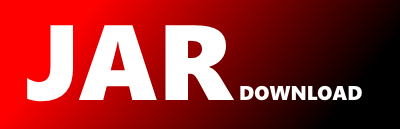
org.camunda.community.rest.client.dto.AuthenticationResult Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.dto;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* AuthenticationResult
*/
@JsonPropertyOrder({
AuthenticationResult.JSON_PROPERTY_AUTHENTICATED_USER,
AuthenticationResult.JSON_PROPERTY_AUTHENTICATED,
AuthenticationResult.JSON_PROPERTY_TENANTS,
AuthenticationResult.JSON_PROPERTY_GROUPS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class AuthenticationResult {
public static final String JSON_PROPERTY_AUTHENTICATED_USER = "authenticatedUser";
private JsonNullable authenticatedUser = JsonNullable.undefined();
public static final String JSON_PROPERTY_AUTHENTICATED = "authenticated";
private JsonNullable authenticated = JsonNullable.undefined();
public static final String JSON_PROPERTY_TENANTS = "tenants";
private JsonNullable> tenants = JsonNullable.>undefined();
public static final String JSON_PROPERTY_GROUPS = "groups";
private JsonNullable> groups = JsonNullable.>undefined();
public AuthenticationResult() {
}
public AuthenticationResult authenticatedUser(String authenticatedUser) {
this.authenticatedUser = JsonNullable.of(authenticatedUser);
return this;
}
/**
* An id of authenticated user.
* @return authenticatedUser
**/
@javax.annotation.Nullable
@JsonIgnore
public String getAuthenticatedUser() {
return authenticatedUser.orElse(null);
}
@JsonProperty(JSON_PROPERTY_AUTHENTICATED_USER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getAuthenticatedUser_JsonNullable() {
return authenticatedUser;
}
@JsonProperty(JSON_PROPERTY_AUTHENTICATED_USER)
public void setAuthenticatedUser_JsonNullable(JsonNullable authenticatedUser) {
this.authenticatedUser = authenticatedUser;
}
public void setAuthenticatedUser(String authenticatedUser) {
this.authenticatedUser = JsonNullable.of(authenticatedUser);
}
public AuthenticationResult authenticated(Boolean authenticated) {
this.authenticated = JsonNullable.of(authenticated);
return this;
}
/**
* A flag indicating if user is authenticated.
* @return authenticated
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getAuthenticated() {
return authenticated.orElse(null);
}
@JsonProperty(JSON_PROPERTY_AUTHENTICATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getAuthenticated_JsonNullable() {
return authenticated;
}
@JsonProperty(JSON_PROPERTY_AUTHENTICATED)
public void setAuthenticated_JsonNullable(JsonNullable authenticated) {
this.authenticated = authenticated;
}
public void setAuthenticated(Boolean authenticated) {
this.authenticated = JsonNullable.of(authenticated);
}
public AuthenticationResult tenants(List tenants) {
this.tenants = JsonNullable.>of(tenants);
return this;
}
public AuthenticationResult addTenantsItem(String tenantsItem) {
if (this.tenants == null || !this.tenants.isPresent()) {
this.tenants = JsonNullable.>of(new ArrayList<>());
}
try {
this.tenants.get().add(tenantsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Will be null.
* @return tenants
**/
@javax.annotation.Nullable
@JsonIgnore
public List getTenants() {
return tenants.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TENANTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getTenants_JsonNullable() {
return tenants;
}
@JsonProperty(JSON_PROPERTY_TENANTS)
public void setTenants_JsonNullable(JsonNullable> tenants) {
this.tenants = tenants;
}
public void setTenants(List tenants) {
this.tenants = JsonNullable.>of(tenants);
}
public AuthenticationResult groups(List groups) {
this.groups = JsonNullable.>of(groups);
return this;
}
public AuthenticationResult addGroupsItem(String groupsItem) {
if (this.groups == null || !this.groups.isPresent()) {
this.groups = JsonNullable.>of(new ArrayList<>());
}
try {
this.groups.get().add(groupsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Will be null.
* @return groups
**/
@javax.annotation.Nullable
@JsonIgnore
public List getGroups() {
return groups.orElse(null);
}
@JsonProperty(JSON_PROPERTY_GROUPS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getGroups_JsonNullable() {
return groups;
}
@JsonProperty(JSON_PROPERTY_GROUPS)
public void setGroups_JsonNullable(JsonNullable> groups) {
this.groups = groups;
}
public void setGroups(List groups) {
this.groups = JsonNullable.>of(groups);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AuthenticationResult authenticationResult = (AuthenticationResult) o;
return equalsNullable(this.authenticatedUser, authenticationResult.authenticatedUser) &&
equalsNullable(this.authenticated, authenticationResult.authenticated) &&
equalsNullable(this.tenants, authenticationResult.tenants) &&
equalsNullable(this.groups, authenticationResult.groups);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(authenticatedUser), hashCodeNullable(authenticated), hashCodeNullable(tenants), hashCodeNullable(groups));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AuthenticationResult {\n");
sb.append(" authenticatedUser: ").append(toIndentedString(authenticatedUser)).append("\n");
sb.append(" authenticated: ").append(toIndentedString(authenticated)).append("\n");
sb.append(" tenants: ").append(toIndentedString(tenants)).append("\n");
sb.append(" groups: ").append(toIndentedString(groups)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `authenticatedUser` to the URL query string
if (getAuthenticatedUser() != null) {
try {
joiner.add(String.format("%sauthenticatedUser%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAuthenticatedUser()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `authenticated` to the URL query string
if (getAuthenticated() != null) {
try {
joiner.add(String.format("%sauthenticated%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAuthenticated()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `tenants` to the URL query string
if (getTenants() != null) {
for (int i = 0; i < getTenants().size(); i++) {
try {
joiner.add(String.format("%stenants%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getTenants().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `groups` to the URL query string
if (getGroups() != null) {
for (int i = 0; i < getGroups().size(); i++) {
try {
joiner.add(String.format("%sgroups%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getGroups().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy