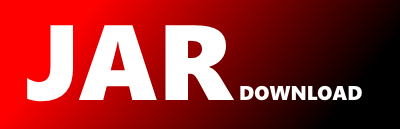
org.camunda.bpm.extension.mockito.query.AbstractQueryMock Maven / Gradle / Ivy
package org.camunda.bpm.extension.mockito.query;
import static com.google.common.base.Throwables.propagate;
import static org.mockito.Mockito.when;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Nonnull;
import org.camunda.bpm.engine.query.Query;
import org.camunda.bpm.extension.mockito.answer.FluentAnswer;
import com.google.common.base.Supplier;
/**
* This looks more complicated than it actually is ... To easily mock the
* behaviour of queries, all common functionality is extracted to this abstract
* super class, the high level of Generics is needed for the fluent api pattern,
* so the abstract mock implementing the generic Query interface must also keep
* a reference to itself.
*
* @param
* the type of the AbstractQueryMock (repeat the type of the class you
* are building). Used to "return this"
* @param
* the type of the query to mock (for example: TaskQuery).
* @param
* the type of the expected result (for example: Execution).
* @param
* the type of the service the query belongs to (used for "forService"
* binding), for Example: TaskService.
*
* @author Jan Galinski
*/
abstract class AbstractQueryMock, Q extends Query, R>, R extends Object, S> implements Supplier {
/**
* The internally stored query instance.
*/
private final Q query;
/**
* Used for mocking the query creation via reflection.
*/
private final Method createMethod;
/**
* Creates a new query mock and mocks fluent api behavior by adding a default
* answer to the mock. Every createMethod will return the mock itself, except
*
* - list() - returns empty ArrayList
* - singeResult() - returns null
*
*
* @param queryType
* the type of the query to mock.
* @param serviceType
* the type of service that generates this query
*/
protected AbstractQueryMock(@Nonnull final Class queryType, @Nonnull final Class serviceType) {
query = FluentAnswer.createMock(queryType);
createMethod = createMethod(queryType, serviceType);
list(new ArrayList());
singleResult(null);
}
private Method createMethod(@Nonnull final Class queryType, @Nonnull Class serviceType) {
try {
return serviceType.getDeclaredMethod("create" + queryType.getSimpleName());
} catch (NoSuchMethodException e) {
throw propagate(e);
}
}
public final Q list(final List result) {
when(query.list()).thenReturn(result);
return get();
}
public final Q singleResult(final R result) {
when(query.singleResult()).thenReturn(result);
return get();
}
public final Q count(long count) {
when(query.count()).thenReturn(count);
return get();
}
public M forService(S service) {
try {
when(createMethod.invoke(service)).thenReturn(get());
} catch (Exception e) {
propagate(e);
}
return (M) this;
}
@Override
public final Q get() {
return query;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy