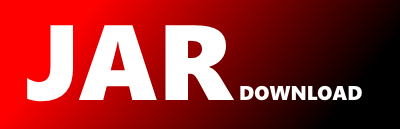
org.camunda.bpm.engine.impl.util.CollectionUtil Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH
* under one or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information regarding copyright
* ownership. Camunda licenses this file to you under the Apache License,
* Version 2.0; you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.camunda.bpm.engine.impl.util;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
/**
* helper/convience methods for working with collections.
*
* @author Joram Barrez
*/
public class CollectionUtil {
// No need to instantiate
private CollectionUtil() {}
/**
* Helper method that creates a singleton map.
*
* Alternative for Collections.singletonMap(), since that method returns a
* generic typed map depending on the input type, but we often need a
* map.
*/
public static Map singletonMap(String key, Object value) {
Map map = new HashMap<>();
map.put(key, value);
return map;
}
/**
* Arrays.asList cannot be reliably used for SQL parameters on MyBatis < 3.3.0
*/
public static List asArrayList(T[] values) {
ArrayList result = new ArrayList<>();
Collections.addAll(result, values);
return result;
}
public static Set asHashSet(T... elements) {
Set set = new HashSet<>();
Collections.addAll(set, elements);
return set;
}
public static void addToMapOfLists(Map> map, S key, T value) {
List list = map.get(key);
if (list == null) {
list = new ArrayList<>();
map.put(key, list);
}
list.add(value);
}
public static void mergeMapsOfLists(Map> map, Map> toAdd) {
for (Entry> entry : toAdd.entrySet()) {
for (T listener : entry.getValue()) {
CollectionUtil.addToMapOfLists(map, entry.getKey(), listener);
}
}
}
public static void addToMapOfSets(Map> map, S key, T value) {
Set set = map.get(key);
if (set == null) {
set = new HashSet<>();
map.put(key, set);
}
set.add(value);
}
public static void addCollectionToMapOfSets(Map> map, S key, Collection values) {
Set set = map.get(key);
if (set == null) {
set = new HashSet<>();
map.put(key, set);
}
set.addAll(values);
}
/**
* Chops a list into non-view sublists of length partitionSize. Note: the argument list
* may be included in the result.
*/
public static List> partition(List list, final int partitionSize) {
List> parts = new ArrayList<>();
final int listSize = list.size();
if (listSize <= partitionSize) {
// no need for partitioning
parts.add(list);
} else {
for (int i = 0; i < listSize; i += partitionSize) {
parts.add(new ArrayList<>(list.subList(i, Math.min(listSize, i + partitionSize))));
}
}
return parts;
}
public static List collectInList(Iterator iterator) {
List result = new ArrayList<>();
while (iterator.hasNext()) {
result.add(iterator.next());
}
return result;
}
public static T getLastElement(final Iterable elements) {
T lastElement = null;
if (elements instanceof List) {
return ((List) elements).get(((List) elements).size() - 1);
}
for (T element : elements) {
lastElement = element;
}
return lastElement;
}
public static boolean isEmpty(Collection> collection) {
return collection == null || collection.isEmpty();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy