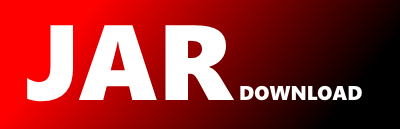
org.bytemechanics.service.repository.ServiceSupplier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of service-repository Show documentation
Show all versions of service-repository Show documentation
Repository to group all available services (stateless and statefull). The intention is have some point where all the internal project services are defined
/*
* Copyright 2017 Byte Mechanics.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.bytemechanics.service.repository;
import java.io.Closeable;
import java.text.MessageFormat;
import java.util.Arrays;
import java.util.Collections;
import java.util.Optional;
import java.util.function.Supplier;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.bytemechanics.service.repository.exceptions.ServiceDisposeException;
import org.bytemechanics.service.repository.exceptions.ServiceInitializationException;
import org.bytemechanics.service.repository.internal.ObjectFactory;
/**
* Service supplier interface to store all service metadata information and the current instance in case of singletons
* @author afarre
* @since 0.1.0
*/
public interface ServiceSupplier extends Supplier {
/**
* Method to return the service name
* @return Service name
*/
public String getName();
/**
* Method to return the service adapter interface that any implementation must accomplish in order to be an eligible implementation
* @return Service adapter interface
*/
public Class getAdapter();
/**
* Method to return if the service must be instantiated as singleton (only on instance in all virtual machine)
* @return true if the this service is a singleton
*/
public boolean isSingleton();
/**
* Method to return the current supplier for this service
* @return the Supplier for this service
* @see Supplier
*/
public Supplier getSupplier();
/**
* Method to retrieve the current singleton service instance
* @return singleton service instance or null if is not a singleton or still not instantiated
*/
public Object getInstance();
/**
* Method to store the new singleton service instance
* @param _instance instance to store
*/
public void setInstance(final Object _instance);
/**
* Method to obtain the service instance, note that if the service is a singleton always returns the same instance
* @return the optional service instance as Object that can be empty if the instance can not be obtained
*/
@SuppressWarnings("DoubleCheckedLocking")
public default Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy