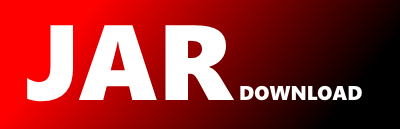
org.bytedeco.tensorrt.nvparsers.IUffParser Maven / Gradle / Ivy
// Targeted by JavaCPP version 1.5.7: DO NOT EDIT THIS FILE
package org.bytedeco.tensorrt.nvparsers;
import java.nio.*;
import org.bytedeco.javacpp.*;
import org.bytedeco.javacpp.annotation.*;
import static org.bytedeco.javacpp.presets.javacpp.*;
import org.bytedeco.cuda.cudart.*;
import static org.bytedeco.cuda.global.cudart.*;
import org.bytedeco.cuda.cublas.*;
import static org.bytedeco.cuda.global.cublas.*;
import org.bytedeco.cuda.cudnn.*;
import static org.bytedeco.cuda.global.cudnn.*;
import org.bytedeco.cuda.nvrtc.*;
import static org.bytedeco.cuda.global.nvrtc.*;
import org.bytedeco.tensorrt.nvinfer.*;
import static org.bytedeco.tensorrt.global.nvinfer.*;
import org.bytedeco.tensorrt.nvinfer_plugin.*;
import static org.bytedeco.tensorrt.global.nvinfer_plugin.*;
import static org.bytedeco.tensorrt.global.nvparsers.*;
/**
* \class IUffParser
*
* \brief Class used for parsing models described using the UFF format.
*
* \warning Do not inherit from this class, as doing so will break forward-compatibility of the API and ABI.
* */
@Namespace("nvuffparser") @Properties(inherit = org.bytedeco.tensorrt.presets.nvparsers.class)
public class IUffParser extends Pointer {
static { Loader.load(); }
/** Pointer cast constructor. Invokes {@link Pointer#Pointer(Pointer)}. */
public IUffParser(Pointer p) { super(p); }
/**
* \brief Register an input name of a UFF network with the associated Dimensions.
*
* @param inputName Input name.
* @param inputDims Input dimensions.
* @param inputOrder Input order on which the framework input was originally.
* */
//!
//!
//!
public native @Cast("bool") @NoException(true) boolean registerInput(String inputName, @ByVal @Cast("nvinfer1::Dims*") Dims32 inputDims, UffInputOrder inputOrder);
public native @Cast("bool") @NoException(true) boolean registerInput(@Cast("const char*") BytePointer inputName, @ByVal @Cast("nvinfer1::Dims*") Dims32 inputDims, @Cast("nvuffparser::UffInputOrder") int inputOrder);
/**
* \brief Register an output name of a UFF network.
*
* @param outputName Output name.
* */
//!
//!
//!
public native @Cast("bool") @NoException(true) boolean registerOutput(String outputName);
public native @Cast("bool") @NoException(true) boolean registerOutput(@Cast("const char*") BytePointer outputName);
/**
* \brief Parse a UFF file.
*
* @param file File name of the UFF file.
* @param network Network in which the UFFParser will fill the layers.
* @param weightsType The type on which the weights will transformed in.
* */
//!
//!
//!
public native @Cast("bool") @NoException(true) boolean parse(String file, @ByRef INetworkDefinition network,
DataType weightsType/*=nvinfer1::DataType::kFLOAT*/);
public native @Cast("bool") @NoException(true) boolean parse(String file, @ByRef INetworkDefinition network);
public native @Cast("bool") @NoException(true) boolean parse(@Cast("const char*") BytePointer file, @ByRef INetworkDefinition network,
@Cast("nvinfer1::DataType") int weightsType/*=nvinfer1::DataType::kFLOAT*/);
public native @Cast("bool") @NoException(true) boolean parse(@Cast("const char*") BytePointer file, @ByRef INetworkDefinition network);
/**
* \brief Parse a UFF buffer, useful if the file already live in memory.
*
* @param buffer Buffer of the UFF file.
* @param size Size of buffer of the UFF file.
* @param network Network in which the UFFParser will fill the layers.
* @param weightsType The type on which the weights will transformed in.
* */
//!
//!
public native @Cast("bool") @NoException(true) boolean parseBuffer(String buffer, @Cast("std::size_t") long size, @ByRef INetworkDefinition network,
DataType weightsType/*=nvinfer1::DataType::kFLOAT*/);
public native @Cast("bool") @NoException(true) boolean parseBuffer(String buffer, @Cast("std::size_t") long size, @ByRef INetworkDefinition network);
public native @Cast("bool") @NoException(true) boolean parseBuffer(@Cast("const char*") BytePointer buffer, @Cast("std::size_t") long size, @ByRef INetworkDefinition network,
@Cast("nvinfer1::DataType") int weightsType/*=nvinfer1::DataType::kFLOAT*/);
public native @Cast("bool") @NoException(true) boolean parseBuffer(@Cast("const char*") BytePointer buffer, @Cast("std::size_t") long size, @ByRef INetworkDefinition network);
/**
* @deprecated Deprecated interface will be removed in TensorRT 10.0.
* */
//!
//!
public native @Deprecated @NoException(true) void destroy();
/**
* \brief Return Version Major of the UFF.
* */
//!
//!
public native @NoException(true) int getUffRequiredVersionMajor();
/**
* \brief Return Version Minor of the UFF.
* */
//!
//!
public native @NoException(true) int getUffRequiredVersionMinor();
/**
* \brief Return Patch Version of the UFF.
* */
//!
//!
public native @NoException(true) int getUffRequiredVersionPatch();
/**
* \brief Set the namespace used to lookup and create plugins in the network.
* */
public native @NoException(true) void setPluginNamespace(String libNamespace);
public native @NoException(true) void setPluginNamespace(@Cast("const char*") BytePointer libNamespace);
/**
* \brief Set the ErrorRecorder for this interface
*
* Assigns the ErrorRecorder to this interface. The ErrorRecorder will track all errors during execution.
* This function will call incRefCount of the registered ErrorRecorder at least once. Setting
* recorder to nullptr unregisters the recorder with the interface, resulting in a call to decRefCount if
* a recorder has been registered.
*
* If an error recorder is not set, messages will be sent to the global log stream.
*
* @param recorder The error recorder to register with this interface. */
//
/** @see getErrorRecorder()
/** */
//!
//!
//!
//!
//!
public native @NoException(true) void setErrorRecorder(IErrorRecorder recorder);
/**
* \brief get the ErrorRecorder assigned to this interface.
*
* Retrieves the assigned error recorder object for the given class. A
* nullptr will be returned if setErrorRecorder has not been called.
*
* @return A pointer to the IErrorRecorder object that has been registered.
*
* @see setErrorRecorder()
* */
public native @NoException(true) IErrorRecorder getErrorRecorder();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy