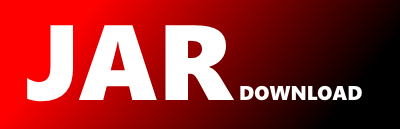
org.camunda.community.rest.client.api.ExecutionApi Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.api;
import com.fasterxml.jackson.core.type.TypeReference;
import org.camunda.community.rest.client.invoker.ApiException;
import org.camunda.community.rest.client.invoker.ApiClient;
import org.camunda.community.rest.client.invoker.Configuration;
import org.camunda.community.rest.client.invoker.Pair;
import org.camunda.community.rest.client.dto.CountResultDto;
import org.camunda.community.rest.client.dto.CreateIncidentDto;
import org.camunda.community.rest.client.dto.EventSubscriptionDto;
import org.camunda.community.rest.client.dto.ExceptionDto;
import org.camunda.community.rest.client.dto.ExecutionDto;
import org.camunda.community.rest.client.dto.ExecutionQueryDto;
import org.camunda.community.rest.client.dto.ExecutionTriggerDto;
import java.io.File;
import org.camunda.community.rest.client.dto.IncidentDto;
import org.camunda.community.rest.client.dto.PatchVariablesDto;
import org.camunda.community.rest.client.dto.VariableValueDto;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class ExecutionApi {
private ApiClient apiClient;
public ExecutionApi() {
this(Configuration.getDefaultApiClient());
}
public ExecutionApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Create Incident
* Creates a custom incident with given properties.
* @param id The id of the execution to create a new incident for. (required)
* @param createIncidentDto (optional)
* @return IncidentDto
* @throws ApiException if fails to make API call
*/
public IncidentDto createIncident(String id, CreateIncidentDto createIncidentDto) throws ApiException {
return this.createIncident(id, createIncidentDto, Collections.emptyMap());
}
/**
* Create Incident
* Creates a custom incident with given properties.
* @param id The id of the execution to create a new incident for. (required)
* @param createIncidentDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @return IncidentDto
* @throws ApiException if fails to make API call
*/
public IncidentDto createIncident(String id, CreateIncidentDto createIncidentDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = createIncidentDto;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling createIncident");
}
// create path and map variables
String localVarPath = "/execution/{id}/create-incident"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Delete Local Execution Variable
* Deletes a variable in the context of a given execution by id. Deletion does not propagate upwards in the execution hierarchy.
* @param id The id of the execution to delete the variable from. (required)
* @param varName The name of the variable to delete. (required)
* @throws ApiException if fails to make API call
*/
public void deleteLocalExecutionVariable(String id, String varName) throws ApiException {
this.deleteLocalExecutionVariable(id, varName, Collections.emptyMap());
}
/**
* Delete Local Execution Variable
* Deletes a variable in the context of a given execution by id. Deletion does not propagate upwards in the execution hierarchy.
* @param id The id of the execution to delete the variable from. (required)
* @param varName The name of the variable to delete. (required)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void deleteLocalExecutionVariable(String id, String varName, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling deleteLocalExecutionVariable");
}
// verify the required parameter 'varName' is set
if (varName == null) {
throw new ApiException(400, "Missing the required parameter 'varName' when calling deleteLocalExecutionVariable");
}
// create path and map variables
String localVarPath = "/execution/{id}/localVariables/{varName}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()))
.replaceAll("\\{" + "varName" + "\\}", apiClient.escapeString(varName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"DELETE",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Get Execution
* Retrieves an execution by id, according to the `Execution` interface in the engine.
* @param id The id of the execution to be retrieved. (required)
* @return ExecutionDto
* @throws ApiException if fails to make API call
*/
public ExecutionDto getExecution(String id) throws ApiException {
return this.getExecution(id, Collections.emptyMap());
}
/**
* Get Execution
* Retrieves an execution by id, according to the `Execution` interface in the engine.
* @param id The id of the execution to be retrieved. (required)
* @param additionalHeaders additionalHeaders for this call
* @return ExecutionDto
* @throws ApiException if fails to make API call
*/
public ExecutionDto getExecution(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getExecution");
}
// create path and map variables
String localVarPath = "/execution/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Executions
* Queries for the executions that fulfill given parameters. Parameters may be static as well as dynamic runtime properties of executions. The size of the result set can be retrieved by using the [Get Execution Count](https://docs.camunda.org/manual/7.21/reference/rest/execution/get-query-count/) method.
* @param businessKey Filter by the business key of the process instances the executions belong to. (optional)
* @param processDefinitionId Filter by the process definition the executions run on. (optional)
* @param processDefinitionKey Filter by the key of the process definition the executions run on. (optional)
* @param processInstanceId Filter by the id of the process instance the execution belongs to. (optional)
* @param activityId Filter by the id of the activity the execution currently executes. (optional)
* @param signalEventSubscriptionName Select only those executions that expect a signal of the given name. (optional)
* @param messageEventSubscriptionName Select only those executions that expect a message of the given name. (optional)
* @param active Only include active executions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param suspended Only include suspended executions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param incidentId Filter by the incident id. (optional)
* @param incidentType Filter by the incident type. See the [User Guide](/manual/develop/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param incidentMessage Filter by the incident message. Exact match. (optional)
* @param incidentMessageLike Filter by the incident message that the parameter is a substring of. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. An execution must have one of the given tenant ids. (optional)
* @param variables Only include executions that have variables with certain values. Variable filtering expressions are comma-separated and are structured as follows: A valid parameter value has the form `key_operator_value`. `key` is the variable name, `operator` is the comparison operator to be used and `value` the variable value. **Note:** Values are always treated as `String` objects on server side. Valid operator values are: `eq` - equal to; `neq` - not equal to; `gt` - greater than; `gteq` - greater than or equal to; `lt` - lower than; `lteq` - lower than or equal to; `like`. `key` and `value` may not contain underscore or comma characters. (optional)
* @param processVariables Only include executions that belong to a process instance with variables with certain values. Variable filtering expressions are comma-separated and are structured as follows: A valid parameter value has the form `key_operator_value`. `key` is the variable name, `operator` is the comparison operator to be used and `value` the variable value. **Note:** Values are always treated as `String` objects on server side. Valid operator values are: `eq` - equal to; `neq` - not equal to. `key` and `value` may not contain underscore or comma characters. (optional)
* @param variableNamesIgnoreCase Match all variable names provided in `variables` and `processVariables` case- insensitively. If set to `true` **variableName** and **variablename** are treated as equal. (optional)
* @param variableValuesIgnoreCase Match all variable values provided in `variables` and `processVariables` case- insensitively. If set to `true` **variableValue** and **variablevalue** are treated as equal. (optional)
* @param sortBy Sort the results lexicographically by a given criterion. Must be used in conjunction with the sortOrder parameter. (optional)
* @param sortOrder Sort the results in a given order. Values may be asc for ascending order or desc for descending order. Must be used in conjunction with the sortBy parameter. (optional)
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @return List<ExecutionDto>
* @throws ApiException if fails to make API call
*/
public List getExecutions(String businessKey, String processDefinitionId, String processDefinitionKey, String processInstanceId, String activityId, String signalEventSubscriptionName, String messageEventSubscriptionName, Boolean active, Boolean suspended, String incidentId, String incidentType, String incidentMessage, String incidentMessageLike, String tenantIdIn, String variables, String processVariables, Boolean variableNamesIgnoreCase, Boolean variableValuesIgnoreCase, String sortBy, String sortOrder, Integer firstResult, Integer maxResults) throws ApiException {
return this.getExecutions(businessKey, processDefinitionId, processDefinitionKey, processInstanceId, activityId, signalEventSubscriptionName, messageEventSubscriptionName, active, suspended, incidentId, incidentType, incidentMessage, incidentMessageLike, tenantIdIn, variables, processVariables, variableNamesIgnoreCase, variableValuesIgnoreCase, sortBy, sortOrder, firstResult, maxResults, Collections.emptyMap());
}
/**
* Get Executions
* Queries for the executions that fulfill given parameters. Parameters may be static as well as dynamic runtime properties of executions. The size of the result set can be retrieved by using the [Get Execution Count](https://docs.camunda.org/manual/7.21/reference/rest/execution/get-query-count/) method.
* @param businessKey Filter by the business key of the process instances the executions belong to. (optional)
* @param processDefinitionId Filter by the process definition the executions run on. (optional)
* @param processDefinitionKey Filter by the key of the process definition the executions run on. (optional)
* @param processInstanceId Filter by the id of the process instance the execution belongs to. (optional)
* @param activityId Filter by the id of the activity the execution currently executes. (optional)
* @param signalEventSubscriptionName Select only those executions that expect a signal of the given name. (optional)
* @param messageEventSubscriptionName Select only those executions that expect a message of the given name. (optional)
* @param active Only include active executions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param suspended Only include suspended executions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param incidentId Filter by the incident id. (optional)
* @param incidentType Filter by the incident type. See the [User Guide](/manual/develop/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param incidentMessage Filter by the incident message. Exact match. (optional)
* @param incidentMessageLike Filter by the incident message that the parameter is a substring of. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. An execution must have one of the given tenant ids. (optional)
* @param variables Only include executions that have variables with certain values. Variable filtering expressions are comma-separated and are structured as follows: A valid parameter value has the form `key_operator_value`. `key` is the variable name, `operator` is the comparison operator to be used and `value` the variable value. **Note:** Values are always treated as `String` objects on server side. Valid operator values are: `eq` - equal to; `neq` - not equal to; `gt` - greater than; `gteq` - greater than or equal to; `lt` - lower than; `lteq` - lower than or equal to; `like`. `key` and `value` may not contain underscore or comma characters. (optional)
* @param processVariables Only include executions that belong to a process instance with variables with certain values. Variable filtering expressions are comma-separated and are structured as follows: A valid parameter value has the form `key_operator_value`. `key` is the variable name, `operator` is the comparison operator to be used and `value` the variable value. **Note:** Values are always treated as `String` objects on server side. Valid operator values are: `eq` - equal to; `neq` - not equal to. `key` and `value` may not contain underscore or comma characters. (optional)
* @param variableNamesIgnoreCase Match all variable names provided in `variables` and `processVariables` case- insensitively. If set to `true` **variableName** and **variablename** are treated as equal. (optional)
* @param variableValuesIgnoreCase Match all variable values provided in `variables` and `processVariables` case- insensitively. If set to `true` **variableValue** and **variablevalue** are treated as equal. (optional)
* @param sortBy Sort the results lexicographically by a given criterion. Must be used in conjunction with the sortOrder parameter. (optional)
* @param sortOrder Sort the results in a given order. Values may be asc for ascending order or desc for descending order. Must be used in conjunction with the sortBy parameter. (optional)
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<ExecutionDto>
* @throws ApiException if fails to make API call
*/
public List getExecutions(String businessKey, String processDefinitionId, String processDefinitionKey, String processInstanceId, String activityId, String signalEventSubscriptionName, String messageEventSubscriptionName, Boolean active, Boolean suspended, String incidentId, String incidentType, String incidentMessage, String incidentMessageLike, String tenantIdIn, String variables, String processVariables, Boolean variableNamesIgnoreCase, Boolean variableValuesIgnoreCase, String sortBy, String sortOrder, Integer firstResult, Integer maxResults, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/execution";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("businessKey", businessKey));
localVarQueryParams.addAll(apiClient.parameterToPair("processDefinitionId", processDefinitionId));
localVarQueryParams.addAll(apiClient.parameterToPair("processDefinitionKey", processDefinitionKey));
localVarQueryParams.addAll(apiClient.parameterToPair("processInstanceId", processInstanceId));
localVarQueryParams.addAll(apiClient.parameterToPair("activityId", activityId));
localVarQueryParams.addAll(apiClient.parameterToPair("signalEventSubscriptionName", signalEventSubscriptionName));
localVarQueryParams.addAll(apiClient.parameterToPair("messageEventSubscriptionName", messageEventSubscriptionName));
localVarQueryParams.addAll(apiClient.parameterToPair("active", active));
localVarQueryParams.addAll(apiClient.parameterToPair("suspended", suspended));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentId", incidentId));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentType", incidentType));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentMessage", incidentMessage));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentMessageLike", incidentMessageLike));
localVarQueryParams.addAll(apiClient.parameterToPair("tenantIdIn", tenantIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("variables", variables));
localVarQueryParams.addAll(apiClient.parameterToPair("processVariables", processVariables));
localVarQueryParams.addAll(apiClient.parameterToPair("variableNamesIgnoreCase", variableNamesIgnoreCase));
localVarQueryParams.addAll(apiClient.parameterToPair("variableValuesIgnoreCase", variableValuesIgnoreCase));
localVarQueryParams.addAll(apiClient.parameterToPair("sortBy", sortBy));
localVarQueryParams.addAll(apiClient.parameterToPair("sortOrder", sortOrder));
localVarQueryParams.addAll(apiClient.parameterToPair("firstResult", firstResult));
localVarQueryParams.addAll(apiClient.parameterToPair("maxResults", maxResults));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Execution Count
* Queries for the number of executions that fulfill given parameters. Takes the same parameters as the [Get Executions](https://docs.camunda.org/manual/7.21/reference/rest/execution/get-query/) method.
* @param businessKey Filter by the business key of the process instances the executions belong to. (optional)
* @param processDefinitionId Filter by the process definition the executions run on. (optional)
* @param processDefinitionKey Filter by the key of the process definition the executions run on. (optional)
* @param processInstanceId Filter by the id of the process instance the execution belongs to. (optional)
* @param activityId Filter by the id of the activity the execution currently executes. (optional)
* @param signalEventSubscriptionName Select only those executions that expect a signal of the given name. (optional)
* @param messageEventSubscriptionName Select only those executions that expect a message of the given name. (optional)
* @param active Only include active executions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param suspended Only include suspended executions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param incidentId Filter by the incident id. (optional)
* @param incidentType Filter by the incident type. See the [User Guide](/manual/develop/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param incidentMessage Filter by the incident message. Exact match. (optional)
* @param incidentMessageLike Filter by the incident message that the parameter is a substring of. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. An execution must have one of the given tenant ids. (optional)
* @param variables Only include executions that have variables with certain values. Variable filtering expressions are comma-separated and are structured as follows: A valid parameter value has the form `key_operator_value`. `key` is the variable name, `operator` is the comparison operator to be used and `value` the variable value. **Note:** Values are always treated as `String` objects on server side. Valid operator values are: `eq` - equal to; `neq` - not equal to; `gt` - greater than; `gteq` - greater than or equal to; `lt` - lower than; `lteq` - lower than or equal to; `like`. `key` and `value` may not contain underscore or comma characters. (optional)
* @param processVariables Only include executions that belong to a process instance with variables with certain values. Variable filtering expressions are comma-separated and are structured as follows: A valid parameter value has the form `key_operator_value`. `key` is the variable name, `operator` is the comparison operator to be used and `value` the variable value. **Note:** Values are always treated as `String` objects on server side. Valid operator values are: `eq` - equal to; `neq` - not equal to. `key` and `value` may not contain underscore or comma characters. (optional)
* @param variableNamesIgnoreCase Match all variable names provided in `variables` and `processVariables` case- insensitively. If set to `true` **variableName** and **variablename** are treated as equal. (optional)
* @param variableValuesIgnoreCase Match all variable values provided in `variables` and `processVariables` case- insensitively. If set to `true` **variableValue** and **variablevalue** are treated as equal. (optional)
* @return CountResultDto
* @throws ApiException if fails to make API call
*/
public CountResultDto getExecutionsCount(String businessKey, String processDefinitionId, String processDefinitionKey, String processInstanceId, String activityId, String signalEventSubscriptionName, String messageEventSubscriptionName, Boolean active, Boolean suspended, String incidentId, String incidentType, String incidentMessage, String incidentMessageLike, String tenantIdIn, String variables, String processVariables, Boolean variableNamesIgnoreCase, Boolean variableValuesIgnoreCase) throws ApiException {
return this.getExecutionsCount(businessKey, processDefinitionId, processDefinitionKey, processInstanceId, activityId, signalEventSubscriptionName, messageEventSubscriptionName, active, suspended, incidentId, incidentType, incidentMessage, incidentMessageLike, tenantIdIn, variables, processVariables, variableNamesIgnoreCase, variableValuesIgnoreCase, Collections.emptyMap());
}
/**
* Get Execution Count
* Queries for the number of executions that fulfill given parameters. Takes the same parameters as the [Get Executions](https://docs.camunda.org/manual/7.21/reference/rest/execution/get-query/) method.
* @param businessKey Filter by the business key of the process instances the executions belong to. (optional)
* @param processDefinitionId Filter by the process definition the executions run on. (optional)
* @param processDefinitionKey Filter by the key of the process definition the executions run on. (optional)
* @param processInstanceId Filter by the id of the process instance the execution belongs to. (optional)
* @param activityId Filter by the id of the activity the execution currently executes. (optional)
* @param signalEventSubscriptionName Select only those executions that expect a signal of the given name. (optional)
* @param messageEventSubscriptionName Select only those executions that expect a message of the given name. (optional)
* @param active Only include active executions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param suspended Only include suspended executions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param incidentId Filter by the incident id. (optional)
* @param incidentType Filter by the incident type. See the [User Guide](/manual/develop/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param incidentMessage Filter by the incident message. Exact match. (optional)
* @param incidentMessageLike Filter by the incident message that the parameter is a substring of. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. An execution must have one of the given tenant ids. (optional)
* @param variables Only include executions that have variables with certain values. Variable filtering expressions are comma-separated and are structured as follows: A valid parameter value has the form `key_operator_value`. `key` is the variable name, `operator` is the comparison operator to be used and `value` the variable value. **Note:** Values are always treated as `String` objects on server side. Valid operator values are: `eq` - equal to; `neq` - not equal to; `gt` - greater than; `gteq` - greater than or equal to; `lt` - lower than; `lteq` - lower than or equal to; `like`. `key` and `value` may not contain underscore or comma characters. (optional)
* @param processVariables Only include executions that belong to a process instance with variables with certain values. Variable filtering expressions are comma-separated and are structured as follows: A valid parameter value has the form `key_operator_value`. `key` is the variable name, `operator` is the comparison operator to be used and `value` the variable value. **Note:** Values are always treated as `String` objects on server side. Valid operator values are: `eq` - equal to; `neq` - not equal to. `key` and `value` may not contain underscore or comma characters. (optional)
* @param variableNamesIgnoreCase Match all variable names provided in `variables` and `processVariables` case- insensitively. If set to `true` **variableName** and **variablename** are treated as equal. (optional)
* @param variableValuesIgnoreCase Match all variable values provided in `variables` and `processVariables` case- insensitively. If set to `true` **variableValue** and **variablevalue** are treated as equal. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return CountResultDto
* @throws ApiException if fails to make API call
*/
public CountResultDto getExecutionsCount(String businessKey, String processDefinitionId, String processDefinitionKey, String processInstanceId, String activityId, String signalEventSubscriptionName, String messageEventSubscriptionName, Boolean active, Boolean suspended, String incidentId, String incidentType, String incidentMessage, String incidentMessageLike, String tenantIdIn, String variables, String processVariables, Boolean variableNamesIgnoreCase, Boolean variableValuesIgnoreCase, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/execution/count";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("businessKey", businessKey));
localVarQueryParams.addAll(apiClient.parameterToPair("processDefinitionId", processDefinitionId));
localVarQueryParams.addAll(apiClient.parameterToPair("processDefinitionKey", processDefinitionKey));
localVarQueryParams.addAll(apiClient.parameterToPair("processInstanceId", processInstanceId));
localVarQueryParams.addAll(apiClient.parameterToPair("activityId", activityId));
localVarQueryParams.addAll(apiClient.parameterToPair("signalEventSubscriptionName", signalEventSubscriptionName));
localVarQueryParams.addAll(apiClient.parameterToPair("messageEventSubscriptionName", messageEventSubscriptionName));
localVarQueryParams.addAll(apiClient.parameterToPair("active", active));
localVarQueryParams.addAll(apiClient.parameterToPair("suspended", suspended));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentId", incidentId));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentType", incidentType));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentMessage", incidentMessage));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentMessageLike", incidentMessageLike));
localVarQueryParams.addAll(apiClient.parameterToPair("tenantIdIn", tenantIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("variables", variables));
localVarQueryParams.addAll(apiClient.parameterToPair("processVariables", processVariables));
localVarQueryParams.addAll(apiClient.parameterToPair("variableNamesIgnoreCase", variableNamesIgnoreCase));
localVarQueryParams.addAll(apiClient.parameterToPair("variableValuesIgnoreCase", variableValuesIgnoreCase));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Local Execution Variable
* Retrieves a variable from the context of a given execution by id. Does not traverse the parent execution hierarchy.
* @param id The id of the execution to retrieve the variable from. (required)
* @param varName The name of the variable to get. (required)
* @param deserializeValue Determines whether serializable variable values (typically variables that store custom Java objects) should be deserialized on server side (default `true`). If set to `true`, a serializable variable will be deserialized on server side and transformed to JSON using [Jackson's](https://github.com/FasterXML/jackson) POJO/bean property introspection feature. Note that this requires the Java classes of the variable value to be on the REST API's classpath. If set to `false`, a serializable variable will be returned in its serialized format. For example, a variable that is serialized as XML will be returned as a JSON string containing XML. **Note:** While `true` is the default value for reasons of backward compatibility, we recommend setting this parameter to `false` when developing web applications that are independent of the Java process applications deployed to the engine. (optional)
* @return VariableValueDto
* @throws ApiException if fails to make API call
*/
public VariableValueDto getLocalExecutionVariable(String id, String varName, Boolean deserializeValue) throws ApiException {
return this.getLocalExecutionVariable(id, varName, deserializeValue, Collections.emptyMap());
}
/**
* Get Local Execution Variable
* Retrieves a variable from the context of a given execution by id. Does not traverse the parent execution hierarchy.
* @param id The id of the execution to retrieve the variable from. (required)
* @param varName The name of the variable to get. (required)
* @param deserializeValue Determines whether serializable variable values (typically variables that store custom Java objects) should be deserialized on server side (default `true`). If set to `true`, a serializable variable will be deserialized on server side and transformed to JSON using [Jackson's](https://github.com/FasterXML/jackson) POJO/bean property introspection feature. Note that this requires the Java classes of the variable value to be on the REST API's classpath. If set to `false`, a serializable variable will be returned in its serialized format. For example, a variable that is serialized as XML will be returned as a JSON string containing XML. **Note:** While `true` is the default value for reasons of backward compatibility, we recommend setting this parameter to `false` when developing web applications that are independent of the Java process applications deployed to the engine. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return VariableValueDto
* @throws ApiException if fails to make API call
*/
public VariableValueDto getLocalExecutionVariable(String id, String varName, Boolean deserializeValue, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getLocalExecutionVariable");
}
// verify the required parameter 'varName' is set
if (varName == null) {
throw new ApiException(400, "Missing the required parameter 'varName' when calling getLocalExecutionVariable");
}
// create path and map variables
String localVarPath = "/execution/{id}/localVariables/{varName}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()))
.replaceAll("\\{" + "varName" + "\\}", apiClient.escapeString(varName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("deserializeValue", deserializeValue));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Local Execution Variable (Binary)
* Retrieves a binary variable from the context of a given execution by id. Does not traverse the parent execution hierarchy. Applicable for byte array and file variables.
* @param id The id of the execution to retrieve the variable from. (required)
* @param varName The name of the variable to get. (required)
* @return File
* @throws ApiException if fails to make API call
*/
public File getLocalExecutionVariableBinary(String id, String varName) throws ApiException {
return this.getLocalExecutionVariableBinary(id, varName, Collections.emptyMap());
}
/**
* Get Local Execution Variable (Binary)
* Retrieves a binary variable from the context of a given execution by id. Does not traverse the parent execution hierarchy. Applicable for byte array and file variables.
* @param id The id of the execution to retrieve the variable from. (required)
* @param varName The name of the variable to get. (required)
* @param additionalHeaders additionalHeaders for this call
* @return File
* @throws ApiException if fails to make API call
*/
public File getLocalExecutionVariableBinary(String id, String varName, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getLocalExecutionVariableBinary");
}
// verify the required parameter 'varName' is set
if (varName == null) {
throw new ApiException(400, "Missing the required parameter 'varName' when calling getLocalExecutionVariableBinary");
}
// create path and map variables
String localVarPath = "/execution/{id}/localVariables/{varName}/data"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()))
.replaceAll("\\{" + "varName" + "\\}", apiClient.escapeString(varName.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/octet-stream", "text/plain", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Local Execution Variables
* Retrieves all variables of a given execution by id.
* @param id The id of the execution to retrieve the variables from. (required)
* @param deserializeValues Determines whether serializable variable values (typically variables that store custom Java objects) should be deserialized on server side (default `true`). If set to `true`, a serializable variable will be deserialized on server side and transformed to JSON using [Jackson's](https://github.com/FasterXML/jackson) POJO/bean property introspection feature. Note that this requires the Java classes of the variable value to be on the REST API's classpath. If set to `false`, a serializable variable will be returned in its serialized format. For example, a variable that is serialized as XML will be returned as a JSON string containing XML. **Note:** While `true` is the default value for reasons of backward compatibility, we recommend setting this parameter to `false` when developing web applications that are independent of the Java process applications deployed to the engine. (optional)
* @return Map<String, VariableValueDto>
* @throws ApiException if fails to make API call
*/
public Map getLocalExecutionVariables(String id, Boolean deserializeValues) throws ApiException {
return this.getLocalExecutionVariables(id, deserializeValues, Collections.emptyMap());
}
/**
* Get Local Execution Variables
* Retrieves all variables of a given execution by id.
* @param id The id of the execution to retrieve the variables from. (required)
* @param deserializeValues Determines whether serializable variable values (typically variables that store custom Java objects) should be deserialized on server side (default `true`). If set to `true`, a serializable variable will be deserialized on server side and transformed to JSON using [Jackson's](https://github.com/FasterXML/jackson) POJO/bean property introspection feature. Note that this requires the Java classes of the variable value to be on the REST API's classpath. If set to `false`, a serializable variable will be returned in its serialized format. For example, a variable that is serialized as XML will be returned as a JSON string containing XML. **Note:** While `true` is the default value for reasons of backward compatibility, we recommend setting this parameter to `false` when developing web applications that are independent of the Java process applications deployed to the engine. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return Map<String, VariableValueDto>
* @throws ApiException if fails to make API call
*/
public Map getLocalExecutionVariables(String id, Boolean deserializeValues, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getLocalExecutionVariables");
}
// create path and map variables
String localVarPath = "/execution/{id}/localVariables"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("deserializeValues", deserializeValues));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy