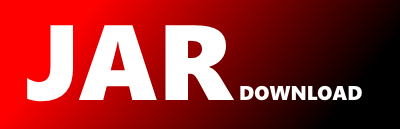
org.cache2k.CacheBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cache2k-api Show documentation
Show all versions of cache2k-api Show documentation
A light weight and high performance Java cache library. API only jar.
package org.cache2k;
/*
* #%L
* cache2k API
* %%
* Copyright (C) 2000 - 2016 headissue GmbH, Munich
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import org.cache2k.configuration.Cache2kConfiguration;
import org.cache2k.configuration.CacheType;
import org.cache2k.expiry.*;
import org.cache2k.event.CacheEntryOperationListener;
import org.cache2k.integration.AdvancedCacheLoader;
import org.cache2k.integration.CacheLoader;
import org.cache2k.integration.CacheWriter;
import org.cache2k.integration.ExceptionPropagator;
import org.cache2k.integration.ExceptionInformation;
import org.cache2k.integration.ResiliencePolicy;
import java.util.Collection;
import java.util.Collections;
import java.util.concurrent.TimeUnit;
/**
* @deprecated Replaced with {@link Cache2kBuilder}
*/
public class CacheBuilder {
public static CacheBuilder,?> newCache() {
return new CacheBuilder(Cache2kBuilder.forUnknownTypes());
}
public static CacheBuilder newCache(Class _keyType, Class _valueType) {
return fromConfig(Cache2kConfiguration.of(_keyType, _valueType));
}
@SuppressWarnings("unchecked")
public static , T> CacheBuilder newCache(
Class _keyType, Class _collectionType, Class _entryType) {
return newCache(_keyType, _collectionType);
}
public static CacheBuilder fromConfig(final Cache2kConfiguration c) {
return new CacheBuilder(Cache2kBuilder.of(c));
}
public CacheBuilder(final Cache2kBuilder _builder) {
builder = _builder;
/** backwards compatible */
builder.permitNullValues(true);
}
Cache2kBuilder builder;
public CacheBuilder addListener(final CacheEntryOperationListener listener) {
builder.addListener(listener);
return this;
}
public CacheBuilder entryCapacity(final int v) {
builder.entryCapacity(v);
return this;
}
public CacheBuilder loaderThreadCount(final int v) {
builder.loaderThreadCount(v);
return this;
}
/**
* @deprecated Replaced by {@link Cache2kBuilder#expireAfterWrite}
*/
public CacheBuilder expiryDuration(final long v, final TimeUnit u) {
builder.expireAfterWrite(v, u);
return this;
}
public CacheBuilder entryExpiryCalculator(final EntryExpiryCalculator c) {
builder.expiryPolicy(c);
return this;
}
public CacheBuilder name(final Class> _class) {
name(_class.getSimpleName());
return this;
}
public CacheBuilder name(final Object _containingObject, final String _fieldName) {
name(_containingObject.getClass(), _fieldName);
return this;
}
public CacheBuilder loader(final CacheLoader l) {
builder.loader(l);
return this;
}
public CacheBuilder sharpExpiry(final boolean f) {
builder.sharpExpiry(f);
return this;
}
public CacheBuilder eternal(final boolean v) {
builder.eternal(v);
return this;
}
public CacheBuilder suppressExceptions(final boolean v) {
builder.suppressExceptions(v);
return this;
}
public CacheBuilder writer(final CacheWriter w) {
builder.writer(w);
return this;
}
public CacheBuilder heapEntryCapacity(final int v) {
builder.toConfiguration().setHeapEntryCapacity(v);
return this;
}
public CacheBuilder valueType(final Class t) {
builder.valueType(t);
return (CacheBuilder) this;
}
public CacheBuilder keyType(final Class t) {
builder.keyType(t);
return (CacheBuilder) this;
}
public CacheBuilder expiryCalculator(final ExpiryPolicy c) {
builder.expiryPolicy(c);
return this;
}
public CacheBuilder name(final Class> _class, final String _fieldName) {
builder.name(_class.getSimpleName() + "." + _fieldName);
return this;
}
public CacheBuilder exceptionExpiryDuration(final long v, final TimeUnit u) {
builder.retryInterval(v, u);
return this;
}
public Cache build() {
return builder.build();
}
public Cache2kConfiguration createConfiguration() {
return builder.toConfiguration();
}
public CacheBuilder manager(final CacheManager m) {
builder.manager(m);
return this;
}
public CacheBuilder storeByReference(final boolean v) {
builder.storeByReference(v);
return this;
}
@Deprecated
public Cache2kConfiguration getConfig() {
return builder.toConfiguration();
}
public CacheBuilder expirySecs(final int v) {
builder.expireAfterWrite(v, TimeUnit.SECONDS);
return this;
}
public CacheBuilder maxSizeBound(final int v) {
return this;
}
public CacheBuilder name(final String v) {
builder.name(v);
return this;
}
public CacheBuilder source(final CacheSourceWithMetaInfo eg) {
builder.loader(new AdvancedCacheLoader() {
@Override
public V load(final K key, final long currentTime, final CacheEntry previousEntry) throws Exception {
try {
if (previousEntry != null && previousEntry.getException() == null) {
return eg.get(key, currentTime, previousEntry.getValue(), previousEntry.getLastModification());
} else {
return eg.get(key, currentTime, null, 0);
}
} catch (Exception e) {
throw e;
} catch (Throwable t) {
throw new RuntimeException("rethrow throwable", t);
}
}
});
return this;
}
/**
* Removed without replacement.
*/
public CacheBuilder implementation(final Class> c) {
return this;
}
public CacheBuilder keyType(final CacheType t) {
builder.keyType(t);
return (CacheBuilder) this;
}
public CacheBuilder expiryMillis(final long v) {
builder.expireAfterWrite(v, TimeUnit.MILLISECONDS);
return this;
}
public CacheBuilder backgroundRefresh(final boolean f) {
builder.refreshAhead(f);
return this;
}
public CacheBuilder source(final CacheSource g) {
builder.loader(new AdvancedCacheLoader() {
@Override
public V load(final K key, final long currentTime, final CacheEntry previousEntry) throws Exception {
try {
return g.get(key);
} catch (Exception e) {
throw e;
} catch (Throwable t) {
throw new RuntimeException("rethrow throwable", t);
}
}
});
return this;
}
public CacheBuilder source(final BulkCacheSource s) {
builder.loader(new AdvancedCacheLoader() {
@Override
public V load(final K key, final long currentTime, final CacheEntry previousEntry) throws Exception {
CacheEntry entry = previousEntry;
if (previousEntry == null)
entry = new CacheEntry() {
@Override
public K getKey() {
return key;
}
@Override
public V getValue() {
return null;
}
@Override
public Throwable getException() {
return null;
}
@Override
public long getLastModification() {
return 0;
}
};
try {
return s.getValues(Collections.singletonList(entry), currentTime).get(0);
} catch (Exception e) {
throw e;
} catch (Throwable t) {
throw new RuntimeException("rethrow throwable", t);
}
}
});
return this;
}
public CacheBuilder exceptionExpiryCalculator(final org.cache2k.ExceptionExpiryCalculator c) {
builder.resiliencePolicy(new ResiliencePolicy() {
@Override
public long suppressExceptionUntil(final K key, final ExceptionInformation exceptionInformation, final CacheEntry cachedContent) {
return c.calculateExpiryTime(key, exceptionInformation.getException(), exceptionInformation.getLoadTime());
}
@Override
public long retryLoadAfter(final K key, final ExceptionInformation exceptionInformation) {
return c.calculateExpiryTime(key, exceptionInformation.getException(), exceptionInformation.getLoadTime());
}
});
return this;
}
public CacheBuilder maxSize(final int v) {
builder.entryCapacity(v);
return this;
}
public CacheBuilder valueType(final CacheType t) {
builder.valueType(t);
return (CacheBuilder) this;
}
public CacheBuilder keepDataAfterExpired(final boolean v) {
builder.keepDataAfterExpired(v);
return this;
}
public CacheBuilder refreshController(final RefreshController lc) {
expiryCalculator(new ExpiryPolicy() {
@Override
public long calculateExpiryTime(K _key, V _value, long _loadTime, CacheEntry _oldEntry) {
if (_oldEntry != null) {
return lc.calculateNextRefreshTime(_oldEntry.getValue(), _value, _oldEntry.getLastModification(), _loadTime);
} else {
return lc.calculateNextRefreshTime(null, _value, 0L, _loadTime);
}
}
});
return this;
}
public CacheBuilder refreshAhead(final boolean f) {
builder.refreshAhead(f);
return this;
}
public CacheBuilder exceptionPropagator(final ExceptionPropagator ep) {
builder.exceptionPropagator(ep);
return this;
}
public CacheBuilder loader(final AdvancedCacheLoader l) {
builder.loader(l);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy