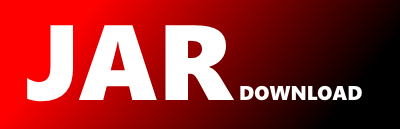
org.camunda.community.rest.client.dto.ActivityInstanceDto Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.dto;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.camunda.community.rest.client.dto.ActivityInstanceIncidentDto;
import org.camunda.community.rest.client.dto.TransitionInstanceDto;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* A JSON object corresponding to the Activity Instance tree of the given process instance.
*/
@JsonPropertyOrder({
ActivityInstanceDto.JSON_PROPERTY_ID,
ActivityInstanceDto.JSON_PROPERTY_PARENT_ACTIVITY_INSTANCE_ID,
ActivityInstanceDto.JSON_PROPERTY_ACTIVITY_ID,
ActivityInstanceDto.JSON_PROPERTY_ACTIVITY_NAME,
ActivityInstanceDto.JSON_PROPERTY_NAME,
ActivityInstanceDto.JSON_PROPERTY_ACTIVITY_TYPE,
ActivityInstanceDto.JSON_PROPERTY_PROCESS_INSTANCE_ID,
ActivityInstanceDto.JSON_PROPERTY_PROCESS_DEFINITION_ID,
ActivityInstanceDto.JSON_PROPERTY_CHILD_ACTIVITY_INSTANCES,
ActivityInstanceDto.JSON_PROPERTY_CHILD_TRANSITION_INSTANCES,
ActivityInstanceDto.JSON_PROPERTY_EXECUTION_IDS,
ActivityInstanceDto.JSON_PROPERTY_INCIDENT_IDS,
ActivityInstanceDto.JSON_PROPERTY_INCIDENTS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class ActivityInstanceDto {
public static final String JSON_PROPERTY_ID = "id";
private JsonNullable id = JsonNullable.undefined();
public static final String JSON_PROPERTY_PARENT_ACTIVITY_INSTANCE_ID = "parentActivityInstanceId";
private JsonNullable parentActivityInstanceId = JsonNullable.undefined();
public static final String JSON_PROPERTY_ACTIVITY_ID = "activityId";
private JsonNullable activityId = JsonNullable.undefined();
public static final String JSON_PROPERTY_ACTIVITY_NAME = "activityName";
private JsonNullable activityName = JsonNullable.undefined();
public static final String JSON_PROPERTY_NAME = "name";
private JsonNullable name = JsonNullable.undefined();
public static final String JSON_PROPERTY_ACTIVITY_TYPE = "activityType";
private JsonNullable activityType = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_INSTANCE_ID = "processInstanceId";
private JsonNullable processInstanceId = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_DEFINITION_ID = "processDefinitionId";
private JsonNullable processDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_CHILD_ACTIVITY_INSTANCES = "childActivityInstances";
private JsonNullable> childActivityInstances = JsonNullable.>undefined();
public static final String JSON_PROPERTY_CHILD_TRANSITION_INSTANCES = "childTransitionInstances";
private JsonNullable> childTransitionInstances = JsonNullable.>undefined();
public static final String JSON_PROPERTY_EXECUTION_IDS = "executionIds";
private JsonNullable> executionIds = JsonNullable.>undefined();
public static final String JSON_PROPERTY_INCIDENT_IDS = "incidentIds";
private JsonNullable> incidentIds = JsonNullable.>undefined();
public static final String JSON_PROPERTY_INCIDENTS = "incidents";
private JsonNullable> incidents = JsonNullable.>undefined();
public ActivityInstanceDto() {
}
public ActivityInstanceDto id(String id) {
this.id = JsonNullable.of(id);
return this;
}
/**
* The id of the activity instance.
* @return id
**/
@javax.annotation.Nullable
@JsonIgnore
public String getId() {
return id.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getId_JsonNullable() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
public void setId_JsonNullable(JsonNullable id) {
this.id = id;
}
public void setId(String id) {
this.id = JsonNullable.of(id);
}
public ActivityInstanceDto parentActivityInstanceId(String parentActivityInstanceId) {
this.parentActivityInstanceId = JsonNullable.of(parentActivityInstanceId);
return this;
}
/**
* The id of the parent activity instance, for example a sub process instance.
* @return parentActivityInstanceId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getParentActivityInstanceId() {
return parentActivityInstanceId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PARENT_ACTIVITY_INSTANCE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getParentActivityInstanceId_JsonNullable() {
return parentActivityInstanceId;
}
@JsonProperty(JSON_PROPERTY_PARENT_ACTIVITY_INSTANCE_ID)
public void setParentActivityInstanceId_JsonNullable(JsonNullable parentActivityInstanceId) {
this.parentActivityInstanceId = parentActivityInstanceId;
}
public void setParentActivityInstanceId(String parentActivityInstanceId) {
this.parentActivityInstanceId = JsonNullable.of(parentActivityInstanceId);
}
public ActivityInstanceDto activityId(String activityId) {
this.activityId = JsonNullable.of(activityId);
return this;
}
/**
* The id of the activity.
* @return activityId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getActivityId() {
return activityId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getActivityId_JsonNullable() {
return activityId;
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_ID)
public void setActivityId_JsonNullable(JsonNullable activityId) {
this.activityId = activityId;
}
public void setActivityId(String activityId) {
this.activityId = JsonNullable.of(activityId);
}
public ActivityInstanceDto activityName(String activityName) {
this.activityName = JsonNullable.of(activityName);
return this;
}
/**
* The name of the activity
* @return activityName
**/
@javax.annotation.Nullable
@JsonIgnore
public String getActivityName() {
return activityName.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getActivityName_JsonNullable() {
return activityName;
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_NAME)
public void setActivityName_JsonNullable(JsonNullable activityName) {
this.activityName = activityName;
}
public void setActivityName(String activityName) {
this.activityName = JsonNullable.of(activityName);
}
public ActivityInstanceDto name(String name) {
this.name = JsonNullable.of(name);
return this;
}
/**
* The name of the activity. This property is deprecated. Please use 'activityName'.
* @return name
**/
@javax.annotation.Nullable
@JsonIgnore
public String getName() {
return name.orElse(null);
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getName_JsonNullable() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
public void setName_JsonNullable(JsonNullable name) {
this.name = name;
}
public void setName(String name) {
this.name = JsonNullable.of(name);
}
public ActivityInstanceDto activityType(String activityType) {
this.activityType = JsonNullable.of(activityType);
return this;
}
/**
* The type of activity (corresponds to the XML element name in the BPMN 2.0, e.g., 'userTask')
* @return activityType
**/
@javax.annotation.Nullable
@JsonIgnore
public String getActivityType() {
return activityType.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getActivityType_JsonNullable() {
return activityType;
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_TYPE)
public void setActivityType_JsonNullable(JsonNullable activityType) {
this.activityType = activityType;
}
public void setActivityType(String activityType) {
this.activityType = JsonNullable.of(activityType);
}
public ActivityInstanceDto processInstanceId(String processInstanceId) {
this.processInstanceId = JsonNullable.of(processInstanceId);
return this;
}
/**
* The id of the process instance this activity instance is part of.
* @return processInstanceId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProcessInstanceId() {
return processInstanceId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessInstanceId_JsonNullable() {
return processInstanceId;
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_ID)
public void setProcessInstanceId_JsonNullable(JsonNullable processInstanceId) {
this.processInstanceId = processInstanceId;
}
public void setProcessInstanceId(String processInstanceId) {
this.processInstanceId = JsonNullable.of(processInstanceId);
}
public ActivityInstanceDto processDefinitionId(String processDefinitionId) {
this.processDefinitionId = JsonNullable.of(processDefinitionId);
return this;
}
/**
* The id of the process definition.
* @return processDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProcessDefinitionId() {
return processDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessDefinitionId_JsonNullable() {
return processDefinitionId;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_ID)
public void setProcessDefinitionId_JsonNullable(JsonNullable processDefinitionId) {
this.processDefinitionId = processDefinitionId;
}
public void setProcessDefinitionId(String processDefinitionId) {
this.processDefinitionId = JsonNullable.of(processDefinitionId);
}
public ActivityInstanceDto childActivityInstances(List childActivityInstances) {
this.childActivityInstances = JsonNullable.>of(childActivityInstances);
return this;
}
public ActivityInstanceDto addChildActivityInstancesItem(ActivityInstanceDto childActivityInstancesItem) {
if (this.childActivityInstances == null || !this.childActivityInstances.isPresent()) {
this.childActivityInstances = JsonNullable.>of(new ArrayList<>());
}
try {
this.childActivityInstances.get().add(childActivityInstancesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* A list of child activity instances.
* @return childActivityInstances
**/
@javax.annotation.Nullable
@JsonIgnore
public List getChildActivityInstances() {
return childActivityInstances.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CHILD_ACTIVITY_INSTANCES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getChildActivityInstances_JsonNullable() {
return childActivityInstances;
}
@JsonProperty(JSON_PROPERTY_CHILD_ACTIVITY_INSTANCES)
public void setChildActivityInstances_JsonNullable(JsonNullable> childActivityInstances) {
this.childActivityInstances = childActivityInstances;
}
public void setChildActivityInstances(List childActivityInstances) {
this.childActivityInstances = JsonNullable.>of(childActivityInstances);
}
public ActivityInstanceDto childTransitionInstances(List childTransitionInstances) {
this.childTransitionInstances = JsonNullable.>of(childTransitionInstances);
return this;
}
public ActivityInstanceDto addChildTransitionInstancesItem(TransitionInstanceDto childTransitionInstancesItem) {
if (this.childTransitionInstances == null || !this.childTransitionInstances.isPresent()) {
this.childTransitionInstances = JsonNullable.>of(new ArrayList<>());
}
try {
this.childTransitionInstances.get().add(childTransitionInstancesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* A list of child transition instances. A transition instance represents an execution waiting in an asynchronous continuation.
* @return childTransitionInstances
**/
@javax.annotation.Nullable
@JsonIgnore
public List getChildTransitionInstances() {
return childTransitionInstances.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CHILD_TRANSITION_INSTANCES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getChildTransitionInstances_JsonNullable() {
return childTransitionInstances;
}
@JsonProperty(JSON_PROPERTY_CHILD_TRANSITION_INSTANCES)
public void setChildTransitionInstances_JsonNullable(JsonNullable> childTransitionInstances) {
this.childTransitionInstances = childTransitionInstances;
}
public void setChildTransitionInstances(List childTransitionInstances) {
this.childTransitionInstances = JsonNullable.>of(childTransitionInstances);
}
public ActivityInstanceDto executionIds(List executionIds) {
this.executionIds = JsonNullable.>of(executionIds);
return this;
}
public ActivityInstanceDto addExecutionIdsItem(String executionIdsItem) {
if (this.executionIds == null || !this.executionIds.isPresent()) {
this.executionIds = JsonNullable.>of(new ArrayList<>());
}
try {
this.executionIds.get().add(executionIdsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* A list of execution ids.
* @return executionIds
**/
@javax.annotation.Nullable
@JsonIgnore
public List getExecutionIds() {
return executionIds.orElse(null);
}
@JsonProperty(JSON_PROPERTY_EXECUTION_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getExecutionIds_JsonNullable() {
return executionIds;
}
@JsonProperty(JSON_PROPERTY_EXECUTION_IDS)
public void setExecutionIds_JsonNullable(JsonNullable> executionIds) {
this.executionIds = executionIds;
}
public void setExecutionIds(List executionIds) {
this.executionIds = JsonNullable.>of(executionIds);
}
public ActivityInstanceDto incidentIds(List incidentIds) {
this.incidentIds = JsonNullable.>of(incidentIds);
return this;
}
public ActivityInstanceDto addIncidentIdsItem(String incidentIdsItem) {
if (this.incidentIds == null || !this.incidentIds.isPresent()) {
this.incidentIds = JsonNullable.>of(new ArrayList<>());
}
try {
this.incidentIds.get().add(incidentIdsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* A list of incident ids.
* @return incidentIds
**/
@javax.annotation.Nullable
@JsonIgnore
public List getIncidentIds() {
return incidentIds.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INCIDENT_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getIncidentIds_JsonNullable() {
return incidentIds;
}
@JsonProperty(JSON_PROPERTY_INCIDENT_IDS)
public void setIncidentIds_JsonNullable(JsonNullable> incidentIds) {
this.incidentIds = incidentIds;
}
public void setIncidentIds(List incidentIds) {
this.incidentIds = JsonNullable.>of(incidentIds);
}
public ActivityInstanceDto incidents(List incidents) {
this.incidents = JsonNullable.>of(incidents);
return this;
}
public ActivityInstanceDto addIncidentsItem(ActivityInstanceIncidentDto incidentsItem) {
if (this.incidents == null || !this.incidents.isPresent()) {
this.incidents = JsonNullable.>of(new ArrayList<>());
}
try {
this.incidents.get().add(incidentsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* A list of JSON objects containing incident specific properties: * `id`: the id of the incident * `activityId`: the activity id in which the incident occurred
* @return incidents
**/
@javax.annotation.Nullable
@JsonIgnore
public List getIncidents() {
return incidents.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INCIDENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getIncidents_JsonNullable() {
return incidents;
}
@JsonProperty(JSON_PROPERTY_INCIDENTS)
public void setIncidents_JsonNullable(JsonNullable> incidents) {
this.incidents = incidents;
}
public void setIncidents(List incidents) {
this.incidents = JsonNullable.>of(incidents);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ActivityInstanceDto activityInstanceDto = (ActivityInstanceDto) o;
return equalsNullable(this.id, activityInstanceDto.id) &&
equalsNullable(this.parentActivityInstanceId, activityInstanceDto.parentActivityInstanceId) &&
equalsNullable(this.activityId, activityInstanceDto.activityId) &&
equalsNullable(this.activityName, activityInstanceDto.activityName) &&
equalsNullable(this.name, activityInstanceDto.name) &&
equalsNullable(this.activityType, activityInstanceDto.activityType) &&
equalsNullable(this.processInstanceId, activityInstanceDto.processInstanceId) &&
equalsNullable(this.processDefinitionId, activityInstanceDto.processDefinitionId) &&
equalsNullable(this.childActivityInstances, activityInstanceDto.childActivityInstances) &&
equalsNullable(this.childTransitionInstances, activityInstanceDto.childTransitionInstances) &&
equalsNullable(this.executionIds, activityInstanceDto.executionIds) &&
equalsNullable(this.incidentIds, activityInstanceDto.incidentIds) &&
equalsNullable(this.incidents, activityInstanceDto.incidents);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(id), hashCodeNullable(parentActivityInstanceId), hashCodeNullable(activityId), hashCodeNullable(activityName), hashCodeNullable(name), hashCodeNullable(activityType), hashCodeNullable(processInstanceId), hashCodeNullable(processDefinitionId), hashCodeNullable(childActivityInstances), hashCodeNullable(childTransitionInstances), hashCodeNullable(executionIds), hashCodeNullable(incidentIds), hashCodeNullable(incidents));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ActivityInstanceDto {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" parentActivityInstanceId: ").append(toIndentedString(parentActivityInstanceId)).append("\n");
sb.append(" activityId: ").append(toIndentedString(activityId)).append("\n");
sb.append(" activityName: ").append(toIndentedString(activityName)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" activityType: ").append(toIndentedString(activityType)).append("\n");
sb.append(" processInstanceId: ").append(toIndentedString(processInstanceId)).append("\n");
sb.append(" processDefinitionId: ").append(toIndentedString(processDefinitionId)).append("\n");
sb.append(" childActivityInstances: ").append(toIndentedString(childActivityInstances)).append("\n");
sb.append(" childTransitionInstances: ").append(toIndentedString(childTransitionInstances)).append("\n");
sb.append(" executionIds: ").append(toIndentedString(executionIds)).append("\n");
sb.append(" incidentIds: ").append(toIndentedString(incidentIds)).append("\n");
sb.append(" incidents: ").append(toIndentedString(incidents)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `id` to the URL query string
if (getId() != null) {
try {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `parentActivityInstanceId` to the URL query string
if (getParentActivityInstanceId() != null) {
try {
joiner.add(String.format("%sparentActivityInstanceId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getParentActivityInstanceId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `activityId` to the URL query string
if (getActivityId() != null) {
try {
joiner.add(String.format("%sactivityId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getActivityId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `activityName` to the URL query string
if (getActivityName() != null) {
try {
joiner.add(String.format("%sactivityName%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getActivityName()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `name` to the URL query string
if (getName() != null) {
try {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getName()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `activityType` to the URL query string
if (getActivityType() != null) {
try {
joiner.add(String.format("%sactivityType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getActivityType()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processInstanceId` to the URL query string
if (getProcessInstanceId() != null) {
try {
joiner.add(String.format("%sprocessInstanceId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessInstanceId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processDefinitionId` to the URL query string
if (getProcessDefinitionId() != null) {
try {
joiner.add(String.format("%sprocessDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `childActivityInstances` to the URL query string
if (getChildActivityInstances() != null) {
for (int i = 0; i < getChildActivityInstances().size(); i++) {
if (getChildActivityInstances().get(i) != null) {
joiner.add(getChildActivityInstances().get(i).toUrlQueryString(String.format("%schildActivityInstances%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `childTransitionInstances` to the URL query string
if (getChildTransitionInstances() != null) {
for (int i = 0; i < getChildTransitionInstances().size(); i++) {
if (getChildTransitionInstances().get(i) != null) {
joiner.add(getChildTransitionInstances().get(i).toUrlQueryString(String.format("%schildTransitionInstances%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `executionIds` to the URL query string
if (getExecutionIds() != null) {
for (int i = 0; i < getExecutionIds().size(); i++) {
try {
joiner.add(String.format("%sexecutionIds%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getExecutionIds().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `incidentIds` to the URL query string
if (getIncidentIds() != null) {
for (int i = 0; i < getIncidentIds().size(); i++) {
try {
joiner.add(String.format("%sincidentIds%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getIncidentIds().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `incidents` to the URL query string
if (getIncidents() != null) {
for (int i = 0; i < getIncidents().size(); i++) {
if (getIncidents().get(i) != null) {
joiner.add(getIncidents().get(i).toUrlQueryString(String.format("%sincidents%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy