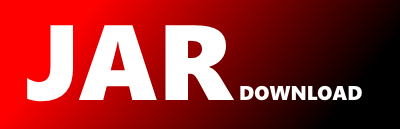
org.camunda.community.rest.client.api.UserApi Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.api;
import com.fasterxml.jackson.core.type.TypeReference;
import org.camunda.community.rest.client.invoker.ApiException;
import org.camunda.community.rest.client.invoker.ApiClient;
import org.camunda.community.rest.client.invoker.Configuration;
import org.camunda.community.rest.client.invoker.Pair;
import org.camunda.community.rest.client.dto.CountResultDto;
import org.camunda.community.rest.client.dto.ExceptionDto;
import org.camunda.community.rest.client.dto.ResourceOptionsDto;
import org.camunda.community.rest.client.dto.UserCredentialsDto;
import org.camunda.community.rest.client.dto.UserDto;
import org.camunda.community.rest.client.dto.UserProfileDto;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class UserApi {
private ApiClient apiClient;
public UserApi() {
this(Configuration.getDefaultApiClient());
}
public UserApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Options
* The `/user` resource supports two custom `OPTIONS` requests, one for the resource as such and one for individual user instances. The `OPTIONS` request allows checking for the set of available operations that the currently authenticated user can perform on the /user resource. If the user can perform an operation or not may depend on various things, including the user's authorizations to interact with this resource and the internal configuration of the process engine. `OPTIONS /user` returns available interactions on the resource.
* @return ResourceOptionsDto
* @throws ApiException if fails to make API call
*/
public ResourceOptionsDto availableOperations() throws ApiException {
return this.availableOperations(Collections.emptyMap());
}
/**
* Options
* The `/user` resource supports two custom `OPTIONS` requests, one for the resource as such and one for individual user instances. The `OPTIONS` request allows checking for the set of available operations that the currently authenticated user can perform on the /user resource. If the user can perform an operation or not may depend on various things, including the user's authorizations to interact with this resource and the internal configuration of the process engine. `OPTIONS /user` returns available interactions on the resource.
* @param additionalHeaders additionalHeaders for this call
* @return ResourceOptionsDto
* @throws ApiException if fails to make API call
*/
public ResourceOptionsDto availableOperations(Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/user";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"OPTIONS",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Options
* The `/user` resource supports two custom `OPTIONS` requests, one for the resource as such and one for individual user instances. The `OPTIONS` request allows checking for the set of available operations that the currently authenticated user can perform on the /user resource. If the user can perform an operation or not may depend on various things, including the user's authorizations to interact with this resource and the internal configuration of the process engine. `OPTIONS /user/{id}` returns available interactions on a resource instance.
* @param id The id of the user to be deleted. (required)
* @return ResourceOptionsDto
* @throws ApiException if fails to make API call
*/
public ResourceOptionsDto availableUserOperations(String id) throws ApiException {
return this.availableUserOperations(id, Collections.emptyMap());
}
/**
* Options
* The `/user` resource supports two custom `OPTIONS` requests, one for the resource as such and one for individual user instances. The `OPTIONS` request allows checking for the set of available operations that the currently authenticated user can perform on the /user resource. If the user can perform an operation or not may depend on various things, including the user's authorizations to interact with this resource and the internal configuration of the process engine. `OPTIONS /user/{id}` returns available interactions on a resource instance.
* @param id The id of the user to be deleted. (required)
* @param additionalHeaders additionalHeaders for this call
* @return ResourceOptionsDto
* @throws ApiException if fails to make API call
*/
public ResourceOptionsDto availableUserOperations(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling availableUserOperations");
}
// create path and map variables
String localVarPath = "/user/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"OPTIONS",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Create
* Create a new user.
* @param userDto (optional)
* @throws ApiException if fails to make API call
*/
public void createUser(UserDto userDto) throws ApiException {
this.createUser(userDto, Collections.emptyMap());
}
/**
* Create
* Create a new user.
* @param userDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void createUser(UserDto userDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = userDto;
// create path and map variables
String localVarPath = "/user/create";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Delete
* Deletes a user by id.
* @param id The id of the user to be deleted. (required)
* @throws ApiException if fails to make API call
*/
public void deleteUser(String id) throws ApiException {
this.deleteUser(id, Collections.emptyMap());
}
/**
* Delete
* Deletes a user by id.
* @param id The id of the user to be deleted. (required)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void deleteUser(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling deleteUser");
}
// create path and map variables
String localVarPath = "/user/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"DELETE",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Get List Count
* Queries for the number of deployments that fulfill given parameters. Takes the same parameters as the [Get Users](https://docs.camunda.org/manual/7.21/reference/rest/user/get-query/) method.
* @param id Filter by user id (optional)
* @param idIn Filter by a comma-separated list of user ids. (optional)
* @param firstName Filter by the first name of the user. Exact match. (optional)
* @param firstNameLike Filter by the first name that the parameter is a substring of. (optional)
* @param lastName Filter by the last name of the user. Exact match. (optional)
* @param lastNameLike Filter by the last name that the parameter is a substring of. (optional)
* @param email Filter by the email of the user. Exact match. (optional)
* @param emailLike Filter by the email that the parameter is a substring of. (optional)
* @param memberOfGroup Filter for users which are members of the given group. (optional)
* @param memberOfTenant Filter for users which are members of the given tenant. (optional)
* @param potentialStarter Only select Users that are potential starter for the given process definition. (optional)
* @return CountResultDto
* @throws ApiException if fails to make API call
*/
public CountResultDto getUserCount(String id, String idIn, String firstName, String firstNameLike, String lastName, String lastNameLike, String email, String emailLike, String memberOfGroup, String memberOfTenant, String potentialStarter) throws ApiException {
return this.getUserCount(id, idIn, firstName, firstNameLike, lastName, lastNameLike, email, emailLike, memberOfGroup, memberOfTenant, potentialStarter, Collections.emptyMap());
}
/**
* Get List Count
* Queries for the number of deployments that fulfill given parameters. Takes the same parameters as the [Get Users](https://docs.camunda.org/manual/7.21/reference/rest/user/get-query/) method.
* @param id Filter by user id (optional)
* @param idIn Filter by a comma-separated list of user ids. (optional)
* @param firstName Filter by the first name of the user. Exact match. (optional)
* @param firstNameLike Filter by the first name that the parameter is a substring of. (optional)
* @param lastName Filter by the last name of the user. Exact match. (optional)
* @param lastNameLike Filter by the last name that the parameter is a substring of. (optional)
* @param email Filter by the email of the user. Exact match. (optional)
* @param emailLike Filter by the email that the parameter is a substring of. (optional)
* @param memberOfGroup Filter for users which are members of the given group. (optional)
* @param memberOfTenant Filter for users which are members of the given tenant. (optional)
* @param potentialStarter Only select Users that are potential starter for the given process definition. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return CountResultDto
* @throws ApiException if fails to make API call
*/
public CountResultDto getUserCount(String id, String idIn, String firstName, String firstNameLike, String lastName, String lastNameLike, String email, String emailLike, String memberOfGroup, String memberOfTenant, String potentialStarter, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/user/count";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("id", id));
localVarQueryParams.addAll(apiClient.parameterToPair("idIn", idIn));
localVarQueryParams.addAll(apiClient.parameterToPair("firstName", firstName));
localVarQueryParams.addAll(apiClient.parameterToPair("firstNameLike", firstNameLike));
localVarQueryParams.addAll(apiClient.parameterToPair("lastName", lastName));
localVarQueryParams.addAll(apiClient.parameterToPair("lastNameLike", lastNameLike));
localVarQueryParams.addAll(apiClient.parameterToPair("email", email));
localVarQueryParams.addAll(apiClient.parameterToPair("emailLike", emailLike));
localVarQueryParams.addAll(apiClient.parameterToPair("memberOfGroup", memberOfGroup));
localVarQueryParams.addAll(apiClient.parameterToPair("memberOfTenant", memberOfTenant));
localVarQueryParams.addAll(apiClient.parameterToPair("potentialStarter", potentialStarter));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get Profile
* Retrieves a user's profile.
* @param id The id of the user to retrieve. (required)
* @return UserProfileDto
* @throws ApiException if fails to make API call
*/
public UserProfileDto getUserProfile(String id) throws ApiException {
return this.getUserProfile(id, Collections.emptyMap());
}
/**
* Get Profile
* Retrieves a user's profile.
* @param id The id of the user to retrieve. (required)
* @param additionalHeaders additionalHeaders for this call
* @return UserProfileDto
* @throws ApiException if fails to make API call
*/
public UserProfileDto getUserProfile(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getUserProfile");
}
// create path and map variables
String localVarPath = "/user/{id}/profile"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get List
* Query for a list of users using a list of parameters. The size of the result set can be retrieved by using the Get User Count method. [Get User Count](https://docs.camunda.org/manual/7.21/reference/rest/user/get-query-count/) method.
* @param id Filter by user id (optional)
* @param idIn Filter by a comma-separated list of user ids. (optional)
* @param firstName Filter by the first name of the user. Exact match. (optional)
* @param firstNameLike Filter by the first name that the parameter is a substring of. (optional)
* @param lastName Filter by the last name of the user. Exact match. (optional)
* @param lastNameLike Filter by the last name that the parameter is a substring of. (optional)
* @param email Filter by the email of the user. Exact match. (optional)
* @param emailLike Filter by the email that the parameter is a substring of. (optional)
* @param memberOfGroup Filter for users which are members of the given group. (optional)
* @param memberOfTenant Filter for users which are members of the given tenant. (optional)
* @param potentialStarter Only select Users that are potential starter for the given process definition. (optional)
* @param sortBy Sort the results lexicographically by a given criterion. Must be used in conjunction with the sortOrder parameter. (optional)
* @param sortOrder Sort the results in a given order. Values may be asc for ascending order or desc for descending order. Must be used in conjunction with the sortBy parameter. (optional)
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @return List<UserProfileDto>
* @throws ApiException if fails to make API call
*/
public List getUsers(String id, String idIn, String firstName, String firstNameLike, String lastName, String lastNameLike, String email, String emailLike, String memberOfGroup, String memberOfTenant, String potentialStarter, String sortBy, String sortOrder, Integer firstResult, Integer maxResults) throws ApiException {
return this.getUsers(id, idIn, firstName, firstNameLike, lastName, lastNameLike, email, emailLike, memberOfGroup, memberOfTenant, potentialStarter, sortBy, sortOrder, firstResult, maxResults, Collections.emptyMap());
}
/**
* Get List
* Query for a list of users using a list of parameters. The size of the result set can be retrieved by using the Get User Count method. [Get User Count](https://docs.camunda.org/manual/7.21/reference/rest/user/get-query-count/) method.
* @param id Filter by user id (optional)
* @param idIn Filter by a comma-separated list of user ids. (optional)
* @param firstName Filter by the first name of the user. Exact match. (optional)
* @param firstNameLike Filter by the first name that the parameter is a substring of. (optional)
* @param lastName Filter by the last name of the user. Exact match. (optional)
* @param lastNameLike Filter by the last name that the parameter is a substring of. (optional)
* @param email Filter by the email of the user. Exact match. (optional)
* @param emailLike Filter by the email that the parameter is a substring of. (optional)
* @param memberOfGroup Filter for users which are members of the given group. (optional)
* @param memberOfTenant Filter for users which are members of the given tenant. (optional)
* @param potentialStarter Only select Users that are potential starter for the given process definition. (optional)
* @param sortBy Sort the results lexicographically by a given criterion. Must be used in conjunction with the sortOrder parameter. (optional)
* @param sortOrder Sort the results in a given order. Values may be asc for ascending order or desc for descending order. Must be used in conjunction with the sortBy parameter. (optional)
* @param firstResult Pagination of results. Specifies the index of the first result to return. (optional)
* @param maxResults Pagination of results. Specifies the maximum number of results to return. Will return less results if there are no more results left. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<UserProfileDto>
* @throws ApiException if fails to make API call
*/
public List getUsers(String id, String idIn, String firstName, String firstNameLike, String lastName, String lastNameLike, String email, String emailLike, String memberOfGroup, String memberOfTenant, String potentialStarter, String sortBy, String sortOrder, Integer firstResult, Integer maxResults, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/user";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("id", id));
localVarQueryParams.addAll(apiClient.parameterToPair("idIn", idIn));
localVarQueryParams.addAll(apiClient.parameterToPair("firstName", firstName));
localVarQueryParams.addAll(apiClient.parameterToPair("firstNameLike", firstNameLike));
localVarQueryParams.addAll(apiClient.parameterToPair("lastName", lastName));
localVarQueryParams.addAll(apiClient.parameterToPair("lastNameLike", lastNameLike));
localVarQueryParams.addAll(apiClient.parameterToPair("email", email));
localVarQueryParams.addAll(apiClient.parameterToPair("emailLike", emailLike));
localVarQueryParams.addAll(apiClient.parameterToPair("memberOfGroup", memberOfGroup));
localVarQueryParams.addAll(apiClient.parameterToPair("memberOfTenant", memberOfTenant));
localVarQueryParams.addAll(apiClient.parameterToPair("potentialStarter", potentialStarter));
localVarQueryParams.addAll(apiClient.parameterToPair("sortBy", sortBy));
localVarQueryParams.addAll(apiClient.parameterToPair("sortOrder", sortOrder));
localVarQueryParams.addAll(apiClient.parameterToPair("firstResult", firstResult));
localVarQueryParams.addAll(apiClient.parameterToPair("maxResults", maxResults));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Unlock User
* Unlocks a user by id.
* @param id The id of the user to be unlocked. (required)
* @throws ApiException if fails to make API call
*/
public void unlockUser(String id) throws ApiException {
this.unlockUser(id, Collections.emptyMap());
}
/**
* Unlock User
* Unlocks a user by id.
* @param id The id of the user to be unlocked. (required)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void unlockUser(String id, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling unlockUser");
}
// create path and map variables
String localVarPath = "/user/{id}/unlock"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Update Credentials
* Updates a user's credentials (password)
* @param id The id of the user to be updated. (required)
* @param userCredentialsDto (optional)
* @throws ApiException if fails to make API call
*/
public void updateCredentials(String id, UserCredentialsDto userCredentialsDto) throws ApiException {
this.updateCredentials(id, userCredentialsDto, Collections.emptyMap());
}
/**
* Update Credentials
* Updates a user's credentials (password)
* @param id The id of the user to be updated. (required)
* @param userCredentialsDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void updateCredentials(String id, UserCredentialsDto userCredentialsDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = userCredentialsDto;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling updateCredentials");
}
// create path and map variables
String localVarPath = "/user/{id}/credentials"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"PUT",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Update User Profile
* Updates the profile information of an already existing user.
* @param id The id of the user. (required)
* @param userProfileDto (optional)
* @throws ApiException if fails to make API call
*/
public void updateProfile(String id, UserProfileDto userProfileDto) throws ApiException {
this.updateProfile(id, userProfileDto, Collections.emptyMap());
}
/**
* Update User Profile
* Updates the profile information of an already existing user.
* @param id The id of the user. (required)
* @param userProfileDto (optional)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void updateProfile(String id, UserProfileDto userProfileDto, Map additionalHeaders) throws ApiException {
Object localVarPostBody = userProfileDto;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling updateProfile");
}
// create path and map variables
String localVarPath = "/user/{id}/profile"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(
localVarPath,
"PUT",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy