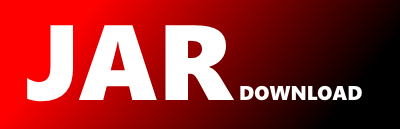
org.camunda.community.rest.client.api.ExternalTaskApi Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.api;
import com.fasterxml.jackson.core.type.TypeReference;
import org.camunda.community.rest.client.invoker.ApiException;
import org.camunda.community.rest.client.invoker.ApiClient;
import org.camunda.community.rest.client.invoker.Configuration;
import org.camunda.community.rest.client.invoker.Pair;
import org.camunda.community.rest.client.dto.BatchDto;
import org.camunda.community.rest.client.dto.CompleteExternalTaskDto;
import org.camunda.community.rest.client.dto.CountResultDto;
import org.camunda.community.rest.client.dto.ExceptionDto;
import org.camunda.community.rest.client.dto.ExtendLockOnExternalTaskDto;
import org.camunda.community.rest.client.dto.ExternalTaskBpmnError;
import org.camunda.community.rest.client.dto.ExternalTaskDto;
import org.camunda.community.rest.client.dto.ExternalTaskFailureDto;
import org.camunda.community.rest.client.dto.ExternalTaskQueryDto;
import org.camunda.community.rest.client.dto.FetchExternalTasksDto;
import org.camunda.community.rest.client.dto.LockExternalTaskDto;
import org.camunda.community.rest.client.dto.LockedExternalTaskDto;
import java.time.OffsetDateTime;
import org.camunda.community.rest.client.dto.PriorityDto;
import org.camunda.community.rest.client.dto.RetriesDto;
import org.camunda.community.rest.client.dto.SetRetriesForExternalTasksDto;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class ExternalTaskApi {
private ApiClient apiClient;
public ExternalTaskApi() {
this(Configuration.getDefaultApiClient());
}
public ExternalTaskApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Complete
* Completes an external task by id and updates process variables.
* @param id The id of the task to complete. (required)
* @param completeExternalTaskDto (optional)
* @throws ApiException if fails to make API call
*/
public void completeExternalTaskResource(String id, CompleteExternalTaskDto completeExternalTaskDto) throws ApiException {
this.completeExternalTaskResource(id, completeExternalTaskDto, Collections.emptyMap());
}
/**
* Complete
* Completes an external task by id and updates process variables.
* @param id The id of the task to complete. (required)
* @param completeExternalTaskDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void completeExternalTaskResource(String id, CompleteExternalTaskDto completeExternalTaskDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = completeExternalTaskDto;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling completeExternalTaskResource");
}
// create path and map variables
String localVarPath = "/external-task/{id}/complete"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Extend Lock
* Extends the timeout of the lock by a given amount of time.
* @param id The id of the external task. (required)
* @param extendLockOnExternalTaskDto (optional)
* @throws ApiException if fails to make API call
*/
public void extendLock(String id, ExtendLockOnExternalTaskDto extendLockOnExternalTaskDto) throws ApiException {
this.extendLock(id, extendLockOnExternalTaskDto, Collections.emptyMap());
}
/**
* Extend Lock
* Extends the timeout of the lock by a given amount of time.
* @param id The id of the external task. (required)
* @param extendLockOnExternalTaskDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void extendLock(String id, ExtendLockOnExternalTaskDto extendLockOnExternalTaskDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = extendLockOnExternalTaskDto;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling extendLock");
}
// create path and map variables
String localVarPath = "/external-task/{id}/extendLock"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Fetch and Lock
* Fetches and locks a specific number of external tasks for execution by a worker. Query can be restricted to specific task topics and for each task topic an individual lock time can be provided.
* @param fetchExternalTasksDto (optional)
* @return List<LockedExternalTaskDto>
* @throws ApiException if fails to make API call
*/
public List fetchAndLock(FetchExternalTasksDto fetchExternalTasksDto) throws ApiException {
return this.fetchAndLock(fetchExternalTasksDto, Collections.emptyMap());
}
/**
* Fetch and Lock
* Fetches and locks a specific number of external tasks for execution by a worker. Query can be restricted to specific task topics and for each task topic an individual lock time can be provided.
* @param fetchExternalTasksDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<LockedExternalTaskDto>
* @throws ApiException if fails to make API call
*/
public List fetchAndLock(FetchExternalTasksDto fetchExternalTasksDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = fetchExternalTasksDto;
// create path and map variables
String localVarPath = "/external-task/fetchAndLock";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get
* Retrieves an external task by id, corresponding to the `ExternalTask` interface in the engine.
* @param id The id of the external task to be retrieved. (required)
* @return ExternalTaskDto
* @throws ApiException if fails to make API call
*/
public ExternalTaskDto getExternalTask(String id) throws ApiException {
return this.getExternalTask(id, Collections.emptyMap());
}
/**
* Get
* Retrieves an external task by id, corresponding to the `ExternalTask` interface in the engine.
* @param id The id of the external task to be retrieved. (required)
* @param additionalHeaders additionalHeaders for this call
* @return ExternalTaskDto
* @throws ApiException if fails to make API call
*/
public ExternalTaskDto getExternalTask(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getExternalTask");
}
// create path and map variables
String localVarPath = "/external-task/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Error Details
* Retrieves the error details in the context of a running external task by id.
* @param id The id of the external task for which the error details should be retrieved. (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String getExternalTaskErrorDetails(String id) throws ApiException {
return this.getExternalTaskErrorDetails(id, Collections.emptyMap());
}
/**
* Get Error Details
* Retrieves the error details in the context of a running external task by id.
* @param id The id of the external task for which the error details should be retrieved. (required)
* @param additionalHeaders additionalHeaders for this call
* @return String
* @throws ApiException if fails to make API call
*/
public String getExternalTaskErrorDetails(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getExternalTaskErrorDetails");
}
// create path and map variables
String localVarPath = "/external-task/{id}/errorDetails"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"text/plain", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get List
* Queries for the external tasks that fulfill given parameters. Parameters may be static as well as dynamic runtime properties of executions. The size of the result set can be retrieved by using the [Get External Task Count](https://docs.camunda.org/manual/7.21/reference/rest/external-task/get-query-count/) method.
* @param externalTaskId Filter by an external task's id. (optional)
* @param externalTaskIdIn Filter by the comma-separated list of external task ids. (optional)
* @param topicName Filter by an external task topic. (optional)
* @param workerId Filter by the id of the worker that the task was most recently locked by. (optional)
* @param locked Only include external tasks that are currently locked (i.e., they have a lock time and it has not expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param notLocked Only include external tasks that are currently not locked (i.e., they have no lock or it has expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param withRetriesLeft Only include external tasks that have a positive (> 0) number of retries (or `null`). Value may only be `true`, as `false` matches any external task. (optional)
* @param noRetriesLeft Only include external tasks that have 0 retries. Value may only be `true`, as `false` matches any external task. (optional)
* @param lockExpirationAfter Restrict to external tasks that have a lock that expires after a given date. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.000+0200`. (optional)
* @param lockExpirationBefore Restrict to external tasks that have a lock that expires before a given date. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.000+0200`. (optional)
* @param activityId Filter by the id of the activity that an external task is created for. (optional)
* @param activityIdIn Filter by the comma-separated list of ids of the activities that an external task is created for. (optional)
* @param executionId Filter by the id of the execution that an external task belongs to. (optional)
* @param processInstanceId Filter by the id of the process instance that an external task belongs to. (optional)
* @param processInstanceIdIn Filter by a comma-separated list of process instance ids that an external task may belong to. (optional)
* @param processDefinitionId Filter by the id of the process definition that an external task belongs to. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. An external task must have one of the given tenant ids. (optional)
* @param active Only include active tasks. Value may only be `true`, as `false` matches any external task. (optional)
* @param suspended Only include suspended tasks. Value may only be `true`, as `false` matches any external task. (optional)
* @param priorityHigherThanOrEquals Only include jobs with a priority higher than or equal to the given value. Value must be a valid `long` value. (optional)
* @param priorityLowerThanOrEquals Only include jobs with a priority lower than or equal to the given value. Value must be a valid `long` value. (optional)
* @param sortBy Sort the results lexicographically by a given criterion. Must be used in conjunction with the sortOrder parameter. (optional)
* @param sortOrder Sort the results in a given order. Values may be asc for ascending order or desc for descending order. Must be used in conjunction with the sortBy parameter. (optional)
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @return List<ExternalTaskDto>
* @throws ApiException if fails to make API call
*/
public List getExternalTasks(String externalTaskId, String externalTaskIdIn, String topicName, String workerId, Boolean locked, Boolean notLocked, Boolean withRetriesLeft, Boolean noRetriesLeft, OffsetDateTime lockExpirationAfter, OffsetDateTime lockExpirationBefore, String activityId, String activityIdIn, String executionId, String processInstanceId, String processInstanceIdIn, String processDefinitionId, String tenantIdIn, Boolean active, Boolean suspended, Long priorityHigherThanOrEquals, Long priorityLowerThanOrEquals, String sortBy, String sortOrder, Integer firstResult, Integer maxResults) throws ApiException {
return this.getExternalTasks(externalTaskId, externalTaskIdIn, topicName, workerId, locked, notLocked, withRetriesLeft, noRetriesLeft, lockExpirationAfter, lockExpirationBefore, activityId, activityIdIn, executionId, processInstanceId, processInstanceIdIn, processDefinitionId, tenantIdIn, active, suspended, priorityHigherThanOrEquals, priorityLowerThanOrEquals, sortBy, sortOrder, firstResult, maxResults, Collections.emptyMap());
}
/**
* Get List
* Queries for the external tasks that fulfill given parameters. Parameters may be static as well as dynamic runtime properties of executions. The size of the result set can be retrieved by using the [Get External Task Count](https://docs.camunda.org/manual/7.21/reference/rest/external-task/get-query-count/) method.
* @param externalTaskId Filter by an external task's id. (optional)
* @param externalTaskIdIn Filter by the comma-separated list of external task ids. (optional)
* @param topicName Filter by an external task topic. (optional)
* @param workerId Filter by the id of the worker that the task was most recently locked by. (optional)
* @param locked Only include external tasks that are currently locked (i.e., they have a lock time and it has not expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param notLocked Only include external tasks that are currently not locked (i.e., they have no lock or it has expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param withRetriesLeft Only include external tasks that have a positive (> 0) number of retries (or `null`). Value may only be `true`, as `false` matches any external task. (optional)
* @param noRetriesLeft Only include external tasks that have 0 retries. Value may only be `true`, as `false` matches any external task. (optional)
* @param lockExpirationAfter Restrict to external tasks that have a lock that expires after a given date. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.000+0200`. (optional)
* @param lockExpirationBefore Restrict to external tasks that have a lock that expires before a given date. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.000+0200`. (optional)
* @param activityId Filter by the id of the activity that an external task is created for. (optional)
* @param activityIdIn Filter by the comma-separated list of ids of the activities that an external task is created for. (optional)
* @param executionId Filter by the id of the execution that an external task belongs to. (optional)
* @param processInstanceId Filter by the id of the process instance that an external task belongs to. (optional)
* @param processInstanceIdIn Filter by a comma-separated list of process instance ids that an external task may belong to. (optional)
* @param processDefinitionId Filter by the id of the process definition that an external task belongs to. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. An external task must have one of the given tenant ids. (optional)
* @param active Only include active tasks. Value may only be `true`, as `false` matches any external task. (optional)
* @param suspended Only include suspended tasks. Value may only be `true`, as `false` matches any external task. (optional)
* @param priorityHigherThanOrEquals Only include jobs with a priority higher than or equal to the given value. Value must be a valid `long` value. (optional)
* @param priorityLowerThanOrEquals Only include jobs with a priority lower than or equal to the given value. Value must be a valid `long` value. (optional)
* @param sortBy Sort the results lexicographically by a given criterion. Must be used in conjunction with the sortOrder parameter. (optional)
* @param sortOrder Sort the results in a given order. Values may be asc for ascending order or desc for descending order. Must be used in conjunction with the sortBy parameter. (optional)
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<ExternalTaskDto>
* @throws ApiException if fails to make API call
*/
public List getExternalTasks(String externalTaskId, String externalTaskIdIn, String topicName, String workerId, Boolean locked, Boolean notLocked, Boolean withRetriesLeft, Boolean noRetriesLeft, OffsetDateTime lockExpirationAfter, OffsetDateTime lockExpirationBefore, String activityId, String activityIdIn, String executionId, String processInstanceId, String processInstanceIdIn, String processDefinitionId, String tenantIdIn, Boolean active, Boolean suspended, Long priorityHigherThanOrEquals, Long priorityLowerThanOrEquals, String sortBy, String sortOrder, Integer firstResult, Integer maxResults, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/external-task";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("externalTaskId", externalTaskId));
localVarQueryParams.addAll(apiClient.parameterToPair("externalTaskIdIn", externalTaskIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("topicName", topicName));
localVarQueryParams.addAll(apiClient.parameterToPair("workerId", workerId));
localVarQueryParams.addAll(apiClient.parameterToPair("locked", locked));
localVarQueryParams.addAll(apiClient.parameterToPair("notLocked", notLocked));
localVarQueryParams.addAll(apiClient.parameterToPair("withRetriesLeft", withRetriesLeft));
localVarQueryParams.addAll(apiClient.parameterToPair("noRetriesLeft", noRetriesLeft));
localVarQueryParams.addAll(apiClient.parameterToPair("lockExpirationAfter", lockExpirationAfter));
localVarQueryParams.addAll(apiClient.parameterToPair("lockExpirationBefore", lockExpirationBefore));
localVarQueryParams.addAll(apiClient.parameterToPair("activityId", activityId));
localVarQueryParams.addAll(apiClient.parameterToPair("activityIdIn", activityIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("executionId", executionId));
localVarQueryParams.addAll(apiClient.parameterToPair("processInstanceId", processInstanceId));
localVarQueryParams.addAll(apiClient.parameterToPair("processInstanceIdIn", processInstanceIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("processDefinitionId", processDefinitionId));
localVarQueryParams.addAll(apiClient.parameterToPair("tenantIdIn", tenantIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("active", active));
localVarQueryParams.addAll(apiClient.parameterToPair("suspended", suspended));
localVarQueryParams.addAll(apiClient.parameterToPair("priorityHigherThanOrEquals", priorityHigherThanOrEquals));
localVarQueryParams.addAll(apiClient.parameterToPair("priorityLowerThanOrEquals", priorityLowerThanOrEquals));
localVarQueryParams.addAll(apiClient.parameterToPair("sortBy", sortBy));
localVarQueryParams.addAll(apiClient.parameterToPair("sortOrder", sortOrder));
localVarQueryParams.addAll(apiClient.parameterToPair("firstResult", firstResult));
localVarQueryParams.addAll(apiClient.parameterToPair("maxResults", maxResults));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get List Count
* Queries for the number of external tasks that fulfill given parameters. Takes the same parameters as the [Get External Tasks](https://docs.camunda.org/manual/7.21/reference/rest/external-task/get-query/) method.
* @param externalTaskId Filter by an external task's id. (optional)
* @param externalTaskIdIn Filter by the comma-separated list of external task ids. (optional)
* @param topicName Filter by an external task topic. (optional)
* @param workerId Filter by the id of the worker that the task was most recently locked by. (optional)
* @param locked Only include external tasks that are currently locked (i.e., they have a lock time and it has not expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param notLocked Only include external tasks that are currently not locked (i.e., they have no lock or it has expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param withRetriesLeft Only include external tasks that have a positive (> 0) number of retries (or `null`). Value may only be `true`, as `false` matches any external task. (optional)
* @param noRetriesLeft Only include external tasks that have 0 retries. Value may only be `true`, as `false` matches any external task. (optional)
* @param lockExpirationAfter Restrict to external tasks that have a lock that expires after a given date. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.000+0200`. (optional)
* @param lockExpirationBefore Restrict to external tasks that have a lock that expires before a given date. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.000+0200`. (optional)
* @param activityId Filter by the id of the activity that an external task is created for. (optional)
* @param activityIdIn Filter by the comma-separated list of ids of the activities that an external task is created for. (optional)
* @param executionId Filter by the id of the execution that an external task belongs to. (optional)
* @param processInstanceId Filter by the id of the process instance that an external task belongs to. (optional)
* @param processInstanceIdIn Filter by a comma-separated list of process instance ids that an external task may belong to. (optional)
* @param processDefinitionId Filter by the id of the process definition that an external task belongs to. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. An external task must have one of the given tenant ids. (optional)
* @param active Only include active tasks. Value may only be `true`, as `false` matches any external task. (optional)
* @param suspended Only include suspended tasks. Value may only be `true`, as `false` matches any external task. (optional)
* @param priorityHigherThanOrEquals Only include jobs with a priority higher than or equal to the given value. Value must be a valid `long` value. (optional)
* @param priorityLowerThanOrEquals Only include jobs with a priority lower than or equal to the given value. Value must be a valid `long` value. (optional)
* @return CountResultDto
* @throws ApiException if fails to make API call
*/
public CountResultDto getExternalTasksCount(String externalTaskId, String externalTaskIdIn, String topicName, String workerId, Boolean locked, Boolean notLocked, Boolean withRetriesLeft, Boolean noRetriesLeft, OffsetDateTime lockExpirationAfter, OffsetDateTime lockExpirationBefore, String activityId, String activityIdIn, String executionId, String processInstanceId, String processInstanceIdIn, String processDefinitionId, String tenantIdIn, Boolean active, Boolean suspended, Long priorityHigherThanOrEquals, Long priorityLowerThanOrEquals) throws ApiException {
return this.getExternalTasksCount(externalTaskId, externalTaskIdIn, topicName, workerId, locked, notLocked, withRetriesLeft, noRetriesLeft, lockExpirationAfter, lockExpirationBefore, activityId, activityIdIn, executionId, processInstanceId, processInstanceIdIn, processDefinitionId, tenantIdIn, active, suspended, priorityHigherThanOrEquals, priorityLowerThanOrEquals, Collections.emptyMap());
}
/**
* Get List Count
* Queries for the number of external tasks that fulfill given parameters. Takes the same parameters as the [Get External Tasks](https://docs.camunda.org/manual/7.21/reference/rest/external-task/get-query/) method.
* @param externalTaskId Filter by an external task's id. (optional)
* @param externalTaskIdIn Filter by the comma-separated list of external task ids. (optional)
* @param topicName Filter by an external task topic. (optional)
* @param workerId Filter by the id of the worker that the task was most recently locked by. (optional)
* @param locked Only include external tasks that are currently locked (i.e., they have a lock time and it has not expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param notLocked Only include external tasks that are currently not locked (i.e., they have no lock or it has expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param withRetriesLeft Only include external tasks that have a positive (> 0) number of retries (or `null`). Value may only be `true`, as `false` matches any external task. (optional)
* @param noRetriesLeft Only include external tasks that have 0 retries. Value may only be `true`, as `false` matches any external task. (optional)
* @param lockExpirationAfter Restrict to external tasks that have a lock that expires after a given date. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.000+0200`. (optional)
* @param lockExpirationBefore Restrict to external tasks that have a lock that expires before a given date. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.000+0200`. (optional)
* @param activityId Filter by the id of the activity that an external task is created for. (optional)
* @param activityIdIn Filter by the comma-separated list of ids of the activities that an external task is created for. (optional)
* @param executionId Filter by the id of the execution that an external task belongs to. (optional)
* @param processInstanceId Filter by the id of the process instance that an external task belongs to. (optional)
* @param processInstanceIdIn Filter by a comma-separated list of process instance ids that an external task may belong to. (optional)
* @param processDefinitionId Filter by the id of the process definition that an external task belongs to. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. An external task must have one of the given tenant ids. (optional)
* @param active Only include active tasks. Value may only be `true`, as `false` matches any external task. (optional)
* @param suspended Only include suspended tasks. Value may only be `true`, as `false` matches any external task. (optional)
* @param priorityHigherThanOrEquals Only include jobs with a priority higher than or equal to the given value. Value must be a valid `long` value. (optional)
* @param priorityLowerThanOrEquals Only include jobs with a priority lower than or equal to the given value. Value must be a valid `long` value. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return CountResultDto
* @throws ApiException if fails to make API call
*/
public CountResultDto getExternalTasksCount(String externalTaskId, String externalTaskIdIn, String topicName, String workerId, Boolean locked, Boolean notLocked, Boolean withRetriesLeft, Boolean noRetriesLeft, OffsetDateTime lockExpirationAfter, OffsetDateTime lockExpirationBefore, String activityId, String activityIdIn, String executionId, String processInstanceId, String processInstanceIdIn, String processDefinitionId, String tenantIdIn, Boolean active, Boolean suspended, Long priorityHigherThanOrEquals, Long priorityLowerThanOrEquals, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/external-task/count";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("externalTaskId", externalTaskId));
localVarQueryParams.addAll(apiClient.parameterToPair("externalTaskIdIn", externalTaskIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("topicName", topicName));
localVarQueryParams.addAll(apiClient.parameterToPair("workerId", workerId));
localVarQueryParams.addAll(apiClient.parameterToPair("locked", locked));
localVarQueryParams.addAll(apiClient.parameterToPair("notLocked", notLocked));
localVarQueryParams.addAll(apiClient.parameterToPair("withRetriesLeft", withRetriesLeft));
localVarQueryParams.addAll(apiClient.parameterToPair("noRetriesLeft", noRetriesLeft));
localVarQueryParams.addAll(apiClient.parameterToPair("lockExpirationAfter", lockExpirationAfter));
localVarQueryParams.addAll(apiClient.parameterToPair("lockExpirationBefore", lockExpirationBefore));
localVarQueryParams.addAll(apiClient.parameterToPair("activityId", activityId));
localVarQueryParams.addAll(apiClient.parameterToPair("activityIdIn", activityIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("executionId", executionId));
localVarQueryParams.addAll(apiClient.parameterToPair("processInstanceId", processInstanceId));
localVarQueryParams.addAll(apiClient.parameterToPair("processInstanceIdIn", processInstanceIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("processDefinitionId", processDefinitionId));
localVarQueryParams.addAll(apiClient.parameterToPair("tenantIdIn", tenantIdIn));
localVarQueryParams.addAll(apiClient.parameterToPair("active", active));
localVarQueryParams.addAll(apiClient.parameterToPair("suspended", suspended));
localVarQueryParams.addAll(apiClient.parameterToPair("priorityHigherThanOrEquals", priorityHigherThanOrEquals));
localVarQueryParams.addAll(apiClient.parameterToPair("priorityLowerThanOrEquals", priorityLowerThanOrEquals));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get External Task Topic Names
* Queries for distinct topic names of external tasks that fulfill given parameters. Query can be restricted to only tasks with retries left, tasks that are locked, or tasks that are unlocked. The parameters withLockedTasks and withUnlockedTasks are exclusive. Setting them both to true will return an empty list. Providing no parameters will return a list of all distinct topic names with external tasks.
* @param withLockedTasks Only include external tasks that are currently locked (i.e., they have a lock time and it has not expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param withUnlockedTasks Only include external tasks that are currently not locked (i.e., they have no lock or it has expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param withRetriesLeft Only include external tasks that have a positive (> 0) number of retries (or `null`). Value may only be `true`, as `false` matches any external task. (optional)
* @return List<String>
* @throws ApiException if fails to make API call
*/
public List getTopicNames(Boolean withLockedTasks, Boolean withUnlockedTasks, Boolean withRetriesLeft) throws ApiException {
return this.getTopicNames(withLockedTasks, withUnlockedTasks, withRetriesLeft, Collections.emptyMap());
}
/**
* Get External Task Topic Names
* Queries for distinct topic names of external tasks that fulfill given parameters. Query can be restricted to only tasks with retries left, tasks that are locked, or tasks that are unlocked. The parameters withLockedTasks and withUnlockedTasks are exclusive. Setting them both to true will return an empty list. Providing no parameters will return a list of all distinct topic names with external tasks.
* @param withLockedTasks Only include external tasks that are currently locked (i.e., they have a lock time and it has not expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param withUnlockedTasks Only include external tasks that are currently not locked (i.e., they have no lock or it has expired). Value may only be `true`, as `false` matches any external task. (optional)
* @param withRetriesLeft Only include external tasks that have a positive (> 0) number of retries (or `null`). Value may only be `true`, as `false` matches any external task. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<String>
* @throws ApiException if fails to make API call
*/
public List getTopicNames(Boolean withLockedTasks, Boolean withUnlockedTasks, Boolean withRetriesLeft, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/external-task/topic-names";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("withLockedTasks", withLockedTasks));
localVarQueryParams.addAll(apiClient.parameterToPair("withUnlockedTasks", withUnlockedTasks));
localVarQueryParams.addAll(apiClient.parameterToPair("withRetriesLeft", withRetriesLeft));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Handle BPMN Error
* Reports a business error in the context of a running external task by id. The error code must be specified to identify the BPMN error handler.
* @param id The id of the external task in which context a BPMN error is reported. (required)
* @param externalTaskBpmnError (optional)
* @throws ApiException if fails to make API call
*/
public void handleExternalTaskBpmnError(String id, ExternalTaskBpmnError externalTaskBpmnError) throws ApiException {
this.handleExternalTaskBpmnError(id, externalTaskBpmnError, Collections.emptyMap());
}
/**
* Handle BPMN Error
* Reports a business error in the context of a running external task by id. The error code must be specified to identify the BPMN error handler.
* @param id The id of the external task in which context a BPMN error is reported. (required)
* @param externalTaskBpmnError (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void handleExternalTaskBpmnError(String id, ExternalTaskBpmnError externalTaskBpmnError, Map additionalHeaders) throws ApiException {
Object localVarPostBody = externalTaskBpmnError;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling handleExternalTaskBpmnError");
}
// create path and map variables
String localVarPath = "/external-task/{id}/bpmnError"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Handle Failure
* Reports a failure to execute an external task by id. A number of retries and a timeout until the task can be retried can be specified. If retries are set to 0, an incident for this task is created.
* @param id The id of the external task to report a failure for. (required)
* @param externalTaskFailureDto (optional)
* @throws ApiException if fails to make API call
*/
public void handleFailure(String id, ExternalTaskFailureDto externalTaskFailureDto) throws ApiException {
this.handleFailure(id, externalTaskFailureDto, Collections.emptyMap());
}
/**
* Handle Failure
* Reports a failure to execute an external task by id. A number of retries and a timeout until the task can be retried can be specified. If retries are set to 0, an incident for this task is created.
* @param id The id of the external task to report a failure for. (required)
* @param externalTaskFailureDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void handleFailure(String id, ExternalTaskFailureDto externalTaskFailureDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = externalTaskFailureDto;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling handleFailure");
}
// create path and map variables
String localVarPath = "/external-task/{id}/failure"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
*
* Lock an external task by a given id for a specified worker and amount of time.
* @param id The id of the external task. (required)
* @param lockExternalTaskDto (optional)
* @throws ApiException if fails to make API call
*/
public void lock(String id, LockExternalTaskDto lockExternalTaskDto) throws ApiException {
this.lock(id, lockExternalTaskDto, Collections.emptyMap());
}
/**
*
* Lock an external task by a given id for a specified worker and amount of time.
* @param id The id of the external task. (required)
* @param lockExternalTaskDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void lock(String id, LockExternalTaskDto lockExternalTaskDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = lockExternalTaskDto;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling lock");
}
// create path and map variables
String localVarPath = "/external-task/{id}/lock"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Get List (POST)
* Queries for external tasks that fulfill given parameters in the form of a JSON object. This method is slightly more powerful than the [Get External Tasks](https://docs.camunda.org/manual/7.21/reference/rest/external-task/get-query/) method because it allows to specify a hierarchical result sorting.
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @param externalTaskQueryDto (optional)
* @return List<ExternalTaskDto>
* @throws ApiException if fails to make API call
*/
public List queryExternalTasks(Integer firstResult, Integer maxResults, ExternalTaskQueryDto externalTaskQueryDto) throws ApiException {
return this.queryExternalTasks(firstResult, maxResults, externalTaskQueryDto, Collections.emptyMap());
}
/**
* Get List (POST)
* Queries for external tasks that fulfill given parameters in the form of a JSON object. This method is slightly more powerful than the [Get External Tasks](https://docs.camunda.org/manual/7.21/reference/rest/external-task/get-query/) method because it allows to specify a hierarchical result sorting.
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @param externalTaskQueryDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<ExternalTaskDto>
* @throws ApiException if fails to make API call
*/
public List queryExternalTasks(Integer firstResult, Integer maxResults, ExternalTaskQueryDto externalTaskQueryDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = externalTaskQueryDto;
// create path and map variables
String localVarPath = "/external-task";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("firstResult", firstResult));
localVarQueryParams.addAll(apiClient.parameterToPair("maxResults", maxResults));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get List Count (POST)
* Queries for the number of external tasks that fulfill given parameters. This method takes the same message body as the [Get External Tasks (POST)](https://docs.camunda.org/manual/7.21/reference/rest/external-task/post-query/) method.
* @param externalTaskQueryDto (optional)
* @return CountResultDto
* @throws ApiException if fails to make API call
*/
public CountResultDto queryExternalTasksCount(ExternalTaskQueryDto externalTaskQueryDto) throws ApiException {
return this.queryExternalTasksCount(externalTaskQueryDto, Collections.emptyMap());
}
/**
* Get List Count (POST)
* Queries for the number of external tasks that fulfill given parameters. This method takes the same message body as the [Get External Tasks (POST)](https://docs.camunda.org/manual/7.21/reference/rest/external-task/post-query/) method.
* @param externalTaskQueryDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @return CountResultDto
* @throws ApiException if fails to make API call
*/
public CountResultDto queryExternalTasksCount(ExternalTaskQueryDto externalTaskQueryDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = externalTaskQueryDto;
// create path and map variables
String localVarPath = "/external-task/count";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Set Priority
* Sets the priority of an existing external task by id. The default value of a priority is 0.
* @param id The id of the external task to set the priority for. (required)
* @param priorityDto (optional)
* @throws ApiException if fails to make API call
*/
public void setExternalTaskResourcePriority(String id, PriorityDto priorityDto) throws ApiException {
this.setExternalTaskResourcePriority(id, priorityDto, Collections.emptyMap());
}
/**
* Set Priority
* Sets the priority of an existing external task by id. The default value of a priority is 0.
* @param id The id of the external task to set the priority for. (required)
* @param priorityDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void setExternalTaskResourcePriority(String id, PriorityDto priorityDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = priorityDto;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling setExternalTaskResourcePriority");
}
// create path and map variables
String localVarPath = "/external-task/{id}/priority"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"PUT",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Set Retries
* Sets the number of retries left to execute an external task by id. If retries are set to 0, an incident is created.
* @param id The id of the external task to set the number of retries for. (required)
* @param retriesDto (optional)
* @throws ApiException if fails to make API call
*/
public void setExternalTaskResourceRetries(String id, RetriesDto retriesDto) throws ApiException {
this.setExternalTaskResourceRetries(id, retriesDto, Collections.emptyMap());
}
/**
* Set Retries
* Sets the number of retries left to execute an external task by id. If retries are set to 0, an incident is created.
* @param id The id of the external task to set the number of retries for. (required)
* @param retriesDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void setExternalTaskResourceRetries(String id, RetriesDto retriesDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = retriesDto;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling setExternalTaskResourceRetries");
}
// create path and map variables
String localVarPath = "/external-task/{id}/retries"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"PUT",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Set Retries Sync
* Sets the number of retries left to execute external tasks by id synchronously. If retries are set to 0, an incident is created.
* @param setRetriesForExternalTasksDto (optional)
* @throws ApiException if fails to make API call
*/
public void setExternalTaskRetries(SetRetriesForExternalTasksDto setRetriesForExternalTasksDto) throws ApiException {
this.setExternalTaskRetries(setRetriesForExternalTasksDto, Collections.emptyMap());
}
/**
* Set Retries Sync
* Sets the number of retries left to execute external tasks by id synchronously. If retries are set to 0, an incident is created.
* @param setRetriesForExternalTasksDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void setExternalTaskRetries(SetRetriesForExternalTasksDto setRetriesForExternalTasksDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = setRetriesForExternalTasksDto;
// create path and map variables
String localVarPath = "/external-task/retries";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"PUT",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Set Retries Async
* Sets the number of retries left to execute external tasks by id asynchronously. If retries are set to 0, an incident is created.
* @param setRetriesForExternalTasksDto (optional)
* @return BatchDto
* @throws ApiException if fails to make API call
*/
public BatchDto setExternalTaskRetriesAsyncOperation(SetRetriesForExternalTasksDto setRetriesForExternalTasksDto) throws ApiException {
return this.setExternalTaskRetriesAsyncOperation(setRetriesForExternalTasksDto, Collections.emptyMap());
}
/**
* Set Retries Async
* Sets the number of retries left to execute external tasks by id asynchronously. If retries are set to 0, an incident is created.
* @param setRetriesForExternalTasksDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @return BatchDto
* @throws ApiException if fails to make API call
*/
public BatchDto setExternalTaskRetriesAsyncOperation(SetRetriesForExternalTasksDto setRetriesForExternalTasksDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = setRetriesForExternalTasksDto;
// create path and map variables
String localVarPath = "/external-task/retries-async";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Unlock
* Unlocks an external task by id. Clears the task's lock expiration time and worker id.
* @param id The id of the external task to unlock. (required)
* @throws ApiException if fails to make API call
*/
public void unlock(String id) throws ApiException {
this.unlock(id, Collections.emptyMap());
}
/**
* Unlock
* Unlocks an external task by id. Clears the task's lock expiration time and worker id.
* @param id The id of the external task to unlock. (required)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void unlock(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling unlock");
}
// create path and map variables
String localVarPath = "/external-task/{id}/unlock"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy