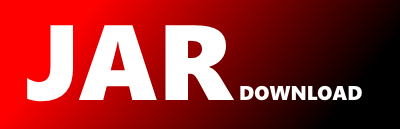
org.bytedeco.tensorflowlite.SignatureRunner Maven / Gradle / Ivy
// Targeted by JavaCPP version 1.5.7: DO NOT EDIT THIS FILE
package org.bytedeco.tensorflowlite;
import java.nio.*;
import org.bytedeco.javacpp.*;
import org.bytedeco.javacpp.annotation.*;
import static org.bytedeco.tensorflowlite.global.tensorflowlite.*;
// Class for friend declarations.
/** WARNING: Experimental interface, subject to change
*
* SignatureRunner class for running TFLite models using SignatureDef.
*
* Usage:
*
*
* // Create model from file. Note that the model instance must outlive the
* // interpreter instance.
* auto model = tflite::FlatBufferModel::BuildFromFile(...);
* if (model == nullptr) {
* // Return error.
* }
*
* // Create an Interpreter with an InterpreterBuilder.
* std::unique_ptr interpreter;
* tflite::ops::builtin::BuiltinOpResolver resolver;
* if (InterpreterBuilder(*model, resolver)(&interpreter) != kTfLiteOk) {
* // Return failure.
* }
*
* // Get the list of signatures and check it.
* auto signature_defs = interpreter->signature_def_names();
* if (signature_defs.empty()) {
* // Return error.
* }
*
* // Get pointer to the SignatureRunner instance corresponding to a signature.
* // Note that the pointed SignatureRunner instance has lifetime same as the
* // Interpreter instance.
* tflite::SignatureRunner* runner =
* interpreter->GetSignatureRunner(signature_defs[0]->c_str());
* if (runner == nullptr) {
* // Return error.
* }
* if (runner->AllocateTensors() != kTfLiteOk) {
* // Return failure.
* }
*
* // Set input data. In this example, the input tensor has float type.
* float* input = runner->input_tensor(0)->data.f;
* for (int i = 0; i < input_size; i++) {
* input[i] = ...; */
// }
/** runner->Invoke();
/**
/**
/** WARNING: This class is *not* thread-safe. The client is responsible for
/** ensuring serialized interaction to avoid data races and undefined behavior.
/**
/** SignatureRunner and Interpreter share the same underlying data. Calling
/** methods on an Interpreter object will affect the state in corresponding
/** SignatureRunner objects. Therefore, it is recommended not to call other
/** Interpreter methods after calling GetSignatureRunner to create
/** SignatureRunner instances. */
@Namespace("tflite") @NoOffset @Properties(inherit = org.bytedeco.tensorflowlite.presets.tensorflowlite.class)
public class SignatureRunner extends Pointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public SignatureRunner(Pointer p) { super(p); }
/** Returns the key for the corresponding signature. */
public native @StdString String signature_key();
/** Returns the number of inputs. */
public native @Cast("size_t") long input_size();
/** Returns the number of outputs. */
public native @Cast("size_t") long output_size();
/** Read-only access to list of signature input names. */
public native @Cast("const char**") @StdVector PointerPointer input_names();
/** Read-only access to list of signature output names. */
public native @Cast("const char**") @StdVector PointerPointer output_names();
/** Returns the input tensor identified by 'input_name' in the
* given signature. Returns nullptr if the given name is not valid. */
public native TfLiteTensor input_tensor(@Cast("const char*") BytePointer input_name);
public native TfLiteTensor input_tensor(String input_name);
/** Returns the output tensor identified by 'output_name' in the
* given signature. Returns nullptr if the given name is not valid. */
public native @Const TfLiteTensor output_tensor(@Cast("const char*") BytePointer output_name);
public native @Const TfLiteTensor output_tensor(String output_name);
/** Change a dimensionality of a given tensor. Note, this is only acceptable
* for tensors that are inputs.
* Returns status of failure or success. Note that this doesn't actually
* resize any existing buffers. A call to AllocateTensors() is required to
* change the tensor input buffer. */
public native @Cast("TfLiteStatus") int ResizeInputTensor(@Cast("const char*") BytePointer input_name,
@StdVector IntPointer new_size);
public native @Cast("TfLiteStatus") int ResizeInputTensor(String input_name,
@StdVector IntBuffer new_size);
public native @Cast("TfLiteStatus") int ResizeInputTensor(@Cast("const char*") BytePointer input_name,
@StdVector int[] new_size);
public native @Cast("TfLiteStatus") int ResizeInputTensor(String input_name,
@StdVector IntPointer new_size);
public native @Cast("TfLiteStatus") int ResizeInputTensor(@Cast("const char*") BytePointer input_name,
@StdVector IntBuffer new_size);
public native @Cast("TfLiteStatus") int ResizeInputTensor(String input_name,
@StdVector int[] new_size);
/** Updates allocations for all tensors, related to the given signature. */
public native @Cast("TfLiteStatus") int AllocateTensors();
/** Invokes the signature runner (run the graph identified by the given
* signature in dependency order). */
public native @Cast("TfLiteStatus") int Invoke();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy