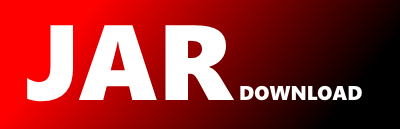
org.caffinitas.ohc.OHCacheBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ohc-core Show documentation
Show all versions of ohc-core Show documentation
Off-Heap concurrent hash map intended to store GBs of serialized data
/*
* Copyright (C) 2014 Robert Stupp, Koeln, Germany, robert-stupp.de
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.caffinitas.ohc;
import java.lang.reflect.InvocationTargetException;
import java.util.concurrent.ScheduledExecutorService;
import org.caffinitas.ohc.linked.OHCacheImpl;
/**
* Configures and builds OHC instance.
*
*
* Field
* Meaning
* Default
*
*
* {@code keySerializer}
* Serializer implementation used for keys
* Must be configured
*
*
* {@code valueSerializer}
* Serializer implementation used for values
* Must be configured
*
*
* {@code executorService}
* Executor service required for get operations using a cache loader. E.g. {@link org.caffinitas.ohc.OHCache#getWithLoaderAsync(Object, CacheLoader)}
* (Not configured by default meaning get operations with cache loader not supported by default)
*
*
* {@code segmentCount}
* Number of segments
* 2 * number of CPUs ({@code java.lang.Runtime.availableProcessors()})
*
*
* {@code hashTableSize}
* Initial size of each segment's hash table
* {@code 8192}
*
*
* {@code loadFactor}
* Hash table load factor. I.e. determines when rehashing occurs.
* {@code .75f}
*
*
* {@code capacity}
* Capacity of the cache in bytes
* 16 MB * number of CPUs ({@code java.lang.Runtime.availableProcessors()}), minimum 64 MB
*
*
* {@code maxEntrySize}
* Maximum size of a hash entry (including header, serialized key + serialized value)
* (not set, defaults to capacity)
*
*
* {@code bucketLength}
* (For tables implementation only) Number of entries per bucket.
* {@code 8}
*
*
*
* You may also use system properties prefixed with {@code org.caffinitas.org.} to other defaults.
* E.g. the system property {@code org.caffinitas.org.segmentCount} configures the default of the number of segments.
*
*
* @param cache key type
* @param cache value type
*/
public class OHCacheBuilder
{
private int segmentCount;
private int hashTableSize = 8192;
private int bucketLength = 8;
private long capacity;
private CacheSerializer keySerializer;
private CacheSerializer valueSerializer;
private float loadFactor = .75f;
private long maxEntrySize;
private Class extends OHCache> type = OHCacheImpl.class;
private ScheduledExecutorService executorService;
private OHCacheBuilder()
{
int cpus = Runtime.getRuntime().availableProcessors();
segmentCount = roundUpToPowerOf2(cpus * 2, 1 << 30);
capacity = Math.min(cpus * 16, 64) * 1024 * 1024;
segmentCount = fromSystemProperties("segmentCount", segmentCount);
hashTableSize = fromSystemProperties("hashTableSize", hashTableSize);
bucketLength = fromSystemProperties("bucketLength", bucketLength);
capacity = fromSystemProperties("capacity", capacity);
loadFactor = fromSystemProperties("loadFactor", loadFactor);
maxEntrySize = fromSystemProperties("maxEntrySize", maxEntrySize);
String t = fromSystemProperties("type", null);
if (t != null)
try
{
type = (Class extends OHCache>) Class.forName(t);
}
catch (ClassNotFoundException x)
{
try
{
type = (Class extends OHCache>) Class.forName("org.caffinitas.ohc." + t + ".OHCacheImpl");
}
catch (ClassNotFoundException e)
{
throw new RuntimeException(e);
}
}
}
public static final String SYSTEM_PROPERTY_PREFIX = "org.caffinitas.org.";
private static float fromSystemProperties(String name, float defaultValue)
{
return Float.parseFloat(System.getProperty(SYSTEM_PROPERTY_PREFIX + name, Float.toString(defaultValue)));
}
private static long fromSystemProperties(String name, long defaultValue)
{
return Long.parseLong(System.getProperty(SYSTEM_PROPERTY_PREFIX + name, Long.toString(defaultValue)));
}
private static int fromSystemProperties(String name, int defaultValue)
{
return Integer.parseInt(System.getProperty(SYSTEM_PROPERTY_PREFIX + name, Integer.toString(defaultValue)));
}
private static String fromSystemProperties(String name, String defaultValue)
{
return System.getProperty(SYSTEM_PROPERTY_PREFIX + name, defaultValue);
}
static int roundUpToPowerOf2(int number, int max)
{
return number >= max
? max
: (number > 1) ? Integer.highestOneBit((number - 1) << 1) : 1;
}
public static OHCacheBuilder newBuilder()
{
return new OHCacheBuilder<>();
}
public OHCache build()
{
try
{
return type.getDeclaredConstructor(OHCacheBuilder.class).newInstance(this);
}
catch (InstantiationException | IllegalAccessException | NoSuchMethodException | InvocationTargetException e)
{
throw new RuntimeException(e);
}
}
public Class extends OHCache> getType()
{
return type;
}
public OHCacheBuilder type(Class extends OHCache> type)
{
this.type = type;
return this;
}
public int getHashTableSize()
{
return hashTableSize;
}
public OHCacheBuilder hashTableSize(int hashTableSize)
{
this.hashTableSize = hashTableSize;
return this;
}
public int getBucketLength()
{
return bucketLength;
}
public OHCacheBuilder bucketLength(int bucketLength)
{
this.bucketLength = bucketLength;
return this;
}
public long getCapacity()
{
return capacity;
}
public OHCacheBuilder capacity(long capacity)
{
this.capacity = capacity;
return this;
}
public CacheSerializer getKeySerializer()
{
return keySerializer;
}
public OHCacheBuilder keySerializer(CacheSerializer keySerializer)
{
this.keySerializer = keySerializer;
return this;
}
public CacheSerializer getValueSerializer()
{
return valueSerializer;
}
public OHCacheBuilder valueSerializer(CacheSerializer valueSerializer)
{
this.valueSerializer = valueSerializer;
return this;
}
public int getSegmentCount()
{
return segmentCount;
}
public OHCacheBuilder segmentCount(int segmentCount)
{
this.segmentCount = segmentCount;
return this;
}
public float getLoadFactor()
{
return loadFactor;
}
public OHCacheBuilder loadFactor(float loadFactor)
{
this.loadFactor = loadFactor;
return this;
}
public long getMaxEntrySize()
{
return maxEntrySize;
}
public OHCacheBuilder maxEntrySize(long maxEntrySize)
{
this.maxEntrySize = maxEntrySize;
return this;
}
public ScheduledExecutorService getExecutorService()
{
return executorService;
}
public OHCacheBuilder executorService(ScheduledExecutorService executorService)
{
this.executorService = executorService;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy