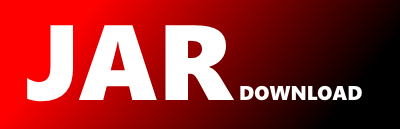
org.bytedeco.cuda.global.nvjpeg Maven / Gradle / Ivy
The newest version!
// Targeted by JavaCPP version 1.5.11: DO NOT EDIT THIS FILE
package org.bytedeco.cuda.global;
import org.bytedeco.cuda.nvjpeg.*;
import java.nio.*;
import org.bytedeco.javacpp.*;
import org.bytedeco.javacpp.annotation.*;
import static org.bytedeco.javacpp.presets.javacpp.*;
import org.bytedeco.cuda.cudart.*;
import static org.bytedeco.cuda.global.cudart.*;
public class nvjpeg extends org.bytedeco.cuda.presets.nvjpeg {
static { Loader.load(); }
// Parsed from
/*
* Copyright 2009-2022 NVIDIA Corporation. All rights reserved.
*
* NOTICE TO LICENSEE:
*
* This source code and/or documentation ("Licensed Deliverables") are
* subject to NVIDIA intellectual property rights under U.S. and
* international Copyright laws.
*
* These Licensed Deliverables contained herein is PROPRIETARY and
* CONFIDENTIAL to NVIDIA and is being provided under the terms and
* conditions of a form of NVIDIA software license agreement by and
* between NVIDIA and Licensee ("License Agreement") or electronically
* accepted by Licensee. Notwithstanding any terms or conditions to
* the contrary in the License Agreement, reproduction or disclosure
* of the Licensed Deliverables to any third party without the express
* written consent of NVIDIA is prohibited.
*
* NOTWITHSTANDING ANY TERMS OR CONDITIONS TO THE CONTRARY IN THE
* LICENSE AGREEMENT, NVIDIA MAKES NO REPRESENTATION ABOUT THE
* SUITABILITY OF THESE LICENSED DELIVERABLES FOR ANY PURPOSE. IT IS
* PROVIDED "AS IS" WITHOUT EXPRESS OR IMPLIED WARRANTY OF ANY KIND.
* NVIDIA DISCLAIMS ALL WARRANTIES WITH REGARD TO THESE LICENSED
* DELIVERABLES, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY,
* NONINFRINGEMENT, AND FITNESS FOR A PARTICULAR PURPOSE.
* NOTWITHSTANDING ANY TERMS OR CONDITIONS TO THE CONTRARY IN THE
* LICENSE AGREEMENT, IN NO EVENT SHALL NVIDIA BE LIABLE FOR ANY
* SPECIAL, INDIRECT, INCIDENTAL, OR CONSEQUENTIAL DAMAGES, OR ANY
* DAMAGES WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS,
* WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS
* ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE
* OF THESE LICENSED DELIVERABLES.
*
* U.S. Government End Users. These Licensed Deliverables are a
* "commercial item" as that term is defined at 48 C.F.R. 2.101 (OCT
* 1995), consisting of "commercial computer software" and "commercial
* computer software documentation" as such terms are used in 48
* C.F.R. 12.212 (SEPT 1995) and is provided to the U.S. Government
* only as a commercial end item. Consistent with 48 C.F.R.12.212 and
* 48 C.F.R. 227.7202-1 through 227.7202-4 (JUNE 1995), all
* U.S. Government End Users acquire the Licensed Deliverables with
* only those rights set forth herein.
*
* Any use of the Licensed Deliverables in individual and commercial
* software must include, in the user documentation and internal
* comments to the code, the above Disclaimer and U.S. Government End
* Users Notice.
*/
// #ifndef NV_JPEG_HEADER
// #define NV_JPEG_HEADER
// #define NVJPEGAPI
// #include "cuda_runtime_api.h"
// #include "library_types.h"
// #include "stdint.h"
// #if defined(__cplusplus)
// #endif
// Maximum number of channels nvjpeg decoder supports
public static final int NVJPEG_MAX_COMPONENT = 4;
// nvjpeg version information
public static final int NVJPEG_VER_MAJOR = 12;
public static final int NVJPEG_VER_MINOR = 3;
public static final int NVJPEG_VER_PATCH = 3;
public static final int NVJPEG_VER_BUILD = 54;
/* nvJPEG status enums, returned by nvJPEG API */
/** enum nvjpegStatus_t */
public static final int
NVJPEG_STATUS_SUCCESS = 0,
NVJPEG_STATUS_NOT_INITIALIZED = 1,
NVJPEG_STATUS_INVALID_PARAMETER = 2,
NVJPEG_STATUS_BAD_JPEG = 3,
NVJPEG_STATUS_JPEG_NOT_SUPPORTED = 4,
NVJPEG_STATUS_ALLOCATOR_FAILURE = 5,
NVJPEG_STATUS_EXECUTION_FAILED = 6,
NVJPEG_STATUS_ARCH_MISMATCH = 7,
NVJPEG_STATUS_INTERNAL_ERROR = 8,
NVJPEG_STATUS_IMPLEMENTATION_NOT_SUPPORTED = 9,
NVJPEG_STATUS_INCOMPLETE_BITSTREAM = 10;
// Enums for EXIF Orientation
/** enum nvjpegExifOrientation_t */
public static final int
NVJPEG_ORIENTATION_UNKNOWN = 0,
NVJPEG_ORIENTATION_NORMAL = 1,
NVJPEG_ORIENTATION_FLIP_HORIZONTAL = 2,
NVJPEG_ORIENTATION_ROTATE_180 = 3,
NVJPEG_ORIENTATION_FLIP_VERTICAL = 4,
NVJPEG_ORIENTATION_TRANSPOSE = 5,
NVJPEG_ORIENTATION_ROTATE_90 = 6,
NVJPEG_ORIENTATION_TRANSVERSE = 7,
NVJPEG_ORIENTATION_ROTATE_270 = 8;
// Enum identifies image chroma subsampling values stored inside JPEG input stream
// In the case of NVJPEG_CSS_GRAY only 1 luminance channel is encoded in JPEG input stream
// Otherwise both chroma planes are present
/** enum nvjpegChromaSubsampling_t */
public static final int
NVJPEG_CSS_444 = 0,
NVJPEG_CSS_422 = 1,
NVJPEG_CSS_420 = 2,
NVJPEG_CSS_440 = 3,
NVJPEG_CSS_411 = 4,
NVJPEG_CSS_410 = 5,
NVJPEG_CSS_GRAY = 6,
NVJPEG_CSS_410V = 7,
NVJPEG_CSS_UNKNOWN = -1;
// Parameter of this type specifies what type of output user wants for image decoding
/** enum nvjpegOutputFormat_t */
public static final int
// return decompressed image as it is - write planar output
NVJPEG_OUTPUT_UNCHANGED = 0,
// return planar luma and chroma, assuming YCbCr colorspace
NVJPEG_OUTPUT_YUV = 1,
// return luma component only, if YCbCr colorspace,
// or try to convert to grayscale,
// writes to 1-st channel of nvjpegImage_t
NVJPEG_OUTPUT_Y = 2,
// convert to planar RGB
NVJPEG_OUTPUT_RGB = 3,
// convert to planar BGR
NVJPEG_OUTPUT_BGR = 4,
// convert to interleaved RGB and write to 1-st channel of nvjpegImage_t
NVJPEG_OUTPUT_RGBI = 5,
// convert to interleaved BGR and write to 1-st channel of nvjpegImage_t
NVJPEG_OUTPUT_BGRI = 6,
// 16 bit unsigned output in interleaved format
NVJPEG_OUTPUT_UNCHANGEDI_U16 = 7,
// maximum allowed value
NVJPEG_OUTPUT_FORMAT_MAX = 7;
// Parameter of this type specifies what type of input user provides for encoding
/** enum nvjpegInputFormat_t */
public static final int
NVJPEG_INPUT_RGB = 3, // Input is RGB - will be converted to YCbCr before encoding
NVJPEG_INPUT_BGR = 4, // Input is RGB - will be converted to YCbCr before encoding
NVJPEG_INPUT_RGBI = 5, // Input is interleaved RGB - will be converted to YCbCr before encoding
NVJPEG_INPUT_BGRI = 6; // Input is interleaved RGB - will be converted to YCbCr before encoding
// Implementation
// NVJPEG_BACKEND_DEFAULT : default value
// NVJPEG_BACKEND_HYBRID : uses CPU for Huffman decode
// NVJPEG_BACKEND_GPU_HYBRID : uses GPU assisted Huffman decode. nvjpegDecodeBatched will use GPU decoding for baseline JPEG bitstreams with
// interleaved scan when batch size is bigger than 50
// NVJPEG_BACKEND_HARDWARE : supports baseline JPEG bitstream with single scan. 410 and 411 sub-samplings are not supported
// NVJPEG_BACKEND_GPU_HYBRID_DEVICE : nvjpegDecodeBatched will support bitstream input on device memory
// NVJPEG_BACKEND_HARDWARE_DEVICE : nvjpegDecodeBatched will support bitstream input on device memory
/** enum nvjpegBackend_t */
public static final int
NVJPEG_BACKEND_DEFAULT = 0,
NVJPEG_BACKEND_HYBRID = 1,
NVJPEG_BACKEND_GPU_HYBRID = 2,
NVJPEG_BACKEND_HARDWARE = 3,
NVJPEG_BACKEND_GPU_HYBRID_DEVICE = 4,
NVJPEG_BACKEND_HARDWARE_DEVICE = 5,
NVJPEG_BACKEND_LOSSLESS_JPEG = 6;
// Currently parseable JPEG encodings (SOF markers)
/** enum nvjpegJpegEncoding_t */
public static final int
NVJPEG_ENCODING_UNKNOWN = 0x0,
NVJPEG_ENCODING_BASELINE_DCT = 0xc0,
NVJPEG_ENCODING_EXTENDED_SEQUENTIAL_DCT_HUFFMAN = 0xc1,
NVJPEG_ENCODING_PROGRESSIVE_DCT_HUFFMAN = 0xc2,
NVJPEG_ENCODING_LOSSLESS_HUFFMAN = 0xc3;
/** enum nvjpegScaleFactor_t */
public static final int
NVJPEG_SCALE_NONE = 0, // decoded output is not scaled
NVJPEG_SCALE_1_BY_2 = 1, // decoded output width and height is scaled by a factor of 1/2
NVJPEG_SCALE_1_BY_4 = 2, // decoded output width and height is scaled by a factor of 1/4
NVJPEG_SCALE_1_BY_8 = 3; // decoded output width and height is scaled by a factor of 1/8
public static final int NVJPEG_FLAGS_DEFAULT = 0;
public static final int NVJPEG_FLAGS_HW_DECODE_NO_PIPELINE = 1;
public static final int NVJPEG_FLAGS_ENABLE_MEMORY_POOLS = 1<<1;
public static final int NVJPEG_FLAGS_BITSTREAM_STRICT = 1<<2;
public static final int NVJPEG_FLAGS_REDUCED_MEMORY_DECODE = 1<<3;
public static final int NVJPEG_FLAGS_REDUCED_MEMORY_DECODE_ZERO_COPY = 1<<4;
public static final int NVJPEG_FLAGS_UPSAMPLING_WITH_INTERPOLATION = 1<<5;
// Targeting ..\nvjpeg\nvjpegImage_t.java
// Targeting ..\nvjpeg\tDevMalloc.java
// Targeting ..\nvjpeg\tDevFree.java
// Targeting ..\nvjpeg\tPinnedMalloc.java
// Targeting ..\nvjpeg\tPinnedFree.java
// Targeting ..\nvjpeg\nvjpegDevAllocator_t.java
// Targeting ..\nvjpeg\nvjpegPinnedAllocator_t.java
// Targeting ..\nvjpeg\tDevMallocV2.java
// Targeting ..\nvjpeg\tDevFreeV2.java
// Targeting ..\nvjpeg\tPinnedMallocV2.java
// Targeting ..\nvjpeg\tPinnedFreeV2.java
// Targeting ..\nvjpeg\nvjpegDevAllocatorV2_t.java
// Targeting ..\nvjpeg\nvjpegPinnedAllocatorV2_t.java
// Targeting ..\nvjpeg\nvjpegHandle.java
// Targeting ..\nvjpeg\nvjpegJpegState.java
// returns library's property values, such as MAJOR_VERSION, MINOR_VERSION or PATCH_LEVEL
public static native @Cast("nvjpegStatus_t") int nvjpegGetProperty(@Cast("libraryPropertyType") int type, IntPointer value);
public static native @Cast("nvjpegStatus_t") int nvjpegGetProperty(@Cast("libraryPropertyType") int type, IntBuffer value);
public static native @Cast("nvjpegStatus_t") int nvjpegGetProperty(@Cast("libraryPropertyType") int type, int[] value);
// returns CUDA Toolkit property values that was used for building library,
// such as MAJOR_VERSION, MINOR_VERSION or PATCH_LEVEL
public static native @Cast("nvjpegStatus_t") int nvjpegGetCudartProperty(@Cast("libraryPropertyType") int type, IntPointer value);
public static native @Cast("nvjpegStatus_t") int nvjpegGetCudartProperty(@Cast("libraryPropertyType") int type, IntBuffer value);
public static native @Cast("nvjpegStatus_t") int nvjpegGetCudartProperty(@Cast("libraryPropertyType") int type, int[] value);
// Initalization of nvjpeg handle. This handle is used for all consecutive calls
// IN backend : Backend to use. Currently Default or Hybrid (which is the same at the moment) is supported.
// IN allocator : Pointer to nvjpegDevAllocator. If NULL - use default cuda calls (cudaMalloc/cudaFree)
// INT/OUT handle : Codec instance, use for other calls
public static native @Cast("nvjpegStatus_t") int nvjpegCreate(@Cast("nvjpegBackend_t") int backend, nvjpegDevAllocator_t dev_allocator, @ByPtrPtr nvjpegHandle handle);
// Initalization of nvjpeg handle with default backend and default memory allocators.
// INT/OUT handle : Codec instance, use for other calls
public static native @Cast("nvjpegStatus_t") int nvjpegCreateSimple(@ByPtrPtr nvjpegHandle handle);
// Initalization of nvjpeg handle with additional parameters. This handle is used for all consecutive nvjpeg calls
// IN backend : Backend to use. Currently Default or Hybrid (which is the same at the moment) is supported.
// IN dev_allocator : Pointer to nvjpegDevAllocator. If NULL - use default cuda calls (cudaMalloc/cudaFree)
// IN pinned_allocator : Pointer to nvjpegPinnedAllocator. If NULL - use default cuda calls (cudaHostAlloc/cudaFreeHost)
// IN flags : Parameters for the operation. Must be 0.
// INT/OUT handle : Codec instance, use for other calls
public static native @Cast("nvjpegStatus_t") int nvjpegCreateEx(@Cast("nvjpegBackend_t") int backend,
nvjpegDevAllocator_t dev_allocator,
nvjpegPinnedAllocator_t pinned_allocator,
@Cast("unsigned int") int flags,
@ByPtrPtr nvjpegHandle handle);
public static native @Cast("nvjpegStatus_t") int nvjpegCreateExV2(@Cast("nvjpegBackend_t") int backend,
nvjpegDevAllocatorV2_t dev_allocator,
nvjpegPinnedAllocatorV2_t pinned_allocator,
@Cast("unsigned int") int flags,
@ByPtrPtr nvjpegHandle handle);
// Release the handle and resources.
// IN/OUT handle: instance handle to release
public static native @Cast("nvjpegStatus_t") int nvjpegDestroy(nvjpegHandle handle);
// Sets padding for device memory allocations. After success on this call any device memory allocation
// would be padded to the multiple of specified number of bytes.
// IN padding: padding size
// IN/OUT handle: instance handle to release
public static native @Cast("nvjpegStatus_t") int nvjpegSetDeviceMemoryPadding(@Cast("size_t") long padding, nvjpegHandle handle);
// Retrieves padding for device memory allocations
// IN/OUT padding: padding size currently used in handle.
// IN/OUT handle: instance handle to release
public static native @Cast("nvjpegStatus_t") int nvjpegGetDeviceMemoryPadding(@Cast("size_t*") SizeTPointer padding, nvjpegHandle handle);
// Sets padding for pinned host memory allocations. After success on this call any pinned host memory allocation
// would be padded to the multiple of specified number of bytes.
// IN padding: padding size
// IN/OUT handle: instance handle to release
public static native @Cast("nvjpegStatus_t") int nvjpegSetPinnedMemoryPadding(@Cast("size_t") long padding, nvjpegHandle handle);
// Retrieves padding for pinned host memory allocations
// IN/OUT padding: padding size currently used in handle.
// IN/OUT handle: instance handle to release
public static native @Cast("nvjpegStatus_t") int nvjpegGetPinnedMemoryPadding(@Cast("size_t*") SizeTPointer padding, nvjpegHandle handle);
public static native @Cast("nvjpegStatus_t") int nvjpegGetHardwareDecoderInfo(nvjpegHandle handle,
@Cast("unsigned int*") IntPointer num_engines,
@Cast("unsigned int*") IntPointer num_cores_per_engine);
public static native @Cast("nvjpegStatus_t") int nvjpegGetHardwareDecoderInfo(nvjpegHandle handle,
@Cast("unsigned int*") IntBuffer num_engines,
@Cast("unsigned int*") IntBuffer num_cores_per_engine);
public static native @Cast("nvjpegStatus_t") int nvjpegGetHardwareDecoderInfo(nvjpegHandle handle,
@Cast("unsigned int*") int[] num_engines,
@Cast("unsigned int*") int[] num_cores_per_engine);
// Initalization of decoding state
// IN handle : Library handle
// INT/OUT jpeg_handle : Decoded jpeg image state handle
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStateCreate(nvjpegHandle handle, @ByPtrPtr nvjpegJpegState jpeg_handle);
// Release the jpeg image handle.
// INT/OUT jpeg_handle : Decoded jpeg image state handle
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStateDestroy(nvjpegJpegState jpeg_handle);
//
// Retrieve the image info, including channel, width and height of each component, and chroma subsampling.
// If less than NVJPEG_MAX_COMPONENT channels are encoded, then zeros would be set to absent channels information
// If the image is 3-channel, all three groups are valid.
// This function is thread safe.
// IN handle : Library handle
// IN data : Pointer to the buffer containing the jpeg stream data to be decoded.
// IN length : Length of the jpeg image buffer.
// OUT nComponent : Number of componenets of the image, currently only supports 1-channel (grayscale) or 3-channel.
// OUT subsampling : Chroma subsampling used in this JPEG, see nvjpegChromaSubsampling_t
// OUT widths : pointer to NVJPEG_MAX_COMPONENT of ints, returns width of each channel. 0 if channel is not encoded
// OUT heights : pointer to NVJPEG_MAX_COMPONENT of ints, returns height of each channel. 0 if channel is not encoded
public static native @Cast("nvjpegStatus_t") int nvjpegGetImageInfo(
nvjpegHandle handle,
@Cast("const unsigned char*") BytePointer data,
@Cast("size_t") long length,
IntPointer nComponents,
@Cast("nvjpegChromaSubsampling_t*") IntPointer subsampling,
IntPointer widths,
IntPointer heights);
public static native @Cast("nvjpegStatus_t") int nvjpegGetImageInfo(
nvjpegHandle handle,
@Cast("const unsigned char*") ByteBuffer data,
@Cast("size_t") long length,
IntBuffer nComponents,
@Cast("nvjpegChromaSubsampling_t*") IntBuffer subsampling,
IntBuffer widths,
IntBuffer heights);
public static native @Cast("nvjpegStatus_t") int nvjpegGetImageInfo(
nvjpegHandle handle,
@Cast("const unsigned char*") byte[] data,
@Cast("size_t") long length,
int[] nComponents,
@Cast("nvjpegChromaSubsampling_t*") int[] subsampling,
int[] widths,
int[] heights);
// Decodes single image. The API is back-end agnostic. It will decide on which implementation to use internally
// Destination buffers should be large enough to be able to store output of specified format.
// For each color plane sizes could be retrieved for image using nvjpegGetImageInfo()
// and minimum required memory buffer for each plane is nPlaneHeight*nPlanePitch where nPlanePitch >= nPlaneWidth for
// planar output formats and nPlanePitch >= nPlaneWidth*nOutputComponents for interleaved output format.
//
// IN/OUT handle : Library handle
// INT/OUT jpeg_handle : Decoded jpeg image state handle
// IN data : Pointer to the buffer containing the jpeg image to be decoded.
// IN length : Length of the jpeg image buffer.
// IN output_format : Output data format. See nvjpegOutputFormat_t for description
// IN/OUT destination : Pointer to structure with information about output buffers. See nvjpegImage_t description.
// IN/OUT stream : CUDA stream where to submit all GPU work
//
// \return NVJPEG_STATUS_SUCCESS if successful
public static native @Cast("nvjpegStatus_t") int nvjpegDecode(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*") BytePointer data,
@Cast("size_t") long length,
@Cast("nvjpegOutputFormat_t") int output_format,
nvjpegImage_t destination,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegDecode(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*") ByteBuffer data,
@Cast("size_t") long length,
@Cast("nvjpegOutputFormat_t") int output_format,
nvjpegImage_t destination,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegDecode(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*") byte[] data,
@Cast("size_t") long length,
@Cast("nvjpegOutputFormat_t") int output_format,
nvjpegImage_t destination,
CUstream_st stream);
//////////////////////////////////////////////
/////////////// Batch decoding ///////////////
//////////////////////////////////////////////
// Resets and initizlizes batch decoder for working on the batches of specified size
// Should be called once for decoding bathes of this specific size, also use to reset failed batches
// IN/OUT handle : Library handle
// INT/OUT jpeg_handle : Decoded jpeg image state handle
// IN batch_size : Size of the batch
// IN max_cpu_threads : Maximum number of CPU threads that will be processing this batch
// IN output_format : Output data format. Will be the same for every image in batch
//
// \return NVJPEG_STATUS_SUCCESS if successful
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedInitialize(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
int batch_size,
int max_cpu_threads,
@Cast("nvjpegOutputFormat_t") int output_format);
// Decodes batch of images. Output buffers should be large enough to be able to store
// outputs of specified format, see single image decoding description for details. Call to
// nvjpegDecodeBatchedInitialize() is required prior to this call, batch size is expected to be the same as
// parameter to this batch initialization function.
//
// IN/OUT handle : Library handle
// INT/OUT jpeg_handle : Decoded jpeg image state handle
// IN data : Array of size batch_size of pointers to the input buffers containing the jpeg images to be decoded.
// IN lengths : Array of size batch_size with lengths of the jpeg images' buffers in the batch.
// IN/OUT destinations : Array of size batch_size with pointers to structure with information about output buffers,
// IN/OUT stream : CUDA stream where to submit all GPU work
//
// \return NVJPEG_STATUS_SUCCESS if successful
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatched(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*const*") PointerPointer data,
@Cast("const size_t*") SizeTPointer lengths,
nvjpegImage_t destinations,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatched(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*const*") @ByPtrPtr BytePointer data,
@Cast("const size_t*") SizeTPointer lengths,
nvjpegImage_t destinations,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatched(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*const*") @ByPtrPtr ByteBuffer data,
@Cast("const size_t*") SizeTPointer lengths,
nvjpegImage_t destinations,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatched(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*const*") @ByPtrPtr byte[] data,
@Cast("const size_t*") SizeTPointer lengths,
nvjpegImage_t destinations,
CUstream_st stream);
// Allocates the internal buffers as a pre-allocation step
// IN handle : Library handle
// IN jpeg_handle : Decoded jpeg image state handle
// IN width : frame width
// IN height : frame height
// IN chroma_subsampling : chroma subsampling of images to be decoded
// IN output_format : out format
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedPreAllocate(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
int batch_size,
int width,
int height,
@Cast("nvjpegChromaSubsampling_t") int chroma_subsampling,
@Cast("nvjpegOutputFormat_t") int output_format);
// Allocates the internal buffers as a pre-allocation step
// IN handle : Library handle
// IN jpeg_handle : Decoded jpeg image state handle
// IN data : jpeg bitstream containing huffman and quantization tables
// IN length : bitstream size in bytes
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedParseJpegTables(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*") BytePointer data,
@Cast("const size_t") long length);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedParseJpegTables(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*") ByteBuffer data,
@Cast("const size_t") long length);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedParseJpegTables(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*") byte[] data,
@Cast("const size_t") long length);
// Targeting ..\nvjpeg\nvjpegEncoderState.java
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderStateCreate(
nvjpegHandle handle,
@ByPtrPtr nvjpegEncoderState encoder_state,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderStateDestroy(nvjpegEncoderState encoder_state);
// Targeting ..\nvjpeg\nvjpegEncoderParams.java
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderParamsCreate(
nvjpegHandle handle,
@ByPtrPtr nvjpegEncoderParams encoder_params,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderParamsDestroy(nvjpegEncoderParams encoder_params);
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderParamsSetQuality(
nvjpegEncoderParams encoder_params,
int quality,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderParamsSetEncoding(
nvjpegEncoderParams encoder_params,
@Cast("nvjpegJpegEncoding_t") int etype,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderParamsSetOptimizedHuffman(
nvjpegEncoderParams encoder_params,
int optimized,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderParamsSetSamplingFactors(
nvjpegEncoderParams encoder_params,
@Cast("const nvjpegChromaSubsampling_t") int chroma_subsampling,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncodeGetBufferSize(
nvjpegHandle handle,
nvjpegEncoderParams encoder_params,
int image_width,
int image_height,
@Cast("size_t*") SizeTPointer max_stream_length);
public static native @Cast("nvjpegStatus_t") int nvjpegEncodeYUV(
nvjpegHandle handle,
nvjpegEncoderState encoder_state,
nvjpegEncoderParams encoder_params,
@Const nvjpegImage_t source,
@Cast("nvjpegChromaSubsampling_t") int chroma_subsampling,
int image_width,
int image_height,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncodeImage(
nvjpegHandle handle,
nvjpegEncoderState encoder_state,
nvjpegEncoderParams encoder_params,
@Const nvjpegImage_t source,
@Cast("nvjpegInputFormat_t") int input_format,
int image_width,
int image_height,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncodeRetrieveBitstreamDevice(
nvjpegHandle handle,
nvjpegEncoderState encoder_state,
@Cast("unsigned char*") BytePointer data,
@Cast("size_t*") SizeTPointer length,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncodeRetrieveBitstreamDevice(
nvjpegHandle handle,
nvjpegEncoderState encoder_state,
@Cast("unsigned char*") ByteBuffer data,
@Cast("size_t*") SizeTPointer length,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncodeRetrieveBitstreamDevice(
nvjpegHandle handle,
nvjpegEncoderState encoder_state,
@Cast("unsigned char*") byte[] data,
@Cast("size_t*") SizeTPointer length,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncodeRetrieveBitstream(
nvjpegHandle handle,
nvjpegEncoderState encoder_state,
@Cast("unsigned char*") BytePointer data,
@Cast("size_t*") SizeTPointer length,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncodeRetrieveBitstream(
nvjpegHandle handle,
nvjpegEncoderState encoder_state,
@Cast("unsigned char*") ByteBuffer data,
@Cast("size_t*") SizeTPointer length,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegEncodeRetrieveBitstream(
nvjpegHandle handle,
nvjpegEncoderState encoder_state,
@Cast("unsigned char*") byte[] data,
@Cast("size_t*") SizeTPointer length,
CUstream_st stream);
// Targeting ..\nvjpeg\nvjpegBufferPinned.java
public static native @Cast("nvjpegStatus_t") int nvjpegBufferPinnedCreate(nvjpegHandle handle,
nvjpegPinnedAllocator_t pinned_allocator,
@ByPtrPtr nvjpegBufferPinned buffer);
public static native @Cast("nvjpegStatus_t") int nvjpegBufferPinnedCreateV2(nvjpegHandle handle,
nvjpegPinnedAllocatorV2_t pinned_allocator,
@ByPtrPtr nvjpegBufferPinned buffer);
public static native @Cast("nvjpegStatus_t") int nvjpegBufferPinnedResize(nvjpegBufferPinned buffer, @Cast("size_t") long size, CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegBufferPinnedDestroy(nvjpegBufferPinned buffer);
// Targeting ..\nvjpeg\nvjpegBufferDevice.java
public static native @Cast("nvjpegStatus_t") int nvjpegBufferDeviceCreate(nvjpegHandle handle,
nvjpegDevAllocator_t device_allocator,
@ByPtrPtr nvjpegBufferDevice buffer);
public static native @Cast("nvjpegStatus_t") int nvjpegBufferDeviceCreateV2(nvjpegHandle handle,
nvjpegDevAllocatorV2_t device_allocator,
@ByPtrPtr nvjpegBufferDevice buffer);
public static native @Cast("nvjpegStatus_t") int nvjpegBufferDeviceResize(nvjpegBufferDevice buffer, @Cast("size_t") long size, CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegBufferDeviceDestroy(nvjpegBufferDevice buffer);
// retrieve buffer size and pointer - this allows reusing buffer when decode is not needed
public static native @Cast("nvjpegStatus_t") int nvjpegBufferPinnedRetrieve(nvjpegBufferPinned buffer, @Cast("size_t*") SizeTPointer size, @Cast("void**") PointerPointer ptr);
public static native @Cast("nvjpegStatus_t") int nvjpegBufferPinnedRetrieve(nvjpegBufferPinned buffer, @Cast("size_t*") SizeTPointer size, @Cast("void**") @ByPtrPtr Pointer ptr);
public static native @Cast("nvjpegStatus_t") int nvjpegBufferDeviceRetrieve(nvjpegBufferDevice buffer, @Cast("size_t*") SizeTPointer size, @Cast("void**") PointerPointer ptr);
public static native @Cast("nvjpegStatus_t") int nvjpegBufferDeviceRetrieve(nvjpegBufferDevice buffer, @Cast("size_t*") SizeTPointer size, @Cast("void**") @ByPtrPtr Pointer ptr);
// this allows attaching same memory buffers to different states, allowing to switch implementations
// without allocating extra memory
public static native @Cast("nvjpegStatus_t") int nvjpegStateAttachPinnedBuffer(nvjpegJpegState decoder_state,
nvjpegBufferPinned pinned_buffer);
public static native @Cast("nvjpegStatus_t") int nvjpegStateAttachDeviceBuffer(nvjpegJpegState decoder_state,
nvjpegBufferDevice device_buffer);
// Targeting ..\nvjpeg\nvjpegJpegStream.java
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamCreate(
nvjpegHandle handle,
@ByPtrPtr nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamDestroy(nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamParse(
nvjpegHandle handle,
@Cast("const unsigned char*") BytePointer data,
@Cast("size_t") long length,
int save_metadata,
int save_stream,
nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamParse(
nvjpegHandle handle,
@Cast("const unsigned char*") ByteBuffer data,
@Cast("size_t") long length,
int save_metadata,
int save_stream,
nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamParse(
nvjpegHandle handle,
@Cast("const unsigned char*") byte[] data,
@Cast("size_t") long length,
int save_metadata,
int save_stream,
nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamParseHeader(
nvjpegHandle handle,
@Cast("const unsigned char*") BytePointer data,
@Cast("size_t") long length,
nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamParseHeader(
nvjpegHandle handle,
@Cast("const unsigned char*") ByteBuffer data,
@Cast("size_t") long length,
nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamParseHeader(
nvjpegHandle handle,
@Cast("const unsigned char*") byte[] data,
@Cast("size_t") long length,
nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamParseTables(
nvjpegHandle handle,
@Cast("const unsigned char*") BytePointer data,
@Cast("size_t") long length,
nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamParseTables(
nvjpegHandle handle,
@Cast("const unsigned char*") ByteBuffer data,
@Cast("size_t") long length,
nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamParseTables(
nvjpegHandle handle,
@Cast("const unsigned char*") byte[] data,
@Cast("size_t") long length,
nvjpegJpegStream jpeg_stream);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetJpegEncoding(
nvjpegJpegStream jpeg_stream,
@Cast("nvjpegJpegEncoding_t*") IntPointer jpeg_encoding);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetJpegEncoding(
nvjpegJpegStream jpeg_stream,
@Cast("nvjpegJpegEncoding_t*") IntBuffer jpeg_encoding);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetJpegEncoding(
nvjpegJpegStream jpeg_stream,
@Cast("nvjpegJpegEncoding_t*") int[] jpeg_encoding);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetFrameDimensions(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int*") IntPointer width,
@Cast("unsigned int*") IntPointer height);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetFrameDimensions(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int*") IntBuffer width,
@Cast("unsigned int*") IntBuffer height);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetFrameDimensions(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int*") int[] width,
@Cast("unsigned int*") int[] height);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetComponentsNum(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int*") IntPointer components_num);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetComponentsNum(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int*") IntBuffer components_num);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetComponentsNum(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int*") int[] components_num);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetComponentDimensions(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int") int component,
@Cast("unsigned int*") IntPointer width,
@Cast("unsigned int*") IntPointer height);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetComponentDimensions(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int") int component,
@Cast("unsigned int*") IntBuffer width,
@Cast("unsigned int*") IntBuffer height);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetComponentDimensions(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int") int component,
@Cast("unsigned int*") int[] width,
@Cast("unsigned int*") int[] height);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetExifOrientation(
nvjpegJpegStream jpeg_stream,
@Cast("nvjpegExifOrientation_t*") IntPointer orientation_flag);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetExifOrientation(
nvjpegJpegStream jpeg_stream,
@Cast("nvjpegExifOrientation_t*") IntBuffer orientation_flag);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetExifOrientation(
nvjpegJpegStream jpeg_stream,
@Cast("nvjpegExifOrientation_t*") int[] orientation_flag);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetSamplePrecision(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int*") IntPointer precision);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetSamplePrecision(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int*") IntBuffer precision);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetSamplePrecision(
nvjpegJpegStream jpeg_stream,
@Cast("unsigned int*") int[] precision);
// if encoded is 1 color component then it assumes 4:0:0 (NVJPEG_CSS_GRAY, grayscale)
// if encoded is 3 color components it tries to assign one of the known subsamplings
// based on the components subsampling infromation
// in case sampling factors are not stadard or number of components is different
// it will return NVJPEG_CSS_UNKNOWN
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetChromaSubsampling(
nvjpegJpegStream jpeg_stream,
@Cast("nvjpegChromaSubsampling_t*") IntPointer chroma_subsampling);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetChromaSubsampling(
nvjpegJpegStream jpeg_stream,
@Cast("nvjpegChromaSubsampling_t*") IntBuffer chroma_subsampling);
public static native @Cast("nvjpegStatus_t") int nvjpegJpegStreamGetChromaSubsampling(
nvjpegJpegStream jpeg_stream,
@Cast("nvjpegChromaSubsampling_t*") int[] chroma_subsampling);
// Targeting ..\nvjpeg\nvjpegDecodeParams.java
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeParamsCreate(
nvjpegHandle handle,
@ByPtrPtr nvjpegDecodeParams decode_params);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeParamsDestroy(nvjpegDecodeParams decode_params);
// set output pixel format - same value as in nvjpegDecode()
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeParamsSetOutputFormat(
nvjpegDecodeParams decode_params,
@Cast("nvjpegOutputFormat_t") int output_format);
// set to desired ROI. set to (0, 0, -1, -1) to disable ROI decode (decode whole image)
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeParamsSetROI(
nvjpegDecodeParams decode_params,
int offset_x, int offset_y, int roi_width, int roi_height);
// set to true to allow conversion from CMYK to RGB or YUV that follows simple subtractive scheme
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeParamsSetAllowCMYK(
nvjpegDecodeParams decode_params,
int allow_cmyk);
// works only with the hardware decoder backend
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeParamsSetScaleFactor(
nvjpegDecodeParams decode_params,
@Cast("nvjpegScaleFactor_t") int scale_factor);
// set the orientation flag to the decode parameters
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeParamsSetExifOrientation(
nvjpegDecodeParams decode_params,
@Cast("nvjpegExifOrientation_t") int orientation);
// Targeting ..\nvjpeg\nvjpegJpegDecoder.java
//creates decoder implementation
public static native @Cast("nvjpegStatus_t") int nvjpegDecoderCreate(nvjpegHandle nvjpeg_handle,
@Cast("nvjpegBackend_t") int implementation,
@ByPtrPtr nvjpegJpegDecoder decoder_handle);
public static native @Cast("nvjpegStatus_t") int nvjpegDecoderDestroy(nvjpegJpegDecoder decoder_handle);
// on return sets is_supported value to 0 if decoder is capable to handle jpeg_stream
// with specified decode parameters
public static native @Cast("nvjpegStatus_t") int nvjpegDecoderJpegSupported(nvjpegJpegDecoder decoder_handle,
nvjpegJpegStream jpeg_stream,
nvjpegDecodeParams decode_params,
IntPointer is_supported);
public static native @Cast("nvjpegStatus_t") int nvjpegDecoderJpegSupported(nvjpegJpegDecoder decoder_handle,
nvjpegJpegStream jpeg_stream,
nvjpegDecodeParams decode_params,
IntBuffer is_supported);
public static native @Cast("nvjpegStatus_t") int nvjpegDecoderJpegSupported(nvjpegJpegDecoder decoder_handle,
nvjpegJpegStream jpeg_stream,
nvjpegDecodeParams decode_params,
int[] is_supported);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedSupported(nvjpegHandle handle,
nvjpegJpegStream jpeg_stream,
IntPointer is_supported);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedSupported(nvjpegHandle handle,
nvjpegJpegStream jpeg_stream,
IntBuffer is_supported);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedSupported(nvjpegHandle handle,
nvjpegJpegStream jpeg_stream,
int[] is_supported);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedSupportedEx(nvjpegHandle handle,
nvjpegJpegStream jpeg_stream,
nvjpegDecodeParams decode_params,
IntPointer is_supported);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedSupportedEx(nvjpegHandle handle,
nvjpegJpegStream jpeg_stream,
nvjpegDecodeParams decode_params,
IntBuffer is_supported);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedSupportedEx(nvjpegHandle handle,
nvjpegJpegStream jpeg_stream,
nvjpegDecodeParams decode_params,
int[] is_supported);
// creates decoder state
public static native @Cast("nvjpegStatus_t") int nvjpegDecoderStateCreate(nvjpegHandle nvjpeg_handle,
nvjpegJpegDecoder decoder_handle,
@ByPtrPtr nvjpegJpegState decoder_state);
///////////////////////////////////////////////////////////////////////////////////
// Decode functions //
///////////////////////////////////////////////////////////////////////////////////
// takes parsed jpeg as input and performs decoding
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeJpeg(
nvjpegHandle handle,
nvjpegJpegDecoder decoder,
nvjpegJpegState decoder_state,
nvjpegJpegStream jpeg_bitstream,
nvjpegImage_t destination,
nvjpegDecodeParams decode_params,
CUstream_st stream);
// starts decoding on host and save decode parameters to the state
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeJpegHost(
nvjpegHandle handle,
nvjpegJpegDecoder decoder,
nvjpegJpegState decoder_state,
nvjpegDecodeParams decode_params,
nvjpegJpegStream jpeg_stream);
// hybrid stage of decoding image, involves device async calls
// note that jpeg stream is a parameter here - because we still might need copy
// parts of bytestream to device
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeJpegTransferToDevice(
nvjpegHandle handle,
nvjpegJpegDecoder decoder,
nvjpegJpegState decoder_state,
nvjpegJpegStream jpeg_stream,
CUstream_st stream);
// finishing async operations on the device
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeJpegDevice(
nvjpegHandle handle,
nvjpegJpegDecoder decoder,
nvjpegJpegState decoder_state,
nvjpegImage_t destination,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedEx(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*const*") PointerPointer data,
@Cast("const size_t*") SizeTPointer lengths,
nvjpegImage_t destinations,
@ByPtrPtr nvjpegDecodeParams decode_params,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedEx(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*const*") @ByPtrPtr BytePointer data,
@Cast("const size_t*") SizeTPointer lengths,
nvjpegImage_t destinations,
@ByPtrPtr nvjpegDecodeParams decode_params,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedEx(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*const*") @ByPtrPtr ByteBuffer data,
@Cast("const size_t*") SizeTPointer lengths,
nvjpegImage_t destinations,
@ByPtrPtr nvjpegDecodeParams decode_params,
CUstream_st stream);
public static native @Cast("nvjpegStatus_t") int nvjpegDecodeBatchedEx(
nvjpegHandle handle,
nvjpegJpegState jpeg_handle,
@Cast("const unsigned char*const*") @ByPtrPtr byte[] data,
@Cast("const size_t*") SizeTPointer lengths,
nvjpegImage_t destinations,
@ByPtrPtr nvjpegDecodeParams decode_params,
CUstream_st stream);
///////////////////////////////////////////////////////////////////////////////////
// JPEG Transcoding Functions //
///////////////////////////////////////////////////////////////////////////////////
// copies metadata (JFIF, APP, EXT, COM markers) from parsed stream
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderParamsCopyMetadata(
nvjpegEncoderState encoder_state,
nvjpegEncoderParams encode_params,
nvjpegJpegStream jpeg_stream,
CUstream_st stream);
// copies quantization tables from parsed stream
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderParamsCopyQuantizationTables(
nvjpegEncoderParams encode_params,
nvjpegJpegStream jpeg_stream,
CUstream_st stream);
// copies huffman tables from parsed stream. should require same scans structure
public static native @Cast("nvjpegStatus_t") int nvjpegEncoderParamsCopyHuffmanTables(
nvjpegEncoderState encoder_state,
nvjpegEncoderParams encode_params,
nvjpegJpegStream jpeg_stream,
CUstream_st stream);
// #if defined(__cplusplus)
// #endif
// #endif /* NV_JPEG_HEADER */
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy