native void LAPACK_chptrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_chptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_chptrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] ap,
int[] ipiv, int[] info );
public static native void LAPACK_zhptrf( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_zhptrf( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_zhptrf( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] ap,
int[] ipiv, int[] info );
public static native void LAPACK_sgetrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const FloatPointer a, IntPointer lda, @Const IntPointer ipiv,
FloatPointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_sgetrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer a, IntBuffer lda, @Const IntBuffer ipiv,
FloatBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_sgetrs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const float[] a, int[] lda, @Const int[] ipiv,
float[] b, int[] ldb, int[] info );
public static native void LAPACK_dgetrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const DoublePointer a, IntPointer lda, @Const IntPointer ipiv,
DoublePointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_dgetrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer a, IntBuffer lda, @Const IntBuffer ipiv,
DoubleBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dgetrs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const double[] a, int[] lda, @Const int[] ipiv,
double[] b, int[] ldb, int[] info );
public static native void LAPACK_cgetrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_cgetrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cgetrs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zgetrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zgetrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zgetrs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_sgbtrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Const FloatPointer ab, IntPointer ldab,
@Const IntPointer ipiv, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_sgbtrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Const FloatBuffer ab, IntBuffer ldab,
@Const IntBuffer ipiv, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_sgbtrs( @Cast("char*") byte[] trans, int[] n, int[] kl, int[] ku,
int[] nrhs, @Const float[] ab, int[] ldab,
@Const int[] ipiv, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dgbtrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Const DoublePointer ab, IntPointer ldab,
@Const IntPointer ipiv, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dgbtrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Const DoubleBuffer ab, IntBuffer ldab,
@Const IntBuffer ipiv, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dgbtrs( @Cast("char*") byte[] trans, int[] n, int[] kl, int[] ku,
int[] nrhs, @Const double[] ab, int[] ldab,
@Const int[] ipiv, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_cgbtrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Cast("const lapack_complex_float*") FloatPointer ab,
IntPointer ldab, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_cgbtrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Cast("const lapack_complex_float*") FloatBuffer ab,
IntBuffer ldab, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_cgbtrs( @Cast("char*") byte[] trans, int[] n, int[] kl, int[] ku,
int[] nrhs, @Cast("const lapack_complex_float*") float[] ab,
int[] ldab, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_zgbtrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Cast("const lapack_complex_double*") DoublePointer ab,
IntPointer ldab, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zgbtrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zgbtrs( @Cast("char*") byte[] trans, int[] n, int[] kl, int[] ku,
int[] nrhs, @Cast("const lapack_complex_double*") double[] ab,
int[] ldab, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_sgttrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const FloatPointer dl, @Const FloatPointer d, @Const FloatPointer du,
@Const FloatPointer du2, @Const IntPointer ipiv, FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_sgttrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer dl, @Const FloatBuffer d, @Const FloatBuffer du,
@Const FloatBuffer du2, @Const IntBuffer ipiv, FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_sgttrs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const float[] dl, @Const float[] d, @Const float[] du,
@Const float[] du2, @Const int[] ipiv, float[] b,
int[] ldb, int[] info );
public static native void LAPACK_dgttrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const DoublePointer dl, @Const DoublePointer d, @Const DoublePointer du,
@Const DoublePointer du2, @Const IntPointer ipiv, DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_dgttrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer dl, @Const DoubleBuffer d, @Const DoubleBuffer du,
@Const DoubleBuffer du2, @Const IntBuffer ipiv, DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dgttrs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const double[] dl, @Const double[] d, @Const double[] du,
@Const double[] du2, @Const int[] ipiv, double[] b,
int[] ldb, int[] info );
public static native void LAPACK_cgttrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("const lapack_complex_float*") FloatPointer du2, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_cgttrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("const lapack_complex_float*") FloatBuffer du2, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_cgttrs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("const lapack_complex_float*") float[] du2, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_zgttrs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("const lapack_complex_double*") DoublePointer du2, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zgttrs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("const lapack_complex_double*") DoubleBuffer du2, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zgttrs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("const lapack_complex_double*") double[] du2, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_spotrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, @Const FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_spotrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, @Const FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_spotrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, @Const float[] a,
int[] lda, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dpotrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer a, IntPointer lda, DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_dpotrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer a, IntBuffer lda, DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dpotrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] a, int[] lda, double[] b,
int[] ldb, int[] info );
public static native void LAPACK_cpotrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_cpotrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_cpotrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_zpotrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zpotrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zpotrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_dpftrs( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer a, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dpftrs( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer a, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dpftrs( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] a, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_spftrs( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const FloatPointer a, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_spftrs( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer a, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_spftrs( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const float[] a, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_zpftrs( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zpftrs( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zpftrs( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_cpftrs( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_cpftrs( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cpftrs( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_spptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const FloatPointer ap, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_spptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer ap, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_spptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const float[] ap, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dpptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer ap, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dpptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer ap, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dpptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] ap, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_cpptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_cpptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cpptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ap, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zpptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zpptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zpptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ap, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_spbtrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Const FloatPointer ab, IntPointer ldab, FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_spbtrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Const FloatBuffer ab, IntBuffer ldab, FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_spbtrs( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Const float[] ab, int[] ldab, float[] b,
int[] ldb, int[] info );
public static native void LAPACK_dpbtrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Const DoublePointer ab, IntPointer ldab, DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_dpbtrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Const DoubleBuffer ab, IntBuffer ldab, DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dpbtrs( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Const double[] ab, int[] ldab, double[] b,
int[] ldb, int[] info );
public static native void LAPACK_cpbtrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_cpbtrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_cpbtrs( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ab, int[] ldab,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_zpbtrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zpbtrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zpbtrs( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ab, int[] ldab,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_spttrs( IntPointer n, IntPointer nrhs, @Const FloatPointer d,
@Const FloatPointer e, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_spttrs( IntBuffer n, IntBuffer nrhs, @Const FloatBuffer d,
@Const FloatBuffer e, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_spttrs( int[] n, int[] nrhs, @Const float[] d,
@Const float[] e, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dpttrs( IntPointer n, IntPointer nrhs, @Const DoublePointer d,
@Const DoublePointer e, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dpttrs( IntBuffer n, IntBuffer nrhs, @Const DoubleBuffer d,
@Const DoubleBuffer e, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dpttrs( int[] n, int[] nrhs, @Const double[] d,
@Const double[] e, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_cpttrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_cpttrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cpttrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zpttrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer d, @Cast("const lapack_complex_double*") DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zpttrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer d, @Cast("const lapack_complex_double*") DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zpttrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] d, @Cast("const lapack_complex_double*") double[] e,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_ssytrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, @Const FloatPointer a,
IntPointer lda, @Const IntPointer ipiv, FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_ssytrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, @Const FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv, FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_ssytrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, @Const float[] a,
int[] lda, @Const int[] ipiv, float[] b,
int[] ldb, int[] info );
public static native void LAPACK_dsytrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer a, IntPointer lda, @Const IntPointer ipiv,
DoublePointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_dsytrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer a, IntBuffer lda, @Const IntBuffer ipiv,
DoubleBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dsytrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] a, int[] lda, @Const int[] ipiv,
double[] b, int[] ldb, int[] info );
public static native void LAPACK_csytrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_csytrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_csytrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zsytrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zsytrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zsytrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_chetrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_chetrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_chetrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zhetrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zhetrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zhetrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_ssptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const FloatPointer ap, @Const IntPointer ipiv, FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_ssptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer ap, @Const IntBuffer ipiv, FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_ssptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const float[] ap, @Const int[] ipiv, float[] b,
int[] ldb, int[] info );
public static native void LAPACK_dsptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer ap, @Const IntPointer ipiv, DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_dsptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer ap, @Const IntBuffer ipiv, DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dsptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] ap, @Const int[] ipiv, double[] b,
int[] ldb, int[] info );
public static native void LAPACK_csptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_csptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_csptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ap, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_zsptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zsptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zsptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ap, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_chptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_chptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_chptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ap, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_zhptrs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zhptrs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zhptrs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ap, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_strtrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Const FloatPointer a, IntPointer lda, FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_strtrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Const FloatBuffer a, IntBuffer lda, FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_strtrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Const float[] a, int[] lda, float[] b,
int[] ldb, int[] info );
public static native void LAPACK_dtrtrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Const DoublePointer a, IntPointer lda,
DoublePointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_dtrtrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Const DoubleBuffer a, IntBuffer lda,
DoubleBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dtrtrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Const double[] a, int[] lda,
double[] b, int[] ldb, int[] info );
public static native void LAPACK_ctrtrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_ctrtrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_ctrtrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_ztrtrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_ztrtrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_ztrtrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_stptrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Const FloatPointer ap, FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_stptrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Const FloatBuffer ap, FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_stptrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Const float[] ap, float[] b,
int[] ldb, int[] info );
public static native void LAPACK_dtptrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Const DoublePointer ap, DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_dtptrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Const DoubleBuffer ap, DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dtptrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Const double[] ap, double[] b,
int[] ldb, int[] info );
public static native void LAPACK_ctptrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_ctptrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_ctptrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_ztptrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_ztptrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_ztptrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_stbtrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, IntPointer nrhs, @Const FloatPointer ab,
IntPointer ldab, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_stbtrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, IntBuffer nrhs, @Const FloatBuffer ab,
IntBuffer ldab, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_stbtrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] kd, int[] nrhs, @Const float[] ab,
int[] ldab, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dtbtrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, IntPointer nrhs, @Const DoublePointer ab,
IntPointer ldab, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dtbtrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, IntBuffer nrhs, @Const DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dtbtrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] kd, int[] nrhs, @Const double[] ab,
int[] ldab, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_ctbtrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_ctbtrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_ctbtrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] kd, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ab, int[] ldab,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_ztbtrs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_ztbtrs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_ztbtrs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] kd, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ab, int[] ldab,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_sgecon( @Cast("char*") BytePointer norm, IntPointer n, @Const FloatPointer a, IntPointer lda,
FloatPointer anorm, FloatPointer rcond, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sgecon( @Cast("char*") ByteBuffer norm, IntBuffer n, @Const FloatBuffer a, IntBuffer lda,
FloatBuffer anorm, FloatBuffer rcond, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sgecon( @Cast("char*") byte[] norm, int[] n, @Const float[] a, int[] lda,
float[] anorm, float[] rcond, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dgecon( @Cast("char*") BytePointer norm, IntPointer n, @Const DoublePointer a, IntPointer lda,
DoublePointer anorm, DoublePointer rcond, DoublePointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dgecon( @Cast("char*") ByteBuffer norm, IntBuffer n, @Const DoubleBuffer a, IntBuffer lda,
DoubleBuffer anorm, DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgecon( @Cast("char*") byte[] norm, int[] n, @Const double[] a, int[] lda,
double[] anorm, double[] rcond, double[] work,
int[] iwork, int[] info );
public static native void LAPACK_cgecon( @Cast("char*") BytePointer norm, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer anorm, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cgecon( @Cast("char*") ByteBuffer norm, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer anorm, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cgecon( @Cast("char*") byte[] norm, int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, float[] anorm, float[] rcond,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zgecon( @Cast("char*") BytePointer norm, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer anorm, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zgecon( @Cast("char*") ByteBuffer norm, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer anorm, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zgecon( @Cast("char*") byte[] norm, int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, double[] anorm, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sgbcon( @Cast("char*") BytePointer norm, IntPointer n, IntPointer kl, IntPointer ku,
@Const FloatPointer ab, IntPointer ldab, @Const IntPointer ipiv,
FloatPointer anorm, FloatPointer rcond, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sgbcon( @Cast("char*") ByteBuffer norm, IntBuffer n, IntBuffer kl, IntBuffer ku,
@Const FloatBuffer ab, IntBuffer ldab, @Const IntBuffer ipiv,
FloatBuffer anorm, FloatBuffer rcond, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sgbcon( @Cast("char*") byte[] norm, int[] n, int[] kl, int[] ku,
@Const float[] ab, int[] ldab, @Const int[] ipiv,
float[] anorm, float[] rcond, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dgbcon( @Cast("char*") BytePointer norm, IntPointer n, IntPointer kl, IntPointer ku,
@Const DoublePointer ab, IntPointer ldab, @Const IntPointer ipiv,
DoublePointer anorm, DoublePointer rcond, DoublePointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dgbcon( @Cast("char*") ByteBuffer norm, IntBuffer n, IntBuffer kl, IntBuffer ku,
@Const DoubleBuffer ab, IntBuffer ldab, @Const IntBuffer ipiv,
DoubleBuffer anorm, DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgbcon( @Cast("char*") byte[] norm, int[] n, int[] kl, int[] ku,
@Const double[] ab, int[] ldab, @Const int[] ipiv,
double[] anorm, double[] rcond, double[] work,
int[] iwork, int[] info );
public static native void LAPACK_cgbcon( @Cast("char*") BytePointer norm, IntPointer n, IntPointer kl, IntPointer ku,
@Cast("const lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Const IntPointer ipiv, FloatPointer anorm, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cgbcon( @Cast("char*") ByteBuffer norm, IntBuffer n, IntBuffer kl, IntBuffer ku,
@Cast("const lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Const IntBuffer ipiv, FloatBuffer anorm, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cgbcon( @Cast("char*") byte[] norm, int[] n, int[] kl, int[] ku,
@Cast("const lapack_complex_float*") float[] ab, int[] ldab,
@Const int[] ipiv, float[] anorm, float[] rcond,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zgbcon( @Cast("char*") BytePointer norm, IntPointer n, IntPointer kl, IntPointer ku,
@Cast("const lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Const IntPointer ipiv, DoublePointer anorm, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zgbcon( @Cast("char*") ByteBuffer norm, IntBuffer n, IntBuffer kl, IntBuffer ku,
@Cast("const lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Const IntBuffer ipiv, DoubleBuffer anorm, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zgbcon( @Cast("char*") byte[] norm, int[] n, int[] kl, int[] ku,
@Cast("const lapack_complex_double*") double[] ab, int[] ldab,
@Const int[] ipiv, double[] anorm, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sgtcon( @Cast("char*") BytePointer norm, IntPointer n, @Const FloatPointer dl, @Const FloatPointer d,
@Const FloatPointer du, @Const FloatPointer du2, @Const IntPointer ipiv,
FloatPointer anorm, FloatPointer rcond, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sgtcon( @Cast("char*") ByteBuffer norm, IntBuffer n, @Const FloatBuffer dl, @Const FloatBuffer d,
@Const FloatBuffer du, @Const FloatBuffer du2, @Const IntBuffer ipiv,
FloatBuffer anorm, FloatBuffer rcond, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sgtcon( @Cast("char*") byte[] norm, int[] n, @Const float[] dl, @Const float[] d,
@Const float[] du, @Const float[] du2, @Const int[] ipiv,
float[] anorm, float[] rcond, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dgtcon( @Cast("char*") BytePointer norm, IntPointer n, @Const DoublePointer dl,
@Const DoublePointer d, @Const DoublePointer du, @Const DoublePointer du2,
@Const IntPointer ipiv, DoublePointer anorm, DoublePointer rcond,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dgtcon( @Cast("char*") ByteBuffer norm, IntBuffer n, @Const DoubleBuffer dl,
@Const DoubleBuffer d, @Const DoubleBuffer du, @Const DoubleBuffer du2,
@Const IntBuffer ipiv, DoubleBuffer anorm, DoubleBuffer rcond,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgtcon( @Cast("char*") byte[] norm, int[] n, @Const double[] dl,
@Const double[] d, @Const double[] du, @Const double[] du2,
@Const int[] ipiv, double[] anorm, double[] rcond,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_cgtcon( @Cast("char*") BytePointer norm, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("const lapack_complex_float*") FloatPointer du2, @Const IntPointer ipiv,
FloatPointer anorm, FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer info );
public static native void LAPACK_cgtcon( @Cast("char*") ByteBuffer norm, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("const lapack_complex_float*") FloatBuffer du2, @Const IntBuffer ipiv,
FloatBuffer anorm, FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer info );
public static native void LAPACK_cgtcon( @Cast("char*") byte[] norm, int[] n, @Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("const lapack_complex_float*") float[] du2, @Const int[] ipiv,
float[] anorm, float[] rcond, @Cast("lapack_complex_float*") float[] work,
int[] info );
public static native void LAPACK_zgtcon( @Cast("char*") BytePointer norm, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("const lapack_complex_double*") DoublePointer du2, @Const IntPointer ipiv,
DoublePointer anorm, DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer info );
public static native void LAPACK_zgtcon( @Cast("char*") ByteBuffer norm, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("const lapack_complex_double*") DoubleBuffer du2, @Const IntBuffer ipiv,
DoubleBuffer anorm, DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zgtcon( @Cast("char*") byte[] norm, int[] n, @Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("const lapack_complex_double*") double[] du2, @Const int[] ipiv,
double[] anorm, double[] rcond, @Cast("lapack_complex_double*") double[] work,
int[] info );
public static native void LAPACK_spocon( @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer a, IntPointer lda,
FloatPointer anorm, FloatPointer rcond, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_spocon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer a, IntBuffer lda,
FloatBuffer anorm, FloatBuffer rcond, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_spocon( @Cast("char*") byte[] uplo, int[] n, @Const float[] a, int[] lda,
float[] anorm, float[] rcond, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dpocon( @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer a, IntPointer lda,
DoublePointer anorm, DoublePointer rcond, DoublePointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dpocon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer a, IntBuffer lda,
DoubleBuffer anorm, DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dpocon( @Cast("char*") byte[] uplo, int[] n, @Const double[] a, int[] lda,
double[] anorm, double[] rcond, double[] work,
int[] iwork, int[] info );
public static native void LAPACK_cpocon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer anorm, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cpocon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer anorm, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cpocon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, float[] anorm, float[] rcond,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zpocon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer anorm, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zpocon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer anorm, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zpocon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, double[] anorm, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sppcon( @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer ap, FloatPointer anorm,
FloatPointer rcond, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sppcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer ap, FloatBuffer anorm,
FloatBuffer rcond, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sppcon( @Cast("char*") byte[] uplo, int[] n, @Const float[] ap, float[] anorm,
float[] rcond, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dppcon( @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer ap, DoublePointer anorm,
DoublePointer rcond, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dppcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer ap, DoubleBuffer anorm,
DoubleBuffer rcond, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dppcon( @Cast("char*") byte[] uplo, int[] n, @Const double[] ap, double[] anorm,
double[] rcond, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_cppcon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer ap,
FloatPointer anorm, FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_cppcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer ap,
FloatBuffer anorm, FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cppcon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] ap,
float[] anorm, float[] rcond, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zppcon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer ap,
DoublePointer anorm, DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zppcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
DoubleBuffer anorm, DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zppcon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] ap,
double[] anorm, double[] rcond, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_spbcon( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, @Const FloatPointer ab,
IntPointer ldab, FloatPointer anorm, FloatPointer rcond, FloatPointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_spbcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, @Const FloatBuffer ab,
IntBuffer ldab, FloatBuffer anorm, FloatBuffer rcond, FloatBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_spbcon( @Cast("char*") byte[] uplo, int[] n, int[] kd, @Const float[] ab,
int[] ldab, float[] anorm, float[] rcond, float[] work,
int[] iwork, int[] info );
public static native void LAPACK_dpbcon( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, @Const DoublePointer ab,
IntPointer ldab, DoublePointer anorm, DoublePointer rcond,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dpbcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, @Const DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer anorm, DoubleBuffer rcond,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dpbcon( @Cast("char*") byte[] uplo, int[] n, int[] kd, @Const double[] ab,
int[] ldab, double[] anorm, double[] rcond,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_cpbcon( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("const lapack_complex_float*") FloatPointer ab, IntPointer ldab,
FloatPointer anorm, FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_cpbcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("const lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
FloatBuffer anorm, FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cpbcon( @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("const lapack_complex_float*") float[] ab, int[] ldab,
float[] anorm, float[] rcond, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zpbcon( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("const lapack_complex_double*") DoublePointer ab, IntPointer ldab,
DoublePointer anorm, DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zpbcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("const lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
DoubleBuffer anorm, DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zpbcon( @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("const lapack_complex_double*") double[] ab, int[] ldab,
double[] anorm, double[] rcond, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_sptcon( IntPointer n, @Const FloatPointer d, @Const FloatPointer e, FloatPointer anorm,
FloatPointer rcond, FloatPointer work, IntPointer info );
public static native void LAPACK_sptcon( IntBuffer n, @Const FloatBuffer d, @Const FloatBuffer e, FloatBuffer anorm,
FloatBuffer rcond, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sptcon( int[] n, @Const float[] d, @Const float[] e, float[] anorm,
float[] rcond, float[] work, int[] info );
public static native void LAPACK_dptcon( IntPointer n, @Const DoublePointer d, @Const DoublePointer e,
DoublePointer anorm, DoublePointer rcond, DoublePointer work,
IntPointer info );
public static native void LAPACK_dptcon( IntBuffer n, @Const DoubleBuffer d, @Const DoubleBuffer e,
DoubleBuffer anorm, DoubleBuffer rcond, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dptcon( int[] n, @Const double[] d, @Const double[] e,
double[] anorm, double[] rcond, double[] work,
int[] info );
public static native void LAPACK_cptcon( IntPointer n, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, FloatPointer anorm, FloatPointer rcond,
FloatPointer work, IntPointer info );
public static native void LAPACK_cptcon( IntBuffer n, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, FloatBuffer anorm, FloatBuffer rcond,
FloatBuffer work, IntBuffer info );
public static native void LAPACK_cptcon( int[] n, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, float[] anorm, float[] rcond,
float[] work, int[] info );
public static native void LAPACK_zptcon( IntPointer n, @Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e, DoublePointer anorm,
DoublePointer rcond, DoublePointer work, IntPointer info );
public static native void LAPACK_zptcon( IntBuffer n, @Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e, DoubleBuffer anorm,
DoubleBuffer rcond, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zptcon( int[] n, @Const double[] d,
@Cast("const lapack_complex_double*") double[] e, double[] anorm,
double[] rcond, double[] work, int[] info );
public static native void LAPACK_ssycon( @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, FloatPointer anorm, FloatPointer rcond,
FloatPointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_ssycon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, FloatBuffer anorm, FloatBuffer rcond,
FloatBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_ssycon( @Cast("char*") byte[] uplo, int[] n, @Const float[] a, int[] lda,
@Const int[] ipiv, float[] anorm, float[] rcond,
float[] work, int[] iwork, int[] info );
public static native void LAPACK_dsycon( @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, DoublePointer anorm, DoublePointer rcond,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dsycon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, DoubleBuffer anorm, DoubleBuffer rcond,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dsycon( @Cast("char*") byte[] uplo, int[] n, @Const double[] a, int[] lda,
@Const int[] ipiv, double[] anorm, double[] rcond,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_csycon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Const IntPointer ipiv, FloatPointer anorm,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer info );
public static native void LAPACK_csycon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv, FloatBuffer anorm,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer info );
public static native void LAPACK_csycon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Const int[] ipiv, float[] anorm,
float[] rcond, @Cast("lapack_complex_float*") float[] work,
int[] info );
public static native void LAPACK_zsycon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Const IntPointer ipiv, DoublePointer anorm,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer info );
public static native void LAPACK_zsycon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Const IntBuffer ipiv, DoubleBuffer anorm,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zsycon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Const int[] ipiv, double[] anorm,
double[] rcond, @Cast("lapack_complex_double*") double[] work,
int[] info );
public static native void LAPACK_checon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Const IntPointer ipiv, FloatPointer anorm,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer info );
public static native void LAPACK_checon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv, FloatBuffer anorm,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer info );
public static native void LAPACK_checon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Const int[] ipiv, float[] anorm,
float[] rcond, @Cast("lapack_complex_float*") float[] work,
int[] info );
public static native void LAPACK_zhecon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Const IntPointer ipiv, DoublePointer anorm,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer info );
public static native void LAPACK_zhecon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Const IntBuffer ipiv, DoubleBuffer anorm,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zhecon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Const int[] ipiv, double[] anorm,
double[] rcond, @Cast("lapack_complex_double*") double[] work,
int[] info );
public static native void LAPACK_sspcon( @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer ap,
@Const IntPointer ipiv, FloatPointer anorm, FloatPointer rcond,
FloatPointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_sspcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer ap,
@Const IntBuffer ipiv, FloatBuffer anorm, FloatBuffer rcond,
FloatBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sspcon( @Cast("char*") byte[] uplo, int[] n, @Const float[] ap,
@Const int[] ipiv, float[] anorm, float[] rcond,
float[] work, int[] iwork, int[] info );
public static native void LAPACK_dspcon( @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer ap,
@Const IntPointer ipiv, DoublePointer anorm, DoublePointer rcond,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dspcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer ap,
@Const IntBuffer ipiv, DoubleBuffer anorm, DoubleBuffer rcond,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dspcon( @Cast("char*") byte[] uplo, int[] n, @Const double[] ap,
@Const int[] ipiv, double[] anorm, double[] rcond,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_cspcon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, FloatPointer anorm, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_cspcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, FloatBuffer anorm, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_cspcon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, float[] anorm, float[] rcond,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zspcon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, DoublePointer anorm, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zspcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, DoubleBuffer anorm, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zspcon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv, double[] anorm, double[] rcond,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_chpcon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, FloatPointer anorm, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_chpcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, FloatBuffer anorm, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_chpcon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] ap,
@Const int[] ipiv, float[] anorm, float[] rcond,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zhpcon( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, DoublePointer anorm, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zhpcon( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, DoubleBuffer anorm, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zhpcon( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] ap,
@Const int[] ipiv, double[] anorm, double[] rcond,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_strcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Const FloatPointer a, IntPointer lda, FloatPointer rcond, FloatPointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_strcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Const FloatBuffer a, IntBuffer lda, FloatBuffer rcond, FloatBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_strcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Const float[] a, int[] lda, float[] rcond, float[] work,
int[] iwork, int[] info );
public static native void LAPACK_dtrcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Const DoublePointer a, IntPointer lda, DoublePointer rcond,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dtrcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Const DoubleBuffer a, IntBuffer lda, DoubleBuffer rcond,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dtrcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Const double[] a, int[] lda, double[] rcond,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_ctrcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_ctrcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_ctrcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
float[] rcond, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_ztrcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
DoublePointer rcond, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_ztrcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
DoubleBuffer rcond, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_ztrcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
double[] rcond, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_stpcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Const FloatPointer ap, FloatPointer rcond, FloatPointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_stpcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Const FloatBuffer ap, FloatBuffer rcond, FloatBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_stpcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Const float[] ap, float[] rcond, float[] work,
int[] iwork, int[] info );
public static native void LAPACK_dtpcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Const DoublePointer ap, DoublePointer rcond, DoublePointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dtpcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Const DoubleBuffer ap, DoubleBuffer rcond, DoubleBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dtpcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Const double[] ap, double[] rcond, double[] work,
int[] iwork, int[] info );
public static native void LAPACK_ctpcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer ap, FloatPointer rcond,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_ctpcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer ap, FloatBuffer rcond,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_ctpcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("const lapack_complex_float*") float[] ap, float[] rcond,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_ztpcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer ap, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_ztpcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer ap, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_ztpcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("const lapack_complex_double*") double[] ap, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_stbcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, @Const FloatPointer ab, IntPointer ldab,
FloatPointer rcond, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_stbcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, @Const FloatBuffer ab, IntBuffer ldab,
FloatBuffer rcond, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_stbcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
int[] kd, @Const float[] ab, int[] ldab,
float[] rcond, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dtbcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, @Const DoublePointer ab, IntPointer ldab,
DoublePointer rcond, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dtbcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, @Const DoubleBuffer ab, IntBuffer ldab,
DoubleBuffer rcond, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dtbcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
int[] kd, @Const double[] ab, int[] ldab,
double[] rcond, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_ctbcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, @Cast("const lapack_complex_float*") FloatPointer ab,
IntPointer ldab, FloatPointer rcond, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_ctbcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, @Cast("const lapack_complex_float*") FloatBuffer ab,
IntBuffer ldab, FloatBuffer rcond, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_ctbcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
int[] kd, @Cast("const lapack_complex_float*") float[] ab,
int[] ldab, float[] rcond, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_ztbcon( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, @Cast("const lapack_complex_double*") DoublePointer ab,
IntPointer ldab, DoublePointer rcond,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_ztbcon( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, @Cast("const lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer rcond,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_ztbcon( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
int[] kd, @Cast("const lapack_complex_double*") double[] ab,
int[] ldab, double[] rcond,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sgerfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const FloatPointer a, IntPointer lda, @Const FloatPointer af,
IntPointer ldaf, @Const IntPointer ipiv, @Const FloatPointer b,
IntPointer ldb, FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sgerfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer a, IntBuffer lda, @Const FloatBuffer af,
IntBuffer ldaf, @Const IntBuffer ipiv, @Const FloatBuffer b,
IntBuffer ldb, FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sgerfs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const float[] a, int[] lda, @Const float[] af,
int[] ldaf, @Const int[] ipiv, @Const float[] b,
int[] ldb, float[] x, int[] ldx, float[] ferr,
float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dgerfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const DoublePointer a, IntPointer lda, @Const DoublePointer af,
IntPointer ldaf, @Const IntPointer ipiv, @Const DoublePointer b,
IntPointer ldb, DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dgerfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer a, IntBuffer lda, @Const DoubleBuffer af,
IntBuffer ldaf, @Const IntBuffer ipiv, @Const DoubleBuffer b,
IntBuffer ldb, DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dgerfs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const double[] a, int[] lda, @Const double[] af,
int[] ldaf, @Const int[] ipiv, @Const double[] b,
int[] ldb, double[] x, int[] ldx, double[] ferr,
double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_cgerfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer af, IntPointer ldaf,
@Const IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
FloatPointer ferr, FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_cgerfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer af, IntBuffer ldaf,
@Const IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
FloatBuffer ferr, FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cgerfs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] af, int[] ldaf,
@Const int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] x, int[] ldx,
float[] ferr, float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zgerfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer af, IntPointer ldaf,
@Const IntPointer ipiv, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgerfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer af, IntBuffer ldaf,
@Const IntBuffer ipiv, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgerfs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] af, int[] ldaf,
@Const int[] ipiv, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_sgbrfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Const FloatPointer ab, IntPointer ldab,
@Const FloatPointer afb, IntPointer ldafb, @Const IntPointer ipiv,
@Const FloatPointer b, IntPointer ldb, FloatPointer x, IntPointer ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sgbrfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Const FloatBuffer ab, IntBuffer ldab,
@Const FloatBuffer afb, IntBuffer ldafb, @Const IntBuffer ipiv,
@Const FloatBuffer b, IntBuffer ldb, FloatBuffer x, IntBuffer ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sgbrfs( @Cast("char*") byte[] trans, int[] n, int[] kl, int[] ku,
int[] nrhs, @Const float[] ab, int[] ldab,
@Const float[] afb, int[] ldafb, @Const int[] ipiv,
@Const float[] b, int[] ldb, float[] x, int[] ldx,
float[] ferr, float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dgbrfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Const DoublePointer ab, IntPointer ldab,
@Const DoublePointer afb, IntPointer ldafb,
@Const IntPointer ipiv, @Const DoublePointer b, IntPointer ldb,
DoublePointer x, IntPointer ldx, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dgbrfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Const DoubleBuffer ab, IntBuffer ldab,
@Const DoubleBuffer afb, IntBuffer ldafb,
@Const IntBuffer ipiv, @Const DoubleBuffer b, IntBuffer ldb,
DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgbrfs( @Cast("char*") byte[] trans, int[] n, int[] kl, int[] ku,
int[] nrhs, @Const double[] ab, int[] ldab,
@Const double[] afb, int[] ldafb,
@Const int[] ipiv, @Const double[] b, int[] ldb,
double[] x, int[] ldx, double[] ferr, double[] berr,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_cgbrfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Cast("const lapack_complex_float*") FloatPointer ab,
IntPointer ldab, @Cast("const lapack_complex_float*") FloatPointer afb,
IntPointer ldafb, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cgbrfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Cast("const lapack_complex_float*") FloatBuffer ab,
IntBuffer ldab, @Cast("const lapack_complex_float*") FloatBuffer afb,
IntBuffer ldafb, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cgbrfs( @Cast("char*") byte[] trans, int[] n, int[] kl, int[] ku,
int[] nrhs, @Cast("const lapack_complex_float*") float[] ab,
int[] ldab, @Cast("const lapack_complex_float*") float[] afb,
int[] ldafb, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zgbrfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Cast("const lapack_complex_double*") DoublePointer ab,
IntPointer ldab, @Cast("const lapack_complex_double*") DoublePointer afb,
IntPointer ldafb, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zgbrfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, @Cast("const lapack_complex_double*") DoubleBuffer afb,
IntBuffer ldafb, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zgbrfs( @Cast("char*") byte[] trans, int[] n, int[] kl, int[] ku,
int[] nrhs, @Cast("const lapack_complex_double*") double[] ab,
int[] ldab, @Cast("const lapack_complex_double*") double[] afb,
int[] ldafb, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sgtrfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const FloatPointer dl, @Const FloatPointer d, @Const FloatPointer du,
@Const FloatPointer dlf, @Const FloatPointer df, @Const FloatPointer duf,
@Const FloatPointer du2, @Const IntPointer ipiv, @Const FloatPointer b,
IntPointer ldb, FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sgtrfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer dl, @Const FloatBuffer d, @Const FloatBuffer du,
@Const FloatBuffer dlf, @Const FloatBuffer df, @Const FloatBuffer duf,
@Const FloatBuffer du2, @Const IntBuffer ipiv, @Const FloatBuffer b,
IntBuffer ldb, FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sgtrfs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const float[] dl, @Const float[] d, @Const float[] du,
@Const float[] dlf, @Const float[] df, @Const float[] duf,
@Const float[] du2, @Const int[] ipiv, @Const float[] b,
int[] ldb, float[] x, int[] ldx, float[] ferr,
float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dgtrfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const DoublePointer dl, @Const DoublePointer d, @Const DoublePointer du,
@Const DoublePointer dlf, @Const DoublePointer df, @Const DoublePointer duf,
@Const DoublePointer du2, @Const IntPointer ipiv, @Const DoublePointer b,
IntPointer ldb, DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dgtrfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer dl, @Const DoubleBuffer d, @Const DoubleBuffer du,
@Const DoubleBuffer dlf, @Const DoubleBuffer df, @Const DoubleBuffer duf,
@Const DoubleBuffer du2, @Const IntBuffer ipiv, @Const DoubleBuffer b,
IntBuffer ldb, DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dgtrfs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const double[] dl, @Const double[] d, @Const double[] du,
@Const double[] dlf, @Const double[] df, @Const double[] duf,
@Const double[] du2, @Const int[] ipiv, @Const double[] b,
int[] ldb, double[] x, int[] ldx, double[] ferr,
double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_cgtrfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du,
@Cast("const lapack_complex_float*") FloatPointer dlf,
@Cast("const lapack_complex_float*") FloatPointer df,
@Cast("const lapack_complex_float*") FloatPointer duf,
@Cast("const lapack_complex_float*") FloatPointer du2, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cgtrfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du,
@Cast("const lapack_complex_float*") FloatBuffer dlf,
@Cast("const lapack_complex_float*") FloatBuffer df,
@Cast("const lapack_complex_float*") FloatBuffer duf,
@Cast("const lapack_complex_float*") FloatBuffer du2, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cgtrfs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du,
@Cast("const lapack_complex_float*") float[] dlf,
@Cast("const lapack_complex_float*") float[] df,
@Cast("const lapack_complex_float*") float[] duf,
@Cast("const lapack_complex_float*") float[] du2, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zgtrfs( @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du,
@Cast("const lapack_complex_double*") DoublePointer dlf,
@Cast("const lapack_complex_double*") DoublePointer df,
@Cast("const lapack_complex_double*") DoublePointer duf,
@Cast("const lapack_complex_double*") DoublePointer du2, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zgtrfs( @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du,
@Cast("const lapack_complex_double*") DoubleBuffer dlf,
@Cast("const lapack_complex_double*") DoubleBuffer df,
@Cast("const lapack_complex_double*") DoubleBuffer duf,
@Cast("const lapack_complex_double*") DoubleBuffer du2, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zgtrfs( @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du,
@Cast("const lapack_complex_double*") double[] dlf,
@Cast("const lapack_complex_double*") double[] df,
@Cast("const lapack_complex_double*") double[] duf,
@Cast("const lapack_complex_double*") double[] du2, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sporfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, @Const FloatPointer a,
IntPointer lda, @Const FloatPointer af, IntPointer ldaf,
@Const FloatPointer b, IntPointer ldb, FloatPointer x, IntPointer ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sporfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, @Const FloatBuffer a,
IntBuffer lda, @Const FloatBuffer af, IntBuffer ldaf,
@Const FloatBuffer b, IntBuffer ldb, FloatBuffer x, IntBuffer ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sporfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, @Const float[] a,
int[] lda, @Const float[] af, int[] ldaf,
@Const float[] b, int[] ldb, float[] x, int[] ldx,
float[] ferr, float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dporfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer a, IntPointer lda, @Const DoublePointer af,
IntPointer ldaf, @Const DoublePointer b, IntPointer ldb,
DoublePointer x, IntPointer ldx, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dporfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer a, IntBuffer lda, @Const DoubleBuffer af,
IntBuffer ldaf, @Const DoubleBuffer b, IntBuffer ldb,
DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dporfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] a, int[] lda, @Const double[] af,
int[] ldaf, @Const double[] b, int[] ldb,
double[] x, int[] ldx, double[] ferr, double[] berr,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_cporfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer af, IntPointer ldaf,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cporfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer af, IntBuffer ldaf,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cporfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] af, int[] ldaf,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zporfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer af, IntPointer ldaf,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zporfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer af, IntBuffer ldaf,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zporfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] af, int[] ldaf,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_spprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const FloatPointer ap, @Const FloatPointer afp, @Const FloatPointer b,
IntPointer ldb, FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_spprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer ap, @Const FloatBuffer afp, @Const FloatBuffer b,
IntBuffer ldb, FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_spprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const float[] ap, @Const float[] afp, @Const float[] b,
int[] ldb, float[] x, int[] ldx, float[] ferr,
float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dpprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer ap, @Const DoublePointer afp, @Const DoublePointer b,
IntPointer ldb, DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dpprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer ap, @Const DoubleBuffer afp, @Const DoubleBuffer b,
IntBuffer ldb, DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dpprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] ap, @Const double[] afp, @Const double[] b,
int[] ldb, double[] x, int[] ldx, double[] ferr,
double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_cpprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer afp,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cpprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer afp,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cpprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] afp,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zpprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer afp,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zpprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer afp,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zpprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] afp,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_spbrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Const FloatPointer ab, IntPointer ldab, @Const FloatPointer afb,
IntPointer ldafb, @Const FloatPointer b, IntPointer ldb,
FloatPointer x, IntPointer ldx, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_spbrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Const FloatBuffer ab, IntBuffer ldab, @Const FloatBuffer afb,
IntBuffer ldafb, @Const FloatBuffer b, IntBuffer ldb,
FloatBuffer x, IntBuffer ldx, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_spbrfs( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Const float[] ab, int[] ldab, @Const float[] afb,
int[] ldafb, @Const float[] b, int[] ldb,
float[] x, int[] ldx, float[] ferr, float[] berr,
float[] work, int[] iwork, int[] info );
public static native void LAPACK_dpbrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Const DoublePointer ab, IntPointer ldab, @Const DoublePointer afb,
IntPointer ldafb, @Const DoublePointer b, IntPointer ldb,
DoublePointer x, IntPointer ldx, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dpbrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Const DoubleBuffer ab, IntBuffer ldab, @Const DoubleBuffer afb,
IntBuffer ldafb, @Const DoubleBuffer b, IntBuffer ldb,
DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dpbrfs( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Const double[] ab, int[] ldab, @Const double[] afb,
int[] ldafb, @Const double[] b, int[] ldb,
double[] x, int[] ldx, double[] ferr, double[] berr,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_cpbrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Cast("const lapack_complex_float*") FloatPointer afb, IntPointer ldafb,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cpbrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Cast("const lapack_complex_float*") FloatBuffer afb, IntBuffer ldafb,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cpbrfs( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ab, int[] ldab,
@Cast("const lapack_complex_float*") float[] afb, int[] ldafb,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zpbrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Cast("const lapack_complex_double*") DoublePointer afb, IntPointer ldafb,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zpbrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Cast("const lapack_complex_double*") DoubleBuffer afb, IntBuffer ldafb,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zpbrfs( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ab, int[] ldab,
@Cast("const lapack_complex_double*") double[] afb, int[] ldafb,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sptrfs( IntPointer n, IntPointer nrhs, @Const FloatPointer d,
@Const FloatPointer e, @Const FloatPointer df, @Const FloatPointer ef,
@Const FloatPointer b, IntPointer ldb, FloatPointer x, IntPointer ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer info );
public static native void LAPACK_sptrfs( IntBuffer n, IntBuffer nrhs, @Const FloatBuffer d,
@Const FloatBuffer e, @Const FloatBuffer df, @Const FloatBuffer ef,
@Const FloatBuffer b, IntBuffer ldb, FloatBuffer x, IntBuffer ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sptrfs( int[] n, int[] nrhs, @Const float[] d,
@Const float[] e, @Const float[] df, @Const float[] ef,
@Const float[] b, int[] ldb, float[] x, int[] ldx,
float[] ferr, float[] berr, float[] work, int[] info );
public static native void LAPACK_dptrfs( IntPointer n, IntPointer nrhs, @Const DoublePointer d,
@Const DoublePointer e, @Const DoublePointer df, @Const DoublePointer ef,
@Const DoublePointer b, IntPointer ldb, DoublePointer x,
IntPointer ldx, DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer info );
public static native void LAPACK_dptrfs( IntBuffer n, IntBuffer nrhs, @Const DoubleBuffer d,
@Const DoubleBuffer e, @Const DoubleBuffer df, @Const DoubleBuffer ef,
@Const DoubleBuffer b, IntBuffer ldb, DoubleBuffer x,
IntBuffer ldx, DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dptrfs( int[] n, int[] nrhs, @Const double[] d,
@Const double[] e, @Const double[] df, @Const double[] ef,
@Const double[] b, int[] ldb, double[] x,
int[] ldx, double[] ferr, double[] berr, double[] work,
int[] info );
public static native void LAPACK_cptrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, @Const FloatPointer df,
@Cast("const lapack_complex_float*") FloatPointer ef,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cptrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, @Const FloatBuffer df,
@Cast("const lapack_complex_float*") FloatBuffer ef,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cptrfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, @Const float[] df,
@Cast("const lapack_complex_float*") float[] ef,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zptrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer d, @Cast("const lapack_complex_double*") DoublePointer e,
@Const DoublePointer df, @Cast("const lapack_complex_double*") DoublePointer ef,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zptrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer d, @Cast("const lapack_complex_double*") DoubleBuffer e,
@Const DoubleBuffer df, @Cast("const lapack_complex_double*") DoubleBuffer ef,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zptrfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] d, @Cast("const lapack_complex_double*") double[] e,
@Const double[] df, @Cast("const lapack_complex_double*") double[] ef,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_ssyrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, @Const FloatPointer a,
IntPointer lda, @Const FloatPointer af, IntPointer ldaf,
@Const IntPointer ipiv, @Const FloatPointer b, IntPointer ldb,
FloatPointer x, IntPointer ldx, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_ssyrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, @Const FloatBuffer a,
IntBuffer lda, @Const FloatBuffer af, IntBuffer ldaf,
@Const IntBuffer ipiv, @Const FloatBuffer b, IntBuffer ldb,
FloatBuffer x, IntBuffer ldx, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_ssyrfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, @Const float[] a,
int[] lda, @Const float[] af, int[] ldaf,
@Const int[] ipiv, @Const float[] b, int[] ldb,
float[] x, int[] ldx, float[] ferr, float[] berr,
float[] work, int[] iwork, int[] info );
public static native void LAPACK_dsyrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer a, IntPointer lda, @Const DoublePointer af,
IntPointer ldaf, @Const IntPointer ipiv, @Const DoublePointer b,
IntPointer ldb, DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dsyrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer a, IntBuffer lda, @Const DoubleBuffer af,
IntBuffer ldaf, @Const IntBuffer ipiv, @Const DoubleBuffer b,
IntBuffer ldb, DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dsyrfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] a, int[] lda, @Const double[] af,
int[] ldaf, @Const int[] ipiv, @Const double[] b,
int[] ldb, double[] x, int[] ldx, double[] ferr,
double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_csyrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer af, IntPointer ldaf,
@Const IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
FloatPointer ferr, FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_csyrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer af, IntBuffer ldaf,
@Const IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
FloatBuffer ferr, FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_csyrfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] af, int[] ldaf,
@Const int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] x, int[] ldx,
float[] ferr, float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zsyrfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer af, IntPointer ldaf,
@Const IntPointer ipiv, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zsyrfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer af, IntBuffer ldaf,
@Const IntBuffer ipiv, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zsyrfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] af, int[] ldaf,
@Const int[] ipiv, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_cherfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer af, IntPointer ldaf,
@Const IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
FloatPointer ferr, FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_cherfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer af, IntBuffer ldaf,
@Const IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
FloatBuffer ferr, FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cherfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] af, int[] ldaf,
@Const int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] x, int[] ldx,
float[] ferr, float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zherfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer af, IntPointer ldaf,
@Const IntPointer ipiv, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zherfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer af, IntBuffer ldaf,
@Const IntBuffer ipiv, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zherfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] af, int[] ldaf,
@Const int[] ipiv, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_ssprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const FloatPointer ap, @Const FloatPointer afp, @Const IntPointer ipiv,
@Const FloatPointer b, IntPointer ldb, FloatPointer x, IntPointer ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_ssprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer ap, @Const FloatBuffer afp, @Const IntBuffer ipiv,
@Const FloatBuffer b, IntBuffer ldb, FloatBuffer x, IntBuffer ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_ssprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const float[] ap, @Const float[] afp, @Const int[] ipiv,
@Const float[] b, int[] ldb, float[] x, int[] ldx,
float[] ferr, float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dsprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer ap, @Const DoublePointer afp, @Const IntPointer ipiv,
@Const DoublePointer b, IntPointer ldb, DoublePointer x,
IntPointer ldx, DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dsprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer ap, @Const DoubleBuffer afp, @Const IntBuffer ipiv,
@Const DoubleBuffer b, IntBuffer ldb, DoubleBuffer x,
IntBuffer ldx, DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dsprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] ap, @Const double[] afp, @Const int[] ipiv,
@Const double[] b, int[] ldb, double[] x,
int[] ldx, double[] ferr, double[] berr, double[] work,
int[] iwork, int[] info );
public static native void LAPACK_csprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer afp, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_csprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer afp, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_csprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] afp, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zsprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer afp, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zsprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer afp, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zsprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] afp, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_chprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer afp, @Const IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_chprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer afp, @Const IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_chprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] afp, @Const int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zhprfs( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer afp, @Const IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zhprfs( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer afp, @Const IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zhprfs( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] afp, @Const int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] ferr,
double[] berr, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_strrfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Const FloatPointer a, IntPointer lda,
@Const FloatPointer b, IntPointer ldb, @Const FloatPointer x,
IntPointer ldx, FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_strrfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Const FloatBuffer a, IntBuffer lda,
@Const FloatBuffer b, IntBuffer ldb, @Const FloatBuffer x,
IntBuffer ldx, FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_strrfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Const float[] a, int[] lda,
@Const float[] b, int[] ldb, @Const float[] x,
int[] ldx, float[] ferr, float[] berr, float[] work,
int[] iwork, int[] info );
public static native void LAPACK_dtrrfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Const DoublePointer a, IntPointer lda,
@Const DoublePointer b, IntPointer ldb, @Const DoublePointer x,
IntPointer ldx, DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dtrrfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Const DoubleBuffer a, IntBuffer lda,
@Const DoubleBuffer b, IntBuffer ldb, @Const DoubleBuffer x,
IntBuffer ldx, DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dtrrfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Const double[] a, int[] lda,
@Const double[] b, int[] ldb, @Const double[] x,
int[] ldx, double[] ferr, double[] berr, double[] work,
int[] iwork, int[] info );
public static native void LAPACK_ctrrfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("const lapack_complex_float*") FloatPointer x,
IntPointer ldx, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_ctrrfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("const lapack_complex_float*") FloatBuffer x,
IntBuffer ldx, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_ctrrfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("const lapack_complex_float*") float[] x,
int[] ldx, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_ztrrfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("const lapack_complex_double*") DoublePointer x,
IntPointer ldx, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_ztrrfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("const lapack_complex_double*") DoubleBuffer x,
IntBuffer ldx, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_ztrrfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("const lapack_complex_double*") double[] x,
int[] ldx, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_stprfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Const FloatPointer ap, @Const FloatPointer b,
IntPointer ldb, @Const FloatPointer x, IntPointer ldx,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_stprfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Const FloatBuffer ap, @Const FloatBuffer b,
IntBuffer ldb, @Const FloatBuffer x, IntBuffer ldx,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_stprfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Const float[] ap, @Const float[] b,
int[] ldb, @Const float[] x, int[] ldx,
float[] ferr, float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dtprfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Const DoublePointer ap, @Const DoublePointer b,
IntPointer ldb, @Const DoublePointer x, IntPointer ldx,
DoublePointer ferr, DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dtprfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Const DoubleBuffer ap, @Const DoubleBuffer b,
IntBuffer ldb, @Const DoubleBuffer x, IntBuffer ldx,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dtprfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Const double[] ap, @Const double[] b,
int[] ldb, @Const double[] x, int[] ldx,
double[] ferr, double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_ctprfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("const lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_ctprfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("const lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_ctprfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("const lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_ztprfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("const lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_ztprfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("const lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_ztprfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] nrhs, @Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("const lapack_complex_double*") double[] x, int[] ldx,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_stbrfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, IntPointer nrhs, @Const FloatPointer ab,
IntPointer ldab, @Const FloatPointer b, IntPointer ldb,
@Const FloatPointer x, IntPointer ldx, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_stbrfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, IntBuffer nrhs, @Const FloatBuffer ab,
IntBuffer ldab, @Const FloatBuffer b, IntBuffer ldb,
@Const FloatBuffer x, IntBuffer ldx, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_stbrfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] kd, int[] nrhs, @Const float[] ab,
int[] ldab, @Const float[] b, int[] ldb,
@Const float[] x, int[] ldx, float[] ferr, float[] berr,
float[] work, int[] iwork, int[] info );
public static native void LAPACK_dtbrfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, IntPointer nrhs, @Const DoublePointer ab,
IntPointer ldab, @Const DoublePointer b, IntPointer ldb,
@Const DoublePointer x, IntPointer ldx, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dtbrfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, IntBuffer nrhs, @Const DoubleBuffer ab,
IntBuffer ldab, @Const DoubleBuffer b, IntBuffer ldb,
@Const DoubleBuffer x, IntBuffer ldx, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dtbrfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] kd, int[] nrhs, @Const double[] ab,
int[] ldab, @Const double[] b, int[] ldb,
@Const double[] x, int[] ldx, double[] ferr,
double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_ctbrfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("const lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer ferr,
FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_ctbrfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("const lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer ferr,
FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_ctbrfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] kd, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ab, int[] ldab,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("const lapack_complex_float*") float[] x, int[] ldx, float[] ferr,
float[] berr, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_ztbrfs( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer diag, IntPointer n,
IntPointer kd, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("const lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_ztbrfs( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer diag, IntBuffer n,
IntBuffer kd, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("const lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_ztbrfs( @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, @Cast("char*") byte[] diag, int[] n,
int[] kd, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ab, int[] ldab,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("const lapack_complex_double*") double[] x, int[] ldx,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_sgetri( IntPointer n, FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sgetri( IntBuffer n, FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sgetri( int[] n, float[] a, int[] lda,
@Const int[] ipiv, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dgetri( IntPointer n, DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dgetri( IntBuffer n, DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dgetri( int[] n, double[] a, int[] lda,
@Const int[] ipiv, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cgetri( IntPointer n, @Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cgetri( IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cgetri( int[] n, @Cast("lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zgetri( IntPointer n, @Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zgetri( IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zgetri( int[] n, @Cast("lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_spotri( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_spotri( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_spotri( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
int[] info );
public static native void LAPACK_dpotri( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_dpotri( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_dpotri( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
int[] info );
public static native void LAPACK_cpotri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_cpotri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_cpotri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] info );
public static native void LAPACK_zpotri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_zpotri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_zpotri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] info );
public static native void LAPACK_dpftri( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a,
IntPointer info );
public static native void LAPACK_dpftri( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a,
IntBuffer info );
public static native void LAPACK_dpftri( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, double[] a,
int[] info );
public static native void LAPACK_spftri( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a,
IntPointer info );
public static native void LAPACK_spftri( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a,
IntBuffer info );
public static native void LAPACK_spftri( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, float[] a,
int[] info );
public static native void LAPACK_zpftri( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer info );
public static native void LAPACK_zpftri( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer info );
public static native void LAPACK_zpftri( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] info );
public static native void LAPACK_cpftri( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer info );
public static native void LAPACK_cpftri( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer info );
public static native void LAPACK_cpftri( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] info );
public static native void LAPACK_spptri( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer ap, IntPointer info );
public static native void LAPACK_spptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer ap, IntBuffer info );
public static native void LAPACK_spptri( @Cast("char*") byte[] uplo, int[] n, float[] ap, int[] info );
public static native void LAPACK_dpptri( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer ap, IntPointer info );
public static native void LAPACK_dpptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer ap, IntBuffer info );
public static native void LAPACK_dpptri( @Cast("char*") byte[] uplo, int[] n, double[] ap, int[] info );
public static native void LAPACK_cpptri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer info );
public static native void LAPACK_cpptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer info );
public static native void LAPACK_cpptri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] ap,
int[] info );
public static native void LAPACK_zpptri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer info );
public static native void LAPACK_zpptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer info );
public static native void LAPACK_zpptri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] ap,
int[] info );
public static native void LAPACK_ssytri( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, FloatPointer work, IntPointer info );
public static native void LAPACK_ssytri( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, FloatBuffer work, IntBuffer info );
public static native void LAPACK_ssytri( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
@Const int[] ipiv, float[] work, int[] info );
public static native void LAPACK_dsytri( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, DoublePointer work, IntPointer info );
public static native void LAPACK_dsytri( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dsytri( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
@Const int[] ipiv, double[] work, int[] info );
public static native void LAPACK_csytri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_csytri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_csytri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zsytri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zsytri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zsytri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_chetri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_chetri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_chetri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zhetri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zhetri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zhetri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_ssptri( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer ap,
@Const IntPointer ipiv, FloatPointer work, IntPointer info );
public static native void LAPACK_ssptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer ap,
@Const IntBuffer ipiv, FloatBuffer work, IntBuffer info );
public static native void LAPACK_ssptri( @Cast("char*") byte[] uplo, int[] n, float[] ap,
@Const int[] ipiv, float[] work, int[] info );
public static native void LAPACK_dsptri( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer ap,
@Const IntPointer ipiv, DoublePointer work, IntPointer info );
public static native void LAPACK_dsptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer ap,
@Const IntBuffer ipiv, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dsptri( @Cast("char*") byte[] uplo, int[] n, double[] ap,
@Const int[] ipiv, double[] work, int[] info );
public static native void LAPACK_csptri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer info );
public static native void LAPACK_csptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer info );
public static native void LAPACK_csptri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] ap,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] work,
int[] info );
public static native void LAPACK_zsptri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer info );
public static native void LAPACK_zsptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zsptri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] ap,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] work,
int[] info );
public static native void LAPACK_chptri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer info );
public static native void LAPACK_chptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer info );
public static native void LAPACK_chptri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] ap,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] work,
int[] info );
public static native void LAPACK_zhptri( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer ap,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer info );
public static native void LAPACK_zhptri( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zhptri( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] ap,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] work,
int[] info );
public static native void LAPACK_strtri( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n, FloatPointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_strtri( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n, FloatBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_strtri( @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n, float[] a,
int[] lda, int[] info );
public static native void LAPACK_dtrtri( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n, DoublePointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_dtrtri( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n, DoubleBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_dtrtri( @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n, double[] a,
int[] lda, int[] info );
public static native void LAPACK_ctrtri( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_ctrtri( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_ctrtri( @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
int[] info );
public static native void LAPACK_ztrtri( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_ztrtri( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_ztrtri( @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
int[] info );
public static native void LAPACK_dtftri( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
DoublePointer a, IntPointer info );
public static native void LAPACK_dtftri( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
DoubleBuffer a, IntBuffer info );
public static native void LAPACK_dtftri( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
double[] a, int[] info );
public static native void LAPACK_stftri( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
FloatPointer a, IntPointer info );
public static native void LAPACK_stftri( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
FloatBuffer a, IntBuffer info );
public static native void LAPACK_stftri( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
float[] a, int[] info );
public static native void LAPACK_ztftri( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer info );
public static native void LAPACK_ztftri( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer info );
public static native void LAPACK_ztftri( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] info );
public static native void LAPACK_ctftri( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer info );
public static native void LAPACK_ctftri( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer info );
public static native void LAPACK_ctftri( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] info );
public static native void LAPACK_stptri( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n, FloatPointer ap,
IntPointer info );
public static native void LAPACK_stptri( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n, FloatBuffer ap,
IntBuffer info );
public static native void LAPACK_stptri( @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n, float[] ap,
int[] info );
public static native void LAPACK_dtptri( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n, DoublePointer ap,
IntPointer info );
public static native void LAPACK_dtptri( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n, DoubleBuffer ap,
IntBuffer info );
public static native void LAPACK_dtptri( @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n, double[] ap,
int[] info );
public static native void LAPACK_ctptri( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer ap, IntPointer info );
public static native void LAPACK_ctptri( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer ap, IntBuffer info );
public static native void LAPACK_ctptri( @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("lapack_complex_float*") float[] ap, int[] info );
public static native void LAPACK_ztptri( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer ap, IntPointer info );
public static native void LAPACK_ztptri( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer ap, IntBuffer info );
public static native void LAPACK_ztptri( @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] n,
@Cast("lapack_complex_double*") double[] ap, int[] info );
public static native void LAPACK_sgeequ( IntPointer m, IntPointer n, @Const FloatPointer a,
IntPointer lda, FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax, IntPointer info );
public static native void LAPACK_sgeequ( IntBuffer m, IntBuffer n, @Const FloatBuffer a,
IntBuffer lda, FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_sgeequ( int[] m, int[] n, @Const float[] a,
int[] lda, float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax, int[] info );
public static native void LAPACK_dgeequ( IntPointer m, IntPointer n, @Const DoublePointer a,
IntPointer lda, DoublePointer r, DoublePointer c, DoublePointer rowcnd,
DoublePointer colcnd, DoublePointer amax, IntPointer info );
public static native void LAPACK_dgeequ( IntBuffer m, IntBuffer n, @Const DoubleBuffer a,
IntBuffer lda, DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd,
DoubleBuffer colcnd, DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_dgeequ( int[] m, int[] n, @Const double[] a,
int[] lda, double[] r, double[] c, double[] rowcnd,
double[] colcnd, double[] amax, int[] info );
public static native void LAPACK_cgeequ( IntPointer m, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax, IntPointer info );
public static native void LAPACK_cgeequ( IntBuffer m, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_cgeequ( int[] m, int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax, int[] info );
public static native void LAPACK_zgeequ( IntPointer m, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer r,
DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd, DoublePointer amax,
IntPointer info );
public static native void LAPACK_zgeequ( IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer r,
DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd, DoubleBuffer amax,
IntBuffer info );
public static native void LAPACK_zgeequ( int[] m, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda, double[] r,
double[] c, double[] rowcnd, double[] colcnd, double[] amax,
int[] info );
public static native void LAPACK_dgeequb( IntPointer m, IntPointer n, @Const DoublePointer a,
IntPointer lda, DoublePointer r, DoublePointer c, DoublePointer rowcnd,
DoublePointer colcnd, DoublePointer amax, IntPointer info );
public static native void LAPACK_dgeequb( IntBuffer m, IntBuffer n, @Const DoubleBuffer a,
IntBuffer lda, DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd,
DoubleBuffer colcnd, DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_dgeequb( int[] m, int[] n, @Const double[] a,
int[] lda, double[] r, double[] c, double[] rowcnd,
double[] colcnd, double[] amax, int[] info );
public static native void LAPACK_sgeequb( IntPointer m, IntPointer n, @Const FloatPointer a,
IntPointer lda, FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax, IntPointer info );
public static native void LAPACK_sgeequb( IntBuffer m, IntBuffer n, @Const FloatBuffer a,
IntBuffer lda, FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_sgeequb( int[] m, int[] n, @Const float[] a,
int[] lda, float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax, int[] info );
public static native void LAPACK_zgeequb( IntPointer m, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer r,
DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd, DoublePointer amax,
IntPointer info );
public static native void LAPACK_zgeequb( IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer r,
DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd, DoubleBuffer amax,
IntBuffer info );
public static native void LAPACK_zgeequb( int[] m, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda, double[] r,
double[] c, double[] rowcnd, double[] colcnd, double[] amax,
int[] info );
public static native void LAPACK_cgeequb( IntPointer m, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer r,
FloatPointer c, FloatPointer rowcnd, FloatPointer colcnd, FloatPointer amax,
IntPointer info );
public static native void LAPACK_cgeequb( IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer r,
FloatBuffer c, FloatBuffer rowcnd, FloatBuffer colcnd, FloatBuffer amax,
IntBuffer info );
public static native void LAPACK_cgeequb( int[] m, int[] n,
@Cast("const lapack_complex_float*") float[] a, int[] lda, float[] r,
float[] c, float[] rowcnd, float[] colcnd, float[] amax,
int[] info );
public static native void LAPACK_sgbequ( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Const FloatPointer ab, IntPointer ldab, FloatPointer r,
FloatPointer c, FloatPointer rowcnd, FloatPointer colcnd, FloatPointer amax,
IntPointer info );
public static native void LAPACK_sgbequ( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Const FloatBuffer ab, IntBuffer ldab, FloatBuffer r,
FloatBuffer c, FloatBuffer rowcnd, FloatBuffer colcnd, FloatBuffer amax,
IntBuffer info );
public static native void LAPACK_sgbequ( int[] m, int[] n, int[] kl,
int[] ku, @Const float[] ab, int[] ldab, float[] r,
float[] c, float[] rowcnd, float[] colcnd, float[] amax,
int[] info );
public static native void LAPACK_dgbequ( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Const DoublePointer ab, IntPointer ldab,
DoublePointer r, DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd,
DoublePointer amax, IntPointer info );
public static native void LAPACK_dgbequ( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Const DoubleBuffer ab, IntBuffer ldab,
DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd,
DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_dgbequ( int[] m, int[] n, int[] kl,
int[] ku, @Const double[] ab, int[] ldab,
double[] r, double[] c, double[] rowcnd, double[] colcnd,
double[] amax, int[] info );
public static native void LAPACK_cgbequ( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Cast("const lapack_complex_float*") FloatPointer ab,
IntPointer ldab, FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax, IntPointer info );
public static native void LAPACK_cgbequ( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Cast("const lapack_complex_float*") FloatBuffer ab,
IntBuffer ldab, FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_cgbequ( int[] m, int[] n, int[] kl,
int[] ku, @Cast("const lapack_complex_float*") float[] ab,
int[] ldab, float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax, int[] info );
public static native void LAPACK_zgbequ( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Cast("const lapack_complex_double*") DoublePointer ab,
IntPointer ldab, DoublePointer r, DoublePointer c, DoublePointer rowcnd,
DoublePointer colcnd, DoublePointer amax, IntPointer info );
public static native void LAPACK_zgbequ( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Cast("const lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd,
DoubleBuffer colcnd, DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_zgbequ( int[] m, int[] n, int[] kl,
int[] ku, @Cast("const lapack_complex_double*") double[] ab,
int[] ldab, double[] r, double[] c, double[] rowcnd,
double[] colcnd, double[] amax, int[] info );
public static native void LAPACK_dgbequb( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Const DoublePointer ab, IntPointer ldab,
DoublePointer r, DoublePointer c, DoublePointer rowcnd, DoublePointer colcnd,
DoublePointer amax, IntPointer info );
public static native void LAPACK_dgbequb( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Const DoubleBuffer ab, IntBuffer ldab,
DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd, DoubleBuffer colcnd,
DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_dgbequb( int[] m, int[] n, int[] kl,
int[] ku, @Const double[] ab, int[] ldab,
double[] r, double[] c, double[] rowcnd, double[] colcnd,
double[] amax, int[] info );
public static native void LAPACK_sgbequb( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Const FloatPointer ab, IntPointer ldab,
FloatPointer r, FloatPointer c, FloatPointer rowcnd, FloatPointer colcnd,
FloatPointer amax, IntPointer info );
public static native void LAPACK_sgbequb( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Const FloatBuffer ab, IntBuffer ldab,
FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd, FloatBuffer colcnd,
FloatBuffer amax, IntBuffer info );
public static native void LAPACK_sgbequb( int[] m, int[] n, int[] kl,
int[] ku, @Const float[] ab, int[] ldab,
float[] r, float[] c, float[] rowcnd, float[] colcnd,
float[] amax, int[] info );
public static native void LAPACK_zgbequb( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Cast("const lapack_complex_double*") DoublePointer ab,
IntPointer ldab, DoublePointer r, DoublePointer c, DoublePointer rowcnd,
DoublePointer colcnd, DoublePointer amax, IntPointer info );
public static native void LAPACK_zgbequb( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Cast("const lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer r, DoubleBuffer c, DoubleBuffer rowcnd,
DoubleBuffer colcnd, DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_zgbequb( int[] m, int[] n, int[] kl,
int[] ku, @Cast("const lapack_complex_double*") double[] ab,
int[] ldab, double[] r, double[] c, double[] rowcnd,
double[] colcnd, double[] amax, int[] info );
public static native void LAPACK_cgbequb( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Cast("const lapack_complex_float*") FloatPointer ab,
IntPointer ldab, FloatPointer r, FloatPointer c, FloatPointer rowcnd,
FloatPointer colcnd, FloatPointer amax, IntPointer info );
public static native void LAPACK_cgbequb( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Cast("const lapack_complex_float*") FloatBuffer ab,
IntBuffer ldab, FloatBuffer r, FloatBuffer c, FloatBuffer rowcnd,
FloatBuffer colcnd, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_cgbequb( int[] m, int[] n, int[] kl,
int[] ku, @Cast("const lapack_complex_float*") float[] ab,
int[] ldab, float[] r, float[] c, float[] rowcnd,
float[] colcnd, float[] amax, int[] info );
public static native void LAPACK_spoequ( IntPointer n, @Const FloatPointer a, IntPointer lda, FloatPointer s,
FloatPointer scond, FloatPointer amax, IntPointer info );
public static native void LAPACK_spoequ( IntBuffer n, @Const FloatBuffer a, IntBuffer lda, FloatBuffer s,
FloatBuffer scond, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_spoequ( int[] n, @Const float[] a, int[] lda, float[] s,
float[] scond, float[] amax, int[] info );
public static native void LAPACK_dpoequ( IntPointer n, @Const DoublePointer a, IntPointer lda, DoublePointer s,
DoublePointer scond, DoublePointer amax, IntPointer info );
public static native void LAPACK_dpoequ( IntBuffer n, @Const DoubleBuffer a, IntBuffer lda, DoubleBuffer s,
DoubleBuffer scond, DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_dpoequ( int[] n, @Const double[] a, int[] lda, double[] s,
double[] scond, double[] amax, int[] info );
public static native void LAPACK_cpoequ( IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer s, FloatPointer scond, FloatPointer amax,
IntPointer info );
public static native void LAPACK_cpoequ( IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer s, FloatBuffer scond, FloatBuffer amax,
IntBuffer info );
public static native void LAPACK_cpoequ( int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, float[] s, float[] scond, float[] amax,
int[] info );
public static native void LAPACK_zpoequ( IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer s, DoublePointer scond, DoublePointer amax,
IntPointer info );
public static native void LAPACK_zpoequ( IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax,
IntBuffer info );
public static native void LAPACK_zpoequ( int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, double[] s, double[] scond, double[] amax,
int[] info );
public static native void LAPACK_dpoequb( IntPointer n, @Const DoublePointer a, IntPointer lda, DoublePointer s,
DoublePointer scond, DoublePointer amax, IntPointer info );
public static native void LAPACK_dpoequb( IntBuffer n, @Const DoubleBuffer a, IntBuffer lda, DoubleBuffer s,
DoubleBuffer scond, DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_dpoequb( int[] n, @Const double[] a, int[] lda, double[] s,
double[] scond, double[] amax, int[] info );
public static native void LAPACK_spoequb( IntPointer n, @Const FloatPointer a, IntPointer lda, FloatPointer s,
FloatPointer scond, FloatPointer amax, IntPointer info );
public static native void LAPACK_spoequb( IntBuffer n, @Const FloatBuffer a, IntBuffer lda, FloatBuffer s,
FloatBuffer scond, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_spoequb( int[] n, @Const float[] a, int[] lda, float[] s,
float[] scond, float[] amax, int[] info );
public static native void LAPACK_zpoequb( IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer s, DoublePointer scond, DoublePointer amax,
IntPointer info );
public static native void LAPACK_zpoequb( IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax,
IntBuffer info );
public static native void LAPACK_zpoequb( int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, double[] s, double[] scond, double[] amax,
int[] info );
public static native void LAPACK_cpoequb( IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer s, FloatPointer scond, FloatPointer amax,
IntPointer info );
public static native void LAPACK_cpoequb( IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer s, FloatBuffer scond, FloatBuffer amax,
IntBuffer info );
public static native void LAPACK_cpoequb( int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, float[] s, float[] scond, float[] amax,
int[] info );
public static native void LAPACK_sppequ( @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer ap, FloatPointer s,
FloatPointer scond, FloatPointer amax, IntPointer info );
public static native void LAPACK_sppequ( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer ap, FloatBuffer s,
FloatBuffer scond, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_sppequ( @Cast("char*") byte[] uplo, int[] n, @Const float[] ap, float[] s,
float[] scond, float[] amax, int[] info );
public static native void LAPACK_dppequ( @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer ap, DoublePointer s,
DoublePointer scond, DoublePointer amax, IntPointer info );
public static native void LAPACK_dppequ( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer ap, DoubleBuffer s,
DoubleBuffer scond, DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_dppequ( @Cast("char*") byte[] uplo, int[] n, @Const double[] ap, double[] s,
double[] scond, double[] amax, int[] info );
public static native void LAPACK_cppequ( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer ap,
FloatPointer s, FloatPointer scond, FloatPointer amax, IntPointer info );
public static native void LAPACK_cppequ( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer ap,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_cppequ( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] ap,
float[] s, float[] scond, float[] amax, int[] info );
public static native void LAPACK_zppequ( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer ap,
DoublePointer s, DoublePointer scond, DoublePointer amax, IntPointer info );
public static native void LAPACK_zppequ( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_zppequ( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] ap,
double[] s, double[] scond, double[] amax, int[] info );
public static native void LAPACK_spbequ( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, @Const FloatPointer ab,
IntPointer ldab, FloatPointer s, FloatPointer scond, FloatPointer amax,
IntPointer info );
public static native void LAPACK_spbequ( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, @Const FloatBuffer ab,
IntBuffer ldab, FloatBuffer s, FloatBuffer scond, FloatBuffer amax,
IntBuffer info );
public static native void LAPACK_spbequ( @Cast("char*") byte[] uplo, int[] n, int[] kd, @Const float[] ab,
int[] ldab, float[] s, float[] scond, float[] amax,
int[] info );
public static native void LAPACK_dpbequ( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, @Const DoublePointer ab,
IntPointer ldab, DoublePointer s, DoublePointer scond, DoublePointer amax,
IntPointer info );
public static native void LAPACK_dpbequ( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, @Const DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax,
IntBuffer info );
public static native void LAPACK_dpbequ( @Cast("char*") byte[] uplo, int[] n, int[] kd, @Const double[] ab,
int[] ldab, double[] s, double[] scond, double[] amax,
int[] info );
public static native void LAPACK_cpbequ( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("const lapack_complex_float*") FloatPointer ab, IntPointer ldab, FloatPointer s,
FloatPointer scond, FloatPointer amax, IntPointer info );
public static native void LAPACK_cpbequ( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("const lapack_complex_float*") FloatBuffer ab, IntBuffer ldab, FloatBuffer s,
FloatBuffer scond, FloatBuffer amax, IntBuffer info );
public static native void LAPACK_cpbequ( @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("const lapack_complex_float*") float[] ab, int[] ldab, float[] s,
float[] scond, float[] amax, int[] info );
public static native void LAPACK_zpbequ( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("const lapack_complex_double*") DoublePointer ab, IntPointer ldab,
DoublePointer s, DoublePointer scond, DoublePointer amax, IntPointer info );
public static native void LAPACK_zpbequ( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("const lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax, IntBuffer info );
public static native void LAPACK_zpbequ( @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("const lapack_complex_double*") double[] ab, int[] ldab,
double[] s, double[] scond, double[] amax, int[] info );
public static native void LAPACK_dsyequb( @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer a,
IntPointer lda, DoublePointer s, DoublePointer scond, DoublePointer amax,
DoublePointer work, IntPointer info );
public static native void LAPACK_dsyequb( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer a,
IntBuffer lda, DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax,
DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dsyequb( @Cast("char*") byte[] uplo, int[] n, @Const double[] a,
int[] lda, double[] s, double[] scond, double[] amax,
double[] work, int[] info );
public static native void LAPACK_ssyequb( @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer a, IntPointer lda,
FloatPointer s, FloatPointer scond, FloatPointer amax, FloatPointer work,
IntPointer info );
public static native void LAPACK_ssyequb( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer a, IntBuffer lda,
FloatBuffer s, FloatBuffer scond, FloatBuffer amax, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_ssyequb( @Cast("char*") byte[] uplo, int[] n, @Const float[] a, int[] lda,
float[] s, float[] scond, float[] amax, float[] work,
int[] info );
public static native void LAPACK_zsyequb( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer s, DoublePointer scond, DoublePointer amax,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zsyequb( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zsyequb( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, double[] s, double[] scond, double[] amax,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_csyequb( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer s, FloatPointer scond, FloatPointer amax,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_csyequb( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer s, FloatBuffer scond, FloatBuffer amax,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_csyequb( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, float[] s, float[] scond, float[] amax,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zheequb( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer s, DoublePointer scond, DoublePointer amax,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zheequb( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer s, DoubleBuffer scond, DoubleBuffer amax,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zheequb( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, double[] s, double[] scond, double[] amax,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_cheequb( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer s, FloatPointer scond, FloatPointer amax,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_cheequb( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer s, FloatBuffer scond, FloatBuffer amax,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_cheequb( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, float[] s, float[] scond, float[] amax,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_sgesv( IntPointer n, IntPointer nrhs, FloatPointer a, IntPointer lda,
IntPointer ipiv, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_sgesv( IntBuffer n, IntBuffer nrhs, FloatBuffer a, IntBuffer lda,
IntBuffer ipiv, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_sgesv( int[] n, int[] nrhs, float[] a, int[] lda,
int[] ipiv, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dgesv( IntPointer n, IntPointer nrhs, DoublePointer a, IntPointer lda,
IntPointer ipiv, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dgesv( IntBuffer n, IntBuffer nrhs, DoubleBuffer a, IntBuffer lda,
IntBuffer ipiv, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dgesv( int[] n, int[] nrhs, double[] a, int[] lda,
int[] ipiv, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_cgesv( IntPointer n, IntPointer nrhs, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_cgesv( IntBuffer n, IntBuffer nrhs, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cgesv( int[] n, int[] nrhs, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zgesv( IntPointer n, IntPointer nrhs, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zgesv( IntBuffer n, IntBuffer nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zgesv( int[] n, int[] nrhs, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_dsgesv( IntPointer n, IntPointer nrhs, DoublePointer a, IntPointer lda,
IntPointer ipiv, DoublePointer b, IntPointer ldb, DoublePointer x,
IntPointer ldx, DoublePointer work, FloatPointer swork,
IntPointer iter, IntPointer info );
public static native void LAPACK_dsgesv( IntBuffer n, IntBuffer nrhs, DoubleBuffer a, IntBuffer lda,
IntBuffer ipiv, DoubleBuffer b, IntBuffer ldb, DoubleBuffer x,
IntBuffer ldx, DoubleBuffer work, FloatBuffer swork,
IntBuffer iter, IntBuffer info );
public static native void LAPACK_dsgesv( int[] n, int[] nrhs, double[] a, int[] lda,
int[] ipiv, double[] b, int[] ldb, double[] x,
int[] ldx, double[] work, float[] swork,
int[] iter, int[] info );
public static native void LAPACK_zcgesv( IntPointer n, IntPointer nrhs, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
@Cast("lapack_complex_double*") DoublePointer work, @Cast("lapack_complex_float*") FloatPointer swork,
DoublePointer rwork, IntPointer iter, IntPointer info );
public static native void LAPACK_zcgesv( IntBuffer n, IntBuffer nrhs, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
@Cast("lapack_complex_double*") DoubleBuffer work, @Cast("lapack_complex_float*") FloatBuffer swork,
DoubleBuffer rwork, IntBuffer iter, IntBuffer info );
public static native void LAPACK_zcgesv( int[] n, int[] nrhs, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
@Cast("lapack_complex_double*") double[] work, @Cast("lapack_complex_float*") float[] swork,
double[] rwork, int[] iter, int[] info );
public static native void LAPACK_sgesvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
FloatPointer a, IntPointer lda, FloatPointer af, IntPointer ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, FloatPointer r, FloatPointer c, FloatPointer b,
IntPointer ldb, FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sgesvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
FloatBuffer a, IntBuffer lda, FloatBuffer af, IntBuffer ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, FloatBuffer r, FloatBuffer c, FloatBuffer b,
IntBuffer ldb, FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sgesvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] nrhs,
float[] a, int[] lda, float[] af, int[] ldaf,
int[] ipiv, @Cast("char*") byte[] equed, float[] r, float[] c, float[] b,
int[] ldb, float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dgesvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
DoublePointer a, IntPointer lda, DoublePointer af, IntPointer ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, DoublePointer r, DoublePointer c,
DoublePointer b, IntPointer ldb, DoublePointer x, IntPointer ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dgesvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
DoubleBuffer a, IntBuffer lda, DoubleBuffer af, IntBuffer ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, DoubleBuffer r, DoubleBuffer c,
DoubleBuffer b, IntBuffer ldb, DoubleBuffer x, IntBuffer ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgesvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] nrhs,
double[] a, int[] lda, double[] af, int[] ldaf,
int[] ipiv, @Cast("char*") byte[] equed, double[] r, double[] c,
double[] b, int[] ldb, double[] x, int[] ldx,
double[] rcond, double[] ferr, double[] berr, double[] work,
int[] iwork, int[] info );
public static native void LAPACK_cgesvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer af, IntPointer ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, FloatPointer r, FloatPointer c,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_cgesvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer af, IntBuffer ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, FloatBuffer r, FloatBuffer c,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cgesvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] af, int[] ldaf,
int[] ipiv, @Cast("char*") byte[] equed, float[] r, float[] c,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zgesvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer af, IntPointer ldaf,
IntPointer ipiv, @Cast("char*") BytePointer equed, DoublePointer r, DoublePointer c,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgesvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer af, IntBuffer ldaf,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, DoubleBuffer r, DoubleBuffer c,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgesvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] af, int[] ldaf,
int[] ipiv, @Cast("char*") byte[] equed, double[] r, double[] c,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] rcond,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_sgbsv( IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, FloatPointer ab, IntPointer ldab,
IntPointer ipiv, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_sgbsv( IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, FloatBuffer ab, IntBuffer ldab,
IntBuffer ipiv, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_sgbsv( int[] n, int[] kl, int[] ku,
int[] nrhs, float[] ab, int[] ldab,
int[] ipiv, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dgbsv( IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, DoublePointer ab, IntPointer ldab,
IntPointer ipiv, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dgbsv( IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, DoubleBuffer ab, IntBuffer ldab,
IntBuffer ipiv, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dgbsv( int[] n, int[] kl, int[] ku,
int[] nrhs, double[] ab, int[] ldab,
int[] ipiv, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_cgbsv( IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_cgbsv( IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_cgbsv( int[] n, int[] kl, int[] ku,
int[] nrhs, @Cast("lapack_complex_float*") float[] ab, int[] ldab,
int[] ipiv, @Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_zgbsv( IntPointer n, IntPointer kl, IntPointer ku,
IntPointer nrhs, @Cast("lapack_complex_double*") DoublePointer ab,
IntPointer ldab, IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zgbsv( IntBuffer n, IntBuffer kl, IntBuffer ku,
IntBuffer nrhs, @Cast("lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zgbsv( int[] n, int[] kl, int[] ku,
int[] nrhs, @Cast("lapack_complex_double*") double[] ab,
int[] ldab, int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_sgbsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl,
IntPointer ku, IntPointer nrhs, FloatPointer ab,
IntPointer ldab, FloatPointer afb, IntPointer ldafb,
IntPointer ipiv, @Cast("char*") BytePointer equed, FloatPointer r, FloatPointer c, FloatPointer b,
IntPointer ldb, FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sgbsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl,
IntBuffer ku, IntBuffer nrhs, FloatBuffer ab,
IntBuffer ldab, FloatBuffer afb, IntBuffer ldafb,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, FloatBuffer r, FloatBuffer c, FloatBuffer b,
IntBuffer ldb, FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sgbsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] kl,
int[] ku, int[] nrhs, float[] ab,
int[] ldab, float[] afb, int[] ldafb,
int[] ipiv, @Cast("char*") byte[] equed, float[] r, float[] c, float[] b,
int[] ldb, float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dgbsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl,
IntPointer ku, IntPointer nrhs, DoublePointer ab,
IntPointer ldab, DoublePointer afb, IntPointer ldafb,
IntPointer ipiv, @Cast("char*") BytePointer equed, DoublePointer r, DoublePointer c,
DoublePointer b, IntPointer ldb, DoublePointer x, IntPointer ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr, DoublePointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dgbsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl,
IntBuffer ku, IntBuffer nrhs, DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer afb, IntBuffer ldafb,
IntBuffer ipiv, @Cast("char*") ByteBuffer equed, DoubleBuffer r, DoubleBuffer c,
DoubleBuffer b, IntBuffer ldb, DoubleBuffer x, IntBuffer ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgbsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] kl,
int[] ku, int[] nrhs, double[] ab,
int[] ldab, double[] afb, int[] ldafb,
int[] ipiv, @Cast("char*") byte[] equed, double[] r, double[] c,
double[] b, int[] ldb, double[] x, int[] ldx,
double[] rcond, double[] ferr, double[] berr, double[] work,
int[] iwork, int[] info );
public static native void LAPACK_cgbsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl,
IntPointer ku, IntPointer nrhs, @Cast("lapack_complex_float*") FloatPointer ab,
IntPointer ldab, @Cast("lapack_complex_float*") FloatPointer afb,
IntPointer ldafb, IntPointer ipiv, @Cast("char*") BytePointer equed, FloatPointer r,
FloatPointer c, @Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_cgbsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl,
IntBuffer ku, IntBuffer nrhs, @Cast("lapack_complex_float*") FloatBuffer ab,
IntBuffer ldab, @Cast("lapack_complex_float*") FloatBuffer afb,
IntBuffer ldafb, IntBuffer ipiv, @Cast("char*") ByteBuffer equed, FloatBuffer r,
FloatBuffer c, @Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cgbsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] kl,
int[] ku, int[] nrhs, @Cast("lapack_complex_float*") float[] ab,
int[] ldab, @Cast("lapack_complex_float*") float[] afb,
int[] ldafb, int[] ipiv, @Cast("char*") byte[] equed, float[] r,
float[] c, @Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zgbsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer kl,
IntPointer ku, IntPointer nrhs, @Cast("lapack_complex_double*") DoublePointer ab,
IntPointer ldab, @Cast("lapack_complex_double*") DoublePointer afb,
IntPointer ldafb, IntPointer ipiv, @Cast("char*") BytePointer equed, DoublePointer r,
DoublePointer c, @Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgbsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer kl,
IntBuffer ku, IntBuffer nrhs, @Cast("lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, @Cast("lapack_complex_double*") DoubleBuffer afb,
IntBuffer ldafb, IntBuffer ipiv, @Cast("char*") ByteBuffer equed, DoubleBuffer r,
DoubleBuffer c, @Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgbsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] kl,
int[] ku, int[] nrhs, @Cast("lapack_complex_double*") double[] ab,
int[] ldab, @Cast("lapack_complex_double*") double[] afb,
int[] ldafb, int[] ipiv, @Cast("char*") byte[] equed, double[] r,
double[] c, @Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] rcond,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_sgtsv( IntPointer n, IntPointer nrhs, FloatPointer dl, FloatPointer d,
FloatPointer du, FloatPointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_sgtsv( IntBuffer n, IntBuffer nrhs, FloatBuffer dl, FloatBuffer d,
FloatBuffer du, FloatBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_sgtsv( int[] n, int[] nrhs, float[] dl, float[] d,
float[] du, float[] b, int[] ldb, int[] info );
public static native void LAPACK_dgtsv( IntPointer n, IntPointer nrhs, DoublePointer dl, DoublePointer d,
DoublePointer du, DoublePointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_dgtsv( IntBuffer n, IntBuffer nrhs, DoubleBuffer dl, DoubleBuffer d,
DoubleBuffer du, DoubleBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dgtsv( int[] n, int[] nrhs, double[] dl, double[] d,
double[] du, double[] b, int[] ldb, int[] info );
public static native void LAPACK_cgtsv( IntPointer n, IntPointer nrhs, @Cast("lapack_complex_float*") FloatPointer dl,
@Cast("lapack_complex_float*") FloatPointer d, @Cast("lapack_complex_float*") FloatPointer du,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_cgtsv( IntBuffer n, IntBuffer nrhs, @Cast("lapack_complex_float*") FloatBuffer dl,
@Cast("lapack_complex_float*") FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer du,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cgtsv( int[] n, int[] nrhs, @Cast("lapack_complex_float*") float[] dl,
@Cast("lapack_complex_float*") float[] d, @Cast("lapack_complex_float*") float[] du,
@Cast("lapack_complex_float*") float[] b, int[] ldb, int[] info );
public static native void LAPACK_zgtsv( IntPointer n, IntPointer nrhs, @Cast("lapack_complex_double*") DoublePointer dl,
@Cast("lapack_complex_double*") DoublePointer d, @Cast("lapack_complex_double*") DoublePointer du,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zgtsv( IntBuffer n, IntBuffer nrhs, @Cast("lapack_complex_double*") DoubleBuffer dl,
@Cast("lapack_complex_double*") DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer du,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zgtsv( int[] n, int[] nrhs, @Cast("lapack_complex_double*") double[] dl,
@Cast("lapack_complex_double*") double[] d, @Cast("lapack_complex_double*") double[] du,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_sgtsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const FloatPointer dl, @Const FloatPointer d, @Const FloatPointer du,
FloatPointer dlf, FloatPointer df, FloatPointer duf, FloatPointer du2,
IntPointer ipiv, @Const FloatPointer b, IntPointer ldb, FloatPointer x,
IntPointer ldx, FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_sgtsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer dl, @Const FloatBuffer d, @Const FloatBuffer du,
FloatBuffer dlf, FloatBuffer df, FloatBuffer duf, FloatBuffer du2,
IntBuffer ipiv, @Const FloatBuffer b, IntBuffer ldb, FloatBuffer x,
IntBuffer ldx, FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sgtsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const float[] dl, @Const float[] d, @Const float[] du,
float[] dlf, float[] df, float[] duf, float[] du2,
int[] ipiv, @Const float[] b, int[] ldb, float[] x,
int[] ldx, float[] rcond, float[] ferr, float[] berr,
float[] work, int[] iwork, int[] info );
public static native void LAPACK_dgtsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Const DoublePointer dl, @Const DoublePointer d, @Const DoublePointer du,
DoublePointer dlf, DoublePointer df, DoublePointer duf, DoublePointer du2,
IntPointer ipiv, @Const DoublePointer b, IntPointer ldb,
DoublePointer x, IntPointer ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dgtsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer dl, @Const DoubleBuffer d, @Const DoubleBuffer du,
DoubleBuffer dlf, DoubleBuffer df, DoubleBuffer duf, DoubleBuffer du2,
IntBuffer ipiv, @Const DoubleBuffer b, IntBuffer ldb,
DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dgtsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Const double[] dl, @Const double[] d, @Const double[] du,
double[] dlf, double[] df, double[] duf, double[] du2,
int[] ipiv, @Const double[] b, int[] ldb,
double[] x, int[] ldx, double[] rcond, double[] ferr,
double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_cgtsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du, @Cast("lapack_complex_float*") FloatPointer dlf,
@Cast("lapack_complex_float*") FloatPointer df, @Cast("lapack_complex_float*") FloatPointer duf,
@Cast("lapack_complex_float*") FloatPointer du2, IntPointer ipiv,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_cgtsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du, @Cast("lapack_complex_float*") FloatBuffer dlf,
@Cast("lapack_complex_float*") FloatBuffer df, @Cast("lapack_complex_float*") FloatBuffer duf,
@Cast("lapack_complex_float*") FloatBuffer du2, IntBuffer ipiv,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cgtsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du, @Cast("lapack_complex_float*") float[] dlf,
@Cast("lapack_complex_float*") float[] df, @Cast("lapack_complex_float*") float[] duf,
@Cast("lapack_complex_float*") float[] du2, int[] ipiv,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zgtsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer trans, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du, @Cast("lapack_complex_double*") DoublePointer dlf,
@Cast("lapack_complex_double*") DoublePointer df, @Cast("lapack_complex_double*") DoublePointer duf,
@Cast("lapack_complex_double*") DoublePointer du2, IntPointer ipiv,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgtsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer trans, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du, @Cast("lapack_complex_double*") DoubleBuffer dlf,
@Cast("lapack_complex_double*") DoubleBuffer df, @Cast("lapack_complex_double*") DoubleBuffer duf,
@Cast("lapack_complex_double*") DoubleBuffer du2, IntBuffer ipiv,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgtsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] trans, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du, @Cast("lapack_complex_double*") double[] dlf,
@Cast("lapack_complex_double*") double[] df, @Cast("lapack_complex_double*") double[] duf,
@Cast("lapack_complex_double*") double[] du2, int[] ipiv,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] rcond,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_sposv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_sposv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_sposv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, float[] a,
int[] lda, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dposv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dposv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dposv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, double[] a,
int[] lda, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_cposv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_cposv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cposv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, int[] info );
public static native void LAPACK_zposv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zposv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zposv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_dsposv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb, DoublePointer x,
IntPointer ldx, DoublePointer work, FloatPointer swork,
IntPointer iter, IntPointer info );
public static native void LAPACK_dsposv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb, DoubleBuffer x,
IntBuffer ldx, DoubleBuffer work, FloatBuffer swork,
IntBuffer iter, IntBuffer info );
public static native void LAPACK_dsposv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, double[] a,
int[] lda, double[] b, int[] ldb, double[] x,
int[] ldx, double[] work, float[] swork,
int[] iter, int[] info );
public static native void LAPACK_zcposv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
@Cast("lapack_complex_double*") DoublePointer work, @Cast("lapack_complex_float*") FloatPointer swork,
DoublePointer rwork, IntPointer iter, IntPointer info );
public static native void LAPACK_zcposv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
@Cast("lapack_complex_double*") DoubleBuffer work, @Cast("lapack_complex_float*") FloatBuffer swork,
DoubleBuffer rwork, IntBuffer iter, IntBuffer info );
public static native void LAPACK_zcposv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx,
@Cast("lapack_complex_double*") double[] work, @Cast("lapack_complex_float*") float[] swork,
double[] rwork, int[] iter, int[] info );
public static native void LAPACK_sposvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
FloatPointer a, IntPointer lda, FloatPointer af, IntPointer ldaf,
@Cast("char*") BytePointer equed, FloatPointer s, FloatPointer b, IntPointer ldb, FloatPointer x,
IntPointer ldx, FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
FloatPointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_sposvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
FloatBuffer a, IntBuffer lda, FloatBuffer af, IntBuffer ldaf,
@Cast("char*") ByteBuffer equed, FloatBuffer s, FloatBuffer b, IntBuffer ldb, FloatBuffer x,
IntBuffer ldx, FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
FloatBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sposvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
float[] a, int[] lda, float[] af, int[] ldaf,
@Cast("char*") byte[] equed, float[] s, float[] b, int[] ldb, float[] x,
int[] ldx, float[] rcond, float[] ferr, float[] berr,
float[] work, int[] iwork, int[] info );
public static native void LAPACK_dposvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
DoublePointer a, IntPointer lda, DoublePointer af, IntPointer ldaf,
@Cast("char*") BytePointer equed, DoublePointer s, DoublePointer b, IntPointer ldb,
DoublePointer x, IntPointer ldx, DoublePointer rcond, DoublePointer ferr,
DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dposvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
DoubleBuffer a, IntBuffer lda, DoubleBuffer af, IntBuffer ldaf,
@Cast("char*") ByteBuffer equed, DoubleBuffer s, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond, DoubleBuffer ferr,
DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dposvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
double[] a, int[] lda, double[] af, int[] ldaf,
@Cast("char*") byte[] equed, double[] s, double[] b, int[] ldb,
double[] x, int[] ldx, double[] rcond, double[] ferr,
double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_cposvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer af, IntPointer ldaf, @Cast("char*") BytePointer equed,
FloatPointer s, @Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_cposvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer af, IntBuffer ldaf, @Cast("char*") ByteBuffer equed,
FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cposvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] af, int[] ldaf, @Cast("char*") byte[] equed,
float[] s, @Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zposvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer af, IntPointer ldaf, @Cast("char*") BytePointer equed,
DoublePointer s, @Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zposvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer af, IntBuffer ldaf, @Cast("char*") ByteBuffer equed,
DoubleBuffer s, @Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zposvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] af, int[] ldaf, @Cast("char*") byte[] equed,
double[] s, @Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] rcond,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_sppsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, FloatPointer ap,
FloatPointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_sppsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, FloatBuffer ap,
FloatBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_sppsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, float[] ap,
float[] b, int[] ldb, int[] info );
public static native void LAPACK_dppsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, DoublePointer ap,
DoublePointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_dppsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, DoubleBuffer ap,
DoubleBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dppsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, double[] ap,
double[] b, int[] ldb, int[] info );
public static native void LAPACK_cppsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer ap, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_cppsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer ap, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cppsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] ap, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zppsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer ap, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zppsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ap, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zppsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] ap, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_sppsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
FloatPointer ap, FloatPointer afp, @Cast("char*") BytePointer equed, FloatPointer s, FloatPointer b,
IntPointer ldb, FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sppsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
FloatBuffer ap, FloatBuffer afp, @Cast("char*") ByteBuffer equed, FloatBuffer s, FloatBuffer b,
IntBuffer ldb, FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sppsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
float[] ap, float[] afp, @Cast("char*") byte[] equed, float[] s, float[] b,
int[] ldb, float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dppsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
DoublePointer ap, DoublePointer afp, @Cast("char*") BytePointer equed, DoublePointer s, DoublePointer b,
IntPointer ldb, DoublePointer x, IntPointer ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dppsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
DoubleBuffer ap, DoubleBuffer afp, @Cast("char*") ByteBuffer equed, DoubleBuffer s, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dppsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
double[] ap, double[] afp, @Cast("char*") byte[] equed, double[] s, double[] b,
int[] ldb, double[] x, int[] ldx, double[] rcond,
double[] ferr, double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_cppsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer ap, @Cast("lapack_complex_float*") FloatPointer afp,
@Cast("char*") BytePointer equed, FloatPointer s, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cppsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer ap, @Cast("lapack_complex_float*") FloatBuffer afp,
@Cast("char*") ByteBuffer equed, FloatBuffer s, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cppsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] ap, @Cast("lapack_complex_float*") float[] afp,
@Cast("char*") byte[] equed, float[] s, @Cast("lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] x, int[] ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zppsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer ap, @Cast("lapack_complex_double*") DoublePointer afp,
@Cast("char*") BytePointer equed, DoublePointer s, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zppsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ap, @Cast("lapack_complex_double*") DoubleBuffer afp,
@Cast("char*") ByteBuffer equed, DoubleBuffer s, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zppsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] ap, @Cast("lapack_complex_double*") double[] afp,
@Cast("char*") byte[] equed, double[] s, @Cast("lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_spbsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
FloatPointer ab, IntPointer ldab, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_spbsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
FloatBuffer ab, IntBuffer ldab, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_spbsv( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
float[] ab, int[] ldab, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dpbsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
DoublePointer ab, IntPointer ldab, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dpbsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
DoubleBuffer ab, IntBuffer ldab, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dpbsv( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
double[] ab, int[] ldab, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_cpbsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_cpbsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cpbsv( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Cast("lapack_complex_float*") float[] ab, int[] ldab,
@Cast("lapack_complex_float*") float[] b, int[] ldb, int[] info );
public static native void LAPACK_zpbsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zpbsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zpbsv( @Cast("char*") byte[] uplo, int[] n, int[] kd, int[] nrhs,
@Cast("lapack_complex_double*") double[] ab, int[] ldab,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_spbsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
IntPointer nrhs, FloatPointer ab, IntPointer ldab, FloatPointer afb,
IntPointer ldafb, @Cast("char*") BytePointer equed, FloatPointer s, FloatPointer b,
IntPointer ldb, FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_spbsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
IntBuffer nrhs, FloatBuffer ab, IntBuffer ldab, FloatBuffer afb,
IntBuffer ldafb, @Cast("char*") ByteBuffer equed, FloatBuffer s, FloatBuffer b,
IntBuffer ldb, FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_spbsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] kd,
int[] nrhs, float[] ab, int[] ldab, float[] afb,
int[] ldafb, @Cast("char*") byte[] equed, float[] s, float[] b,
int[] ldb, float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dpbsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
IntPointer nrhs, DoublePointer ab, IntPointer ldab, DoublePointer afb,
IntPointer ldafb, @Cast("char*") BytePointer equed, DoublePointer s, DoublePointer b,
IntPointer ldb, DoublePointer x, IntPointer ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dpbsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
IntBuffer nrhs, DoubleBuffer ab, IntBuffer ldab, DoubleBuffer afb,
IntBuffer ldafb, @Cast("char*") ByteBuffer equed, DoubleBuffer s, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dpbsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] kd,
int[] nrhs, double[] ab, int[] ldab, double[] afb,
int[] ldafb, @Cast("char*") byte[] equed, double[] s, double[] b,
int[] ldb, double[] x, int[] ldx, double[] rcond,
double[] ferr, double[] berr, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_cpbsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
IntPointer nrhs, @Cast("lapack_complex_float*") FloatPointer ab,
IntPointer ldab, @Cast("lapack_complex_float*") FloatPointer afb,
IntPointer ldafb, @Cast("char*") BytePointer equed, FloatPointer s,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, @Cast("lapack_complex_float*") FloatPointer work,
FloatPointer rwork, IntPointer info );
public static native void LAPACK_cpbsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
IntBuffer nrhs, @Cast("lapack_complex_float*") FloatBuffer ab,
IntBuffer ldab, @Cast("lapack_complex_float*") FloatBuffer afb,
IntBuffer ldafb, @Cast("char*") ByteBuffer equed, FloatBuffer s,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, @Cast("lapack_complex_float*") FloatBuffer work,
FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cpbsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] kd,
int[] nrhs, @Cast("lapack_complex_float*") float[] ab,
int[] ldab, @Cast("lapack_complex_float*") float[] afb,
int[] ldafb, @Cast("char*") byte[] equed, float[] s,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, @Cast("lapack_complex_float*") float[] work,
float[] rwork, int[] info );
public static native void LAPACK_zpbsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
IntPointer nrhs, @Cast("lapack_complex_double*") DoublePointer ab,
IntPointer ldab, @Cast("lapack_complex_double*") DoublePointer afb,
IntPointer ldafb, @Cast("char*") BytePointer equed, DoublePointer s,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr, @Cast("lapack_complex_double*") DoublePointer work,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zpbsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
IntBuffer nrhs, @Cast("lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, @Cast("lapack_complex_double*") DoubleBuffer afb,
IntBuffer ldafb, @Cast("char*") ByteBuffer equed, DoubleBuffer s,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr, @Cast("lapack_complex_double*") DoubleBuffer work,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zpbsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] kd,
int[] nrhs, @Cast("lapack_complex_double*") double[] ab,
int[] ldab, @Cast("lapack_complex_double*") double[] afb,
int[] ldafb, @Cast("char*") byte[] equed, double[] s,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] x, int[] ldx, double[] rcond,
double[] ferr, double[] berr, @Cast("lapack_complex_double*") double[] work,
double[] rwork, int[] info );
public static native void LAPACK_sptsv( IntPointer n, IntPointer nrhs, FloatPointer d, FloatPointer e,
FloatPointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_sptsv( IntBuffer n, IntBuffer nrhs, FloatBuffer d, FloatBuffer e,
FloatBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_sptsv( int[] n, int[] nrhs, float[] d, float[] e,
float[] b, int[] ldb, int[] info );
public static native void LAPACK_dptsv( IntPointer n, IntPointer nrhs, DoublePointer d, DoublePointer e,
DoublePointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_dptsv( IntBuffer n, IntBuffer nrhs, DoubleBuffer d, DoubleBuffer e,
DoubleBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dptsv( int[] n, int[] nrhs, double[] d, double[] e,
double[] b, int[] ldb, int[] info );
public static native void LAPACK_cptsv( IntPointer n, IntPointer nrhs, FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer e, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_cptsv( IntBuffer n, IntBuffer nrhs, FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cptsv( int[] n, int[] nrhs, float[] d,
@Cast("lapack_complex_float*") float[] e, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zptsv( IntPointer n, IntPointer nrhs, DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer e, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zptsv( IntBuffer n, IntBuffer nrhs, DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zptsv( int[] n, int[] nrhs, double[] d,
@Cast("lapack_complex_double*") double[] e, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_sptsvx( @Cast("char*") BytePointer fact, IntPointer n, IntPointer nrhs, @Const FloatPointer d,
@Const FloatPointer e, FloatPointer df, FloatPointer ef, @Const FloatPointer b,
IntPointer ldb, FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer info );
public static native void LAPACK_sptsvx( @Cast("char*") ByteBuffer fact, IntBuffer n, IntBuffer nrhs, @Const FloatBuffer d,
@Const FloatBuffer e, FloatBuffer df, FloatBuffer ef, @Const FloatBuffer b,
IntBuffer ldb, FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sptsvx( @Cast("char*") byte[] fact, int[] n, int[] nrhs, @Const float[] d,
@Const float[] e, float[] df, float[] ef, @Const float[] b,
int[] ldb, float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, float[] work, int[] info );
public static native void LAPACK_dptsvx( @Cast("char*") BytePointer fact, IntPointer n, IntPointer nrhs,
@Const DoublePointer d, @Const DoublePointer e, DoublePointer df, DoublePointer ef,
@Const DoublePointer b, IntPointer ldb, DoublePointer x,
IntPointer ldx, DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer info );
public static native void LAPACK_dptsvx( @Cast("char*") ByteBuffer fact, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer d, @Const DoubleBuffer e, DoubleBuffer df, DoubleBuffer ef,
@Const DoubleBuffer b, IntBuffer ldb, DoubleBuffer x,
IntBuffer ldx, DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dptsvx( @Cast("char*") byte[] fact, int[] n, int[] nrhs,
@Const double[] d, @Const double[] e, double[] df, double[] ef,
@Const double[] b, int[] ldb, double[] x,
int[] ldx, double[] rcond, double[] ferr, double[] berr,
double[] work, int[] info );
public static native void LAPACK_cptsvx( @Cast("char*") BytePointer fact, IntPointer n, IntPointer nrhs, @Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e, FloatPointer df,
@Cast("lapack_complex_float*") FloatPointer ef, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cptsvx( @Cast("char*") ByteBuffer fact, IntBuffer n, IntBuffer nrhs, @Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e, FloatBuffer df,
@Cast("lapack_complex_float*") FloatBuffer ef, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cptsvx( @Cast("char*") byte[] fact, int[] n, int[] nrhs, @Const float[] d,
@Cast("const lapack_complex_float*") float[] e, float[] df,
@Cast("lapack_complex_float*") float[] ef, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] x, int[] ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zptsvx( @Cast("char*") BytePointer fact, IntPointer n, IntPointer nrhs,
@Const DoublePointer d, @Cast("const lapack_complex_double*") DoublePointer e, DoublePointer df,
@Cast("lapack_complex_double*") DoublePointer ef, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zptsvx( @Cast("char*") ByteBuffer fact, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer d, @Cast("const lapack_complex_double*") DoubleBuffer e, DoubleBuffer df,
@Cast("lapack_complex_double*") DoubleBuffer ef, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zptsvx( @Cast("char*") byte[] fact, int[] n, int[] nrhs,
@Const double[] d, @Cast("const lapack_complex_double*") double[] e, double[] df,
@Cast("lapack_complex_double*") double[] ef, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_ssysv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, FloatPointer a,
IntPointer lda, IntPointer ipiv, FloatPointer b, IntPointer ldb,
FloatPointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_ssysv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, FloatBuffer a,
IntBuffer lda, IntBuffer ipiv, FloatBuffer b, IntBuffer ldb,
FloatBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_ssysv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, float[] a,
int[] lda, int[] ipiv, float[] b, int[] ldb,
float[] work, int[] lwork, int[] info );
public static native void LAPACK_dsysv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, DoublePointer a,
IntPointer lda, IntPointer ipiv, DoublePointer b,
IntPointer ldb, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dsysv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dsysv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, double[] a,
int[] lda, int[] ipiv, double[] b,
int[] ldb, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_csysv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_csysv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_csysv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zsysv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zsysv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zsysv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_ssysvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const FloatPointer a, IntPointer lda, FloatPointer af,
IntPointer ldaf, IntPointer ipiv, @Const FloatPointer b,
IntPointer ldb, FloatPointer x, IntPointer ldx, FloatPointer rcond,
FloatPointer ferr, FloatPointer berr, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_ssysvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer a, IntBuffer lda, FloatBuffer af,
IntBuffer ldaf, IntBuffer ipiv, @Const FloatBuffer b,
IntBuffer ldb, FloatBuffer x, IntBuffer ldx, FloatBuffer rcond,
FloatBuffer ferr, FloatBuffer berr, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_ssysvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const float[] a, int[] lda, float[] af,
int[] ldaf, int[] ipiv, @Const float[] b,
int[] ldb, float[] x, int[] ldx, float[] rcond,
float[] ferr, float[] berr, float[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_dsysvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer a, IntPointer lda, DoublePointer af,
IntPointer ldaf, IntPointer ipiv, @Const DoublePointer b,
IntPointer ldb, DoublePointer x, IntPointer ldx, DoublePointer rcond,
DoublePointer ferr, DoublePointer berr, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dsysvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer a, IntBuffer lda, DoubleBuffer af,
IntBuffer ldaf, IntBuffer ipiv, @Const DoubleBuffer b,
IntBuffer ldb, DoubleBuffer x, IntBuffer ldx, DoubleBuffer rcond,
DoubleBuffer ferr, DoubleBuffer berr, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dsysvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] a, int[] lda, double[] af,
int[] ldaf, int[] ipiv, @Const double[] b,
int[] ldb, double[] x, int[] ldx, double[] rcond,
double[] ferr, double[] berr, double[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_csysvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer af, IntPointer ldaf,
IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_csysvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer af, IntBuffer ldaf,
IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_csysvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] af, int[] ldaf,
int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] x, int[] ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zsysvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer af, IntPointer ldaf,
IntPointer ipiv, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zsysvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer af, IntBuffer ldaf,
IntBuffer ipiv, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zsysvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] af, int[] ldaf,
int[] ipiv, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_chesv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_chesv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_chesv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zhesv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zhesv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zhesv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_chesvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer af, IntPointer ldaf,
IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_chesvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer af, IntBuffer ldaf,
IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_chesvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] af, int[] ldaf,
int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] x, int[] ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zhesvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer af, IntPointer ldaf,
IntPointer ipiv, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zhesvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer af, IntBuffer ldaf,
IntBuffer ipiv, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zhesvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] af, int[] ldaf,
int[] ipiv, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_sspsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, FloatPointer ap,
IntPointer ipiv, FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_sspsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, FloatBuffer ap,
IntBuffer ipiv, FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_sspsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, float[] ap,
int[] ipiv, float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dspsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, DoublePointer ap,
IntPointer ipiv, DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dspsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, DoubleBuffer ap,
IntBuffer ipiv, DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dspsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, double[] ap,
int[] ipiv, double[] b, int[] ldb,
int[] info );
public static native void LAPACK_cspsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer ap, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_cspsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer ap, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_cspsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] ap, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb, int[] info );
public static native void LAPACK_zspsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer ap, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zspsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ap, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zspsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] ap, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_sspsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const FloatPointer ap, FloatPointer afp, IntPointer ipiv,
@Const FloatPointer b, IntPointer ldb, FloatPointer x, IntPointer ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr, FloatPointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_sspsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const FloatBuffer ap, FloatBuffer afp, IntBuffer ipiv,
@Const FloatBuffer b, IntBuffer ldb, FloatBuffer x, IntBuffer ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr, FloatBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sspsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const float[] ap, float[] afp, int[] ipiv,
@Const float[] b, int[] ldb, float[] x, int[] ldx,
float[] rcond, float[] ferr, float[] berr, float[] work,
int[] iwork, int[] info );
public static native void LAPACK_dspsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer ap, DoublePointer afp, IntPointer ipiv,
@Const DoublePointer b, IntPointer ldb, DoublePointer x,
IntPointer ldx, DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dspsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer ap, DoubleBuffer afp, IntBuffer ipiv,
@Const DoubleBuffer b, IntBuffer ldb, DoubleBuffer x,
IntBuffer ldx, DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dspsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] ap, double[] afp, int[] ipiv,
@Const double[] b, int[] ldb, double[] x,
int[] ldx, double[] rcond, double[] ferr, double[] berr,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_cspsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap, @Cast("lapack_complex_float*") FloatPointer afp,
IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cspsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap, @Cast("lapack_complex_float*") FloatBuffer afp,
IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cspsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ap, @Cast("lapack_complex_float*") float[] afp,
int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] x, int[] ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zspsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap, @Cast("lapack_complex_double*") DoublePointer afp,
IntPointer ipiv, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zspsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap, @Cast("lapack_complex_double*") DoubleBuffer afp,
IntBuffer ipiv, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zspsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ap, @Cast("lapack_complex_double*") double[] afp,
int[] ipiv, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_chpsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer ap, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_chpsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer ap, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_chpsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] ap, int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb, int[] info );
public static native void LAPACK_zhpsv( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer ap, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zhpsv( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer ap, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zhpsv( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] ap, int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_chpsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer ap, @Cast("lapack_complex_float*") FloatPointer afp,
IntPointer ipiv, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
FloatPointer rcond, FloatPointer ferr, FloatPointer berr,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_chpsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer ap, @Cast("lapack_complex_float*") FloatBuffer afp,
IntBuffer ipiv, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
FloatBuffer rcond, FloatBuffer ferr, FloatBuffer berr,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_chpsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] ap, @Cast("lapack_complex_float*") float[] afp,
int[] ipiv, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] x, int[] ldx,
float[] rcond, float[] ferr, float[] berr,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zhpsvx( @Cast("char*") BytePointer fact, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer ap, @Cast("lapack_complex_double*") DoublePointer afp,
IntPointer ipiv, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
DoublePointer rcond, DoublePointer ferr, DoublePointer berr,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zhpsvx( @Cast("char*") ByteBuffer fact, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer ap, @Cast("lapack_complex_double*") DoubleBuffer afp,
IntBuffer ipiv, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
DoubleBuffer rcond, DoubleBuffer ferr, DoubleBuffer berr,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zhpsvx( @Cast("char*") byte[] fact, @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] ap, @Cast("lapack_complex_double*") double[] afp,
int[] ipiv, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] x, int[] ldx,
double[] rcond, double[] ferr, double[] berr,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sgeqrf( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer tau, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sgeqrf( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer tau, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sgeqrf( int[] m, int[] n, float[] a, int[] lda,
float[] tau, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dgeqrf( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer tau, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dgeqrf( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer tau, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dgeqrf( int[] m, int[] n, double[] a, int[] lda,
double[] tau, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cgeqrf( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cgeqrf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cgeqrf( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zgeqrf( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zgeqrf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zgeqrf( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sgeqp3( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer jpvt, FloatPointer tau, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sgeqp3( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer jpvt, FloatBuffer tau, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sgeqp3( int[] m, int[] n, float[] a, int[] lda,
int[] jpvt, float[] tau, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dgeqp3( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer jpvt, DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dgeqp3( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer jpvt, DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dgeqp3( int[] m, int[] n, double[] a, int[] lda,
int[] jpvt, double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cgeqp3( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer jpvt,
@Cast("lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, FloatPointer rwork, IntPointer info );
public static native void LAPACK_cgeqp3( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer jpvt,
@Cast("lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cgeqp3( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] jpvt,
@Cast("lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] work,
int[] lwork, float[] rwork, int[] info );
public static native void LAPACK_zgeqp3( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer jpvt,
@Cast("lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgeqp3( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer jpvt,
@Cast("lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgeqp3( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] jpvt,
@Cast("lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] work,
int[] lwork, double[] rwork, int[] info );
public static native void LAPACK_sorgqr( IntPointer m, IntPointer n, IntPointer k, FloatPointer a,
IntPointer lda, @Const FloatPointer tau, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sorgqr( IntBuffer m, IntBuffer n, IntBuffer k, FloatBuffer a,
IntBuffer lda, @Const FloatBuffer tau, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sorgqr( int[] m, int[] n, int[] k, float[] a,
int[] lda, @Const float[] tau, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dorgqr( IntPointer m, IntPointer n, IntPointer k, DoublePointer a,
IntPointer lda, @Const DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dorgqr( IntBuffer m, IntBuffer n, IntBuffer k, DoubleBuffer a,
IntBuffer lda, @Const DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dorgqr( int[] m, int[] n, int[] k, double[] a,
int[] lda, @Const double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sormqr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Const FloatPointer a, IntPointer lda,
@Const FloatPointer tau, FloatPointer c, IntPointer ldc, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sormqr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Const FloatBuffer a, IntBuffer lda,
@Const FloatBuffer tau, FloatBuffer c, IntBuffer ldc, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sormqr( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Const float[] a, int[] lda,
@Const float[] tau, float[] c, int[] ldc, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dormqr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Const DoublePointer a, IntPointer lda,
@Const DoublePointer tau, DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dormqr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Const DoubleBuffer a, IntBuffer lda,
@Const DoubleBuffer tau, DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dormqr( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Const double[] a, int[] lda,
@Const double[] tau, double[] c, int[] ldc, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cungqr( IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cungqr( IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cungqr( int[] m, int[] n, int[] k,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zungqr( IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zungqr( IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zungqr( int[] m, int[] n, int[] k,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cunmqr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cunmqr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cunmqr( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zunmqr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zunmqr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zunmqr( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sgelqf( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer tau, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sgelqf( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer tau, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sgelqf( int[] m, int[] n, float[] a, int[] lda,
float[] tau, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dgelqf( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer tau, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dgelqf( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer tau, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dgelqf( int[] m, int[] n, double[] a, int[] lda,
double[] tau, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cgelqf( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cgelqf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cgelqf( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zgelqf( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zgelqf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zgelqf( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sorglq( IntPointer m, IntPointer n, IntPointer k, FloatPointer a,
IntPointer lda, @Const FloatPointer tau, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sorglq( IntBuffer m, IntBuffer n, IntBuffer k, FloatBuffer a,
IntBuffer lda, @Const FloatBuffer tau, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sorglq( int[] m, int[] n, int[] k, float[] a,
int[] lda, @Const float[] tau, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dorglq( IntPointer m, IntPointer n, IntPointer k, DoublePointer a,
IntPointer lda, @Const DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dorglq( IntBuffer m, IntBuffer n, IntBuffer k, DoubleBuffer a,
IntBuffer lda, @Const DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dorglq( int[] m, int[] n, int[] k, double[] a,
int[] lda, @Const double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sormlq( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Const FloatPointer a, IntPointer lda,
@Const FloatPointer tau, FloatPointer c, IntPointer ldc, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sormlq( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Const FloatBuffer a, IntBuffer lda,
@Const FloatBuffer tau, FloatBuffer c, IntBuffer ldc, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sormlq( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Const float[] a, int[] lda,
@Const float[] tau, float[] c, int[] ldc, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dormlq( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Const DoublePointer a, IntPointer lda,
@Const DoublePointer tau, DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dormlq( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Const DoubleBuffer a, IntBuffer lda,
@Const DoubleBuffer tau, DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dormlq( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Const double[] a, int[] lda,
@Const double[] tau, double[] c, int[] ldc, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cunglq( IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cunglq( IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cunglq( int[] m, int[] n, int[] k,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zunglq( IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zunglq( IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zunglq( int[] m, int[] n, int[] k,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cunmlq( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cunmlq( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cunmlq( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zunmlq( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zunmlq( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zunmlq( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sgeqlf( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer tau, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sgeqlf( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer tau, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sgeqlf( int[] m, int[] n, float[] a, int[] lda,
float[] tau, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dgeqlf( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer tau, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dgeqlf( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer tau, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dgeqlf( int[] m, int[] n, double[] a, int[] lda,
double[] tau, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cgeqlf( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cgeqlf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cgeqlf( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zgeqlf( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zgeqlf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zgeqlf( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sorgql( IntPointer m, IntPointer n, IntPointer k, FloatPointer a,
IntPointer lda, @Const FloatPointer tau, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sorgql( IntBuffer m, IntBuffer n, IntBuffer k, FloatBuffer a,
IntBuffer lda, @Const FloatBuffer tau, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sorgql( int[] m, int[] n, int[] k, float[] a,
int[] lda, @Const float[] tau, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dorgql( IntPointer m, IntPointer n, IntPointer k, DoublePointer a,
IntPointer lda, @Const DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dorgql( IntBuffer m, IntBuffer n, IntBuffer k, DoubleBuffer a,
IntBuffer lda, @Const DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dorgql( int[] m, int[] n, int[] k, double[] a,
int[] lda, @Const double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cungql( IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cungql( IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cungql( int[] m, int[] n, int[] k,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zungql( IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zungql( IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zungql( int[] m, int[] n, int[] k,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sormql( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Const FloatPointer a, IntPointer lda,
@Const FloatPointer tau, FloatPointer c, IntPointer ldc, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sormql( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Const FloatBuffer a, IntBuffer lda,
@Const FloatBuffer tau, FloatBuffer c, IntBuffer ldc, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sormql( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Const float[] a, int[] lda,
@Const float[] tau, float[] c, int[] ldc, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dormql( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Const DoublePointer a, IntPointer lda,
@Const DoublePointer tau, DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dormql( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Const DoubleBuffer a, IntBuffer lda,
@Const DoubleBuffer tau, DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dormql( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Const double[] a, int[] lda,
@Const double[] tau, double[] c, int[] ldc, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cunmql( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cunmql( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cunmql( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zunmql( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zunmql( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zunmql( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sgerqf( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer tau, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sgerqf( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer tau, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sgerqf( int[] m, int[] n, float[] a, int[] lda,
float[] tau, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dgerqf( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer tau, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dgerqf( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer tau, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dgerqf( int[] m, int[] n, double[] a, int[] lda,
double[] tau, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cgerqf( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cgerqf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cgerqf( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zgerqf( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zgerqf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zgerqf( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sorgrq( IntPointer m, IntPointer n, IntPointer k, FloatPointer a,
IntPointer lda, @Const FloatPointer tau, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sorgrq( IntBuffer m, IntBuffer n, IntBuffer k, FloatBuffer a,
IntBuffer lda, @Const FloatBuffer tau, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sorgrq( int[] m, int[] n, int[] k, float[] a,
int[] lda, @Const float[] tau, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dorgrq( IntPointer m, IntPointer n, IntPointer k, DoublePointer a,
IntPointer lda, @Const DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dorgrq( IntBuffer m, IntBuffer n, IntBuffer k, DoubleBuffer a,
IntBuffer lda, @Const DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dorgrq( int[] m, int[] n, int[] k, double[] a,
int[] lda, @Const double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cungrq( IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cungrq( IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cungrq( int[] m, int[] n, int[] k,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zungrq( IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zungrq( IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zungrq( int[] m, int[] n, int[] k,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sormrq( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Const FloatPointer a, IntPointer lda,
@Const FloatPointer tau, FloatPointer c, IntPointer ldc, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sormrq( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Const FloatBuffer a, IntBuffer lda,
@Const FloatBuffer tau, FloatBuffer c, IntBuffer ldc, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sormrq( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Const float[] a, int[] lda,
@Const float[] tau, float[] c, int[] ldc, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dormrq( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Const DoublePointer a, IntPointer lda,
@Const DoublePointer tau, DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dormrq( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Const DoubleBuffer a, IntBuffer lda,
@Const DoubleBuffer tau, DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dormrq( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Const double[] a, int[] lda,
@Const double[] tau, double[] c, int[] ldc, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cunmrq( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cunmrq( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cunmrq( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zunmrq( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zunmrq( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zunmrq( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_stzrzf( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer tau, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_stzrzf( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer tau, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_stzrzf( int[] m, int[] n, float[] a, int[] lda,
float[] tau, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dtzrzf( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer tau, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dtzrzf( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer tau, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dtzrzf( int[] m, int[] n, double[] a, int[] lda,
double[] tau, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_ctzrzf( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_ctzrzf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_ctzrzf( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_ztzrzf( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_ztzrzf( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_ztzrzf( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sormrz( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer l, @Const FloatPointer a,
IntPointer lda, @Const FloatPointer tau, FloatPointer c,
IntPointer ldc, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sormrz( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer l, @Const FloatBuffer a,
IntBuffer lda, @Const FloatBuffer tau, FloatBuffer c,
IntBuffer ldc, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sormrz( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] l, @Const float[] a,
int[] lda, @Const float[] tau, float[] c,
int[] ldc, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dormrz( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer l, @Const DoublePointer a,
IntPointer lda, @Const DoublePointer tau, DoublePointer c,
IntPointer ldc, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dormrz( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer l, @Const DoubleBuffer a,
IntBuffer lda, @Const DoubleBuffer tau, DoubleBuffer c,
IntBuffer ldc, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dormrz( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] l, @Const double[] a,
int[] lda, @Const double[] tau, double[] c,
int[] ldc, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cunmrz( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer l, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cunmrz( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer l, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cunmrz( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] l, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zunmrz( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer l,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer c,
IntPointer ldc, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zunmrz( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer l,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer c,
IntBuffer ldc, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zunmrz( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] l,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] c,
int[] ldc, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sggqrf( IntPointer n, IntPointer m, IntPointer p, FloatPointer a,
IntPointer lda, FloatPointer taua, FloatPointer b, IntPointer ldb,
FloatPointer taub, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sggqrf( IntBuffer n, IntBuffer m, IntBuffer p, FloatBuffer a,
IntBuffer lda, FloatBuffer taua, FloatBuffer b, IntBuffer ldb,
FloatBuffer taub, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sggqrf( int[] n, int[] m, int[] p, float[] a,
int[] lda, float[] taua, float[] b, int[] ldb,
float[] taub, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dggqrf( IntPointer n, IntPointer m, IntPointer p, DoublePointer a,
IntPointer lda, DoublePointer taua, DoublePointer b, IntPointer ldb,
DoublePointer taub, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dggqrf( IntBuffer n, IntBuffer m, IntBuffer p, DoubleBuffer a,
IntBuffer lda, DoubleBuffer taua, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer taub, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dggqrf( int[] n, int[] m, int[] p, double[] a,
int[] lda, double[] taua, double[] b, int[] ldb,
double[] taub, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cggqrf( IntPointer n, IntPointer m, IntPointer p,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer taua, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer taub,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cggqrf( IntBuffer n, IntBuffer m, IntBuffer p,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer taua, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer taub,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cggqrf( int[] n, int[] m, int[] p,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] taua, @Cast("lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] taub,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zggqrf( IntPointer n, IntPointer m, IntPointer p,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer taua, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer taub,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zggqrf( IntBuffer n, IntBuffer m, IntBuffer p,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer taua, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer taub,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zggqrf( int[] n, int[] m, int[] p,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] taua, @Cast("lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] taub,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sggrqf( IntPointer m, IntPointer p, IntPointer n, FloatPointer a,
IntPointer lda, FloatPointer taua, FloatPointer b, IntPointer ldb,
FloatPointer taub, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sggrqf( IntBuffer m, IntBuffer p, IntBuffer n, FloatBuffer a,
IntBuffer lda, FloatBuffer taua, FloatBuffer b, IntBuffer ldb,
FloatBuffer taub, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sggrqf( int[] m, int[] p, int[] n, float[] a,
int[] lda, float[] taua, float[] b, int[] ldb,
float[] taub, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dggrqf( IntPointer m, IntPointer p, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer taua, DoublePointer b, IntPointer ldb,
DoublePointer taub, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dggrqf( IntBuffer m, IntBuffer p, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer taua, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer taub, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dggrqf( int[] m, int[] p, int[] n, double[] a,
int[] lda, double[] taua, double[] b, int[] ldb,
double[] taub, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cggrqf( IntPointer m, IntPointer p, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer taua, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer taub,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cggrqf( IntBuffer m, IntBuffer p, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer taua, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer taub,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cggrqf( int[] m, int[] p, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] taua, @Cast("lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] taub,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zggrqf( IntPointer m, IntPointer p, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer taua, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer taub,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zggrqf( IntBuffer m, IntBuffer p, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer taua, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer taub,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zggrqf( int[] m, int[] p, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] taua, @Cast("lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] taub,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sgebrd( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer d, FloatPointer e, FloatPointer tauq, FloatPointer taup, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sgebrd( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer d, FloatBuffer e, FloatBuffer tauq, FloatBuffer taup, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sgebrd( int[] m, int[] n, float[] a, int[] lda,
float[] d, float[] e, float[] tauq, float[] taup, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dgebrd( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer d, DoublePointer e, DoublePointer tauq, DoublePointer taup,
DoublePointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_dgebrd( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer tauq, DoubleBuffer taup,
DoubleBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dgebrd( int[] m, int[] n, double[] a, int[] lda,
double[] d, double[] e, double[] tauq, double[] taup,
double[] work, int[] lwork, int[] info );
public static native void LAPACK_cgebrd( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer tauq, @Cast("lapack_complex_float*") FloatPointer taup,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cgebrd( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer tauq, @Cast("lapack_complex_float*") FloatBuffer taup,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cgebrd( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] tauq, @Cast("lapack_complex_float*") float[] taup,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zgebrd( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer tauq, @Cast("lapack_complex_double*") DoublePointer taup,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zgebrd( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer tauq, @Cast("lapack_complex_double*") DoubleBuffer taup,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zgebrd( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] tauq, @Cast("lapack_complex_double*") double[] taup,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sgbbrd( @Cast("char*") BytePointer vect, IntPointer m, IntPointer n, IntPointer ncc,
IntPointer kl, IntPointer ku, FloatPointer ab, IntPointer ldab,
FloatPointer d, FloatPointer e, FloatPointer q, IntPointer ldq, FloatPointer pt,
IntPointer ldpt, FloatPointer c, IntPointer ldc, FloatPointer work,
IntPointer info );
public static native void LAPACK_sgbbrd( @Cast("char*") ByteBuffer vect, IntBuffer m, IntBuffer n, IntBuffer ncc,
IntBuffer kl, IntBuffer ku, FloatBuffer ab, IntBuffer ldab,
FloatBuffer d, FloatBuffer e, FloatBuffer q, IntBuffer ldq, FloatBuffer pt,
IntBuffer ldpt, FloatBuffer c, IntBuffer ldc, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_sgbbrd( @Cast("char*") byte[] vect, int[] m, int[] n, int[] ncc,
int[] kl, int[] ku, float[] ab, int[] ldab,
float[] d, float[] e, float[] q, int[] ldq, float[] pt,
int[] ldpt, float[] c, int[] ldc, float[] work,
int[] info );
public static native void LAPACK_dgbbrd( @Cast("char*") BytePointer vect, IntPointer m, IntPointer n, IntPointer ncc,
IntPointer kl, IntPointer ku, DoublePointer ab,
IntPointer ldab, DoublePointer d, DoublePointer e, DoublePointer q,
IntPointer ldq, DoublePointer pt, IntPointer ldpt, DoublePointer c,
IntPointer ldc, DoublePointer work, IntPointer info );
public static native void LAPACK_dgbbrd( @Cast("char*") ByteBuffer vect, IntBuffer m, IntBuffer n, IntBuffer ncc,
IntBuffer kl, IntBuffer ku, DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer d, DoubleBuffer e, DoubleBuffer q,
IntBuffer ldq, DoubleBuffer pt, IntBuffer ldpt, DoubleBuffer c,
IntBuffer ldc, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dgbbrd( @Cast("char*") byte[] vect, int[] m, int[] n, int[] ncc,
int[] kl, int[] ku, double[] ab,
int[] ldab, double[] d, double[] e, double[] q,
int[] ldq, double[] pt, int[] ldpt, double[] c,
int[] ldc, double[] work, int[] info );
public static native void LAPACK_cgbbrd( @Cast("char*") BytePointer vect, IntPointer m, IntPointer n, IntPointer ncc,
IntPointer kl, IntPointer ku, @Cast("lapack_complex_float*") FloatPointer ab,
IntPointer ldab, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer pt, IntPointer ldpt,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cgbbrd( @Cast("char*") ByteBuffer vect, IntBuffer m, IntBuffer n, IntBuffer ncc,
IntBuffer kl, IntBuffer ku, @Cast("lapack_complex_float*") FloatBuffer ab,
IntBuffer ldab, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer pt, IntBuffer ldpt,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cgbbrd( @Cast("char*") byte[] vect, int[] m, int[] n, int[] ncc,
int[] kl, int[] ku, @Cast("lapack_complex_float*") float[] ab,
int[] ldab, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] pt, int[] ldpt,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zgbbrd( @Cast("char*") BytePointer vect, IntPointer m, IntPointer n, IntPointer ncc,
IntPointer kl, IntPointer ku, @Cast("lapack_complex_double*") DoublePointer ab,
IntPointer ldab, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer pt, IntPointer ldpt,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zgbbrd( @Cast("char*") ByteBuffer vect, IntBuffer m, IntBuffer n, IntBuffer ncc,
IntBuffer kl, IntBuffer ku, @Cast("lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer pt, IntBuffer ldpt,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zgbbrd( @Cast("char*") byte[] vect, int[] m, int[] n, int[] ncc,
int[] kl, int[] ku, @Cast("lapack_complex_double*") double[] ab,
int[] ldab, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] pt, int[] ldpt,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sorgbr( @Cast("char*") BytePointer vect, IntPointer m, IntPointer n, IntPointer k,
FloatPointer a, IntPointer lda, @Const FloatPointer tau, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sorgbr( @Cast("char*") ByteBuffer vect, IntBuffer m, IntBuffer n, IntBuffer k,
FloatBuffer a, IntBuffer lda, @Const FloatBuffer tau, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sorgbr( @Cast("char*") byte[] vect, int[] m, int[] n, int[] k,
float[] a, int[] lda, @Const float[] tau, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dorgbr( @Cast("char*") BytePointer vect, IntPointer m, IntPointer n, IntPointer k,
DoublePointer a, IntPointer lda, @Const DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dorgbr( @Cast("char*") ByteBuffer vect, IntBuffer m, IntBuffer n, IntBuffer k,
DoubleBuffer a, IntBuffer lda, @Const DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dorgbr( @Cast("char*") byte[] vect, int[] m, int[] n, int[] k,
double[] a, int[] lda, @Const double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sormbr( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, IntPointer k, @Const FloatPointer a,
IntPointer lda, @Const FloatPointer tau, FloatPointer c,
IntPointer ldc, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sormbr( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, IntBuffer k, @Const FloatBuffer a,
IntBuffer lda, @Const FloatBuffer tau, FloatBuffer c,
IntBuffer ldc, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sormbr( @Cast("char*") byte[] vect, @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m,
int[] n, int[] k, @Const float[] a,
int[] lda, @Const float[] tau, float[] c,
int[] ldc, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dormbr( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, IntPointer k, @Const DoublePointer a,
IntPointer lda, @Const DoublePointer tau, DoublePointer c,
IntPointer ldc, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dormbr( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, IntBuffer k, @Const DoubleBuffer a,
IntBuffer lda, @Const DoubleBuffer tau, DoubleBuffer c,
IntBuffer ldc, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dormbr( @Cast("char*") byte[] vect, @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m,
int[] n, int[] k, @Const double[] a,
int[] lda, @Const double[] tau, double[] c,
int[] ldc, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cungbr( @Cast("char*") BytePointer vect, IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cungbr( @Cast("char*") ByteBuffer vect, IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cungbr( @Cast("char*") byte[] vect, int[] m, int[] n, int[] k,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zungbr( @Cast("char*") BytePointer vect, IntPointer m, IntPointer n, IntPointer k,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zungbr( @Cast("char*") ByteBuffer vect, IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zungbr( @Cast("char*") byte[] vect, int[] m, int[] n, int[] k,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cunmbr( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, IntPointer k, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cunmbr( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, IntBuffer k, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cunmbr( @Cast("char*") byte[] vect, @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m,
int[] n, int[] k, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zunmbr( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, IntPointer k,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer c,
IntPointer ldc, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zunmbr( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, IntBuffer k,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer c,
IntBuffer ldc, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zunmbr( @Cast("char*") byte[] vect, @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m,
int[] n, int[] k,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] c,
int[] ldc, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sbdsqr( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ncvt,
IntPointer nru, IntPointer ncc, FloatPointer d, FloatPointer e,
FloatPointer vt, IntPointer ldvt, FloatPointer u, IntPointer ldu,
FloatPointer c, IntPointer ldc, FloatPointer work, IntPointer info );
public static native void LAPACK_sbdsqr( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ncvt,
IntBuffer nru, IntBuffer ncc, FloatBuffer d, FloatBuffer e,
FloatBuffer vt, IntBuffer ldvt, FloatBuffer u, IntBuffer ldu,
FloatBuffer c, IntBuffer ldc, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sbdsqr( @Cast("char*") byte[] uplo, int[] n, int[] ncvt,
int[] nru, int[] ncc, float[] d, float[] e,
float[] vt, int[] ldvt, float[] u, int[] ldu,
float[] c, int[] ldc, float[] work, int[] info );
public static native void LAPACK_dbdsqr( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ncvt,
IntPointer nru, IntPointer ncc, DoublePointer d, DoublePointer e,
DoublePointer vt, IntPointer ldvt, DoublePointer u, IntPointer ldu,
DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer info );
public static native void LAPACK_dbdsqr( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ncvt,
IntBuffer nru, IntBuffer ncc, DoubleBuffer d, DoubleBuffer e,
DoubleBuffer vt, IntBuffer ldvt, DoubleBuffer u, IntBuffer ldu,
DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dbdsqr( @Cast("char*") byte[] uplo, int[] n, int[] ncvt,
int[] nru, int[] ncc, double[] d, double[] e,
double[] vt, int[] ldvt, double[] u, int[] ldu,
double[] c, int[] ldc, double[] work,
int[] info );
public static native void LAPACK_cbdsqr( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ncvt,
IntPointer nru, IntPointer ncc, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer vt, IntPointer ldvt,
@Cast("lapack_complex_float*") FloatPointer u, IntPointer ldu,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc, FloatPointer work,
IntPointer info );
public static native void LAPACK_cbdsqr( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ncvt,
IntBuffer nru, IntBuffer ncc, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer vt, IntBuffer ldvt,
@Cast("lapack_complex_float*") FloatBuffer u, IntBuffer ldu,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_cbdsqr( @Cast("char*") byte[] uplo, int[] n, int[] ncvt,
int[] nru, int[] ncc, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] vt, int[] ldvt,
@Cast("lapack_complex_float*") float[] u, int[] ldu,
@Cast("lapack_complex_float*") float[] c, int[] ldc, float[] work,
int[] info );
public static native void LAPACK_zbdsqr( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ncvt,
IntPointer nru, IntPointer ncc, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer vt, IntPointer ldvt,
@Cast("lapack_complex_double*") DoublePointer u, IntPointer ldu,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer info );
public static native void LAPACK_zbdsqr( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ncvt,
IntBuffer nru, IntBuffer ncc, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer vt, IntBuffer ldvt,
@Cast("lapack_complex_double*") DoubleBuffer u, IntBuffer ldu,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zbdsqr( @Cast("char*") byte[] uplo, int[] n, int[] ncvt,
int[] nru, int[] ncc, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] vt, int[] ldvt,
@Cast("lapack_complex_double*") double[] u, int[] ldu,
@Cast("lapack_complex_double*") double[] c, int[] ldc, double[] work,
int[] info );
public static native void LAPACK_sbdsdc( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer compq, IntPointer n, FloatPointer d, FloatPointer e,
FloatPointer u, IntPointer ldu, FloatPointer vt, IntPointer ldvt,
FloatPointer q, IntPointer iq, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sbdsdc( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer compq, IntBuffer n, FloatBuffer d, FloatBuffer e,
FloatBuffer u, IntBuffer ldu, FloatBuffer vt, IntBuffer ldvt,
FloatBuffer q, IntBuffer iq, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sbdsdc( @Cast("char*") byte[] uplo, @Cast("char*") byte[] compq, int[] n, float[] d, float[] e,
float[] u, int[] ldu, float[] vt, int[] ldvt,
float[] q, int[] iq, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dbdsdc( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer compq, IntPointer n, DoublePointer d,
DoublePointer e, DoublePointer u, IntPointer ldu, DoublePointer vt,
IntPointer ldvt, DoublePointer q, IntPointer iq, DoublePointer work,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dbdsdc( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer compq, IntBuffer n, DoubleBuffer d,
DoubleBuffer e, DoubleBuffer u, IntBuffer ldu, DoubleBuffer vt,
IntBuffer ldvt, DoubleBuffer q, IntBuffer iq, DoubleBuffer work,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dbdsdc( @Cast("char*") byte[] uplo, @Cast("char*") byte[] compq, int[] n, double[] d,
double[] e, double[] u, int[] ldu, double[] vt,
int[] ldvt, double[] q, int[] iq, double[] work,
int[] iwork, int[] info );
public static native void LAPACK_sbdsvdx( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range,
IntPointer n, FloatPointer d, FloatPointer e,
IntPointer vl, IntPointer vu,
IntPointer il, IntPointer iu, IntPointer ns,
FloatPointer s, FloatPointer z, IntPointer ldz,
FloatPointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_sbdsvdx( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range,
IntBuffer n, FloatBuffer d, FloatBuffer e,
IntBuffer vl, IntBuffer vu,
IntBuffer il, IntBuffer iu, IntBuffer ns,
FloatBuffer s, FloatBuffer z, IntBuffer ldz,
FloatBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sbdsvdx( @Cast("char*") byte[] uplo, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range,
int[] n, float[] d, float[] e,
int[] vl, int[] vu,
int[] il, int[] iu, int[] ns,
float[] s, float[] z, int[] ldz,
float[] work, int[] iwork, int[] info );
public static native void LAPACK_dbdsvdx( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range,
IntPointer n, DoublePointer d, DoublePointer e,
IntPointer vl, IntPointer vu,
IntPointer il, IntPointer iu, IntPointer ns,
DoublePointer s, DoublePointer z, IntPointer ldz,
DoublePointer work, IntPointer iwork, IntPointer info );
public static native void LAPACK_dbdsvdx( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range,
IntBuffer n, DoubleBuffer d, DoubleBuffer e,
IntBuffer vl, IntBuffer vu,
IntBuffer il, IntBuffer iu, IntBuffer ns,
DoubleBuffer s, DoubleBuffer z, IntBuffer ldz,
DoubleBuffer work, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dbdsvdx( @Cast("char*") byte[] uplo, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range,
int[] n, double[] d, double[] e,
int[] vl, int[] vu,
int[] il, int[] iu, int[] ns,
double[] s, double[] z, int[] ldz,
double[] work, int[] iwork, int[] info );
public static native void LAPACK_ssytrd( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer d, FloatPointer e, FloatPointer tau, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_ssytrd( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer d, FloatBuffer e, FloatBuffer tau, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_ssytrd( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
float[] d, float[] e, float[] tau, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dsytrd( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer d, DoublePointer e, DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dsytrd( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer d, DoubleBuffer e, DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dsytrd( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
double[] d, double[] e, double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sorgtr( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
@Const FloatPointer tau, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sorgtr( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
@Const FloatBuffer tau, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sorgtr( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
@Const float[] tau, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dorgtr( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
@Const DoublePointer tau, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dorgtr( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
@Const DoubleBuffer tau, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dorgtr( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
@Const double[] tau, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sormtr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, @Const FloatPointer a, IntPointer lda,
@Const FloatPointer tau, FloatPointer c, IntPointer ldc, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sormtr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, @Const FloatBuffer a, IntBuffer lda,
@Const FloatBuffer tau, FloatBuffer c, IntBuffer ldc, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sormtr( @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] m,
int[] n, @Const float[] a, int[] lda,
@Const float[] tau, float[] c, int[] ldc, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dormtr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, @Const DoublePointer a, IntPointer lda,
@Const DoublePointer tau, DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dormtr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, @Const DoubleBuffer a, IntBuffer lda,
@Const DoubleBuffer tau, DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dormtr( @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] m,
int[] n, @Const double[] a, int[] lda,
@Const double[] tau, double[] c, int[] ldc, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_chetrd( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_chetrd( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_chetrd( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zhetrd( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zhetrd( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zhetrd( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cungtr( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cungtr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cungtr( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zungtr( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zungtr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zungtr( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cunmtr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cunmtr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cunmtr( @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] m,
int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zunmtr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zunmtr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zunmtr( @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] m,
int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_ssptrd( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer ap, FloatPointer d, FloatPointer e,
FloatPointer tau, IntPointer info );
public static native void LAPACK_ssptrd( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer ap, FloatBuffer d, FloatBuffer e,
FloatBuffer tau, IntBuffer info );
public static native void LAPACK_ssptrd( @Cast("char*") byte[] uplo, int[] n, float[] ap, float[] d, float[] e,
float[] tau, int[] info );
public static native void LAPACK_dsptrd( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer ap, DoublePointer d, DoublePointer e,
DoublePointer tau, IntPointer info );
public static native void LAPACK_dsptrd( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer ap, DoubleBuffer d, DoubleBuffer e,
DoubleBuffer tau, IntBuffer info );
public static native void LAPACK_dsptrd( @Cast("char*") byte[] uplo, int[] n, double[] ap, double[] d, double[] e,
double[] tau, int[] info );
public static native void LAPACK_sopgtr( @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer ap,
@Const FloatPointer tau, FloatPointer q, IntPointer ldq, FloatPointer work,
IntPointer info );
public static native void LAPACK_sopgtr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer ap,
@Const FloatBuffer tau, FloatBuffer q, IntBuffer ldq, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_sopgtr( @Cast("char*") byte[] uplo, int[] n, @Const float[] ap,
@Const float[] tau, float[] q, int[] ldq, float[] work,
int[] info );
public static native void LAPACK_dopgtr( @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer ap,
@Const DoublePointer tau, DoublePointer q, IntPointer ldq, DoublePointer work,
IntPointer info );
public static native void LAPACK_dopgtr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer ap,
@Const DoubleBuffer tau, DoubleBuffer q, IntBuffer ldq, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dopgtr( @Cast("char*") byte[] uplo, int[] n, @Const double[] ap,
@Const double[] tau, double[] q, int[] ldq, double[] work,
int[] info );
public static native void LAPACK_sopmtr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, @Const FloatPointer ap, @Const FloatPointer tau, FloatPointer c,
IntPointer ldc, FloatPointer work, IntPointer info );
public static native void LAPACK_sopmtr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, @Const FloatBuffer ap, @Const FloatBuffer tau, FloatBuffer c,
IntBuffer ldc, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sopmtr( @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] m,
int[] n, @Const float[] ap, @Const float[] tau, float[] c,
int[] ldc, float[] work, int[] info );
public static native void LAPACK_dopmtr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, @Const DoublePointer ap, @Const DoublePointer tau,
DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer info );
public static native void LAPACK_dopmtr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, @Const DoubleBuffer ap, @Const DoubleBuffer tau,
DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dopmtr( @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] m,
int[] n, @Const double[] ap, @Const double[] tau,
double[] c, int[] ldc, double[] work,
int[] info );
public static native void LAPACK_chptrd( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer ap,
FloatPointer d, FloatPointer e, @Cast("lapack_complex_float*") FloatPointer tau,
IntPointer info );
public static native void LAPACK_chptrd( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer ap,
FloatBuffer d, FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer tau,
IntBuffer info );
public static native void LAPACK_chptrd( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] ap,
float[] d, float[] e, @Cast("lapack_complex_float*") float[] tau,
int[] info );
public static native void LAPACK_zhptrd( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer ap,
DoublePointer d, DoublePointer e, @Cast("lapack_complex_double*") DoublePointer tau,
IntPointer info );
public static native void LAPACK_zhptrd( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer ap,
DoubleBuffer d, DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer tau,
IntBuffer info );
public static native void LAPACK_zhptrd( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] ap,
double[] d, double[] e, @Cast("lapack_complex_double*") double[] tau,
int[] info );
public static native void LAPACK_cupgtr( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer q,
IntPointer ldq, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer info );
public static native void LAPACK_cupgtr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer q,
IntBuffer ldq, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer info );
public static native void LAPACK_cupgtr( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] q,
int[] ldq, @Cast("lapack_complex_float*") float[] work,
int[] info );
public static native void LAPACK_zupgtr( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer q,
IntPointer ldq, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer info );
public static native void LAPACK_zupgtr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer q,
IntBuffer ldq, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zupgtr( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] q,
int[] ldq, @Cast("lapack_complex_double*") double[] work,
int[] info );
public static native void LAPACK_cupmtr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer c,
IntPointer ldc, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer info );
public static native void LAPACK_cupmtr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer c,
IntBuffer ldc, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer info );
public static native void LAPACK_cupmtr( @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] m,
int[] n, @Cast("const lapack_complex_float*") float[] ap,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] c,
int[] ldc, @Cast("lapack_complex_float*") float[] work,
int[] info );
public static native void LAPACK_zupmtr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer m,
IntPointer n, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("const lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer c,
IntPointer ldc, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer info );
public static native void LAPACK_zupmtr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer m,
IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("const lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer c,
IntBuffer ldc, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zupmtr( @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] m,
int[] n, @Cast("const lapack_complex_double*") double[] ap,
@Cast("const lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] c,
int[] ldc, @Cast("lapack_complex_double*") double[] work,
int[] info );
public static native void LAPACK_ssbtrd( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
FloatPointer ab, IntPointer ldab, FloatPointer d, FloatPointer e, FloatPointer q,
IntPointer ldq, FloatPointer work, IntPointer info );
public static native void LAPACK_ssbtrd( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
FloatBuffer ab, IntBuffer ldab, FloatBuffer d, FloatBuffer e, FloatBuffer q,
IntBuffer ldq, FloatBuffer work, IntBuffer info );
public static native void LAPACK_ssbtrd( @Cast("char*") byte[] vect, @Cast("char*") byte[] uplo, int[] n, int[] kd,
float[] ab, int[] ldab, float[] d, float[] e, float[] q,
int[] ldq, float[] work, int[] info );
public static native void LAPACK_dsbtrd( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
DoublePointer ab, IntPointer ldab, DoublePointer d, DoublePointer e,
DoublePointer q, IntPointer ldq, DoublePointer work,
IntPointer info );
public static native void LAPACK_dsbtrd( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
DoubleBuffer ab, IntBuffer ldab, DoubleBuffer d, DoubleBuffer e,
DoubleBuffer q, IntBuffer ldq, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dsbtrd( @Cast("char*") byte[] vect, @Cast("char*") byte[] uplo, int[] n, int[] kd,
double[] ab, int[] ldab, double[] d, double[] e,
double[] q, int[] ldq, double[] work,
int[] info );
public static native void LAPACK_chbtrd( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab, FloatPointer d,
FloatPointer e, @Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_chbtrd( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab, FloatBuffer d,
FloatBuffer e, @Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_chbtrd( @Cast("char*") byte[] vect, @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("lapack_complex_float*") float[] ab, int[] ldab, float[] d,
float[] e, @Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zhbtrd( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab, DoublePointer d,
DoublePointer e, @Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zhbtrd( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab, DoubleBuffer d,
DoubleBuffer e, @Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zhbtrd( @Cast("char*") byte[] vect, @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("lapack_complex_double*") double[] ab, int[] ldab, double[] d,
double[] e, @Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_ssterf( IntPointer n, FloatPointer d, FloatPointer e, IntPointer info );
public static native void LAPACK_ssterf( IntBuffer n, FloatBuffer d, FloatBuffer e, IntBuffer info );
public static native void LAPACK_ssterf( int[] n, float[] d, float[] e, int[] info );
public static native void LAPACK_dsterf( IntPointer n, DoublePointer d, DoublePointer e, IntPointer info );
public static native void LAPACK_dsterf( IntBuffer n, DoubleBuffer d, DoubleBuffer e, IntBuffer info );
public static native void LAPACK_dsterf( int[] n, double[] d, double[] e, int[] info );
public static native void LAPACK_ssteqr( @Cast("char*") BytePointer compz, IntPointer n, FloatPointer d, FloatPointer e, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer info );
public static native void LAPACK_ssteqr( @Cast("char*") ByteBuffer compz, IntBuffer n, FloatBuffer d, FloatBuffer e, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer info );
public static native void LAPACK_ssteqr( @Cast("char*") byte[] compz, int[] n, float[] d, float[] e, float[] z,
int[] ldz, float[] work, int[] info );
public static native void LAPACK_dsteqr( @Cast("char*") BytePointer compz, IntPointer n, DoublePointer d, DoublePointer e, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer info );
public static native void LAPACK_dsteqr( @Cast("char*") ByteBuffer compz, IntBuffer n, DoubleBuffer d, DoubleBuffer e, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dsteqr( @Cast("char*") byte[] compz, int[] n, double[] d, double[] e, double[] z,
int[] ldz, double[] work, int[] info );
public static native void LAPACK_csteqr( @Cast("char*") BytePointer compz, IntPointer n, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz, FloatPointer work,
IntPointer info );
public static native void LAPACK_csteqr( @Cast("char*") ByteBuffer compz, IntBuffer n, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_csteqr( @Cast("char*") byte[] compz, int[] n, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] z, int[] ldz, float[] work,
int[] info );
public static native void LAPACK_zsteqr( @Cast("char*") BytePointer compz, IntPointer n, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz, DoublePointer work,
IntPointer info );
public static native void LAPACK_zsteqr( @Cast("char*") ByteBuffer compz, IntBuffer n, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zsteqr( @Cast("char*") byte[] compz, int[] n, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] z, int[] ldz, double[] work,
int[] info );
public static native void LAPACK_sstemr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, FloatPointer d, FloatPointer e,
FloatPointer vl, FloatPointer vu, IntPointer il, IntPointer iu,
IntPointer m, FloatPointer w, FloatPointer z, IntPointer ldz,
IntPointer nzc, IntPointer isuppz, IntPointer tryrac,
FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_sstemr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, FloatBuffer d, FloatBuffer e,
FloatBuffer vl, FloatBuffer vu, IntBuffer il, IntBuffer iu,
IntBuffer m, FloatBuffer w, FloatBuffer z, IntBuffer ldz,
IntBuffer nzc, IntBuffer isuppz, IntBuffer tryrac,
FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_sstemr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, float[] d, float[] e,
float[] vl, float[] vu, int[] il, int[] iu,
int[] m, float[] w, float[] z, int[] ldz,
int[] nzc, int[] isuppz, int[] tryrac,
float[] work, int[] lwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_dstemr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, DoublePointer d,
DoublePointer e, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, IntPointer m, DoublePointer w, DoublePointer z,
IntPointer ldz, IntPointer nzc, IntPointer isuppz,
IntPointer tryrac, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dstemr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, DoubleBuffer d,
DoubleBuffer e, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, IntBuffer m, DoubleBuffer w, DoubleBuffer z,
IntBuffer ldz, IntBuffer nzc, IntBuffer isuppz,
IntBuffer tryrac, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dstemr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, double[] d,
double[] e, double[] vl, double[] vu, int[] il,
int[] iu, int[] m, double[] w, double[] z,
int[] ldz, int[] nzc, int[] isuppz,
int[] tryrac, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_cstemr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, FloatPointer d, FloatPointer e,
FloatPointer vl, FloatPointer vu, IntPointer il, IntPointer iu,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, IntPointer nzc, IntPointer isuppz,
IntPointer tryrac, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_cstemr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, FloatBuffer d, FloatBuffer e,
FloatBuffer vl, FloatBuffer vu, IntBuffer il, IntBuffer iu,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, IntBuffer nzc, IntBuffer isuppz,
IntBuffer tryrac, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_cstemr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, float[] d, float[] e,
float[] vl, float[] vu, int[] il, int[] iu,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, int[] nzc, int[] isuppz,
int[] tryrac, float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_zstemr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, DoublePointer d,
DoublePointer e, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz, IntPointer nzc,
IntPointer isuppz, IntPointer tryrac, DoublePointer work,
IntPointer lwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_zstemr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, DoubleBuffer d,
DoubleBuffer e, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz, IntBuffer nzc,
IntBuffer isuppz, IntBuffer tryrac, DoubleBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_zstemr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, double[] d,
double[] e, double[] vl, double[] vu, int[] il,
int[] iu, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int[] ldz, int[] nzc,
int[] isuppz, int[] tryrac, double[] work,
int[] lwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_sstedc( @Cast("char*") BytePointer compz, IntPointer n, FloatPointer d, FloatPointer e, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_sstedc( @Cast("char*") ByteBuffer compz, IntBuffer n, FloatBuffer d, FloatBuffer e, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_sstedc( @Cast("char*") byte[] compz, int[] n, float[] d, float[] e, float[] z,
int[] ldz, float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_dstedc( @Cast("char*") BytePointer compz, IntPointer n, DoublePointer d, DoublePointer e, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dstedc( @Cast("char*") ByteBuffer compz, IntBuffer n, DoubleBuffer d, DoubleBuffer e, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dstedc( @Cast("char*") byte[] compz, int[] n, double[] d, double[] e, double[] z,
int[] ldz, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_cstedc( @Cast("char*") BytePointer compz, IntPointer n, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer lrwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_cstedc( @Cast("char*") ByteBuffer compz, IntBuffer n, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer lrwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_cstedc( @Cast("char*") byte[] compz, int[] n, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] lrwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_zstedc( @Cast("char*") BytePointer compz, IntPointer n, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_zstedc( @Cast("char*") ByteBuffer compz, IntBuffer n, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_zstedc( @Cast("char*") byte[] compz, int[] n, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_sstegr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, FloatPointer d, FloatPointer e,
FloatPointer vl, FloatPointer vu, IntPointer il, IntPointer iu,
FloatPointer abstol, IntPointer m, FloatPointer w, FloatPointer z,
IntPointer ldz, IntPointer isuppz, FloatPointer work,
IntPointer lwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_sstegr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, FloatBuffer d, FloatBuffer e,
FloatBuffer vl, FloatBuffer vu, IntBuffer il, IntBuffer iu,
FloatBuffer abstol, IntBuffer m, FloatBuffer w, FloatBuffer z,
IntBuffer ldz, IntBuffer isuppz, FloatBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_sstegr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, float[] d, float[] e,
float[] vl, float[] vu, int[] il, int[] iu,
float[] abstol, int[] m, float[] w, float[] z,
int[] ldz, int[] isuppz, float[] work,
int[] lwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_dstegr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, DoublePointer d,
DoublePointer e, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, DoublePointer abstol, IntPointer m, DoublePointer w,
DoublePointer z, IntPointer ldz, IntPointer isuppz,
DoublePointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_dstegr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, DoubleBuffer d,
DoubleBuffer e, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, DoubleBuffer abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, IntBuffer ldz, IntBuffer isuppz,
DoubleBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dstegr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, double[] d,
double[] e, double[] vl, double[] vu, int[] il,
int[] iu, double[] abstol, int[] m, double[] w,
double[] z, int[] ldz, int[] isuppz,
double[] work, int[] lwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_cstegr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, FloatPointer d, FloatPointer e,
FloatPointer vl, FloatPointer vu, IntPointer il, IntPointer iu,
FloatPointer abstol, IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
IntPointer isuppz, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_cstegr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, FloatBuffer d, FloatBuffer e,
FloatBuffer vl, FloatBuffer vu, IntBuffer il, IntBuffer iu,
FloatBuffer abstol, IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
IntBuffer isuppz, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_cstegr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, float[] d, float[] e,
float[] vl, float[] vu, int[] il, int[] iu,
float[] abstol, int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
int[] isuppz, float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_zstegr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, DoublePointer d,
DoublePointer e, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, DoublePointer abstol, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
IntPointer isuppz, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_zstegr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, DoubleBuffer d,
DoubleBuffer e, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, DoubleBuffer abstol, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
IntBuffer isuppz, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_zstegr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, double[] d,
double[] e, double[] vl, double[] vu, int[] il,
int[] iu, double[] abstol, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
int[] isuppz, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_spteqr( @Cast("char*") BytePointer compz, IntPointer n, FloatPointer d, FloatPointer e, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer info );
public static native void LAPACK_spteqr( @Cast("char*") ByteBuffer compz, IntBuffer n, FloatBuffer d, FloatBuffer e, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer info );
public static native void LAPACK_spteqr( @Cast("char*") byte[] compz, int[] n, float[] d, float[] e, float[] z,
int[] ldz, float[] work, int[] info );
public static native void LAPACK_dpteqr( @Cast("char*") BytePointer compz, IntPointer n, DoublePointer d, DoublePointer e, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer info );
public static native void LAPACK_dpteqr( @Cast("char*") ByteBuffer compz, IntBuffer n, DoubleBuffer d, DoubleBuffer e, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dpteqr( @Cast("char*") byte[] compz, int[] n, double[] d, double[] e, double[] z,
int[] ldz, double[] work, int[] info );
public static native void LAPACK_cpteqr( @Cast("char*") BytePointer compz, IntPointer n, FloatPointer d, FloatPointer e,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz, FloatPointer work,
IntPointer info );
public static native void LAPACK_cpteqr( @Cast("char*") ByteBuffer compz, IntBuffer n, FloatBuffer d, FloatBuffer e,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_cpteqr( @Cast("char*") byte[] compz, int[] n, float[] d, float[] e,
@Cast("lapack_complex_float*") float[] z, int[] ldz, float[] work,
int[] info );
public static native void LAPACK_zpteqr( @Cast("char*") BytePointer compz, IntPointer n, DoublePointer d, DoublePointer e,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz, DoublePointer work,
IntPointer info );
public static native void LAPACK_zpteqr( @Cast("char*") ByteBuffer compz, IntBuffer n, DoubleBuffer d, DoubleBuffer e,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zpteqr( @Cast("char*") byte[] compz, int[] n, double[] d, double[] e,
@Cast("lapack_complex_double*") double[] z, int[] ldz, double[] work,
int[] info );
public static native void LAPACK_sstebz( @Cast("char*") BytePointer range, @Cast("char*") BytePointer order, IntPointer n, FloatPointer vl,
FloatPointer vu, IntPointer il, IntPointer iu, FloatPointer abstol,
@Const FloatPointer d, @Const FloatPointer e, IntPointer m,
IntPointer nsplit, FloatPointer w, IntPointer iblock,
IntPointer isplit, FloatPointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sstebz( @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer order, IntBuffer n, FloatBuffer vl,
FloatBuffer vu, IntBuffer il, IntBuffer iu, FloatBuffer abstol,
@Const FloatBuffer d, @Const FloatBuffer e, IntBuffer m,
IntBuffer nsplit, FloatBuffer w, IntBuffer iblock,
IntBuffer isplit, FloatBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sstebz( @Cast("char*") byte[] range, @Cast("char*") byte[] order, int[] n, float[] vl,
float[] vu, int[] il, int[] iu, float[] abstol,
@Const float[] d, @Const float[] e, int[] m,
int[] nsplit, float[] w, int[] iblock,
int[] isplit, float[] work, int[] iwork,
int[] info );
public static native void LAPACK_dstebz( @Cast("char*") BytePointer range, @Cast("char*") BytePointer order, IntPointer n, DoublePointer vl,
DoublePointer vu, IntPointer il, IntPointer iu, DoublePointer abstol,
@Const DoublePointer d, @Const DoublePointer e, IntPointer m,
IntPointer nsplit, DoublePointer w, IntPointer iblock,
IntPointer isplit, DoublePointer work, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dstebz( @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer order, IntBuffer n, DoubleBuffer vl,
DoubleBuffer vu, IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
@Const DoubleBuffer d, @Const DoubleBuffer e, IntBuffer m,
IntBuffer nsplit, DoubleBuffer w, IntBuffer iblock,
IntBuffer isplit, DoubleBuffer work, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dstebz( @Cast("char*") byte[] range, @Cast("char*") byte[] order, int[] n, double[] vl,
double[] vu, int[] il, int[] iu, double[] abstol,
@Const double[] d, @Const double[] e, int[] m,
int[] nsplit, double[] w, int[] iblock,
int[] isplit, double[] work, int[] iwork,
int[] info );
public static native void LAPACK_sstein( IntPointer n, @Const FloatPointer d, @Const FloatPointer e,
IntPointer m, @Const FloatPointer w, @Const IntPointer iblock,
@Const IntPointer isplit, FloatPointer z, IntPointer ldz,
FloatPointer work, IntPointer iwork, IntPointer ifailv,
IntPointer info );
public static native void LAPACK_sstein( IntBuffer n, @Const FloatBuffer d, @Const FloatBuffer e,
IntBuffer m, @Const FloatBuffer w, @Const IntBuffer iblock,
@Const IntBuffer isplit, FloatBuffer z, IntBuffer ldz,
FloatBuffer work, IntBuffer iwork, IntBuffer ifailv,
IntBuffer info );
public static native void LAPACK_sstein( int[] n, @Const float[] d, @Const float[] e,
int[] m, @Const float[] w, @Const int[] iblock,
@Const int[] isplit, float[] z, int[] ldz,
float[] work, int[] iwork, int[] ifailv,
int[] info );
public static native void LAPACK_dstein( IntPointer n, @Const DoublePointer d, @Const DoublePointer e,
IntPointer m, @Const DoublePointer w, @Const IntPointer iblock,
@Const IntPointer isplit, DoublePointer z, IntPointer ldz,
DoublePointer work, IntPointer iwork, IntPointer ifailv,
IntPointer info );
public static native void LAPACK_dstein( IntBuffer n, @Const DoubleBuffer d, @Const DoubleBuffer e,
IntBuffer m, @Const DoubleBuffer w, @Const IntBuffer iblock,
@Const IntBuffer isplit, DoubleBuffer z, IntBuffer ldz,
DoubleBuffer work, IntBuffer iwork, IntBuffer ifailv,
IntBuffer info );
public static native void LAPACK_dstein( int[] n, @Const double[] d, @Const double[] e,
int[] m, @Const double[] w, @Const int[] iblock,
@Const int[] isplit, double[] z, int[] ldz,
double[] work, int[] iwork, int[] ifailv,
int[] info );
public static native void LAPACK_cstein( IntPointer n, @Const FloatPointer d, @Const FloatPointer e,
IntPointer m, @Const FloatPointer w, @Const IntPointer iblock,
@Const IntPointer isplit, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer iwork,
IntPointer ifailv, IntPointer info );
public static native void LAPACK_cstein( IntBuffer n, @Const FloatBuffer d, @Const FloatBuffer e,
IntBuffer m, @Const FloatBuffer w, @Const IntBuffer iblock,
@Const IntBuffer isplit, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifailv, IntBuffer info );
public static native void LAPACK_cstein( int[] n, @Const float[] d, @Const float[] e,
int[] m, @Const float[] w, @Const int[] iblock,
@Const int[] isplit, @Cast("lapack_complex_float*") float[] z,
int[] ldz, float[] work, int[] iwork,
int[] ifailv, int[] info );
public static native void LAPACK_zstein( IntPointer n, @Const DoublePointer d, @Const DoublePointer e,
IntPointer m, @Const DoublePointer w, @Const IntPointer iblock,
@Const IntPointer isplit, @Cast("lapack_complex_double*") DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer iwork,
IntPointer ifailv, IntPointer info );
public static native void LAPACK_zstein( IntBuffer n, @Const DoubleBuffer d, @Const DoubleBuffer e,
IntBuffer m, @Const DoubleBuffer w, @Const IntBuffer iblock,
@Const IntBuffer isplit, @Cast("lapack_complex_double*") DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer iwork,
IntBuffer ifailv, IntBuffer info );
public static native void LAPACK_zstein( int[] n, @Const double[] d, @Const double[] e,
int[] m, @Const double[] w, @Const int[] iblock,
@Const int[] isplit, @Cast("lapack_complex_double*") double[] z,
int[] ldz, double[] work, int[] iwork,
int[] ifailv, int[] info );
public static native void LAPACK_sdisna( @Cast("char*") BytePointer job, IntPointer m, IntPointer n, @Const FloatPointer d,
FloatPointer sep, IntPointer info );
public static native void LAPACK_sdisna( @Cast("char*") ByteBuffer job, IntBuffer m, IntBuffer n, @Const FloatBuffer d,
FloatBuffer sep, IntBuffer info );
public static native void LAPACK_sdisna( @Cast("char*") byte[] job, int[] m, int[] n, @Const float[] d,
float[] sep, int[] info );
public static native void LAPACK_ddisna( @Cast("char*") BytePointer job, IntPointer m, IntPointer n, @Const DoublePointer d,
DoublePointer sep, IntPointer info );
public static native void LAPACK_ddisna( @Cast("char*") ByteBuffer job, IntBuffer m, IntBuffer n, @Const DoubleBuffer d,
DoubleBuffer sep, IntBuffer info );
public static native void LAPACK_ddisna( @Cast("char*") byte[] job, int[] m, int[] n, @Const double[] d,
double[] sep, int[] info );
public static native void LAPACK_ssygst( IntPointer itype, @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a,
IntPointer lda, @Const FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_ssygst( IntBuffer itype, @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a,
IntBuffer lda, @Const FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_ssygst( int[] itype, @Cast("char*") byte[] uplo, int[] n, float[] a,
int[] lda, @Const float[] b, int[] ldb,
int[] info );
public static native void LAPACK_dsygst( IntPointer itype, @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a,
IntPointer lda, @Const DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_dsygst( IntBuffer itype, @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a,
IntBuffer lda, @Const DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_dsygst( int[] itype, @Cast("char*") byte[] uplo, int[] n, double[] a,
int[] lda, @Const double[] b, int[] ldb,
int[] info );
public static native void LAPACK_chegst( IntPointer itype, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_chegst( IntBuffer itype, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_chegst( int[] itype, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
int[] info );
public static native void LAPACK_zhegst( IntPointer itype, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer info );
public static native void LAPACK_zhegst( IntBuffer itype, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer info );
public static native void LAPACK_zhegst( int[] itype, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
int[] info );
public static native void LAPACK_sspgst( IntPointer itype, @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer ap,
@Const FloatPointer bp, IntPointer info );
public static native void LAPACK_sspgst( IntBuffer itype, @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer ap,
@Const FloatBuffer bp, IntBuffer info );
public static native void LAPACK_sspgst( int[] itype, @Cast("char*") byte[] uplo, int[] n, float[] ap,
@Const float[] bp, int[] info );
public static native void LAPACK_dspgst( IntPointer itype, @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer ap,
@Const DoublePointer bp, IntPointer info );
public static native void LAPACK_dspgst( IntBuffer itype, @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer ap,
@Const DoubleBuffer bp, IntBuffer info );
public static native void LAPACK_dspgst( int[] itype, @Cast("char*") byte[] uplo, int[] n, double[] ap,
@Const double[] bp, int[] info );
public static native void LAPACK_chpgst( IntPointer itype, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer ap, @Cast("const lapack_complex_float*") FloatPointer bp,
IntPointer info );
public static native void LAPACK_chpgst( IntBuffer itype, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer ap, @Cast("const lapack_complex_float*") FloatBuffer bp,
IntBuffer info );
public static native void LAPACK_chpgst( int[] itype, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] ap, @Cast("const lapack_complex_float*") float[] bp,
int[] info );
public static native void LAPACK_zhpgst( IntPointer itype, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer ap, @Cast("const lapack_complex_double*") DoublePointer bp,
IntPointer info );
public static native void LAPACK_zhpgst( IntBuffer itype, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer ap, @Cast("const lapack_complex_double*") DoubleBuffer bp,
IntBuffer info );
public static native void LAPACK_zhpgst( int[] itype, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] ap, @Cast("const lapack_complex_double*") double[] bp,
int[] info );
public static native void LAPACK_ssbgst( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, FloatPointer ab, IntPointer ldab,
@Const FloatPointer bb, IntPointer ldbb, FloatPointer x,
IntPointer ldx, FloatPointer work, IntPointer info );
public static native void LAPACK_ssbgst( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, FloatBuffer ab, IntBuffer ldab,
@Const FloatBuffer bb, IntBuffer ldbb, FloatBuffer x,
IntBuffer ldx, FloatBuffer work, IntBuffer info );
public static native void LAPACK_ssbgst( @Cast("char*") byte[] vect, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, float[] ab, int[] ldab,
@Const float[] bb, int[] ldbb, float[] x,
int[] ldx, float[] work, int[] info );
public static native void LAPACK_dsbgst( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, DoublePointer ab, IntPointer ldab,
@Const DoublePointer bb, IntPointer ldbb, DoublePointer x,
IntPointer ldx, DoublePointer work, IntPointer info );
public static native void LAPACK_dsbgst( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, DoubleBuffer ab, IntBuffer ldab,
@Const DoubleBuffer bb, IntBuffer ldbb, DoubleBuffer x,
IntBuffer ldx, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dsbgst( @Cast("char*") byte[] vect, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, double[] ab, int[] ldab,
@Const double[] bb, int[] ldbb, double[] x,
int[] ldx, double[] work, int[] info );
public static native void LAPACK_chbgst( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, @Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Cast("const lapack_complex_float*") FloatPointer bb, IntPointer ldbb,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_chbgst( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, @Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Cast("const lapack_complex_float*") FloatBuffer bb, IntBuffer ldbb,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_chbgst( @Cast("char*") byte[] vect, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, @Cast("lapack_complex_float*") float[] ab, int[] ldab,
@Cast("const lapack_complex_float*") float[] bb, int[] ldbb,
@Cast("lapack_complex_float*") float[] x, int[] ldx,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zhbgst( @Cast("char*") BytePointer vect, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, @Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Cast("const lapack_complex_double*") DoublePointer bb, IntPointer ldbb,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zhbgst( @Cast("char*") ByteBuffer vect, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, @Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Cast("const lapack_complex_double*") DoubleBuffer bb, IntBuffer ldbb,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zhbgst( @Cast("char*") byte[] vect, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, @Cast("lapack_complex_double*") double[] ab, int[] ldab,
@Cast("const lapack_complex_double*") double[] bb, int[] ldbb,
@Cast("lapack_complex_double*") double[] x, int[] ldx,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_spbstf( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kb, FloatPointer bb,
IntPointer ldbb, IntPointer info );
public static native void LAPACK_spbstf( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kb, FloatBuffer bb,
IntBuffer ldbb, IntBuffer info );
public static native void LAPACK_spbstf( @Cast("char*") byte[] uplo, int[] n, int[] kb, float[] bb,
int[] ldbb, int[] info );
public static native void LAPACK_dpbstf( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kb, DoublePointer bb,
IntPointer ldbb, IntPointer info );
public static native void LAPACK_dpbstf( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kb, DoubleBuffer bb,
IntBuffer ldbb, IntBuffer info );
public static native void LAPACK_dpbstf( @Cast("char*") byte[] uplo, int[] n, int[] kb, double[] bb,
int[] ldbb, int[] info );
public static native void LAPACK_cpbstf( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kb,
@Cast("lapack_complex_float*") FloatPointer bb, IntPointer ldbb,
IntPointer info );
public static native void LAPACK_cpbstf( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kb,
@Cast("lapack_complex_float*") FloatBuffer bb, IntBuffer ldbb,
IntBuffer info );
public static native void LAPACK_cpbstf( @Cast("char*") byte[] uplo, int[] n, int[] kb,
@Cast("lapack_complex_float*") float[] bb, int[] ldbb,
int[] info );
public static native void LAPACK_zpbstf( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kb,
@Cast("lapack_complex_double*") DoublePointer bb, IntPointer ldbb,
IntPointer info );
public static native void LAPACK_zpbstf( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kb,
@Cast("lapack_complex_double*") DoubleBuffer bb, IntBuffer ldbb,
IntBuffer info );
public static native void LAPACK_zpbstf( @Cast("char*") byte[] uplo, int[] n, int[] kb,
@Cast("lapack_complex_double*") double[] bb, int[] ldbb,
int[] info );
public static native void LAPACK_sgehrd( IntPointer n, IntPointer ilo, IntPointer ihi, FloatPointer a,
IntPointer lda, FloatPointer tau, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sgehrd( IntBuffer n, IntBuffer ilo, IntBuffer ihi, FloatBuffer a,
IntBuffer lda, FloatBuffer tau, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sgehrd( int[] n, int[] ilo, int[] ihi, float[] a,
int[] lda, float[] tau, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dgehrd( IntPointer n, IntPointer ilo, IntPointer ihi, DoublePointer a,
IntPointer lda, DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dgehrd( IntBuffer n, IntBuffer ilo, IntBuffer ihi, DoubleBuffer a,
IntBuffer lda, DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dgehrd( int[] n, int[] ilo, int[] ihi, double[] a,
int[] lda, double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cgehrd( IntPointer n, IntPointer ilo, IntPointer ihi,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cgehrd( IntBuffer n, IntBuffer ilo, IntBuffer ihi,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cgehrd( int[] n, int[] ilo, int[] ihi,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zgehrd( IntPointer n, IntPointer ilo, IntPointer ihi,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zgehrd( IntBuffer n, IntBuffer ilo, IntBuffer ihi,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zgehrd( int[] n, int[] ilo, int[] ihi,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sorghr( IntPointer n, IntPointer ilo, IntPointer ihi, FloatPointer a,
IntPointer lda, @Const FloatPointer tau, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sorghr( IntBuffer n, IntBuffer ilo, IntBuffer ihi, FloatBuffer a,
IntBuffer lda, @Const FloatBuffer tau, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sorghr( int[] n, int[] ilo, int[] ihi, float[] a,
int[] lda, @Const float[] tau, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dorghr( IntPointer n, IntPointer ilo, IntPointer ihi, DoublePointer a,
IntPointer lda, @Const DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dorghr( IntBuffer n, IntBuffer ilo, IntBuffer ihi, DoubleBuffer a,
IntBuffer lda, @Const DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dorghr( int[] n, int[] ilo, int[] ihi, double[] a,
int[] lda, @Const double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sormhr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer ilo, IntPointer ihi, @Const FloatPointer a,
IntPointer lda, @Const FloatPointer tau, FloatPointer c,
IntPointer ldc, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sormhr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer ilo, IntBuffer ihi, @Const FloatBuffer a,
IntBuffer lda, @Const FloatBuffer tau, FloatBuffer c,
IntBuffer ldc, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sormhr( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] ilo, int[] ihi, @Const float[] a,
int[] lda, @Const float[] tau, float[] c,
int[] ldc, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dormhr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer ilo, IntPointer ihi, @Const DoublePointer a,
IntPointer lda, @Const DoublePointer tau, DoublePointer c,
IntPointer ldc, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dormhr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer ilo, IntBuffer ihi, @Const DoubleBuffer a,
IntBuffer lda, @Const DoubleBuffer tau, DoubleBuffer c,
IntBuffer ldc, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dormhr( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] ilo, int[] ihi, @Const double[] a,
int[] lda, @Const double[] tau, double[] c,
int[] ldc, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cunghr( IntPointer n, IntPointer ilo, IntPointer ihi,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cunghr( IntBuffer n, IntBuffer ilo, IntBuffer ihi,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cunghr( int[] n, int[] ilo, int[] ihi,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zunghr( IntPointer n, IntPointer ilo, IntPointer ihi,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zunghr( IntBuffer n, IntBuffer ilo, IntBuffer ihi,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zunghr( int[] n, int[] ilo, int[] ihi,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cunmhr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer ilo, IntPointer ihi,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer c,
IntPointer ldc, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cunmhr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer ilo, IntBuffer ihi,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer c,
IntBuffer ldc, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cunmhr( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] ilo, int[] ihi,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] c,
int[] ldc, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zunmhr( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer ilo, IntPointer ihi,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer c,
IntPointer ldc, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zunmhr( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer ilo, IntBuffer ihi,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer c,
IntBuffer ldc, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zunmhr( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] ilo, int[] ihi,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] c,
int[] ldc, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sgebal( @Cast("char*") BytePointer job, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer ilo, IntPointer ihi, FloatPointer scale,
IntPointer info );
public static native void LAPACK_sgebal( @Cast("char*") ByteBuffer job, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer ilo, IntBuffer ihi, FloatBuffer scale,
IntBuffer info );
public static native void LAPACK_sgebal( @Cast("char*") byte[] job, int[] n, float[] a, int[] lda,
int[] ilo, int[] ihi, float[] scale,
int[] info );
public static native void LAPACK_dgebal( @Cast("char*") BytePointer job, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer ilo, IntPointer ihi, DoublePointer scale,
IntPointer info );
public static native void LAPACK_dgebal( @Cast("char*") ByteBuffer job, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer scale,
IntBuffer info );
public static native void LAPACK_dgebal( @Cast("char*") byte[] job, int[] n, double[] a, int[] lda,
int[] ilo, int[] ihi, double[] scale,
int[] info );
public static native void LAPACK_cgebal( @Cast("char*") BytePointer job, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer ilo, IntPointer ihi,
FloatPointer scale, IntPointer info );
public static native void LAPACK_cgebal( @Cast("char*") ByteBuffer job, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer ilo, IntBuffer ihi,
FloatBuffer scale, IntBuffer info );
public static native void LAPACK_cgebal( @Cast("char*") byte[] job, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] ilo, int[] ihi,
float[] scale, int[] info );
public static native void LAPACK_zgebal( @Cast("char*") BytePointer job, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ilo, IntPointer ihi,
DoublePointer scale, IntPointer info );
public static native void LAPACK_zgebal( @Cast("char*") ByteBuffer job, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ilo, IntBuffer ihi,
DoubleBuffer scale, IntBuffer info );
public static native void LAPACK_zgebal( @Cast("char*") byte[] job, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ilo, int[] ihi,
double[] scale, int[] info );
public static native void LAPACK_sgebak( @Cast("char*") BytePointer job, @Cast("char*") BytePointer side, IntPointer n, IntPointer ilo,
IntPointer ihi, @Const FloatPointer scale, IntPointer m,
FloatPointer v, IntPointer ldv, IntPointer info );
public static native void LAPACK_sgebak( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer side, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Const FloatBuffer scale, IntBuffer m,
FloatBuffer v, IntBuffer ldv, IntBuffer info );
public static native void LAPACK_sgebak( @Cast("char*") byte[] job, @Cast("char*") byte[] side, int[] n, int[] ilo,
int[] ihi, @Const float[] scale, int[] m,
float[] v, int[] ldv, int[] info );
public static native void LAPACK_dgebak( @Cast("char*") BytePointer job, @Cast("char*") BytePointer side, IntPointer n, IntPointer ilo,
IntPointer ihi, @Const DoublePointer scale, IntPointer m,
DoublePointer v, IntPointer ldv, IntPointer info );
public static native void LAPACK_dgebak( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer side, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Const DoubleBuffer scale, IntBuffer m,
DoubleBuffer v, IntBuffer ldv, IntBuffer info );
public static native void LAPACK_dgebak( @Cast("char*") byte[] job, @Cast("char*") byte[] side, int[] n, int[] ilo,
int[] ihi, @Const double[] scale, int[] m,
double[] v, int[] ldv, int[] info );
public static native void LAPACK_cgebak( @Cast("char*") BytePointer job, @Cast("char*") BytePointer side, IntPointer n, IntPointer ilo,
IntPointer ihi, @Const FloatPointer scale, IntPointer m,
@Cast("lapack_complex_float*") FloatPointer v, IntPointer ldv,
IntPointer info );
public static native void LAPACK_cgebak( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer side, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Const FloatBuffer scale, IntBuffer m,
@Cast("lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
IntBuffer info );
public static native void LAPACK_cgebak( @Cast("char*") byte[] job, @Cast("char*") byte[] side, int[] n, int[] ilo,
int[] ihi, @Const float[] scale, int[] m,
@Cast("lapack_complex_float*") float[] v, int[] ldv,
int[] info );
public static native void LAPACK_zgebak( @Cast("char*") BytePointer job, @Cast("char*") BytePointer side, IntPointer n, IntPointer ilo,
IntPointer ihi, @Const DoublePointer scale, IntPointer m,
@Cast("lapack_complex_double*") DoublePointer v, IntPointer ldv,
IntPointer info );
public static native void LAPACK_zgebak( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer side, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Const DoubleBuffer scale, IntBuffer m,
@Cast("lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
IntBuffer info );
public static native void LAPACK_zgebak( @Cast("char*") byte[] job, @Cast("char*") byte[] side, int[] n, int[] ilo,
int[] ihi, @Const double[] scale, int[] m,
@Cast("lapack_complex_double*") double[] v, int[] ldv,
int[] info );
public static native void LAPACK_shseqr( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, FloatPointer h, IntPointer ldh, FloatPointer wr,
FloatPointer wi, FloatPointer z, IntPointer ldz, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_shseqr( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, FloatBuffer h, IntBuffer ldh, FloatBuffer wr,
FloatBuffer wi, FloatBuffer z, IntBuffer ldz, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_shseqr( @Cast("char*") byte[] job, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, float[] h, int[] ldh, float[] wr,
float[] wi, float[] z, int[] ldz, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dhseqr( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, DoublePointer h, IntPointer ldh, DoublePointer wr,
DoublePointer wi, DoublePointer z, IntPointer ldz, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dhseqr( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, DoubleBuffer h, IntBuffer ldh, DoubleBuffer wr,
DoubleBuffer wi, DoubleBuffer z, IntBuffer ldz, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dhseqr( @Cast("char*") byte[] job, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, double[] h, int[] ldh, double[] wr,
double[] wi, double[] z, int[] ldz, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_chseqr( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, @Cast("lapack_complex_float*") FloatPointer h, IntPointer ldh,
@Cast("lapack_complex_float*") FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_chseqr( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Cast("lapack_complex_float*") FloatBuffer h, IntBuffer ldh,
@Cast("lapack_complex_float*") FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_chseqr( @Cast("char*") byte[] job, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, @Cast("lapack_complex_float*") float[] h, int[] ldh,
@Cast("lapack_complex_float*") float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zhseqr( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, @Cast("lapack_complex_double*") DoublePointer h, IntPointer ldh,
@Cast("lapack_complex_double*") DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
IntPointer ldz, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zhseqr( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Cast("lapack_complex_double*") DoubleBuffer h, IntBuffer ldh,
@Cast("lapack_complex_double*") DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
IntBuffer ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zhseqr( @Cast("char*") byte[] job, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, @Cast("lapack_complex_double*") double[] h, int[] ldh,
@Cast("lapack_complex_double*") double[] w, @Cast("lapack_complex_double*") double[] z,
int[] ldz, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_shsein( @Cast("char*") BytePointer job, @Cast("char*") BytePointer eigsrc, @Cast("char*") BytePointer initv,
IntPointer select, IntPointer n, @Const FloatPointer h,
IntPointer ldh, FloatPointer wr, @Const FloatPointer wi, FloatPointer vl,
IntPointer ldvl, FloatPointer vr, IntPointer ldvr,
IntPointer mm, IntPointer m, FloatPointer work,
IntPointer ifaill, IntPointer ifailr, IntPointer info );
public static native void LAPACK_shsein( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer eigsrc, @Cast("char*") ByteBuffer initv,
IntBuffer select, IntBuffer n, @Const FloatBuffer h,
IntBuffer ldh, FloatBuffer wr, @Const FloatBuffer wi, FloatBuffer vl,
IntBuffer ldvl, FloatBuffer vr, IntBuffer ldvr,
IntBuffer mm, IntBuffer m, FloatBuffer work,
IntBuffer ifaill, IntBuffer ifailr, IntBuffer info );
public static native void LAPACK_shsein( @Cast("char*") byte[] job, @Cast("char*") byte[] eigsrc, @Cast("char*") byte[] initv,
int[] select, int[] n, @Const float[] h,
int[] ldh, float[] wr, @Const float[] wi, float[] vl,
int[] ldvl, float[] vr, int[] ldvr,
int[] mm, int[] m, float[] work,
int[] ifaill, int[] ifailr, int[] info );
public static native void LAPACK_dhsein( @Cast("char*") BytePointer job, @Cast("char*") BytePointer eigsrc, @Cast("char*") BytePointer initv,
IntPointer select, IntPointer n, @Const DoublePointer h,
IntPointer ldh, DoublePointer wr, @Const DoublePointer wi, DoublePointer vl,
IntPointer ldvl, DoublePointer vr, IntPointer ldvr,
IntPointer mm, IntPointer m, DoublePointer work,
IntPointer ifaill, IntPointer ifailr, IntPointer info );
public static native void LAPACK_dhsein( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer eigsrc, @Cast("char*") ByteBuffer initv,
IntBuffer select, IntBuffer n, @Const DoubleBuffer h,
IntBuffer ldh, DoubleBuffer wr, @Const DoubleBuffer wi, DoubleBuffer vl,
IntBuffer ldvl, DoubleBuffer vr, IntBuffer ldvr,
IntBuffer mm, IntBuffer m, DoubleBuffer work,
IntBuffer ifaill, IntBuffer ifailr, IntBuffer info );
public static native void LAPACK_dhsein( @Cast("char*") byte[] job, @Cast("char*") byte[] eigsrc, @Cast("char*") byte[] initv,
int[] select, int[] n, @Const double[] h,
int[] ldh, double[] wr, @Const double[] wi, double[] vl,
int[] ldvl, double[] vr, int[] ldvr,
int[] mm, int[] m, double[] work,
int[] ifaill, int[] ifailr, int[] info );
public static native void LAPACK_chsein( @Cast("char*") BytePointer job, @Cast("char*") BytePointer eigsrc, @Cast("char*") BytePointer initv,
@Const IntPointer select, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer h, IntPointer ldh,
@Cast("lapack_complex_float*") FloatPointer w, @Cast("lapack_complex_float*") FloatPointer vl,
IntPointer ldvl, @Cast("lapack_complex_float*") FloatPointer vr,
IntPointer ldvr, IntPointer mm, IntPointer m,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer ifaill, IntPointer ifailr, IntPointer info );
public static native void LAPACK_chsein( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer eigsrc, @Cast("char*") ByteBuffer initv,
@Const IntBuffer select, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer h, IntBuffer ldh,
@Cast("lapack_complex_float*") FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer vl,
IntBuffer ldvl, @Cast("lapack_complex_float*") FloatBuffer vr,
IntBuffer ldvr, IntBuffer mm, IntBuffer m,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer ifaill, IntBuffer ifailr, IntBuffer info );
public static native void LAPACK_chsein( @Cast("char*") byte[] job, @Cast("char*") byte[] eigsrc, @Cast("char*") byte[] initv,
@Const int[] select, int[] n,
@Cast("const lapack_complex_float*") float[] h, int[] ldh,
@Cast("lapack_complex_float*") float[] w, @Cast("lapack_complex_float*") float[] vl,
int[] ldvl, @Cast("lapack_complex_float*") float[] vr,
int[] ldvr, int[] mm, int[] m,
@Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] ifaill, int[] ifailr, int[] info );
public static native void LAPACK_zhsein( @Cast("char*") BytePointer job, @Cast("char*") BytePointer eigsrc, @Cast("char*") BytePointer initv,
@Const IntPointer select, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer h, IntPointer ldh,
@Cast("lapack_complex_double*") DoublePointer w, @Cast("lapack_complex_double*") DoublePointer vl,
IntPointer ldvl, @Cast("lapack_complex_double*") DoublePointer vr,
IntPointer ldvr, IntPointer mm, IntPointer m,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer ifaill, IntPointer ifailr, IntPointer info );
public static native void LAPACK_zhsein( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer eigsrc, @Cast("char*") ByteBuffer initv,
@Const IntBuffer select, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer h, IntBuffer ldh,
@Cast("lapack_complex_double*") DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer vl,
IntBuffer ldvl, @Cast("lapack_complex_double*") DoubleBuffer vr,
IntBuffer ldvr, IntBuffer mm, IntBuffer m,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer ifaill, IntBuffer ifailr, IntBuffer info );
public static native void LAPACK_zhsein( @Cast("char*") byte[] job, @Cast("char*") byte[] eigsrc, @Cast("char*") byte[] initv,
@Const int[] select, int[] n,
@Cast("const lapack_complex_double*") double[] h, int[] ldh,
@Cast("lapack_complex_double*") double[] w, @Cast("lapack_complex_double*") double[] vl,
int[] ldvl, @Cast("lapack_complex_double*") double[] vr,
int[] ldvr, int[] mm, int[] m,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] ifaill, int[] ifailr, int[] info );
public static native void LAPACK_strevc( @Cast("char*") BytePointer side, @Cast("char*") BytePointer howmny, IntPointer select,
IntPointer n, @Const FloatPointer t, IntPointer ldt, FloatPointer vl,
IntPointer ldvl, FloatPointer vr, IntPointer ldvr,
IntPointer mm, IntPointer m, FloatPointer work,
IntPointer info );
public static native void LAPACK_strevc( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer howmny, IntBuffer select,
IntBuffer n, @Const FloatBuffer t, IntBuffer ldt, FloatBuffer vl,
IntBuffer ldvl, FloatBuffer vr, IntBuffer ldvr,
IntBuffer mm, IntBuffer m, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_strevc( @Cast("char*") byte[] side, @Cast("char*") byte[] howmny, int[] select,
int[] n, @Const float[] t, int[] ldt, float[] vl,
int[] ldvl, float[] vr, int[] ldvr,
int[] mm, int[] m, float[] work,
int[] info );
public static native void LAPACK_dtrevc( @Cast("char*") BytePointer side, @Cast("char*") BytePointer howmny, IntPointer select,
IntPointer n, @Const DoublePointer t, IntPointer ldt, DoublePointer vl,
IntPointer ldvl, DoublePointer vr, IntPointer ldvr,
IntPointer mm, IntPointer m, DoublePointer work,
IntPointer info );
public static native void LAPACK_dtrevc( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer howmny, IntBuffer select,
IntBuffer n, @Const DoubleBuffer t, IntBuffer ldt, DoubleBuffer vl,
IntBuffer ldvl, DoubleBuffer vr, IntBuffer ldvr,
IntBuffer mm, IntBuffer m, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dtrevc( @Cast("char*") byte[] side, @Cast("char*") byte[] howmny, int[] select,
int[] n, @Const double[] t, int[] ldt, double[] vl,
int[] ldvl, double[] vr, int[] ldvr,
int[] mm, int[] m, double[] work,
int[] info );
public static native void LAPACK_ctrevc( @Cast("char*") BytePointer side, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer t, IntPointer ldt,
@Cast("lapack_complex_float*") FloatPointer vl, IntPointer ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, IntPointer ldvr, IntPointer mm,
IntPointer m, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_ctrevc( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
@Cast("lapack_complex_float*") FloatBuffer vl, IntBuffer ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, IntBuffer ldvr, IntBuffer mm,
IntBuffer m, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_ctrevc( @Cast("char*") byte[] side, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Cast("lapack_complex_float*") float[] t, int[] ldt,
@Cast("lapack_complex_float*") float[] vl, int[] ldvl,
@Cast("lapack_complex_float*") float[] vr, int[] ldvr, int[] mm,
int[] m, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_ztrevc( @Cast("char*") BytePointer side, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer t, IntPointer ldt,
@Cast("lapack_complex_double*") DoublePointer vl, IntPointer ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, IntPointer ldvr, IntPointer mm,
IntPointer m, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_ztrevc( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
@Cast("lapack_complex_double*") DoubleBuffer vl, IntBuffer ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, IntBuffer ldvr, IntBuffer mm,
IntBuffer m, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_ztrevc( @Cast("char*") byte[] side, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Cast("lapack_complex_double*") double[] t, int[] ldt,
@Cast("lapack_complex_double*") double[] vl, int[] ldvl,
@Cast("lapack_complex_double*") double[] vr, int[] ldvr, int[] mm,
int[] m, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_strsna( @Cast("char*") BytePointer job, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Const FloatPointer t, IntPointer ldt,
@Const FloatPointer vl, IntPointer ldvl, @Const FloatPointer vr,
IntPointer ldvr, FloatPointer s, FloatPointer sep, IntPointer mm,
IntPointer m, FloatPointer work, IntPointer ldwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_strsna( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Const FloatBuffer t, IntBuffer ldt,
@Const FloatBuffer vl, IntBuffer ldvl, @Const FloatBuffer vr,
IntBuffer ldvr, FloatBuffer s, FloatBuffer sep, IntBuffer mm,
IntBuffer m, FloatBuffer work, IntBuffer ldwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_strsna( @Cast("char*") byte[] job, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Const float[] t, int[] ldt,
@Const float[] vl, int[] ldvl, @Const float[] vr,
int[] ldvr, float[] s, float[] sep, int[] mm,
int[] m, float[] work, int[] ldwork,
int[] iwork, int[] info );
public static native void LAPACK_dtrsna( @Cast("char*") BytePointer job, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Const DoublePointer t, IntPointer ldt,
@Const DoublePointer vl, IntPointer ldvl, @Const DoublePointer vr,
IntPointer ldvr, DoublePointer s, DoublePointer sep, IntPointer mm,
IntPointer m, DoublePointer work, IntPointer ldwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dtrsna( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Const DoubleBuffer t, IntBuffer ldt,
@Const DoubleBuffer vl, IntBuffer ldvl, @Const DoubleBuffer vr,
IntBuffer ldvr, DoubleBuffer s, DoubleBuffer sep, IntBuffer mm,
IntBuffer m, DoubleBuffer work, IntBuffer ldwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dtrsna( @Cast("char*") byte[] job, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Const double[] t, int[] ldt,
@Const double[] vl, int[] ldvl, @Const double[] vr,
int[] ldvr, double[] s, double[] sep, int[] mm,
int[] m, double[] work, int[] ldwork,
int[] iwork, int[] info );
public static native void LAPACK_ctrsna( @Cast("char*") BytePointer job, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Cast("const lapack_complex_float*") FloatPointer t,
IntPointer ldt, @Cast("const lapack_complex_float*") FloatPointer vl,
IntPointer ldvl, @Cast("const lapack_complex_float*") FloatPointer vr,
IntPointer ldvr, FloatPointer s, FloatPointer sep, IntPointer mm,
IntPointer m, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer ldwork, FloatPointer rwork, IntPointer info );
public static native void LAPACK_ctrsna( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer t,
IntBuffer ldt, @Cast("const lapack_complex_float*") FloatBuffer vl,
IntBuffer ldvl, @Cast("const lapack_complex_float*") FloatBuffer vr,
IntBuffer ldvr, FloatBuffer s, FloatBuffer sep, IntBuffer mm,
IntBuffer m, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer ldwork, FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_ctrsna( @Cast("char*") byte[] job, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Cast("const lapack_complex_float*") float[] t,
int[] ldt, @Cast("const lapack_complex_float*") float[] vl,
int[] ldvl, @Cast("const lapack_complex_float*") float[] vr,
int[] ldvr, float[] s, float[] sep, int[] mm,
int[] m, @Cast("lapack_complex_float*") float[] work,
int[] ldwork, float[] rwork, int[] info );
public static native void LAPACK_ztrsna( @Cast("char*") BytePointer job, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Cast("const lapack_complex_double*") DoublePointer t,
IntPointer ldt, @Cast("const lapack_complex_double*") DoublePointer vl,
IntPointer ldvl, @Cast("const lapack_complex_double*") DoublePointer vr,
IntPointer ldvr, DoublePointer s, DoublePointer sep, IntPointer mm,
IntPointer m, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer ldwork, DoublePointer rwork, IntPointer info );
public static native void LAPACK_ztrsna( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer t,
IntBuffer ldt, @Cast("const lapack_complex_double*") DoubleBuffer vl,
IntBuffer ldvl, @Cast("const lapack_complex_double*") DoubleBuffer vr,
IntBuffer ldvr, DoubleBuffer s, DoubleBuffer sep, IntBuffer mm,
IntBuffer m, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer ldwork, DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_ztrsna( @Cast("char*") byte[] job, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Cast("const lapack_complex_double*") double[] t,
int[] ldt, @Cast("const lapack_complex_double*") double[] vl,
int[] ldvl, @Cast("const lapack_complex_double*") double[] vr,
int[] ldvr, double[] s, double[] sep, int[] mm,
int[] m, @Cast("lapack_complex_double*") double[] work,
int[] ldwork, double[] rwork, int[] info );
public static native void LAPACK_strexc( @Cast("char*") BytePointer compq, IntPointer n, FloatPointer t, IntPointer ldt,
FloatPointer q, IntPointer ldq, IntPointer ifst,
IntPointer ilst, FloatPointer work, IntPointer info );
public static native void LAPACK_strexc( @Cast("char*") ByteBuffer compq, IntBuffer n, FloatBuffer t, IntBuffer ldt,
FloatBuffer q, IntBuffer ldq, IntBuffer ifst,
IntBuffer ilst, FloatBuffer work, IntBuffer info );
public static native void LAPACK_strexc( @Cast("char*") byte[] compq, int[] n, float[] t, int[] ldt,
float[] q, int[] ldq, int[] ifst,
int[] ilst, float[] work, int[] info );
public static native void LAPACK_dtrexc( @Cast("char*") BytePointer compq, IntPointer n, DoublePointer t, IntPointer ldt,
DoublePointer q, IntPointer ldq, IntPointer ifst,
IntPointer ilst, DoublePointer work, IntPointer info );
public static native void LAPACK_dtrexc( @Cast("char*") ByteBuffer compq, IntBuffer n, DoubleBuffer t, IntBuffer ldt,
DoubleBuffer q, IntBuffer ldq, IntBuffer ifst,
IntBuffer ilst, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dtrexc( @Cast("char*") byte[] compq, int[] n, double[] t, int[] ldt,
double[] q, int[] ldq, int[] ifst,
int[] ilst, double[] work, int[] info );
public static native void LAPACK_ctrexc( @Cast("char*") BytePointer compq, IntPointer n, @Cast("lapack_complex_float*") FloatPointer t,
IntPointer ldt, @Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
IntPointer ifst, IntPointer ilst, IntPointer info );
public static native void LAPACK_ctrexc( @Cast("char*") ByteBuffer compq, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer t,
IntBuffer ldt, @Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
IntBuffer ifst, IntBuffer ilst, IntBuffer info );
public static native void LAPACK_ctrexc( @Cast("char*") byte[] compq, int[] n, @Cast("lapack_complex_float*") float[] t,
int[] ldt, @Cast("lapack_complex_float*") float[] q, int[] ldq,
int[] ifst, int[] ilst, int[] info );
public static native void LAPACK_ztrexc( @Cast("char*") BytePointer compq, IntPointer n, @Cast("lapack_complex_double*") DoublePointer t,
IntPointer ldt, @Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
IntPointer ifst, IntPointer ilst, IntPointer info );
public static native void LAPACK_ztrexc( @Cast("char*") ByteBuffer compq, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer t,
IntBuffer ldt, @Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
IntBuffer ifst, IntBuffer ilst, IntBuffer info );
public static native void LAPACK_ztrexc( @Cast("char*") byte[] compq, int[] n, @Cast("lapack_complex_double*") double[] t,
int[] ldt, @Cast("lapack_complex_double*") double[] q, int[] ldq,
int[] ifst, int[] ilst, int[] info );
public static native void LAPACK_strsen( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compq, @Const IntPointer select,
IntPointer n, FloatPointer t, IntPointer ldt, FloatPointer q,
IntPointer ldq, FloatPointer wr, FloatPointer wi, IntPointer m,
FloatPointer s, FloatPointer sep, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_strsen( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compq, @Const IntBuffer select,
IntBuffer n, FloatBuffer t, IntBuffer ldt, FloatBuffer q,
IntBuffer ldq, FloatBuffer wr, FloatBuffer wi, IntBuffer m,
FloatBuffer s, FloatBuffer sep, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_strsen( @Cast("char*") byte[] job, @Cast("char*") byte[] compq, @Const int[] select,
int[] n, float[] t, int[] ldt, float[] q,
int[] ldq, float[] wr, float[] wi, int[] m,
float[] s, float[] sep, float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_dtrsen( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compq, @Const IntPointer select,
IntPointer n, DoublePointer t, IntPointer ldt, DoublePointer q,
IntPointer ldq, DoublePointer wr, DoublePointer wi, IntPointer m,
DoublePointer s, DoublePointer sep, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dtrsen( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compq, @Const IntBuffer select,
IntBuffer n, DoubleBuffer t, IntBuffer ldt, DoubleBuffer q,
IntBuffer ldq, DoubleBuffer wr, DoubleBuffer wi, IntBuffer m,
DoubleBuffer s, DoubleBuffer sep, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dtrsen( @Cast("char*") byte[] job, @Cast("char*") byte[] compq, @Const int[] select,
int[] n, double[] t, int[] ldt, double[] q,
int[] ldq, double[] wr, double[] wi, int[] m,
double[] s, double[] sep, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_ctrsen( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compq, @Const IntPointer select,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer t, IntPointer ldt,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer w, IntPointer m, FloatPointer s,
FloatPointer sep, @Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_ctrsen( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compq, @Const IntBuffer select,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer w, IntBuffer m, FloatBuffer s,
FloatBuffer sep, @Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_ctrsen( @Cast("char*") byte[] job, @Cast("char*") byte[] compq, @Const int[] select,
int[] n, @Cast("lapack_complex_float*") float[] t, int[] ldt,
@Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] w, int[] m, float[] s,
float[] sep, @Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_ztrsen( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compq, @Const IntPointer select,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer t, IntPointer ldt,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer w, IntPointer m, DoublePointer s,
DoublePointer sep, @Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_ztrsen( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compq, @Const IntBuffer select,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer w, IntBuffer m, DoubleBuffer s,
DoubleBuffer sep, @Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_ztrsen( @Cast("char*") byte[] job, @Cast("char*") byte[] compq, @Const int[] select,
int[] n, @Cast("lapack_complex_double*") double[] t, int[] ldt,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] w, int[] m, double[] s,
double[] sep, @Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_strsyl( @Cast("char*") BytePointer trana, @Cast("char*") BytePointer tranb, IntPointer isgn, IntPointer m,
IntPointer n, @Const FloatPointer a, IntPointer lda,
@Const FloatPointer b, IntPointer ldb, FloatPointer c, IntPointer ldc,
FloatPointer scale, IntPointer info );
public static native void LAPACK_strsyl( @Cast("char*") ByteBuffer trana, @Cast("char*") ByteBuffer tranb, IntBuffer isgn, IntBuffer m,
IntBuffer n, @Const FloatBuffer a, IntBuffer lda,
@Const FloatBuffer b, IntBuffer ldb, FloatBuffer c, IntBuffer ldc,
FloatBuffer scale, IntBuffer info );
public static native void LAPACK_strsyl( @Cast("char*") byte[] trana, @Cast("char*") byte[] tranb, int[] isgn, int[] m,
int[] n, @Const float[] a, int[] lda,
@Const float[] b, int[] ldb, float[] c, int[] ldc,
float[] scale, int[] info );
public static native void LAPACK_dtrsyl( @Cast("char*") BytePointer trana, @Cast("char*") BytePointer tranb, IntPointer isgn, IntPointer m,
IntPointer n, @Const DoublePointer a, IntPointer lda,
@Const DoublePointer b, IntPointer ldb, DoublePointer c,
IntPointer ldc, DoublePointer scale, IntPointer info );
public static native void LAPACK_dtrsyl( @Cast("char*") ByteBuffer trana, @Cast("char*") ByteBuffer tranb, IntBuffer isgn, IntBuffer m,
IntBuffer n, @Const DoubleBuffer a, IntBuffer lda,
@Const DoubleBuffer b, IntBuffer ldb, DoubleBuffer c,
IntBuffer ldc, DoubleBuffer scale, IntBuffer info );
public static native void LAPACK_dtrsyl( @Cast("char*") byte[] trana, @Cast("char*") byte[] tranb, int[] isgn, int[] m,
int[] n, @Const double[] a, int[] lda,
@Const double[] b, int[] ldb, double[] c,
int[] ldc, double[] scale, int[] info );
public static native void LAPACK_ctrsyl( @Cast("char*") BytePointer trana, @Cast("char*") BytePointer tranb, IntPointer isgn, IntPointer m,
IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
FloatPointer scale, IntPointer info );
public static native void LAPACK_ctrsyl( @Cast("char*") ByteBuffer trana, @Cast("char*") ByteBuffer tranb, IntBuffer isgn, IntBuffer m,
IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
FloatBuffer scale, IntBuffer info );
public static native void LAPACK_ctrsyl( @Cast("char*") byte[] trana, @Cast("char*") byte[] tranb, int[] isgn, int[] m,
int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] c, int[] ldc,
float[] scale, int[] info );
public static native void LAPACK_ztrsyl( @Cast("char*") BytePointer trana, @Cast("char*") BytePointer tranb, IntPointer isgn, IntPointer m,
IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
DoublePointer scale, IntPointer info );
public static native void LAPACK_ztrsyl( @Cast("char*") ByteBuffer trana, @Cast("char*") ByteBuffer tranb, IntBuffer isgn, IntBuffer m,
IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
DoubleBuffer scale, IntBuffer info );
public static native void LAPACK_ztrsyl( @Cast("char*") byte[] trana, @Cast("char*") byte[] tranb, int[] isgn, int[] m,
int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] c, int[] ldc,
double[] scale, int[] info );
public static native void LAPACK_sgghrd( @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, FloatPointer a, IntPointer lda, FloatPointer b,
IntPointer ldb, FloatPointer q, IntPointer ldq, FloatPointer z,
IntPointer ldz, IntPointer info );
public static native void LAPACK_sgghrd( @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, FloatBuffer a, IntBuffer lda, FloatBuffer b,
IntBuffer ldb, FloatBuffer q, IntBuffer ldq, FloatBuffer z,
IntBuffer ldz, IntBuffer info );
public static native void LAPACK_sgghrd( @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, float[] a, int[] lda, float[] b,
int[] ldb, float[] q, int[] ldq, float[] z,
int[] ldz, int[] info );
public static native void LAPACK_dgghrd( @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, DoublePointer a, IntPointer lda, DoublePointer b,
IntPointer ldb, DoublePointer q, IntPointer ldq, DoublePointer z,
IntPointer ldz, IntPointer info );
public static native void LAPACK_dgghrd( @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, DoubleBuffer a, IntBuffer lda, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer q, IntBuffer ldq, DoubleBuffer z,
IntBuffer ldz, IntBuffer info );
public static native void LAPACK_dgghrd( @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, double[] a, int[] lda, double[] b,
int[] ldb, double[] q, int[] ldq, double[] z,
int[] ldz, int[] info );
public static native void LAPACK_cgghrd( @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, @Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
IntPointer info );
public static native void LAPACK_cgghrd( @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
IntBuffer info );
public static native void LAPACK_cgghrd( @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, @Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
int[] info );
public static native void LAPACK_zgghrd( @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, @Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
IntPointer info );
public static native void LAPACK_zgghrd( @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
IntBuffer info );
public static native void LAPACK_zgghrd( @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, @Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
int[] info );
public static native void LAPACK_sgghd3( @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, FloatPointer a, IntPointer lda, FloatPointer b,
IntPointer ldb, FloatPointer q, IntPointer ldq, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sgghd3( @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, FloatBuffer a, IntBuffer lda, FloatBuffer b,
IntBuffer ldb, FloatBuffer q, IntBuffer ldq, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sgghd3( @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, float[] a, int[] lda, float[] b,
int[] ldb, float[] q, int[] ldq, float[] z,
int[] ldz, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dgghd3( @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n, IntPointer ilo,
IntPointer ihi, DoublePointer a, IntPointer lda, DoublePointer b,
IntPointer ldb, DoublePointer q, IntPointer ldq, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dgghd3( @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, DoubleBuffer a, IntBuffer lda, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer q, IntBuffer ldq, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dgghd3( @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n, int[] ilo,
int[] ihi, double[] a, int[] lda, double[] b,
int[] ldb, double[] q, int[] ldq, double[] z,
int[] ldz, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cgghd3( @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n,
IntPointer ilo, IntPointer ihi,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cgghd3( @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n,
IntBuffer ilo, IntBuffer ihi,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cgghd3( @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n,
int[] ilo, int[] ihi,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zgghd3( @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n,
IntPointer ilo, IntPointer ihi,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zgghd3( @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n,
IntBuffer ilo, IntBuffer ihi,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zgghd3( @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n,
int[] ilo, int[] ihi,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sggbal( @Cast("char*") BytePointer job, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer b, IntPointer ldb, IntPointer ilo, IntPointer ihi,
FloatPointer lscale, FloatPointer rscale, FloatPointer work,
IntPointer info );
public static native void LAPACK_sggbal( @Cast("char*") ByteBuffer job, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer b, IntBuffer ldb, IntBuffer ilo, IntBuffer ihi,
FloatBuffer lscale, FloatBuffer rscale, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_sggbal( @Cast("char*") byte[] job, int[] n, float[] a, int[] lda,
float[] b, int[] ldb, int[] ilo, int[] ihi,
float[] lscale, float[] rscale, float[] work,
int[] info );
public static native void LAPACK_dggbal( @Cast("char*") BytePointer job, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer b, IntPointer ldb, IntPointer ilo,
IntPointer ihi, DoublePointer lscale, DoublePointer rscale,
DoublePointer work, IntPointer info );
public static native void LAPACK_dggbal( @Cast("char*") ByteBuffer job, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer b, IntBuffer ldb, IntBuffer ilo,
IntBuffer ihi, DoubleBuffer lscale, DoubleBuffer rscale,
DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dggbal( @Cast("char*") byte[] job, int[] n, double[] a, int[] lda,
double[] b, int[] ldb, int[] ilo,
int[] ihi, double[] lscale, double[] rscale,
double[] work, int[] info );
public static native void LAPACK_cggbal( @Cast("char*") BytePointer job, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer ilo, IntPointer ihi, FloatPointer lscale,
FloatPointer rscale, FloatPointer work, IntPointer info );
public static native void LAPACK_cggbal( @Cast("char*") ByteBuffer job, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer ilo, IntBuffer ihi, FloatBuffer lscale,
FloatBuffer rscale, FloatBuffer work, IntBuffer info );
public static native void LAPACK_cggbal( @Cast("char*") byte[] job, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] ilo, int[] ihi, float[] lscale,
float[] rscale, float[] work, int[] info );
public static native void LAPACK_zggbal( @Cast("char*") BytePointer job, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
IntPointer ilo, IntPointer ihi, DoublePointer lscale,
DoublePointer rscale, DoublePointer work, IntPointer info );
public static native void LAPACK_zggbal( @Cast("char*") ByteBuffer job, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer lscale,
DoubleBuffer rscale, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zggbal( @Cast("char*") byte[] job, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] b, int[] ldb,
int[] ilo, int[] ihi, double[] lscale,
double[] rscale, double[] work, int[] info );
public static native void LAPACK_sggbak( @Cast("char*") BytePointer job, @Cast("char*") BytePointer side, IntPointer n, IntPointer ilo,
IntPointer ihi, @Const FloatPointer lscale, @Const FloatPointer rscale,
IntPointer m, FloatPointer v, IntPointer ldv,
IntPointer info );
public static native void LAPACK_sggbak( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer side, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Const FloatBuffer lscale, @Const FloatBuffer rscale,
IntBuffer m, FloatBuffer v, IntBuffer ldv,
IntBuffer info );
public static native void LAPACK_sggbak( @Cast("char*") byte[] job, @Cast("char*") byte[] side, int[] n, int[] ilo,
int[] ihi, @Const float[] lscale, @Const float[] rscale,
int[] m, float[] v, int[] ldv,
int[] info );
public static native void LAPACK_dggbak( @Cast("char*") BytePointer job, @Cast("char*") BytePointer side, IntPointer n, IntPointer ilo,
IntPointer ihi, @Const DoublePointer lscale, @Const DoublePointer rscale,
IntPointer m, DoublePointer v, IntPointer ldv,
IntPointer info );
public static native void LAPACK_dggbak( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer side, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Const DoubleBuffer lscale, @Const DoubleBuffer rscale,
IntBuffer m, DoubleBuffer v, IntBuffer ldv,
IntBuffer info );
public static native void LAPACK_dggbak( @Cast("char*") byte[] job, @Cast("char*") byte[] side, int[] n, int[] ilo,
int[] ihi, @Const double[] lscale, @Const double[] rscale,
int[] m, double[] v, int[] ldv,
int[] info );
public static native void LAPACK_cggbak( @Cast("char*") BytePointer job, @Cast("char*") BytePointer side, IntPointer n, IntPointer ilo,
IntPointer ihi, @Const FloatPointer lscale, @Const FloatPointer rscale,
IntPointer m, @Cast("lapack_complex_float*") FloatPointer v, IntPointer ldv,
IntPointer info );
public static native void LAPACK_cggbak( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer side, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Const FloatBuffer lscale, @Const FloatBuffer rscale,
IntBuffer m, @Cast("lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
IntBuffer info );
public static native void LAPACK_cggbak( @Cast("char*") byte[] job, @Cast("char*") byte[] side, int[] n, int[] ilo,
int[] ihi, @Const float[] lscale, @Const float[] rscale,
int[] m, @Cast("lapack_complex_float*") float[] v, int[] ldv,
int[] info );
public static native void LAPACK_zggbak( @Cast("char*") BytePointer job, @Cast("char*") BytePointer side, IntPointer n, IntPointer ilo,
IntPointer ihi, @Const DoublePointer lscale, @Const DoublePointer rscale,
IntPointer m, @Cast("lapack_complex_double*") DoublePointer v, IntPointer ldv,
IntPointer info );
public static native void LAPACK_zggbak( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer side, IntBuffer n, IntBuffer ilo,
IntBuffer ihi, @Const DoubleBuffer lscale, @Const DoubleBuffer rscale,
IntBuffer m, @Cast("lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
IntBuffer info );
public static native void LAPACK_zggbak( @Cast("char*") byte[] job, @Cast("char*") byte[] side, int[] n, int[] ilo,
int[] ihi, @Const double[] lscale, @Const double[] rscale,
int[] m, @Cast("lapack_complex_double*") double[] v, int[] ldv,
int[] info );
public static native void LAPACK_shgeqz( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n,
IntPointer ilo, IntPointer ihi, FloatPointer h, IntPointer ldh,
FloatPointer t, IntPointer ldt, FloatPointer alphar, FloatPointer alphai,
FloatPointer beta, FloatPointer q, IntPointer ldq, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_shgeqz( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n,
IntBuffer ilo, IntBuffer ihi, FloatBuffer h, IntBuffer ldh,
FloatBuffer t, IntBuffer ldt, FloatBuffer alphar, FloatBuffer alphai,
FloatBuffer beta, FloatBuffer q, IntBuffer ldq, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_shgeqz( @Cast("char*") byte[] job, @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n,
int[] ilo, int[] ihi, float[] h, int[] ldh,
float[] t, int[] ldt, float[] alphar, float[] alphai,
float[] beta, float[] q, int[] ldq, float[] z,
int[] ldz, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dhgeqz( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n,
IntPointer ilo, IntPointer ihi, DoublePointer h,
IntPointer ldh, DoublePointer t, IntPointer ldt, DoublePointer alphar,
DoublePointer alphai, DoublePointer beta, DoublePointer q, IntPointer ldq,
DoublePointer z, IntPointer ldz, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dhgeqz( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer h,
IntBuffer ldh, DoubleBuffer t, IntBuffer ldt, DoubleBuffer alphar,
DoubleBuffer alphai, DoubleBuffer beta, DoubleBuffer q, IntBuffer ldq,
DoubleBuffer z, IntBuffer ldz, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dhgeqz( @Cast("char*") byte[] job, @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n,
int[] ilo, int[] ihi, double[] h,
int[] ldh, double[] t, int[] ldt, double[] alphar,
double[] alphai, double[] beta, double[] q, int[] ldq,
double[] z, int[] ldz, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_chgeqz( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n,
IntPointer ilo, IntPointer ihi, @Cast("lapack_complex_float*") FloatPointer h,
IntPointer ldh, @Cast("lapack_complex_float*") FloatPointer t, IntPointer ldt,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_chgeqz( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n,
IntBuffer ilo, IntBuffer ihi, @Cast("lapack_complex_float*") FloatBuffer h,
IntBuffer ldh, @Cast("lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_chgeqz( @Cast("char*") byte[] job, @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n,
int[] ilo, int[] ihi, @Cast("lapack_complex_float*") float[] h,
int[] ldh, @Cast("lapack_complex_float*") float[] t, int[] ldt,
@Cast("lapack_complex_float*") float[] alpha, @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zhgeqz( @Cast("char*") BytePointer job, @Cast("char*") BytePointer compq, @Cast("char*") BytePointer compz, IntPointer n,
IntPointer ilo, IntPointer ihi, @Cast("lapack_complex_double*") DoublePointer h,
IntPointer ldh, @Cast("lapack_complex_double*") DoublePointer t, IntPointer ldt,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zhgeqz( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer compq, @Cast("char*") ByteBuffer compz, IntBuffer n,
IntBuffer ilo, IntBuffer ihi, @Cast("lapack_complex_double*") DoubleBuffer h,
IntBuffer ldh, @Cast("lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zhgeqz( @Cast("char*") byte[] job, @Cast("char*") byte[] compq, @Cast("char*") byte[] compz, int[] n,
int[] ilo, int[] ihi, @Cast("lapack_complex_double*") double[] h,
int[] ldh, @Cast("lapack_complex_double*") double[] t, int[] ldt,
@Cast("lapack_complex_double*") double[] alpha, @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_stgevc( @Cast("char*") BytePointer side, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Const FloatPointer s, IntPointer lds,
@Const FloatPointer p, IntPointer ldp, FloatPointer vl,
IntPointer ldvl, FloatPointer vr, IntPointer ldvr,
IntPointer mm, IntPointer m, FloatPointer work,
IntPointer info );
public static native void LAPACK_stgevc( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Const FloatBuffer s, IntBuffer lds,
@Const FloatBuffer p, IntBuffer ldp, FloatBuffer vl,
IntBuffer ldvl, FloatBuffer vr, IntBuffer ldvr,
IntBuffer mm, IntBuffer m, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_stgevc( @Cast("char*") byte[] side, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Const float[] s, int[] lds,
@Const float[] p, int[] ldp, float[] vl,
int[] ldvl, float[] vr, int[] ldvr,
int[] mm, int[] m, float[] work,
int[] info );
public static native void LAPACK_dtgevc( @Cast("char*") BytePointer side, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Const DoublePointer s, IntPointer lds,
@Const DoublePointer p, IntPointer ldp, DoublePointer vl,
IntPointer ldvl, DoublePointer vr, IntPointer ldvr,
IntPointer mm, IntPointer m, DoublePointer work,
IntPointer info );
public static native void LAPACK_dtgevc( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Const DoubleBuffer s, IntBuffer lds,
@Const DoubleBuffer p, IntBuffer ldp, DoubleBuffer vl,
IntBuffer ldvl, DoubleBuffer vr, IntBuffer ldvr,
IntBuffer mm, IntBuffer m, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dtgevc( @Cast("char*") byte[] side, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Const double[] s, int[] lds,
@Const double[] p, int[] ldp, double[] vl,
int[] ldvl, double[] vr, int[] ldvr,
int[] mm, int[] m, double[] work,
int[] info );
public static native void LAPACK_ctgevc( @Cast("char*") BytePointer side, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Cast("const lapack_complex_float*") FloatPointer s,
IntPointer lds, @Cast("const lapack_complex_float*") FloatPointer p,
IntPointer ldp, @Cast("lapack_complex_float*") FloatPointer vl, IntPointer ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, IntPointer ldvr, IntPointer mm,
IntPointer m, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_ctgevc( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer s,
IntBuffer lds, @Cast("const lapack_complex_float*") FloatBuffer p,
IntBuffer ldp, @Cast("lapack_complex_float*") FloatBuffer vl, IntBuffer ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, IntBuffer ldvr, IntBuffer mm,
IntBuffer m, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_ctgevc( @Cast("char*") byte[] side, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Cast("const lapack_complex_float*") float[] s,
int[] lds, @Cast("const lapack_complex_float*") float[] p,
int[] ldp, @Cast("lapack_complex_float*") float[] vl, int[] ldvl,
@Cast("lapack_complex_float*") float[] vr, int[] ldvr, int[] mm,
int[] m, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_ztgevc( @Cast("char*") BytePointer side, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Cast("const lapack_complex_double*") DoublePointer s,
IntPointer lds, @Cast("const lapack_complex_double*") DoublePointer p,
IntPointer ldp, @Cast("lapack_complex_double*") DoublePointer vl,
IntPointer ldvl, @Cast("lapack_complex_double*") DoublePointer vr,
IntPointer ldvr, IntPointer mm, IntPointer m,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_ztgevc( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer s,
IntBuffer lds, @Cast("const lapack_complex_double*") DoubleBuffer p,
IntBuffer ldp, @Cast("lapack_complex_double*") DoubleBuffer vl,
IntBuffer ldvl, @Cast("lapack_complex_double*") DoubleBuffer vr,
IntBuffer ldvr, IntBuffer mm, IntBuffer m,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_ztgevc( @Cast("char*") byte[] side, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Cast("const lapack_complex_double*") double[] s,
int[] lds, @Cast("const lapack_complex_double*") double[] p,
int[] ldp, @Cast("lapack_complex_double*") double[] vl,
int[] ldvl, @Cast("lapack_complex_double*") double[] vr,
int[] ldvr, int[] mm, int[] m,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_stgexc( IntPointer wantq, IntPointer wantz, IntPointer n,
FloatPointer a, IntPointer lda, FloatPointer b, IntPointer ldb,
FloatPointer q, IntPointer ldq, FloatPointer z, IntPointer ldz,
IntPointer ifst, IntPointer ilst, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_stgexc( IntBuffer wantq, IntBuffer wantz, IntBuffer n,
FloatBuffer a, IntBuffer lda, FloatBuffer b, IntBuffer ldb,
FloatBuffer q, IntBuffer ldq, FloatBuffer z, IntBuffer ldz,
IntBuffer ifst, IntBuffer ilst, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_stgexc( int[] wantq, int[] wantz, int[] n,
float[] a, int[] lda, float[] b, int[] ldb,
float[] q, int[] ldq, float[] z, int[] ldz,
int[] ifst, int[] ilst, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dtgexc( IntPointer wantq, IntPointer wantz, IntPointer n,
DoublePointer a, IntPointer lda, DoublePointer b, IntPointer ldb,
DoublePointer q, IntPointer ldq, DoublePointer z, IntPointer ldz,
IntPointer ifst, IntPointer ilst, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dtgexc( IntBuffer wantq, IntBuffer wantz, IntBuffer n,
DoubleBuffer a, IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer q, IntBuffer ldq, DoubleBuffer z, IntBuffer ldz,
IntBuffer ifst, IntBuffer ilst, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dtgexc( int[] wantq, int[] wantz, int[] n,
double[] a, int[] lda, double[] b, int[] ldb,
double[] q, int[] ldq, double[] z, int[] ldz,
int[] ifst, int[] ilst, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_ctgexc( IntPointer wantq, IntPointer wantz, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz, IntPointer ifst,
IntPointer ilst, IntPointer info );
public static native void LAPACK_ctgexc( IntBuffer wantq, IntBuffer wantz, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz, IntBuffer ifst,
IntBuffer ilst, IntBuffer info );
public static native void LAPACK_ctgexc( int[] wantq, int[] wantz, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] z, int[] ldz, int[] ifst,
int[] ilst, int[] info );
public static native void LAPACK_ztgexc( IntPointer wantq, IntPointer wantz, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz, IntPointer ifst,
IntPointer ilst, IntPointer info );
public static native void LAPACK_ztgexc( IntBuffer wantq, IntBuffer wantz, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz, IntBuffer ifst,
IntBuffer ilst, IntBuffer info );
public static native void LAPACK_ztgexc( int[] wantq, int[] wantz, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] z, int[] ldz, int[] ifst,
int[] ilst, int[] info );
public static native void LAPACK_stgsen( IntPointer ijob, IntPointer wantq,
IntPointer wantz, @Const IntPointer select,
IntPointer n, FloatPointer a, IntPointer lda, FloatPointer b,
IntPointer ldb, FloatPointer alphar, FloatPointer alphai, FloatPointer beta,
FloatPointer q, IntPointer ldq, FloatPointer z, IntPointer ldz,
IntPointer m, FloatPointer pl, FloatPointer pr, FloatPointer dif,
FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_stgsen( IntBuffer ijob, IntBuffer wantq,
IntBuffer wantz, @Const IntBuffer select,
IntBuffer n, FloatBuffer a, IntBuffer lda, FloatBuffer b,
IntBuffer ldb, FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta,
FloatBuffer q, IntBuffer ldq, FloatBuffer z, IntBuffer ldz,
IntBuffer m, FloatBuffer pl, FloatBuffer pr, FloatBuffer dif,
FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_stgsen( int[] ijob, int[] wantq,
int[] wantz, @Const int[] select,
int[] n, float[] a, int[] lda, float[] b,
int[] ldb, float[] alphar, float[] alphai, float[] beta,
float[] q, int[] ldq, float[] z, int[] ldz,
int[] m, float[] pl, float[] pr, float[] dif,
float[] work, int[] lwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_dtgsen( IntPointer ijob, IntPointer wantq,
IntPointer wantz, @Const IntPointer select,
IntPointer n, DoublePointer a, IntPointer lda, DoublePointer b,
IntPointer ldb, DoublePointer alphar, DoublePointer alphai,
DoublePointer beta, DoublePointer q, IntPointer ldq, DoublePointer z,
IntPointer ldz, IntPointer m, DoublePointer pl, DoublePointer pr,
DoublePointer dif, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dtgsen( IntBuffer ijob, IntBuffer wantq,
IntBuffer wantz, @Const IntBuffer select,
IntBuffer n, DoubleBuffer a, IntBuffer lda, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer alphar, DoubleBuffer alphai,
DoubleBuffer beta, DoubleBuffer q, IntBuffer ldq, DoubleBuffer z,
IntBuffer ldz, IntBuffer m, DoubleBuffer pl, DoubleBuffer pr,
DoubleBuffer dif, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dtgsen( int[] ijob, int[] wantq,
int[] wantz, @Const int[] select,
int[] n, double[] a, int[] lda, double[] b,
int[] ldb, double[] alphar, double[] alphai,
double[] beta, double[] q, int[] ldq, double[] z,
int[] ldz, int[] m, double[] pl, double[] pr,
double[] dif, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_ctgsen( IntPointer ijob, IntPointer wantq,
IntPointer wantz, @Const IntPointer select,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz, IntPointer m,
FloatPointer pl, FloatPointer pr, FloatPointer dif,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_ctgsen( IntBuffer ijob, IntBuffer wantq,
IntBuffer wantz, @Const IntBuffer select,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz, IntBuffer m,
FloatBuffer pl, FloatBuffer pr, FloatBuffer dif,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_ctgsen( int[] ijob, int[] wantq,
int[] wantz, @Const int[] select,
int[] n, @Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] alpha, @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] z, int[] ldz, int[] m,
float[] pl, float[] pr, float[] dif,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_ztgsen( IntPointer ijob, IntPointer wantq,
IntPointer wantz, @Const IntPointer select,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz, IntPointer m,
DoublePointer pl, DoublePointer pr, DoublePointer dif,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_ztgsen( IntBuffer ijob, IntBuffer wantq,
IntBuffer wantz, @Const IntBuffer select,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz, IntBuffer m,
DoubleBuffer pl, DoubleBuffer pr, DoubleBuffer dif,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_ztgsen( int[] ijob, int[] wantq,
int[] wantz, @Const int[] select,
int[] n, @Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] alpha, @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] z, int[] ldz, int[] m,
double[] pl, double[] pr, double[] dif,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_stgsyl( @Cast("char*") BytePointer trans, IntPointer ijob, IntPointer m, IntPointer n,
@Const FloatPointer a, IntPointer lda, @Const FloatPointer b,
IntPointer ldb, FloatPointer c, IntPointer ldc, @Const FloatPointer d,
IntPointer ldd, @Const FloatPointer e, IntPointer lde, FloatPointer f,
IntPointer ldf, FloatPointer scale, FloatPointer dif, FloatPointer work,
IntPointer lwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_stgsyl( @Cast("char*") ByteBuffer trans, IntBuffer ijob, IntBuffer m, IntBuffer n,
@Const FloatBuffer a, IntBuffer lda, @Const FloatBuffer b,
IntBuffer ldb, FloatBuffer c, IntBuffer ldc, @Const FloatBuffer d,
IntBuffer ldd, @Const FloatBuffer e, IntBuffer lde, FloatBuffer f,
IntBuffer ldf, FloatBuffer scale, FloatBuffer dif, FloatBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_stgsyl( @Cast("char*") byte[] trans, int[] ijob, int[] m, int[] n,
@Const float[] a, int[] lda, @Const float[] b,
int[] ldb, float[] c, int[] ldc, @Const float[] d,
int[] ldd, @Const float[] e, int[] lde, float[] f,
int[] ldf, float[] scale, float[] dif, float[] work,
int[] lwork, int[] iwork, int[] info );
public static native void LAPACK_dtgsyl( @Cast("char*") BytePointer trans, IntPointer ijob, IntPointer m, IntPointer n,
@Const DoublePointer a, IntPointer lda, @Const DoublePointer b,
IntPointer ldb, DoublePointer c, IntPointer ldc,
@Const DoublePointer d, IntPointer ldd, @Const DoublePointer e,
IntPointer lde, DoublePointer f, IntPointer ldf, DoublePointer scale,
DoublePointer dif, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dtgsyl( @Cast("char*") ByteBuffer trans, IntBuffer ijob, IntBuffer m, IntBuffer n,
@Const DoubleBuffer a, IntBuffer lda, @Const DoubleBuffer b,
IntBuffer ldb, DoubleBuffer c, IntBuffer ldc,
@Const DoubleBuffer d, IntBuffer ldd, @Const DoubleBuffer e,
IntBuffer lde, DoubleBuffer f, IntBuffer ldf, DoubleBuffer scale,
DoubleBuffer dif, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dtgsyl( @Cast("char*") byte[] trans, int[] ijob, int[] m, int[] n,
@Const double[] a, int[] lda, @Const double[] b,
int[] ldb, double[] c, int[] ldc,
@Const double[] d, int[] ldd, @Const double[] e,
int[] lde, double[] f, int[] ldf, double[] scale,
double[] dif, double[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_ctgsyl( @Cast("char*") BytePointer trans, IntPointer ijob, IntPointer m, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("const lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("const lapack_complex_float*") FloatPointer d, IntPointer ldd,
@Cast("const lapack_complex_float*") FloatPointer e, IntPointer lde,
@Cast("lapack_complex_float*") FloatPointer f, IntPointer ldf, FloatPointer scale,
FloatPointer dif, @Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_ctgsyl( @Cast("char*") ByteBuffer trans, IntBuffer ijob, IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("const lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("const lapack_complex_float*") FloatBuffer d, IntBuffer ldd,
@Cast("const lapack_complex_float*") FloatBuffer e, IntBuffer lde,
@Cast("lapack_complex_float*") FloatBuffer f, IntBuffer ldf, FloatBuffer scale,
FloatBuffer dif, @Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_ctgsyl( @Cast("char*") byte[] trans, int[] ijob, int[] m, int[] n,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("const lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("const lapack_complex_float*") float[] d, int[] ldd,
@Cast("const lapack_complex_float*") float[] e, int[] lde,
@Cast("lapack_complex_float*") float[] f, int[] ldf, float[] scale,
float[] dif, @Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_ztgsyl( @Cast("char*") BytePointer trans, IntPointer ijob, IntPointer m, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("const lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("const lapack_complex_double*") DoublePointer d, IntPointer ldd,
@Cast("const lapack_complex_double*") DoublePointer e, IntPointer lde,
@Cast("lapack_complex_double*") DoublePointer f, IntPointer ldf, DoublePointer scale,
DoublePointer dif, @Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_ztgsyl( @Cast("char*") ByteBuffer trans, IntBuffer ijob, IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("const lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("const lapack_complex_double*") DoubleBuffer d, IntBuffer ldd,
@Cast("const lapack_complex_double*") DoubleBuffer e, IntBuffer lde,
@Cast("lapack_complex_double*") DoubleBuffer f, IntBuffer ldf, DoubleBuffer scale,
DoubleBuffer dif, @Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_ztgsyl( @Cast("char*") byte[] trans, int[] ijob, int[] m, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("const lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("const lapack_complex_double*") double[] d, int[] ldd,
@Cast("const lapack_complex_double*") double[] e, int[] lde,
@Cast("lapack_complex_double*") double[] f, int[] ldf, double[] scale,
double[] dif, @Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_stgsna( @Cast("char*") BytePointer job, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Const FloatPointer a, IntPointer lda,
@Const FloatPointer b, IntPointer ldb, @Const FloatPointer vl,
IntPointer ldvl, @Const FloatPointer vr, IntPointer ldvr,
FloatPointer s, FloatPointer dif, IntPointer mm, IntPointer m,
FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer info );
public static native void LAPACK_stgsna( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Const FloatBuffer a, IntBuffer lda,
@Const FloatBuffer b, IntBuffer ldb, @Const FloatBuffer vl,
IntBuffer ldvl, @Const FloatBuffer vr, IntBuffer ldvr,
FloatBuffer s, FloatBuffer dif, IntBuffer mm, IntBuffer m,
FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_stgsna( @Cast("char*") byte[] job, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Const float[] a, int[] lda,
@Const float[] b, int[] ldb, @Const float[] vl,
int[] ldvl, @Const float[] vr, int[] ldvr,
float[] s, float[] dif, int[] mm, int[] m,
float[] work, int[] lwork, int[] iwork,
int[] info );
public static native void LAPACK_dtgsna( @Cast("char*") BytePointer job, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Const DoublePointer a, IntPointer lda,
@Const DoublePointer b, IntPointer ldb, @Const DoublePointer vl,
IntPointer ldvl, @Const DoublePointer vr, IntPointer ldvr,
DoublePointer s, DoublePointer dif, IntPointer mm, IntPointer m,
DoublePointer work, IntPointer lwork, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dtgsna( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Const DoubleBuffer a, IntBuffer lda,
@Const DoubleBuffer b, IntBuffer ldb, @Const DoubleBuffer vl,
IntBuffer ldvl, @Const DoubleBuffer vr, IntBuffer ldvr,
DoubleBuffer s, DoubleBuffer dif, IntBuffer mm, IntBuffer m,
DoubleBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dtgsna( @Cast("char*") byte[] job, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Const double[] a, int[] lda,
@Const double[] b, int[] ldb, @Const double[] vl,
int[] ldvl, @Const double[] vr, int[] ldvr,
double[] s, double[] dif, int[] mm, int[] m,
double[] work, int[] lwork, int[] iwork,
int[] info );
public static native void LAPACK_ctgsna( @Cast("char*") BytePointer job, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("const lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("const lapack_complex_float*") FloatPointer vl,
IntPointer ldvl, @Cast("const lapack_complex_float*") FloatPointer vr,
IntPointer ldvr, FloatPointer s, FloatPointer dif, IntPointer mm,
IntPointer m, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_ctgsna( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("const lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("const lapack_complex_float*") FloatBuffer vl,
IntBuffer ldvl, @Cast("const lapack_complex_float*") FloatBuffer vr,
IntBuffer ldvr, FloatBuffer s, FloatBuffer dif, IntBuffer mm,
IntBuffer m, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_ctgsna( @Cast("char*") byte[] job, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("const lapack_complex_float*") float[] b,
int[] ldb, @Cast("const lapack_complex_float*") float[] vl,
int[] ldvl, @Cast("const lapack_complex_float*") float[] vr,
int[] ldvr, float[] s, float[] dif, int[] mm,
int[] m, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] iwork, int[] info );
public static native void LAPACK_ztgsna( @Cast("char*") BytePointer job, @Cast("char*") BytePointer howmny, @Const IntPointer select,
IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("const lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("const lapack_complex_double*") DoublePointer vl,
IntPointer ldvl, @Cast("const lapack_complex_double*") DoublePointer vr,
IntPointer ldvr, DoublePointer s, DoublePointer dif, IntPointer mm,
IntPointer m, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_ztgsna( @Cast("char*") ByteBuffer job, @Cast("char*") ByteBuffer howmny, @Const IntBuffer select,
IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("const lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("const lapack_complex_double*") DoubleBuffer vl,
IntBuffer ldvl, @Cast("const lapack_complex_double*") DoubleBuffer vr,
IntBuffer ldvr, DoubleBuffer s, DoubleBuffer dif, IntBuffer mm,
IntBuffer m, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_ztgsna( @Cast("char*") byte[] job, @Cast("char*") byte[] howmny, @Const int[] select,
int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("const lapack_complex_double*") double[] b,
int[] ldb, @Cast("const lapack_complex_double*") double[] vl,
int[] ldvl, @Cast("const lapack_complex_double*") double[] vr,
int[] ldvr, double[] s, double[] dif, int[] mm,
int[] m, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] iwork, int[] info );
public static native void LAPACK_sggsvp3( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer p, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer b, IntPointer ldb, FloatPointer tola, FloatPointer tolb,
IntPointer k, IntPointer l, FloatPointer u, IntPointer ldu,
FloatPointer v, IntPointer ldv, FloatPointer q, IntPointer ldq,
IntPointer iwork, FloatPointer tau, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sggsvp3( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer p, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer b, IntBuffer ldb, FloatBuffer tola, FloatBuffer tolb,
IntBuffer k, IntBuffer l, FloatBuffer u, IntBuffer ldu,
FloatBuffer v, IntBuffer ldv, FloatBuffer q, IntBuffer ldq,
IntBuffer iwork, FloatBuffer tau, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sggsvp3( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] p, int[] n, float[] a, int[] lda,
float[] b, int[] ldb, float[] tola, float[] tolb,
int[] k, int[] l, float[] u, int[] ldu,
float[] v, int[] ldv, float[] q, int[] ldq,
int[] iwork, float[] tau, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dggsvp3( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer p, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer b, IntPointer ldb, DoublePointer tola, DoublePointer tolb,
IntPointer k, IntPointer l, DoublePointer u, IntPointer ldu,
DoublePointer v, IntPointer ldv, DoublePointer q, IntPointer ldq,
IntPointer iwork, DoublePointer tau, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dggsvp3( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer p, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer b, IntBuffer ldb, DoubleBuffer tola, DoubleBuffer tolb,
IntBuffer k, IntBuffer l, DoubleBuffer u, IntBuffer ldu,
DoubleBuffer v, IntBuffer ldv, DoubleBuffer q, IntBuffer ldq,
IntBuffer iwork, DoubleBuffer tau, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dggsvp3( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] p, int[] n, double[] a, int[] lda,
double[] b, int[] ldb, double[] tola, double[] tolb,
int[] k, int[] l, double[] u, int[] ldu,
double[] v, int[] ldv, double[] q, int[] ldq,
int[] iwork, double[] tau, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cggsvp3( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer p, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
FloatPointer tola, FloatPointer tolb, IntPointer k, IntPointer l,
@Cast("lapack_complex_float*") FloatPointer u, IntPointer ldu,
@Cast("lapack_complex_float*") FloatPointer v, IntPointer ldv,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq, IntPointer iwork,
FloatPointer rwork, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cggsvp3( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer p, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
FloatBuffer tola, FloatBuffer tolb, IntBuffer k, IntBuffer l,
@Cast("lapack_complex_float*") FloatBuffer u, IntBuffer ldu,
@Cast("lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq, IntBuffer iwork,
FloatBuffer rwork, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cggsvp3( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] p, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] b, int[] ldb,
float[] tola, float[] tolb, int[] k, int[] l,
@Cast("lapack_complex_float*") float[] u, int[] ldu,
@Cast("lapack_complex_float*") float[] v, int[] ldv,
@Cast("lapack_complex_float*") float[] q, int[] ldq, int[] iwork,
float[] rwork, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zggsvp3( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer p, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
DoublePointer tola, DoublePointer tolb, IntPointer k, IntPointer l,
@Cast("lapack_complex_double*") DoublePointer u, IntPointer ldu,
@Cast("lapack_complex_double*") DoublePointer v, IntPointer ldv,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
IntPointer iwork, DoublePointer rwork,
@Cast("lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zggsvp3( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer p, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
DoubleBuffer tola, DoubleBuffer tolb, IntBuffer k, IntBuffer l,
@Cast("lapack_complex_double*") DoubleBuffer u, IntBuffer ldu,
@Cast("lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
IntBuffer iwork, DoubleBuffer rwork,
@Cast("lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zggsvp3( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] p, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] b, int[] ldb,
double[] tola, double[] tolb, int[] k, int[] l,
@Cast("lapack_complex_double*") double[] u, int[] ldu,
@Cast("lapack_complex_double*") double[] v, int[] ldv,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
int[] iwork, double[] rwork,
@Cast("lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_stgsja( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer p, IntPointer n, IntPointer k, IntPointer l,
FloatPointer a, IntPointer lda, FloatPointer b, IntPointer ldb,
FloatPointer tola, FloatPointer tolb, FloatPointer alpha, FloatPointer beta,
FloatPointer u, IntPointer ldu, FloatPointer v, IntPointer ldv,
FloatPointer q, IntPointer ldq, FloatPointer work, IntPointer ncycle,
IntPointer info );
public static native void LAPACK_stgsja( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer p, IntBuffer n, IntBuffer k, IntBuffer l,
FloatBuffer a, IntBuffer lda, FloatBuffer b, IntBuffer ldb,
FloatBuffer tola, FloatBuffer tolb, FloatBuffer alpha, FloatBuffer beta,
FloatBuffer u, IntBuffer ldu, FloatBuffer v, IntBuffer ldv,
FloatBuffer q, IntBuffer ldq, FloatBuffer work, IntBuffer ncycle,
IntBuffer info );
public static native void LAPACK_stgsja( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] p, int[] n, int[] k, int[] l,
float[] a, int[] lda, float[] b, int[] ldb,
float[] tola, float[] tolb, float[] alpha, float[] beta,
float[] u, int[] ldu, float[] v, int[] ldv,
float[] q, int[] ldq, float[] work, int[] ncycle,
int[] info );
public static native void LAPACK_dtgsja( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer p, IntPointer n, IntPointer k, IntPointer l,
DoublePointer a, IntPointer lda, DoublePointer b, IntPointer ldb,
DoublePointer tola, DoublePointer tolb, DoublePointer alpha, DoublePointer beta,
DoublePointer u, IntPointer ldu, DoublePointer v, IntPointer ldv,
DoublePointer q, IntPointer ldq, DoublePointer work,
IntPointer ncycle, IntPointer info );
public static native void LAPACK_dtgsja( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer p, IntBuffer n, IntBuffer k, IntBuffer l,
DoubleBuffer a, IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer tola, DoubleBuffer tolb, DoubleBuffer alpha, DoubleBuffer beta,
DoubleBuffer u, IntBuffer ldu, DoubleBuffer v, IntBuffer ldv,
DoubleBuffer q, IntBuffer ldq, DoubleBuffer work,
IntBuffer ncycle, IntBuffer info );
public static native void LAPACK_dtgsja( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] p, int[] n, int[] k, int[] l,
double[] a, int[] lda, double[] b, int[] ldb,
double[] tola, double[] tolb, double[] alpha, double[] beta,
double[] u, int[] ldu, double[] v, int[] ldv,
double[] q, int[] ldq, double[] work,
int[] ncycle, int[] info );
public static native void LAPACK_ctgsja( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer p, IntPointer n, IntPointer k, IntPointer l,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, FloatPointer tola,
FloatPointer tolb, FloatPointer alpha, FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer u, IntPointer ldu,
@Cast("lapack_complex_float*") FloatPointer v, IntPointer ldv,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer ncycle,
IntPointer info );
public static native void LAPACK_ctgsja( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer p, IntBuffer n, IntBuffer k, IntBuffer l,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, FloatBuffer tola,
FloatBuffer tolb, FloatBuffer alpha, FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer u, IntBuffer ldu,
@Cast("lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer ncycle,
IntBuffer info );
public static native void LAPACK_ctgsja( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] p, int[] n, int[] k, int[] l,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, float[] tola,
float[] tolb, float[] alpha, float[] beta,
@Cast("lapack_complex_float*") float[] u, int[] ldu,
@Cast("lapack_complex_float*") float[] v, int[] ldv,
@Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] work, int[] ncycle,
int[] info );
public static native void LAPACK_ztgsja( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer p, IntPointer n, IntPointer k, IntPointer l,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, DoublePointer tola,
DoublePointer tolb, DoublePointer alpha, DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer u, IntPointer ldu,
@Cast("lapack_complex_double*") DoublePointer v, IntPointer ldv,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer ncycle,
IntPointer info );
public static native void LAPACK_ztgsja( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer p, IntBuffer n, IntBuffer k, IntBuffer l,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, DoubleBuffer tola,
DoubleBuffer tolb, DoubleBuffer alpha, DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer u, IntBuffer ldu,
@Cast("lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer ncycle,
IntBuffer info );
public static native void LAPACK_ztgsja( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] p, int[] n, int[] k, int[] l,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, double[] tola,
double[] tolb, double[] alpha, double[] beta,
@Cast("lapack_complex_double*") double[] u, int[] ldu,
@Cast("lapack_complex_double*") double[] v, int[] ldv,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] work, int[] ncycle,
int[] info );
public static native void LAPACK_sgels( @Cast("char*") BytePointer trans, IntPointer m, IntPointer n, IntPointer nrhs,
FloatPointer a, IntPointer lda, FloatPointer b, IntPointer ldb,
FloatPointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_sgels( @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n, IntBuffer nrhs,
FloatBuffer a, IntBuffer lda, FloatBuffer b, IntBuffer ldb,
FloatBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sgels( @Cast("char*") byte[] trans, int[] m, int[] n, int[] nrhs,
float[] a, int[] lda, float[] b, int[] ldb,
float[] work, int[] lwork, int[] info );
public static native void LAPACK_dgels( @Cast("char*") BytePointer trans, IntPointer m, IntPointer n, IntPointer nrhs,
DoublePointer a, IntPointer lda, DoublePointer b, IntPointer ldb,
DoublePointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_dgels( @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n, IntBuffer nrhs,
DoubleBuffer a, IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dgels( @Cast("char*") byte[] trans, int[] m, int[] n, int[] nrhs,
double[] a, int[] lda, double[] b, int[] ldb,
double[] work, int[] lwork, int[] info );
public static native void LAPACK_cgels( @Cast("char*") BytePointer trans, IntPointer m, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cgels( @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cgels( @Cast("char*") byte[] trans, int[] m, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zgels( @Cast("char*") BytePointer trans, IntPointer m, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zgels( @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zgels( @Cast("char*") byte[] trans, int[] m, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_sgelsy( IntPointer m, IntPointer n, IntPointer nrhs, FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb,
IntPointer jpvt, FloatPointer rcond, IntPointer rank,
FloatPointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_sgelsy( IntBuffer m, IntBuffer n, IntBuffer nrhs, FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb,
IntBuffer jpvt, FloatBuffer rcond, IntBuffer rank,
FloatBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sgelsy( int[] m, int[] n, int[] nrhs, float[] a,
int[] lda, float[] b, int[] ldb,
int[] jpvt, float[] rcond, int[] rank,
float[] work, int[] lwork, int[] info );
public static native void LAPACK_dgelsy( IntPointer m, IntPointer n, IntPointer nrhs, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb,
IntPointer jpvt, DoublePointer rcond, IntPointer rank,
DoublePointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_dgelsy( IntBuffer m, IntBuffer n, IntBuffer nrhs, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
IntBuffer jpvt, DoubleBuffer rcond, IntBuffer rank,
DoubleBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dgelsy( int[] m, int[] n, int[] nrhs, double[] a,
int[] lda, double[] b, int[] ldb,
int[] jpvt, double[] rcond, int[] rank,
double[] work, int[] lwork, int[] info );
public static native void LAPACK_cgelsy( IntPointer m, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, IntPointer jpvt,
FloatPointer rcond, IntPointer rank, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, FloatPointer rwork, IntPointer info );
public static native void LAPACK_cgelsy( IntBuffer m, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, IntBuffer jpvt,
FloatBuffer rcond, IntBuffer rank, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cgelsy( int[] m, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, int[] jpvt,
float[] rcond, int[] rank, @Cast("lapack_complex_float*") float[] work,
int[] lwork, float[] rwork, int[] info );
public static native void LAPACK_zgelsy( IntPointer m, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, IntPointer jpvt,
DoublePointer rcond, IntPointer rank,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgelsy( IntBuffer m, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, IntBuffer jpvt,
DoubleBuffer rcond, IntBuffer rank,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgelsy( int[] m, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, int[] jpvt,
double[] rcond, int[] rank,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_sgelss( IntPointer m, IntPointer n, IntPointer nrhs, FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb, FloatPointer s,
FloatPointer rcond, IntPointer rank, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sgelss( IntBuffer m, IntBuffer n, IntBuffer nrhs, FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb, FloatBuffer s,
FloatBuffer rcond, IntBuffer rank, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sgelss( int[] m, int[] n, int[] nrhs, float[] a,
int[] lda, float[] b, int[] ldb, float[] s,
float[] rcond, int[] rank, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dgelss( IntPointer m, IntPointer n, IntPointer nrhs, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb, DoublePointer s,
DoublePointer rcond, IntPointer rank, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dgelss( IntBuffer m, IntBuffer n, IntBuffer nrhs, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb, DoubleBuffer s,
DoubleBuffer rcond, IntBuffer rank, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dgelss( int[] m, int[] n, int[] nrhs, double[] a,
int[] lda, double[] b, int[] ldb, double[] s,
double[] rcond, int[] rank, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cgelss( IntPointer m, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, FloatPointer s,
FloatPointer rcond, IntPointer rank, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, FloatPointer rwork, IntPointer info );
public static native void LAPACK_cgelss( IntBuffer m, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, FloatBuffer s,
FloatBuffer rcond, IntBuffer rank, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_cgelss( int[] m, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, float[] s,
float[] rcond, int[] rank, @Cast("lapack_complex_float*") float[] work,
int[] lwork, float[] rwork, int[] info );
public static native void LAPACK_zgelss( IntPointer m, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, DoublePointer s,
DoublePointer rcond, IntPointer rank,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgelss( IntBuffer m, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, DoubleBuffer s,
DoubleBuffer rcond, IntBuffer rank,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgelss( int[] m, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, double[] s,
double[] rcond, int[] rank,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_sgelsd( IntPointer m, IntPointer n, IntPointer nrhs, FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb, FloatPointer s,
FloatPointer rcond, IntPointer rank, FloatPointer work,
IntPointer lwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_sgelsd( IntBuffer m, IntBuffer n, IntBuffer nrhs, FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb, FloatBuffer s,
FloatBuffer rcond, IntBuffer rank, FloatBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sgelsd( int[] m, int[] n, int[] nrhs, float[] a,
int[] lda, float[] b, int[] ldb, float[] s,
float[] rcond, int[] rank, float[] work,
int[] lwork, int[] iwork, int[] info );
public static native void LAPACK_dgelsd( IntPointer m, IntPointer n, IntPointer nrhs, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb, DoublePointer s,
DoublePointer rcond, IntPointer rank, DoublePointer work,
IntPointer lwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_dgelsd( IntBuffer m, IntBuffer n, IntBuffer nrhs, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb, DoubleBuffer s,
DoubleBuffer rcond, IntBuffer rank, DoubleBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgelsd( int[] m, int[] n, int[] nrhs, double[] a,
int[] lda, double[] b, int[] ldb, double[] s,
double[] rcond, int[] rank, double[] work,
int[] lwork, int[] iwork, int[] info );
public static native void LAPACK_cgelsd( IntPointer m, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, FloatPointer s,
FloatPointer rcond, IntPointer rank, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, FloatPointer rwork, IntPointer iwork,
IntPointer info );
public static native void LAPACK_cgelsd( IntBuffer m, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, FloatBuffer s,
FloatBuffer rcond, IntBuffer rank, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, FloatBuffer rwork, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_cgelsd( int[] m, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, float[] s,
float[] rcond, int[] rank, @Cast("lapack_complex_float*") float[] work,
int[] lwork, float[] rwork, int[] iwork,
int[] info );
public static native void LAPACK_zgelsd( IntPointer m, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, DoublePointer s,
DoublePointer rcond, IntPointer rank,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_zgelsd( IntBuffer m, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, DoubleBuffer s,
DoubleBuffer rcond, IntBuffer rank,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_zgelsd( int[] m, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, double[] s,
double[] rcond, int[] rank,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] iwork, int[] info );
public static native void LAPACK_sgglse( IntPointer m, IntPointer n, IntPointer p, FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb, FloatPointer c,
FloatPointer d, FloatPointer x, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sgglse( IntBuffer m, IntBuffer n, IntBuffer p, FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb, FloatBuffer c,
FloatBuffer d, FloatBuffer x, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sgglse( int[] m, int[] n, int[] p, float[] a,
int[] lda, float[] b, int[] ldb, float[] c,
float[] d, float[] x, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dgglse( IntPointer m, IntPointer n, IntPointer p, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb, DoublePointer c,
DoublePointer d, DoublePointer x, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dgglse( IntBuffer m, IntBuffer n, IntBuffer p, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb, DoubleBuffer c,
DoubleBuffer d, DoubleBuffer x, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dgglse( int[] m, int[] n, int[] p, double[] a,
int[] lda, double[] b, int[] ldb, double[] c,
double[] d, double[] x, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cgglse( IntPointer m, IntPointer n, IntPointer p,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer c, @Cast("lapack_complex_float*") FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer x, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cgglse( IntBuffer m, IntBuffer n, IntBuffer p,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer c, @Cast("lapack_complex_float*") FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer x, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cgglse( int[] m, int[] n, int[] p,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] c, @Cast("lapack_complex_float*") float[] d,
@Cast("lapack_complex_float*") float[] x, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zgglse( IntPointer m, IntPointer n, IntPointer p,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer c, @Cast("lapack_complex_double*") DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer x, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zgglse( IntBuffer m, IntBuffer n, IntBuffer p,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer c, @Cast("lapack_complex_double*") DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer x, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zgglse( int[] m, int[] n, int[] p,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] c, @Cast("lapack_complex_double*") double[] d,
@Cast("lapack_complex_double*") double[] x, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sggglm( IntPointer n, IntPointer m, IntPointer p, FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb, FloatPointer d,
FloatPointer x, FloatPointer y, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sggglm( IntBuffer n, IntBuffer m, IntBuffer p, FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb, FloatBuffer d,
FloatBuffer x, FloatBuffer y, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sggglm( int[] n, int[] m, int[] p, float[] a,
int[] lda, float[] b, int[] ldb, float[] d,
float[] x, float[] y, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dggglm( IntPointer n, IntPointer m, IntPointer p, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb, DoublePointer d,
DoublePointer x, DoublePointer y, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dggglm( IntBuffer n, IntBuffer m, IntBuffer p, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb, DoubleBuffer d,
DoubleBuffer x, DoubleBuffer y, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dggglm( int[] n, int[] m, int[] p, double[] a,
int[] lda, double[] b, int[] ldb, double[] d,
double[] x, double[] y, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cggglm( IntPointer n, IntPointer m, IntPointer p,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer d, @Cast("lapack_complex_float*") FloatPointer x,
@Cast("lapack_complex_float*") FloatPointer y, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_cggglm( IntBuffer n, IntBuffer m, IntBuffer p,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer x,
@Cast("lapack_complex_float*") FloatBuffer y, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cggglm( int[] n, int[] m, int[] p,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] d, @Cast("lapack_complex_float*") float[] x,
@Cast("lapack_complex_float*") float[] y, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_zggglm( IntPointer n, IntPointer m, IntPointer p,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer d, @Cast("lapack_complex_double*") DoublePointer x,
@Cast("lapack_complex_double*") DoublePointer y, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zggglm( IntBuffer n, IntBuffer m, IntBuffer p,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer x,
@Cast("lapack_complex_double*") DoubleBuffer y, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zggglm( int[] n, int[] m, int[] p,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] d, @Cast("lapack_complex_double*") double[] x,
@Cast("lapack_complex_double*") double[] y, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_ssyev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a,
IntPointer lda, FloatPointer w, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_ssyev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a,
IntBuffer lda, FloatBuffer w, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_ssyev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, float[] a,
int[] lda, float[] w, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dsyev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer w, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dsyev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer w, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dsyev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, double[] a,
int[] lda, double[] w, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cheev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cheev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cheev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda, float[] w,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zheev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zheev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zheev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda, double[] w,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_ssyevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a,
IntPointer lda, FloatPointer w, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_ssyevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a,
IntBuffer lda, FloatBuffer w, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_ssyevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, float[] a,
int[] lda, float[] w, float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_dsyevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer w, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dsyevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer w, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dsyevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, double[] a,
int[] lda, double[] w, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_cheevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer lrwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_cheevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer lrwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_cheevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda, float[] w,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] lrwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_zheevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_zheevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_zheevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda, double[] w,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_ssyevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer a, IntPointer lda, FloatPointer vl, FloatPointer vu,
IntPointer il, IntPointer iu, FloatPointer abstol,
IntPointer m, FloatPointer w, FloatPointer z, IntPointer ldz,
FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_ssyevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer a, IntBuffer lda, FloatBuffer vl, FloatBuffer vu,
IntBuffer il, IntBuffer iu, FloatBuffer abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, IntBuffer ldz,
FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_ssyevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
float[] a, int[] lda, float[] vl, float[] vu,
int[] il, int[] iu, float[] abstol,
int[] m, float[] w, float[] z, int[] ldz,
float[] work, int[] lwork, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_dsyevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer a, IntPointer lda, DoublePointer vl, DoublePointer vu,
IntPointer il, IntPointer iu, DoublePointer abstol,
IntPointer m, DoublePointer w, DoublePointer z, IntPointer ldz,
DoublePointer work, IntPointer lwork, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_dsyevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer a, IntBuffer lda, DoubleBuffer vl, DoubleBuffer vu,
IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
IntBuffer m, DoubleBuffer w, DoubleBuffer z, IntBuffer ldz,
DoubleBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_dsyevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
double[] a, int[] lda, double[] vl, double[] vu,
int[] il, int[] iu, double[] abstol,
int[] m, double[] w, double[] z, int[] ldz,
double[] work, int[] lwork, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_cheevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer vl,
FloatPointer vu, IntPointer il, IntPointer iu, FloatPointer abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, FloatPointer rwork, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_cheevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer vl,
FloatBuffer vu, IntBuffer il, IntBuffer iu, FloatBuffer abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, FloatBuffer rwork, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_cheevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda, float[] vl,
float[] vu, int[] il, int[] iu, float[] abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, @Cast("lapack_complex_float*") float[] work,
int[] lwork, float[] rwork, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_zheevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer vl,
DoublePointer vu, IntPointer il, IntPointer iu, DoublePointer abstol,
IntPointer m, DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
IntPointer ldz, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, DoublePointer rwork, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_zheevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer vl,
DoubleBuffer vu, IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
IntBuffer m, DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
IntBuffer ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, DoubleBuffer rwork, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_zheevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda, double[] vl,
double[] vu, int[] il, int[] iu, double[] abstol,
int[] m, double[] w, @Cast("lapack_complex_double*") double[] z,
int[] ldz, @Cast("lapack_complex_double*") double[] work,
int[] lwork, double[] rwork, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_ssyevr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer a, IntPointer lda, FloatPointer vl, FloatPointer vu,
IntPointer il, IntPointer iu, FloatPointer abstol,
IntPointer m, FloatPointer w, FloatPointer z, IntPointer ldz,
IntPointer isuppz, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_ssyevr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer a, IntBuffer lda, FloatBuffer vl, FloatBuffer vu,
IntBuffer il, IntBuffer iu, FloatBuffer abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, IntBuffer ldz,
IntBuffer isuppz, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_ssyevr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
float[] a, int[] lda, float[] vl, float[] vu,
int[] il, int[] iu, float[] abstol,
int[] m, float[] w, float[] z, int[] ldz,
int[] isuppz, float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_dsyevr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer a, IntPointer lda, DoublePointer vl, DoublePointer vu,
IntPointer il, IntPointer iu, DoublePointer abstol,
IntPointer m, DoublePointer w, DoublePointer z, IntPointer ldz,
IntPointer isuppz, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dsyevr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer a, IntBuffer lda, DoubleBuffer vl, DoubleBuffer vu,
IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
IntBuffer m, DoubleBuffer w, DoubleBuffer z, IntBuffer ldz,
IntBuffer isuppz, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dsyevr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
double[] a, int[] lda, double[] vl, double[] vu,
int[] il, int[] iu, double[] abstol,
int[] m, double[] w, double[] z, int[] ldz,
int[] isuppz, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_cheevr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer vl,
FloatPointer vu, IntPointer il, IntPointer iu, FloatPointer abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, IntPointer isuppz,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer lrwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_cheevr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer vl,
FloatBuffer vu, IntBuffer il, IntBuffer iu, FloatBuffer abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, IntBuffer isuppz,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer lrwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_cheevr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda, float[] vl,
float[] vu, int[] il, int[] iu, float[] abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, int[] isuppz,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] lrwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_zheevr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer vl,
DoublePointer vu, IntPointer il, IntPointer iu, DoublePointer abstol,
IntPointer m, DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
IntPointer ldz, IntPointer isuppz,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_zheevr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer vl,
DoubleBuffer vu, IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
IntBuffer m, DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
IntBuffer ldz, IntBuffer isuppz,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_zheevr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda, double[] vl,
double[] vu, int[] il, int[] iu, double[] abstol,
int[] m, double[] w, @Cast("lapack_complex_double*") double[] z,
int[] ldz, int[] isuppz,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_sspev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer ap, FloatPointer w,
FloatPointer z, IntPointer ldz, FloatPointer work, IntPointer info );
public static native void LAPACK_sspev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer ap, FloatBuffer w,
FloatBuffer z, IntBuffer ldz, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sspev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, float[] ap, float[] w,
float[] z, int[] ldz, float[] work, int[] info );
public static native void LAPACK_dspev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer ap, DoublePointer w,
DoublePointer z, IntPointer ldz, DoublePointer work, IntPointer info );
public static native void LAPACK_dspev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer ap, DoubleBuffer w,
DoubleBuffer z, IntBuffer ldz, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dspev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, double[] ap, double[] w,
double[] z, int[] ldz, double[] work, int[] info );
public static native void LAPACK_chpev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer ap, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_chpev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer ap, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_chpev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] ap, float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] info );
public static native void LAPACK_zhpev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer ap, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zhpev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer ap, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zhpev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] ap, double[] w,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sspevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer ap, FloatPointer w,
FloatPointer z, IntPointer ldz, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_sspevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer ap, FloatBuffer w,
FloatBuffer z, IntBuffer ldz, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_sspevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, float[] ap, float[] w,
float[] z, int[] ldz, float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_dspevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer ap,
DoublePointer w, DoublePointer z, IntPointer ldz, DoublePointer work,
IntPointer lwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_dspevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer ap,
DoubleBuffer w, DoubleBuffer z, IntBuffer ldz, DoubleBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_dspevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, double[] ap,
double[] w, double[] z, int[] ldz, double[] work,
int[] lwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_chpevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer ap, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, FloatPointer rwork, IntPointer lrwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_chpevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer ap, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, FloatBuffer rwork, IntBuffer lrwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_chpevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] ap, float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, @Cast("lapack_complex_float*") float[] work,
int[] lwork, float[] rwork, int[] lrwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_zhpevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer ap, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_zhpevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer ap, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_zhpevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] ap, double[] w,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_sspevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer ap, FloatPointer vl, FloatPointer vu, IntPointer il,
IntPointer iu, FloatPointer abstol, IntPointer m, FloatPointer w,
FloatPointer z, IntPointer ldz, FloatPointer work, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_sspevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer ap, FloatBuffer vl, FloatBuffer vu, IntBuffer il,
IntBuffer iu, FloatBuffer abstol, IntBuffer m, FloatBuffer w,
FloatBuffer z, IntBuffer ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_sspevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
float[] ap, float[] vl, float[] vu, int[] il,
int[] iu, float[] abstol, int[] m, float[] w,
float[] z, int[] ldz, float[] work, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_dspevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer ap, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, DoublePointer abstol, IntPointer m, DoublePointer w,
DoublePointer z, IntPointer ldz, DoublePointer work, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_dspevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer ap, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, DoubleBuffer abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, IntBuffer ldz, DoubleBuffer work, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_dspevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
double[] ap, double[] vl, double[] vu, int[] il,
int[] iu, double[] abstol, int[] m, double[] w,
double[] z, int[] ldz, double[] work, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_chpevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer ap, FloatPointer vl, FloatPointer vu,
IntPointer il, IntPointer iu, FloatPointer abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer iwork, IntPointer ifail, IntPointer info );
public static native void LAPACK_chpevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer ap, FloatBuffer vl, FloatBuffer vu,
IntBuffer il, IntBuffer iu, FloatBuffer abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer iwork, IntBuffer ifail, IntBuffer info );
public static native void LAPACK_chpevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] ap, float[] vl, float[] vu,
int[] il, int[] iu, float[] abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] iwork, int[] ifail, int[] info );
public static native void LAPACK_zhpevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer ap, DoublePointer vl, DoublePointer vu,
IntPointer il, IntPointer iu, DoublePointer abstol,
IntPointer m, DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
IntPointer ldz, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer iwork, IntPointer ifail, IntPointer info );
public static native void LAPACK_zhpevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer ap, DoubleBuffer vl, DoubleBuffer vu,
IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
IntBuffer m, DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
IntBuffer ldz, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer iwork, IntBuffer ifail, IntBuffer info );
public static native void LAPACK_zhpevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] ap, double[] vl, double[] vu,
int[] il, int[] iu, double[] abstol,
int[] m, double[] w, @Cast("lapack_complex_double*") double[] z,
int[] ldz, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] iwork, int[] ifail, int[] info );
public static native void LAPACK_ssbev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
FloatPointer ab, IntPointer ldab, FloatPointer w, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer info );
public static native void LAPACK_ssbev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
FloatBuffer ab, IntBuffer ldab, FloatBuffer w, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer info );
public static native void LAPACK_ssbev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] kd,
float[] ab, int[] ldab, float[] w, float[] z,
int[] ldz, float[] work, int[] info );
public static native void LAPACK_dsbev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
DoublePointer ab, IntPointer ldab, DoublePointer w, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer info );
public static native void LAPACK_dsbev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
DoubleBuffer ab, IntBuffer ldab, DoubleBuffer w, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dsbev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] kd,
double[] ab, int[] ldab, double[] w, double[] z,
int[] ldz, double[] work, int[] info );
public static native void LAPACK_chbev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork, IntPointer info );
public static native void LAPACK_chbev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_chbev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("lapack_complex_float*") float[] ab, int[] ldab, float[] w,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, float[] rwork, int[] info );
public static native void LAPACK_zhbev( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zhbev( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zhbev( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("lapack_complex_double*") double[] ab, int[] ldab, double[] w,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_ssbevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
FloatPointer ab, IntPointer ldab, FloatPointer w, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_ssbevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
FloatBuffer ab, IntBuffer ldab, FloatBuffer w, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_ssbevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] kd,
float[] ab, int[] ldab, float[] w, float[] z,
int[] ldz, float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_dsbevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
DoublePointer ab, IntPointer ldab, DoublePointer w, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dsbevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
DoubleBuffer ab, IntBuffer ldab, DoubleBuffer w, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dsbevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] kd,
double[] ab, int[] ldab, double[] w, double[] z,
int[] ldz, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_chbevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer lrwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_chbevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer lrwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_chbevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("lapack_complex_float*") float[] ab, int[] ldab, float[] w,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] lrwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_zhbevd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer kd,
@Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_zhbevd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer kd,
@Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_zhbevd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] kd,
@Cast("lapack_complex_double*") double[] ab, int[] ldab, double[] w,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_ssbevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer kd, FloatPointer ab, IntPointer ldab, FloatPointer q,
IntPointer ldq, FloatPointer vl, FloatPointer vu, IntPointer il,
IntPointer iu, FloatPointer abstol, IntPointer m, FloatPointer w,
FloatPointer z, IntPointer ldz, FloatPointer work, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_ssbevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer kd, FloatBuffer ab, IntBuffer ldab, FloatBuffer q,
IntBuffer ldq, FloatBuffer vl, FloatBuffer vu, IntBuffer il,
IntBuffer iu, FloatBuffer abstol, IntBuffer m, FloatBuffer w,
FloatBuffer z, IntBuffer ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_ssbevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
int[] kd, float[] ab, int[] ldab, float[] q,
int[] ldq, float[] vl, float[] vu, int[] il,
int[] iu, float[] abstol, int[] m, float[] w,
float[] z, int[] ldz, float[] work, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_dsbevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer kd, DoublePointer ab, IntPointer ldab, DoublePointer q,
IntPointer ldq, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, DoublePointer abstol, IntPointer m, DoublePointer w,
DoublePointer z, IntPointer ldz, DoublePointer work, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_dsbevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer kd, DoubleBuffer ab, IntBuffer ldab, DoubleBuffer q,
IntBuffer ldq, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, DoubleBuffer abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, IntBuffer ldz, DoubleBuffer work, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_dsbevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
int[] kd, double[] ab, int[] ldab, double[] q,
int[] ldq, double[] vl, double[] vu, int[] il,
int[] iu, double[] abstol, int[] m, double[] w,
double[] z, int[] ldz, double[] work, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_chbevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer kd, @Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq, FloatPointer vl,
FloatPointer vu, IntPointer il, IntPointer iu, FloatPointer abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer iwork, IntPointer ifail, IntPointer info );
public static native void LAPACK_chbevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer kd, @Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq, FloatBuffer vl,
FloatBuffer vu, IntBuffer il, IntBuffer iu, FloatBuffer abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer iwork, IntBuffer ifail, IntBuffer info );
public static native void LAPACK_chbevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
int[] kd, @Cast("lapack_complex_float*") float[] ab, int[] ldab,
@Cast("lapack_complex_float*") float[] q, int[] ldq, float[] vl,
float[] vu, int[] il, int[] iu, float[] abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] iwork, int[] ifail, int[] info );
public static native void LAPACK_zhbevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer kd, @Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq, DoublePointer vl,
DoublePointer vu, IntPointer il, IntPointer iu, DoublePointer abstol,
IntPointer m, DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
IntPointer ldz, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer iwork, IntPointer ifail, IntPointer info );
public static native void LAPACK_zhbevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer kd, @Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq, DoubleBuffer vl,
DoubleBuffer vu, IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
IntBuffer m, DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
IntBuffer ldz, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer iwork, IntBuffer ifail, IntBuffer info );
public static native void LAPACK_zhbevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
int[] kd, @Cast("lapack_complex_double*") double[] ab, int[] ldab,
@Cast("lapack_complex_double*") double[] q, int[] ldq, double[] vl,
double[] vu, int[] il, int[] iu, double[] abstol,
int[] m, double[] w, @Cast("lapack_complex_double*") double[] z,
int[] ldz, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] iwork, int[] ifail, int[] info );
public static native void LAPACK_sstev( @Cast("char*") BytePointer jobz, IntPointer n, FloatPointer d, FloatPointer e, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer info );
public static native void LAPACK_sstev( @Cast("char*") ByteBuffer jobz, IntBuffer n, FloatBuffer d, FloatBuffer e, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sstev( @Cast("char*") byte[] jobz, int[] n, float[] d, float[] e, float[] z,
int[] ldz, float[] work, int[] info );
public static native void LAPACK_dstev( @Cast("char*") BytePointer jobz, IntPointer n, DoublePointer d, DoublePointer e, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer info );
public static native void LAPACK_dstev( @Cast("char*") ByteBuffer jobz, IntBuffer n, DoubleBuffer d, DoubleBuffer e, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dstev( @Cast("char*") byte[] jobz, int[] n, double[] d, double[] e, double[] z,
int[] ldz, double[] work, int[] info );
public static native void LAPACK_sstevd( @Cast("char*") BytePointer jobz, IntPointer n, FloatPointer d, FloatPointer e, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_sstevd( @Cast("char*") ByteBuffer jobz, IntBuffer n, FloatBuffer d, FloatBuffer e, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_sstevd( @Cast("char*") byte[] jobz, int[] n, float[] d, float[] e, float[] z,
int[] ldz, float[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_dstevd( @Cast("char*") BytePointer jobz, IntPointer n, DoublePointer d, DoublePointer e, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dstevd( @Cast("char*") ByteBuffer jobz, IntBuffer n, DoubleBuffer d, DoubleBuffer e, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dstevd( @Cast("char*") byte[] jobz, int[] n, double[] d, double[] e, double[] z,
int[] ldz, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_sstevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, FloatPointer d, FloatPointer e,
FloatPointer vl, FloatPointer vu, IntPointer il, IntPointer iu,
FloatPointer abstol, IntPointer m, FloatPointer w, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_sstevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, FloatBuffer d, FloatBuffer e,
FloatBuffer vl, FloatBuffer vu, IntBuffer il, IntBuffer iu,
FloatBuffer abstol, IntBuffer m, FloatBuffer w, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_sstevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, float[] d, float[] e,
float[] vl, float[] vu, int[] il, int[] iu,
float[] abstol, int[] m, float[] w, float[] z,
int[] ldz, float[] work, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_dstevx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, DoublePointer d,
DoublePointer e, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, DoublePointer abstol, IntPointer m, DoublePointer w,
DoublePointer z, IntPointer ldz, DoublePointer work, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_dstevx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, DoubleBuffer d,
DoubleBuffer e, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, DoubleBuffer abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, IntBuffer ldz, DoubleBuffer work, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_dstevx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, double[] d,
double[] e, double[] vl, double[] vu, int[] il,
int[] iu, double[] abstol, int[] m, double[] w,
double[] z, int[] ldz, double[] work, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_sstevr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, FloatPointer d, FloatPointer e,
FloatPointer vl, FloatPointer vu, IntPointer il, IntPointer iu,
FloatPointer abstol, IntPointer m, FloatPointer w, FloatPointer z,
IntPointer ldz, IntPointer isuppz, FloatPointer work,
IntPointer lwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_sstevr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, FloatBuffer d, FloatBuffer e,
FloatBuffer vl, FloatBuffer vu, IntBuffer il, IntBuffer iu,
FloatBuffer abstol, IntBuffer m, FloatBuffer w, FloatBuffer z,
IntBuffer ldz, IntBuffer isuppz, FloatBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_sstevr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, float[] d, float[] e,
float[] vl, float[] vu, int[] il, int[] iu,
float[] abstol, int[] m, float[] w, float[] z,
int[] ldz, int[] isuppz, float[] work,
int[] lwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_dstevr( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, IntPointer n, DoublePointer d,
DoublePointer e, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, DoublePointer abstol, IntPointer m, DoublePointer w,
DoublePointer z, IntPointer ldz, IntPointer isuppz,
DoublePointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_dstevr( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, IntBuffer n, DoubleBuffer d,
DoubleBuffer e, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, DoubleBuffer abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, IntBuffer ldz, IntBuffer isuppz,
DoubleBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dstevr( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, int[] n, double[] d,
double[] e, double[] vl, double[] vu, int[] il,
int[] iu, double[] abstol, int[] m, double[] w,
double[] z, int[] ldz, int[] isuppz,
double[] work, int[] lwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_sgees( @Cast("char*") BytePointer jobvs, @Cast("char*") BytePointer sort, LAPACK_S_SELECT2 select,
IntPointer n, FloatPointer a, IntPointer lda, IntPointer sdim,
FloatPointer wr, FloatPointer wi, FloatPointer vs, IntPointer ldvs,
FloatPointer work, IntPointer lwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_sgees( @Cast("char*") ByteBuffer jobvs, @Cast("char*") ByteBuffer sort, LAPACK_S_SELECT2 select,
IntBuffer n, FloatBuffer a, IntBuffer lda, IntBuffer sdim,
FloatBuffer wr, FloatBuffer wi, FloatBuffer vs, IntBuffer ldvs,
FloatBuffer work, IntBuffer lwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_sgees( @Cast("char*") byte[] jobvs, @Cast("char*") byte[] sort, LAPACK_S_SELECT2 select,
int[] n, float[] a, int[] lda, int[] sdim,
float[] wr, float[] wi, float[] vs, int[] ldvs,
float[] work, int[] lwork, int[] bwork,
int[] info );
public static native void LAPACK_dgees( @Cast("char*") BytePointer jobvs, @Cast("char*") BytePointer sort, LAPACK_D_SELECT2 select,
IntPointer n, DoublePointer a, IntPointer lda, IntPointer sdim,
DoublePointer wr, DoublePointer wi, DoublePointer vs, IntPointer ldvs,
DoublePointer work, IntPointer lwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_dgees( @Cast("char*") ByteBuffer jobvs, @Cast("char*") ByteBuffer sort, LAPACK_D_SELECT2 select,
IntBuffer n, DoubleBuffer a, IntBuffer lda, IntBuffer sdim,
DoubleBuffer wr, DoubleBuffer wi, DoubleBuffer vs, IntBuffer ldvs,
DoubleBuffer work, IntBuffer lwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_dgees( @Cast("char*") byte[] jobvs, @Cast("char*") byte[] sort, LAPACK_D_SELECT2 select,
int[] n, double[] a, int[] lda, int[] sdim,
double[] wr, double[] wi, double[] vs, int[] ldvs,
double[] work, int[] lwork, int[] bwork,
int[] info );
public static native void LAPACK_cgees( @Cast("char*") BytePointer jobvs, @Cast("char*") BytePointer sort, LAPACK_C_SELECT1 select,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
IntPointer sdim, @Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer vs, IntPointer ldvs,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer bwork, IntPointer info );
public static native void LAPACK_cgees( @Cast("char*") ByteBuffer jobvs, @Cast("char*") ByteBuffer sort, LAPACK_C_SELECT1 select,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
IntBuffer sdim, @Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer vs, IntBuffer ldvs,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer bwork, IntBuffer info );
public static native void LAPACK_cgees( @Cast("char*") byte[] jobvs, @Cast("char*") byte[] sort, LAPACK_C_SELECT1 select,
int[] n, @Cast("lapack_complex_float*") float[] a, int[] lda,
int[] sdim, @Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] vs, int[] ldvs,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] bwork, int[] info );
public static native void LAPACK_zgees( @Cast("char*") BytePointer jobvs, @Cast("char*") BytePointer sort, LAPACK_Z_SELECT1 select,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
IntPointer sdim, @Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vs, IntPointer ldvs,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer bwork, IntPointer info );
public static native void LAPACK_zgees( @Cast("char*") ByteBuffer jobvs, @Cast("char*") ByteBuffer sort, LAPACK_Z_SELECT1 select,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
IntBuffer sdim, @Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vs, IntBuffer ldvs,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer bwork, IntBuffer info );
public static native void LAPACK_zgees( @Cast("char*") byte[] jobvs, @Cast("char*") byte[] sort, LAPACK_Z_SELECT1 select,
int[] n, @Cast("lapack_complex_double*") double[] a, int[] lda,
int[] sdim, @Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vs, int[] ldvs,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] bwork, int[] info );
public static native void LAPACK_sgeesx( @Cast("char*") BytePointer jobvs, @Cast("char*") BytePointer sort, LAPACK_S_SELECT2 select,
@Cast("char*") BytePointer sense, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer sdim, FloatPointer wr, FloatPointer wi, FloatPointer vs,
IntPointer ldvs, FloatPointer rconde, FloatPointer rcondv, FloatPointer work,
IntPointer lwork, IntPointer iwork, IntPointer liwork,
IntPointer bwork, IntPointer info );
public static native void LAPACK_sgeesx( @Cast("char*") ByteBuffer jobvs, @Cast("char*") ByteBuffer sort, LAPACK_S_SELECT2 select,
@Cast("char*") ByteBuffer sense, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer sdim, FloatBuffer wr, FloatBuffer wi, FloatBuffer vs,
IntBuffer ldvs, FloatBuffer rconde, FloatBuffer rcondv, FloatBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer bwork, IntBuffer info );
public static native void LAPACK_sgeesx( @Cast("char*") byte[] jobvs, @Cast("char*") byte[] sort, LAPACK_S_SELECT2 select,
@Cast("char*") byte[] sense, int[] n, float[] a, int[] lda,
int[] sdim, float[] wr, float[] wi, float[] vs,
int[] ldvs, float[] rconde, float[] rcondv, float[] work,
int[] lwork, int[] iwork, int[] liwork,
int[] bwork, int[] info );
public static native void LAPACK_dgeesx( @Cast("char*") BytePointer jobvs, @Cast("char*") BytePointer sort, LAPACK_D_SELECT2 select,
@Cast("char*") BytePointer sense, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer sdim, DoublePointer wr, DoublePointer wi, DoublePointer vs,
IntPointer ldvs, DoublePointer rconde, DoublePointer rcondv,
DoublePointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_dgeesx( @Cast("char*") ByteBuffer jobvs, @Cast("char*") ByteBuffer sort, LAPACK_D_SELECT2 select,
@Cast("char*") ByteBuffer sense, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer sdim, DoubleBuffer wr, DoubleBuffer wi, DoubleBuffer vs,
IntBuffer ldvs, DoubleBuffer rconde, DoubleBuffer rcondv,
DoubleBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_dgeesx( @Cast("char*") byte[] jobvs, @Cast("char*") byte[] sort, LAPACK_D_SELECT2 select,
@Cast("char*") byte[] sense, int[] n, double[] a, int[] lda,
int[] sdim, double[] wr, double[] wi, double[] vs,
int[] ldvs, double[] rconde, double[] rcondv,
double[] work, int[] lwork, int[] iwork,
int[] liwork, int[] bwork,
int[] info );
public static native void LAPACK_cgeesx( @Cast("char*") BytePointer jobvs, @Cast("char*") BytePointer sort, LAPACK_C_SELECT1 select,
@Cast("char*") BytePointer sense, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer sdim, @Cast("lapack_complex_float*") FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer vs, IntPointer ldvs, FloatPointer rconde,
FloatPointer rcondv, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, FloatPointer rwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_cgeesx( @Cast("char*") ByteBuffer jobvs, @Cast("char*") ByteBuffer sort, LAPACK_C_SELECT1 select,
@Cast("char*") ByteBuffer sense, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer sdim, @Cast("lapack_complex_float*") FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer vs, IntBuffer ldvs, FloatBuffer rconde,
FloatBuffer rcondv, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, FloatBuffer rwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_cgeesx( @Cast("char*") byte[] jobvs, @Cast("char*") byte[] sort, LAPACK_C_SELECT1 select,
@Cast("char*") byte[] sense, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] sdim, @Cast("lapack_complex_float*") float[] w,
@Cast("lapack_complex_float*") float[] vs, int[] ldvs, float[] rconde,
float[] rcondv, @Cast("lapack_complex_float*") float[] work,
int[] lwork, float[] rwork, int[] bwork,
int[] info );
public static native void LAPACK_zgeesx( @Cast("char*") BytePointer jobvs, @Cast("char*") BytePointer sort, LAPACK_Z_SELECT1 select,
@Cast("char*") BytePointer sense, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer sdim, @Cast("lapack_complex_double*") DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer vs, IntPointer ldvs, DoublePointer rconde,
DoublePointer rcondv, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, DoublePointer rwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_zgeesx( @Cast("char*") ByteBuffer jobvs, @Cast("char*") ByteBuffer sort, LAPACK_Z_SELECT1 select,
@Cast("char*") ByteBuffer sense, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer sdim, @Cast("lapack_complex_double*") DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer vs, IntBuffer ldvs, DoubleBuffer rconde,
DoubleBuffer rcondv, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, DoubleBuffer rwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_zgeesx( @Cast("char*") byte[] jobvs, @Cast("char*") byte[] sort, LAPACK_Z_SELECT1 select,
@Cast("char*") byte[] sense, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] sdim, @Cast("lapack_complex_double*") double[] w,
@Cast("lapack_complex_double*") double[] vs, int[] ldvs, double[] rconde,
double[] rcondv, @Cast("lapack_complex_double*") double[] work,
int[] lwork, double[] rwork, int[] bwork,
int[] info );
public static native void LAPACK_sgeev( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n, FloatPointer a,
IntPointer lda, FloatPointer wr, FloatPointer wi, FloatPointer vl,
IntPointer ldvl, FloatPointer vr, IntPointer ldvr, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sgeev( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n, FloatBuffer a,
IntBuffer lda, FloatBuffer wr, FloatBuffer wi, FloatBuffer vl,
IntBuffer ldvl, FloatBuffer vr, IntBuffer ldvr, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sgeev( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n, float[] a,
int[] lda, float[] wr, float[] wi, float[] vl,
int[] ldvl, float[] vr, int[] ldvr, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dgeev( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer wr, DoublePointer wi, DoublePointer vl,
IntPointer ldvl, DoublePointer vr, IntPointer ldvr, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dgeev( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer wr, DoubleBuffer wi, DoubleBuffer vl,
IntBuffer ldvl, DoubleBuffer vr, IntBuffer ldvr, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dgeev( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n, double[] a,
int[] lda, double[] wr, double[] wi, double[] vl,
int[] ldvl, double[] vr, int[] ldvr, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cgeev( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer w, @Cast("lapack_complex_float*") FloatPointer vl,
IntPointer ldvl, @Cast("lapack_complex_float*") FloatPointer vr, IntPointer ldvr,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cgeev( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer vl,
IntBuffer ldvl, @Cast("lapack_complex_float*") FloatBuffer vr, IntBuffer ldvr,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cgeev( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] w, @Cast("lapack_complex_float*") float[] vl,
int[] ldvl, @Cast("lapack_complex_float*") float[] vr, int[] ldvr,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zgeev( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer w, @Cast("lapack_complex_double*") DoublePointer vl,
IntPointer ldvl, @Cast("lapack_complex_double*") DoublePointer vr,
IntPointer ldvr, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgeev( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer vl,
IntBuffer ldvl, @Cast("lapack_complex_double*") DoubleBuffer vr,
IntBuffer ldvr, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgeev( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] w, @Cast("lapack_complex_double*") double[] vl,
int[] ldvl, @Cast("lapack_complex_double*") double[] vr,
int[] ldvr, @Cast("lapack_complex_double*") double[] work,
int[] lwork, double[] rwork, int[] info );
public static native void LAPACK_sgeevx( @Cast("char*") BytePointer balanc, @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, @Cast("char*") BytePointer sense,
IntPointer n, FloatPointer a, IntPointer lda, FloatPointer wr,
FloatPointer wi, FloatPointer vl, IntPointer ldvl, FloatPointer vr,
IntPointer ldvr, IntPointer ilo, IntPointer ihi,
FloatPointer scale, FloatPointer abnrm, FloatPointer rconde, FloatPointer rcondv,
FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sgeevx( @Cast("char*") ByteBuffer balanc, @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, @Cast("char*") ByteBuffer sense,
IntBuffer n, FloatBuffer a, IntBuffer lda, FloatBuffer wr,
FloatBuffer wi, FloatBuffer vl, IntBuffer ldvl, FloatBuffer vr,
IntBuffer ldvr, IntBuffer ilo, IntBuffer ihi,
FloatBuffer scale, FloatBuffer abnrm, FloatBuffer rconde, FloatBuffer rcondv,
FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sgeevx( @Cast("char*") byte[] balanc, @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, @Cast("char*") byte[] sense,
int[] n, float[] a, int[] lda, float[] wr,
float[] wi, float[] vl, int[] ldvl, float[] vr,
int[] ldvr, int[] ilo, int[] ihi,
float[] scale, float[] abnrm, float[] rconde, float[] rcondv,
float[] work, int[] lwork, int[] iwork,
int[] info );
public static native void LAPACK_dgeevx( @Cast("char*") BytePointer balanc, @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, @Cast("char*") BytePointer sense,
IntPointer n, DoublePointer a, IntPointer lda, DoublePointer wr,
DoublePointer wi, DoublePointer vl, IntPointer ldvl, DoublePointer vr,
IntPointer ldvr, IntPointer ilo, IntPointer ihi,
DoublePointer scale, DoublePointer abnrm, DoublePointer rconde,
DoublePointer rcondv, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dgeevx( @Cast("char*") ByteBuffer balanc, @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, @Cast("char*") ByteBuffer sense,
IntBuffer n, DoubleBuffer a, IntBuffer lda, DoubleBuffer wr,
DoubleBuffer wi, DoubleBuffer vl, IntBuffer ldvl, DoubleBuffer vr,
IntBuffer ldvr, IntBuffer ilo, IntBuffer ihi,
DoubleBuffer scale, DoubleBuffer abnrm, DoubleBuffer rconde,
DoubleBuffer rcondv, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgeevx( @Cast("char*") byte[] balanc, @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, @Cast("char*") byte[] sense,
int[] n, double[] a, int[] lda, double[] wr,
double[] wi, double[] vl, int[] ldvl, double[] vr,
int[] ldvr, int[] ilo, int[] ihi,
double[] scale, double[] abnrm, double[] rconde,
double[] rcondv, double[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_cgeevx( @Cast("char*") BytePointer balanc, @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, @Cast("char*") BytePointer sense,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer w, @Cast("lapack_complex_float*") FloatPointer vl,
IntPointer ldvl, @Cast("lapack_complex_float*") FloatPointer vr,
IntPointer ldvr, IntPointer ilo, IntPointer ihi,
FloatPointer scale, FloatPointer abnrm, FloatPointer rconde, FloatPointer rcondv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cgeevx( @Cast("char*") ByteBuffer balanc, @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, @Cast("char*") ByteBuffer sense,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer vl,
IntBuffer ldvl, @Cast("lapack_complex_float*") FloatBuffer vr,
IntBuffer ldvr, IntBuffer ilo, IntBuffer ihi,
FloatBuffer scale, FloatBuffer abnrm, FloatBuffer rconde, FloatBuffer rcondv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cgeevx( @Cast("char*") byte[] balanc, @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, @Cast("char*") byte[] sense,
int[] n, @Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] w, @Cast("lapack_complex_float*") float[] vl,
int[] ldvl, @Cast("lapack_complex_float*") float[] vr,
int[] ldvr, int[] ilo, int[] ihi,
float[] scale, float[] abnrm, float[] rconde, float[] rcondv,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zgeevx( @Cast("char*") BytePointer balanc, @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, @Cast("char*") BytePointer sense,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer w, @Cast("lapack_complex_double*") DoublePointer vl,
IntPointer ldvl, @Cast("lapack_complex_double*") DoublePointer vr,
IntPointer ldvr, IntPointer ilo, IntPointer ihi,
DoublePointer scale, DoublePointer abnrm, DoublePointer rconde,
DoublePointer rcondv, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgeevx( @Cast("char*") ByteBuffer balanc, @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, @Cast("char*") ByteBuffer sense,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer vl,
IntBuffer ldvl, @Cast("lapack_complex_double*") DoubleBuffer vr,
IntBuffer ldvr, IntBuffer ilo, IntBuffer ihi,
DoubleBuffer scale, DoubleBuffer abnrm, DoubleBuffer rconde,
DoubleBuffer rcondv, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgeevx( @Cast("char*") byte[] balanc, @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, @Cast("char*") byte[] sense,
int[] n, @Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] w, @Cast("lapack_complex_double*") double[] vl,
int[] ldvl, @Cast("lapack_complex_double*") double[] vr,
int[] ldvr, int[] ilo, int[] ihi,
double[] scale, double[] abnrm, double[] rconde,
double[] rcondv, @Cast("lapack_complex_double*") double[] work,
int[] lwork, double[] rwork, int[] info );
public static native void LAPACK_sgesvd( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobvt, IntPointer m, IntPointer n,
FloatPointer a, IntPointer lda, FloatPointer s, FloatPointer u,
IntPointer ldu, FloatPointer vt, IntPointer ldvt, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sgesvd( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobvt, IntBuffer m, IntBuffer n,
FloatBuffer a, IntBuffer lda, FloatBuffer s, FloatBuffer u,
IntBuffer ldu, FloatBuffer vt, IntBuffer ldvt, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sgesvd( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobvt, int[] m, int[] n,
float[] a, int[] lda, float[] s, float[] u,
int[] ldu, float[] vt, int[] ldvt, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_dgesvd( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobvt, IntPointer m, IntPointer n,
DoublePointer a, IntPointer lda, DoublePointer s, DoublePointer u,
IntPointer ldu, DoublePointer vt, IntPointer ldvt, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dgesvd( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobvt, IntBuffer m, IntBuffer n,
DoubleBuffer a, IntBuffer lda, DoubleBuffer s, DoubleBuffer u,
IntBuffer ldu, DoubleBuffer vt, IntBuffer ldvt, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dgesvd( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobvt, int[] m, int[] n,
double[] a, int[] lda, double[] s, double[] u,
int[] ldu, double[] vt, int[] ldvt, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cgesvd( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobvt, IntPointer m, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer s,
@Cast("lapack_complex_float*") FloatPointer u, IntPointer ldu,
@Cast("lapack_complex_float*") FloatPointer vt, IntPointer ldvt,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cgesvd( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobvt, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer s,
@Cast("lapack_complex_float*") FloatBuffer u, IntBuffer ldu,
@Cast("lapack_complex_float*") FloatBuffer vt, IntBuffer ldvt,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cgesvd( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobvt, int[] m, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda, float[] s,
@Cast("lapack_complex_float*") float[] u, int[] ldu,
@Cast("lapack_complex_float*") float[] vt, int[] ldvt,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zgesvd( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobvt, IntPointer m, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer s,
@Cast("lapack_complex_double*") DoublePointer u, IntPointer ldu,
@Cast("lapack_complex_double*") DoublePointer vt, IntPointer ldvt,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zgesvd( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobvt, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer s,
@Cast("lapack_complex_double*") DoubleBuffer u, IntBuffer ldu,
@Cast("lapack_complex_double*") DoubleBuffer vt, IntBuffer ldvt,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zgesvd( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobvt, int[] m, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda, double[] s,
@Cast("lapack_complex_double*") double[] u, int[] ldu,
@Cast("lapack_complex_double*") double[] vt, int[] ldvt,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_sgesvdx( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobvt, @Cast("char*") BytePointer range, IntPointer m, IntPointer n,
FloatPointer a, IntPointer lda, IntPointer vl, IntPointer vu,
IntPointer il, IntPointer iu, IntPointer ns, FloatPointer s, FloatPointer u,
IntPointer ldu, FloatPointer vt, IntPointer ldvt, FloatPointer work,
IntPointer lwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_sgesvdx( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobvt, @Cast("char*") ByteBuffer range, IntBuffer m, IntBuffer n,
FloatBuffer a, IntBuffer lda, IntBuffer vl, IntBuffer vu,
IntBuffer il, IntBuffer iu, IntBuffer ns, FloatBuffer s, FloatBuffer u,
IntBuffer ldu, FloatBuffer vt, IntBuffer ldvt, FloatBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sgesvdx( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobvt, @Cast("char*") byte[] range, int[] m, int[] n,
float[] a, int[] lda, int[] vl, int[] vu,
int[] il, int[] iu, int[] ns, float[] s, float[] u,
int[] ldu, float[] vt, int[] ldvt, float[] work,
int[] lwork, int[] iwork, int[] info );
public static native void LAPACK_dgesvdx( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobvt, @Cast("char*") BytePointer range, IntPointer m, IntPointer n,
DoublePointer a, IntPointer lda, IntPointer vl, IntPointer vu,
IntPointer il, IntPointer iu, IntPointer ns, DoublePointer s, DoublePointer u,
IntPointer ldu, DoublePointer vt, IntPointer ldvt, DoublePointer work,
IntPointer lwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_dgesvdx( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobvt, @Cast("char*") ByteBuffer range, IntBuffer m, IntBuffer n,
DoubleBuffer a, IntBuffer lda, IntBuffer vl, IntBuffer vu,
IntBuffer il, IntBuffer iu, IntBuffer ns, DoubleBuffer s, DoubleBuffer u,
IntBuffer ldu, DoubleBuffer vt, IntBuffer ldvt, DoubleBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgesvdx( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobvt, @Cast("char*") byte[] range, int[] m, int[] n,
double[] a, int[] lda, int[] vl, int[] vu,
int[] il, int[] iu, int[] ns, double[] s, double[] u,
int[] ldu, double[] vt, int[] ldvt, double[] work,
int[] lwork, int[] iwork, int[] info );
public static native void LAPACK_cgesvdx( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobvt, @Cast("char*") BytePointer range, IntPointer m, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, IntPointer vl, IntPointer vu,
IntPointer il, IntPointer iu, IntPointer ns, FloatPointer s,
@Cast("lapack_complex_float*") FloatPointer u, IntPointer ldu,
@Cast("lapack_complex_float*") FloatPointer vt, IntPointer ldvt,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_cgesvdx( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobvt, @Cast("char*") ByteBuffer range, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, IntBuffer vl, IntBuffer vu,
IntBuffer il, IntBuffer iu, IntBuffer ns, FloatBuffer s,
@Cast("lapack_complex_float*") FloatBuffer u, IntBuffer ldu,
@Cast("lapack_complex_float*") FloatBuffer vt, IntBuffer ldvt,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_cgesvdx( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobvt, @Cast("char*") byte[] range, int[] m, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda, int[] vl, int[] vu,
int[] il, int[] iu, int[] ns, float[] s,
@Cast("lapack_complex_float*") float[] u, int[] ldu,
@Cast("lapack_complex_float*") float[] vt, int[] ldvt,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] iwork, int[] info );
public static native void LAPACK_zgesvdx( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobvt, @Cast("char*") BytePointer range, IntPointer m, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, IntPointer vl, IntPointer vu,
IntPointer il, IntPointer iu, IntPointer ns, DoublePointer s,
@Cast("lapack_complex_double*") DoublePointer u, IntPointer ldu,
@Cast("lapack_complex_double*") DoublePointer vt, IntPointer ldvt,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_zgesvdx( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobvt, @Cast("char*") ByteBuffer range, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, IntBuffer vl, IntBuffer vu,
IntBuffer il, IntBuffer iu, IntBuffer ns, DoubleBuffer s,
@Cast("lapack_complex_double*") DoubleBuffer u, IntBuffer ldu,
@Cast("lapack_complex_double*") DoubleBuffer vt, IntBuffer ldvt,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_zgesvdx( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobvt, @Cast("char*") byte[] range, int[] m, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda, int[] vl, int[] vu,
int[] il, int[] iu, int[] ns, double[] s,
@Cast("lapack_complex_double*") double[] u, int[] ldu,
@Cast("lapack_complex_double*") double[] vt, int[] ldvt,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] iwork, int[] info );
public static native void LAPACK_sgesdd( @Cast("char*") BytePointer jobz, IntPointer m, IntPointer n, FloatPointer a,
IntPointer lda, FloatPointer s, FloatPointer u, IntPointer ldu,
FloatPointer vt, IntPointer ldvt, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_sgesdd( @Cast("char*") ByteBuffer jobz, IntBuffer m, IntBuffer n, FloatBuffer a,
IntBuffer lda, FloatBuffer s, FloatBuffer u, IntBuffer ldu,
FloatBuffer vt, IntBuffer ldvt, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sgesdd( @Cast("char*") byte[] jobz, int[] m, int[] n, float[] a,
int[] lda, float[] s, float[] u, int[] ldu,
float[] vt, int[] ldvt, float[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_dgesdd( @Cast("char*") BytePointer jobz, IntPointer m, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer s, DoublePointer u, IntPointer ldu,
DoublePointer vt, IntPointer ldvt, DoublePointer work,
IntPointer lwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_dgesdd( @Cast("char*") ByteBuffer jobz, IntBuffer m, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer s, DoubleBuffer u, IntBuffer ldu,
DoubleBuffer vt, IntBuffer ldvt, DoubleBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgesdd( @Cast("char*") byte[] jobz, int[] m, int[] n, double[] a,
int[] lda, double[] s, double[] u, int[] ldu,
double[] vt, int[] ldvt, double[] work,
int[] lwork, int[] iwork, int[] info );
public static native void LAPACK_cgesdd( @Cast("char*") BytePointer jobz, IntPointer m, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer s,
@Cast("lapack_complex_float*") FloatPointer u, IntPointer ldu,
@Cast("lapack_complex_float*") FloatPointer vt, IntPointer ldvt,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_cgesdd( @Cast("char*") ByteBuffer jobz, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer s,
@Cast("lapack_complex_float*") FloatBuffer u, IntBuffer ldu,
@Cast("lapack_complex_float*") FloatBuffer vt, IntBuffer ldvt,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_cgesdd( @Cast("char*") byte[] jobz, int[] m, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda, float[] s,
@Cast("lapack_complex_float*") float[] u, int[] ldu,
@Cast("lapack_complex_float*") float[] vt, int[] ldvt,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] iwork, int[] info );
public static native void LAPACK_zgesdd( @Cast("char*") BytePointer jobz, IntPointer m, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer s,
@Cast("lapack_complex_double*") DoublePointer u, IntPointer ldu,
@Cast("lapack_complex_double*") DoublePointer vt, IntPointer ldvt,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_zgesdd( @Cast("char*") ByteBuffer jobz, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer s,
@Cast("lapack_complex_double*") DoubleBuffer u, IntBuffer ldu,
@Cast("lapack_complex_double*") DoubleBuffer vt, IntBuffer ldvt,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_zgesdd( @Cast("char*") byte[] jobz, int[] m, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda, double[] s,
@Cast("lapack_complex_double*") double[] u, int[] ldu,
@Cast("lapack_complex_double*") double[] vt, int[] ldvt,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] iwork, int[] info );
public static native void LAPACK_dgejsv( @Cast("char*") BytePointer joba, @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobr, @Cast("char*") BytePointer jobt,
@Cast("char*") BytePointer jobp, IntPointer m, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer sva, DoublePointer u, IntPointer ldu,
DoublePointer v, IntPointer ldv, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dgejsv( @Cast("char*") ByteBuffer joba, @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobr, @Cast("char*") ByteBuffer jobt,
@Cast("char*") ByteBuffer jobp, IntBuffer m, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer sva, DoubleBuffer u, IntBuffer ldu,
DoubleBuffer v, IntBuffer ldv, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dgejsv( @Cast("char*") byte[] joba, @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobr, @Cast("char*") byte[] jobt,
@Cast("char*") byte[] jobp, int[] m, int[] n, double[] a,
int[] lda, double[] sva, double[] u, int[] ldu,
double[] v, int[] ldv, double[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_sgejsv( @Cast("char*") BytePointer joba, @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobr, @Cast("char*") BytePointer jobt,
@Cast("char*") BytePointer jobp, IntPointer m, IntPointer n, FloatPointer a,
IntPointer lda, FloatPointer sva, FloatPointer u, IntPointer ldu,
FloatPointer v, IntPointer ldv, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_sgejsv( @Cast("char*") ByteBuffer joba, @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobr, @Cast("char*") ByteBuffer jobt,
@Cast("char*") ByteBuffer jobp, IntBuffer m, IntBuffer n, FloatBuffer a,
IntBuffer lda, FloatBuffer sva, FloatBuffer u, IntBuffer ldu,
FloatBuffer v, IntBuffer ldv, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sgejsv( @Cast("char*") byte[] joba, @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobr, @Cast("char*") byte[] jobt,
@Cast("char*") byte[] jobp, int[] m, int[] n, float[] a,
int[] lda, float[] sva, float[] u, int[] ldu,
float[] v, int[] ldv, float[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_cgejsv( @Cast("char*") BytePointer joba, @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobr, @Cast("char*") BytePointer jobt,
@Cast("char*") BytePointer jobp, IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer sva, @Cast("lapack_complex_float*") FloatPointer u, IntPointer ldu,
@Cast("lapack_complex_float*") FloatPointer v, IntPointer ldv, @Cast("lapack_complex_float*") FloatPointer cwork,
IntPointer lwork, FloatPointer work, IntPointer lrwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_cgejsv( @Cast("char*") ByteBuffer joba, @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobr, @Cast("char*") ByteBuffer jobt,
@Cast("char*") ByteBuffer jobp, IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer sva, @Cast("lapack_complex_float*") FloatBuffer u, IntBuffer ldu,
@Cast("lapack_complex_float*") FloatBuffer v, IntBuffer ldv, @Cast("lapack_complex_float*") FloatBuffer cwork,
IntBuffer lwork, FloatBuffer work, IntBuffer lrwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_cgejsv( @Cast("char*") byte[] joba, @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobr, @Cast("char*") byte[] jobt,
@Cast("char*") byte[] jobp, int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, float[] sva, @Cast("lapack_complex_float*") float[] u, int[] ldu,
@Cast("lapack_complex_float*") float[] v, int[] ldv, @Cast("lapack_complex_float*") float[] cwork,
int[] lwork, float[] work, int[] lrwork,
int[] iwork, int[] info );
public static native void LAPACK_zgejsv( @Cast("char*") BytePointer joba, @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobr, @Cast("char*") BytePointer jobt,
@Cast("char*") BytePointer jobp, IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer sva, @Cast("lapack_complex_double*") DoublePointer u, IntPointer ldu,
@Cast("lapack_complex_double*") DoublePointer v, IntPointer ldv, @Cast("lapack_complex_double*") DoublePointer cwork,
IntPointer lwork, DoublePointer work, IntPointer lrwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_zgejsv( @Cast("char*") ByteBuffer joba, @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobr, @Cast("char*") ByteBuffer jobt,
@Cast("char*") ByteBuffer jobp, IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer sva, @Cast("lapack_complex_double*") DoubleBuffer u, IntBuffer ldu,
@Cast("lapack_complex_double*") DoubleBuffer v, IntBuffer ldv, @Cast("lapack_complex_double*") DoubleBuffer cwork,
IntBuffer lwork, DoubleBuffer work, IntBuffer lrwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_zgejsv( @Cast("char*") byte[] joba, @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobr, @Cast("char*") byte[] jobt,
@Cast("char*") byte[] jobp, int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, double[] sva, @Cast("lapack_complex_double*") double[] u, int[] ldu,
@Cast("lapack_complex_double*") double[] v, int[] ldv, @Cast("lapack_complex_double*") double[] cwork,
int[] lwork, double[] work, int[] lrwork,
int[] iwork, int[] info );
public static native void LAPACK_dgesvj( @Cast("char*") BytePointer joba, @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, IntPointer m,
IntPointer n, DoublePointer a, IntPointer lda, DoublePointer sva,
IntPointer mv, DoublePointer v, IntPointer ldv, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dgesvj( @Cast("char*") ByteBuffer joba, @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, IntBuffer m,
IntBuffer n, DoubleBuffer a, IntBuffer lda, DoubleBuffer sva,
IntBuffer mv, DoubleBuffer v, IntBuffer ldv, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dgesvj( @Cast("char*") byte[] joba, @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, int[] m,
int[] n, double[] a, int[] lda, double[] sva,
int[] mv, double[] v, int[] ldv, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_sgesvj( @Cast("char*") BytePointer joba, @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, IntPointer m,
IntPointer n, FloatPointer a, IntPointer lda, FloatPointer sva,
IntPointer mv, FloatPointer v, IntPointer ldv, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sgesvj( @Cast("char*") ByteBuffer joba, @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, IntBuffer m,
IntBuffer n, FloatBuffer a, IntBuffer lda, FloatBuffer sva,
IntBuffer mv, FloatBuffer v, IntBuffer ldv, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sgesvj( @Cast("char*") byte[] joba, @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, int[] m,
int[] n, float[] a, int[] lda, float[] sva,
int[] mv, float[] v, int[] ldv, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_cgesvj( @Cast("char*") BytePointer joba, @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, IntPointer m,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer sva,
IntPointer mv, @Cast("lapack_complex_float*") FloatPointer v, IntPointer ldv,
@Cast("lapack_complex_float*") FloatPointer cwork, IntPointer lwork, FloatPointer rwork,
IntPointer lrwork, IntPointer info );
public static native void LAPACK_cgesvj( @Cast("char*") ByteBuffer joba, @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, IntBuffer m,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer sva,
IntBuffer mv, @Cast("lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
@Cast("lapack_complex_float*") FloatBuffer cwork, IntBuffer lwork, FloatBuffer rwork,
IntBuffer lrwork, IntBuffer info );
public static native void LAPACK_cgesvj( @Cast("char*") byte[] joba, @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, int[] m,
int[] n, @Cast("lapack_complex_float*") float[] a, int[] lda, float[] sva,
int[] mv, @Cast("lapack_complex_float*") float[] v, int[] ldv,
@Cast("lapack_complex_float*") float[] cwork, int[] lwork, float[] rwork,
int[] lrwork, int[] info );
public static native void LAPACK_zgesvj( @Cast("char*") BytePointer joba, @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, IntPointer m,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer sva,
IntPointer mv, @Cast("lapack_complex_double*") DoublePointer v, IntPointer ldv,
@Cast("lapack_complex_double*") DoublePointer cwork, IntPointer lwork, DoublePointer rwork,
IntPointer lrwork, IntPointer info );
public static native void LAPACK_zgesvj( @Cast("char*") ByteBuffer joba, @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, IntBuffer m,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer sva,
IntBuffer mv, @Cast("lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
@Cast("lapack_complex_double*") DoubleBuffer cwork, IntBuffer lwork, DoubleBuffer rwork,
IntBuffer lrwork, IntBuffer info );
public static native void LAPACK_zgesvj( @Cast("char*") byte[] joba, @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, int[] m,
int[] n, @Cast("lapack_complex_double*") double[] a, int[] lda, double[] sva,
int[] mv, @Cast("lapack_complex_double*") double[] v, int[] ldv,
@Cast("lapack_complex_double*") double[] cwork, int[] lwork, double[] rwork,
int[] lrwork, int[] info );
public static native void LAPACK_sggsvd3( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer n, IntPointer p, IntPointer k, IntPointer l,
FloatPointer a, IntPointer lda, FloatPointer b, IntPointer ldb,
FloatPointer alpha, FloatPointer beta, FloatPointer u, IntPointer ldu,
FloatPointer v, IntPointer ldv, FloatPointer q, IntPointer ldq,
FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer info );
public static native void LAPACK_sggsvd3( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer n, IntBuffer p, IntBuffer k, IntBuffer l,
FloatBuffer a, IntBuffer lda, FloatBuffer b, IntBuffer ldb,
FloatBuffer alpha, FloatBuffer beta, FloatBuffer u, IntBuffer ldu,
FloatBuffer v, IntBuffer ldv, FloatBuffer q, IntBuffer ldq,
FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_sggsvd3( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] n, int[] p, int[] k, int[] l,
float[] a, int[] lda, float[] b, int[] ldb,
float[] alpha, float[] beta, float[] u, int[] ldu,
float[] v, int[] ldv, float[] q, int[] ldq,
float[] work, int[] lwork, int[] iwork,
int[] info );
public static native void LAPACK_dggsvd3( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer n, IntPointer p, IntPointer k, IntPointer l,
DoublePointer a, IntPointer lda, DoublePointer b, IntPointer ldb,
DoublePointer alpha, DoublePointer beta, DoublePointer u, IntPointer ldu,
DoublePointer v, IntPointer ldv, DoublePointer q, IntPointer ldq,
DoublePointer work, IntPointer lwork, IntPointer iwork,
IntPointer info );
public static native void LAPACK_dggsvd3( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer n, IntBuffer p, IntBuffer k, IntBuffer l,
DoubleBuffer a, IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer alpha, DoubleBuffer beta, DoubleBuffer u, IntBuffer ldu,
DoubleBuffer v, IntBuffer ldv, DoubleBuffer q, IntBuffer ldq,
DoubleBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer info );
public static native void LAPACK_dggsvd3( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] n, int[] p, int[] k, int[] l,
double[] a, int[] lda, double[] b, int[] ldb,
double[] alpha, double[] beta, double[] u, int[] ldu,
double[] v, int[] ldv, double[] q, int[] ldq,
double[] work, int[] lwork, int[] iwork,
int[] info );
public static native void LAPACK_cggsvd3( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer n, IntPointer p, IntPointer k, IntPointer l,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, FloatPointer alpha,
FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer u, IntPointer ldu,
@Cast("lapack_complex_float*") FloatPointer v, IntPointer ldv,
@Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_cggsvd3( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer n, IntBuffer p, IntBuffer k, IntBuffer l,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, FloatBuffer alpha,
FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer u, IntBuffer ldu,
@Cast("lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
@Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_cggsvd3( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] n, int[] p, int[] k, int[] l,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, float[] alpha,
float[] beta, @Cast("lapack_complex_float*") float[] u, int[] ldu,
@Cast("lapack_complex_float*") float[] v, int[] ldv,
@Cast("lapack_complex_float*") float[] q, int[] ldq,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] iwork, int[] info );
public static native void LAPACK_zggsvd3( @Cast("char*") BytePointer jobu, @Cast("char*") BytePointer jobv, @Cast("char*") BytePointer jobq, IntPointer m,
IntPointer n, IntPointer p, IntPointer k, IntPointer l,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, DoublePointer alpha,
DoublePointer beta, @Cast("lapack_complex_double*") DoublePointer u, IntPointer ldu,
@Cast("lapack_complex_double*") DoublePointer v, IntPointer ldv,
@Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer iwork, IntPointer info );
public static native void LAPACK_zggsvd3( @Cast("char*") ByteBuffer jobu, @Cast("char*") ByteBuffer jobv, @Cast("char*") ByteBuffer jobq, IntBuffer m,
IntBuffer n, IntBuffer p, IntBuffer k, IntBuffer l,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, DoubleBuffer alpha,
DoubleBuffer beta, @Cast("lapack_complex_double*") DoubleBuffer u, IntBuffer ldu,
@Cast("lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
@Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer iwork, IntBuffer info );
public static native void LAPACK_zggsvd3( @Cast("char*") byte[] jobu, @Cast("char*") byte[] jobv, @Cast("char*") byte[] jobq, int[] m,
int[] n, int[] p, int[] k, int[] l,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, double[] alpha,
double[] beta, @Cast("lapack_complex_double*") double[] u, int[] ldu,
@Cast("lapack_complex_double*") double[] v, int[] ldv,
@Cast("lapack_complex_double*") double[] q, int[] ldq,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] iwork, int[] info );
public static native void LAPACK_ssygv( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer a, IntPointer lda, FloatPointer b, IntPointer ldb,
FloatPointer w, FloatPointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_ssygv( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer a, IntBuffer lda, FloatBuffer b, IntBuffer ldb,
FloatBuffer w, FloatBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_ssygv( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
float[] a, int[] lda, float[] b, int[] ldb,
float[] w, float[] work, int[] lwork, int[] info );
public static native void LAPACK_dsygv( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer a, IntPointer lda, DoublePointer b, IntPointer ldb,
DoublePointer w, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dsygv( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer a, IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer w, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dsygv( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
double[] a, int[] lda, double[] b, int[] ldb,
double[] w, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_chegv( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_chegv( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_chegv( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, float[] w,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zhegv( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zhegv( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zhegv( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, double[] w,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_ssygvd( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer a, IntPointer lda, FloatPointer b, IntPointer ldb,
FloatPointer w, FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_ssygvd( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer a, IntBuffer lda, FloatBuffer b, IntBuffer ldb,
FloatBuffer w, FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_ssygvd( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
float[] a, int[] lda, float[] b, int[] ldb,
float[] w, float[] work, int[] lwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_dsygvd( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer a, IntPointer lda, DoublePointer b, IntPointer ldb,
DoublePointer w, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dsygvd( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer a, IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer w, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dsygvd( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
double[] a, int[] lda, double[] b, int[] ldb,
double[] w, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_chegvd( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer lrwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_chegvd( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer lrwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_chegvd( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, float[] w,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] lrwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_zhegvd( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_zhegvd( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_zhegvd( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, double[] w,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_ssygvx( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo,
IntPointer n, FloatPointer a, IntPointer lda, FloatPointer b,
IntPointer ldb, FloatPointer vl, FloatPointer vu, IntPointer il,
IntPointer iu, FloatPointer abstol, IntPointer m, FloatPointer w,
FloatPointer z, IntPointer ldz, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer ifail, IntPointer info );
public static native void LAPACK_ssygvx( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo,
IntBuffer n, FloatBuffer a, IntBuffer lda, FloatBuffer b,
IntBuffer ldb, FloatBuffer vl, FloatBuffer vu, IntBuffer il,
IntBuffer iu, FloatBuffer abstol, IntBuffer m, FloatBuffer w,
FloatBuffer z, IntBuffer ldz, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer ifail, IntBuffer info );
public static native void LAPACK_ssygvx( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo,
int[] n, float[] a, int[] lda, float[] b,
int[] ldb, float[] vl, float[] vu, int[] il,
int[] iu, float[] abstol, int[] m, float[] w,
float[] z, int[] ldz, float[] work, int[] lwork,
int[] iwork, int[] ifail, int[] info );
public static native void LAPACK_dsygvx( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo,
IntPointer n, DoublePointer a, IntPointer lda, DoublePointer b,
IntPointer ldb, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, DoublePointer abstol, IntPointer m, DoublePointer w,
DoublePointer z, IntPointer ldz, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer ifail, IntPointer info );
public static native void LAPACK_dsygvx( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo,
IntBuffer n, DoubleBuffer a, IntBuffer lda, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, DoubleBuffer abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, IntBuffer ldz, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer ifail, IntBuffer info );
public static native void LAPACK_dsygvx( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo,
int[] n, double[] a, int[] lda, double[] b,
int[] ldb, double[] vl, double[] vu, int[] il,
int[] iu, double[] abstol, int[] m, double[] w,
double[] z, int[] ldz, double[] work, int[] lwork,
int[] iwork, int[] ifail, int[] info );
public static native void LAPACK_chegvx( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, FloatPointer vl,
FloatPointer vu, IntPointer il, IntPointer iu, FloatPointer abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, FloatPointer rwork, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_chegvx( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, FloatBuffer vl,
FloatBuffer vu, IntBuffer il, IntBuffer iu, FloatBuffer abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, FloatBuffer rwork, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_chegvx( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo,
int[] n, @Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, float[] vl,
float[] vu, int[] il, int[] iu, float[] abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, @Cast("lapack_complex_float*") float[] work,
int[] lwork, float[] rwork, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_zhegvx( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, DoublePointer vl,
DoublePointer vu, IntPointer il, IntPointer iu, DoublePointer abstol,
IntPointer m, DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
IntPointer ldz, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, DoublePointer rwork, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_zhegvx( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, DoubleBuffer vl,
DoubleBuffer vu, IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
IntBuffer m, DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
IntBuffer ldz, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, DoubleBuffer rwork, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_zhegvx( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo,
int[] n, @Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, double[] vl,
double[] vu, int[] il, int[] iu, double[] abstol,
int[] m, double[] w, @Cast("lapack_complex_double*") double[] z,
int[] ldz, @Cast("lapack_complex_double*") double[] work,
int[] lwork, double[] rwork, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_sspgv( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer ap, FloatPointer bp, FloatPointer w, FloatPointer z, IntPointer ldz,
FloatPointer work, IntPointer info );
public static native void LAPACK_sspgv( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer ap, FloatBuffer bp, FloatBuffer w, FloatBuffer z, IntBuffer ldz,
FloatBuffer work, IntBuffer info );
public static native void LAPACK_sspgv( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
float[] ap, float[] bp, float[] w, float[] z, int[] ldz,
float[] work, int[] info );
public static native void LAPACK_dspgv( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer ap, DoublePointer bp, DoublePointer w, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer info );
public static native void LAPACK_dspgv( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer ap, DoubleBuffer bp, DoubleBuffer w, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dspgv( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
double[] ap, double[] bp, double[] w, double[] z,
int[] ldz, double[] work, int[] info );
public static native void LAPACK_chpgv( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer ap, @Cast("lapack_complex_float*") FloatPointer bp, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork, IntPointer info );
public static native void LAPACK_chpgv( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer ap, @Cast("lapack_complex_float*") FloatBuffer bp, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_chpgv( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] ap, @Cast("lapack_complex_float*") float[] bp, float[] w,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, float[] rwork, int[] info );
public static native void LAPACK_zhpgv( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer ap, @Cast("lapack_complex_double*") DoublePointer bp,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zhpgv( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer ap, @Cast("lapack_complex_double*") DoubleBuffer bp,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zhpgv( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] ap, @Cast("lapack_complex_double*") double[] bp,
double[] w, @Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_sspgvd( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer ap, FloatPointer bp, FloatPointer w, FloatPointer z, IntPointer ldz,
FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_sspgvd( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer ap, FloatBuffer bp, FloatBuffer w, FloatBuffer z, IntBuffer ldz,
FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_sspgvd( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
float[] ap, float[] bp, float[] w, float[] z, int[] ldz,
float[] work, int[] lwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_dspgvd( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer ap, DoublePointer bp, DoublePointer w, DoublePointer z,
IntPointer ldz, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer liwork, IntPointer info );
public static native void LAPACK_dspgvd( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer ap, DoubleBuffer bp, DoubleBuffer w, DoubleBuffer z,
IntBuffer ldz, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dspgvd( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
double[] ap, double[] bp, double[] w, double[] z,
int[] ldz, double[] work, int[] lwork,
int[] iwork, int[] liwork, int[] info );
public static native void LAPACK_chpgvd( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer ap, @Cast("lapack_complex_float*") FloatPointer bp,
FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer lrwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_chpgvd( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer ap, @Cast("lapack_complex_float*") FloatBuffer bp,
FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer lrwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_chpgvd( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] ap, @Cast("lapack_complex_float*") float[] bp,
float[] w, @Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] lrwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_zhpgvd( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer ap, @Cast("lapack_complex_double*") DoublePointer bp,
DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_zhpgvd( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer ap, @Cast("lapack_complex_double*") DoubleBuffer bp,
DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_zhpgvd( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] ap, @Cast("lapack_complex_double*") double[] bp,
double[] w, @Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_sspgvx( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo,
IntPointer n, FloatPointer ap, FloatPointer bp, FloatPointer vl, FloatPointer vu,
IntPointer il, IntPointer iu, FloatPointer abstol,
IntPointer m, FloatPointer w, FloatPointer z, IntPointer ldz,
FloatPointer work, IntPointer iwork, IntPointer ifail,
IntPointer info );
public static native void LAPACK_sspgvx( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo,
IntBuffer n, FloatBuffer ap, FloatBuffer bp, FloatBuffer vl, FloatBuffer vu,
IntBuffer il, IntBuffer iu, FloatBuffer abstol,
IntBuffer m, FloatBuffer w, FloatBuffer z, IntBuffer ldz,
FloatBuffer work, IntBuffer iwork, IntBuffer ifail,
IntBuffer info );
public static native void LAPACK_sspgvx( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo,
int[] n, float[] ap, float[] bp, float[] vl, float[] vu,
int[] il, int[] iu, float[] abstol,
int[] m, float[] w, float[] z, int[] ldz,
float[] work, int[] iwork, int[] ifail,
int[] info );
public static native void LAPACK_dspgvx( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo,
IntPointer n, DoublePointer ap, DoublePointer bp, DoublePointer vl,
DoublePointer vu, IntPointer il, IntPointer iu, DoublePointer abstol,
IntPointer m, DoublePointer w, DoublePointer z, IntPointer ldz,
DoublePointer work, IntPointer iwork, IntPointer ifail,
IntPointer info );
public static native void LAPACK_dspgvx( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo,
IntBuffer n, DoubleBuffer ap, DoubleBuffer bp, DoubleBuffer vl,
DoubleBuffer vu, IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
IntBuffer m, DoubleBuffer w, DoubleBuffer z, IntBuffer ldz,
DoubleBuffer work, IntBuffer iwork, IntBuffer ifail,
IntBuffer info );
public static native void LAPACK_dspgvx( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo,
int[] n, double[] ap, double[] bp, double[] vl,
double[] vu, int[] il, int[] iu, double[] abstol,
int[] m, double[] w, double[] z, int[] ldz,
double[] work, int[] iwork, int[] ifail,
int[] info );
public static native void LAPACK_chpgvx( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer bp, FloatPointer vl, FloatPointer vu,
IntPointer il, IntPointer iu, FloatPointer abstol,
IntPointer m, FloatPointer w, @Cast("lapack_complex_float*") FloatPointer z,
IntPointer ldz, @Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork,
IntPointer iwork, IntPointer ifail, IntPointer info );
public static native void LAPACK_chpgvx( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer bp, FloatBuffer vl, FloatBuffer vu,
IntBuffer il, IntBuffer iu, FloatBuffer abstol,
IntBuffer m, FloatBuffer w, @Cast("lapack_complex_float*") FloatBuffer z,
IntBuffer ldz, @Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork,
IntBuffer iwork, IntBuffer ifail, IntBuffer info );
public static native void LAPACK_chpgvx( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo,
int[] n, @Cast("lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] bp, float[] vl, float[] vu,
int[] il, int[] iu, float[] abstol,
int[] m, float[] w, @Cast("lapack_complex_float*") float[] z,
int[] ldz, @Cast("lapack_complex_float*") float[] work, float[] rwork,
int[] iwork, int[] ifail, int[] info );
public static native void LAPACK_zhpgvx( IntPointer itype, @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer bp, DoublePointer vl, DoublePointer vu,
IntPointer il, IntPointer iu, DoublePointer abstol,
IntPointer m, DoublePointer w, @Cast("lapack_complex_double*") DoublePointer z,
IntPointer ldz, @Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer iwork, IntPointer ifail, IntPointer info );
public static native void LAPACK_zhpgvx( IntBuffer itype, @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer bp, DoubleBuffer vl, DoubleBuffer vu,
IntBuffer il, IntBuffer iu, DoubleBuffer abstol,
IntBuffer m, DoubleBuffer w, @Cast("lapack_complex_double*") DoubleBuffer z,
IntBuffer ldz, @Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer iwork, IntBuffer ifail, IntBuffer info );
public static native void LAPACK_zhpgvx( int[] itype, @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo,
int[] n, @Cast("lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] bp, double[] vl, double[] vu,
int[] il, int[] iu, double[] abstol,
int[] m, double[] w, @Cast("lapack_complex_double*") double[] z,
int[] ldz, @Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] iwork, int[] ifail, int[] info );
public static native void LAPACK_ssbgv( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, FloatPointer ab, IntPointer ldab, FloatPointer bb,
IntPointer ldbb, FloatPointer w, FloatPointer z, IntPointer ldz,
FloatPointer work, IntPointer info );
public static native void LAPACK_ssbgv( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, FloatBuffer ab, IntBuffer ldab, FloatBuffer bb,
IntBuffer ldbb, FloatBuffer w, FloatBuffer z, IntBuffer ldz,
FloatBuffer work, IntBuffer info );
public static native void LAPACK_ssbgv( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, float[] ab, int[] ldab, float[] bb,
int[] ldbb, float[] w, float[] z, int[] ldz,
float[] work, int[] info );
public static native void LAPACK_dsbgv( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, DoublePointer ab, IntPointer ldab, DoublePointer bb,
IntPointer ldbb, DoublePointer w, DoublePointer z, IntPointer ldz,
DoublePointer work, IntPointer info );
public static native void LAPACK_dsbgv( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, DoubleBuffer ab, IntBuffer ldab, DoubleBuffer bb,
IntBuffer ldbb, DoubleBuffer w, DoubleBuffer z, IntBuffer ldz,
DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dsbgv( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, double[] ab, int[] ldab, double[] bb,
int[] ldbb, double[] w, double[] z, int[] ldz,
double[] work, int[] info );
public static native void LAPACK_chbgv( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, @Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Cast("lapack_complex_float*") FloatPointer bb, IntPointer ldbb, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork, IntPointer info );
public static native void LAPACK_chbgv( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, @Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_float*") FloatBuffer bb, IntBuffer ldbb, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork, IntBuffer info );
public static native void LAPACK_chbgv( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, @Cast("lapack_complex_float*") float[] ab, int[] ldab,
@Cast("lapack_complex_float*") float[] bb, int[] ldbb, float[] w,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, float[] rwork, int[] info );
public static native void LAPACK_zhbgv( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, @Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Cast("lapack_complex_double*") DoublePointer bb, IntPointer ldbb, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer info );
public static native void LAPACK_zhbgv( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, @Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_double*") DoubleBuffer bb, IntBuffer ldbb, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer info );
public static native void LAPACK_zhbgv( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, @Cast("lapack_complex_double*") double[] ab, int[] ldab,
@Cast("lapack_complex_double*") double[] bb, int[] ldbb, double[] w,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] info );
public static native void LAPACK_ssbgvd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, FloatPointer ab, IntPointer ldab, FloatPointer bb,
IntPointer ldbb, FloatPointer w, FloatPointer z, IntPointer ldz,
FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_ssbgvd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, FloatBuffer ab, IntBuffer ldab, FloatBuffer bb,
IntBuffer ldbb, FloatBuffer w, FloatBuffer z, IntBuffer ldz,
FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_ssbgvd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, float[] ab, int[] ldab, float[] bb,
int[] ldbb, float[] w, float[] z, int[] ldz,
float[] work, int[] lwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_dsbgvd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, DoublePointer ab, IntPointer ldab, DoublePointer bb,
IntPointer ldbb, DoublePointer w, DoublePointer z, IntPointer ldz,
DoublePointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_dsbgvd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, DoubleBuffer ab, IntBuffer ldab, DoubleBuffer bb,
IntBuffer ldbb, DoubleBuffer w, DoubleBuffer z, IntBuffer ldz,
DoubleBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_dsbgvd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, double[] ab, int[] ldab, double[] bb,
int[] ldbb, double[] w, double[] z, int[] ldz,
double[] work, int[] lwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_chbgvd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, @Cast("lapack_complex_float*") FloatPointer ab, IntPointer ldab,
@Cast("lapack_complex_float*") FloatPointer bb, IntPointer ldbb, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer lrwork, IntPointer iwork, IntPointer liwork,
IntPointer info );
public static native void LAPACK_chbgvd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, @Cast("lapack_complex_float*") FloatBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_float*") FloatBuffer bb, IntBuffer ldbb, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer lrwork, IntBuffer iwork, IntBuffer liwork,
IntBuffer info );
public static native void LAPACK_chbgvd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, @Cast("lapack_complex_float*") float[] ab, int[] ldab,
@Cast("lapack_complex_float*") float[] bb, int[] ldbb, float[] w,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] lrwork, int[] iwork, int[] liwork,
int[] info );
public static native void LAPACK_zhbgvd( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer uplo, IntPointer n, IntPointer ka,
IntPointer kb, @Cast("lapack_complex_double*") DoublePointer ab, IntPointer ldab,
@Cast("lapack_complex_double*") DoublePointer bb, IntPointer ldbb, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork, IntPointer iwork,
IntPointer liwork, IntPointer info );
public static native void LAPACK_zhbgvd( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer ka,
IntBuffer kb, @Cast("lapack_complex_double*") DoubleBuffer ab, IntBuffer ldab,
@Cast("lapack_complex_double*") DoubleBuffer bb, IntBuffer ldbb, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer info );
public static native void LAPACK_zhbgvd( @Cast("char*") byte[] jobz, @Cast("char*") byte[] uplo, int[] n, int[] ka,
int[] kb, @Cast("lapack_complex_double*") double[] ab, int[] ldab,
@Cast("lapack_complex_double*") double[] bb, int[] ldbb, double[] w,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork, int[] iwork,
int[] liwork, int[] info );
public static native void LAPACK_ssbgvx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer ka, IntPointer kb, FloatPointer ab, IntPointer ldab,
FloatPointer bb, IntPointer ldbb, FloatPointer q, IntPointer ldq,
FloatPointer vl, FloatPointer vu, IntPointer il, IntPointer iu,
FloatPointer abstol, IntPointer m, FloatPointer w, FloatPointer z,
IntPointer ldz, FloatPointer work, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_ssbgvx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer ka, IntBuffer kb, FloatBuffer ab, IntBuffer ldab,
FloatBuffer bb, IntBuffer ldbb, FloatBuffer q, IntBuffer ldq,
FloatBuffer vl, FloatBuffer vu, IntBuffer il, IntBuffer iu,
FloatBuffer abstol, IntBuffer m, FloatBuffer w, FloatBuffer z,
IntBuffer ldz, FloatBuffer work, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_ssbgvx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
int[] ka, int[] kb, float[] ab, int[] ldab,
float[] bb, int[] ldbb, float[] q, int[] ldq,
float[] vl, float[] vu, int[] il, int[] iu,
float[] abstol, int[] m, float[] w, float[] z,
int[] ldz, float[] work, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_dsbgvx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer ka, IntPointer kb, DoublePointer ab,
IntPointer ldab, DoublePointer bb, IntPointer ldbb, DoublePointer q,
IntPointer ldq, DoublePointer vl, DoublePointer vu, IntPointer il,
IntPointer iu, DoublePointer abstol, IntPointer m, DoublePointer w,
DoublePointer z, IntPointer ldz, DoublePointer work, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_dsbgvx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer ka, IntBuffer kb, DoubleBuffer ab,
IntBuffer ldab, DoubleBuffer bb, IntBuffer ldbb, DoubleBuffer q,
IntBuffer ldq, DoubleBuffer vl, DoubleBuffer vu, IntBuffer il,
IntBuffer iu, DoubleBuffer abstol, IntBuffer m, DoubleBuffer w,
DoubleBuffer z, IntBuffer ldz, DoubleBuffer work, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_dsbgvx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
int[] ka, int[] kb, double[] ab,
int[] ldab, double[] bb, int[] ldbb, double[] q,
int[] ldq, double[] vl, double[] vu, int[] il,
int[] iu, double[] abstol, int[] m, double[] w,
double[] z, int[] ldz, double[] work, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_chbgvx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer ka, IntPointer kb, @Cast("lapack_complex_float*") FloatPointer ab,
IntPointer ldab, @Cast("lapack_complex_float*") FloatPointer bb,
IntPointer ldbb, @Cast("lapack_complex_float*") FloatPointer q, IntPointer ldq,
FloatPointer vl, FloatPointer vu, IntPointer il, IntPointer iu,
FloatPointer abstol, IntPointer m, FloatPointer w,
@Cast("lapack_complex_float*") FloatPointer z, IntPointer ldz,
@Cast("lapack_complex_float*") FloatPointer work, FloatPointer rwork, IntPointer iwork,
IntPointer ifail, IntPointer info );
public static native void LAPACK_chbgvx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer ka, IntBuffer kb, @Cast("lapack_complex_float*") FloatBuffer ab,
IntBuffer ldab, @Cast("lapack_complex_float*") FloatBuffer bb,
IntBuffer ldbb, @Cast("lapack_complex_float*") FloatBuffer q, IntBuffer ldq,
FloatBuffer vl, FloatBuffer vu, IntBuffer il, IntBuffer iu,
FloatBuffer abstol, IntBuffer m, FloatBuffer w,
@Cast("lapack_complex_float*") FloatBuffer z, IntBuffer ldz,
@Cast("lapack_complex_float*") FloatBuffer work, FloatBuffer rwork, IntBuffer iwork,
IntBuffer ifail, IntBuffer info );
public static native void LAPACK_chbgvx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
int[] ka, int[] kb, @Cast("lapack_complex_float*") float[] ab,
int[] ldab, @Cast("lapack_complex_float*") float[] bb,
int[] ldbb, @Cast("lapack_complex_float*") float[] q, int[] ldq,
float[] vl, float[] vu, int[] il, int[] iu,
float[] abstol, int[] m, float[] w,
@Cast("lapack_complex_float*") float[] z, int[] ldz,
@Cast("lapack_complex_float*") float[] work, float[] rwork, int[] iwork,
int[] ifail, int[] info );
public static native void LAPACK_zhbgvx( @Cast("char*") BytePointer jobz, @Cast("char*") BytePointer range, @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer ka, IntPointer kb, @Cast("lapack_complex_double*") DoublePointer ab,
IntPointer ldab, @Cast("lapack_complex_double*") DoublePointer bb,
IntPointer ldbb, @Cast("lapack_complex_double*") DoublePointer q, IntPointer ldq,
DoublePointer vl, DoublePointer vu, IntPointer il, IntPointer iu,
DoublePointer abstol, IntPointer m, DoublePointer w,
@Cast("lapack_complex_double*") DoublePointer z, IntPointer ldz,
@Cast("lapack_complex_double*") DoublePointer work, DoublePointer rwork,
IntPointer iwork, IntPointer ifail, IntPointer info );
public static native void LAPACK_zhbgvx( @Cast("char*") ByteBuffer jobz, @Cast("char*") ByteBuffer range, @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer ka, IntBuffer kb, @Cast("lapack_complex_double*") DoubleBuffer ab,
IntBuffer ldab, @Cast("lapack_complex_double*") DoubleBuffer bb,
IntBuffer ldbb, @Cast("lapack_complex_double*") DoubleBuffer q, IntBuffer ldq,
DoubleBuffer vl, DoubleBuffer vu, IntBuffer il, IntBuffer iu,
DoubleBuffer abstol, IntBuffer m, DoubleBuffer w,
@Cast("lapack_complex_double*") DoubleBuffer z, IntBuffer ldz,
@Cast("lapack_complex_double*") DoubleBuffer work, DoubleBuffer rwork,
IntBuffer iwork, IntBuffer ifail, IntBuffer info );
public static native void LAPACK_zhbgvx( @Cast("char*") byte[] jobz, @Cast("char*") byte[] range, @Cast("char*") byte[] uplo, int[] n,
int[] ka, int[] kb, @Cast("lapack_complex_double*") double[] ab,
int[] ldab, @Cast("lapack_complex_double*") double[] bb,
int[] ldbb, @Cast("lapack_complex_double*") double[] q, int[] ldq,
double[] vl, double[] vu, int[] il, int[] iu,
double[] abstol, int[] m, double[] w,
@Cast("lapack_complex_double*") double[] z, int[] ldz,
@Cast("lapack_complex_double*") double[] work, double[] rwork,
int[] iwork, int[] ifail, int[] info );
public static native void LAPACK_sgges( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_S_SELECT3 selctg, IntPointer n, FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb, IntPointer sdim,
FloatPointer alphar, FloatPointer alphai, FloatPointer beta, FloatPointer vsl,
IntPointer ldvsl, FloatPointer vsr, IntPointer ldvsr,
FloatPointer work, IntPointer lwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_sgges( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_S_SELECT3 selctg, IntBuffer n, FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb, IntBuffer sdim,
FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta, FloatBuffer vsl,
IntBuffer ldvsl, FloatBuffer vsr, IntBuffer ldvsr,
FloatBuffer work, IntBuffer lwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_sgges( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_S_SELECT3 selctg, int[] n, float[] a,
int[] lda, float[] b, int[] ldb, int[] sdim,
float[] alphar, float[] alphai, float[] beta, float[] vsl,
int[] ldvsl, float[] vsr, int[] ldvsr,
float[] work, int[] lwork, int[] bwork,
int[] info );
public static native void LAPACK_dgges( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_D_SELECT3 selctg, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb,
IntPointer sdim, DoublePointer alphar, DoublePointer alphai,
DoublePointer beta, DoublePointer vsl, IntPointer ldvsl, DoublePointer vsr,
IntPointer ldvsr, DoublePointer work, IntPointer lwork,
IntPointer bwork, IntPointer info );
public static native void LAPACK_dgges( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_D_SELECT3 selctg, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
IntBuffer sdim, DoubleBuffer alphar, DoubleBuffer alphai,
DoubleBuffer beta, DoubleBuffer vsl, IntBuffer ldvsl, DoubleBuffer vsr,
IntBuffer ldvsr, DoubleBuffer work, IntBuffer lwork,
IntBuffer bwork, IntBuffer info );
public static native void LAPACK_dgges( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_D_SELECT3 selctg, int[] n, double[] a,
int[] lda, double[] b, int[] ldb,
int[] sdim, double[] alphar, double[] alphai,
double[] beta, double[] vsl, int[] ldvsl, double[] vsr,
int[] ldvsr, double[] work, int[] lwork,
int[] bwork, int[] info );
public static native void LAPACK_cgges( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_C_SELECT2 selctg, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, IntPointer sdim,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vsl, IntPointer ldvsl,
@Cast("lapack_complex_float*") FloatPointer vsr, IntPointer ldvsr,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer bwork, IntPointer info );
public static native void LAPACK_cgges( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_C_SELECT2 selctg, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, IntBuffer sdim,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vsl, IntBuffer ldvsl,
@Cast("lapack_complex_float*") FloatBuffer vsr, IntBuffer ldvsr,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer bwork, IntBuffer info );
public static native void LAPACK_cgges( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_C_SELECT2 selctg, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, int[] sdim,
@Cast("lapack_complex_float*") float[] alpha, @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vsl, int[] ldvsl,
@Cast("lapack_complex_float*") float[] vsr, int[] ldvsr,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] bwork, int[] info );
public static native void LAPACK_zgges( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_Z_SELECT2 selctg, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, IntPointer sdim,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vsl, IntPointer ldvsl,
@Cast("lapack_complex_double*") DoublePointer vsr, IntPointer ldvsr,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer bwork, IntPointer info );
public static native void LAPACK_zgges( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_Z_SELECT2 selctg, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, IntBuffer sdim,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vsl, IntBuffer ldvsl,
@Cast("lapack_complex_double*") DoubleBuffer vsr, IntBuffer ldvsr,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer bwork, IntBuffer info );
public static native void LAPACK_zgges( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_Z_SELECT2 selctg, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, int[] sdim,
@Cast("lapack_complex_double*") double[] alpha, @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vsl, int[] ldvsl,
@Cast("lapack_complex_double*") double[] vsr, int[] ldvsr,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] bwork, int[] info );
public static native void LAPACK_sgges3( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_S_SELECT3 selctg, IntPointer n,
FloatPointer a, IntPointer lda, FloatPointer b, IntPointer ldb,
IntPointer sdim, FloatPointer alphar, FloatPointer alphai,
FloatPointer beta, FloatPointer vsl, IntPointer ldvsl,
FloatPointer vsr, IntPointer ldvsr,
FloatPointer work, IntPointer lwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_sgges3( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_S_SELECT3 selctg, IntBuffer n,
FloatBuffer a, IntBuffer lda, FloatBuffer b, IntBuffer ldb,
IntBuffer sdim, FloatBuffer alphar, FloatBuffer alphai,
FloatBuffer beta, FloatBuffer vsl, IntBuffer ldvsl,
FloatBuffer vsr, IntBuffer ldvsr,
FloatBuffer work, IntBuffer lwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_sgges3( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_S_SELECT3 selctg, int[] n,
float[] a, int[] lda, float[] b, int[] ldb,
int[] sdim, float[] alphar, float[] alphai,
float[] beta, float[] vsl, int[] ldvsl,
float[] vsr, int[] ldvsr,
float[] work, int[] lwork, int[] bwork,
int[] info );
public static native void LAPACK_dgges3( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_D_SELECT3 selctg, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb,
IntPointer sdim, DoublePointer alphar, DoublePointer alphai,
DoublePointer beta, DoublePointer vsl, IntPointer ldvsl, DoublePointer vsr,
IntPointer ldvsr, DoublePointer work, IntPointer lwork,
IntPointer bwork, IntPointer info );
public static native void LAPACK_dgges3( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_D_SELECT3 selctg, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
IntBuffer sdim, DoubleBuffer alphar, DoubleBuffer alphai,
DoubleBuffer beta, DoubleBuffer vsl, IntBuffer ldvsl, DoubleBuffer vsr,
IntBuffer ldvsr, DoubleBuffer work, IntBuffer lwork,
IntBuffer bwork, IntBuffer info );
public static native void LAPACK_dgges3( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_D_SELECT3 selctg, int[] n, double[] a,
int[] lda, double[] b, int[] ldb,
int[] sdim, double[] alphar, double[] alphai,
double[] beta, double[] vsl, int[] ldvsl, double[] vsr,
int[] ldvsr, double[] work, int[] lwork,
int[] bwork, int[] info );
public static native void LAPACK_cgges3( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_C_SELECT2 selctg, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
IntPointer sdim,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vsl, IntPointer ldvsl,
@Cast("lapack_complex_float*") FloatPointer vsr, IntPointer ldvsr,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer bwork, IntPointer info );
public static native void LAPACK_cgges3( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_C_SELECT2 selctg, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
IntBuffer sdim,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vsl, IntBuffer ldvsl,
@Cast("lapack_complex_float*") FloatBuffer vsr, IntBuffer ldvsr,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer bwork, IntBuffer info );
public static native void LAPACK_cgges3( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_C_SELECT2 selctg, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
int[] sdim,
@Cast("lapack_complex_float*") float[] alpha, @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vsl, int[] ldvsl,
@Cast("lapack_complex_float*") float[] vsr, int[] ldvsr,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] bwork, int[] info );
public static native void LAPACK_zgges3( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_Z_SELECT2 selctg, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, IntPointer sdim,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vsl, IntPointer ldvsl,
@Cast("lapack_complex_double*") DoublePointer vsr, IntPointer ldvsr,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer bwork, IntPointer info );
public static native void LAPACK_zgges3( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_Z_SELECT2 selctg, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, IntBuffer sdim,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vsl, IntBuffer ldvsl,
@Cast("lapack_complex_double*") DoubleBuffer vsr, IntBuffer ldvsr,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer bwork, IntBuffer info );
public static native void LAPACK_zgges3( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_Z_SELECT2 selctg, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, int[] sdim,
@Cast("lapack_complex_double*") double[] alpha, @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vsl, int[] ldvsl,
@Cast("lapack_complex_double*") double[] vsr, int[] ldvsr,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] bwork, int[] info );
public static native void LAPACK_sggesx( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_S_SELECT3 selctg, @Cast("char*") BytePointer sense, IntPointer n,
FloatPointer a, IntPointer lda, FloatPointer b, IntPointer ldb,
IntPointer sdim, FloatPointer alphar, FloatPointer alphai, FloatPointer beta,
FloatPointer vsl, IntPointer ldvsl, FloatPointer vsr,
IntPointer ldvsr, FloatPointer rconde, FloatPointer rcondv,
FloatPointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_sggesx( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_S_SELECT3 selctg, @Cast("char*") ByteBuffer sense, IntBuffer n,
FloatBuffer a, IntBuffer lda, FloatBuffer b, IntBuffer ldb,
IntBuffer sdim, FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta,
FloatBuffer vsl, IntBuffer ldvsl, FloatBuffer vsr,
IntBuffer ldvsr, FloatBuffer rconde, FloatBuffer rcondv,
FloatBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_sggesx( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_S_SELECT3 selctg, @Cast("char*") byte[] sense, int[] n,
float[] a, int[] lda, float[] b, int[] ldb,
int[] sdim, float[] alphar, float[] alphai, float[] beta,
float[] vsl, int[] ldvsl, float[] vsr,
int[] ldvsr, float[] rconde, float[] rcondv,
float[] work, int[] lwork, int[] iwork,
int[] liwork, int[] bwork,
int[] info );
public static native void LAPACK_dggesx( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_D_SELECT3 selctg, @Cast("char*") BytePointer sense, IntPointer n,
DoublePointer a, IntPointer lda, DoublePointer b, IntPointer ldb,
IntPointer sdim, DoublePointer alphar, DoublePointer alphai,
DoublePointer beta, DoublePointer vsl, IntPointer ldvsl, DoublePointer vsr,
IntPointer ldvsr, DoublePointer rconde, DoublePointer rcondv,
DoublePointer work, IntPointer lwork, IntPointer iwork,
IntPointer liwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_dggesx( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_D_SELECT3 selctg, @Cast("char*") ByteBuffer sense, IntBuffer n,
DoubleBuffer a, IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
IntBuffer sdim, DoubleBuffer alphar, DoubleBuffer alphai,
DoubleBuffer beta, DoubleBuffer vsl, IntBuffer ldvsl, DoubleBuffer vsr,
IntBuffer ldvsr, DoubleBuffer rconde, DoubleBuffer rcondv,
DoubleBuffer work, IntBuffer lwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_dggesx( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_D_SELECT3 selctg, @Cast("char*") byte[] sense, int[] n,
double[] a, int[] lda, double[] b, int[] ldb,
int[] sdim, double[] alphar, double[] alphai,
double[] beta, double[] vsl, int[] ldvsl, double[] vsr,
int[] ldvsr, double[] rconde, double[] rcondv,
double[] work, int[] lwork, int[] iwork,
int[] liwork, int[] bwork,
int[] info );
public static native void LAPACK_cggesx( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_C_SELECT2 selctg, @Cast("char*") BytePointer sense, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb, IntPointer sdim,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vsl, IntPointer ldvsl,
@Cast("lapack_complex_float*") FloatPointer vsr, IntPointer ldvsr, FloatPointer rconde,
FloatPointer rcondv, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, FloatPointer rwork, IntPointer iwork,
IntPointer liwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_cggesx( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_C_SELECT2 selctg, @Cast("char*") ByteBuffer sense, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb, IntBuffer sdim,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vsl, IntBuffer ldvsl,
@Cast("lapack_complex_float*") FloatBuffer vsr, IntBuffer ldvsr, FloatBuffer rconde,
FloatBuffer rcondv, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, FloatBuffer rwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_cggesx( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_C_SELECT2 selctg, @Cast("char*") byte[] sense, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb, int[] sdim,
@Cast("lapack_complex_float*") float[] alpha, @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vsl, int[] ldvsl,
@Cast("lapack_complex_float*") float[] vsr, int[] ldvsr, float[] rconde,
float[] rcondv, @Cast("lapack_complex_float*") float[] work,
int[] lwork, float[] rwork, int[] iwork,
int[] liwork, int[] bwork,
int[] info );
public static native void LAPACK_zggesx( @Cast("char*") BytePointer jobvsl, @Cast("char*") BytePointer jobvsr, @Cast("char*") BytePointer sort,
LAPACK_Z_SELECT2 selctg, @Cast("char*") BytePointer sense, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb, IntPointer sdim,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vsl, IntPointer ldvsl,
@Cast("lapack_complex_double*") DoublePointer vsr, IntPointer ldvsr,
DoublePointer rconde, DoublePointer rcondv, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, DoublePointer rwork, IntPointer iwork,
IntPointer liwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_zggesx( @Cast("char*") ByteBuffer jobvsl, @Cast("char*") ByteBuffer jobvsr, @Cast("char*") ByteBuffer sort,
LAPACK_Z_SELECT2 selctg, @Cast("char*") ByteBuffer sense, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb, IntBuffer sdim,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vsl, IntBuffer ldvsl,
@Cast("lapack_complex_double*") DoubleBuffer vsr, IntBuffer ldvsr,
DoubleBuffer rconde, DoubleBuffer rcondv, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, DoubleBuffer rwork, IntBuffer iwork,
IntBuffer liwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_zggesx( @Cast("char*") byte[] jobvsl, @Cast("char*") byte[] jobvsr, @Cast("char*") byte[] sort,
LAPACK_Z_SELECT2 selctg, @Cast("char*") byte[] sense, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb, int[] sdim,
@Cast("lapack_complex_double*") double[] alpha, @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vsl, int[] ldvsl,
@Cast("lapack_complex_double*") double[] vsr, int[] ldvsr,
double[] rconde, double[] rcondv, @Cast("lapack_complex_double*") double[] work,
int[] lwork, double[] rwork, int[] iwork,
int[] liwork, int[] bwork,
int[] info );
public static native void LAPACK_sggev( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n, FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb, FloatPointer alphar,
FloatPointer alphai, FloatPointer beta, FloatPointer vl, IntPointer ldvl,
FloatPointer vr, IntPointer ldvr, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sggev( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n, FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb, FloatBuffer alphar,
FloatBuffer alphai, FloatBuffer beta, FloatBuffer vl, IntBuffer ldvl,
FloatBuffer vr, IntBuffer ldvr, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sggev( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n, float[] a,
int[] lda, float[] b, int[] ldb, float[] alphar,
float[] alphai, float[] beta, float[] vl, int[] ldvl,
float[] vr, int[] ldvr, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dggev( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb, DoublePointer alphar,
DoublePointer alphai, DoublePointer beta, DoublePointer vl, IntPointer ldvl,
DoublePointer vr, IntPointer ldvr, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dggev( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb, DoubleBuffer alphar,
DoubleBuffer alphai, DoubleBuffer beta, DoubleBuffer vl, IntBuffer ldvl,
DoubleBuffer vr, IntBuffer ldvr, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dggev( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n, double[] a,
int[] lda, double[] b, int[] ldb, double[] alphar,
double[] alphai, double[] beta, double[] vl, int[] ldvl,
double[] vr, int[] ldvr, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cggev( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vl, IntPointer ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, IntPointer ldvr,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cggev( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vl, IntBuffer ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, IntBuffer ldvr,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cggev( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] alpha, @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vl, int[] ldvl,
@Cast("lapack_complex_float*") float[] vr, int[] ldvr,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zggev( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vl, IntPointer ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, IntPointer ldvr,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zggev( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vl, IntBuffer ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, IntBuffer ldvr,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zggev( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] alpha, @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vl, int[] ldvl,
@Cast("lapack_complex_double*") double[] vr, int[] ldvr,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_sggev3( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n, FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb, FloatPointer alphar,
FloatPointer alphai, FloatPointer beta, FloatPointer vl, IntPointer ldvl,
FloatPointer vr, IntPointer ldvr, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sggev3( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n, FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb, FloatBuffer alphar,
FloatBuffer alphai, FloatBuffer beta, FloatBuffer vl, IntBuffer ldvl,
FloatBuffer vr, IntBuffer ldvr, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sggev3( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n, float[] a,
int[] lda, float[] b, int[] ldb, float[] alphar,
float[] alphai, float[] beta, float[] vl, int[] ldvl,
float[] vr, int[] ldvr, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dggev3( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n, DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb, DoublePointer alphar,
DoublePointer alphai, DoublePointer beta, DoublePointer vl, IntPointer ldvl,
DoublePointer vr, IntPointer ldvr, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dggev3( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n, DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb, DoubleBuffer alphar,
DoubleBuffer alphai, DoubleBuffer beta, DoubleBuffer vl, IntBuffer ldvl,
DoubleBuffer vr, IntBuffer ldvr, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dggev3( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n, double[] a,
int[] lda, double[] b, int[] ldb, double[] alphar,
double[] alphai, double[] beta, double[] vl, int[] ldvl,
double[] vr, int[] ldvr, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_cggev3( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vl, IntPointer ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, IntPointer ldvr,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer info );
public static native void LAPACK_cggev3( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vl, IntBuffer ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, IntBuffer ldvr,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer info );
public static native void LAPACK_cggev3( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] alpha, @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vl, int[] ldvl,
@Cast("lapack_complex_float*") float[] vr, int[] ldvr,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] info );
public static native void LAPACK_zggev3( @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vl, IntPointer ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, IntPointer ldvr,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer info );
public static native void LAPACK_zggev3( @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vl, IntBuffer ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, IntBuffer ldvr,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer info );
public static native void LAPACK_zggev3( @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] alpha, @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vl, int[] ldvl,
@Cast("lapack_complex_double*") double[] vr, int[] ldvr,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] info );
public static native void LAPACK_sggevx( @Cast("char*") BytePointer balanc, @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, @Cast("char*") BytePointer sense,
IntPointer n, FloatPointer a, IntPointer lda, FloatPointer b,
IntPointer ldb, FloatPointer alphar, FloatPointer alphai, FloatPointer beta,
FloatPointer vl, IntPointer ldvl, FloatPointer vr, IntPointer ldvr,
IntPointer ilo, IntPointer ihi, FloatPointer lscale,
FloatPointer rscale, FloatPointer abnrm, FloatPointer bbnrm, FloatPointer rconde,
FloatPointer rcondv, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_sggevx( @Cast("char*") ByteBuffer balanc, @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, @Cast("char*") ByteBuffer sense,
IntBuffer n, FloatBuffer a, IntBuffer lda, FloatBuffer b,
IntBuffer ldb, FloatBuffer alphar, FloatBuffer alphai, FloatBuffer beta,
FloatBuffer vl, IntBuffer ldvl, FloatBuffer vr, IntBuffer ldvr,
IntBuffer ilo, IntBuffer ihi, FloatBuffer lscale,
FloatBuffer rscale, FloatBuffer abnrm, FloatBuffer bbnrm, FloatBuffer rconde,
FloatBuffer rcondv, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_sggevx( @Cast("char*") byte[] balanc, @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, @Cast("char*") byte[] sense,
int[] n, float[] a, int[] lda, float[] b,
int[] ldb, float[] alphar, float[] alphai, float[] beta,
float[] vl, int[] ldvl, float[] vr, int[] ldvr,
int[] ilo, int[] ihi, float[] lscale,
float[] rscale, float[] abnrm, float[] bbnrm, float[] rconde,
float[] rcondv, float[] work, int[] lwork,
int[] iwork, int[] bwork,
int[] info );
public static native void LAPACK_dggevx( @Cast("char*") BytePointer balanc, @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, @Cast("char*") BytePointer sense,
IntPointer n, DoublePointer a, IntPointer lda, DoublePointer b,
IntPointer ldb, DoublePointer alphar, DoublePointer alphai,
DoublePointer beta, DoublePointer vl, IntPointer ldvl, DoublePointer vr,
IntPointer ldvr, IntPointer ilo, IntPointer ihi,
DoublePointer lscale, DoublePointer rscale, DoublePointer abnrm,
DoublePointer bbnrm, DoublePointer rconde, DoublePointer rcondv, DoublePointer work,
IntPointer lwork, IntPointer iwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_dggevx( @Cast("char*") ByteBuffer balanc, @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, @Cast("char*") ByteBuffer sense,
IntBuffer n, DoubleBuffer a, IntBuffer lda, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer alphar, DoubleBuffer alphai,
DoubleBuffer beta, DoubleBuffer vl, IntBuffer ldvl, DoubleBuffer vr,
IntBuffer ldvr, IntBuffer ilo, IntBuffer ihi,
DoubleBuffer lscale, DoubleBuffer rscale, DoubleBuffer abnrm,
DoubleBuffer bbnrm, DoubleBuffer rconde, DoubleBuffer rcondv, DoubleBuffer work,
IntBuffer lwork, IntBuffer iwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_dggevx( @Cast("char*") byte[] balanc, @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, @Cast("char*") byte[] sense,
int[] n, double[] a, int[] lda, double[] b,
int[] ldb, double[] alphar, double[] alphai,
double[] beta, double[] vl, int[] ldvl, double[] vr,
int[] ldvr, int[] ilo, int[] ihi,
double[] lscale, double[] rscale, double[] abnrm,
double[] bbnrm, double[] rconde, double[] rcondv, double[] work,
int[] lwork, int[] iwork, int[] bwork,
int[] info );
public static native void LAPACK_cggevx( @Cast("char*") BytePointer balanc, @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, @Cast("char*") BytePointer sense,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer vl, IntPointer ldvl,
@Cast("lapack_complex_float*") FloatPointer vr, IntPointer ldvr, IntPointer ilo,
IntPointer ihi, FloatPointer lscale, FloatPointer rscale, FloatPointer abnrm,
FloatPointer bbnrm, FloatPointer rconde, FloatPointer rcondv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, FloatPointer rwork,
IntPointer iwork, IntPointer bwork,
IntPointer info );
public static native void LAPACK_cggevx( @Cast("char*") ByteBuffer balanc, @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, @Cast("char*") ByteBuffer sense,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer vl, IntBuffer ldvl,
@Cast("lapack_complex_float*") FloatBuffer vr, IntBuffer ldvr, IntBuffer ilo,
IntBuffer ihi, FloatBuffer lscale, FloatBuffer rscale, FloatBuffer abnrm,
FloatBuffer bbnrm, FloatBuffer rconde, FloatBuffer rcondv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, FloatBuffer rwork,
IntBuffer iwork, IntBuffer bwork,
IntBuffer info );
public static native void LAPACK_cggevx( @Cast("char*") byte[] balanc, @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, @Cast("char*") byte[] sense,
int[] n, @Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] alpha, @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] vl, int[] ldvl,
@Cast("lapack_complex_float*") float[] vr, int[] ldvr, int[] ilo,
int[] ihi, float[] lscale, float[] rscale, float[] abnrm,
float[] bbnrm, float[] rconde, float[] rcondv,
@Cast("lapack_complex_float*") float[] work, int[] lwork, float[] rwork,
int[] iwork, int[] bwork,
int[] info );
public static native void LAPACK_zggevx( @Cast("char*") BytePointer balanc, @Cast("char*") BytePointer jobvl, @Cast("char*") BytePointer jobvr, @Cast("char*") BytePointer sense,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer vl, IntPointer ldvl,
@Cast("lapack_complex_double*") DoublePointer vr, IntPointer ldvr,
IntPointer ilo, IntPointer ihi, DoublePointer lscale,
DoublePointer rscale, DoublePointer abnrm, DoublePointer bbnrm,
DoublePointer rconde, DoublePointer rcondv, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, DoublePointer rwork, IntPointer iwork,
IntPointer bwork, IntPointer info );
public static native void LAPACK_zggevx( @Cast("char*") ByteBuffer balanc, @Cast("char*") ByteBuffer jobvl, @Cast("char*") ByteBuffer jobvr, @Cast("char*") ByteBuffer sense,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer vl, IntBuffer ldvl,
@Cast("lapack_complex_double*") DoubleBuffer vr, IntBuffer ldvr,
IntBuffer ilo, IntBuffer ihi, DoubleBuffer lscale,
DoubleBuffer rscale, DoubleBuffer abnrm, DoubleBuffer bbnrm,
DoubleBuffer rconde, DoubleBuffer rcondv, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, DoubleBuffer rwork, IntBuffer iwork,
IntBuffer bwork, IntBuffer info );
public static native void LAPACK_zggevx( @Cast("char*") byte[] balanc, @Cast("char*") byte[] jobvl, @Cast("char*") byte[] jobvr, @Cast("char*") byte[] sense,
int[] n, @Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] alpha, @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] vl, int[] ldvl,
@Cast("lapack_complex_double*") double[] vr, int[] ldvr,
int[] ilo, int[] ihi, double[] lscale,
double[] rscale, double[] abnrm, double[] bbnrm,
double[] rconde, double[] rcondv, @Cast("lapack_complex_double*") double[] work,
int[] lwork, double[] rwork, int[] iwork,
int[] bwork, int[] info );
public static native void LAPACK_dsfrk( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer n,
IntPointer k, DoublePointer alpha, @Const DoublePointer a,
IntPointer lda, DoublePointer beta, DoublePointer c );
public static native void LAPACK_dsfrk( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer n,
IntBuffer k, DoubleBuffer alpha, @Const DoubleBuffer a,
IntBuffer lda, DoubleBuffer beta, DoubleBuffer c );
public static native void LAPACK_dsfrk( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] n,
int[] k, double[] alpha, @Const double[] a,
int[] lda, double[] beta, double[] c );
public static native void LAPACK_ssfrk( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer n,
IntPointer k, FloatPointer alpha, @Const FloatPointer a, IntPointer lda,
FloatPointer beta, FloatPointer c );
public static native void LAPACK_ssfrk( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer n,
IntBuffer k, FloatBuffer alpha, @Const FloatBuffer a, IntBuffer lda,
FloatBuffer beta, FloatBuffer c );
public static native void LAPACK_ssfrk( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] n,
int[] k, float[] alpha, @Const float[] a, int[] lda,
float[] beta, float[] c );
public static native void LAPACK_zhfrk( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer n,
IntPointer k, DoublePointer alpha, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, DoublePointer beta, @Cast("lapack_complex_double*") DoublePointer c );
public static native void LAPACK_zhfrk( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer n,
IntBuffer k, DoubleBuffer alpha, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, DoubleBuffer beta, @Cast("lapack_complex_double*") DoubleBuffer c );
public static native void LAPACK_zhfrk( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] n,
int[] k, double[] alpha, @Cast("const lapack_complex_double*") double[] a,
int[] lda, double[] beta, @Cast("lapack_complex_double*") double[] c );
public static native void LAPACK_chfrk( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans, IntPointer n,
IntPointer k, FloatPointer alpha, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, FloatPointer beta, @Cast("lapack_complex_float*") FloatPointer c );
public static native void LAPACK_chfrk( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans, IntBuffer n,
IntBuffer k, FloatBuffer alpha, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, FloatBuffer beta, @Cast("lapack_complex_float*") FloatBuffer c );
public static native void LAPACK_chfrk( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans, int[] n,
int[] k, float[] alpha, @Cast("const lapack_complex_float*") float[] a,
int[] lda, float[] beta, @Cast("lapack_complex_float*") float[] c );
public static native void LAPACK_dtfsm( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans,
@Cast("char*") BytePointer diag, IntPointer m, IntPointer n, DoublePointer alpha,
@Const DoublePointer a, DoublePointer b, IntPointer ldb );
public static native void LAPACK_dtfsm( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans,
@Cast("char*") ByteBuffer diag, IntBuffer m, IntBuffer n, DoubleBuffer alpha,
@Const DoubleBuffer a, DoubleBuffer b, IntBuffer ldb );
public static native void LAPACK_dtfsm( @Cast("char*") byte[] transr, @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans,
@Cast("char*") byte[] diag, int[] m, int[] n, double[] alpha,
@Const double[] a, double[] b, int[] ldb );
public static native void LAPACK_stfsm( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans,
@Cast("char*") BytePointer diag, IntPointer m, IntPointer n, FloatPointer alpha,
@Const FloatPointer a, FloatPointer b, IntPointer ldb );
public static native void LAPACK_stfsm( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans,
@Cast("char*") ByteBuffer diag, IntBuffer m, IntBuffer n, FloatBuffer alpha,
@Const FloatBuffer a, FloatBuffer b, IntBuffer ldb );
public static native void LAPACK_stfsm( @Cast("char*") byte[] transr, @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans,
@Cast("char*") byte[] diag, int[] m, int[] n, float[] alpha,
@Const float[] a, float[] b, int[] ldb );
public static native void LAPACK_ztfsm( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans,
@Cast("char*") BytePointer diag, IntPointer m, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("const lapack_complex_double*") DoublePointer a,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb );
public static native void LAPACK_ztfsm( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans,
@Cast("char*") ByteBuffer diag, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("const lapack_complex_double*") DoubleBuffer a,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb );
public static native void LAPACK_ztfsm( @Cast("char*") byte[] transr, @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans,
@Cast("char*") byte[] diag, int[] m, int[] n,
@Cast("lapack_complex_double*") double[] alpha, @Cast("const lapack_complex_double*") double[] a,
@Cast("lapack_complex_double*") double[] b, int[] ldb );
public static native void LAPACK_ctfsm( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer side, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer trans,
@Cast("char*") BytePointer diag, IntPointer m, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("const lapack_complex_float*") FloatPointer a,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb );
public static native void LAPACK_ctfsm( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer trans,
@Cast("char*") ByteBuffer diag, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("const lapack_complex_float*") FloatBuffer a,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb );
public static native void LAPACK_ctfsm( @Cast("char*") byte[] transr, @Cast("char*") byte[] side, @Cast("char*") byte[] uplo, @Cast("char*") byte[] trans,
@Cast("char*") byte[] diag, int[] m, int[] n,
@Cast("lapack_complex_float*") float[] alpha, @Cast("const lapack_complex_float*") float[] a,
@Cast("lapack_complex_float*") float[] b, int[] ldb );
public static native void LAPACK_dtfttp( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer arf,
DoublePointer ap, IntPointer info );
public static native void LAPACK_dtfttp( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer arf,
DoubleBuffer ap, IntBuffer info );
public static native void LAPACK_dtfttp( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, @Const double[] arf,
double[] ap, int[] info );
public static native void LAPACK_stfttp( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer arf,
FloatPointer ap, IntPointer info );
public static native void LAPACK_stfttp( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer arf,
FloatBuffer ap, IntBuffer info );
public static native void LAPACK_stfttp( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, @Const float[] arf,
float[] ap, int[] info );
public static native void LAPACK_ztfttp( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer arf, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer info );
public static native void LAPACK_ztfttp( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer arf, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer info );
public static native void LAPACK_ztfttp( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_double*") double[] arf, @Cast("lapack_complex_double*") double[] ap,
int[] info );
public static native void LAPACK_ctfttp( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer arf, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer info );
public static native void LAPACK_ctfttp( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer arf, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer info );
public static native void LAPACK_ctfttp( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_float*") float[] arf, @Cast("lapack_complex_float*") float[] ap,
int[] info );
public static native void LAPACK_dtfttr( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer arf,
DoublePointer a, IntPointer lda, IntPointer info );
public static native void LAPACK_dtfttr( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer arf,
DoubleBuffer a, IntBuffer lda, IntBuffer info );
public static native void LAPACK_dtfttr( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, @Const double[] arf,
double[] a, int[] lda, int[] info );
public static native void LAPACK_stfttr( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer arf,
FloatPointer a, IntPointer lda, IntPointer info );
public static native void LAPACK_stfttr( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer arf,
FloatBuffer a, IntBuffer lda, IntBuffer info );
public static native void LAPACK_stfttr( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, @Const float[] arf,
float[] a, int[] lda, int[] info );
public static native void LAPACK_ztfttr( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer arf, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_ztfttr( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer arf, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_ztfttr( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_double*") double[] arf, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] info );
public static native void LAPACK_ctfttr( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer arf, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_ctfttr( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer arf, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_ctfttr( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_float*") float[] arf, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] info );
public static native void LAPACK_dtpttf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer ap,
DoublePointer arf, IntPointer info );
public static native void LAPACK_dtpttf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer ap,
DoubleBuffer arf, IntBuffer info );
public static native void LAPACK_dtpttf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, @Const double[] ap,
double[] arf, int[] info );
public static native void LAPACK_stpttf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer ap,
FloatPointer arf, IntPointer info );
public static native void LAPACK_stpttf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer ap,
FloatBuffer arf, IntBuffer info );
public static native void LAPACK_stpttf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, @Const float[] ap,
float[] arf, int[] info );
public static native void LAPACK_ztpttf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer ap, @Cast("lapack_complex_double*") DoublePointer arf,
IntPointer info );
public static native void LAPACK_ztpttf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer ap, @Cast("lapack_complex_double*") DoubleBuffer arf,
IntBuffer info );
public static native void LAPACK_ztpttf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_double*") double[] ap, @Cast("lapack_complex_double*") double[] arf,
int[] info );
public static native void LAPACK_ctpttf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer ap, @Cast("lapack_complex_float*") FloatPointer arf,
IntPointer info );
public static native void LAPACK_ctpttf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer ap, @Cast("lapack_complex_float*") FloatBuffer arf,
IntBuffer info );
public static native void LAPACK_ctpttf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_float*") float[] ap, @Cast("lapack_complex_float*") float[] arf,
int[] info );
public static native void LAPACK_dtpttr( @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer ap, DoublePointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_dtpttr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer ap, DoubleBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_dtpttr( @Cast("char*") byte[] uplo, int[] n, @Const double[] ap, double[] a,
int[] lda, int[] info );
public static native void LAPACK_stpttr( @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer ap, FloatPointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_stpttr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer ap, FloatBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_stpttr( @Cast("char*") byte[] uplo, int[] n, @Const float[] ap, float[] a,
int[] lda, int[] info );
public static native void LAPACK_ztpttr( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer ap,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_ztpttr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer ap,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_ztpttr( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] ap,
@Cast("lapack_complex_double*") double[] a, int[] lda,
int[] info );
public static native void LAPACK_ctpttr( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer ap,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_ctpttr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer ap,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_ctpttr( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] ap,
@Cast("lapack_complex_float*") float[] a, int[] lda,
int[] info );
public static native void LAPACK_dtrttf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer a,
IntPointer lda, DoublePointer arf, IntPointer info );
public static native void LAPACK_dtrttf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer a,
IntBuffer lda, DoubleBuffer arf, IntBuffer info );
public static native void LAPACK_dtrttf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, @Const double[] a,
int[] lda, double[] arf, int[] info );
public static native void LAPACK_strttf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer a,
IntPointer lda, FloatPointer arf, IntPointer info );
public static native void LAPACK_strttf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer a,
IntBuffer lda, FloatBuffer arf, IntBuffer info );
public static native void LAPACK_strttf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n, @Const float[] a,
int[] lda, float[] arf, int[] info );
public static native void LAPACK_ztrttf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer arf, IntPointer info );
public static native void LAPACK_ztrttf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer arf, IntBuffer info );
public static native void LAPACK_ztrttf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] arf, int[] info );
public static native void LAPACK_ctrttf( @Cast("char*") BytePointer transr, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer arf, IntPointer info );
public static native void LAPACK_ctrttf( @Cast("char*") ByteBuffer transr, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer arf, IntBuffer info );
public static native void LAPACK_ctrttf( @Cast("char*") byte[] transr, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] arf, int[] info );
public static native void LAPACK_dtrttp( @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer a, IntPointer lda,
DoublePointer ap, IntPointer info );
public static native void LAPACK_dtrttp( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer a, IntBuffer lda,
DoubleBuffer ap, IntBuffer info );
public static native void LAPACK_dtrttp( @Cast("char*") byte[] uplo, int[] n, @Const double[] a, int[] lda,
double[] ap, int[] info );
public static native void LAPACK_strttp( @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer a, IntPointer lda,
FloatPointer ap, IntPointer info );
public static native void LAPACK_strttp( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer a, IntBuffer lda,
FloatBuffer ap, IntBuffer info );
public static native void LAPACK_strttp( @Cast("char*") byte[] uplo, int[] n, @Const float[] a, int[] lda,
float[] ap, int[] info );
public static native void LAPACK_ztrttp( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer ap,
IntPointer info );
public static native void LAPACK_ztrttp( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer ap,
IntBuffer info );
public static native void LAPACK_ztrttp( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] ap,
int[] info );
public static native void LAPACK_ctrttp( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer ap,
IntPointer info );
public static native void LAPACK_ctrttp( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer ap,
IntBuffer info );
public static native void LAPACK_ctrttp( @Cast("char*") byte[] uplo, int[] n, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] ap,
int[] info );
public static native void LAPACK_sgeqrfp( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer tau, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_sgeqrfp( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer tau, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_sgeqrfp( int[] m, int[] n, float[] a, int[] lda,
float[] tau, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dgeqrfp( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer tau, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dgeqrfp( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer tau, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dgeqrfp( int[] m, int[] n, double[] a, int[] lda,
double[] tau, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_cgeqrfp( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_cgeqrfp( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_cgeqrfp( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zgeqrfp( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zgeqrfp( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zgeqrfp( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_clacgv( IntPointer n, @Cast("lapack_complex_float*") FloatPointer x, IntPointer incx );
public static native void LAPACK_clacgv( IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer x, IntBuffer incx );
public static native void LAPACK_clacgv( int[] n, @Cast("lapack_complex_float*") float[] x, int[] incx );
public static native void LAPACK_zlacgv( IntPointer n, @Cast("lapack_complex_double*") DoublePointer x, IntPointer incx );
public static native void LAPACK_zlacgv( IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer incx );
public static native void LAPACK_zlacgv( int[] n, @Cast("lapack_complex_double*") double[] x, int[] incx );
public static native void LAPACK_slarnv( IntPointer idist, IntPointer iseed, IntPointer n,
FloatPointer x );
public static native void LAPACK_slarnv( IntBuffer idist, IntBuffer iseed, IntBuffer n,
FloatBuffer x );
public static native void LAPACK_slarnv( int[] idist, int[] iseed, int[] n,
float[] x );
public static native void LAPACK_dlarnv( IntPointer idist, IntPointer iseed, IntPointer n,
DoublePointer x );
public static native void LAPACK_dlarnv( IntBuffer idist, IntBuffer iseed, IntBuffer n,
DoubleBuffer x );
public static native void LAPACK_dlarnv( int[] idist, int[] iseed, int[] n,
double[] x );
public static native void LAPACK_clarnv( IntPointer idist, IntPointer iseed, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer x );
public static native void LAPACK_clarnv( IntBuffer idist, IntBuffer iseed, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer x );
public static native void LAPACK_clarnv( int[] idist, int[] iseed, int[] n,
@Cast("lapack_complex_float*") float[] x );
public static native void LAPACK_zlarnv( IntPointer idist, IntPointer iseed, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer x );
public static native void LAPACK_zlarnv( IntBuffer idist, IntBuffer iseed, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer x );
public static native void LAPACK_zlarnv( int[] idist, int[] iseed, int[] n,
@Cast("lapack_complex_double*") double[] x );
public static native void LAPACK_sgeqr2( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer tau, FloatPointer work, IntPointer info );
public static native void LAPACK_sgeqr2( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer tau, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sgeqr2( int[] m, int[] n, float[] a, int[] lda,
float[] tau, float[] work, int[] info );
public static native void LAPACK_dgeqr2( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer tau, DoublePointer work, IntPointer info );
public static native void LAPACK_dgeqr2( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer tau, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dgeqr2( int[] m, int[] n, double[] a, int[] lda,
double[] tau, double[] work, int[] info );
public static native void LAPACK_cgeqr2( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_cgeqr2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_cgeqr2( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zgeqr2( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zgeqr2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zgeqr2( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_slacn2( IntPointer n, FloatPointer v, FloatPointer x, IntPointer isgn,
FloatPointer est, IntPointer kase, IntPointer isave );
public static native void LAPACK_slacn2( IntBuffer n, FloatBuffer v, FloatBuffer x, IntBuffer isgn,
FloatBuffer est, IntBuffer kase, IntBuffer isave );
public static native void LAPACK_slacn2( int[] n, float[] v, float[] x, int[] isgn,
float[] est, int[] kase, int[] isave );
public static native void LAPACK_dlacn2( IntPointer n, DoublePointer v, DoublePointer x, IntPointer isgn,
DoublePointer est, IntPointer kase, IntPointer isave );
public static native void LAPACK_dlacn2( IntBuffer n, DoubleBuffer v, DoubleBuffer x, IntBuffer isgn,
DoubleBuffer est, IntBuffer kase, IntBuffer isave );
public static native void LAPACK_dlacn2( int[] n, double[] v, double[] x, int[] isgn,
double[] est, int[] kase, int[] isave );
public static native void LAPACK_clacn2( IntPointer n, @Cast("lapack_complex_float*") FloatPointer v,
@Cast("lapack_complex_float*") FloatPointer x, FloatPointer est,
IntPointer kase, IntPointer isave );
public static native void LAPACK_clacn2( IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer v,
@Cast("lapack_complex_float*") FloatBuffer x, FloatBuffer est,
IntBuffer kase, IntBuffer isave );
public static native void LAPACK_clacn2( int[] n, @Cast("lapack_complex_float*") float[] v,
@Cast("lapack_complex_float*") float[] x, float[] est,
int[] kase, int[] isave );
public static native void LAPACK_zlacn2( IntPointer n, @Cast("lapack_complex_double*") DoublePointer v,
@Cast("lapack_complex_double*") DoublePointer x, DoublePointer est,
IntPointer kase, IntPointer isave );
public static native void LAPACK_zlacn2( IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer v,
@Cast("lapack_complex_double*") DoubleBuffer x, DoubleBuffer est,
IntBuffer kase, IntBuffer isave );
public static native void LAPACK_zlacn2( int[] n, @Cast("lapack_complex_double*") double[] v,
@Cast("lapack_complex_double*") double[] x, double[] est,
int[] kase, int[] isave );
public static native void LAPACK_slacpy( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n, @Const FloatPointer a,
IntPointer lda, FloatPointer b, IntPointer ldb );
public static native void LAPACK_slacpy( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n, @Const FloatBuffer a,
IntBuffer lda, FloatBuffer b, IntBuffer ldb );
public static native void LAPACK_slacpy( @Cast("char*") byte[] uplo, int[] m, int[] n, @Const float[] a,
int[] lda, float[] b, int[] ldb );
public static native void LAPACK_dlacpy( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n, @Const DoublePointer a,
IntPointer lda, DoublePointer b, IntPointer ldb );
public static native void LAPACK_dlacpy( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n, @Const DoubleBuffer a,
IntBuffer lda, DoubleBuffer b, IntBuffer ldb );
public static native void LAPACK_dlacpy( @Cast("char*") byte[] uplo, int[] m, int[] n, @Const double[] a,
int[] lda, double[] b, int[] ldb );
public static native void LAPACK_clacpy( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb );
public static native void LAPACK_clacpy( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb );
public static native void LAPACK_clacpy( @Cast("char*") byte[] uplo, int[] m, int[] n,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb );
public static native void LAPACK_zlacpy( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb );
public static native void LAPACK_zlacpy( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb );
public static native void LAPACK_zlacpy( @Cast("char*") byte[] uplo, int[] m, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb );
public static native void LAPACK_clacp2( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n, @Const FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb );
public static native void LAPACK_clacp2( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n, @Const FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb );
public static native void LAPACK_clacp2( @Cast("char*") byte[] uplo, int[] m, int[] n, @Const float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] b, int[] ldb );
public static native void LAPACK_zlacp2( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n, @Const DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb );
public static native void LAPACK_zlacp2( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n, @Const DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb );
public static native void LAPACK_zlacp2( @Cast("char*") byte[] uplo, int[] m, int[] n, @Const double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] b,
int[] ldb );
public static native void LAPACK_sgetf2( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_sgetf2( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_sgetf2( int[] m, int[] n, float[] a, int[] lda,
int[] ipiv, int[] info );
public static native void LAPACK_dgetf2( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer ipiv, IntPointer info );
public static native void LAPACK_dgetf2( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_dgetf2( int[] m, int[] n, double[] a, int[] lda,
int[] ipiv, int[] info );
public static native void LAPACK_cgetf2( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer ipiv, IntPointer info );
public static native void LAPACK_cgetf2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_cgetf2( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] ipiv, int[] info );
public static native void LAPACK_zgetf2( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ipiv, IntPointer info );
public static native void LAPACK_zgetf2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv, IntBuffer info );
public static native void LAPACK_zgetf2( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ipiv, int[] info );
public static native void LAPACK_slaswp( IntPointer n, FloatPointer a, IntPointer lda, IntPointer k1,
IntPointer k2, @Const IntPointer ipiv, IntPointer incx );
public static native void LAPACK_slaswp( IntBuffer n, FloatBuffer a, IntBuffer lda, IntBuffer k1,
IntBuffer k2, @Const IntBuffer ipiv, IntBuffer incx );
public static native void LAPACK_slaswp( int[] n, float[] a, int[] lda, int[] k1,
int[] k2, @Const int[] ipiv, int[] incx );
public static native void LAPACK_dlaswp( IntPointer n, DoublePointer a, IntPointer lda, IntPointer k1,
IntPointer k2, @Const IntPointer ipiv, IntPointer incx );
public static native void LAPACK_dlaswp( IntBuffer n, DoubleBuffer a, IntBuffer lda, IntBuffer k1,
IntBuffer k2, @Const IntBuffer ipiv, IntBuffer incx );
public static native void LAPACK_dlaswp( int[] n, double[] a, int[] lda, int[] k1,
int[] k2, @Const int[] ipiv, int[] incx );
public static native void LAPACK_claswp( IntPointer n, @Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
IntPointer k1, IntPointer k2, @Const IntPointer ipiv,
IntPointer incx );
public static native void LAPACK_claswp( IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
IntBuffer k1, IntBuffer k2, @Const IntBuffer ipiv,
IntBuffer incx );
public static native void LAPACK_claswp( int[] n, @Cast("lapack_complex_float*") float[] a, int[] lda,
int[] k1, int[] k2, @Const int[] ipiv,
int[] incx );
public static native void LAPACK_zlaswp( IntPointer n, @Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
IntPointer k1, IntPointer k2, @Const IntPointer ipiv,
IntPointer incx );
public static native void LAPACK_zlaswp( IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
IntBuffer k1, IntBuffer k2, @Const IntBuffer ipiv,
IntBuffer incx );
public static native void LAPACK_zlaswp( int[] n, @Cast("lapack_complex_double*") double[] a, int[] lda,
int[] k1, int[] k2, @Const int[] ipiv,
int[] incx );
public static native float LAPACK_slange( @Cast("char*") BytePointer norm, IntPointer m, IntPointer n, @Const FloatPointer a,
IntPointer lda, FloatPointer work );
public static native float LAPACK_slange( @Cast("char*") ByteBuffer norm, IntBuffer m, IntBuffer n, @Const FloatBuffer a,
IntBuffer lda, FloatBuffer work );
public static native float LAPACK_slange( @Cast("char*") byte[] norm, int[] m, int[] n, @Const float[] a,
int[] lda, float[] work );
public static native double LAPACK_dlange( @Cast("char*") BytePointer norm, IntPointer m, IntPointer n, @Const DoublePointer a,
IntPointer lda, DoublePointer work );
public static native double LAPACK_dlange( @Cast("char*") ByteBuffer norm, IntBuffer m, IntBuffer n, @Const DoubleBuffer a,
IntBuffer lda, DoubleBuffer work );
public static native double LAPACK_dlange( @Cast("char*") byte[] norm, int[] m, int[] n, @Const double[] a,
int[] lda, double[] work );
public static native float LAPACK_clange( @Cast("char*") BytePointer norm, IntPointer m, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer work );
public static native float LAPACK_clange( @Cast("char*") ByteBuffer norm, IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer work );
public static native float LAPACK_clange( @Cast("char*") byte[] norm, int[] m, int[] n,
@Cast("const lapack_complex_float*") float[] a, int[] lda, float[] work );
public static native double LAPACK_zlange( @Cast("char*") BytePointer norm, IntPointer m, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer work );
public static native double LAPACK_zlange( @Cast("char*") ByteBuffer norm, IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer work );
public static native double LAPACK_zlange( @Cast("char*") byte[] norm, int[] m, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda, double[] work );
public static native float LAPACK_clanhe( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer work );
public static native float LAPACK_clanhe( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer work );
public static native float LAPACK_clanhe( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_float*") float[] a, int[] lda, float[] work );
public static native double LAPACK_zlanhe( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer work );
public static native double LAPACK_zlanhe( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer work );
public static native double LAPACK_zlanhe( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda, double[] work );
public static native float LAPACK_slansy( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, IntPointer n, @Const FloatPointer a,
IntPointer lda, FloatPointer work );
public static native float LAPACK_slansy( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const FloatBuffer a,
IntBuffer lda, FloatBuffer work );
public static native float LAPACK_slansy( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, int[] n, @Const float[] a,
int[] lda, float[] work );
public static native double LAPACK_dlansy( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, IntPointer n, @Const DoublePointer a,
IntPointer lda, DoublePointer work );
public static native double LAPACK_dlansy( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, IntBuffer n, @Const DoubleBuffer a,
IntBuffer lda, DoubleBuffer work );
public static native double LAPACK_dlansy( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, int[] n, @Const double[] a,
int[] lda, double[] work );
public static native float LAPACK_clansy( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda, FloatPointer work );
public static native float LAPACK_clansy( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda, FloatBuffer work );
public static native float LAPACK_clansy( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_float*") float[] a, int[] lda, float[] work );
public static native double LAPACK_zlansy( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda, DoublePointer work );
public static native double LAPACK_zlansy( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda, DoubleBuffer work );
public static native double LAPACK_zlansy( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda, double[] work );
public static native float LAPACK_slantr( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer m,
IntPointer n, @Const FloatPointer a, IntPointer lda, FloatPointer work );
public static native float LAPACK_slantr( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer m,
IntBuffer n, @Const FloatBuffer a, IntBuffer lda, FloatBuffer work );
public static native float LAPACK_slantr( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] m,
int[] n, @Const float[] a, int[] lda, float[] work );
public static native double LAPACK_dlantr( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer m,
IntPointer n, @Const DoublePointer a, IntPointer lda, DoublePointer work );
public static native double LAPACK_dlantr( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer m,
IntBuffer n, @Const DoubleBuffer a, IntBuffer lda, DoubleBuffer work );
public static native double LAPACK_dlantr( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] m,
int[] n, @Const double[] a, int[] lda, double[] work );
public static native float LAPACK_clantr( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer m,
IntPointer n, @Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
FloatPointer work );
public static native float LAPACK_clantr( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer m,
IntBuffer n, @Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
FloatBuffer work );
public static native float LAPACK_clantr( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] m,
int[] n, @Cast("const lapack_complex_float*") float[] a, int[] lda,
float[] work );
public static native double LAPACK_zlantr( @Cast("char*") BytePointer norm, @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer diag, IntPointer m,
IntPointer n, @Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
DoublePointer work );
public static native double LAPACK_zlantr( @Cast("char*") ByteBuffer norm, @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer diag, IntBuffer m,
IntBuffer n, @Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
DoubleBuffer work );
public static native double LAPACK_zlantr( @Cast("char*") byte[] norm, @Cast("char*") byte[] uplo, @Cast("char*") byte[] diag, int[] m,
int[] n, @Cast("const lapack_complex_double*") double[] a, int[] lda,
double[] work );
public static native float LAPACK_slamch( @Cast("char*") BytePointer cmach );
public static native float LAPACK_slamch( @Cast("char*") ByteBuffer cmach );
public static native float LAPACK_slamch( @Cast("char*") byte[] cmach );
public static native double LAPACK_dlamch( @Cast("char*") BytePointer cmach );
public static native double LAPACK_dlamch( @Cast("char*") ByteBuffer cmach );
public static native double LAPACK_dlamch( @Cast("char*") byte[] cmach );
public static native void LAPACK_sgelq2( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer tau, FloatPointer work, IntPointer info );
public static native void LAPACK_sgelq2( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer tau, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sgelq2( int[] m, int[] n, float[] a, int[] lda,
float[] tau, float[] work, int[] info );
public static native void LAPACK_dgelq2( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer tau, DoublePointer work, IntPointer info );
public static native void LAPACK_dgelq2( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer tau, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dgelq2( int[] m, int[] n, double[] a, int[] lda,
double[] tau, double[] work, int[] info );
public static native void LAPACK_cgelq2( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_cgelq2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_cgelq2( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zgelq2( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zgelq2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zgelq2( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_slarfb( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev,
IntPointer m, IntPointer n, IntPointer k, @Const FloatPointer v,
IntPointer ldv, @Const FloatPointer t, IntPointer ldt, FloatPointer c,
IntPointer ldc, FloatPointer work, IntPointer ldwork );
public static native void LAPACK_slarfb( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev,
IntBuffer m, IntBuffer n, IntBuffer k, @Const FloatBuffer v,
IntBuffer ldv, @Const FloatBuffer t, IntBuffer ldt, FloatBuffer c,
IntBuffer ldc, FloatBuffer work, IntBuffer ldwork );
public static native void LAPACK_slarfb( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, @Cast("char*") byte[] direct, @Cast("char*") byte[] storev,
int[] m, int[] n, int[] k, @Const float[] v,
int[] ldv, @Const float[] t, int[] ldt, float[] c,
int[] ldc, float[] work, int[] ldwork );
public static native void LAPACK_dlarfb( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev,
IntPointer m, IntPointer n, IntPointer k,
@Const DoublePointer v, IntPointer ldv, @Const DoublePointer t,
IntPointer ldt, DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer ldwork );
public static native void LAPACK_dlarfb( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev,
IntBuffer m, IntBuffer n, IntBuffer k,
@Const DoubleBuffer v, IntBuffer ldv, @Const DoubleBuffer t,
IntBuffer ldt, DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer ldwork );
public static native void LAPACK_dlarfb( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, @Cast("char*") byte[] direct, @Cast("char*") byte[] storev,
int[] m, int[] n, int[] k,
@Const double[] v, int[] ldv, @Const double[] t,
int[] ldt, double[] c, int[] ldc, double[] work,
int[] ldwork );
public static native void LAPACK_clarfb( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev,
IntPointer m, IntPointer n, IntPointer k,
@Cast("const lapack_complex_float*") FloatPointer v, IntPointer ldv,
@Cast("const lapack_complex_float*") FloatPointer t, IntPointer ldt,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer ldwork );
public static native void LAPACK_clarfb( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev,
IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("const lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer ldwork );
public static native void LAPACK_clarfb( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, @Cast("char*") byte[] direct, @Cast("char*") byte[] storev,
int[] m, int[] n, int[] k,
@Cast("const lapack_complex_float*") float[] v, int[] ldv,
@Cast("const lapack_complex_float*") float[] t, int[] ldt,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, int[] ldwork );
public static native void LAPACK_zlarfb( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev,
IntPointer m, IntPointer n, IntPointer k,
@Cast("const lapack_complex_double*") DoublePointer v, IntPointer ldv,
@Cast("const lapack_complex_double*") DoublePointer t, IntPointer ldt,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer ldwork );
public static native void LAPACK_zlarfb( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev,
IntBuffer m, IntBuffer n, IntBuffer k,
@Cast("const lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer ldwork );
public static native void LAPACK_zlarfb( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, @Cast("char*") byte[] direct, @Cast("char*") byte[] storev,
int[] m, int[] n, int[] k,
@Cast("const lapack_complex_double*") double[] v, int[] ldv,
@Cast("const lapack_complex_double*") double[] t, int[] ldt,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("lapack_complex_double*") double[] work, int[] ldwork );
public static native void LAPACK_slarfg( IntPointer n, FloatPointer alpha, FloatPointer x, IntPointer incx,
FloatPointer tau );
public static native void LAPACK_slarfg( IntBuffer n, FloatBuffer alpha, FloatBuffer x, IntBuffer incx,
FloatBuffer tau );
public static native void LAPACK_slarfg( int[] n, float[] alpha, float[] x, int[] incx,
float[] tau );
public static native void LAPACK_dlarfg( IntPointer n, DoublePointer alpha, DoublePointer x, IntPointer incx,
DoublePointer tau );
public static native void LAPACK_dlarfg( IntBuffer n, DoubleBuffer alpha, DoubleBuffer x, IntBuffer incx,
DoubleBuffer tau );
public static native void LAPACK_dlarfg( int[] n, double[] alpha, double[] x, int[] incx,
double[] tau );
public static native void LAPACK_clarfg( IntPointer n, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer incx,
@Cast("lapack_complex_float*") FloatPointer tau );
public static native void LAPACK_clarfg( IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer incx,
@Cast("lapack_complex_float*") FloatBuffer tau );
public static native void LAPACK_clarfg( int[] n, @Cast("lapack_complex_float*") float[] alpha,
@Cast("lapack_complex_float*") float[] x, int[] incx,
@Cast("lapack_complex_float*") float[] tau );
public static native void LAPACK_zlarfg( IntPointer n, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer incx,
@Cast("lapack_complex_double*") DoublePointer tau );
public static native void LAPACK_zlarfg( IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer incx,
@Cast("lapack_complex_double*") DoubleBuffer tau );
public static native void LAPACK_zlarfg( int[] n, @Cast("lapack_complex_double*") double[] alpha,
@Cast("lapack_complex_double*") double[] x, int[] incx,
@Cast("lapack_complex_double*") double[] tau );
public static native void LAPACK_slarft( @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev, IntPointer n, IntPointer k,
@Const FloatPointer v, IntPointer ldv, @Const FloatPointer tau, FloatPointer t,
IntPointer ldt );
public static native void LAPACK_slarft( @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev, IntBuffer n, IntBuffer k,
@Const FloatBuffer v, IntBuffer ldv, @Const FloatBuffer tau, FloatBuffer t,
IntBuffer ldt );
public static native void LAPACK_slarft( @Cast("char*") byte[] direct, @Cast("char*") byte[] storev, int[] n, int[] k,
@Const float[] v, int[] ldv, @Const float[] tau, float[] t,
int[] ldt );
public static native void LAPACK_dlarft( @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev, IntPointer n, IntPointer k,
@Const DoublePointer v, IntPointer ldv, @Const DoublePointer tau,
DoublePointer t, IntPointer ldt );
public static native void LAPACK_dlarft( @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev, IntBuffer n, IntBuffer k,
@Const DoubleBuffer v, IntBuffer ldv, @Const DoubleBuffer tau,
DoubleBuffer t, IntBuffer ldt );
public static native void LAPACK_dlarft( @Cast("char*") byte[] direct, @Cast("char*") byte[] storev, int[] n, int[] k,
@Const double[] v, int[] ldv, @Const double[] tau,
double[] t, int[] ldt );
public static native void LAPACK_clarft( @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev, IntPointer n, IntPointer k,
@Cast("const lapack_complex_float*") FloatPointer v, IntPointer ldv,
@Cast("const lapack_complex_float*") FloatPointer tau, @Cast("lapack_complex_float*") FloatPointer t,
IntPointer ldt );
public static native void LAPACK_clarft( @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev, IntBuffer n, IntBuffer k,
@Cast("const lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_float*") FloatBuffer tau, @Cast("lapack_complex_float*") FloatBuffer t,
IntBuffer ldt );
public static native void LAPACK_clarft( @Cast("char*") byte[] direct, @Cast("char*") byte[] storev, int[] n, int[] k,
@Cast("const lapack_complex_float*") float[] v, int[] ldv,
@Cast("const lapack_complex_float*") float[] tau, @Cast("lapack_complex_float*") float[] t,
int[] ldt );
public static native void LAPACK_zlarft( @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev, IntPointer n, IntPointer k,
@Cast("const lapack_complex_double*") DoublePointer v, IntPointer ldv,
@Cast("const lapack_complex_double*") DoublePointer tau, @Cast("lapack_complex_double*") DoublePointer t,
IntPointer ldt );
public static native void LAPACK_zlarft( @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev, IntBuffer n, IntBuffer k,
@Cast("const lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_double*") DoubleBuffer tau, @Cast("lapack_complex_double*") DoubleBuffer t,
IntBuffer ldt );
public static native void LAPACK_zlarft( @Cast("char*") byte[] direct, @Cast("char*") byte[] storev, int[] n, int[] k,
@Cast("const lapack_complex_double*") double[] v, int[] ldv,
@Cast("const lapack_complex_double*") double[] tau, @Cast("lapack_complex_double*") double[] t,
int[] ldt );
public static native void LAPACK_slarfx( @Cast("char*") BytePointer side, IntPointer m, IntPointer n, @Const FloatPointer v,
FloatPointer tau, FloatPointer c, IntPointer ldc, FloatPointer work );
public static native void LAPACK_slarfx( @Cast("char*") ByteBuffer side, IntBuffer m, IntBuffer n, @Const FloatBuffer v,
FloatBuffer tau, FloatBuffer c, IntBuffer ldc, FloatBuffer work );
public static native void LAPACK_slarfx( @Cast("char*") byte[] side, int[] m, int[] n, @Const float[] v,
float[] tau, float[] c, int[] ldc, float[] work );
public static native void LAPACK_dlarfx( @Cast("char*") BytePointer side, IntPointer m, IntPointer n, @Const DoublePointer v,
DoublePointer tau, DoublePointer c, IntPointer ldc, DoublePointer work );
public static native void LAPACK_dlarfx( @Cast("char*") ByteBuffer side, IntBuffer m, IntBuffer n, @Const DoubleBuffer v,
DoubleBuffer tau, DoubleBuffer c, IntBuffer ldc, DoubleBuffer work );
public static native void LAPACK_dlarfx( @Cast("char*") byte[] side, int[] m, int[] n, @Const double[] v,
double[] tau, double[] c, int[] ldc, double[] work );
public static native void LAPACK_clarfx( @Cast("char*") BytePointer side, IntPointer m, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer v, @Cast("lapack_complex_float*") FloatPointer tau,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work );
public static native void LAPACK_clarfx( @Cast("char*") ByteBuffer side, IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer v, @Cast("lapack_complex_float*") FloatBuffer tau,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work );
public static native void LAPACK_clarfx( @Cast("char*") byte[] side, int[] m, int[] n,
@Cast("const lapack_complex_float*") float[] v, @Cast("lapack_complex_float*") float[] tau,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work );
public static native void LAPACK_zlarfx( @Cast("char*") BytePointer side, IntPointer m, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer v, @Cast("lapack_complex_double*") DoublePointer tau,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("lapack_complex_double*") DoublePointer work );
public static native void LAPACK_zlarfx( @Cast("char*") ByteBuffer side, IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer v, @Cast("lapack_complex_double*") DoubleBuffer tau,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("lapack_complex_double*") DoubleBuffer work );
public static native void LAPACK_zlarfx( @Cast("char*") byte[] side, int[] m, int[] n,
@Cast("const lapack_complex_double*") double[] v, @Cast("lapack_complex_double*") double[] tau,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("lapack_complex_double*") double[] work );
public static native void LAPACK_slatms( IntPointer m, IntPointer n, @Cast("char*") BytePointer dist, IntPointer iseed,
@Cast("char*") BytePointer sym, FloatPointer d, IntPointer mode, FloatPointer cond,
FloatPointer dmax, IntPointer kl, IntPointer ku, @Cast("char*") BytePointer pack,
FloatPointer a, IntPointer lda, FloatPointer work, IntPointer info );
public static native void LAPACK_slatms( IntBuffer m, IntBuffer n, @Cast("char*") ByteBuffer dist, IntBuffer iseed,
@Cast("char*") ByteBuffer sym, FloatBuffer d, IntBuffer mode, FloatBuffer cond,
FloatBuffer dmax, IntBuffer kl, IntBuffer ku, @Cast("char*") ByteBuffer pack,
FloatBuffer a, IntBuffer lda, FloatBuffer work, IntBuffer info );
public static native void LAPACK_slatms( int[] m, int[] n, @Cast("char*") byte[] dist, int[] iseed,
@Cast("char*") byte[] sym, float[] d, int[] mode, float[] cond,
float[] dmax, int[] kl, int[] ku, @Cast("char*") byte[] pack,
float[] a, int[] lda, float[] work, int[] info );
public static native void LAPACK_dlatms( IntPointer m, IntPointer n, @Cast("char*") BytePointer dist, IntPointer iseed,
@Cast("char*") BytePointer sym, DoublePointer d, IntPointer mode, DoublePointer cond,
DoublePointer dmax, IntPointer kl, IntPointer ku, @Cast("char*") BytePointer pack,
DoublePointer a, IntPointer lda, DoublePointer work,
IntPointer info );
public static native void LAPACK_dlatms( IntBuffer m, IntBuffer n, @Cast("char*") ByteBuffer dist, IntBuffer iseed,
@Cast("char*") ByteBuffer sym, DoubleBuffer d, IntBuffer mode, DoubleBuffer cond,
DoubleBuffer dmax, IntBuffer kl, IntBuffer ku, @Cast("char*") ByteBuffer pack,
DoubleBuffer a, IntBuffer lda, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dlatms( int[] m, int[] n, @Cast("char*") byte[] dist, int[] iseed,
@Cast("char*") byte[] sym, double[] d, int[] mode, double[] cond,
double[] dmax, int[] kl, int[] ku, @Cast("char*") byte[] pack,
double[] a, int[] lda, double[] work,
int[] info );
public static native void LAPACK_clatms( IntPointer m, IntPointer n, @Cast("char*") BytePointer dist, IntPointer iseed,
@Cast("char*") BytePointer sym, FloatPointer d, IntPointer mode, FloatPointer cond,
FloatPointer dmax, IntPointer kl, IntPointer ku, @Cast("char*") BytePointer pack,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_clatms( IntBuffer m, IntBuffer n, @Cast("char*") ByteBuffer dist, IntBuffer iseed,
@Cast("char*") ByteBuffer sym, FloatBuffer d, IntBuffer mode, FloatBuffer cond,
FloatBuffer dmax, IntBuffer kl, IntBuffer ku, @Cast("char*") ByteBuffer pack,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_clatms( int[] m, int[] n, @Cast("char*") byte[] dist, int[] iseed,
@Cast("char*") byte[] sym, float[] d, int[] mode, float[] cond,
float[] dmax, int[] kl, int[] ku, @Cast("char*") byte[] pack,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zlatms( IntPointer m, IntPointer n, @Cast("char*") BytePointer dist, IntPointer iseed,
@Cast("char*") BytePointer sym, DoublePointer d, IntPointer mode, DoublePointer cond,
DoublePointer dmax, IntPointer kl, IntPointer ku, @Cast("char*") BytePointer pack,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zlatms( IntBuffer m, IntBuffer n, @Cast("char*") ByteBuffer dist, IntBuffer iseed,
@Cast("char*") ByteBuffer sym, DoubleBuffer d, IntBuffer mode, DoubleBuffer cond,
DoubleBuffer dmax, IntBuffer kl, IntBuffer ku, @Cast("char*") ByteBuffer pack,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zlatms( int[] m, int[] n, @Cast("char*") byte[] dist, int[] iseed,
@Cast("char*") byte[] sym, double[] d, int[] mode, double[] cond,
double[] dmax, int[] kl, int[] ku, @Cast("char*") byte[] pack,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_slag2d( IntPointer m, IntPointer n, @Const FloatPointer sa,
IntPointer ldsa, DoublePointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_slag2d( IntBuffer m, IntBuffer n, @Const FloatBuffer sa,
IntBuffer ldsa, DoubleBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_slag2d( int[] m, int[] n, @Const float[] sa,
int[] ldsa, double[] a, int[] lda,
int[] info );
public static native void LAPACK_dlag2s( IntPointer m, IntPointer n, @Const DoublePointer a,
IntPointer lda, FloatPointer sa, IntPointer ldsa,
IntPointer info );
public static native void LAPACK_dlag2s( IntBuffer m, IntBuffer n, @Const DoubleBuffer a,
IntBuffer lda, FloatBuffer sa, IntBuffer ldsa,
IntBuffer info );
public static native void LAPACK_dlag2s( int[] m, int[] n, @Const double[] a,
int[] lda, float[] sa, int[] ldsa,
int[] info );
public static native void LAPACK_clag2z( IntPointer m, IntPointer n,
@Cast("const lapack_complex_float*") FloatPointer sa, IntPointer ldsa,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_clag2z( IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_float*") FloatBuffer sa, IntBuffer ldsa,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_clag2z( int[] m, int[] n,
@Cast("const lapack_complex_float*") float[] sa, int[] ldsa,
@Cast("lapack_complex_double*") double[] a, int[] lda,
int[] info );
public static native void LAPACK_zlag2c( IntPointer m, IntPointer n,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer sa, IntPointer ldsa,
IntPointer info );
public static native void LAPACK_zlag2c( IntBuffer m, IntBuffer n,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer sa, IntBuffer ldsa,
IntBuffer info );
public static native void LAPACK_zlag2c( int[] m, int[] n,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_float*") float[] sa, int[] ldsa,
int[] info );
public static native void LAPACK_slauum( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_slauum( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_slauum( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
int[] info );
public static native void LAPACK_dlauum( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer info );
public static native void LAPACK_dlauum( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer info );
public static native void LAPACK_dlauum( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
int[] info );
public static native void LAPACK_clauum( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_clauum( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_clauum( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] info );
public static native void LAPACK_zlauum( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_zlauum( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_zlauum( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] info );
public static native void LAPACK_slagge( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Const FloatPointer d, FloatPointer a, IntPointer lda,
IntPointer iseed, FloatPointer work, IntPointer info );
public static native void LAPACK_slagge( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Const FloatBuffer d, FloatBuffer a, IntBuffer lda,
IntBuffer iseed, FloatBuffer work, IntBuffer info );
public static native void LAPACK_slagge( int[] m, int[] n, int[] kl,
int[] ku, @Const float[] d, float[] a, int[] lda,
int[] iseed, float[] work, int[] info );
public static native void LAPACK_dlagge( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Const DoublePointer d, DoublePointer a, IntPointer lda,
IntPointer iseed, DoublePointer work, IntPointer info );
public static native void LAPACK_dlagge( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Const DoubleBuffer d, DoubleBuffer a, IntBuffer lda,
IntBuffer iseed, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dlagge( int[] m, int[] n, int[] kl,
int[] ku, @Const double[] d, double[] a, int[] lda,
int[] iseed, double[] work, int[] info );
public static native void LAPACK_clagge( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Const FloatPointer d, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer iseed,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_clagge( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Const FloatBuffer d, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer iseed,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_clagge( int[] m, int[] n, int[] kl,
int[] ku, @Const float[] d, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] iseed,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zlagge( IntPointer m, IntPointer n, IntPointer kl,
IntPointer ku, @Const DoublePointer d, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer iseed,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zlagge( IntBuffer m, IntBuffer n, IntBuffer kl,
IntBuffer ku, @Const DoubleBuffer d, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer iseed,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zlagge( int[] m, int[] n, int[] kl,
int[] ku, @Const double[] d, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] iseed,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_slascl( @Cast("char*") BytePointer type, IntPointer kl, IntPointer ku, FloatPointer cfrom,
FloatPointer cto, IntPointer m, IntPointer n, FloatPointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_slascl( @Cast("char*") ByteBuffer type, IntBuffer kl, IntBuffer ku, FloatBuffer cfrom,
FloatBuffer cto, IntBuffer m, IntBuffer n, FloatBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_slascl( @Cast("char*") byte[] type, int[] kl, int[] ku, float[] cfrom,
float[] cto, int[] m, int[] n, float[] a,
int[] lda, int[] info );
public static native void LAPACK_dlascl( @Cast("char*") BytePointer type, IntPointer kl, IntPointer ku, DoublePointer cfrom,
DoublePointer cto, IntPointer m, IntPointer n, DoublePointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_dlascl( @Cast("char*") ByteBuffer type, IntBuffer kl, IntBuffer ku, DoubleBuffer cfrom,
DoubleBuffer cto, IntBuffer m, IntBuffer n, DoubleBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_dlascl( @Cast("char*") byte[] type, int[] kl, int[] ku, double[] cfrom,
double[] cto, int[] m, int[] n, double[] a,
int[] lda, int[] info );
public static native void LAPACK_clascl( @Cast("char*") BytePointer type, IntPointer kl, IntPointer ku, FloatPointer cfrom,
FloatPointer cto, IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_clascl( @Cast("char*") ByteBuffer type, IntBuffer kl, IntBuffer ku, FloatBuffer cfrom,
FloatBuffer cto, IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_clascl( @Cast("char*") byte[] type, int[] kl, int[] ku, float[] cfrom,
float[] cto, int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] info );
public static native void LAPACK_zlascl( @Cast("char*") BytePointer type, IntPointer kl, IntPointer ku, DoublePointer cfrom,
DoublePointer cto, IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer info );
public static native void LAPACK_zlascl( @Cast("char*") ByteBuffer type, IntBuffer kl, IntBuffer ku, DoubleBuffer cfrom,
DoubleBuffer cto, IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer info );
public static native void LAPACK_zlascl( @Cast("char*") byte[] type, int[] kl, int[] ku, double[] cfrom,
double[] cto, int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] info );
public static native void LAPACK_slaset( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n, FloatPointer alpha,
FloatPointer beta, FloatPointer a, IntPointer lda );
public static native void LAPACK_slaset( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n, FloatBuffer alpha,
FloatBuffer beta, FloatBuffer a, IntBuffer lda );
public static native void LAPACK_slaset( @Cast("char*") byte[] uplo, int[] m, int[] n, float[] alpha,
float[] beta, float[] a, int[] lda );
public static native void LAPACK_dlaset( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n, DoublePointer alpha,
DoublePointer beta, DoublePointer a, IntPointer lda );
public static native void LAPACK_dlaset( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n, DoubleBuffer alpha,
DoubleBuffer beta, DoubleBuffer a, IntBuffer lda );
public static native void LAPACK_dlaset( @Cast("char*") byte[] uplo, int[] m, int[] n, double[] alpha,
double[] beta, double[] a, int[] lda );
public static native void LAPACK_claset( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer alpha, @Cast("lapack_complex_float*") FloatPointer beta,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda );
public static native void LAPACK_claset( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer alpha, @Cast("lapack_complex_float*") FloatBuffer beta,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda );
public static native void LAPACK_claset( @Cast("char*") byte[] uplo, int[] m, int[] n,
@Cast("lapack_complex_float*") float[] alpha, @Cast("lapack_complex_float*") float[] beta,
@Cast("lapack_complex_float*") float[] a, int[] lda );
public static native void LAPACK_zlaset( @Cast("char*") BytePointer uplo, IntPointer m, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer alpha, @Cast("lapack_complex_double*") DoublePointer beta,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda );
public static native void LAPACK_zlaset( @Cast("char*") ByteBuffer uplo, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer alpha, @Cast("lapack_complex_double*") DoubleBuffer beta,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda );
public static native void LAPACK_zlaset( @Cast("char*") byte[] uplo, int[] m, int[] n,
@Cast("lapack_complex_double*") double[] alpha, @Cast("lapack_complex_double*") double[] beta,
@Cast("lapack_complex_double*") double[] a, int[] lda );
public static native void LAPACK_slasrt( @Cast("char*") BytePointer id, IntPointer n, FloatPointer d, IntPointer info );
public static native void LAPACK_slasrt( @Cast("char*") ByteBuffer id, IntBuffer n, FloatBuffer d, IntBuffer info );
public static native void LAPACK_slasrt( @Cast("char*") byte[] id, int[] n, float[] d, int[] info );
public static native void LAPACK_dlasrt( @Cast("char*") BytePointer id, IntPointer n, DoublePointer d, IntPointer info );
public static native void LAPACK_dlasrt( @Cast("char*") ByteBuffer id, IntBuffer n, DoubleBuffer d, IntBuffer info );
public static native void LAPACK_dlasrt( @Cast("char*") byte[] id, int[] n, double[] d, int[] info );
public static native void LAPACK_claghe( IntPointer n, IntPointer k, @Const FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, IntPointer iseed,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_claghe( IntBuffer n, IntBuffer k, @Const FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, IntBuffer iseed,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_claghe( int[] n, int[] k, @Const float[] d,
@Cast("lapack_complex_float*") float[] a, int[] lda, int[] iseed,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zlaghe( IntPointer n, IntPointer k, @Const DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
IntPointer iseed, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer info );
public static native void LAPACK_zlaghe( IntBuffer n, IntBuffer k, @Const DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
IntBuffer iseed, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zlaghe( int[] n, int[] k, @Const double[] d,
@Cast("lapack_complex_double*") double[] a, int[] lda,
int[] iseed, @Cast("lapack_complex_double*") double[] work,
int[] info );
public static native void LAPACK_slagsy( IntPointer n, IntPointer k, @Const FloatPointer d, FloatPointer a,
IntPointer lda, IntPointer iseed, FloatPointer work,
IntPointer info );
public static native void LAPACK_slagsy( IntBuffer n, IntBuffer k, @Const FloatBuffer d, FloatBuffer a,
IntBuffer lda, IntBuffer iseed, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_slagsy( int[] n, int[] k, @Const float[] d, float[] a,
int[] lda, int[] iseed, float[] work,
int[] info );
public static native void LAPACK_dlagsy( IntPointer n, IntPointer k, @Const DoublePointer d, DoublePointer a,
IntPointer lda, IntPointer iseed, DoublePointer work,
IntPointer info );
public static native void LAPACK_dlagsy( IntBuffer n, IntBuffer k, @Const DoubleBuffer d, DoubleBuffer a,
IntBuffer lda, IntBuffer iseed, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dlagsy( int[] n, int[] k, @Const double[] d, double[] a,
int[] lda, int[] iseed, double[] work,
int[] info );
public static native void LAPACK_clagsy( IntPointer n, IntPointer k, @Const FloatPointer d,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda, IntPointer iseed,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_clagsy( IntBuffer n, IntBuffer k, @Const FloatBuffer d,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda, IntBuffer iseed,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_clagsy( int[] n, int[] k, @Const float[] d,
@Cast("lapack_complex_float*") float[] a, int[] lda, int[] iseed,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zlagsy( IntPointer n, IntPointer k, @Const DoublePointer d,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
IntPointer iseed, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer info );
public static native void LAPACK_zlagsy( IntBuffer n, IntBuffer k, @Const DoubleBuffer d,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
IntBuffer iseed, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_zlagsy( int[] n, int[] k, @Const double[] d,
@Cast("lapack_complex_double*") double[] a, int[] lda,
int[] iseed, @Cast("lapack_complex_double*") double[] work,
int[] info );
public static native void LAPACK_slapmr( IntPointer forwrd, IntPointer m, IntPointer n,
FloatPointer x, IntPointer ldx, IntPointer k );
public static native void LAPACK_slapmr( IntBuffer forwrd, IntBuffer m, IntBuffer n,
FloatBuffer x, IntBuffer ldx, IntBuffer k );
public static native void LAPACK_slapmr( int[] forwrd, int[] m, int[] n,
float[] x, int[] ldx, int[] k );
public static native void LAPACK_dlapmr( IntPointer forwrd, IntPointer m, IntPointer n,
DoublePointer x, IntPointer ldx, IntPointer k );
public static native void LAPACK_dlapmr( IntBuffer forwrd, IntBuffer m, IntBuffer n,
DoubleBuffer x, IntBuffer ldx, IntBuffer k );
public static native void LAPACK_dlapmr( int[] forwrd, int[] m, int[] n,
double[] x, int[] ldx, int[] k );
public static native void LAPACK_clapmr( IntPointer forwrd, IntPointer m, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, IntPointer k );
public static native void LAPACK_clapmr( IntBuffer forwrd, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, IntBuffer k );
public static native void LAPACK_clapmr( int[] forwrd, int[] m, int[] n,
@Cast("lapack_complex_float*") float[] x, int[] ldx, int[] k );
public static native void LAPACK_zlapmr( IntPointer forwrd, IntPointer m, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, IntPointer k );
public static native void LAPACK_zlapmr( IntBuffer forwrd, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, IntBuffer k );
public static native void LAPACK_zlapmr( int[] forwrd, int[] m, int[] n,
@Cast("lapack_complex_double*") double[] x, int[] ldx, int[] k );
public static native void LAPACK_slapmt( IntPointer forwrd, IntPointer m, IntPointer n,
FloatPointer x, IntPointer ldx, IntPointer k );
public static native void LAPACK_slapmt( IntBuffer forwrd, IntBuffer m, IntBuffer n,
FloatBuffer x, IntBuffer ldx, IntBuffer k );
public static native void LAPACK_slapmt( int[] forwrd, int[] m, int[] n,
float[] x, int[] ldx, int[] k );
public static native void LAPACK_dlapmt( IntPointer forwrd, IntPointer m, IntPointer n,
DoublePointer x, IntPointer ldx, IntPointer k );
public static native void LAPACK_dlapmt( IntBuffer forwrd, IntBuffer m, IntBuffer n,
DoubleBuffer x, IntBuffer ldx, IntBuffer k );
public static native void LAPACK_dlapmt( int[] forwrd, int[] m, int[] n,
double[] x, int[] ldx, int[] k );
public static native void LAPACK_clapmt( IntPointer forwrd, IntPointer m, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer x, IntPointer ldx, IntPointer k );
public static native void LAPACK_clapmt( IntBuffer forwrd, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer x, IntBuffer ldx, IntBuffer k );
public static native void LAPACK_clapmt( int[] forwrd, int[] m, int[] n,
@Cast("lapack_complex_float*") float[] x, int[] ldx, int[] k );
public static native void LAPACK_zlapmt( IntPointer forwrd, IntPointer m, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer x, IntPointer ldx, IntPointer k );
public static native void LAPACK_zlapmt( IntBuffer forwrd, IntBuffer m, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer x, IntBuffer ldx, IntBuffer k );
public static native void LAPACK_zlapmt( int[] forwrd, int[] m, int[] n,
@Cast("lapack_complex_double*") double[] x, int[] ldx, int[] k );
public static native float LAPACK_slapy2( FloatPointer x, FloatPointer y );
public static native float LAPACK_slapy2( FloatBuffer x, FloatBuffer y );
public static native float LAPACK_slapy2( float[] x, float[] y );
public static native double LAPACK_dlapy2( DoublePointer x, DoublePointer y );
public static native double LAPACK_dlapy2( DoubleBuffer x, DoubleBuffer y );
public static native double LAPACK_dlapy2( double[] x, double[] y );
public static native float LAPACK_slapy3( FloatPointer x, FloatPointer y, FloatPointer z );
public static native float LAPACK_slapy3( FloatBuffer x, FloatBuffer y, FloatBuffer z );
public static native float LAPACK_slapy3( float[] x, float[] y, float[] z );
public static native double LAPACK_dlapy3( DoublePointer x, DoublePointer y, DoublePointer z );
public static native double LAPACK_dlapy3( DoubleBuffer x, DoubleBuffer y, DoubleBuffer z );
public static native double LAPACK_dlapy3( double[] x, double[] y, double[] z );
public static native void LAPACK_slartgp( FloatPointer f, FloatPointer g, FloatPointer cs, FloatPointer sn, FloatPointer r );
public static native void LAPACK_slartgp( FloatBuffer f, FloatBuffer g, FloatBuffer cs, FloatBuffer sn, FloatBuffer r );
public static native void LAPACK_slartgp( float[] f, float[] g, float[] cs, float[] sn, float[] r );
public static native void LAPACK_dlartgp( DoublePointer f, DoublePointer g, DoublePointer cs, DoublePointer sn, DoublePointer r );
public static native void LAPACK_dlartgp( DoubleBuffer f, DoubleBuffer g, DoubleBuffer cs, DoubleBuffer sn, DoubleBuffer r );
public static native void LAPACK_dlartgp( double[] f, double[] g, double[] cs, double[] sn, double[] r );
public static native void LAPACK_slartgs( FloatPointer x, FloatPointer y, FloatPointer sigma, FloatPointer cs, FloatPointer sn );
public static native void LAPACK_slartgs( FloatBuffer x, FloatBuffer y, FloatBuffer sigma, FloatBuffer cs, FloatBuffer sn );
public static native void LAPACK_slartgs( float[] x, float[] y, float[] sigma, float[] cs, float[] sn );
public static native void LAPACK_dlartgs( DoublePointer x, DoublePointer y, DoublePointer sigma, DoublePointer cs,
DoublePointer sn );
public static native void LAPACK_dlartgs( DoubleBuffer x, DoubleBuffer y, DoubleBuffer sigma, DoubleBuffer cs,
DoubleBuffer sn );
public static native void LAPACK_dlartgs( double[] x, double[] y, double[] sigma, double[] cs,
double[] sn );
// LAPACK 3.3.0
public static native void LAPACK_cbbcsd( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, @Cast("char*") BytePointer jobv2t, @Cast("char*") BytePointer trans,
IntPointer m, IntPointer p, IntPointer q,
FloatPointer theta, FloatPointer phi,
@Cast("lapack_complex_float*") FloatPointer u1, IntPointer ldu1,
@Cast("lapack_complex_float*") FloatPointer u2, IntPointer ldu2,
@Cast("lapack_complex_float*") FloatPointer v1t, IntPointer ldv1t,
@Cast("lapack_complex_float*") FloatPointer v2t, IntPointer ldv2t,
FloatPointer b11d, FloatPointer b11e, FloatPointer b12d,
FloatPointer b12e, FloatPointer b21d, FloatPointer b21e,
FloatPointer b22d, FloatPointer b22e, FloatPointer rwork,
IntPointer lrwork, IntPointer info );
public static native void LAPACK_cbbcsd( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, @Cast("char*") ByteBuffer jobv2t, @Cast("char*") ByteBuffer trans,
IntBuffer m, IntBuffer p, IntBuffer q,
FloatBuffer theta, FloatBuffer phi,
@Cast("lapack_complex_float*") FloatBuffer u1, IntBuffer ldu1,
@Cast("lapack_complex_float*") FloatBuffer u2, IntBuffer ldu2,
@Cast("lapack_complex_float*") FloatBuffer v1t, IntBuffer ldv1t,
@Cast("lapack_complex_float*") FloatBuffer v2t, IntBuffer ldv2t,
FloatBuffer b11d, FloatBuffer b11e, FloatBuffer b12d,
FloatBuffer b12e, FloatBuffer b21d, FloatBuffer b21e,
FloatBuffer b22d, FloatBuffer b22e, FloatBuffer rwork,
IntBuffer lrwork, IntBuffer info );
public static native void LAPACK_cbbcsd( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, @Cast("char*") byte[] jobv2t, @Cast("char*") byte[] trans,
int[] m, int[] p, int[] q,
float[] theta, float[] phi,
@Cast("lapack_complex_float*") float[] u1, int[] ldu1,
@Cast("lapack_complex_float*") float[] u2, int[] ldu2,
@Cast("lapack_complex_float*") float[] v1t, int[] ldv1t,
@Cast("lapack_complex_float*") float[] v2t, int[] ldv2t,
float[] b11d, float[] b11e, float[] b12d,
float[] b12e, float[] b21d, float[] b21e,
float[] b22d, float[] b22e, float[] rwork,
int[] lrwork, int[] info );
public static native void LAPACK_cheswapr( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer i1,
IntPointer i2 );
public static native void LAPACK_cheswapr( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer i1,
IntBuffer i2 );
public static native void LAPACK_cheswapr( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] i1,
int[] i2 );
public static native void LAPACK_chetri2( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_chetri2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_chetri2( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] lwork, int[] info );
public static native void LAPACK_chetri2x( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer nb, IntPointer info );
public static native void LAPACK_chetri2x( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer nb, IntBuffer info );
public static native void LAPACK_chetri2x( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] nb, int[] info );
public static native void LAPACK_chetrs2( @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_chetrs2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_chetrs2( @Cast("char*") byte[] uplo, int[] n,
int[] nrhs, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_csyconv( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer way,
IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_csyconv( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer way,
IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_csyconv( @Cast("char*") byte[] uplo, @Cast("char*") byte[] way,
int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_csyswapr( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer i1,
IntPointer i2 );
public static native void LAPACK_csyswapr( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer i1,
IntBuffer i2 );
public static native void LAPACK_csyswapr( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] i1,
int[] i2 );
public static native void LAPACK_csytri2( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_csytri2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_csytri2( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] lwork, int[] info );
public static native void LAPACK_csytri2x( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer nb, IntPointer info );
public static native void LAPACK_csytri2x( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer nb, IntBuffer info );
public static native void LAPACK_csytri2x( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] nb, int[] info );
public static native void LAPACK_csytrs2( @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer nrhs, @Cast("const lapack_complex_float*") FloatPointer a,
IntPointer lda, @Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_csytrs2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer nrhs, @Cast("const lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_csytrs2( @Cast("char*") byte[] uplo, int[] n,
int[] nrhs, @Cast("const lapack_complex_float*") float[] a,
int[] lda, @Const int[] ipiv,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_cunbdb( @Cast("char*") BytePointer trans, @Cast("char*") BytePointer signs,
IntPointer m, IntPointer p, IntPointer q,
@Cast("lapack_complex_float*") FloatPointer x11, IntPointer ldx11,
@Cast("lapack_complex_float*") FloatPointer x12, IntPointer ldx12,
@Cast("lapack_complex_float*") FloatPointer x21, IntPointer ldx21,
@Cast("lapack_complex_float*") FloatPointer x22, IntPointer ldx22,
FloatPointer theta, FloatPointer phi,
@Cast("lapack_complex_float*") FloatPointer taup1,
@Cast("lapack_complex_float*") FloatPointer taup2,
@Cast("lapack_complex_float*") FloatPointer tauq1,
@Cast("lapack_complex_float*") FloatPointer tauq2,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_cunbdb( @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer signs,
IntBuffer m, IntBuffer p, IntBuffer q,
@Cast("lapack_complex_float*") FloatBuffer x11, IntBuffer ldx11,
@Cast("lapack_complex_float*") FloatBuffer x12, IntBuffer ldx12,
@Cast("lapack_complex_float*") FloatBuffer x21, IntBuffer ldx21,
@Cast("lapack_complex_float*") FloatBuffer x22, IntBuffer ldx22,
FloatBuffer theta, FloatBuffer phi,
@Cast("lapack_complex_float*") FloatBuffer taup1,
@Cast("lapack_complex_float*") FloatBuffer taup2,
@Cast("lapack_complex_float*") FloatBuffer tauq1,
@Cast("lapack_complex_float*") FloatBuffer tauq2,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_cunbdb( @Cast("char*") byte[] trans, @Cast("char*") byte[] signs,
int[] m, int[] p, int[] q,
@Cast("lapack_complex_float*") float[] x11, int[] ldx11,
@Cast("lapack_complex_float*") float[] x12, int[] ldx12,
@Cast("lapack_complex_float*") float[] x21, int[] ldx21,
@Cast("lapack_complex_float*") float[] x22, int[] ldx22,
float[] theta, float[] phi,
@Cast("lapack_complex_float*") float[] taup1,
@Cast("lapack_complex_float*") float[] taup2,
@Cast("lapack_complex_float*") float[] tauq1,
@Cast("lapack_complex_float*") float[] tauq2,
@Cast("lapack_complex_float*") float[] work, int[] lwork, int[] info );
public static native void LAPACK_cuncsd( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, @Cast("char*") BytePointer jobv2t, @Cast("char*") BytePointer trans,
@Cast("char*") BytePointer signs, IntPointer m, IntPointer p,
IntPointer q, @Cast("lapack_complex_float*") FloatPointer x11,
IntPointer ldx11, @Cast("lapack_complex_float*") FloatPointer x12,
IntPointer ldx12, @Cast("lapack_complex_float*") FloatPointer x21,
IntPointer ldx21, @Cast("lapack_complex_float*") FloatPointer x22,
IntPointer ldx22, FloatPointer theta,
@Cast("lapack_complex_float*") FloatPointer u1, IntPointer ldu1,
@Cast("lapack_complex_float*") FloatPointer u2, IntPointer ldu2,
@Cast("lapack_complex_float*") FloatPointer v1t, IntPointer ldv1t,
@Cast("lapack_complex_float*") FloatPointer v2t, IntPointer ldv2t,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
FloatPointer rwork, IntPointer lrwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_cuncsd( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, @Cast("char*") ByteBuffer jobv2t, @Cast("char*") ByteBuffer trans,
@Cast("char*") ByteBuffer signs, IntBuffer m, IntBuffer p,
IntBuffer q, @Cast("lapack_complex_float*") FloatBuffer x11,
IntBuffer ldx11, @Cast("lapack_complex_float*") FloatBuffer x12,
IntBuffer ldx12, @Cast("lapack_complex_float*") FloatBuffer x21,
IntBuffer ldx21, @Cast("lapack_complex_float*") FloatBuffer x22,
IntBuffer ldx22, FloatBuffer theta,
@Cast("lapack_complex_float*") FloatBuffer u1, IntBuffer ldu1,
@Cast("lapack_complex_float*") FloatBuffer u2, IntBuffer ldu2,
@Cast("lapack_complex_float*") FloatBuffer v1t, IntBuffer ldv1t,
@Cast("lapack_complex_float*") FloatBuffer v2t, IntBuffer ldv2t,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
FloatBuffer rwork, IntBuffer lrwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_cuncsd( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, @Cast("char*") byte[] jobv2t, @Cast("char*") byte[] trans,
@Cast("char*") byte[] signs, int[] m, int[] p,
int[] q, @Cast("lapack_complex_float*") float[] x11,
int[] ldx11, @Cast("lapack_complex_float*") float[] x12,
int[] ldx12, @Cast("lapack_complex_float*") float[] x21,
int[] ldx21, @Cast("lapack_complex_float*") float[] x22,
int[] ldx22, float[] theta,
@Cast("lapack_complex_float*") float[] u1, int[] ldu1,
@Cast("lapack_complex_float*") float[] u2, int[] ldu2,
@Cast("lapack_complex_float*") float[] v1t, int[] ldv1t,
@Cast("lapack_complex_float*") float[] v2t, int[] ldv2t,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
float[] rwork, int[] lrwork,
int[] iwork, int[] info );
public static native void LAPACK_cuncsd2by1( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, IntPointer m, IntPointer p,
IntPointer q, @Cast("lapack_complex_float*") FloatPointer x11,
IntPointer ldx11, @Cast("lapack_complex_float*") FloatPointer x21,
IntPointer ldx21, @Cast("lapack_complex_float*") FloatPointer theta,
@Cast("lapack_complex_float*") FloatPointer u1, IntPointer ldu1,
@Cast("lapack_complex_float*") FloatPointer u2, IntPointer ldu2,
@Cast("lapack_complex_float*") FloatPointer v1t, IntPointer ldv1t,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
FloatPointer rwork, IntPointer lrwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_cuncsd2by1( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, IntBuffer m, IntBuffer p,
IntBuffer q, @Cast("lapack_complex_float*") FloatBuffer x11,
IntBuffer ldx11, @Cast("lapack_complex_float*") FloatBuffer x21,
IntBuffer ldx21, @Cast("lapack_complex_float*") FloatBuffer theta,
@Cast("lapack_complex_float*") FloatBuffer u1, IntBuffer ldu1,
@Cast("lapack_complex_float*") FloatBuffer u2, IntBuffer ldu2,
@Cast("lapack_complex_float*") FloatBuffer v1t, IntBuffer ldv1t,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
FloatBuffer rwork, IntBuffer lrwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_cuncsd2by1( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, int[] m, int[] p,
int[] q, @Cast("lapack_complex_float*") float[] x11,
int[] ldx11, @Cast("lapack_complex_float*") float[] x21,
int[] ldx21, @Cast("lapack_complex_float*") float[] theta,
@Cast("lapack_complex_float*") float[] u1, int[] ldu1,
@Cast("lapack_complex_float*") float[] u2, int[] ldu2,
@Cast("lapack_complex_float*") float[] v1t, int[] ldv1t,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
float[] rwork, int[] lrwork,
int[] iwork, int[] info );
public static native void LAPACK_dbbcsd( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, @Cast("char*") BytePointer jobv2t, @Cast("char*") BytePointer trans,
IntPointer m, IntPointer p, IntPointer q,
DoublePointer theta, DoublePointer phi, DoublePointer u1,
IntPointer ldu1, DoublePointer u2, IntPointer ldu2,
DoublePointer v1t, IntPointer ldv1t, DoublePointer v2t,
IntPointer ldv2t, DoublePointer b11d, DoublePointer b11e,
DoublePointer b12d, DoublePointer b12e, DoublePointer b21d,
DoublePointer b21e, DoublePointer b22d, DoublePointer b22e,
DoublePointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_dbbcsd( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, @Cast("char*") ByteBuffer jobv2t, @Cast("char*") ByteBuffer trans,
IntBuffer m, IntBuffer p, IntBuffer q,
DoubleBuffer theta, DoubleBuffer phi, DoubleBuffer u1,
IntBuffer ldu1, DoubleBuffer u2, IntBuffer ldu2,
DoubleBuffer v1t, IntBuffer ldv1t, DoubleBuffer v2t,
IntBuffer ldv2t, DoubleBuffer b11d, DoubleBuffer b11e,
DoubleBuffer b12d, DoubleBuffer b12e, DoubleBuffer b21d,
DoubleBuffer b21e, DoubleBuffer b22d, DoubleBuffer b22e,
DoubleBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dbbcsd( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, @Cast("char*") byte[] jobv2t, @Cast("char*") byte[] trans,
int[] m, int[] p, int[] q,
double[] theta, double[] phi, double[] u1,
int[] ldu1, double[] u2, int[] ldu2,
double[] v1t, int[] ldv1t, double[] v2t,
int[] ldv2t, double[] b11d, double[] b11e,
double[] b12d, double[] b12e, double[] b21d,
double[] b21e, double[] b22d, double[] b22e,
double[] work, int[] lwork, int[] info );
public static native void LAPACK_dorbdb( @Cast("char*") BytePointer trans, @Cast("char*") BytePointer signs,
IntPointer m, IntPointer p, IntPointer q,
DoublePointer x11, IntPointer ldx11, DoublePointer x12,
IntPointer ldx12, DoublePointer x21, IntPointer ldx21,
DoublePointer x22, IntPointer ldx22, DoublePointer theta,
DoublePointer phi, DoublePointer taup1, DoublePointer taup2,
DoublePointer tauq1, DoublePointer tauq2, DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_dorbdb( @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer signs,
IntBuffer m, IntBuffer p, IntBuffer q,
DoubleBuffer x11, IntBuffer ldx11, DoubleBuffer x12,
IntBuffer ldx12, DoubleBuffer x21, IntBuffer ldx21,
DoubleBuffer x22, IntBuffer ldx22, DoubleBuffer theta,
DoubleBuffer phi, DoubleBuffer taup1, DoubleBuffer taup2,
DoubleBuffer tauq1, DoubleBuffer tauq2, DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dorbdb( @Cast("char*") byte[] trans, @Cast("char*") byte[] signs,
int[] m, int[] p, int[] q,
double[] x11, int[] ldx11, double[] x12,
int[] ldx12, double[] x21, int[] ldx21,
double[] x22, int[] ldx22, double[] theta,
double[] phi, double[] taup1, double[] taup2,
double[] tauq1, double[] tauq2, double[] work,
int[] lwork, int[] info );
public static native void LAPACK_dorcsd( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, @Cast("char*") BytePointer jobv2t, @Cast("char*") BytePointer trans,
@Cast("char*") BytePointer signs, IntPointer m, IntPointer p,
IntPointer q, DoublePointer x11, IntPointer ldx11,
DoublePointer x12, IntPointer ldx12, DoublePointer x21,
IntPointer ldx21, DoublePointer x22, IntPointer ldx22,
DoublePointer theta, DoublePointer u1, IntPointer ldu1,
DoublePointer u2, IntPointer ldu2, DoublePointer v1t,
IntPointer ldv1t, DoublePointer v2t, IntPointer ldv2t,
DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dorcsd( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, @Cast("char*") ByteBuffer jobv2t, @Cast("char*") ByteBuffer trans,
@Cast("char*") ByteBuffer signs, IntBuffer m, IntBuffer p,
IntBuffer q, DoubleBuffer x11, IntBuffer ldx11,
DoubleBuffer x12, IntBuffer ldx12, DoubleBuffer x21,
IntBuffer ldx21, DoubleBuffer x22, IntBuffer ldx22,
DoubleBuffer theta, DoubleBuffer u1, IntBuffer ldu1,
DoubleBuffer u2, IntBuffer ldu2, DoubleBuffer v1t,
IntBuffer ldv1t, DoubleBuffer v2t, IntBuffer ldv2t,
DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dorcsd( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, @Cast("char*") byte[] jobv2t, @Cast("char*") byte[] trans,
@Cast("char*") byte[] signs, int[] m, int[] p,
int[] q, double[] x11, int[] ldx11,
double[] x12, int[] ldx12, double[] x21,
int[] ldx21, double[] x22, int[] ldx22,
double[] theta, double[] u1, int[] ldu1,
double[] u2, int[] ldu2, double[] v1t,
int[] ldv1t, double[] v2t, int[] ldv2t,
double[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_dorcsd2by1( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, IntPointer m, IntPointer p,
IntPointer q, DoublePointer x11, IntPointer ldx11,
DoublePointer x21, IntPointer ldx21,
DoublePointer theta, DoublePointer u1, IntPointer ldu1,
DoublePointer u2, IntPointer ldu2, DoublePointer v1t,
IntPointer ldv1t, DoublePointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_dorcsd2by1( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, IntBuffer m, IntBuffer p,
IntBuffer q, DoubleBuffer x11, IntBuffer ldx11,
DoubleBuffer x21, IntBuffer ldx21,
DoubleBuffer theta, DoubleBuffer u1, IntBuffer ldu1,
DoubleBuffer u2, IntBuffer ldu2, DoubleBuffer v1t,
IntBuffer ldv1t, DoubleBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_dorcsd2by1( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, int[] m, int[] p,
int[] q, double[] x11, int[] ldx11,
double[] x21, int[] ldx21,
double[] theta, double[] u1, int[] ldu1,
double[] u2, int[] ldu2, double[] v1t,
int[] ldv1t, double[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_dsyconv( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer way,
IntPointer n, DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, DoublePointer work, IntPointer info );
public static native void LAPACK_dsyconv( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer way,
IntBuffer n, DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dsyconv( @Cast("char*") byte[] uplo, @Cast("char*") byte[] way,
int[] n, double[] a, int[] lda,
@Const int[] ipiv, double[] work, int[] info );
public static native void LAPACK_dsyswapr( @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer a, IntPointer i1, IntPointer i2 );
public static native void LAPACK_dsyswapr( @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer a, IntBuffer i1, IntBuffer i2 );
public static native void LAPACK_dsyswapr( @Cast("char*") byte[] uplo, int[] n,
double[] a, int[] i1, int[] i2 );
public static native void LAPACK_dsytri2( @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_dsytri2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_dsytri2( @Cast("char*") byte[] uplo, int[] n,
double[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] lwork, int[] info );
public static native void LAPACK_dsytri2x( @Cast("char*") BytePointer uplo, IntPointer n,
DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, DoublePointer work,
IntPointer nb, IntPointer info );
public static native void LAPACK_dsytri2x( @Cast("char*") ByteBuffer uplo, IntBuffer n,
DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, DoubleBuffer work,
IntBuffer nb, IntBuffer info );
public static native void LAPACK_dsytri2x( @Cast("char*") byte[] uplo, int[] n,
double[] a, int[] lda,
@Const int[] ipiv, double[] work,
int[] nb, int[] info );
public static native void LAPACK_dsytrs2( @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer nrhs, @Const DoublePointer a,
IntPointer lda, @Const IntPointer ipiv,
DoublePointer b, IntPointer ldb, DoublePointer work, IntPointer info );
public static native void LAPACK_dsytrs2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer nrhs, @Const DoubleBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
DoubleBuffer b, IntBuffer ldb, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dsytrs2( @Cast("char*") byte[] uplo, int[] n,
int[] nrhs, @Const double[] a,
int[] lda, @Const int[] ipiv,
double[] b, int[] ldb, double[] work, int[] info );
public static native void LAPACK_sbbcsd( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, @Cast("char*") BytePointer jobv2t, @Cast("char*") BytePointer trans,
IntPointer m, IntPointer p, IntPointer q,
FloatPointer theta, FloatPointer phi, FloatPointer u1,
IntPointer ldu1, FloatPointer u2, IntPointer ldu2,
FloatPointer v1t, IntPointer ldv1t, FloatPointer v2t,
IntPointer ldv2t, FloatPointer b11d, FloatPointer b11e,
FloatPointer b12d, FloatPointer b12e, FloatPointer b21d,
FloatPointer b21e, FloatPointer b22d, FloatPointer b22e,
FloatPointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_sbbcsd( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, @Cast("char*") ByteBuffer jobv2t, @Cast("char*") ByteBuffer trans,
IntBuffer m, IntBuffer p, IntBuffer q,
FloatBuffer theta, FloatBuffer phi, FloatBuffer u1,
IntBuffer ldu1, FloatBuffer u2, IntBuffer ldu2,
FloatBuffer v1t, IntBuffer ldv1t, FloatBuffer v2t,
IntBuffer ldv2t, FloatBuffer b11d, FloatBuffer b11e,
FloatBuffer b12d, FloatBuffer b12e, FloatBuffer b21d,
FloatBuffer b21e, FloatBuffer b22d, FloatBuffer b22e,
FloatBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sbbcsd( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, @Cast("char*") byte[] jobv2t, @Cast("char*") byte[] trans,
int[] m, int[] p, int[] q,
float[] theta, float[] phi, float[] u1,
int[] ldu1, float[] u2, int[] ldu2,
float[] v1t, int[] ldv1t, float[] v2t,
int[] ldv2t, float[] b11d, float[] b11e,
float[] b12d, float[] b12e, float[] b21d,
float[] b21e, float[] b22d, float[] b22e,
float[] work, int[] lwork, int[] info );
public static native void LAPACK_sorbdb( @Cast("char*") BytePointer trans, @Cast("char*") BytePointer signs,
IntPointer m, IntPointer p, IntPointer q,
FloatPointer x11, IntPointer ldx11, FloatPointer x12,
IntPointer ldx12, FloatPointer x21, IntPointer ldx21,
FloatPointer x22, IntPointer ldx22, FloatPointer theta,
FloatPointer phi, FloatPointer taup1, FloatPointer taup2,
FloatPointer tauq1, FloatPointer tauq2, FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_sorbdb( @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer signs,
IntBuffer m, IntBuffer p, IntBuffer q,
FloatBuffer x11, IntBuffer ldx11, FloatBuffer x12,
IntBuffer ldx12, FloatBuffer x21, IntBuffer ldx21,
FloatBuffer x22, IntBuffer ldx22, FloatBuffer theta,
FloatBuffer phi, FloatBuffer taup1, FloatBuffer taup2,
FloatBuffer tauq1, FloatBuffer tauq2, FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_sorbdb( @Cast("char*") byte[] trans, @Cast("char*") byte[] signs,
int[] m, int[] p, int[] q,
float[] x11, int[] ldx11, float[] x12,
int[] ldx12, float[] x21, int[] ldx21,
float[] x22, int[] ldx22, float[] theta,
float[] phi, float[] taup1, float[] taup2,
float[] tauq1, float[] tauq2, float[] work,
int[] lwork, int[] info );
public static native void LAPACK_sorcsd( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, @Cast("char*") BytePointer jobv2t, @Cast("char*") BytePointer trans,
@Cast("char*") BytePointer signs, IntPointer m, IntPointer p,
IntPointer q, FloatPointer x11, IntPointer ldx11,
FloatPointer x12, IntPointer ldx12, FloatPointer x21,
IntPointer ldx21, FloatPointer x22, IntPointer ldx22,
FloatPointer theta, FloatPointer u1, IntPointer ldu1,
FloatPointer u2, IntPointer ldu2, FloatPointer v1t,
IntPointer ldv1t, FloatPointer v2t, IntPointer ldv2t,
FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_sorcsd( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, @Cast("char*") ByteBuffer jobv2t, @Cast("char*") ByteBuffer trans,
@Cast("char*") ByteBuffer signs, IntBuffer m, IntBuffer p,
IntBuffer q, FloatBuffer x11, IntBuffer ldx11,
FloatBuffer x12, IntBuffer ldx12, FloatBuffer x21,
IntBuffer ldx21, FloatBuffer x22, IntBuffer ldx22,
FloatBuffer theta, FloatBuffer u1, IntBuffer ldu1,
FloatBuffer u2, IntBuffer ldu2, FloatBuffer v1t,
IntBuffer ldv1t, FloatBuffer v2t, IntBuffer ldv2t,
FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sorcsd( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, @Cast("char*") byte[] jobv2t, @Cast("char*") byte[] trans,
@Cast("char*") byte[] signs, int[] m, int[] p,
int[] q, float[] x11, int[] ldx11,
float[] x12, int[] ldx12, float[] x21,
int[] ldx21, float[] x22, int[] ldx22,
float[] theta, float[] u1, int[] ldu1,
float[] u2, int[] ldu2, float[] v1t,
int[] ldv1t, float[] v2t, int[] ldv2t,
float[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_sorcsd2by1( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, IntPointer m, IntPointer p,
IntPointer q, FloatPointer x11, IntPointer ldx11,
FloatPointer x21, IntPointer ldx21,
FloatPointer theta, FloatPointer u1, IntPointer ldu1,
FloatPointer u2, IntPointer ldu2, FloatPointer v1t,
IntPointer ldv1t, FloatPointer work, IntPointer lwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_sorcsd2by1( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, IntBuffer m, IntBuffer p,
IntBuffer q, FloatBuffer x11, IntBuffer ldx11,
FloatBuffer x21, IntBuffer ldx21,
FloatBuffer theta, FloatBuffer u1, IntBuffer ldu1,
FloatBuffer u2, IntBuffer ldu2, FloatBuffer v1t,
IntBuffer ldv1t, FloatBuffer work, IntBuffer lwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_sorcsd2by1( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, int[] m, int[] p,
int[] q, float[] x11, int[] ldx11,
float[] x21, int[] ldx21,
float[] theta, float[] u1, int[] ldu1,
float[] u2, int[] ldu2, float[] v1t,
int[] ldv1t, float[] work, int[] lwork,
int[] iwork, int[] info );
public static native void LAPACK_ssyconv( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer way,
IntPointer n, FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, FloatPointer work, IntPointer info );
public static native void LAPACK_ssyconv( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer way,
IntBuffer n, FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, FloatBuffer work, IntBuffer info );
public static native void LAPACK_ssyconv( @Cast("char*") byte[] uplo, @Cast("char*") byte[] way,
int[] n, float[] a, int[] lda,
@Const int[] ipiv, float[] work, int[] info );
public static native void LAPACK_ssyswapr( @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer a, IntPointer i1, IntPointer i2 );
public static native void LAPACK_ssyswapr( @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer a, IntBuffer i1, IntBuffer i2 );
public static native void LAPACK_ssyswapr( @Cast("char*") byte[] uplo, int[] n,
float[] a, int[] i1, int[] i2 );
public static native void LAPACK_ssytri2( @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_ssytri2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_ssytri2( @Cast("char*") byte[] uplo, int[] n,
float[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] lwork, int[] info );
public static native void LAPACK_ssytri2x( @Cast("char*") BytePointer uplo, IntPointer n,
FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, FloatPointer work,
IntPointer nb, IntPointer info );
public static native void LAPACK_ssytri2x( @Cast("char*") ByteBuffer uplo, IntBuffer n,
FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, FloatBuffer work,
IntBuffer nb, IntBuffer info );
public static native void LAPACK_ssytri2x( @Cast("char*") byte[] uplo, int[] n,
float[] a, int[] lda,
@Const int[] ipiv, float[] work,
int[] nb, int[] info );
public static native void LAPACK_ssytrs2( @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer nrhs, @Const FloatPointer a,
IntPointer lda, @Const IntPointer ipiv,
FloatPointer b, IntPointer ldb, FloatPointer work, IntPointer info );
public static native void LAPACK_ssytrs2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer nrhs, @Const FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
FloatBuffer b, IntBuffer ldb, FloatBuffer work, IntBuffer info );
public static native void LAPACK_ssytrs2( @Cast("char*") byte[] uplo, int[] n,
int[] nrhs, @Const float[] a,
int[] lda, @Const int[] ipiv,
float[] b, int[] ldb, float[] work, int[] info );
public static native void LAPACK_zbbcsd( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, @Cast("char*") BytePointer jobv2t, @Cast("char*") BytePointer trans,
IntPointer m, IntPointer p, IntPointer q,
DoublePointer theta, DoublePointer phi,
@Cast("lapack_complex_double*") DoublePointer u1, IntPointer ldu1,
@Cast("lapack_complex_double*") DoublePointer u2, IntPointer ldu2,
@Cast("lapack_complex_double*") DoublePointer v1t, IntPointer ldv1t,
@Cast("lapack_complex_double*") DoublePointer v2t, IntPointer ldv2t,
DoublePointer b11d, DoublePointer b11e, DoublePointer b12d,
DoublePointer b12e, DoublePointer b21d, DoublePointer b21e,
DoublePointer b22d, DoublePointer b22e, DoublePointer rwork,
IntPointer lrwork, IntPointer info );
public static native void LAPACK_zbbcsd( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, @Cast("char*") ByteBuffer jobv2t, @Cast("char*") ByteBuffer trans,
IntBuffer m, IntBuffer p, IntBuffer q,
DoubleBuffer theta, DoubleBuffer phi,
@Cast("lapack_complex_double*") DoubleBuffer u1, IntBuffer ldu1,
@Cast("lapack_complex_double*") DoubleBuffer u2, IntBuffer ldu2,
@Cast("lapack_complex_double*") DoubleBuffer v1t, IntBuffer ldv1t,
@Cast("lapack_complex_double*") DoubleBuffer v2t, IntBuffer ldv2t,
DoubleBuffer b11d, DoubleBuffer b11e, DoubleBuffer b12d,
DoubleBuffer b12e, DoubleBuffer b21d, DoubleBuffer b21e,
DoubleBuffer b22d, DoubleBuffer b22e, DoubleBuffer rwork,
IntBuffer lrwork, IntBuffer info );
public static native void LAPACK_zbbcsd( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, @Cast("char*") byte[] jobv2t, @Cast("char*") byte[] trans,
int[] m, int[] p, int[] q,
double[] theta, double[] phi,
@Cast("lapack_complex_double*") double[] u1, int[] ldu1,
@Cast("lapack_complex_double*") double[] u2, int[] ldu2,
@Cast("lapack_complex_double*") double[] v1t, int[] ldv1t,
@Cast("lapack_complex_double*") double[] v2t, int[] ldv2t,
double[] b11d, double[] b11e, double[] b12d,
double[] b12e, double[] b21d, double[] b21e,
double[] b22d, double[] b22e, double[] rwork,
int[] lrwork, int[] info );
public static native void LAPACK_zheswapr( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer i1,
IntPointer i2 );
public static native void LAPACK_zheswapr( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer i1,
IntBuffer i2 );
public static native void LAPACK_zheswapr( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] i1,
int[] i2 );
public static native void LAPACK_zhetri2( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_zhetri2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zhetri2( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] lwork, int[] info );
public static native void LAPACK_zhetri2x( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer nb, IntPointer info );
public static native void LAPACK_zhetri2x( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer nb, IntBuffer info );
public static native void LAPACK_zhetri2x( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] nb, int[] info );
public static native void LAPACK_zhetrs2( @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zhetrs2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zhetrs2( @Cast("char*") byte[] uplo, int[] n,
int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_zsyconv( @Cast("char*") BytePointer uplo, @Cast("char*") BytePointer way,
IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zsyconv( @Cast("char*") ByteBuffer uplo, @Cast("char*") ByteBuffer way,
IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zsyconv( @Cast("char*") byte[] uplo, @Cast("char*") byte[] way,
int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_zsyswapr( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer i1,
IntPointer i2 );
public static native void LAPACK_zsyswapr( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer i1,
IntBuffer i2 );
public static native void LAPACK_zsyswapr( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] i1,
int[] i2 );
public static native void LAPACK_zsytri2( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_zsytri2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zsytri2( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] lwork, int[] info );
public static native void LAPACK_zsytri2x( @Cast("char*") BytePointer uplo, IntPointer n,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer nb, IntPointer info );
public static native void LAPACK_zsytri2x( @Cast("char*") ByteBuffer uplo, IntBuffer n,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer nb, IntBuffer info );
public static native void LAPACK_zsytri2x( @Cast("char*") byte[] uplo, int[] n,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] nb, int[] info );
public static native void LAPACK_zsytrs2( @Cast("char*") BytePointer uplo, IntPointer n,
IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zsytrs2( @Cast("char*") ByteBuffer uplo, IntBuffer n,
IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zsytrs2( @Cast("char*") byte[] uplo, int[] n,
int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_zunbdb( @Cast("char*") BytePointer trans, @Cast("char*") BytePointer signs,
IntPointer m, IntPointer p, IntPointer q,
@Cast("lapack_complex_double*") DoublePointer x11, IntPointer ldx11,
@Cast("lapack_complex_double*") DoublePointer x12, IntPointer ldx12,
@Cast("lapack_complex_double*") DoublePointer x21, IntPointer ldx21,
@Cast("lapack_complex_double*") DoublePointer x22, IntPointer ldx22,
DoublePointer theta, DoublePointer phi,
@Cast("lapack_complex_double*") DoublePointer taup1,
@Cast("lapack_complex_double*") DoublePointer taup2,
@Cast("lapack_complex_double*") DoublePointer tauq1,
@Cast("lapack_complex_double*") DoublePointer tauq2,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork, IntPointer info );
public static native void LAPACK_zunbdb( @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer signs,
IntBuffer m, IntBuffer p, IntBuffer q,
@Cast("lapack_complex_double*") DoubleBuffer x11, IntBuffer ldx11,
@Cast("lapack_complex_double*") DoubleBuffer x12, IntBuffer ldx12,
@Cast("lapack_complex_double*") DoubleBuffer x21, IntBuffer ldx21,
@Cast("lapack_complex_double*") DoubleBuffer x22, IntBuffer ldx22,
DoubleBuffer theta, DoubleBuffer phi,
@Cast("lapack_complex_double*") DoubleBuffer taup1,
@Cast("lapack_complex_double*") DoubleBuffer taup2,
@Cast("lapack_complex_double*") DoubleBuffer tauq1,
@Cast("lapack_complex_double*") DoubleBuffer tauq2,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zunbdb( @Cast("char*") byte[] trans, @Cast("char*") byte[] signs,
int[] m, int[] p, int[] q,
@Cast("lapack_complex_double*") double[] x11, int[] ldx11,
@Cast("lapack_complex_double*") double[] x12, int[] ldx12,
@Cast("lapack_complex_double*") double[] x21, int[] ldx21,
@Cast("lapack_complex_double*") double[] x22, int[] ldx22,
double[] theta, double[] phi,
@Cast("lapack_complex_double*") double[] taup1,
@Cast("lapack_complex_double*") double[] taup2,
@Cast("lapack_complex_double*") double[] tauq1,
@Cast("lapack_complex_double*") double[] tauq2,
@Cast("lapack_complex_double*") double[] work, int[] lwork, int[] info );
public static native void LAPACK_zuncsd( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, @Cast("char*") BytePointer jobv2t, @Cast("char*") BytePointer trans,
@Cast("char*") BytePointer signs, IntPointer m, IntPointer p,
IntPointer q, @Cast("lapack_complex_double*") DoublePointer x11,
IntPointer ldx11, @Cast("lapack_complex_double*") DoublePointer x12,
IntPointer ldx12, @Cast("lapack_complex_double*") DoublePointer x21,
IntPointer ldx21, @Cast("lapack_complex_double*") DoublePointer x22,
IntPointer ldx22, DoublePointer theta,
@Cast("lapack_complex_double*") DoublePointer u1, IntPointer ldu1,
@Cast("lapack_complex_double*") DoublePointer u2, IntPointer ldu2,
@Cast("lapack_complex_double*") DoublePointer v1t, IntPointer ldv1t,
@Cast("lapack_complex_double*") DoublePointer v2t, IntPointer ldv2t,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_zuncsd( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, @Cast("char*") ByteBuffer jobv2t, @Cast("char*") ByteBuffer trans,
@Cast("char*") ByteBuffer signs, IntBuffer m, IntBuffer p,
IntBuffer q, @Cast("lapack_complex_double*") DoubleBuffer x11,
IntBuffer ldx11, @Cast("lapack_complex_double*") DoubleBuffer x12,
IntBuffer ldx12, @Cast("lapack_complex_double*") DoubleBuffer x21,
IntBuffer ldx21, @Cast("lapack_complex_double*") DoubleBuffer x22,
IntBuffer ldx22, DoubleBuffer theta,
@Cast("lapack_complex_double*") DoubleBuffer u1, IntBuffer ldu1,
@Cast("lapack_complex_double*") DoubleBuffer u2, IntBuffer ldu2,
@Cast("lapack_complex_double*") DoubleBuffer v1t, IntBuffer ldv1t,
@Cast("lapack_complex_double*") DoubleBuffer v2t, IntBuffer ldv2t,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_zuncsd( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, @Cast("char*") byte[] jobv2t, @Cast("char*") byte[] trans,
@Cast("char*") byte[] signs, int[] m, int[] p,
int[] q, @Cast("lapack_complex_double*") double[] x11,
int[] ldx11, @Cast("lapack_complex_double*") double[] x12,
int[] ldx12, @Cast("lapack_complex_double*") double[] x21,
int[] ldx21, @Cast("lapack_complex_double*") double[] x22,
int[] ldx22, double[] theta,
@Cast("lapack_complex_double*") double[] u1, int[] ldu1,
@Cast("lapack_complex_double*") double[] u2, int[] ldu2,
@Cast("lapack_complex_double*") double[] v1t, int[] ldv1t,
@Cast("lapack_complex_double*") double[] v2t, int[] ldv2t,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork,
int[] iwork, int[] info );
public static native void LAPACK_zuncsd2by1( @Cast("char*") BytePointer jobu1, @Cast("char*") BytePointer jobu2,
@Cast("char*") BytePointer jobv1t, IntPointer m, IntPointer p,
IntPointer q, @Cast("lapack_complex_double*") DoublePointer x11,
IntPointer ldx11, @Cast("lapack_complex_double*") DoublePointer x21,
IntPointer ldx21, @Cast("lapack_complex_double*") DoublePointer theta,
@Cast("lapack_complex_double*") DoublePointer u1, IntPointer ldu1,
@Cast("lapack_complex_double*") DoublePointer u2, IntPointer ldu2,
@Cast("lapack_complex_double*") DoublePointer v1t, IntPointer ldv1t,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
DoublePointer rwork, IntPointer lrwork,
IntPointer iwork, IntPointer info );
public static native void LAPACK_zuncsd2by1( @Cast("char*") ByteBuffer jobu1, @Cast("char*") ByteBuffer jobu2,
@Cast("char*") ByteBuffer jobv1t, IntBuffer m, IntBuffer p,
IntBuffer q, @Cast("lapack_complex_double*") DoubleBuffer x11,
IntBuffer ldx11, @Cast("lapack_complex_double*") DoubleBuffer x21,
IntBuffer ldx21, @Cast("lapack_complex_double*") DoubleBuffer theta,
@Cast("lapack_complex_double*") DoubleBuffer u1, IntBuffer ldu1,
@Cast("lapack_complex_double*") DoubleBuffer u2, IntBuffer ldu2,
@Cast("lapack_complex_double*") DoubleBuffer v1t, IntBuffer ldv1t,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
DoubleBuffer rwork, IntBuffer lrwork,
IntBuffer iwork, IntBuffer info );
public static native void LAPACK_zuncsd2by1( @Cast("char*") byte[] jobu1, @Cast("char*") byte[] jobu2,
@Cast("char*") byte[] jobv1t, int[] m, int[] p,
int[] q, @Cast("lapack_complex_double*") double[] x11,
int[] ldx11, @Cast("lapack_complex_double*") double[] x21,
int[] ldx21, @Cast("lapack_complex_double*") double[] theta,
@Cast("lapack_complex_double*") double[] u1, int[] ldu1,
@Cast("lapack_complex_double*") double[] u2, int[] ldu2,
@Cast("lapack_complex_double*") double[] v1t, int[] ldv1t,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
double[] rwork, int[] lrwork,
int[] iwork, int[] info );
// LAPACK 3.4.0
public static native void LAPACK_sgemqrt( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer nb, @Const FloatPointer v,
IntPointer ldv, @Const FloatPointer t, IntPointer ldt, FloatPointer c,
IntPointer ldc, FloatPointer work, IntPointer info );
public static native void LAPACK_sgemqrt( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer nb, @Const FloatBuffer v,
IntBuffer ldv, @Const FloatBuffer t, IntBuffer ldt, FloatBuffer c,
IntBuffer ldc, FloatBuffer work, IntBuffer info );
public static native void LAPACK_sgemqrt( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] nb, @Const float[] v,
int[] ldv, @Const float[] t, int[] ldt, float[] c,
int[] ldc, float[] work, int[] info );
public static native void LAPACK_dgemqrt( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer nb, @Const DoublePointer v,
IntPointer ldv, @Const DoublePointer t, IntPointer ldt,
DoublePointer c, IntPointer ldc, DoublePointer work,
IntPointer info );
public static native void LAPACK_dgemqrt( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer nb, @Const DoubleBuffer v,
IntBuffer ldv, @Const DoubleBuffer t, IntBuffer ldt,
DoubleBuffer c, IntBuffer ldc, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dgemqrt( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] nb, @Const double[] v,
int[] ldv, @Const double[] t, int[] ldt,
double[] c, int[] ldc, double[] work,
int[] info );
public static native void LAPACK_cgemqrt( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer nb,
@Cast("const lapack_complex_float*") FloatPointer v, IntPointer ldv,
@Cast("const lapack_complex_float*") FloatPointer t, IntPointer ldt,
@Cast("lapack_complex_float*") FloatPointer c, IntPointer ldc,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_cgemqrt( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer nb,
@Cast("const lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
@Cast("lapack_complex_float*") FloatBuffer c, IntBuffer ldc,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_cgemqrt( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] nb,
@Cast("const lapack_complex_float*") float[] v, int[] ldv,
@Cast("const lapack_complex_float*") float[] t, int[] ldt,
@Cast("lapack_complex_float*") float[] c, int[] ldc,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zgemqrt( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer nb,
@Cast("const lapack_complex_double*") DoublePointer v, IntPointer ldv,
@Cast("const lapack_complex_double*") DoublePointer t, IntPointer ldt,
@Cast("lapack_complex_double*") DoublePointer c, IntPointer ldc,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zgemqrt( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer nb,
@Cast("const lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
@Cast("lapack_complex_double*") DoubleBuffer c, IntBuffer ldc,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zgemqrt( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] nb,
@Cast("const lapack_complex_double*") double[] v, int[] ldv,
@Cast("const lapack_complex_double*") double[] t, int[] ldt,
@Cast("lapack_complex_double*") double[] c, int[] ldc,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_sgeqrt( IntPointer m, IntPointer n, IntPointer nb, FloatPointer a,
IntPointer lda, FloatPointer t, IntPointer ldt, FloatPointer work,
IntPointer info );
public static native void LAPACK_sgeqrt( IntBuffer m, IntBuffer n, IntBuffer nb, FloatBuffer a,
IntBuffer lda, FloatBuffer t, IntBuffer ldt, FloatBuffer work,
IntBuffer info );
public static native void LAPACK_sgeqrt( int[] m, int[] n, int[] nb, float[] a,
int[] lda, float[] t, int[] ldt, float[] work,
int[] info );
public static native void LAPACK_dgeqrt( IntPointer m, IntPointer n, IntPointer nb, DoublePointer a,
IntPointer lda, DoublePointer t, IntPointer ldt, DoublePointer work,
IntPointer info );
public static native void LAPACK_dgeqrt( IntBuffer m, IntBuffer n, IntBuffer nb, DoubleBuffer a,
IntBuffer lda, DoubleBuffer t, IntBuffer ldt, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dgeqrt( int[] m, int[] n, int[] nb, double[] a,
int[] lda, double[] t, int[] ldt, double[] work,
int[] info );
public static native void LAPACK_cgeqrt( IntPointer m, IntPointer n, IntPointer nb,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer t, IntPointer ldt,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_cgeqrt( IntBuffer m, IntBuffer n, IntBuffer nb,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_cgeqrt( int[] m, int[] n, int[] nb,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] t, int[] ldt,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_zgeqrt( IntPointer m, IntPointer n, IntPointer nb,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer t, IntPointer ldt,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_zgeqrt( IntBuffer m, IntBuffer n, IntBuffer nb,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_zgeqrt( int[] m, int[] n, int[] nb,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] t, int[] ldt,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_sgeqrt2( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer t, IntPointer ldt, IntPointer info );
public static native void LAPACK_sgeqrt2( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer t, IntBuffer ldt, IntBuffer info );
public static native void LAPACK_sgeqrt2( int[] m, int[] n, float[] a, int[] lda,
float[] t, int[] ldt, int[] info );
public static native void LAPACK_dgeqrt2( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer t, IntPointer ldt, IntPointer info );
public static native void LAPACK_dgeqrt2( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer t, IntBuffer ldt, IntBuffer info );
public static native void LAPACK_dgeqrt2( int[] m, int[] n, double[] a, int[] lda,
double[] t, int[] ldt, int[] info );
public static native void LAPACK_cgeqrt2( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer t, IntPointer ldt,
IntPointer info );
public static native void LAPACK_cgeqrt2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
IntBuffer info );
public static native void LAPACK_cgeqrt2( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] t, int[] ldt,
int[] info );
public static native void LAPACK_zgeqrt2( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer t, IntPointer ldt,
IntPointer info );
public static native void LAPACK_zgeqrt2( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
IntBuffer info );
public static native void LAPACK_zgeqrt2( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] t, int[] ldt,
int[] info );
public static native void LAPACK_sgeqrt3( IntPointer m, IntPointer n, FloatPointer a, IntPointer lda,
FloatPointer t, IntPointer ldt, IntPointer info );
public static native void LAPACK_sgeqrt3( IntBuffer m, IntBuffer n, FloatBuffer a, IntBuffer lda,
FloatBuffer t, IntBuffer ldt, IntBuffer info );
public static native void LAPACK_sgeqrt3( int[] m, int[] n, float[] a, int[] lda,
float[] t, int[] ldt, int[] info );
public static native void LAPACK_dgeqrt3( IntPointer m, IntPointer n, DoublePointer a, IntPointer lda,
DoublePointer t, IntPointer ldt, IntPointer info );
public static native void LAPACK_dgeqrt3( IntBuffer m, IntBuffer n, DoubleBuffer a, IntBuffer lda,
DoubleBuffer t, IntBuffer ldt, IntBuffer info );
public static native void LAPACK_dgeqrt3( int[] m, int[] n, double[] a, int[] lda,
double[] t, int[] ldt, int[] info );
public static native void LAPACK_cgeqrt3( IntPointer m, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, @Cast("lapack_complex_float*") FloatPointer t, IntPointer ldt,
IntPointer info );
public static native void LAPACK_cgeqrt3( IntBuffer m, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, @Cast("lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
IntBuffer info );
public static native void LAPACK_cgeqrt3( int[] m, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, @Cast("lapack_complex_float*") float[] t, int[] ldt,
int[] info );
public static native void LAPACK_zgeqrt3( IntPointer m, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, @Cast("lapack_complex_double*") DoublePointer t, IntPointer ldt,
IntPointer info );
public static native void LAPACK_zgeqrt3( IntBuffer m, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, @Cast("lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
IntBuffer info );
public static native void LAPACK_zgeqrt3( int[] m, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, @Cast("lapack_complex_double*") double[] t, int[] ldt,
int[] info );
public static native void LAPACK_stpmqrt( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer l, IntPointer nb,
@Const FloatPointer v, IntPointer ldv, @Const FloatPointer t,
IntPointer ldt, FloatPointer a, IntPointer lda, FloatPointer b,
IntPointer ldb, FloatPointer work, IntPointer info );
public static native void LAPACK_stpmqrt( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer l, IntBuffer nb,
@Const FloatBuffer v, IntBuffer ldv, @Const FloatBuffer t,
IntBuffer ldt, FloatBuffer a, IntBuffer lda, FloatBuffer b,
IntBuffer ldb, FloatBuffer work, IntBuffer info );
public static native void LAPACK_stpmqrt( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] l, int[] nb,
@Const float[] v, int[] ldv, @Const float[] t,
int[] ldt, float[] a, int[] lda, float[] b,
int[] ldb, float[] work, int[] info );
public static native void LAPACK_dtpmqrt( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer l, IntPointer nb,
@Const DoublePointer v, IntPointer ldv, @Const DoublePointer t,
IntPointer ldt, DoublePointer a, IntPointer lda, DoublePointer b,
IntPointer ldb, DoublePointer work, IntPointer info );
public static native void LAPACK_dtpmqrt( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer l, IntBuffer nb,
@Const DoubleBuffer v, IntBuffer ldv, @Const DoubleBuffer t,
IntBuffer ldt, DoubleBuffer a, IntBuffer lda, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer work, IntBuffer info );
public static native void LAPACK_dtpmqrt( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] l, int[] nb,
@Const double[] v, int[] ldv, @Const double[] t,
int[] ldt, double[] a, int[] lda, double[] b,
int[] ldb, double[] work, int[] info );
public static native void LAPACK_ctpmqrt( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer l, IntPointer nb,
@Cast("const lapack_complex_float*") FloatPointer v, IntPointer ldv,
@Cast("const lapack_complex_float*") FloatPointer t, IntPointer ldt,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_ctpmqrt( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer l, IntBuffer nb,
@Cast("const lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_ctpmqrt( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] l, int[] nb,
@Cast("const lapack_complex_float*") float[] v, int[] ldv,
@Cast("const lapack_complex_float*") float[] t, int[] ldt,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_ztpmqrt( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, IntPointer m, IntPointer n,
IntPointer k, IntPointer l, IntPointer nb,
@Cast("const lapack_complex_double*") DoublePointer v, IntPointer ldv,
@Cast("const lapack_complex_double*") DoublePointer t, IntPointer ldt,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_ztpmqrt( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, IntBuffer m, IntBuffer n,
IntBuffer k, IntBuffer l, IntBuffer nb,
@Cast("const lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_ztpmqrt( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, int[] m, int[] n,
int[] k, int[] l, int[] nb,
@Cast("const lapack_complex_double*") double[] v, int[] ldv,
@Cast("const lapack_complex_double*") double[] t, int[] ldt,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_stpqrt( IntPointer m, IntPointer n, IntPointer l, IntPointer nb,
FloatPointer a, IntPointer lda, FloatPointer b, IntPointer ldb,
FloatPointer t, IntPointer ldt, FloatPointer work, IntPointer info );
public static native void LAPACK_stpqrt( IntBuffer m, IntBuffer n, IntBuffer l, IntBuffer nb,
FloatBuffer a, IntBuffer lda, FloatBuffer b, IntBuffer ldb,
FloatBuffer t, IntBuffer ldt, FloatBuffer work, IntBuffer info );
public static native void LAPACK_stpqrt( int[] m, int[] n, int[] l, int[] nb,
float[] a, int[] lda, float[] b, int[] ldb,
float[] t, int[] ldt, float[] work, int[] info );
public static native void LAPACK_dtpqrt( IntPointer m, IntPointer n, IntPointer l, IntPointer nb,
DoublePointer a, IntPointer lda, DoublePointer b, IntPointer ldb,
DoublePointer t, IntPointer ldt, DoublePointer work,
IntPointer info );
public static native void LAPACK_dtpqrt( IntBuffer m, IntBuffer n, IntBuffer l, IntBuffer nb,
DoubleBuffer a, IntBuffer lda, DoubleBuffer b, IntBuffer ldb,
DoubleBuffer t, IntBuffer ldt, DoubleBuffer work,
IntBuffer info );
public static native void LAPACK_dtpqrt( int[] m, int[] n, int[] l, int[] nb,
double[] a, int[] lda, double[] b, int[] ldb,
double[] t, int[] ldt, double[] work,
int[] info );
public static native void LAPACK_ctpqrt( IntPointer m, IntPointer n, IntPointer l, IntPointer nb,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer t, IntPointer ldt,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer info );
public static native void LAPACK_ctpqrt( IntBuffer m, IntBuffer n, IntBuffer l, IntBuffer nb,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer info );
public static native void LAPACK_ctpqrt( int[] m, int[] n, int[] l, int[] nb,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] t, int[] ldt,
@Cast("lapack_complex_float*") float[] work, int[] info );
public static native void LAPACK_ztpqrt( IntPointer m, IntPointer n, IntPointer l, IntPointer nb,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer t, IntPointer ldt,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer info );
public static native void LAPACK_ztpqrt( IntBuffer m, IntBuffer n, IntBuffer l, IntBuffer nb,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer info );
public static native void LAPACK_ztpqrt( int[] m, int[] n, int[] l, int[] nb,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] t, int[] ldt,
@Cast("lapack_complex_double*") double[] work, int[] info );
public static native void LAPACK_stpqrt2( IntPointer m, IntPointer n, IntPointer l,
FloatPointer a, IntPointer lda,
FloatPointer b, IntPointer ldb,
FloatPointer t, IntPointer ldt,
IntPointer info );
public static native void LAPACK_stpqrt2( IntBuffer m, IntBuffer n, IntBuffer l,
FloatBuffer a, IntBuffer lda,
FloatBuffer b, IntBuffer ldb,
FloatBuffer t, IntBuffer ldt,
IntBuffer info );
public static native void LAPACK_stpqrt2( int[] m, int[] n, int[] l,
float[] a, int[] lda,
float[] b, int[] ldb,
float[] t, int[] ldt,
int[] info );
public static native void LAPACK_dtpqrt2( IntPointer m, IntPointer n, IntPointer l,
DoublePointer a, IntPointer lda,
DoublePointer b, IntPointer ldb,
DoublePointer t, IntPointer ldt,
IntPointer info );
public static native void LAPACK_dtpqrt2( IntBuffer m, IntBuffer n, IntBuffer l,
DoubleBuffer a, IntBuffer lda,
DoubleBuffer b, IntBuffer ldb,
DoubleBuffer t, IntBuffer ldt,
IntBuffer info );
public static native void LAPACK_dtpqrt2( int[] m, int[] n, int[] l,
double[] a, int[] lda,
double[] b, int[] ldb,
double[] t, int[] ldt,
int[] info );
public static native void LAPACK_ctpqrt2( IntPointer m, IntPointer n, IntPointer l,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer t, IntPointer ldt,
IntPointer info );
public static native void LAPACK_ctpqrt2( IntBuffer m, IntBuffer n, IntBuffer l,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
IntBuffer info );
public static native void LAPACK_ctpqrt2( int[] m, int[] n, int[] l,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] t, int[] ldt,
int[] info );
public static native void LAPACK_ztpqrt2( IntPointer m, IntPointer n, IntPointer l,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer t, IntPointer ldt,
IntPointer info );
public static native void LAPACK_ztpqrt2( IntBuffer m, IntBuffer n, IntBuffer l,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
IntBuffer info );
public static native void LAPACK_ztpqrt2( int[] m, int[] n, int[] l,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] t, int[] ldt,
int[] info );
public static native void LAPACK_stprfb( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev,
IntPointer m, IntPointer n, IntPointer k, IntPointer l,
@Const FloatPointer v, IntPointer ldv, @Const FloatPointer t,
IntPointer ldt, FloatPointer a, IntPointer lda, FloatPointer b,
IntPointer ldb, @Const FloatPointer work,
IntPointer ldwork );
public static native void LAPACK_stprfb( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev,
IntBuffer m, IntBuffer n, IntBuffer k, IntBuffer l,
@Const FloatBuffer v, IntBuffer ldv, @Const FloatBuffer t,
IntBuffer ldt, FloatBuffer a, IntBuffer lda, FloatBuffer b,
IntBuffer ldb, @Const FloatBuffer work,
IntBuffer ldwork );
public static native void LAPACK_stprfb( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, @Cast("char*") byte[] direct, @Cast("char*") byte[] storev,
int[] m, int[] n, int[] k, int[] l,
@Const float[] v, int[] ldv, @Const float[] t,
int[] ldt, float[] a, int[] lda, float[] b,
int[] ldb, @Const float[] work,
int[] ldwork );
public static native void LAPACK_dtprfb( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev,
IntPointer m, IntPointer n, IntPointer k, IntPointer l,
@Const DoublePointer v, IntPointer ldv, @Const DoublePointer t,
IntPointer ldt, DoublePointer a, IntPointer lda, DoublePointer b,
IntPointer ldb, @Const DoublePointer work,
IntPointer ldwork );
public static native void LAPACK_dtprfb( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev,
IntBuffer m, IntBuffer n, IntBuffer k, IntBuffer l,
@Const DoubleBuffer v, IntBuffer ldv, @Const DoubleBuffer t,
IntBuffer ldt, DoubleBuffer a, IntBuffer lda, DoubleBuffer b,
IntBuffer ldb, @Const DoubleBuffer work,
IntBuffer ldwork );
public static native void LAPACK_dtprfb( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, @Cast("char*") byte[] direct, @Cast("char*") byte[] storev,
int[] m, int[] n, int[] k, int[] l,
@Const double[] v, int[] ldv, @Const double[] t,
int[] ldt, double[] a, int[] lda, double[] b,
int[] ldb, @Const double[] work,
int[] ldwork );
public static native void LAPACK_ctprfb( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev,
IntPointer m, IntPointer n, IntPointer k, IntPointer l,
@Cast("const lapack_complex_float*") FloatPointer v, IntPointer ldv,
@Cast("const lapack_complex_float*") FloatPointer t, IntPointer ldt,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
@Cast("lapack_complex_float*") FloatPointer b, IntPointer ldb,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer ldwork );
public static native void LAPACK_ctprfb( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev,
IntBuffer m, IntBuffer n, IntBuffer k, IntBuffer l,
@Cast("const lapack_complex_float*") FloatBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_float*") FloatBuffer t, IntBuffer ldt,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Cast("lapack_complex_float*") FloatBuffer b, IntBuffer ldb,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer ldwork );
public static native void LAPACK_ctprfb( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, @Cast("char*") byte[] direct, @Cast("char*") byte[] storev,
int[] m, int[] n, int[] k, int[] l,
@Cast("const lapack_complex_float*") float[] v, int[] ldv,
@Cast("const lapack_complex_float*") float[] t, int[] ldt,
@Cast("lapack_complex_float*") float[] a, int[] lda,
@Cast("lapack_complex_float*") float[] b, int[] ldb,
@Cast("lapack_complex_float*") float[] work, int[] ldwork );
public static native void LAPACK_ztprfb( @Cast("char*") BytePointer side, @Cast("char*") BytePointer trans, @Cast("char*") BytePointer direct, @Cast("char*") BytePointer storev,
IntPointer m, IntPointer n, IntPointer k, IntPointer l,
@Cast("const lapack_complex_double*") DoublePointer v, IntPointer ldv,
@Cast("const lapack_complex_double*") DoublePointer t, IntPointer ldt,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
@Cast("lapack_complex_double*") DoublePointer b, IntPointer ldb,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer ldwork );
public static native void LAPACK_ztprfb( @Cast("char*") ByteBuffer side, @Cast("char*") ByteBuffer trans, @Cast("char*") ByteBuffer direct, @Cast("char*") ByteBuffer storev,
IntBuffer m, IntBuffer n, IntBuffer k, IntBuffer l,
@Cast("const lapack_complex_double*") DoubleBuffer v, IntBuffer ldv,
@Cast("const lapack_complex_double*") DoubleBuffer t, IntBuffer ldt,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Cast("lapack_complex_double*") DoubleBuffer b, IntBuffer ldb,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer ldwork );
public static native void LAPACK_ztprfb( @Cast("char*") byte[] side, @Cast("char*") byte[] trans, @Cast("char*") byte[] direct, @Cast("char*") byte[] storev,
int[] m, int[] n, int[] k, int[] l,
@Cast("const lapack_complex_double*") double[] v, int[] ldv,
@Cast("const lapack_complex_double*") double[] t, int[] ldt,
@Cast("lapack_complex_double*") double[] a, int[] lda,
@Cast("lapack_complex_double*") double[] b, int[] ldb,
@Cast("lapack_complex_double*") double[] work, int[] ldwork );
// LAPACK 3.5.0
public static native void LAPACK_ssysv_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, FloatPointer a,
IntPointer lda, IntPointer ipiv, FloatPointer b,
IntPointer ldb, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_ssysv_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, FloatBuffer a,
IntBuffer lda, IntBuffer ipiv, FloatBuffer b,
IntBuffer ldb, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_ssysv_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, float[] a,
int[] lda, int[] ipiv, float[] b,
int[] ldb, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_ssytrf_rook( @Cast("char*") BytePointer uplo, IntPointer n, FloatPointer a, IntPointer lda,
IntPointer ipiv, FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_ssytrf_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, FloatBuffer a, IntBuffer lda,
IntBuffer ipiv, FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_ssytrf_rook( @Cast("char*") byte[] uplo, int[] n, float[] a, int[] lda,
int[] ipiv, float[] work, int[] lwork,
int[] info );
public static native void LAPACK_dsysv_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, DoublePointer a,
IntPointer lda, IntPointer ipiv, DoublePointer b,
IntPointer ldb, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dsysv_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv, DoubleBuffer b,
IntBuffer ldb, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dsysv_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, double[] a,
int[] lda, int[] ipiv, double[] b,
int[] ldb, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_dsytrf_rook( @Cast("char*") BytePointer uplo, IntPointer n, DoublePointer a, IntPointer lda,
IntPointer ipiv, DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_dsytrf_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, DoubleBuffer a, IntBuffer lda,
IntBuffer ipiv, DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_dsytrf_rook( @Cast("char*") byte[] uplo, int[] n, double[] a, int[] lda,
int[] ipiv, double[] work, int[] lwork,
int[] info );
public static native void LAPACK_csysv_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda,
IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, @Cast("lapack_complex_float*") FloatPointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_csysv_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda,
IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, @Cast("lapack_complex_float*") FloatBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_csysv_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_float*") float[] a, int[] lda,
int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int[] ldb, @Cast("lapack_complex_float*") float[] work,
int[] lwork, int[] info );
public static native void LAPACK_csytrf_rook( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_csytrf_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_csytrf_rook( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zsysv_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda,
IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, @Cast("lapack_complex_double*") DoublePointer work,
IntPointer lwork, IntPointer info );
public static native void LAPACK_zsysv_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, @Cast("lapack_complex_double*") DoubleBuffer work,
IntBuffer lwork, IntBuffer info );
public static native void LAPACK_zsysv_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("lapack_complex_double*") double[] a, int[] lda,
int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int[] ldb, @Cast("lapack_complex_double*") double[] work,
int[] lwork, int[] info );
public static native void LAPACK_zsytrf_rook( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zsytrf_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zsytrf_rook( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_ssytrs_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs, @Const FloatPointer a,
IntPointer lda, @Const IntPointer ipiv, FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_ssytrs_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs, @Const FloatBuffer a,
IntBuffer lda, @Const IntBuffer ipiv, FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_ssytrs_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs, @Const float[] a,
int[] lda, @Const int[] ipiv, float[] b,
int[] ldb, int[] info );
public static native void LAPACK_dsytrs_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Const DoublePointer a, IntPointer lda, @Const IntPointer ipiv,
DoublePointer b, IntPointer ldb, IntPointer info );
public static native void LAPACK_dsytrs_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Const DoubleBuffer a, IntBuffer lda, @Const IntBuffer ipiv,
DoubleBuffer b, IntBuffer ldb, IntBuffer info );
public static native void LAPACK_dsytrs_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Const double[] a, int[] lda, @Const int[] ipiv,
double[] b, int[] ldb, int[] info );
public static native void LAPACK_csytrs_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_csytrs_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_csytrs_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zsytrs_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zsytrs_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zsytrs_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_chetrf_rook( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer a,
IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_float*") FloatPointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_chetrf_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer a,
IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_float*") FloatBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_chetrf_rook( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] a,
int[] lda, int[] ipiv,
@Cast("lapack_complex_float*") float[] work, int[] lwork,
int[] info );
public static native void LAPACK_zhetrf_rook( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer a,
IntPointer lda, IntPointer ipiv,
@Cast("lapack_complex_double*") DoublePointer work, IntPointer lwork,
IntPointer info );
public static native void LAPACK_zhetrf_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer a,
IntBuffer lda, IntBuffer ipiv,
@Cast("lapack_complex_double*") DoubleBuffer work, IntBuffer lwork,
IntBuffer info );
public static native void LAPACK_zhetrf_rook( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] a,
int[] lda, int[] ipiv,
@Cast("lapack_complex_double*") double[] work, int[] lwork,
int[] info );
public static native void LAPACK_chetrs_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_float*") FloatPointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_float*") FloatPointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_chetrs_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_float*") FloatBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_float*") FloatBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_chetrs_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_float*") float[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_float*") float[] b,
int[] ldb, int[] info );
public static native void LAPACK_zhetrs_rook( @Cast("char*") BytePointer uplo, IntPointer n, IntPointer nrhs,
@Cast("const lapack_complex_double*") DoublePointer a, IntPointer lda,
@Const IntPointer ipiv, @Cast("lapack_complex_double*") DoublePointer b,
IntPointer ldb, IntPointer info );
public static native void LAPACK_zhetrs_rook( @Cast("char*") ByteBuffer uplo, IntBuffer n, IntBuffer nrhs,
@Cast("const lapack_complex_double*") DoubleBuffer a, IntBuffer lda,
@Const IntBuffer ipiv, @Cast("lapack_complex_double*") DoubleBuffer b,
IntBuffer ldb, IntBuffer info );
public static native void LAPACK_zhetrs_rook( @Cast("char*") byte[] uplo, int[] n, int[] nrhs,
@Cast("const lapack_complex_double*") double[] a, int[] lda,
@Const int[] ipiv, @Cast("lapack_complex_double*") double[] b,
int[] ldb, int[] info );
public static native void LAPACK_csyr( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_float*") FloatPointer alpha,
@Cast("const lapack_complex_float*") FloatPointer x, IntPointer incx,
@Cast("lapack_complex_float*") FloatPointer a, IntPointer lda );
public static native void LAPACK_csyr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_float*") FloatBuffer alpha,
@Cast("const lapack_complex_float*") FloatBuffer x, IntBuffer incx,
@Cast("lapack_complex_float*") FloatBuffer a, IntBuffer lda );
public static native void LAPACK_csyr( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_float*") float[] alpha,
@Cast("const lapack_complex_float*") float[] x, int[] incx,
@Cast("lapack_complex_float*") float[] a, int[] lda );
public static native void LAPACK_zsyr( @Cast("char*") BytePointer uplo, IntPointer n, @Cast("lapack_complex_double*") DoublePointer alpha,
@Cast("const lapack_complex_double*") DoublePointer x, IntPointer incx,
@Cast("lapack_complex_double*") DoublePointer a, IntPointer lda );
public static native void LAPACK_zsyr( @Cast("char*") ByteBuffer uplo, IntBuffer n, @Cast("lapack_complex_double*") DoubleBuffer alpha,
@Cast("const lapack_complex_double*") DoubleBuffer x, IntBuffer incx,
@Cast("lapack_complex_double*") DoubleBuffer a, IntBuffer lda );
public static native void LAPACK_zsyr( @Cast("char*") byte[] uplo, int[] n, @Cast("lapack_complex_double*") double[] alpha,
@Cast("const lapack_complex_double*") double[] x, int[] incx,
@Cast("lapack_complex_double*") double[] a, int[] lda );
public static native void LAPACK_ilaver( @Const IntPointer vers_major, @Const IntPointer vers_minor,
@Const IntPointer vers_patch );
public static native void LAPACK_ilaver( @Const IntBuffer vers_major, @Const IntBuffer vers_minor,
@Const IntBuffer vers_patch );
public static native void LAPACK_ilaver( @Const int[] vers_major, @Const int[] vers_minor,
@Const int[] vers_patch );
// #ifdef __cplusplus
// #endif /* __cplusplus */
// #endif /* _LAPACKE_H_ */
// Parsed from lapacke_utils.h
/*****************************************************************************
Copyright (c) 2014, Intel Corp.
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice,
this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
* Neither the name of Intel Corporation nor the names of its contributors
may be used to endorse or promote products derived from this software
without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF
THE POSSIBILITY OF SUCH DAMAGE.
******************************************************************************
* Contents: Native C interface to LAPACK utility functions
* Author: Intel Corporation
* Created in January, 2010
*****************************************************************************/
// #ifndef _LAPACKE_UTILS_H_
// #define _LAPACKE_UTILS_H_
// #include "lapacke.h"
// #ifdef __cplusplus
// #endif /* __cplusplus */
// #ifndef ABS
// #define ABS(x) (((x) < 0) ? -(x) : (x))
// #endif
// #ifndef MAX
// #define MAX(x,y) (((x) > (y)) ? (x) : (y))
// #endif
// #ifndef MIN
// #define MIN(x,y) (((x) < (y)) ? (x) : (y))
// #endif
// #ifndef MAX3
// #define MAX3(x,y,z) (((x) > MAX(y,z)) ? (x) : MAX(y,z))
// #endif
// #ifndef MIN3
// #define MIN3(x,y,z) (((x) < MIN(y,z)) ? (x) : MIN(y,z))
// #endif
// #define IS_S_NONZERO(x) ( (x) < 0 || (x) > 0 )
// #define IS_D_NONZERO(x) ( (x) < 0 || (x) > 0 )
// #define IS_C_NONZERO(x) ( IS_S_NONZERO(*((float*)&x)) ||
// IS_S_NONZERO(*(((float*)&x)+1)) )
// #define IS_Z_NONZERO(x) ( IS_D_NONZERO(*((double*)&x)) ||
// IS_D_NONZERO(*(((double*)&x)+1)) )
/* Error handler */
public static native void LAPACKE_xerbla( @Cast("const char*") BytePointer name, int info );
public static native void LAPACKE_xerbla( String name, int info );
/* Compare two chars (case-insensitive) */
public static native int LAPACKE_lsame( @Cast("char") byte ca, @Cast("char") byte cb );
/* Functions to convert column-major to row-major 2d arrays and vice versa. */
public static native void LAPACKE_cgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_cgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_cgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_cge_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_cge_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_cge_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_cgg_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_cgg_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_cgg_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_chb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_chb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_chb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_che_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_che_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_che_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_chp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer in,
@Cast("lapack_complex_float*") FloatPointer out );
public static native void LAPACKE_chp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer in,
@Cast("lapack_complex_float*") FloatBuffer out );
public static native void LAPACKE_chp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] in,
@Cast("lapack_complex_float*") float[] out );
public static native void LAPACKE_chs_trans( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_chs_trans( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_chs_trans( int matrix_layout, int n,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_cpb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_cpb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_cpb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_cpf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatPointer in,
@Cast("lapack_complex_float*") FloatPointer out );
public static native void LAPACKE_cpf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") FloatBuffer in,
@Cast("lapack_complex_float*") FloatBuffer out );
public static native void LAPACKE_cpf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_float*") float[] in,
@Cast("lapack_complex_float*") float[] out );
public static native void LAPACKE_cpo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_cpo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_cpo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_cpp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer in,
@Cast("lapack_complex_float*") FloatPointer out );
public static native void LAPACKE_cpp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer in,
@Cast("lapack_complex_float*") FloatBuffer out );
public static native void LAPACKE_cpp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] in,
@Cast("lapack_complex_float*") float[] out );
public static native void LAPACKE_csp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer in,
@Cast("lapack_complex_float*") FloatPointer out );
public static native void LAPACKE_csp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer in,
@Cast("lapack_complex_float*") FloatBuffer out );
public static native void LAPACKE_csp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] in,
@Cast("lapack_complex_float*") float[] out );
public static native void LAPACKE_csy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_csy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_csy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_ctb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_ctb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_ctb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_ctf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") FloatPointer in,
@Cast("lapack_complex_float*") FloatPointer out );
public static native void LAPACKE_ctf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") FloatBuffer in,
@Cast("lapack_complex_float*") FloatBuffer out );
public static native void LAPACKE_ctf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") float[] in,
@Cast("lapack_complex_float*") float[] out );
public static native void LAPACKE_ctp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") FloatPointer in,
@Cast("lapack_complex_float*") FloatPointer out );
public static native void LAPACKE_ctp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") FloatBuffer in,
@Cast("lapack_complex_float*") FloatBuffer out );
public static native void LAPACKE_ctp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_float*") float[] in,
@Cast("lapack_complex_float*") float[] out );
public static native void LAPACKE_ctr_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("const lapack_complex_float*") FloatPointer in, int ldin,
@Cast("lapack_complex_float*") FloatPointer out, int ldout );
public static native void LAPACKE_ctr_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("const lapack_complex_float*") FloatBuffer in, int ldin,
@Cast("lapack_complex_float*") FloatBuffer out, int ldout );
public static native void LAPACKE_ctr_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("const lapack_complex_float*") float[] in, int ldin,
@Cast("lapack_complex_float*") float[] out, int ldout );
public static native void LAPACKE_dgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_dge_trans( int matrix_layout, int m, int n,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dge_trans( int matrix_layout, int m, int n,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dge_trans( int matrix_layout, int m, int n,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_dgg_trans( int matrix_layout, int m, int n,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dgg_trans( int matrix_layout, int m, int n,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dgg_trans( int matrix_layout, int m, int n,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_dhs_trans( int matrix_layout, int n,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dhs_trans( int matrix_layout, int n,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dhs_trans( int matrix_layout, int n,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_dpb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dpb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dpb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_dpf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoublePointer in,
DoublePointer out );
public static native void LAPACKE_dpf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const DoubleBuffer in,
DoubleBuffer out );
public static native void LAPACKE_dpf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const double[] in,
double[] out );
public static native void LAPACKE_dpo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dpo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dpo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_dpp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer in,
DoublePointer out );
public static native void LAPACKE_dpp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer in,
DoubleBuffer out );
public static native void LAPACKE_dpp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] in,
double[] out );
public static native void LAPACKE_dsb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dsb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dsb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_dsp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer in,
DoublePointer out );
public static native void LAPACKE_dsp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer in,
DoubleBuffer out );
public static native void LAPACKE_dsp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] in,
double[] out );
public static native void LAPACKE_dsy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dsy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dsy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_dtb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dtb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dtb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_dtf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const DoublePointer in,
DoublePointer out );
public static native void LAPACKE_dtf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const DoubleBuffer in,
DoubleBuffer out );
public static native void LAPACKE_dtf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const double[] in,
double[] out );
public static native void LAPACKE_dtp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const DoublePointer in,
DoublePointer out );
public static native void LAPACKE_dtp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const DoubleBuffer in,
DoubleBuffer out );
public static native void LAPACKE_dtp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const double[] in,
double[] out );
public static native void LAPACKE_dtr_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Const DoublePointer in, int ldin,
DoublePointer out, int ldout );
public static native void LAPACKE_dtr_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Const DoubleBuffer in, int ldin,
DoubleBuffer out, int ldout );
public static native void LAPACKE_dtr_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Const double[] in, int ldin,
double[] out, int ldout );
public static native void LAPACKE_sgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_sgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_sgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_sge_trans( int matrix_layout, int m, int n,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_sge_trans( int matrix_layout, int m, int n,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_sge_trans( int matrix_layout, int m, int n,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_sgg_trans( int matrix_layout, int m, int n,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_sgg_trans( int matrix_layout, int m, int n,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_sgg_trans( int matrix_layout, int m, int n,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_shs_trans( int matrix_layout, int n,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_shs_trans( int matrix_layout, int n,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_shs_trans( int matrix_layout, int n,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_spb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_spb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_spb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_spf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatPointer in,
FloatPointer out );
public static native void LAPACKE_spf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const FloatBuffer in,
FloatBuffer out );
public static native void LAPACKE_spf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Const float[] in,
float[] out );
public static native void LAPACKE_spo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_spo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_spo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_spp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer in,
FloatPointer out );
public static native void LAPACKE_spp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer in,
FloatBuffer out );
public static native void LAPACKE_spp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] in,
float[] out );
public static native void LAPACKE_ssb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_ssb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_ssb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_ssp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer in,
FloatPointer out );
public static native void LAPACKE_ssp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer in,
FloatBuffer out );
public static native void LAPACKE_ssp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] in,
float[] out );
public static native void LAPACKE_ssy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_ssy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_ssy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_stb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_stb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_stb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_stf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const FloatPointer in,
FloatPointer out );
public static native void LAPACKE_stf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const FloatBuffer in,
FloatBuffer out );
public static native void LAPACKE_stf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const float[] in,
float[] out );
public static native void LAPACKE_stp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const FloatPointer in,
FloatPointer out );
public static native void LAPACKE_stp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const FloatBuffer in,
FloatBuffer out );
public static native void LAPACKE_stp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Const float[] in,
float[] out );
public static native void LAPACKE_str_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Const FloatPointer in, int ldin,
FloatPointer out, int ldout );
public static native void LAPACKE_str_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Const FloatBuffer in, int ldin,
FloatBuffer out, int ldout );
public static native void LAPACKE_str_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Const float[] in, int ldin,
float[] out, int ldout );
public static native void LAPACKE_zgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_zgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_zgb_trans( int matrix_layout, int m, int n,
int kl, int ku,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_zge_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_zge_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_zge_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_zgg_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_zgg_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_zgg_trans( int matrix_layout, int m, int n,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_zhb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_zhb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_zhb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_zhe_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_zhe_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_zhe_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_zhp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer in,
@Cast("lapack_complex_double*") DoublePointer out );
public static native void LAPACKE_zhp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in,
@Cast("lapack_complex_double*") DoubleBuffer out );
public static native void LAPACKE_zhp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] in,
@Cast("lapack_complex_double*") double[] out );
public static native void LAPACKE_zhs_trans( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_zhs_trans( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_zhs_trans( int matrix_layout, int n,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_zpb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_zpb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_zpb_trans( int matrix_layout, @Cast("char") byte uplo, int n,
int kd,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_zpf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoublePointer in,
@Cast("lapack_complex_double*") DoublePointer out );
public static native void LAPACKE_zpf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") DoubleBuffer in,
@Cast("lapack_complex_double*") DoubleBuffer out );
public static native void LAPACKE_zpf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo,
int n, @Cast("const lapack_complex_double*") double[] in,
@Cast("lapack_complex_double*") double[] out );
public static native void LAPACKE_zpo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_zpo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_zpo_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_zpp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer in,
@Cast("lapack_complex_double*") DoublePointer out );
public static native void LAPACKE_zpp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in,
@Cast("lapack_complex_double*") DoubleBuffer out );
public static native void LAPACKE_zpp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] in,
@Cast("lapack_complex_double*") double[] out );
public static native void LAPACKE_zsp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer in,
@Cast("lapack_complex_double*") DoublePointer out );
public static native void LAPACKE_zsp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in,
@Cast("lapack_complex_double*") DoubleBuffer out );
public static native void LAPACKE_zsp_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] in,
@Cast("lapack_complex_double*") double[] out );
public static native void LAPACKE_zsy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_zsy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_zsy_trans( int matrix_layout, @Cast("char") byte uplo, int n,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_ztb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_ztb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_ztb_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
public static native void LAPACKE_ztf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") DoublePointer in,
@Cast("lapack_complex_double*") DoublePointer out );
public static native void LAPACKE_ztf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") DoubleBuffer in,
@Cast("lapack_complex_double*") DoubleBuffer out );
public static native void LAPACKE_ztf_trans( int matrix_layout, @Cast("char") byte transr, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") double[] in,
@Cast("lapack_complex_double*") double[] out );
public static native void LAPACKE_ztp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") DoublePointer in,
@Cast("lapack_complex_double*") DoublePointer out );
public static native void LAPACKE_ztp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") DoubleBuffer in,
@Cast("lapack_complex_double*") DoubleBuffer out );
public static native void LAPACKE_ztp_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, @Cast("const lapack_complex_double*") double[] in,
@Cast("lapack_complex_double*") double[] out );
public static native void LAPACKE_ztr_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("const lapack_complex_double*") DoublePointer in, int ldin,
@Cast("lapack_complex_double*") DoublePointer out, int ldout );
public static native void LAPACKE_ztr_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("const lapack_complex_double*") DoubleBuffer in, int ldin,
@Cast("lapack_complex_double*") DoubleBuffer out, int ldout );
public static native void LAPACKE_ztr_trans( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag, int n,
@Cast("const lapack_complex_double*") double[] in, int ldin,
@Cast("lapack_complex_double*") double[] out, int ldout );
/* NaN checkers */
// #define LAPACK_SISNAN( x ) ( x != x )
// #define LAPACK_DISNAN( x ) ( x != x )
// #define LAPACK_CISNAN( x ) ( LAPACK_SISNAN(*((float*) &x)) ||
// LAPACK_SISNAN(*(((float*) &x)+1)) )
// #define LAPACK_ZISNAN( x ) ( LAPACK_DISNAN(*((double*)&x)) ||
// LAPACK_DISNAN(*(((double*)&x)+1)) )
/* NaN checkers for vectors */
public static native int LAPACKE_c_nancheck( int n,
@Cast("const lapack_complex_float*") FloatPointer x,
int incx );
public static native int LAPACKE_c_nancheck( int n,
@Cast("const lapack_complex_float*") FloatBuffer x,
int incx );
public static native int LAPACKE_c_nancheck( int n,
@Cast("const lapack_complex_float*") float[] x,
int incx );
public static native int LAPACKE_d_nancheck( int n,
@Const DoublePointer x,
int incx );
public static native int LAPACKE_d_nancheck( int n,
@Const DoubleBuffer x,
int incx );
public static native int LAPACKE_d_nancheck( int n,
@Const double[] x,
int incx );
public static native int LAPACKE_s_nancheck( int n,
@Const FloatPointer x,
int incx );
public static native int LAPACKE_s_nancheck( int n,
@Const FloatBuffer x,
int incx );
public static native int LAPACKE_s_nancheck( int n,
@Const float[] x,
int incx );
public static native int LAPACKE_z_nancheck( int n,
@Cast("const lapack_complex_double*") DoublePointer x,
int incx );
public static native int LAPACKE_z_nancheck( int n,
@Cast("const lapack_complex_double*") DoubleBuffer x,
int incx );
public static native int LAPACKE_z_nancheck( int n,
@Cast("const lapack_complex_double*") double[] x,
int incx );
/* NaN checkers for matrices */
public static native int LAPACKE_cgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Cast("const lapack_complex_float*") FloatPointer ab,
int ldab );
public static native int LAPACKE_cgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab );
public static native int LAPACKE_cgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Cast("const lapack_complex_float*") float[] ab,
int ldab );
public static native int LAPACKE_cge_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_cge_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_cge_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_cgg_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_cgg_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_cgg_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_cgt_nancheck( int n,
@Cast("const lapack_complex_float*") FloatPointer dl,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer du );
public static native int LAPACKE_cgt_nancheck( int n,
@Cast("const lapack_complex_float*") FloatBuffer dl,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer du );
public static native int LAPACKE_cgt_nancheck( int n,
@Cast("const lapack_complex_float*") float[] dl,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] du );
public static native int LAPACKE_chb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_float*") FloatPointer ab,
int ldab );
public static native int LAPACKE_chb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab );
public static native int LAPACKE_chb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_float*") float[] ab,
int ldab );
public static native int LAPACKE_che_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_che_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_che_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_chp_nancheck( int n,
@Cast("const lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_chp_nancheck( int n,
@Cast("const lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_chp_nancheck( int n,
@Cast("const lapack_complex_float*") float[] ap );
public static native int LAPACKE_chs_nancheck( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_chs_nancheck( int matrix_layout, int n,
@Cast("const lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_chs_nancheck( int matrix_layout, int n,
@Cast("const lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_cpb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_float*") FloatPointer ab,
int ldab );
public static native int LAPACKE_cpb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab );
public static native int LAPACKE_cpb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_float*") float[] ab,
int ldab );
public static native int LAPACKE_cpf_nancheck( int n,
@Cast("const lapack_complex_float*") FloatPointer a );
public static native int LAPACKE_cpf_nancheck( int n,
@Cast("const lapack_complex_float*") FloatBuffer a );
public static native int LAPACKE_cpf_nancheck( int n,
@Cast("const lapack_complex_float*") float[] a );
public static native int LAPACKE_cpo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_cpo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_cpo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_cpp_nancheck( int n,
@Cast("const lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_cpp_nancheck( int n,
@Cast("const lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_cpp_nancheck( int n,
@Cast("const lapack_complex_float*") float[] ap );
public static native int LAPACKE_cpt_nancheck( int n,
@Const FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e );
public static native int LAPACKE_cpt_nancheck( int n,
@Const FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e );
public static native int LAPACKE_cpt_nancheck( int n,
@Const float[] d,
@Cast("const lapack_complex_float*") float[] e );
public static native int LAPACKE_csp_nancheck( int n,
@Cast("const lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_csp_nancheck( int n,
@Cast("const lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_csp_nancheck( int n,
@Cast("const lapack_complex_float*") float[] ap );
public static native int LAPACKE_cst_nancheck( int n,
@Cast("const lapack_complex_float*") FloatPointer d,
@Cast("const lapack_complex_float*") FloatPointer e );
public static native int LAPACKE_cst_nancheck( int n,
@Cast("const lapack_complex_float*") FloatBuffer d,
@Cast("const lapack_complex_float*") FloatBuffer e );
public static native int LAPACKE_cst_nancheck( int n,
@Cast("const lapack_complex_float*") float[] d,
@Cast("const lapack_complex_float*") float[] e );
public static native int LAPACKE_csy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_csy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_csy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_ctb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_float*") FloatPointer ab,
int ldab );
public static native int LAPACKE_ctb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_float*") FloatBuffer ab,
int ldab );
public static native int LAPACKE_ctb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_float*") float[] ab,
int ldab );
public static native int LAPACKE_ctf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_float*") FloatPointer a );
public static native int LAPACKE_ctf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_float*") FloatBuffer a );
public static native int LAPACKE_ctf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_float*") float[] a );
public static native int LAPACKE_ctp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_float*") FloatPointer ap );
public static native int LAPACKE_ctp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_float*") FloatBuffer ap );
public static native int LAPACKE_ctp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_float*") float[] ap );
public static native int LAPACKE_ctr_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_float*") FloatPointer a,
int lda );
public static native int LAPACKE_ctr_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_float*") FloatBuffer a,
int lda );
public static native int LAPACKE_ctr_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_float*") float[] a,
int lda );
public static native int LAPACKE_dgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Const DoublePointer ab,
int ldab );
public static native int LAPACKE_dgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Const DoubleBuffer ab,
int ldab );
public static native int LAPACKE_dgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Const double[] ab,
int ldab );
public static native int LAPACKE_dge_nancheck( int matrix_layout, int m,
int n,
@Const DoublePointer a,
int lda );
public static native int LAPACKE_dge_nancheck( int matrix_layout, int m,
int n,
@Const DoubleBuffer a,
int lda );
public static native int LAPACKE_dge_nancheck( int matrix_layout, int m,
int n,
@Const double[] a,
int lda );
public static native int LAPACKE_dgg_nancheck( int matrix_layout, int m,
int n,
@Const DoublePointer a,
int lda );
public static native int LAPACKE_dgg_nancheck( int matrix_layout, int m,
int n,
@Const DoubleBuffer a,
int lda );
public static native int LAPACKE_dgg_nancheck( int matrix_layout, int m,
int n,
@Const double[] a,
int lda );
public static native int LAPACKE_dgt_nancheck( int n,
@Const DoublePointer dl,
@Const DoublePointer d,
@Const DoublePointer du );
public static native int LAPACKE_dgt_nancheck( int n,
@Const DoubleBuffer dl,
@Const DoubleBuffer d,
@Const DoubleBuffer du );
public static native int LAPACKE_dgt_nancheck( int n,
@Const double[] dl,
@Const double[] d,
@Const double[] du );
public static native int LAPACKE_dhs_nancheck( int matrix_layout, int n,
@Const DoublePointer a,
int lda );
public static native int LAPACKE_dhs_nancheck( int matrix_layout, int n,
@Const DoubleBuffer a,
int lda );
public static native int LAPACKE_dhs_nancheck( int matrix_layout, int n,
@Const double[] a,
int lda );
public static native int LAPACKE_dpb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const DoublePointer ab,
int ldab );
public static native int LAPACKE_dpb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const DoubleBuffer ab,
int ldab );
public static native int LAPACKE_dpb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const double[] ab,
int ldab );
public static native int LAPACKE_dpf_nancheck( int n,
@Const DoublePointer a );
public static native int LAPACKE_dpf_nancheck( int n,
@Const DoubleBuffer a );
public static native int LAPACKE_dpf_nancheck( int n,
@Const double[] a );
public static native int LAPACKE_dpo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const DoublePointer a,
int lda );
public static native int LAPACKE_dpo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const DoubleBuffer a,
int lda );
public static native int LAPACKE_dpo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const double[] a,
int lda );
public static native int LAPACKE_dpp_nancheck( int n,
@Const DoublePointer ap );
public static native int LAPACKE_dpp_nancheck( int n,
@Const DoubleBuffer ap );
public static native int LAPACKE_dpp_nancheck( int n,
@Const double[] ap );
public static native int LAPACKE_dpt_nancheck( int n,
@Const DoublePointer d,
@Const DoublePointer e );
public static native int LAPACKE_dpt_nancheck( int n,
@Const DoubleBuffer d,
@Const DoubleBuffer e );
public static native int LAPACKE_dpt_nancheck( int n,
@Const double[] d,
@Const double[] e );
public static native int LAPACKE_dsb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const DoublePointer ab,
int ldab );
public static native int LAPACKE_dsb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const DoubleBuffer ab,
int ldab );
public static native int LAPACKE_dsb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const double[] ab,
int ldab );
public static native int LAPACKE_dsp_nancheck( int n,
@Const DoublePointer ap );
public static native int LAPACKE_dsp_nancheck( int n,
@Const DoubleBuffer ap );
public static native int LAPACKE_dsp_nancheck( int n,
@Const double[] ap );
public static native int LAPACKE_dst_nancheck( int n,
@Const DoublePointer d,
@Const DoublePointer e );
public static native int LAPACKE_dst_nancheck( int n,
@Const DoubleBuffer d,
@Const DoubleBuffer e );
public static native int LAPACKE_dst_nancheck( int n,
@Const double[] d,
@Const double[] e );
public static native int LAPACKE_dsy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const DoublePointer a,
int lda );
public static native int LAPACKE_dsy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const DoubleBuffer a,
int lda );
public static native int LAPACKE_dsy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const double[] a,
int lda );
public static native int LAPACKE_dtb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const DoublePointer ab,
int ldab );
public static native int LAPACKE_dtb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const DoubleBuffer ab,
int ldab );
public static native int LAPACKE_dtb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const double[] ab,
int ldab );
public static native int LAPACKE_dtf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const DoublePointer a );
public static native int LAPACKE_dtf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const DoubleBuffer a );
public static native int LAPACKE_dtf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const double[] a );
public static native int LAPACKE_dtp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const DoublePointer ap );
public static native int LAPACKE_dtp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const DoubleBuffer ap );
public static native int LAPACKE_dtp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const double[] ap );
public static native int LAPACKE_dtr_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const DoublePointer a,
int lda );
public static native int LAPACKE_dtr_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const DoubleBuffer a,
int lda );
public static native int LAPACKE_dtr_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const double[] a,
int lda );
public static native int LAPACKE_sgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Const FloatPointer ab,
int ldab );
public static native int LAPACKE_sgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Const FloatBuffer ab,
int ldab );
public static native int LAPACKE_sgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Const float[] ab,
int ldab );
public static native int LAPACKE_sge_nancheck( int matrix_layout, int m,
int n,
@Const FloatPointer a,
int lda );
public static native int LAPACKE_sge_nancheck( int matrix_layout, int m,
int n,
@Const FloatBuffer a,
int lda );
public static native int LAPACKE_sge_nancheck( int matrix_layout, int m,
int n,
@Const float[] a,
int lda );
public static native int LAPACKE_sgg_nancheck( int matrix_layout, int m,
int n,
@Const FloatPointer a,
int lda );
public static native int LAPACKE_sgg_nancheck( int matrix_layout, int m,
int n,
@Const FloatBuffer a,
int lda );
public static native int LAPACKE_sgg_nancheck( int matrix_layout, int m,
int n,
@Const float[] a,
int lda );
public static native int LAPACKE_sgt_nancheck( int n,
@Const FloatPointer dl,
@Const FloatPointer d,
@Const FloatPointer du );
public static native int LAPACKE_sgt_nancheck( int n,
@Const FloatBuffer dl,
@Const FloatBuffer d,
@Const FloatBuffer du );
public static native int LAPACKE_sgt_nancheck( int n,
@Const float[] dl,
@Const float[] d,
@Const float[] du );
public static native int LAPACKE_shs_nancheck( int matrix_layout, int n,
@Const FloatPointer a,
int lda );
public static native int LAPACKE_shs_nancheck( int matrix_layout, int n,
@Const FloatBuffer a,
int lda );
public static native int LAPACKE_shs_nancheck( int matrix_layout, int n,
@Const float[] a,
int lda );
public static native int LAPACKE_spb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const FloatPointer ab,
int ldab );
public static native int LAPACKE_spb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const FloatBuffer ab,
int ldab );
public static native int LAPACKE_spb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const float[] ab,
int ldab );
public static native int LAPACKE_spf_nancheck( int n,
@Const FloatPointer a );
public static native int LAPACKE_spf_nancheck( int n,
@Const FloatBuffer a );
public static native int LAPACKE_spf_nancheck( int n,
@Const float[] a );
public static native int LAPACKE_spo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const FloatPointer a,
int lda );
public static native int LAPACKE_spo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const FloatBuffer a,
int lda );
public static native int LAPACKE_spo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const float[] a,
int lda );
public static native int LAPACKE_spp_nancheck( int n,
@Const FloatPointer ap );
public static native int LAPACKE_spp_nancheck( int n,
@Const FloatBuffer ap );
public static native int LAPACKE_spp_nancheck( int n,
@Const float[] ap );
public static native int LAPACKE_spt_nancheck( int n,
@Const FloatPointer d,
@Const FloatPointer e );
public static native int LAPACKE_spt_nancheck( int n,
@Const FloatBuffer d,
@Const FloatBuffer e );
public static native int LAPACKE_spt_nancheck( int n,
@Const float[] d,
@Const float[] e );
public static native int LAPACKE_ssb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const FloatPointer ab,
int ldab );
public static native int LAPACKE_ssb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const FloatBuffer ab,
int ldab );
public static native int LAPACKE_ssb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Const float[] ab,
int ldab );
public static native int LAPACKE_ssp_nancheck( int n,
@Const FloatPointer ap );
public static native int LAPACKE_ssp_nancheck( int n,
@Const FloatBuffer ap );
public static native int LAPACKE_ssp_nancheck( int n,
@Const float[] ap );
public static native int LAPACKE_sst_nancheck( int n,
@Const FloatPointer d,
@Const FloatPointer e );
public static native int LAPACKE_sst_nancheck( int n,
@Const FloatBuffer d,
@Const FloatBuffer e );
public static native int LAPACKE_sst_nancheck( int n,
@Const float[] d,
@Const float[] e );
public static native int LAPACKE_ssy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const FloatPointer a,
int lda );
public static native int LAPACKE_ssy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const FloatBuffer a,
int lda );
public static native int LAPACKE_ssy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Const float[] a,
int lda );
public static native int LAPACKE_stb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const FloatPointer ab,
int ldab );
public static native int LAPACKE_stb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const FloatBuffer ab,
int ldab );
public static native int LAPACKE_stb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Const float[] ab,
int ldab );
public static native int LAPACKE_stf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const FloatPointer a );
public static native int LAPACKE_stf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const FloatBuffer a );
public static native int LAPACKE_stf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const float[] a );
public static native int LAPACKE_stp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const FloatPointer ap );
public static native int LAPACKE_stp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const FloatBuffer ap );
public static native int LAPACKE_stp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const float[] ap );
public static native int LAPACKE_str_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const FloatPointer a,
int lda );
public static native int LAPACKE_str_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const FloatBuffer a,
int lda );
public static native int LAPACKE_str_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Const float[] a,
int lda );
public static native int LAPACKE_zgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab );
public static native int LAPACKE_zgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab );
public static native int LAPACKE_zgb_nancheck( int matrix_layout, int m,
int n, int kl,
int ku,
@Cast("const lapack_complex_double*") double[] ab,
int ldab );
public static native int LAPACKE_zge_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zge_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zge_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_zgg_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zgg_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zgg_nancheck( int matrix_layout, int m,
int n,
@Cast("const lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_zgt_nancheck( int n,
@Cast("const lapack_complex_double*") DoublePointer dl,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer du );
public static native int LAPACKE_zgt_nancheck( int n,
@Cast("const lapack_complex_double*") DoubleBuffer dl,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer du );
public static native int LAPACKE_zgt_nancheck( int n,
@Cast("const lapack_complex_double*") double[] dl,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] du );
public static native int LAPACKE_zhb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab );
public static native int LAPACKE_zhb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab );
public static native int LAPACKE_zhb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_double*") double[] ab,
int ldab );
public static native int LAPACKE_zhe_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zhe_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zhe_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_zhp_nancheck( int n,
@Cast("const lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_zhp_nancheck( int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_zhp_nancheck( int n,
@Cast("const lapack_complex_double*") double[] ap );
public static native int LAPACKE_zhs_nancheck( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zhs_nancheck( int matrix_layout, int n,
@Cast("const lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zhs_nancheck( int matrix_layout, int n,
@Cast("const lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_zpb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab );
public static native int LAPACKE_zpb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab );
public static native int LAPACKE_zpb_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n, int kd,
@Cast("const lapack_complex_double*") double[] ab,
int ldab );
public static native int LAPACKE_zpf_nancheck( int n,
@Cast("const lapack_complex_double*") DoublePointer a );
public static native int LAPACKE_zpf_nancheck( int n,
@Cast("const lapack_complex_double*") DoubleBuffer a );
public static native int LAPACKE_zpf_nancheck( int n,
@Cast("const lapack_complex_double*") double[] a );
public static native int LAPACKE_zpo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zpo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zpo_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_zpp_nancheck( int n,
@Cast("const lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_zpp_nancheck( int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_zpp_nancheck( int n,
@Cast("const lapack_complex_double*") double[] ap );
public static native int LAPACKE_zpt_nancheck( int n,
@Const DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e );
public static native int LAPACKE_zpt_nancheck( int n,
@Const DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e );
public static native int LAPACKE_zpt_nancheck( int n,
@Const double[] d,
@Cast("const lapack_complex_double*") double[] e );
public static native int LAPACKE_zsp_nancheck( int n,
@Cast("const lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_zsp_nancheck( int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_zsp_nancheck( int n,
@Cast("const lapack_complex_double*") double[] ap );
public static native int LAPACKE_zst_nancheck( int n,
@Cast("const lapack_complex_double*") DoublePointer d,
@Cast("const lapack_complex_double*") DoublePointer e );
public static native int LAPACKE_zst_nancheck( int n,
@Cast("const lapack_complex_double*") DoubleBuffer d,
@Cast("const lapack_complex_double*") DoubleBuffer e );
public static native int LAPACKE_zst_nancheck( int n,
@Cast("const lapack_complex_double*") double[] d,
@Cast("const lapack_complex_double*") double[] e );
public static native int LAPACKE_zsy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_zsy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_zsy_nancheck( int matrix_layout, @Cast("char") byte uplo,
int n,
@Cast("const lapack_complex_double*") double[] a,
int lda );
public static native int LAPACKE_ztb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_double*") DoublePointer ab,
int ldab );
public static native int LAPACKE_ztb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_double*") DoubleBuffer ab,
int ldab );
public static native int LAPACKE_ztb_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n, int kd,
@Cast("const lapack_complex_double*") double[] ab,
int ldab );
public static native int LAPACKE_ztf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_double*") DoublePointer a );
public static native int LAPACKE_ztf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_double*") DoubleBuffer a );
public static native int LAPACKE_ztf_nancheck( int matrix_layout, @Cast("char") byte transr,
@Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_double*") double[] a );
public static native int LAPACKE_ztp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_double*") DoublePointer ap );
public static native int LAPACKE_ztp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_double*") DoubleBuffer ap );
public static native int LAPACKE_ztp_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_double*") double[] ap );
public static native int LAPACKE_ztr_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_double*") DoublePointer a,
int lda );
public static native int LAPACKE_ztr_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_double*") DoubleBuffer a,
int lda );
public static native int LAPACKE_ztr_nancheck( int matrix_layout, @Cast("char") byte uplo, @Cast("char") byte diag,
int n,
@Cast("const lapack_complex_double*") double[] a,
int lda );
// #ifdef __cplusplus
// #endif /* __cplusplus */
// #endif /* _LAPACKE_UTILS_H_ */
}