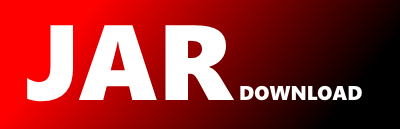
org.camunda.bpm.engine.impl.OptimizeService Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH
* under one or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information regarding copyright
* ownership. Camunda licenses this file to you under the Apache License,
* Version 2.0; you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.camunda.bpm.engine.impl;
import org.camunda.bpm.engine.history.HistoricActivityInstance;
import org.camunda.bpm.engine.history.HistoricDecisionInstance;
import org.camunda.bpm.engine.history.HistoricProcessInstance;
import org.camunda.bpm.engine.history.HistoricTaskInstance;
import org.camunda.bpm.engine.history.HistoricVariableUpdate;
import org.camunda.bpm.engine.history.UserOperationLogEntry;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeCompletedHistoricActivityInstanceQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeCompletedHistoricIncidentsQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeCompletedHistoricProcessInstanceQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeCompletedHistoricTaskInstanceQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeHistoricDecisionInstanceQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeHistoricIdentityLinkLogQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeHistoricUserOperationsLogQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeHistoricVariableUpdateQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeOpenHistoricIncidentsQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeRunningHistoricActivityInstanceQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeRunningHistoricProcessInstanceQueryCmd;
import org.camunda.bpm.engine.impl.cmd.optimize.OptimizeRunningHistoricTaskInstanceQueryCmd;
import org.camunda.bpm.engine.impl.persistence.entity.HistoricIncidentEntity;
import org.camunda.bpm.engine.impl.persistence.entity.optimize.OptimizeHistoricIdentityLinkLogEntity;
import java.util.Date;
import java.util.List;
public class OptimizeService extends ServiceImpl {
public List getCompletedHistoricActivityInstances(Date finishedAfter,
Date finishedAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeCompletedHistoricActivityInstanceQueryCmd(finishedAfter, finishedAt, maxResults)
);
}
public List getRunningHistoricActivityInstances(Date startedAfter,
Date startedAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeRunningHistoricActivityInstanceQueryCmd(startedAfter, startedAt, maxResults)
);
}
public List getCompletedHistoricTaskInstances(Date finishedAfter,
Date finishedAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeCompletedHistoricTaskInstanceQueryCmd(finishedAfter, finishedAt, maxResults)
);
}
public List getRunningHistoricTaskInstances(Date startedAfter,
Date startedAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeRunningHistoricTaskInstanceQueryCmd(startedAfter, startedAt, maxResults)
);
}
public List getHistoricUserOperationLogs(Date occurredAfter,
Date occurredAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeHistoricUserOperationsLogQueryCmd(occurredAfter, occurredAt, maxResults)
);
}
public List getHistoricIdentityLinkLogs(Date occurredAfter,
Date occurredAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeHistoricIdentityLinkLogQueryCmd(occurredAfter, occurredAt, maxResults)
);
}
public List getCompletedHistoricProcessInstances(Date finishedAfter,
Date finishedAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeCompletedHistoricProcessInstanceQueryCmd(finishedAfter, finishedAt, maxResults)
);
}
public List getRunningHistoricProcessInstances(Date startedAfter,
Date startedAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeRunningHistoricProcessInstanceQueryCmd(startedAfter, startedAt, maxResults)
);
}
public List getHistoricVariableUpdates(Date occurredAfter,
Date occurredAt,
boolean excludeObjectValues,
int maxResults) {
return commandExecutor.execute(
new OptimizeHistoricVariableUpdateQueryCmd(occurredAfter, occurredAt, excludeObjectValues, maxResults)
);
}
public List getCompletedHistoricIncidents(Date finishedAfter,
Date finishedAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeCompletedHistoricIncidentsQueryCmd(finishedAfter, finishedAt, maxResults)
);
}
public List getOpenHistoricIncidents(Date createdAfter,
Date createdAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeOpenHistoricIncidentsQueryCmd(createdAfter, createdAt, maxResults)
);
}
public List getHistoricDecisionInstances(Date evaluatedAfter,
Date evaluatedAt,
int maxResults) {
return commandExecutor.execute(
new OptimizeHistoricDecisionInstanceQueryCmd(evaluatedAfter, evaluatedAt, maxResults)
);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy