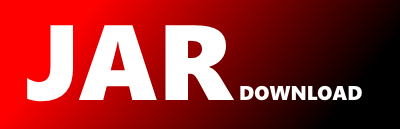
org.bytedeco.opencv.opencv_objdetect.CharucoBoard Maven / Gradle / Ivy
// Targeted by JavaCPP version 1.5.11: DO NOT EDIT THIS FILE
package org.bytedeco.opencv.opencv_objdetect;
import java.nio.*;
import org.bytedeco.javacpp.*;
import org.bytedeco.javacpp.annotation.*;
import static org.bytedeco.javacpp.presets.javacpp.*;
import static org.bytedeco.openblas.global.openblas_nolapack.*;
import static org.bytedeco.openblas.global.openblas.*;
import org.bytedeco.opencv.opencv_core.*;
import static org.bytedeco.opencv.global.opencv_core.*;
import org.bytedeco.opencv.opencv_imgproc.*;
import static org.bytedeco.opencv.global.opencv_imgproc.*;
import static org.bytedeco.opencv.global.opencv_imgcodecs.*;
import org.bytedeco.opencv.opencv_videoio.*;
import static org.bytedeco.opencv.global.opencv_videoio.*;
import org.bytedeco.opencv.opencv_highgui.*;
import static org.bytedeco.opencv.global.opencv_highgui.*;
import org.bytedeco.opencv.opencv_flann.*;
import static org.bytedeco.opencv.global.opencv_flann.*;
import org.bytedeco.opencv.opencv_features2d.*;
import static org.bytedeco.opencv.global.opencv_features2d.*;
import org.bytedeco.opencv.opencv_calib3d.*;
import static org.bytedeco.opencv.global.opencv_calib3d.*;
import org.bytedeco.opencv.opencv_dnn.*;
import static org.bytedeco.opencv.global.opencv_dnn.*;
import static org.bytedeco.opencv.global.opencv_objdetect.*;
/**
* \brief ChArUco board is a planar chessboard where the markers are placed inside the white squares of a chessboard.
*
* The benefits of ChArUco boards is that they provide both, ArUco markers versatility and chessboard corner precision,
* which is important for calibration and pose estimation. The board image can be drawn using generateImage() method.
*/
@Namespace("cv::aruco") @Properties(inherit = org.bytedeco.opencv.presets.opencv_objdetect.class)
public class CharucoBoard extends Board {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public CharucoBoard(Pointer p) { super(p); }
/** Native array allocator. Access with {@link Pointer#position(long)}. */
public CharucoBoard(long size) { super((Pointer)null); allocateArray(size); }
private native void allocateArray(long size);
@Override public CharucoBoard position(long position) {
return (CharucoBoard)super.position(position);
}
@Override public CharucoBoard getPointer(long i) {
return new CharucoBoard((Pointer)this).offsetAddress(i);
}
/** \brief CharucoBoard constructor
*
* @param size number of chessboard squares in x and y directions
* @param squareLength squareLength chessboard square side length (normally in meters)
* @param markerLength marker side length (same unit than squareLength)
* @param dictionary dictionary of markers indicating the type of markers
* @param ids array of id used markers
* The first markers in the dictionary are used to fill the white chessboard squares.
*/
public CharucoBoard(@Const @ByRef Size size, float squareLength, float markerLength,
@Const @ByRef Dictionary dictionary, @ByVal(nullValue = "cv::InputArray(cv::noArray())") Mat ids) { super((Pointer)null); allocate(size, squareLength, markerLength, dictionary, ids); }
private native void allocate(@Const @ByRef Size size, float squareLength, float markerLength,
@Const @ByRef Dictionary dictionary, @ByVal(nullValue = "cv::InputArray(cv::noArray())") Mat ids);
public CharucoBoard(@Const @ByRef Size size, float squareLength, float markerLength,
@Const @ByRef Dictionary dictionary) { super((Pointer)null); allocate(size, squareLength, markerLength, dictionary); }
private native void allocate(@Const @ByRef Size size, float squareLength, float markerLength,
@Const @ByRef Dictionary dictionary);
public CharucoBoard(@Const @ByRef Size size, float squareLength, float markerLength,
@Const @ByRef Dictionary dictionary, @ByVal(nullValue = "cv::InputArray(cv::noArray())") UMat ids) { super((Pointer)null); allocate(size, squareLength, markerLength, dictionary, ids); }
private native void allocate(@Const @ByRef Size size, float squareLength, float markerLength,
@Const @ByRef Dictionary dictionary, @ByVal(nullValue = "cv::InputArray(cv::noArray())") UMat ids);
public CharucoBoard(@Const @ByRef Size size, float squareLength, float markerLength,
@Const @ByRef Dictionary dictionary, @ByVal(nullValue = "cv::InputArray(cv::noArray())") GpuMat ids) { super((Pointer)null); allocate(size, squareLength, markerLength, dictionary, ids); }
private native void allocate(@Const @ByRef Size size, float squareLength, float markerLength,
@Const @ByRef Dictionary dictionary, @ByVal(nullValue = "cv::InputArray(cv::noArray())") GpuMat ids);
/** \brief set legacy chessboard pattern.
*
* Legacy setting creates chessboard patterns starting with a white box in the upper left corner
* if there is an even row count of chessboard boxes, otherwise it starts with a black box.
* This setting ensures compatibility to patterns created with OpenCV versions prior OpenCV 4.6.0.
* See https://github.com/opencv/opencv/issues/23152.
*
* Default value: false.
*/
public native void setLegacyPattern(@Cast("bool") boolean legacyPattern);
public native @Cast("bool") boolean getLegacyPattern();
public native @ByVal Size getChessboardSize();
public native float getSquareLength();
public native float getMarkerLength();
/** \brief get CharucoBoard::chessboardCorners
*/
public native @ByVal @Cast("std::vector*") Point3fVector getChessboardCorners();
/** \brief get CharucoBoard::nearestMarkerIdx, for each charuco corner, nearest marker index in ids array
*/
public native @ByVal IntVectorVector getNearestMarkerIdx();
/** \brief get CharucoBoard::nearestMarkerCorners, for each charuco corner, nearest marker corner id of each marker
*/
public native @ByVal IntVectorVector getNearestMarkerCorners();
/** \brief check whether the ChArUco markers are collinear
*
* @param charucoIds list of identifiers for each corner in charucoCorners per frame.
* @return bool value, 1 (true) if detected corners form a line, 0 (false) if they do not.
* solvePnP, calibration functions will fail if the corners are collinear (true).
*
* The number of ids in charucoIDs should be <= the number of chessboard corners in the board.
* This functions checks whether the charuco corners are on a straight line (returns true, if so), or not (false).
* Axis parallel, as well as diagonal and other straight lines detected. Degenerate cases:
* for number of charucoIDs <= 2,the function returns true.
*/
public native @Cast("bool") boolean checkCharucoCornersCollinear(@ByVal Mat charucoIds);
public native @Cast("bool") boolean checkCharucoCornersCollinear(@ByVal UMat charucoIds);
public native @Cast("bool") boolean checkCharucoCornersCollinear(@ByVal GpuMat charucoIds);
@Deprecated public CharucoBoard() { super((Pointer)null); allocate(); }
@Deprecated private native void allocate();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy