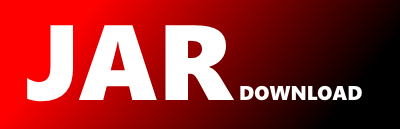
org.camunda.bpm.container.impl.jmx.MBeanServiceContainer Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH
* under one or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information regarding copyright
* ownership. Camunda licenses this file to you under the Apache License,
* Version 2.0; you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.camunda.bpm.container.impl.jmx;
import java.lang.management.ManagementFactory;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.Stack;
import java.util.concurrent.ConcurrentHashMap;
import javax.management.MBeanServer;
import javax.management.ObjectName;
import org.camunda.bpm.container.impl.ContainerIntegrationLogger;
import org.camunda.bpm.container.impl.spi.DeploymentOperation;
import org.camunda.bpm.container.impl.spi.DeploymentOperation.DeploymentOperationBuilder;
import org.camunda.bpm.container.impl.spi.PlatformService;
import org.camunda.bpm.container.impl.spi.PlatformServiceContainer;
import org.camunda.bpm.engine.ProcessEngineException;
import org.camunda.bpm.engine.impl.ProcessEngineLogger;
import static org.camunda.bpm.engine.impl.util.EnsureUtil.ensureNotNull;
/**
* A simple Service Container that delegates to the JVM's {@link MBeanServer}.
*
* @author Daniel Meyer
*
*/
public class MBeanServiceContainer implements PlatformServiceContainer {
private final static ContainerIntegrationLogger LOG = ProcessEngineLogger.CONTAINER_INTEGRATION_LOGGER;
protected MBeanServer mBeanServer;
protected Map> servicesByName = new ConcurrentHashMap>();
/** set if the current thread is performing a composite deployment operation */
protected ThreadLocal> activeDeploymentOperations = new ThreadLocal>();
public final static String SERVICE_NAME_EXECUTOR = "executor-service";
public synchronized void startService(ServiceType serviceType, String localName, PlatformService service) {
String serviceName = composeLocalName(serviceType, localName);
startService(serviceName, service);
}
public synchronized void startService(String name, PlatformService service) {
ObjectName serviceName = getObjectName(name);
if (getService(serviceName) != null) {
throw new ProcessEngineException("Cannot register service " + serviceName + " with MBeans Container, service with same name already registered.");
}
final MBeanServer beanServer = getmBeanServer();
// call the service-provided start behavior
service.start(this);
try {
beanServer.registerMBean(service, serviceName);
servicesByName.put(serviceName, service);
Stack currentOperationContext = activeDeploymentOperations.get();
if (currentOperationContext != null) {
currentOperationContext.peek().serviceAdded(name);
}
}
catch (Exception e) {
throw LOG.cannotRegisterService(serviceName, e);
}
}
public static ObjectName getObjectName(String serviceName) {
try {
return new ObjectName(serviceName);
}
catch(Exception e) {
throw LOG.cannotComposeNameFor(serviceName, e);
}
}
public static String composeLocalName(ServiceType type, String localName) {
return type.getTypeName() + ":type=" + localName;
}
public synchronized void stopService(ServiceType serviceType, String localName) {
String globalName = composeLocalName(serviceType, localName);
stopService(globalName);
}
public synchronized void stopService(String name) {
final MBeanServer mBeanServer = getmBeanServer();
ObjectName serviceName = getObjectName(name);
final PlatformService
© 2015 - 2024 Weber Informatics LLC | Privacy Policy