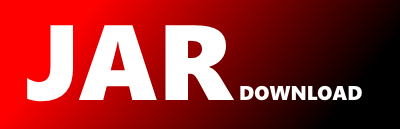
org.bytedeco.cuda.global.nvToolsExt Maven / Gradle / Ivy
The newest version!
// Targeted by JavaCPP version 1.5.11: DO NOT EDIT THIS FILE
package org.bytedeco.cuda.global;
import org.bytedeco.cuda.nvToolsExt.*;
import java.nio.*;
import org.bytedeco.javacpp.*;
import org.bytedeco.javacpp.annotation.*;
import static org.bytedeco.javacpp.presets.javacpp.*;
import org.bytedeco.cuda.cudart.*;
import static org.bytedeco.cuda.global.cudart.*;
public class nvToolsExt extends org.bytedeco.cuda.presets.nvToolsExt {
static { Loader.load(); }
// Parsed from
/*
* Copyright 2009-2012 NVIDIA Corporation. All rights reserved.
*
* NOTICE TO USER:
*
* This source code is subject to NVIDIA ownership rights under U.S. and
* international Copyright laws.
*
* This software and the information contained herein is PROPRIETARY and
* CONFIDENTIAL to NVIDIA and is being provided under the terms and conditions
* of a form of NVIDIA software license agreement.
*
* NVIDIA MAKES NO REPRESENTATION ABOUT THE SUITABILITY OF THIS SOURCE
* CODE FOR ANY PURPOSE. IT IS PROVIDED "AS IS" WITHOUT EXPRESS OR
* IMPLIED WARRANTY OF ANY KIND. NVIDIA DISCLAIMS ALL WARRANTIES WITH
* REGARD TO THIS SOURCE CODE, INCLUDING ALL IMPLIED WARRANTIES OF
* MERCHANTABILITY, NONINFRINGEMENT, AND FITNESS FOR A PARTICULAR PURPOSE.
* IN NO EVENT SHALL NVIDIA BE LIABLE FOR ANY SPECIAL, INDIRECT, INCIDENTAL,
* OR CONSEQUENTIAL DAMAGES, OR ANY DAMAGES WHATSOEVER RESULTING FROM LOSS
* OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE
* OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE
* OR PERFORMANCE OF THIS SOURCE CODE.
*
* U.S. Government End Users. This source code is a "commercial item" as
* that term is defined at 48 C.F.R. 2.101 (OCT 1995), consisting of
* "commercial computer software" and "commercial computer software
* documentation" as such terms are used in 48 C.F.R. 12.212 (SEPT 1995)
* and is provided to the U.S. Government only as a commercial end item.
* Consistent with 48 C.F.R.12.212 and 48 C.F.R. 227.7202-1 through
* 227.7202-4 (JUNE 1995), all U.S. Government End Users acquire the
* source code with only those rights set forth herein.
*
* Any use of this source code in individual and commercial software must
* include, in the user documentation and internal comments to the code,
* the above Disclaimer and U.S. Government End Users Notice.
*/
/** \mainpage
* \section Introduction
* The NVIDIA Tools Extension library is a set of functions that a
* developer can use to provide additional information to tools.
* The additional information is used by the tool to improve
* analysis and visualization of data.
*
* The library introduces close to zero overhead if no tool is
* attached to the application. The overhead when a tool is
* attached is specific to the tool.
*/
// #ifndef NVTOOLSEXT_H_
// #define NVTOOLSEXT_H_
// #if defined(_MSC_VER) /* Microsoft Visual C++ Compiler */
// #ifdef NVTX_EXPORTS
// #define NVTX_DECLSPEC
// #else
// #define NVTX_DECLSPEC __declspec(dllimport)
// #endif /* NVTX_EXPORTS */
// #define NVTX_API __stdcall
// #else /* GCC and most other compilers */
// #define NVTX_DECLSPEC
// #define NVTX_API
// #endif /* Platform */
/**
* The nvToolsExt library depends on stdint.h. If the build tool chain in use
* does not include stdint.h then define NVTX_STDINT_TYPES_ALREADY_DEFINED
* and define the following types:
*
* - uint8_t
*
- int8_t
*
- uint16_t
*
- int16_t
*
- uint32_t
*
- int32_t
*
- uint64_t
*
- int64_t
*
- uintptr_t
*
- intptr_t
*
#define NVTX_STDINT_TYPES_ALREADY_DEFINED if you are using your own header file.
*/
// #ifndef NVTX_STDINT_TYPES_ALREADY_DEFINED
// #include
// #endif
// #ifdef __cplusplus
// #endif /* __cplusplus */
/**
* Tools Extension API version
*/
public static final int NVTX_VERSION = 1;
/**
* Size of the nvtxEventAttributes_t structure.
*/
public static native @MemberGetter int NVTX_EVENT_ATTRIB_STRUCT_SIZE();
public static final int NVTX_EVENT_ATTRIB_STRUCT_SIZE = NVTX_EVENT_ATTRIB_STRUCT_SIZE();
public static final int NVTX_NO_PUSH_POP_TRACKING = ((int)-2);
/** \page EVENT_ATTRIBUTES Event Attributes
*
* \ref MARKER_AND_RANGES can be annotated with various attributes to provide
* additional information for an event or to guide the tool's visualization of
* the data. Each of the attributes is optional and if left unused the
* attributes fall back to a default value.
*
* To specify any attribute other than the text message, the \ref
* EVENT_ATTRIBUTE_STRUCTURE "Event Attribute Structure" must be used.
*/
/** ---------------------------------------------------------------------------
* Color Types
* ------------------------------------------------------------------------- */
/** enum nvtxColorType_t */
public static final int
/** Color attribute is unused. */
NVTX_COLOR_UNKNOWN = 0,
/** An ARGB color is provided. */
NVTX_COLOR_ARGB = 1;
/** ---------------------------------------------------------------------------
* Payload Types
* ------------------------------------------------------------------------- */
/** enum nvtxPayloadType_t */
public static final int
/** Color payload is unused. */
NVTX_PAYLOAD_UNKNOWN = 0,
/** A unsigned integer value is used as payload. */
NVTX_PAYLOAD_TYPE_UNSIGNED_INT64 = 1,
/** A signed integer value is used as payload. */
NVTX_PAYLOAD_TYPE_INT64 = 2,
/** A floating point value is used as payload. */
NVTX_PAYLOAD_TYPE_DOUBLE = 3;
/** ---------------------------------------------------------------------------
* Message Types
* ------------------------------------------------------------------------- */
/** enum nvtxMessageType_t */
public static final int
/** Message payload is unused. */
NVTX_MESSAGE_UNKNOWN = 0,
/** A character sequence is used as payload. */
NVTX_MESSAGE_TYPE_ASCII = 1,
/** A wide character sequence is used as payload. */
NVTX_MESSAGE_TYPE_UNICODE = 2;
// Targeting ..\nvToolsExt\nvtxEventAttributes_t.java
/* ========================================================================= */
/** \defgroup MARKER_AND_RANGES Marker and Ranges
*
* Markers and ranges are used to describe events at a specific time (markers)
* or over a time span (ranges) during the execution of the application
* respectively. The additional information is presented alongside all other
* captured data and facilitates understanding of the collected information.
*/
/* ========================================================================= */
/** \name Markers
*/
/** \name Markers
*/
/** \addtogroup MARKER_AND_RANGES
* \section MARKER Marker
*
* A marker describes a single point in time. A marker event has no side effect
* on other events.
*
* \{
*/
/* ------------------------------------------------------------------------- */
/** \brief Marks an instantaneous event in the application.
*
* A marker can contain a text message or specify additional information
* using the event attributes structure. These attributes include a text
* message, color, category, and a payload. Each of the attributes is optional
* and can only be sent out using the \ref nvtxMarkEx function.
* If \ref nvtxMarkA or \ref nvtxMarkW are used to specify the the marker
* or if an attribute is unspecified then a default value will be used.
*
* @param eventAttrib - The event attribute structure defining the marker's
* attribute types and attribute values.
*
* \par Example:
* {@code
* // zero the structure
* nvtxEventAttributes_t eventAttrib = {0};
* // set the version and the size information
* eventAttrib.version = NVTX_VERSION;
* eventAttrib.size = NVTX_EVENT_ATTRIB_STRUCT_SIZE;
* // configure the attributes. 0 is the default for all attributes.
* eventAttrib.colorType = NVTX_COLOR_ARGB;
* eventAttrib.color = 0xFF880000;
* eventAttrib.messageType = NVTX_MESSAGE_TYPE_ASCII;
* eventAttrib.message.ascii = "Example nvtxMarkEx";
* nvtxMarkEx(&eventAttrib);
* }
*
* @version \NVTX_VERSION_1
* \{ */
public static native void nvtxMarkEx(@Const nvtxEventAttributes_t eventAttrib);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Marks an instantaneous event in the application.
*
* A marker created using \ref nvtxMarkA or \ref nvtxMarkW contains only a
* text message.
*
* @param message - The message associated to this marker event.
*
* \par Example:
* {@code
* nvtxMarkA("Example nvtxMarkA");
* nvtxMarkW(L"Example nvtxMarkW");
* }
*
* @version \NVTX_VERSION_0
* \{ */
public static native void nvtxMarkA(@Cast("const char*") BytePointer message);
public static native void nvtxMarkA(String message);
public static native void nvtxMarkW(@Cast("const wchar_t*") CharPointer message);
public static native void nvtxMarkW(@Cast("const wchar_t*") IntPointer message);
/** \} */
/** \} */ /* END MARKER_AND_RANGES */
/* ========================================================================= */
/** \name Start/Stop Ranges
*/
/** \addtogroup MARKER_AND_RANGES
* \section INDEPENDENT_RANGES Start/Stop Ranges
*
* Start/Stop ranges denote a time span that can expose arbitrary concurrency -
* opposed to Push/Pop ranges that only support nesting. In addition the start
* of a range can happen on a different thread than the end. For the
* correlation of a start/end pair an unique correlation ID is used that is
* returned from the start API call and needs to be passed into the end API
* call.
*
* \{
*/
/* ------------------------------------------------------------------------- */
/** \brief Marks the start of a range.
*
* @param eventAttrib - The event attribute structure defining the range's
* attribute types and attribute values.
*
* @return The unique ID used to correlate a pair of Start and End events.
*
* \remarks Ranges defined by Start/End can overlap.
*
* \par Example:
* {@code
* nvtxEventAttributes_t eventAttrib = {0};
* eventAttrib.version = NVTX_VERSION;
* eventAttrib.size = NVTX_EVENT_ATTRIB_STRUCT_SIZE;
* eventAttrib.category = 3;
* eventAttrib.colorType = NVTX_COLOR_ARGB;
* eventAttrib.color = 0xFF0088FF;
* eventAttrib.messageType = NVTX_MESSAGE_TYPE_ASCII;
* eventAttrib.message.ascii = "Example RangeStartEnd";
* nvtxRangeId_t rangeId = nvtxRangeStartEx(&eventAttrib);
* // ...
* nvtxRangeEnd(rangeId);
* }
*
* @see
* ::nvtxRangeEnd
*
* @version \NVTX_VERSION_1
* \{ */
public static native @Cast("nvtxRangeId_t") long nvtxRangeStartEx(@Const nvtxEventAttributes_t eventAttrib);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Marks the start of a range.
*
* @param message - The event message associated to this range event.
*
* @return The unique ID used to correlate a pair of Start and End events.
*
* \remarks Ranges defined by Start/End can overlap.
*
* \par Example:
* {@code
* nvtxRangeId_t r1 = nvtxRangeStartA("Range 1");
* nvtxRangeId_t r2 = nvtxRangeStartW(L"Range 2");
* nvtxRangeEnd(r1);
* nvtxRangeEnd(r2);
* }
* @see
* ::nvtxRangeEnd
*
* @version \NVTX_VERSION_0
* \{ */
public static native @Cast("nvtxRangeId_t") long nvtxRangeStartA(@Cast("const char*") BytePointer message);
public static native @Cast("nvtxRangeId_t") long nvtxRangeStartA(String message);
public static native @Cast("nvtxRangeId_t") long nvtxRangeStartW(@Cast("const wchar_t*") CharPointer message);
public static native @Cast("nvtxRangeId_t") long nvtxRangeStartW(@Cast("const wchar_t*") IntPointer message);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Marks the end of a range.
*
* @param id - The correlation ID returned from a nvtxRangeStart call.
*
* @see
* ::nvtxRangeStartEx
* ::nvtxRangeStartA
* ::nvtxRangeStartW
*
* @version \NVTX_VERSION_0
* \{ */
public static native void nvtxRangeEnd(@Cast("nvtxRangeId_t") long id);
/** \} */
/** \} */
/* ========================================================================= */
/** \name Push/Pop Ranges
*/
/** \addtogroup MARKER_AND_RANGES
* \section PUSH_POP_RANGES Push/Pop Ranges
*
* Push/Pop ranges denote nested time ranges. Nesting is maintained per thread
* and does not require any additional correlation mechanism. The duration of a
* push/pop range is defined by the corresponding pair of Push/Pop API calls.
*
* \{
*/
/* ------------------------------------------------------------------------- */
/** \brief Marks the start of a nested range
*
* @param eventAttrib - The event attribute structure defining the range's
* attribute types and attribute values.
*
* @return The 0 based level of range being started. If an error occurs a
* negative value is returned.
*
* \par Example:
* {@code
* nvtxEventAttributes_t eventAttrib = {0};
* eventAttrib.version = NVTX_VERSION;
* eventAttrib.size = NVTX_EVENT_ATTRIB_STRUCT_SIZE;
* eventAttrib.colorType = NVTX_COLOR_ARGB;
* eventAttrib.color = 0xFFFF0000;
* eventAttrib.messageType = NVTX_MESSAGE_TYPE_ASCII;
* eventAttrib.message.ascii = "Level 0";
* nvtxRangePushEx(&eventAttrib);
*
* // Re-use eventAttrib
* eventAttrib.messageType = NVTX_MESSAGE_TYPE_UNICODE;
* eventAttrib.message.unicode = L"Level 1";
* nvtxRangePushEx(&eventAttrib);
*
* nvtxRangePop();
* nvtxRangePop();
* }
*
* @see
* ::nvtxRangePop
*
* @version \NVTX_VERSION_1
* \{ */
public static native int nvtxRangePushEx(@Const nvtxEventAttributes_t eventAttrib);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Marks the start of a nested range
*
* @param message - The event message associated to this range event.
*
* @return The 0 based level of range being started. If an error occurs a
* negative value is returned.
*
* \par Example:
* {@code
* nvtxRangePushA("Level 0");
* nvtxRangePushW(L"Level 1");
* nvtxRangePop();
* nvtxRangePop();
* }
*
* @see
* ::nvtxRangePop
*
* @version \NVTX_VERSION_0
* \{ */
public static native int nvtxRangePushA(@Cast("const char*") BytePointer message);
public static native int nvtxRangePushA(String message);
public static native int nvtxRangePushW(@Cast("const wchar_t*") CharPointer message);
public static native int nvtxRangePushW(@Cast("const wchar_t*") IntPointer message);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Marks the end of a nested range
*
* @return The level of the range being ended. If an error occurs a negative
* value is returned on the current thread.
*
* @see
* ::nvtxRangePushEx
* ::nvtxRangePushA
* ::nvtxRangePushW
*
* @version \NVTX_VERSION_0
* \{ */
public static native int nvtxRangePop();
/** \} */
/** \} */
/* ========================================================================= */
/** \defgroup RESOURCE_NAMING Resource Naming
*
* This section covers calls that allow to annotate objects with user-provided
* names in order to allow for a better analysis of complex trace data. All of
* the functions take the handle or the ID of the object to name and the name.
* The functions can be called multiple times during the execution of an
* application, however, in that case it is implementation dependent which
* name will be reported by the tool.
*
* \section RESOURCE_NAMING_NVTX NVTX Resource Naming
* The NVIDIA Tools Extension library allows to attribute events with additional
* information such as category IDs. These category IDs can be annotated with
* user-provided names using the respective resource naming functions.
*
* \section RESOURCE_NAMING_OS OS Resource Naming
* In order to enable a tool to report system threads not just by their thread
* identifier, the NVIDIA Tools Extension library allows to provide user-given
* names to these OS resources.
* \{
*/
/* ------------------------------------------------------------------------- */
/** \name Functions for NVTX Resource Naming
*/
/** \{
* \brief Annotate an NVTX category.
*
* Categories are used to group sets of events. Each category is identified
* through a unique ID and that ID is passed into any of the marker/range
* events to assign that event to a specific category. The nvtxNameCategory
* function calls allow the user to assign a name to a category ID.
*
* @param category - The category ID to name.
* @param name - The name of the category.
*
* \remarks The category names are tracked per process.
*
* \par Example:
* {@code
* nvtxNameCategory(1, "Memory Allocation");
* nvtxNameCategory(2, "Memory Transfer");
* nvtxNameCategory(3, "Memory Object Lifetime");
* }
*
* @version \NVTX_VERSION_1
*/
public static native void nvtxNameCategoryA(@Cast("uint32_t") int category, @Cast("const char*") BytePointer name);
public static native void nvtxNameCategoryA(@Cast("uint32_t") int category, String name);
public static native void nvtxNameCategoryW(@Cast("uint32_t") int category, @Cast("const wchar_t*") CharPointer name);
public static native void nvtxNameCategoryW(@Cast("uint32_t") int category, @Cast("const wchar_t*") IntPointer name);
/** \} */
/* ------------------------------------------------------------------------- */
/** \name Functions for OS Resource Naming
*/
/** \{
* \brief Annotate an OS thread.
*
* Allows the user to name an active thread of the current process. If an
* invalid thread ID is provided or a thread ID from a different process is
* used the behavior of the tool is implementation dependent.
*
* @param threadId - The ID of the thread to name.
* @param name - The name of the thread.
*
* \par Example:
* {@code
* nvtxNameOsThread(GetCurrentThreadId(), "MAIN_THREAD");
* }
*
* @version \NVTX_VERSION_1
*/
public static native void nvtxNameOsThreadA(@Cast("uint32_t") int threadId, @Cast("const char*") BytePointer name);
public static native void nvtxNameOsThreadA(@Cast("uint32_t") int threadId, String name);
public static native void nvtxNameOsThreadW(@Cast("uint32_t") int threadId, @Cast("const wchar_t*") CharPointer name);
public static native void nvtxNameOsThreadW(@Cast("uint32_t") int threadId, @Cast("const wchar_t*") IntPointer name);
/** \} */
/** \} */ /* END RESOURCE_NAMING */
/* ========================================================================= */
// #ifdef __cplusplus
// #endif /* __cplusplus */
// #endif /* NVTOOLSEXT_H_ */
// Parsed from
/*
* Copyright 2009-2012 NVIDIA Corporation. All rights reserved.
*
* NOTICE TO USER:
*
* This source code is subject to NVIDIA ownership rights under U.S. and
* international Copyright laws.
*
* This software and the information contained herein is PROPRIETARY and
* CONFIDENTIAL to NVIDIA and is being provided under the terms and conditions
* of a form of NVIDIA software license agreement.
*
* NVIDIA MAKES NO REPRESENTATION ABOUT THE SUITABILITY OF THIS SOURCE
* CODE FOR ANY PURPOSE. IT IS PROVIDED "AS IS" WITHOUT EXPRESS OR
* IMPLIED WARRANTY OF ANY KIND. NVIDIA DISCLAIMS ALL WARRANTIES WITH
* REGARD TO THIS SOURCE CODE, INCLUDING ALL IMPLIED WARRANTIES OF
* MERCHANTABILITY, NONINFRINGEMENT, AND FITNESS FOR A PARTICULAR PURPOSE.
* IN NO EVENT SHALL NVIDIA BE LIABLE FOR ANY SPECIAL, INDIRECT, INCIDENTAL,
* OR CONSEQUENTIAL DAMAGES, OR ANY DAMAGES WHATSOEVER RESULTING FROM LOSS
* OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE
* OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE
* OR PERFORMANCE OF THIS SOURCE CODE.
*
* U.S. Government End Users. This source code is a "commercial item" as
* that term is defined at 48 C.F.R. 2.101 (OCT 1995), consisting of
* "commercial computer software" and "commercial computer software
* documentation" as such terms are used in 48 C.F.R. 12.212 (SEPT 1995)
* and is provided to the U.S. Government only as a commercial end item.
* Consistent with 48 C.F.R.12.212 and 48 C.F.R. 227.7202-1 through
* 227.7202-4 (JUNE 1995), all U.S. Government End Users acquire the
* source code with only those rights set forth herein.
*
* Any use of this source code in individual and commercial software must
* include, in the user documentation and internal comments to the code,
* the above Disclaimer and U.S. Government End Users Notice.
*/
// #ifndef NVTOOLSEXT_CUDA_H_
// #define NVTOOLSEXT_CUDA_H_
// #include "cuda.h"
// #include "nvToolsExt.h"
// #ifdef __cplusplus
// #endif /* __cplusplus */
/* ========================================================================= */
/** \name Functions for CUDA Resource Naming
*/
/** \addtogroup RESOURCE_NAMING
* \section RESOURCE_NAMING_CUDA CUDA Resource Naming
*
* This section covers the API functions that allow to annotate CUDA resources
* with user-provided names.
*
* \{
*/
/* ------------------------------------------------------------------------- */
/** \brief Annotates a CUDA device.
*
* Allows the user to associate a CUDA device with a user-provided name.
*
* @param device - The handle of the CUDA device to name.
* @param name - The name of the CUDA device.
*
* @version \NVTX_VERSION_1
* \{ */
public static native void nvtxNameCuDeviceA(@Cast("CUdevice") int device, @Cast("const char*") BytePointer name);
public static native void nvtxNameCuDeviceA(@Cast("CUdevice") int device, String name);
public static native void nvtxNameCuDeviceW(@Cast("CUdevice") int device, @Cast("const wchar_t*") CharPointer name);
public static native void nvtxNameCuDeviceW(@Cast("CUdevice") int device, @Cast("const wchar_t*") IntPointer name);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Annotates a CUDA context.
*
* Allows the user to associate a CUDA context with a user-provided name.
*
* @param context - The handle of the CUDA context to name.
* @param name - The name of the CUDA context.
*
* \par Example:
* {@code
* CUresult status = cuCtxCreate( &cuContext, 0, cuDevice );
* if ( CUDA_SUCCESS != status )
* goto Error;
* nvtxNameCuContext(cuContext, "CTX_NAME");
* }
*
* @version \NVTX_VERSION_1
* \{ */
public static native void nvtxNameCuContextA(CUctx_st context, @Cast("const char*") BytePointer name);
public static native void nvtxNameCuContextA(CUctx_st context, String name);
public static native void nvtxNameCuContextW(CUctx_st context, @Cast("const wchar_t*") CharPointer name);
public static native void nvtxNameCuContextW(CUctx_st context, @Cast("const wchar_t*") IntPointer name);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Annotates a CUDA stream.
*
* Allows the user to associate a CUDA stream with a user-provided name.
*
* @param stream - The handle of the CUDA stream to name.
* @param name - The name of the CUDA stream.
*
* @version \NVTX_VERSION_1
* \{ */
public static native void nvtxNameCuStreamA(CUstream_st stream, @Cast("const char*") BytePointer name);
public static native void nvtxNameCuStreamA(CUstream_st stream, String name);
public static native void nvtxNameCuStreamW(CUstream_st stream, @Cast("const wchar_t*") CharPointer name);
public static native void nvtxNameCuStreamW(CUstream_st stream, @Cast("const wchar_t*") IntPointer name);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Annotates a CUDA event.
*
* Allows the user to associate a CUDA event with a user-provided name.
*
* @param event - The handle of the CUDA event to name.
* @param name - The name of the CUDA event.
*
* @version \NVTX_VERSION_1
* \{ */
public static native void nvtxNameCuEventA(CUevent_st event, @Cast("const char*") BytePointer name);
public static native void nvtxNameCuEventA(CUevent_st event, String name);
public static native void nvtxNameCuEventW(CUevent_st event, @Cast("const wchar_t*") CharPointer name);
public static native void nvtxNameCuEventW(CUevent_st event, @Cast("const wchar_t*") IntPointer name);
/** \} */
/** \} */ /* END RESOURCE_NAMING */
/* ========================================================================= */
// #ifdef __cplusplus
// #endif /* __cplusplus */
// #endif /* NVTOOLSEXT_CUDA_H_ */
// Parsed from
/*
* Copyright 2012 NVIDIA Corporation. All rights reserved.
*
* NOTICE TO USER:
*
* This source code is subject to NVIDIA ownership rights under U.S. and
* international Copyright laws.
*
* This software and the information contained herein is PROPRIETARY and
* CONFIDENTIAL to NVIDIA and is being provided under the terms and conditions
* of a form of NVIDIA software license agreement.
*
* NVIDIA MAKES NO REPRESENTATION ABOUT THE SUITABILITY OF THIS SOURCE
* CODE FOR ANY PURPOSE. IT IS PROVIDED "AS IS" WITHOUT EXPRESS OR
* IMPLIED WARRANTY OF ANY KIND. NVIDIA DISCLAIMS ALL WARRANTIES WITH
* REGARD TO THIS SOURCE CODE, INCLUDING ALL IMPLIED WARRANTIES OF
* MERCHANTABILITY, NONINFRINGEMENT, AND FITNESS FOR A PARTICULAR PURPOSE.
* IN NO EVENT SHALL NVIDIA BE LIABLE FOR ANY SPECIAL, INDIRECT, INCIDENTAL,
* OR CONSEQUENTIAL DAMAGES, OR ANY DAMAGES WHATSOEVER RESULTING FROM LOSS
* OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE
* OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE
* OR PERFORMANCE OF THIS SOURCE CODE.
*
* U.S. Government End Users. This source code is a "commercial item" as
* that term is defined at 48 C.F.R. 2.101 (OCT 1995), consisting of
* "commercial computer software" and "commercial computer software
* documentation" as such terms are used in 48 C.F.R. 12.212 (SEPT 1995)
* and is provided to the U.S. Government only as a commercial end item.
* Consistent with 48 C.F.R.12.212 and 48 C.F.R. 227.7202-1 through
* 227.7202-4 (JUNE 1995), all U.S. Government End Users acquire the
* source code with only those rights set forth herein.
*
* Any use of this source code in individual and commercial software must
* include, in the user documentation and internal comments to the code,
* the above Disclaimer and U.S. Government End Users Notice.
*/
// #ifndef NVTOOLSEXT_CUDART_H_
// #define NVTOOLSEXT_CUDART_H_
// #include "cuda.h"
// #include "driver_types.h"
// #include "nvToolsExt.h"
// #ifdef __cplusplus
// #endif /* __cplusplus */
/* ========================================================================= */
/** \name Functions for CUDA Resource Naming
*/
/** \addtogroup RESOURCE_NAMING
* \section RESOURCE_NAMING_CUDA CUDA Resource Naming
*
* This section covers the API functions that allow to annotate CUDA resources
* with user-provided names.
*
* \{
*/
/* ------------------------------------------------------------------------- */
/** \brief Annotates a CUDA device.
*
* Allows the user to associate a CUDA device with a user-provided name.
*
* @param device - The id of the CUDA device to name.
* @param name - The name of the CUDA device.
*
* @version \NVTX_VERSION_1
* \{ */
public static native void nvtxNameCudaDeviceA(int device, @Cast("const char*") BytePointer name);
public static native void nvtxNameCudaDeviceA(int device, String name);
public static native void nvtxNameCudaDeviceW(int device, @Cast("const wchar_t*") CharPointer name);
public static native void nvtxNameCudaDeviceW(int device, @Cast("const wchar_t*") IntPointer name);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Annotates a CUDA stream.
*
* Allows the user to associate a CUDA stream with a user-provided name.
*
* @param stream - The handle of the CUDA stream to name.
* @param name - The name of the CUDA stream.
*
* @version \NVTX_VERSION_1
* \{ */
public static native void nvtxNameCudaStreamA(CUstream_st stream, @Cast("const char*") BytePointer name);
public static native void nvtxNameCudaStreamA(CUstream_st stream, String name);
public static native void nvtxNameCudaStreamW(CUstream_st stream, @Cast("const wchar_t*") CharPointer name);
public static native void nvtxNameCudaStreamW(CUstream_st stream, @Cast("const wchar_t*") IntPointer name);
/** \} */
/* ------------------------------------------------------------------------- */
/** \brief Annotates a CUDA event.
*
* Allows the user to associate a CUDA event with a user-provided name.
*
* @param event - The handle of the CUDA event to name.
* @param name - The name of the CUDA event.
*
* @version \NVTX_VERSION_1
* \{ */
public static native void nvtxNameCudaEventA(CUevent_st event, @Cast("const char*") BytePointer name);
public static native void nvtxNameCudaEventA(CUevent_st event, String name);
public static native void nvtxNameCudaEventW(CUevent_st event, @Cast("const wchar_t*") CharPointer name);
public static native void nvtxNameCudaEventW(CUevent_st event, @Cast("const wchar_t*") IntPointer name);
/** \} */
/** \} */ /* END RESOURCE_NAMING */
/* ========================================================================= */
// #ifdef __cplusplus
// #endif /* __cplusplus */
// #endif /* NVTOOLSEXT_CUDART_H_ */
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy