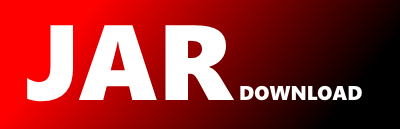
org.camunda.bpm.extension.keycloak.cache.CaffeineCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camunda-bpm-identity-keycloak Show documentation
Show all versions of camunda-bpm-identity-keycloak Show documentation
A Camunda Identity Provider Plugin for Keycloak
package org.camunda.bpm.extension.keycloak.cache;
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
import com.github.benmanes.caffeine.cache.Ticker;
import java.util.concurrent.ConcurrentMap;
import java.util.function.Function;
/**
* An implementation of QueryCache backed by Caffeine.
*/
public class CaffeineCache implements QueryCache {
/** The cache. */
private final Cache cache;
/**
* Creates a new Caffeine backed cache.
* @param config the cach configuration
*/
public CaffeineCache(CacheConfiguration config) {
this(config, Ticker.systemTicker());
}
/**
* Creates a new Caffeine backed cache.
* @param config the cache configuration
* @param ticker ticker to be used by the cache to measure durations
*/
public CaffeineCache(CacheConfiguration config, Ticker ticker) {
this.cache = Caffeine.newBuilder().ticker(ticker).maximumSize(config.getMaxSize())
.expireAfterWrite(config.getExpirationTimeout()).build();
}
/**
* {@inheritDoc}
*/
@Override
public V getOrCompute(K key, Function computation) {
return this.cache.get(key, computation);
}
/**
* {@inheritDoc}
*/
@Override
public void clear() {
this.cache.invalidateAll();
}
/**
* To be used in tests to trigger any pending eviction tasks. This is required
* since caffeine performs these asynchronously. To make tests predictable and
* deterministic, this method should be called after every potential cacheable
* action.
*/
public void cleanUp() {
this.cache.cleanUp();
}
/**
* Returns cached entries as a map. Useful for asserting entries in tests.
*/
public ConcurrentMap asMap() {
return this.cache.asMap();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy