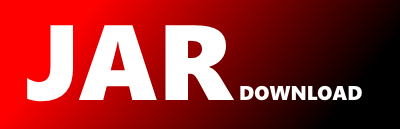
org.bytedeco.bullet.BulletDynamics.btHingeConstraint Maven / Gradle / Ivy
// Targeted by JavaCPP version 1.5.8: DO NOT EDIT THIS FILE
package org.bytedeco.bullet.BulletDynamics;
import java.nio.*;
import org.bytedeco.javacpp.*;
import org.bytedeco.javacpp.annotation.*;
import static org.bytedeco.javacpp.presets.javacpp.*;
import org.bytedeco.bullet.LinearMath.*;
import static org.bytedeco.bullet.global.LinearMath.*;
import org.bytedeco.bullet.BulletCollision.*;
import static org.bytedeco.bullet.global.BulletCollision.*;
import static org.bytedeco.bullet.global.BulletDynamics.*;
/** hinge constraint between two rigidbodies each with a pivotpoint that descibes the axis location in local space
* axis defines the orientation of the hinge axis */
@NoOffset @Properties(inherit = org.bytedeco.bullet.presets.BulletDynamics.class)
public class btHingeConstraint extends btTypedConstraint {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public btHingeConstraint(Pointer p) { super(p); }
public btHingeConstraint(@ByRef btRigidBody rbA, @ByRef btRigidBody rbB, @Const @ByRef btVector3 pivotInA, @Const @ByRef btVector3 pivotInB, @Const @ByRef btVector3 axisInA, @Const @ByRef btVector3 axisInB, @Cast("bool") boolean useReferenceFrameA/*=false*/) { super((Pointer)null); allocate(rbA, rbB, pivotInA, pivotInB, axisInA, axisInB, useReferenceFrameA); }
private native void allocate(@ByRef btRigidBody rbA, @ByRef btRigidBody rbB, @Const @ByRef btVector3 pivotInA, @Const @ByRef btVector3 pivotInB, @Const @ByRef btVector3 axisInA, @Const @ByRef btVector3 axisInB, @Cast("bool") boolean useReferenceFrameA/*=false*/);
public btHingeConstraint(@ByRef btRigidBody rbA, @ByRef btRigidBody rbB, @Const @ByRef btVector3 pivotInA, @Const @ByRef btVector3 pivotInB, @Const @ByRef btVector3 axisInA, @Const @ByRef btVector3 axisInB) { super((Pointer)null); allocate(rbA, rbB, pivotInA, pivotInB, axisInA, axisInB); }
private native void allocate(@ByRef btRigidBody rbA, @ByRef btRigidBody rbB, @Const @ByRef btVector3 pivotInA, @Const @ByRef btVector3 pivotInB, @Const @ByRef btVector3 axisInA, @Const @ByRef btVector3 axisInB);
public btHingeConstraint(@ByRef btRigidBody rbA, @Const @ByRef btVector3 pivotInA, @Const @ByRef btVector3 axisInA, @Cast("bool") boolean useReferenceFrameA/*=false*/) { super((Pointer)null); allocate(rbA, pivotInA, axisInA, useReferenceFrameA); }
private native void allocate(@ByRef btRigidBody rbA, @Const @ByRef btVector3 pivotInA, @Const @ByRef btVector3 axisInA, @Cast("bool") boolean useReferenceFrameA/*=false*/);
public btHingeConstraint(@ByRef btRigidBody rbA, @Const @ByRef btVector3 pivotInA, @Const @ByRef btVector3 axisInA) { super((Pointer)null); allocate(rbA, pivotInA, axisInA); }
private native void allocate(@ByRef btRigidBody rbA, @Const @ByRef btVector3 pivotInA, @Const @ByRef btVector3 axisInA);
public btHingeConstraint(@ByRef btRigidBody rbA, @ByRef btRigidBody rbB, @Const @ByRef btTransform rbAFrame, @Const @ByRef btTransform rbBFrame, @Cast("bool") boolean useReferenceFrameA/*=false*/) { super((Pointer)null); allocate(rbA, rbB, rbAFrame, rbBFrame, useReferenceFrameA); }
private native void allocate(@ByRef btRigidBody rbA, @ByRef btRigidBody rbB, @Const @ByRef btTransform rbAFrame, @Const @ByRef btTransform rbBFrame, @Cast("bool") boolean useReferenceFrameA/*=false*/);
public btHingeConstraint(@ByRef btRigidBody rbA, @ByRef btRigidBody rbB, @Const @ByRef btTransform rbAFrame, @Const @ByRef btTransform rbBFrame) { super((Pointer)null); allocate(rbA, rbB, rbAFrame, rbBFrame); }
private native void allocate(@ByRef btRigidBody rbA, @ByRef btRigidBody rbB, @Const @ByRef btTransform rbAFrame, @Const @ByRef btTransform rbBFrame);
public btHingeConstraint(@ByRef btRigidBody rbA, @Const @ByRef btTransform rbAFrame, @Cast("bool") boolean useReferenceFrameA/*=false*/) { super((Pointer)null); allocate(rbA, rbAFrame, useReferenceFrameA); }
private native void allocate(@ByRef btRigidBody rbA, @Const @ByRef btTransform rbAFrame, @Cast("bool") boolean useReferenceFrameA/*=false*/);
public btHingeConstraint(@ByRef btRigidBody rbA, @Const @ByRef btTransform rbAFrame) { super((Pointer)null); allocate(rbA, rbAFrame); }
private native void allocate(@ByRef btRigidBody rbA, @Const @ByRef btTransform rbAFrame);
public native void buildJacobian();
public native void getInfo1(btConstraintInfo1 info);
public native void getInfo1NonVirtual(btConstraintInfo1 info);
public native void getInfo2(btConstraintInfo2 info);
public native void getInfo2NonVirtual(btConstraintInfo2 info, @Const @ByRef btTransform transA, @Const @ByRef btTransform transB, @Const @ByRef btVector3 angVelA, @Const @ByRef btVector3 angVelB);
public native void getInfo2Internal(btConstraintInfo2 info, @Const @ByRef btTransform transA, @Const @ByRef btTransform transB, @Const @ByRef btVector3 angVelA, @Const @ByRef btVector3 angVelB);
public native void getInfo2InternalUsingFrameOffset(btConstraintInfo2 info, @Const @ByRef btTransform transA, @Const @ByRef btTransform transB, @Const @ByRef btVector3 angVelA, @Const @ByRef btVector3 angVelB);
public native void updateRHS(@Cast("btScalar") double timeStep);
public native @ByRef btRigidBody getRigidBodyA();
public native @ByRef btRigidBody getRigidBodyB();
public native @ByRef btTransform getFrameOffsetA();
public native @ByRef btTransform getFrameOffsetB();
public native void setFrames(@Const @ByRef btTransform frameA, @Const @ByRef btTransform frameB);
public native void setAngularOnly(@Cast("bool") boolean angularOnly);
public native void enableAngularMotor(@Cast("bool") boolean enableMotor, @Cast("btScalar") double targetVelocity, @Cast("btScalar") double maxMotorImpulse);
// extra motor API, including ability to set a target rotation (as opposed to angular velocity)
// note: setMotorTarget sets angular velocity under the hood, so you must call it every tick to
// maintain a given angular target.
public native void enableMotor(@Cast("bool") boolean enableMotor);
public native void setMaxMotorImpulse(@Cast("btScalar") double maxMotorImpulse);
public native void setMotorTargetVelocity(@Cast("btScalar") double motorTargetVelocity);
public native void setMotorTarget(@Const @ByRef btQuaternion qAinB, @Cast("btScalar") double dt); // qAinB is rotation of body A wrt body B.
public native void setMotorTarget(@Cast("btScalar") double targetAngle, @Cast("btScalar") double dt);
public native void setLimit(@Cast("btScalar") double low, @Cast("btScalar") double high, @Cast("btScalar") double _softness/*=0.9f*/, @Cast("btScalar") double _biasFactor/*=0.3f*/, @Cast("btScalar") double _relaxationFactor/*=1.0f*/);
public native void setLimit(@Cast("btScalar") double low, @Cast("btScalar") double high);
public native @Cast("btScalar") double getLimitSoftness();
public native @Cast("btScalar") double getLimitBiasFactor();
public native @Cast("btScalar") double getLimitRelaxationFactor();
public native void setAxis(@ByRef btVector3 axisInA);
public native @Cast("bool") boolean hasLimit();
public native @Cast("btScalar") double getLowerLimit();
public native @Cast("btScalar") double getUpperLimit();
/**The getHingeAngle gives the hinge angle in range [-PI,PI] */
public native @Cast("btScalar") double getHingeAngle();
public native @Cast("btScalar") double getHingeAngle(@Const @ByRef btTransform transA, @Const @ByRef btTransform transB);
public native void testLimit(@Const @ByRef btTransform transA, @Const @ByRef btTransform transB);
public native @ByRef btTransform getAFrame();
public native @ByRef btTransform getBFrame();
public native int getSolveLimit();
public native @Cast("btScalar") double getLimitSign();
public native @Cast("bool") boolean getAngularOnly();
public native @Cast("bool") boolean getEnableAngularMotor();
public native @Cast("btScalar") double getMotorTargetVelocity();
public native @Cast("btScalar") double getMaxMotorImpulse();
// access for UseFrameOffset
public native @Cast("bool") boolean getUseFrameOffset();
public native void setUseFrameOffset(@Cast("bool") boolean frameOffsetOnOff);
// access for UseReferenceFrameA
public native @Cast("bool") boolean getUseReferenceFrameA();
public native void setUseReferenceFrameA(@Cast("bool") boolean useReferenceFrameA);
/**override the default global value of a parameter (such as ERP or CFM), optionally provide the axis (0..5).
* If no axis is provided, it uses the default axis for this constraint. */
public native void setParam(int num, @Cast("btScalar") double value, int axis/*=-1*/);
public native void setParam(int num, @Cast("btScalar") double value);
/**return the local value of parameter */
public native @Cast("btScalar") double getParam(int num, int axis/*=-1*/);
public native @Cast("btScalar") double getParam(int num);
public native int getFlags();
public native int calculateSerializeBufferSize();
/**fills the dataBuffer and returns the struct name (and 0 on failure) */
public native @Cast("const char*") BytePointer serialize(Pointer dataBuffer, btSerializer serializer);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy