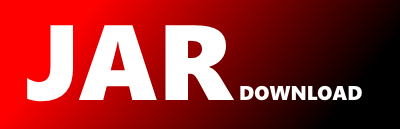
org.camunda.bpm.engine.impl.util.JsonUtil Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH
* under one or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information regarding copyright
* ownership. Camunda licenses this file to you under the Apache License,
* Version 2.0; you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.camunda.bpm.engine.impl.util;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Supplier;
import org.camunda.bpm.engine.impl.ProcessEngineLogger;
import org.camunda.bpm.engine.impl.json.JsonObjectConverter;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonIOException;
import com.google.gson.JsonNull;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.JsonPrimitive;
import com.google.gson.JsonSyntaxException;
import com.google.gson.internal.LazilyParsedNumber;
/**
* @author Tassilo Weidner
*/
public final class JsonUtil {
private static final EngineUtilLogger LOG = ProcessEngineLogger.UTIL_LOGGER;
protected static Gson gsonMapper = createGsonMapper();
public static void addFieldRawValue(JsonObject jsonObject, String memberName, Object rawValue) {
if (rawValue != null) {
JsonElement jsonNode = null;
try {
jsonNode = getGsonMapper().toJsonTree(rawValue);
} catch (JsonIOException e) {
LOG.logJsonException(e);
}
if (jsonNode != null) {
jsonObject.add(memberName, jsonNode);
}
}
}
public static void addField(JsonObject jsonObject, String name, JsonObjectConverter converter, T value) {
if (jsonObject != null && name != null && converter != null && value != null) {
jsonObject.add(name, converter.toJsonObject(value));
}
}
public static void addListField(JsonObject jsonObject, String name, List list) {
if (jsonObject != null && name != null && list != null) {
jsonObject.add(name, asArray(list));
}
}
public static void addArrayField(JsonObject jsonObject, String name, String[] array) {
if (jsonObject != null && name != null && array != null) {
addListField(jsonObject, name, Arrays.asList(array));
}
}
public static void addDateField(JsonObject jsonObject, String name, Date date) {
if (jsonObject != null && name != null && date != null) {
jsonObject.addProperty(name, date.getTime());
}
}
public static void addElement(JsonArray jsonObject, JsonObjectConverter converter, T value) {
if (jsonObject != null && converter != null && value != null) {
JsonObject jsonElement = converter.toJsonObject(value);
if (jsonElement != null) {
jsonObject.add(jsonElement);
}
}
}
public static void addListField(JsonObject jsonObject, String name, JsonObjectConverter converter, List list) {
if (jsonObject != null && name != null && converter != null && list != null) {
JsonArray arrayNode = createArray();
for (T item : list) {
if (item != null) {
JsonObject jsonElement = converter.toJsonObject(item);
if (jsonElement != null) {
arrayNode.add(jsonElement);
}
}
}
jsonObject.add(name, arrayNode);
}
}
public static T asJavaObject(JsonObject jsonObject, JsonObjectConverter converter) {
if (jsonObject != null && converter != null) {
return converter.toObject(jsonObject);
} else {
return null;
}
}
public static void addNullField(JsonObject jsonObject, String name) {
if (jsonObject != null && name != null) {
jsonObject.add(name, JsonNull.INSTANCE);
}
}
public static void addField(JsonObject jsonObject, String name, JsonArray value) {
if (jsonObject != null && name != null && value != null) {
jsonObject.add(name, value);
}
}
public static void addField(JsonObject jsonObject, String name, String value) {
if (jsonObject != null && name != null && value != null) {
jsonObject.addProperty(name, value);
}
}
public static void addField(JsonObject jsonObject, String name, Boolean value) {
if (jsonObject != null && name != null && value != null) {
jsonObject.addProperty(name, value);
}
}
public static void addField(JsonObject jsonObject, String name, Integer value) {
if (jsonObject != null && name != null && value != null) {
jsonObject.addProperty(name, value);
}
}
public static void addField(JsonObject jsonObject, String name, Short value) {
if (jsonObject != null && name != null && value != null) {
jsonObject.addProperty(name, value);
}
}
public static void addField(JsonObject jsonObject, String name, Long value) {
if (jsonObject != null && name != null && value != null) {
jsonObject.addProperty(name, value);
}
}
public static void addField(JsonObject jsonObject, String name, Double value) {
if (jsonObject != null && name != null && value != null) {
jsonObject.addProperty(name, value);
}
}
public static void addDefaultField(JsonObject jsonObject, String name, boolean defaultValue, Boolean value) {
if (jsonObject != null && name != null && value != null && !value.equals(defaultValue)) {
addField(jsonObject, name, value);
}
}
public static byte[] asBytes(JsonElement jsonObject) {
String jsonString = null;
if (jsonObject != null) {
try {
jsonString = getGsonMapper().toJson(jsonObject);
} catch (JsonIOException e) {
LOG.logJsonException(e);
}
}
if (jsonString == null) {
jsonString = "";
}
return StringUtil.toByteArray(jsonString);
}
public static JsonObject asObject(byte[] byteArray) {
String stringValue = null;
if (byteArray != null) {
stringValue = StringUtil.fromBytes(byteArray);
}
if (stringValue == null) {
return createObject();
}
JsonObject jsonObject = null;
try {
jsonObject = getGsonMapper().fromJson(stringValue, JsonObject.class);
} catch (JsonParseException e) {
LOG.logJsonException(e);
}
if (jsonObject != null) {
return jsonObject;
} else {
return createObject();
}
}
public static JsonObject asObject(String jsonString) {
JsonObject jsonObject = null;
if (jsonString != null) {
try {
jsonObject = getGsonMapper().fromJson(jsonString, JsonObject.class);
} catch (JsonParseException | ClassCastException e) {
LOG.logJsonException(e);
}
}
if (jsonObject != null) {
return jsonObject;
} else {
return createObject();
}
}
public static JsonObject asObject(Map properties) {
if (properties != null) {
JsonObject jsonObject = null;
try {
jsonObject = (JsonObject) getGsonMapper().toJsonTree(properties);
} catch (JsonIOException | ClassCastException e) {
LOG.logJsonException(e);
}
if (jsonObject != null) {
return jsonObject;
} else {
return createObject();
}
} else {
return createObject();
}
}
public static List asStringList(JsonElement jsonObject) {
JsonArray jsonArray = null;
if (jsonObject != null) {
try {
jsonArray = jsonObject.getAsJsonArray();
} catch (IllegalStateException | ClassCastException e) {
LOG.logJsonException(e);
}
}
if (jsonArray == null) {
return Collections.emptyList();
}
List list = new ArrayList<>();
for (JsonElement entry : jsonArray) {
String stringValue = null;
try {
stringValue = entry.getAsString();
} catch (IllegalStateException | ClassCastException e) {
LOG.logJsonException(e);
}
if (stringValue != null) {
list.add(stringValue);
}
}
return list;
}
@SuppressWarnings("unchecked")
public static > S asList(JsonArray jsonArray, JsonObjectConverter converter, Supplier listSupplier) {
if (jsonArray == null || converter == null) {
return (S) Collections.emptyList();
}
S list = listSupplier.get();
for (JsonElement element : jsonArray) {
JsonObject jsonObject = null;
try {
jsonObject = element.getAsJsonObject();
} catch (IllegalStateException | ClassCastException e) {
LOG.logJsonException(e);
}
if (jsonObject != null) {
T rawObject = converter.toObject(jsonObject);
if (rawObject != null) {
list.add(rawObject);
}
}
}
return list;
}
public static List asList(JsonArray jsonArray, JsonObjectConverter converter) {
return asList(jsonArray, converter, ArrayList::new);
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy