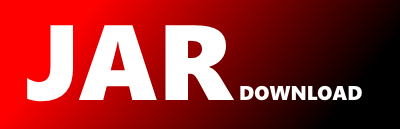
org.bytedeco.tensorrt.nvinfer.IScaleLayer Maven / Gradle / Ivy
The newest version!
// Targeted by JavaCPP version 1.5.11: DO NOT EDIT THIS FILE
package org.bytedeco.tensorrt.nvinfer;
import java.nio.*;
import org.bytedeco.javacpp.*;
import org.bytedeco.javacpp.annotation.*;
import static org.bytedeco.javacpp.presets.javacpp.*;
import org.bytedeco.cuda.cudart.*;
import static org.bytedeco.cuda.global.cudart.*;
import org.bytedeco.cuda.cublas.*;
import static org.bytedeco.cuda.global.cublas.*;
import org.bytedeco.cuda.cudnn.*;
import static org.bytedeco.cuda.global.cudnn.*;
import org.bytedeco.cuda.nvrtc.*;
import static org.bytedeco.cuda.global.nvrtc.*;
import static org.bytedeco.tensorrt.global.nvinfer.*;
/**
* \class IScaleLayer
*
* \brief A Scale layer in a network definition.
*
* This layer applies a per-element computation to its input:
*
* \p output = (\p input* \p scale + \p shift)^ \p power
*
* The coefficients can be applied on a per-tensor, per-channel, or per-element basis.
*
* \note If the number of weights is 0, then a default value is used for shift, power, and scale.
* The default shift is 0, the default power is 1, and the default scale is 1.
*
* The output size is the same as the input size.
*
* \note The input tensor is required to have at least 4 dimensions.
*
* A scale layer may be used as an INT8 quantization node in a graph, if the output is constrained to INT8 and
* the input to FP32. Quantization rounds ties to even, and clamps to [-128, 127].
*
* @see ScaleMode
*
* \warning Do not inherit from this class, as doing so will break forward-compatibility of the API and ABI.
* */
@Namespace("nvinfer1") @NoOffset @Properties(inherit = org.bytedeco.tensorrt.presets.nvinfer.class)
public class IScaleLayer extends ILayer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public IScaleLayer(Pointer p) { super(p); }
/**
* \brief Set the scale mode.
*
* @see getMode()
* */
//!
//!
//!
public native @NoException(true) void setMode(ScaleMode mode);
public native @NoException(true) void setMode(@Cast("nvinfer1::ScaleMode") int mode);
/**
* \brief Get the scale mode.
*
* @see setMode()
* */
//!
//!
//!
public native @NoException(true) ScaleMode getMode();
/**
* \brief Set the shift value.
*
* @see getShift()
* */
//!
//!
//!
public native @NoException(true) void setShift(@ByVal Weights shift);
/**
* \brief Get the shift value.
*
* @see setShift()
* */
//!
//!
//!
public native @ByVal @NoException(true) Weights getShift();
/**
* \brief Set the scale value.
*
* @see getScale()
* */
//!
//!
//!
public native @NoException(true) void setScale(@ByVal Weights scale);
/**
* \brief Get the scale value.
*
* @see setScale()
* */
//!
//!
//!
public native @ByVal @NoException(true) Weights getScale();
/**
* \brief Set the power value.
*
* @see getPower()
* */
//!
//!
//!
public native @NoException(true) void setPower(@ByVal Weights power);
/**
* \brief Get the power value.
*
* @see setPower()
* */
//!
//!
//!
//!
//!
public native @ByVal @NoException(true) Weights getPower();
/**
* \brief Get the channel axis.
*
* @return channelAxis parameter passed to addScaleNd() or set by setChannelAxis()
*
* The value is the index of the channel axis in the input tensor's dimensions.
* Scaling happens along the channel axis when ScaleMode::kCHANNEL is enabled.
*
* @see addScaleNd()
* */
//!
//!
//!
//!
//!
//!
public native @NoException(true) int getChannelAxis();
/**
* \brief Set the channel axis.
*
* The value is the index of the channel axis in the input tensor's dimensions.
*
* For ScaleMode::kCHANNEL, there can be distinct scale, shift, and power weights for each channel coordinate.
* For ScaleMode::kELEMENTWISE, there can be distinct scale, shift, and power weights for each combination of
* coordinates from the channel axis and axes after it.
*
* For example, suppose the input tensor has dimensions [10,20,30,40] and the channel axis is 1.
* Let [n,c,h,w] denote an input coordinate.
* For ScaleMode::kCHANNEL, the scale, shift, and power weights are indexed by c.
* For ScaleMode::kELEMENTWISE, the scale, shift, and power weights are indexed by [c,h,w].
*
* @see addScaleNd()
* */
public native @NoException(true) void setChannelAxis(int channelAxis);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy