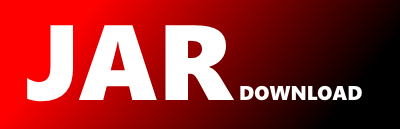
org.cache2k.processor.MutableCacheEntry Maven / Gradle / Ivy
Show all versions of cache2k-api Show documentation
package org.cache2k.processor;
/*
* #%L
* cache2k API
* %%
* Copyright (C) 2000 - 2016 headissue GmbH, Munich
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import org.cache2k.CacheEntry;
import org.cache2k.integration.CacheLoader;
/**
* A mutable entry is used inside the {@link EntryProcessor} to perform
* updates and retrieve information if the entry is existing in the cache.
*
* A mutation is only done if a method for mutation is called, e.g.
* {@code setValue} or {@code remove}. If multiple mutate methods
* are called only the last method will be accounted for.
*
*
One instance is only intended for usage by a single thread.
*
* @see EntryProcessor
* @author Jens Wilke
*/
public interface MutableCacheEntry extends CacheEntry {
/**
* Returns the value to which the cache associated the key,
* or {@code null} if the cache contains no mapping for this key.
* {@code null} is also returned if this entry contains an exception.
*
*
If the cache does permit null values, then a return value of
* {@code null} does not necessarily indicate that the cache
* contained no mapping for the key. It is also possible that the cache
* explicitly associated the key to the value {@code null}. Use {@link #exists()}
* to check whether an entry is existing instead of a null check.
*
*
If read through operation is enabled and the entry not yet existing
* in the cache, the call to this method triggers a call to the cache loader.
*
*
In contrast to the main Cache interface there is no no peekValue method,
* since the same effect can be achieved by the combination of {@link #exists()}
* and {@link #getValue()}.
*
* @throws RestartException If the information is not yet available and the cache
* needs to do an asynchronous operation to supply it.
* After completion, the entry processor will be
* executed again.
* @see CacheLoader
*/
@Override
V getValue();
/**
* True if a mapping exists in the cache, never invokes the loader / cache source.
*
*
After this method returns true, a call to {@code getValue} will always
* return the cached value and never invoke the loader. The potential expiry
* of the value is only checked once and the return values of this method and
* {@code getValue} will be consistent.
*
* @throws RestartException If the information is not yet available and the cache
* needs to do an asynchronous operation to supply it.
* After completion, the entry processor will be
* executed again.
*/
boolean exists();
/**
* Insert or updates the cache value assigned to this key. After calling this method
* {@code exists} will return true and {@code getValue} will return the set value.
*
*
If a writer is registered, the {@link org.cache2k.integration.CacheWriter#write(Object, Object)}
* is called.
*/
void setValue(V v);
/**
* Removes an entry from the cache.
*
*
In case a writer is registered, {@link org.cache2k.integration.CacheWriter#delete}
* is called. If a remove is performed on a not existing cache entry the writer
* method will also be called.
*
* @see © 2015 - 2025 Weber Informatics LLC | Privacy Policy