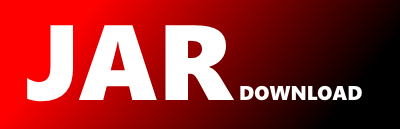
org.camunda.bpm.dmn.xlsx.XlsxConverter Maven / Gradle / Ivy
/* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.camunda.bpm.dmn.xlsx;
import java.io.InputStream;
import org.apache.fop.fo.properties.Property;
import org.camunda.bpm.model.dmn.DmnModelInstance;
import org.docx4j.docProps.core.CoreProperties;
import org.docx4j.docProps.extended.Properties;
import org.docx4j.openpackaging.exceptions.Docx4JException;
import org.docx4j.openpackaging.packages.OpcPackage;
import org.docx4j.openpackaging.packages.SpreadsheetMLPackage;
import org.docx4j.openpackaging.parts.SpreadsheetML.SharedStrings;
import org.docx4j.openpackaging.parts.SpreadsheetML.WorkbookPart;
import org.docx4j.openpackaging.parts.SpreadsheetML.WorksheetPart;
import org.xlsx4j.exceptions.Xlsx4jException;
import org.xlsx4j.sml.Worksheet;
/**
* @author Thorben Lindhauer
*
*/
public class XlsxConverter {
protected InputOutputDetectionStrategy ioDetectionStrategy = new SimpleInputOutputDetectionStrategy();
public DmnModelInstance convert(InputStream inputStream) {
SpreadsheetMLPackage spreadSheetPackage = null;
try {
spreadSheetPackage = (SpreadsheetMLPackage) SpreadsheetMLPackage.load(inputStream);
} catch (Docx4JException e) {
// TODO: checked exception
throw new RuntimeException("cannot load document", e);
}
WorkbookPart workbookPart = spreadSheetPackage.getWorkbookPart();
// TODO: exception when no worksheet present
// TODO: make worksheet number configurable/import all worksheets?
XlsxWorksheetContext worksheetContext = null;
WorksheetPart worksheetPart;
try {
Properties properties = spreadSheetPackage.getDocPropsExtendedPart().getContents();
String worksheetName = (String) properties.getTitlesOfParts().getVector().getVariantOrI1OrI2().get(0).getValue();
worksheetPart = workbookPart.getWorksheet(0);
SharedStrings sharedStrings = workbookPart.getSharedStrings();
worksheetContext = new XlsxWorksheetContext(sharedStrings.getContents(), worksheetPart.getContents(), worksheetName);
} catch (Exception e) {
throw new RuntimeException("Could not determine worksheet", e);
}
return new XlsxWorksheetConverter(worksheetContext, ioDetectionStrategy).convert();
}
public InputOutputDetectionStrategy getIoDetectionStrategy() {
return ioDetectionStrategy;
}
public void setIoDetectionStrategy(InputOutputDetectionStrategy ioDetectionStrategy) {
this.ioDetectionStrategy = ioDetectionStrategy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy