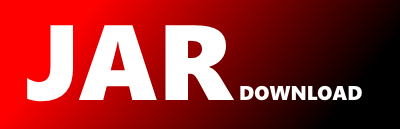
org.camunda.community.rest.client.api.ProcessDefinitionApi Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.api;
import com.fasterxml.jackson.core.type.TypeReference;
import org.camunda.community.rest.client.invoker.ApiException;
import org.camunda.community.rest.client.invoker.ApiClient;
import org.camunda.community.rest.client.invoker.Configuration;
import org.camunda.community.rest.client.invoker.Pair;
import org.camunda.community.rest.client.dto.ActivityStatisticsResultDto;
import org.camunda.community.rest.client.dto.AuthorizationExceptionDto;
import org.camunda.community.rest.client.dto.BatchDto;
import org.camunda.community.rest.client.dto.CalledProcessDefinitionDto;
import org.camunda.community.rest.client.dto.CountResultDto;
import org.camunda.community.rest.client.dto.ExceptionDto;
import java.io.File;
import org.camunda.community.rest.client.dto.FormDto;
import org.camunda.community.rest.client.dto.HistoryTimeToLiveDto;
import java.time.OffsetDateTime;
import org.camunda.community.rest.client.dto.ProcessDefinitionDiagramDto;
import org.camunda.community.rest.client.dto.ProcessDefinitionDto;
import org.camunda.community.rest.client.dto.ProcessDefinitionStatisticsResultDto;
import org.camunda.community.rest.client.dto.ProcessDefinitionSuspensionStateDto;
import org.camunda.community.rest.client.dto.ProcessInstanceDto;
import org.camunda.community.rest.client.dto.ProcessInstanceWithVariablesDto;
import org.camunda.community.rest.client.dto.RestartProcessInstanceDto;
import org.camunda.community.rest.client.dto.StartProcessInstanceDto;
import org.camunda.community.rest.client.dto.StartProcessInstanceFormDto;
import org.camunda.community.rest.client.dto.VariableValueDto;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class ProcessDefinitionApi {
private ApiClient apiClient;
public ProcessDefinitionApi() {
this(Configuration.getDefaultApiClient());
}
public ProcessDefinitionApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Delete
* Deletes a running process instance by id.
* @param id The id of the process definition to be deleted. (required)
* @param cascade `true`, if all process instances, historic process instances and jobs for this process definition should be deleted. (optional)
* @param skipCustomListeners `true`, if only the built-in ExecutionListeners should be notified with the end event. (optional, default to false)
* @param skipIoMappings A boolean value to control whether input/output mappings should be executed during deletion. `true`, if input/output mappings should not be invoked. (optional, default to false)
* @throws ApiException if fails to make API call
*/
public void deleteProcessDefinition(String id, Boolean cascade, Boolean skipCustomListeners, Boolean skipIoMappings) throws ApiException {
this.deleteProcessDefinition(id, cascade, skipCustomListeners, skipIoMappings, Collections.emptyMap());
}
/**
* Delete
* Deletes a running process instance by id.
* @param id The id of the process definition to be deleted. (required)
* @param cascade `true`, if all process instances, historic process instances and jobs for this process definition should be deleted. (optional)
* @param skipCustomListeners `true`, if only the built-in ExecutionListeners should be notified with the end event. (optional, default to false)
* @param skipIoMappings A boolean value to control whether input/output mappings should be executed during deletion. `true`, if input/output mappings should not be invoked. (optional, default to false)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void deleteProcessDefinition(String id, Boolean cascade, Boolean skipCustomListeners, Boolean skipIoMappings, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling deleteProcessDefinition");
}
// create path and map variables
String localVarPath = "/process-definition/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("cascade", cascade));
localVarQueryParams.addAll(apiClient.parameterToPair("skipCustomListeners", skipCustomListeners));
localVarQueryParams.addAll(apiClient.parameterToPair("skipIoMappings", skipIoMappings));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"DELETE",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Delete By Key
* Deletes process definitions by a given key which belong to no tenant id.
* @param key The key of the process definitions to be deleted. (required)
* @param cascade `true`, if all process instances, historic process instances and jobs for this process definition should be deleted. (optional)
* @param skipCustomListeners `true`, if only the built-in ExecutionListeners should be notified with the end event. (optional, default to false)
* @param skipIoMappings A boolean value to control whether input/output mappings should be executed during deletion. `true`, if input/output mappings should not be invoked. (optional, default to false)
* @throws ApiException if fails to make API call
*/
public void deleteProcessDefinitionsByKey(String key, Boolean cascade, Boolean skipCustomListeners, Boolean skipIoMappings) throws ApiException {
this.deleteProcessDefinitionsByKey(key, cascade, skipCustomListeners, skipIoMappings, Collections.emptyMap());
}
/**
* Delete By Key
* Deletes process definitions by a given key which belong to no tenant id.
* @param key The key of the process definitions to be deleted. (required)
* @param cascade `true`, if all process instances, historic process instances and jobs for this process definition should be deleted. (optional)
* @param skipCustomListeners `true`, if only the built-in ExecutionListeners should be notified with the end event. (optional, default to false)
* @param skipIoMappings A boolean value to control whether input/output mappings should be executed during deletion. `true`, if input/output mappings should not be invoked. (optional, default to false)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void deleteProcessDefinitionsByKey(String key, Boolean cascade, Boolean skipCustomListeners, Boolean skipIoMappings, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling deleteProcessDefinitionsByKey");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("cascade", cascade));
localVarQueryParams.addAll(apiClient.parameterToPair("skipCustomListeners", skipCustomListeners));
localVarQueryParams.addAll(apiClient.parameterToPair("skipIoMappings", skipIoMappings));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"DELETE",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Delete By Key
* Deletes process definitions by a given key and which belong to a tenant id.
* @param key The key of the process definitions to be deleted. (required)
* @param tenantId The id of the tenant the process definitions belong to. (required)
* @param cascade `true`, if all process instances, historic process instances and jobs for this process definition should be deleted. (optional)
* @param skipCustomListeners `true`, if only the built-in ExecutionListeners should be notified with the end event. (optional, default to false)
* @param skipIoMappings A boolean value to control whether input/output mappings should be executed during deletion. `true`, if input/output mappings should not be invoked. (optional, default to false)
* @throws ApiException if fails to make API call
*/
public void deleteProcessDefinitionsByKeyAndTenantId(String key, String tenantId, Boolean cascade, Boolean skipCustomListeners, Boolean skipIoMappings) throws ApiException {
this.deleteProcessDefinitionsByKeyAndTenantId(key, tenantId, cascade, skipCustomListeners, skipIoMappings, Collections.emptyMap());
}
/**
* Delete By Key
* Deletes process definitions by a given key and which belong to a tenant id.
* @param key The key of the process definitions to be deleted. (required)
* @param tenantId The id of the tenant the process definitions belong to. (required)
* @param cascade `true`, if all process instances, historic process instances and jobs for this process definition should be deleted. (optional)
* @param skipCustomListeners `true`, if only the built-in ExecutionListeners should be notified with the end event. (optional, default to false)
* @param skipIoMappings A boolean value to control whether input/output mappings should be executed during deletion. `true`, if input/output mappings should not be invoked. (optional, default to false)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void deleteProcessDefinitionsByKeyAndTenantId(String key, String tenantId, Boolean cascade, Boolean skipCustomListeners, Boolean skipIoMappings, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling deleteProcessDefinitionsByKeyAndTenantId");
}
// verify the required parameter 'tenantId' is set
if (tenantId == null) {
throw new ApiException(400, "Missing the required parameter 'tenantId' when calling deleteProcessDefinitionsByKeyAndTenantId");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/tenant-id/{tenant-id}"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()))
.replaceAll("\\{" + "tenant-id" + "\\}", apiClient.escapeString(tenantId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("cascade", cascade));
localVarQueryParams.addAll(apiClient.parameterToPair("skipCustomListeners", skipCustomListeners));
localVarQueryParams.addAll(apiClient.parameterToPair("skipIoMappings", skipIoMappings));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"DELETE",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Get Activity Instance Statistics
* Retrieves runtime statistics of a given process definition, grouped by activities. These statistics include the number of running activity instances, optionally the number of failed jobs and also optionally the number of incidents either grouped by incident types or for a specific incident type. **Note**: This does not include historic data.
* @param id The id of the process definition. (required)
* @param failedJobs Whether to include the number of failed jobs in the result or not. Valid values are `true` or `false`. (optional)
* @param incidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType`. (optional)
* @param incidentsForType If this property has been set with any incident type (i.e., a string value) the result will only include the number of incidents for the assigned incident type. Cannot be used in combination with `incidents`. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @return List<ActivityStatisticsResultDto>
* @throws ApiException if fails to make API call
*/
public List getActivityStatistics(String id, Boolean failedJobs, Boolean incidents, String incidentsForType) throws ApiException {
return this.getActivityStatistics(id, failedJobs, incidents, incidentsForType, Collections.emptyMap());
}
/**
* Get Activity Instance Statistics
* Retrieves runtime statistics of a given process definition, grouped by activities. These statistics include the number of running activity instances, optionally the number of failed jobs and also optionally the number of incidents either grouped by incident types or for a specific incident type. **Note**: This does not include historic data.
* @param id The id of the process definition. (required)
* @param failedJobs Whether to include the number of failed jobs in the result or not. Valid values are `true` or `false`. (optional)
* @param incidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType`. (optional)
* @param incidentsForType If this property has been set with any incident type (i.e., a string value) the result will only include the number of incidents for the assigned incident type. Cannot be used in combination with `incidents`. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<ActivityStatisticsResultDto>
* @throws ApiException if fails to make API call
*/
public List getActivityStatistics(String id, Boolean failedJobs, Boolean incidents, String incidentsForType, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getActivityStatistics");
}
// create path and map variables
String localVarPath = "/process-definition/{id}/statistics"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("failedJobs", failedJobs));
localVarQueryParams.addAll(apiClient.parameterToPair("incidents", incidents));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentsForType", incidentsForType));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Activity Instance Statistics
* Retrieves runtime statistics of the latest version of the given process definition which belongs to no tenant, grouped by activities. These statistics include the number of running activity instances, optionally the number of failed jobs and also optionally the number of incidents either grouped by incident types or for a specific incident type. **Note**: This does not include historic data.
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param failedJobs Whether to include the number of failed jobs in the result or not. Valid values are `true` or `false`. (optional)
* @param incidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType`. (optional)
* @param incidentsForType If this property has been set with any incident type (i.e., a string value) the result will only include the number of incidents for the assigned incident type. Cannot be used in combination with `incidents`. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @return List<ActivityStatisticsResultDto>
* @throws ApiException if fails to make API call
*/
public List getActivityStatisticsByProcessDefinitionKey(String key, Boolean failedJobs, Boolean incidents, String incidentsForType) throws ApiException {
return this.getActivityStatisticsByProcessDefinitionKey(key, failedJobs, incidents, incidentsForType, Collections.emptyMap());
}
/**
* Get Activity Instance Statistics
* Retrieves runtime statistics of the latest version of the given process definition which belongs to no tenant, grouped by activities. These statistics include the number of running activity instances, optionally the number of failed jobs and also optionally the number of incidents either grouped by incident types or for a specific incident type. **Note**: This does not include historic data.
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param failedJobs Whether to include the number of failed jobs in the result or not. Valid values are `true` or `false`. (optional)
* @param incidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType`. (optional)
* @param incidentsForType If this property has been set with any incident type (i.e., a string value) the result will only include the number of incidents for the assigned incident type. Cannot be used in combination with `incidents`. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<ActivityStatisticsResultDto>
* @throws ApiException if fails to make API call
*/
public List getActivityStatisticsByProcessDefinitionKey(String key, Boolean failedJobs, Boolean incidents, String incidentsForType, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getActivityStatisticsByProcessDefinitionKey");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/statistics"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("failedJobs", failedJobs));
localVarQueryParams.addAll(apiClient.parameterToPair("incidents", incidents));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentsForType", incidentsForType));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Activity Instance Statistics
* Retrieves runtime statistics of the latest version of the given process definition for a tenant, grouped by activities. These statistics include the number of running activity instances, optionally the number of failed jobs and also optionally the number of incidents either grouped by incident types or for a specific incident type. **Note**: This does not include historic data.
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param tenantId The id of the tenant the process definition belongs to. (required)
* @param failedJobs Whether to include the number of failed jobs in the result or not. Valid values are `true` or `false`. (optional)
* @param incidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType`. (optional)
* @param incidentsForType If this property has been set with any incident type (i.e., a string value) the result will only include the number of incidents for the assigned incident type. Cannot be used in combination with `incidents`. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @return List<ActivityStatisticsResultDto>
* @throws ApiException if fails to make API call
*/
public List getActivityStatisticsByProcessDefinitionKeyAndTenantId(String key, String tenantId, Boolean failedJobs, Boolean incidents, String incidentsForType) throws ApiException {
return this.getActivityStatisticsByProcessDefinitionKeyAndTenantId(key, tenantId, failedJobs, incidents, incidentsForType, Collections.emptyMap());
}
/**
* Get Activity Instance Statistics
* Retrieves runtime statistics of the latest version of the given process definition for a tenant, grouped by activities. These statistics include the number of running activity instances, optionally the number of failed jobs and also optionally the number of incidents either grouped by incident types or for a specific incident type. **Note**: This does not include historic data.
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param tenantId The id of the tenant the process definition belongs to. (required)
* @param failedJobs Whether to include the number of failed jobs in the result or not. Valid values are `true` or `false`. (optional)
* @param incidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType`. (optional)
* @param incidentsForType If this property has been set with any incident type (i.e., a string value) the result will only include the number of incidents for the assigned incident type. Cannot be used in combination with `incidents`. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<ActivityStatisticsResultDto>
* @throws ApiException if fails to make API call
*/
public List getActivityStatisticsByProcessDefinitionKeyAndTenantId(String key, String tenantId, Boolean failedJobs, Boolean incidents, String incidentsForType, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getActivityStatisticsByProcessDefinitionKeyAndTenantId");
}
// verify the required parameter 'tenantId' is set
if (tenantId == null) {
throw new ApiException(400, "Missing the required parameter 'tenantId' when calling getActivityStatisticsByProcessDefinitionKeyAndTenantId");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/tenant-id/{tenant-id}/statistics"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()))
.replaceAll("\\{" + "tenant-id" + "\\}", apiClient.escapeString(tenantId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("failedJobs", failedJobs));
localVarQueryParams.addAll(apiClient.parameterToPair("incidents", incidents));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentsForType", incidentsForType));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Deployed Start Form
* Retrieves the deployed form that can be referenced from a start event. For further information please refer to [User Guide](https://docs.camunda.org/manual/7.21/user-guide/task-forms/#embedded-task-forms).
* @param id The id of the process definition to get the deployed start form for. (required)
* @return File
* @throws ApiException if fails to make API call
*/
public File getDeployedStartForm(String id) throws ApiException {
return this.getDeployedStartForm(id, Collections.emptyMap());
}
/**
* Get Deployed Start Form
* Retrieves the deployed form that can be referenced from a start event. For further information please refer to [User Guide](https://docs.camunda.org/manual/7.21/user-guide/task-forms/#embedded-task-forms).
* @param id The id of the process definition to get the deployed start form for. (required)
* @param additionalHeaders additionalHeaders for this call
* @return File
* @throws ApiException if fails to make API call
*/
public File getDeployedStartForm(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getDeployedStartForm");
}
// create path and map variables
String localVarPath = "/process-definition/{id}/deployed-start-form"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/xhtml+xml", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Deployed Start Form
* Retrieves the deployed form that can be referenced from a start event. For further information please refer to [User Guide](https://docs.camunda.org/manual/7.21/user-guide/task-forms/#embedded-task-forms).
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @return File
* @throws ApiException if fails to make API call
*/
public File getDeployedStartFormByKey(String key) throws ApiException {
return this.getDeployedStartFormByKey(key, Collections.emptyMap());
}
/**
* Get Deployed Start Form
* Retrieves the deployed form that can be referenced from a start event. For further information please refer to [User Guide](https://docs.camunda.org/manual/7.21/user-guide/task-forms/#embedded-task-forms).
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param additionalHeaders additionalHeaders for this call
* @return File
* @throws ApiException if fails to make API call
*/
public File getDeployedStartFormByKey(String key, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getDeployedStartFormByKey");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/deployed-start-form"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/xhtml+xml", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Deployed Start Form
* Retrieves the deployed form that can be referenced from a start event. For further information please refer to [User Guide](https://docs.camunda.org/manual/7.21/user-guide/task-forms/#embedded-task-forms).
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param tenantId The id of the tenant the process definitions belong to. (required)
* @return File
* @throws ApiException if fails to make API call
*/
public File getDeployedStartFormByKeyAndTenantId(String key, String tenantId) throws ApiException {
return this.getDeployedStartFormByKeyAndTenantId(key, tenantId, Collections.emptyMap());
}
/**
* Get Deployed Start Form
* Retrieves the deployed form that can be referenced from a start event. For further information please refer to [User Guide](https://docs.camunda.org/manual/7.21/user-guide/task-forms/#embedded-task-forms).
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param tenantId The id of the tenant the process definitions belong to. (required)
* @param additionalHeaders additionalHeaders for this call
* @return File
* @throws ApiException if fails to make API call
*/
public File getDeployedStartFormByKeyAndTenantId(String key, String tenantId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getDeployedStartFormByKeyAndTenantId");
}
// verify the required parameter 'tenantId' is set
if (tenantId == null) {
throw new ApiException(400, "Missing the required parameter 'tenantId' when calling getDeployedStartFormByKeyAndTenantId");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/tenant-id/{tenant-id}/deployed-start-form"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()))
.replaceAll("\\{" + "tenant-id" + "\\}", apiClient.escapeString(tenantId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/xhtml+xml", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get
* Retrieves the latest version of the process definition for tenant according to the `ProcessDefinition` interface in the engine.
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param tenantId The id of the tenant the process definition belongs to. (required)
* @return ProcessDefinitionDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDto getLatestProcessDefinitionByTenantId(String key, String tenantId) throws ApiException {
return this.getLatestProcessDefinitionByTenantId(key, tenantId, Collections.emptyMap());
}
/**
* Get
* Retrieves the latest version of the process definition for tenant according to the `ProcessDefinition` interface in the engine.
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param tenantId The id of the tenant the process definition belongs to. (required)
* @param additionalHeaders additionalHeaders for this call
* @return ProcessDefinitionDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDto getLatestProcessDefinitionByTenantId(String key, String tenantId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getLatestProcessDefinitionByTenantId");
}
// verify the required parameter 'tenantId' is set
if (tenantId == null) {
throw new ApiException(400, "Missing the required parameter 'tenantId' when calling getLatestProcessDefinitionByTenantId");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/tenant-id/{tenant-id}"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()))
.replaceAll("\\{" + "tenant-id" + "\\}", apiClient.escapeString(tenantId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get
* Retrieves a process definition according to the `ProcessDefinition` interface in the engine.
* @param id The id of the process definition to be retrieved. (required)
* @return ProcessDefinitionDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDto getProcessDefinition(String id) throws ApiException {
return this.getProcessDefinition(id, Collections.emptyMap());
}
/**
* Get
* Retrieves a process definition according to the `ProcessDefinition` interface in the engine.
* @param id The id of the process definition to be retrieved. (required)
* @param additionalHeaders additionalHeaders for this call
* @return ProcessDefinitionDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDto getProcessDefinition(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getProcessDefinition");
}
// create path and map variables
String localVarPath = "/process-definition/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get XML
* Retrieves the BPMN 2.0 XML of a process definition.
* @param id The id of the process definition. (required)
* @return ProcessDefinitionDiagramDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDiagramDto getProcessDefinitionBpmn20Xml(String id) throws ApiException {
return this.getProcessDefinitionBpmn20Xml(id, Collections.emptyMap());
}
/**
* Get XML
* Retrieves the BPMN 2.0 XML of a process definition.
* @param id The id of the process definition. (required)
* @param additionalHeaders additionalHeaders for this call
* @return ProcessDefinitionDiagramDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDiagramDto getProcessDefinitionBpmn20Xml(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getProcessDefinitionBpmn20Xml");
}
// create path and map variables
String localVarPath = "/process-definition/{id}/xml"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get XML
* Retrieves latest version the BPMN 2.0 XML of a process definition.
* @param key The key of the process definition (the latest version thereof) whose XML should be retrieved. (required)
* @return ProcessDefinitionDiagramDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDiagramDto getProcessDefinitionBpmn20XmlByKey(String key) throws ApiException {
return this.getProcessDefinitionBpmn20XmlByKey(key, Collections.emptyMap());
}
/**
* Get XML
* Retrieves latest version the BPMN 2.0 XML of a process definition.
* @param key The key of the process definition (the latest version thereof) whose XML should be retrieved. (required)
* @param additionalHeaders additionalHeaders for this call
* @return ProcessDefinitionDiagramDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDiagramDto getProcessDefinitionBpmn20XmlByKey(String key, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getProcessDefinitionBpmn20XmlByKey");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/xml"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get XML
* Retrieves latest version the BPMN 2.0 XML of a process definition. Returns the XML for the latest version of the process definition for tenant.
* @param key The key of the process definition (the latest version thereof) whose XML should be retrieved. (required)
* @param tenantId The id of the tenant the process definition belongs to. (required)
* @return ProcessDefinitionDiagramDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDiagramDto getProcessDefinitionBpmn20XmlByKeyAndTenantId(String key, String tenantId) throws ApiException {
return this.getProcessDefinitionBpmn20XmlByKeyAndTenantId(key, tenantId, Collections.emptyMap());
}
/**
* Get XML
* Retrieves latest version the BPMN 2.0 XML of a process definition. Returns the XML for the latest version of the process definition for tenant.
* @param key The key of the process definition (the latest version thereof) whose XML should be retrieved. (required)
* @param tenantId The id of the tenant the process definition belongs to. (required)
* @param additionalHeaders additionalHeaders for this call
* @return ProcessDefinitionDiagramDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDiagramDto getProcessDefinitionBpmn20XmlByKeyAndTenantId(String key, String tenantId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getProcessDefinitionBpmn20XmlByKeyAndTenantId");
}
// verify the required parameter 'tenantId' is set
if (tenantId == null) {
throw new ApiException(400, "Missing the required parameter 'tenantId' when calling getProcessDefinitionBpmn20XmlByKeyAndTenantId");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/tenant-id/{tenant-id}/xml"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()))
.replaceAll("\\{" + "tenant-id" + "\\}", apiClient.escapeString(tenantId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get
* Retrieves the latest version of the process definition which belongs to no tenant according to the `ProcessDefinition` interface in the engine.
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @return ProcessDefinitionDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDto getProcessDefinitionByKey(String key) throws ApiException {
return this.getProcessDefinitionByKey(key, Collections.emptyMap());
}
/**
* Get
* Retrieves the latest version of the process definition which belongs to no tenant according to the `ProcessDefinition` interface in the engine.
* @param key The key of the process definition (the latest version thereof) to be retrieved. (required)
* @param additionalHeaders additionalHeaders for this call
* @return ProcessDefinitionDto
* @throws ApiException if fails to make API call
*/
public ProcessDefinitionDto getProcessDefinitionByKey(String key, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getProcessDefinitionByKey");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Diagram
* Retrieves the diagram of a process definition. If the process definition's deployment contains an image resource with the same file name as the process definition, the deployed image will be returned by the Get Diagram endpoint. Example: `someProcess.bpmn` and `someProcess.png`. Supported file extentions for the image are: `svg`, `png`, `jpg`, and `gif`.
* @param id The id of the process definition. (required)
* @return File
* @throws ApiException if fails to make API call
*/
public File getProcessDefinitionDiagram(String id) throws ApiException {
return this.getProcessDefinitionDiagram(id, Collections.emptyMap());
}
/**
* Get Diagram
* Retrieves the diagram of a process definition. If the process definition's deployment contains an image resource with the same file name as the process definition, the deployed image will be returned by the Get Diagram endpoint. Example: `someProcess.bpmn` and `someProcess.png`. Supported file extentions for the image are: `svg`, `png`, `jpg`, and `gif`.
* @param id The id of the process definition. (required)
* @param additionalHeaders additionalHeaders for this call
* @return File
* @throws ApiException if fails to make API call
*/
public File getProcessDefinitionDiagram(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getProcessDefinitionDiagram");
}
// create path and map variables
String localVarPath = "/process-definition/{id}/diagram"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/octet-stream", "*/*", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Diagram
* Retrieves the diagram for the latest version of the process definition which belongs to no tenant. If the process definition's deployment contains an image resource with the same file name as the process definition, the deployed image will be returned by the Get Diagram endpoint. Example: `someProcess.bpmn` and `someProcess.png`. Supported file extentions for the image are: `svg`, `png`, `jpg`, and `gif`.
* @param key The key of the process definition. (required)
* @return File
* @throws ApiException if fails to make API call
*/
public File getProcessDefinitionDiagramByKey(String key) throws ApiException {
return this.getProcessDefinitionDiagramByKey(key, Collections.emptyMap());
}
/**
* Get Diagram
* Retrieves the diagram for the latest version of the process definition which belongs to no tenant. If the process definition's deployment contains an image resource with the same file name as the process definition, the deployed image will be returned by the Get Diagram endpoint. Example: `someProcess.bpmn` and `someProcess.png`. Supported file extentions for the image are: `svg`, `png`, `jpg`, and `gif`.
* @param key The key of the process definition. (required)
* @param additionalHeaders additionalHeaders for this call
* @return File
* @throws ApiException if fails to make API call
*/
public File getProcessDefinitionDiagramByKey(String key, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getProcessDefinitionDiagramByKey");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/diagram"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/octet-stream", "*/*", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Diagram
* Retrieves the diagram for the latest version of the process definition for tenant. If the process definition's deployment contains an image resource with the same file name as the process definition, the deployed image will be returned by the Get Diagram endpoint. Example: `someProcess.bpmn` and `someProcess.png`. Supported file extentions for the image are: `svg`, `png`, `jpg`, and `gif`.
* @param key The key of the process definition. (required)
* @param tenantId The id of the tenant the process definition belongs to. (required)
* @return File
* @throws ApiException if fails to make API call
*/
public File getProcessDefinitionDiagramByKeyAndTenantId(String key, String tenantId) throws ApiException {
return this.getProcessDefinitionDiagramByKeyAndTenantId(key, tenantId, Collections.emptyMap());
}
/**
* Get Diagram
* Retrieves the diagram for the latest version of the process definition for tenant. If the process definition's deployment contains an image resource with the same file name as the process definition, the deployed image will be returned by the Get Diagram endpoint. Example: `someProcess.bpmn` and `someProcess.png`. Supported file extentions for the image are: `svg`, `png`, `jpg`, and `gif`.
* @param key The key of the process definition. (required)
* @param tenantId The id of the tenant the process definition belongs to. (required)
* @param additionalHeaders additionalHeaders for this call
* @return File
* @throws ApiException if fails to make API call
*/
public File getProcessDefinitionDiagramByKeyAndTenantId(String key, String tenantId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new ApiException(400, "Missing the required parameter 'key' when calling getProcessDefinitionDiagramByKeyAndTenantId");
}
// verify the required parameter 'tenantId' is set
if (tenantId == null) {
throw new ApiException(400, "Missing the required parameter 'tenantId' when calling getProcessDefinitionDiagramByKeyAndTenantId");
}
// create path and map variables
String localVarPath = "/process-definition/key/{key}/tenant-id/{tenant-id}/diagram"
.replaceAll("\\{" + "key" + "\\}", apiClient.escapeString(key.toString()))
.replaceAll("\\{" + "tenant-id" + "\\}", apiClient.escapeString(tenantId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/octet-stream", "*/*", "application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Process Instance Statistics
* Retrieves runtime statistics of the process engine, grouped by process definitions. These statistics include the number of running process instances, optionally the number of failed jobs and also optionally the number of incidents either grouped by incident types or for a specific incident type. **Note**: This does not include historic data.
* @param failedJobs Whether to include the number of failed jobs in the result or not. Valid values are `true` or `false`. (optional)
* @param incidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType`. (optional)
* @param incidentsForType If this property has been set with any incident type (i.e., a string value) the result will only include the number of incidents for the assigned incident type. Cannot be used in combination with `incidents`. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param rootIncidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of root incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType` or `incidents`. (optional)
* @return List<ProcessDefinitionStatisticsResultDto>
* @throws ApiException if fails to make API call
*/
public List getProcessDefinitionStatistics(Boolean failedJobs, Boolean incidents, String incidentsForType, Boolean rootIncidents) throws ApiException {
return this.getProcessDefinitionStatistics(failedJobs, incidents, incidentsForType, rootIncidents, Collections.emptyMap());
}
/**
* Get Process Instance Statistics
* Retrieves runtime statistics of the process engine, grouped by process definitions. These statistics include the number of running process instances, optionally the number of failed jobs and also optionally the number of incidents either grouped by incident types or for a specific incident type. **Note**: This does not include historic data.
* @param failedJobs Whether to include the number of failed jobs in the result or not. Valid values are `true` or `false`. (optional)
* @param incidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType`. (optional)
* @param incidentsForType If this property has been set with any incident type (i.e., a string value) the result will only include the number of incidents for the assigned incident type. Cannot be used in combination with `incidents`. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param rootIncidents Valid values for this property are `true` or `false`. If this property has been set to `true` the result will include the corresponding number of root incidents for each occurred incident type. If it is set to `false`, the incidents will not be included in the result. Cannot be used in combination with `incidentsForType` or `incidents`. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<ProcessDefinitionStatisticsResultDto>
* @throws ApiException if fails to make API call
*/
public List getProcessDefinitionStatistics(Boolean failedJobs, Boolean incidents, String incidentsForType, Boolean rootIncidents, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/process-definition/statistics";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("failedJobs", failedJobs));
localVarQueryParams.addAll(apiClient.parameterToPair("incidents", incidents));
localVarQueryParams.addAll(apiClient.parameterToPair("incidentsForType", incidentsForType));
localVarQueryParams.addAll(apiClient.parameterToPair("rootIncidents", rootIncidents));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get List
* Queries for process definitions that fulfill given parameters. Parameters may be the properties of process definitions, such as the name, key or version. The size of the result set can be retrieved by using the [Get Definition Count](https://docs.camunda.org/manual/7.21/reference/rest/process-definition/get-query-count/) method.
* @param processDefinitionId Filter by process definition id. (optional)
* @param processDefinitionIdIn Filter by a comma-separated list of process definition ids. (optional)
* @param name Filter by process definition name. (optional)
* @param nameLike Filter by process definition names that the parameter is a substring of. (optional)
* @param deploymentId Filter by the deployment the id belongs to. (optional)
* @param deployedAfter Filter by the deploy time of the deployment the process definition belongs to. Only selects process definitions that have been deployed after (exclusive) a specific time. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.546+0200`. (optional)
* @param deployedAt Filter by the deploy time of the deployment the process definition belongs to. Only selects process definitions that have been deployed at a specific time (exact match). By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.546+0200`. (optional)
* @param key Filter by process definition key, i.e., the id in the BPMN 2.0 XML. Exact match. (optional)
* @param keysIn Filter by a comma-separated list of process definition keys. (optional)
* @param keyLike Filter by process definition keys that the parameter is a substring of. (optional)
* @param category Filter by process definition category. Exact match. (optional)
* @param categoryLike Filter by process definition categories that the parameter is a substring of. (optional)
* @param version Filter by process definition version. (optional)
* @param latestVersion Only include those process definitions that are latest versions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param resourceName Filter by the name of the process definition resource. Exact match. (optional)
* @param resourceNameLike Filter by names of those process definition resources that the parameter is a substring of. (optional)
* @param startableBy Filter by a user name who is allowed to start the process. (optional)
* @param active Only include active process definitions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param suspended Only include suspended process definitions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param incidentId Filter by the incident id. (optional)
* @param incidentType Filter by the incident type. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param incidentMessage Filter by the incident message. Exact match. (optional)
* @param incidentMessageLike Filter by the incident message that the parameter is a substring of. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. A process definition must have one of the given tenant ids. (optional)
* @param withoutTenantId Only include process definitions which belong to no tenant. Value may only be true, as false is the default behavior. (optional)
* @param includeProcessDefinitionsWithoutTenantId Include process definitions which belong to no tenant. Can be used in combination with `tenantIdIn`. Value may only be `true`, as `false` is the default behavior. (optional)
* @param versionTag Filter by the version tag. (optional)
* @param versionTagLike Filter by the version tag that the parameter is a substring of. (optional)
* @param withoutVersionTag Only include process definitions without a `versionTag`. (optional)
* @param startableInTasklist Filter by process definitions which are startable in Tasklist.. (optional)
* @param notStartableInTasklist Filter by process definitions which are not startable in Tasklist. (optional)
* @param startablePermissionCheck Filter by process definitions which the user is allowed to start in Tasklist. If the user doesn't have these permissions the result will be empty list. The permissions are: * `CREATE` permission for all Process instances * `CREATE_INSTANCE` and `READ` permission on Process definition level (optional)
* @param sortBy Sort the results lexicographically by a given criterion. Must be used in conjunction with the sortOrder parameter. (optional)
* @param sortOrder Sort the results in a given order. Values may be asc for ascending order or desc for descending order. Must be used in conjunction with the sortBy parameter. (optional)
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @return List<ProcessDefinitionDto>
* @throws ApiException if fails to make API call
*/
public List getProcessDefinitions(String processDefinitionId, String processDefinitionIdIn, String name, String nameLike, String deploymentId, OffsetDateTime deployedAfter, OffsetDateTime deployedAt, String key, String keysIn, String keyLike, String category, String categoryLike, Integer version, Boolean latestVersion, String resourceName, String resourceNameLike, String startableBy, Boolean active, Boolean suspended, String incidentId, String incidentType, String incidentMessage, String incidentMessageLike, String tenantIdIn, Boolean withoutTenantId, Boolean includeProcessDefinitionsWithoutTenantId, String versionTag, String versionTagLike, Boolean withoutVersionTag, Boolean startableInTasklist, Boolean notStartableInTasklist, Boolean startablePermissionCheck, String sortBy, String sortOrder, Integer firstResult, Integer maxResults) throws ApiException {
return this.getProcessDefinitions(processDefinitionId, processDefinitionIdIn, name, nameLike, deploymentId, deployedAfter, deployedAt, key, keysIn, keyLike, category, categoryLike, version, latestVersion, resourceName, resourceNameLike, startableBy, active, suspended, incidentId, incidentType, incidentMessage, incidentMessageLike, tenantIdIn, withoutTenantId, includeProcessDefinitionsWithoutTenantId, versionTag, versionTagLike, withoutVersionTag, startableInTasklist, notStartableInTasklist, startablePermissionCheck, sortBy, sortOrder, firstResult, maxResults, Collections.emptyMap());
}
/**
* Get List
* Queries for process definitions that fulfill given parameters. Parameters may be the properties of process definitions, such as the name, key or version. The size of the result set can be retrieved by using the [Get Definition Count](https://docs.camunda.org/manual/7.21/reference/rest/process-definition/get-query-count/) method.
* @param processDefinitionId Filter by process definition id. (optional)
* @param processDefinitionIdIn Filter by a comma-separated list of process definition ids. (optional)
* @param name Filter by process definition name. (optional)
* @param nameLike Filter by process definition names that the parameter is a substring of. (optional)
* @param deploymentId Filter by the deployment the id belongs to. (optional)
* @param deployedAfter Filter by the deploy time of the deployment the process definition belongs to. Only selects process definitions that have been deployed after (exclusive) a specific time. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.546+0200`. (optional)
* @param deployedAt Filter by the deploy time of the deployment the process definition belongs to. Only selects process definitions that have been deployed at a specific time (exact match). By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.546+0200`. (optional)
* @param key Filter by process definition key, i.e., the id in the BPMN 2.0 XML. Exact match. (optional)
* @param keysIn Filter by a comma-separated list of process definition keys. (optional)
* @param keyLike Filter by process definition keys that the parameter is a substring of. (optional)
* @param category Filter by process definition category. Exact match. (optional)
* @param categoryLike Filter by process definition categories that the parameter is a substring of. (optional)
* @param version Filter by process definition version. (optional)
* @param latestVersion Only include those process definitions that are latest versions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param resourceName Filter by the name of the process definition resource. Exact match. (optional)
* @param resourceNameLike Filter by names of those process definition resources that the parameter is a substring of. (optional)
* @param startableBy Filter by a user name who is allowed to start the process. (optional)
* @param active Only include active process definitions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param suspended Only include suspended process definitions. Value may only be `true`, as `false` is the default behavior. (optional)
* @param incidentId Filter by the incident id. (optional)
* @param incidentType Filter by the incident type. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types. (optional)
* @param incidentMessage Filter by the incident message. Exact match. (optional)
* @param incidentMessageLike Filter by the incident message that the parameter is a substring of. (optional)
* @param tenantIdIn Filter by a comma-separated list of tenant ids. A process definition must have one of the given tenant ids. (optional)
* @param withoutTenantId Only include process definitions which belong to no tenant. Value may only be true, as false is the default behavior. (optional)
* @param includeProcessDefinitionsWithoutTenantId Include process definitions which belong to no tenant. Can be used in combination with `tenantIdIn`. Value may only be `true`, as `false` is the default behavior. (optional)
* @param versionTag Filter by the version tag. (optional)
* @param versionTagLike Filter by the version tag that the parameter is a substring of. (optional)
* @param withoutVersionTag Only include process definitions without a `versionTag`. (optional)
* @param startableInTasklist Filter by process definitions which are startable in Tasklist.. (optional)
* @param notStartableInTasklist Filter by process definitions which are not startable in Tasklist. (optional)
* @param startablePermissionCheck Filter by process definitions which the user is allowed to start in Tasklist. If the user doesn't have these permissions the result will be empty list. The permissions are: * `CREATE` permission for all Process instances * `CREATE_INSTANCE` and `READ` permission on Process definition level (optional)
* @param sortBy Sort the results lexicographically by a given criterion. Must be used in conjunction with the sortOrder parameter. (optional)
* @param sortOrder Sort the results in a given order. Values may be asc for ascending order or desc for descending order. Must be used in conjunction with the sortBy parameter. (optional)
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<ProcessDefinitionDto>
* @throws ApiException if fails to make API call
*/
public List