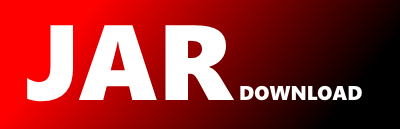
org.camunda.community.rest.client.dto.BatchDto Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.dto;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* BatchDto
*/
@JsonPropertyOrder({
BatchDto.JSON_PROPERTY_ID,
BatchDto.JSON_PROPERTY_TYPE,
BatchDto.JSON_PROPERTY_TOTAL_JOBS,
BatchDto.JSON_PROPERTY_JOBS_CREATED,
BatchDto.JSON_PROPERTY_BATCH_JOBS_PER_SEED,
BatchDto.JSON_PROPERTY_INVOCATIONS_PER_BATCH_JOB,
BatchDto.JSON_PROPERTY_SEED_JOB_DEFINITION_ID,
BatchDto.JSON_PROPERTY_MONITOR_JOB_DEFINITION_ID,
BatchDto.JSON_PROPERTY_BATCH_JOB_DEFINITION_ID,
BatchDto.JSON_PROPERTY_SUSPENDED,
BatchDto.JSON_PROPERTY_TENANT_ID,
BatchDto.JSON_PROPERTY_CREATE_USER_ID,
BatchDto.JSON_PROPERTY_START_TIME,
BatchDto.JSON_PROPERTY_EXECUTION_START_TIME
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class BatchDto {
public static final String JSON_PROPERTY_ID = "id";
private JsonNullable id = JsonNullable.undefined();
public static final String JSON_PROPERTY_TYPE = "type";
private JsonNullable type = JsonNullable.undefined();
public static final String JSON_PROPERTY_TOTAL_JOBS = "totalJobs";
private JsonNullable totalJobs = JsonNullable.undefined();
public static final String JSON_PROPERTY_JOBS_CREATED = "jobsCreated";
private JsonNullable jobsCreated = JsonNullable.undefined();
public static final String JSON_PROPERTY_BATCH_JOBS_PER_SEED = "batchJobsPerSeed";
private JsonNullable batchJobsPerSeed = JsonNullable.undefined();
public static final String JSON_PROPERTY_INVOCATIONS_PER_BATCH_JOB = "invocationsPerBatchJob";
private JsonNullable invocationsPerBatchJob = JsonNullable.undefined();
public static final String JSON_PROPERTY_SEED_JOB_DEFINITION_ID = "seedJobDefinitionId";
private JsonNullable seedJobDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_MONITOR_JOB_DEFINITION_ID = "monitorJobDefinitionId";
private JsonNullable monitorJobDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_BATCH_JOB_DEFINITION_ID = "batchJobDefinitionId";
private JsonNullable batchJobDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_SUSPENDED = "suspended";
private JsonNullable suspended = JsonNullable.undefined();
public static final String JSON_PROPERTY_TENANT_ID = "tenantId";
private JsonNullable tenantId = JsonNullable.undefined();
public static final String JSON_PROPERTY_CREATE_USER_ID = "createUserId";
private JsonNullable createUserId = JsonNullable.undefined();
public static final String JSON_PROPERTY_START_TIME = "startTime";
private JsonNullable startTime = JsonNullable.undefined();
public static final String JSON_PROPERTY_EXECUTION_START_TIME = "executionStartTime";
private JsonNullable executionStartTime = JsonNullable.undefined();
public BatchDto() {
}
public BatchDto id(String id) {
this.id = JsonNullable.of(id);
return this;
}
/**
* The id of the batch.
* @return id
**/
@javax.annotation.Nullable
@JsonIgnore
public String getId() {
return id.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getId_JsonNullable() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
public void setId_JsonNullable(JsonNullable id) {
this.id = id;
}
public void setId(String id) {
this.id = JsonNullable.of(id);
}
public BatchDto type(String type) {
this.type = JsonNullable.of(type);
return this;
}
/**
* The type of the batch. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/batch/#creating-a-batch) for more information about batch types.
* @return type
**/
@javax.annotation.Nullable
@JsonIgnore
public String getType() {
return type.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getType_JsonNullable() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
public void setType_JsonNullable(JsonNullable type) {
this.type = type;
}
public void setType(String type) {
this.type = JsonNullable.of(type);
}
public BatchDto totalJobs(Integer totalJobs) {
this.totalJobs = JsonNullable.of(totalJobs);
return this;
}
/**
* The total jobs of a batch is the number of batch execution jobs required to complete the batch.
* @return totalJobs
**/
@javax.annotation.Nullable
@JsonIgnore
public Integer getTotalJobs() {
return totalJobs.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TOTAL_JOBS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getTotalJobs_JsonNullable() {
return totalJobs;
}
@JsonProperty(JSON_PROPERTY_TOTAL_JOBS)
public void setTotalJobs_JsonNullable(JsonNullable totalJobs) {
this.totalJobs = totalJobs;
}
public void setTotalJobs(Integer totalJobs) {
this.totalJobs = JsonNullable.of(totalJobs);
}
public BatchDto jobsCreated(Integer jobsCreated) {
this.jobsCreated = JsonNullable.of(jobsCreated);
return this;
}
/**
* The number of batch execution jobs already created by the seed job.
* @return jobsCreated
**/
@javax.annotation.Nullable
@JsonIgnore
public Integer getJobsCreated() {
return jobsCreated.orElse(null);
}
@JsonProperty(JSON_PROPERTY_JOBS_CREATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getJobsCreated_JsonNullable() {
return jobsCreated;
}
@JsonProperty(JSON_PROPERTY_JOBS_CREATED)
public void setJobsCreated_JsonNullable(JsonNullable jobsCreated) {
this.jobsCreated = jobsCreated;
}
public void setJobsCreated(Integer jobsCreated) {
this.jobsCreated = JsonNullable.of(jobsCreated);
}
public BatchDto batchJobsPerSeed(Integer batchJobsPerSeed) {
this.batchJobsPerSeed = JsonNullable.of(batchJobsPerSeed);
return this;
}
/**
* The number of batch execution jobs created per seed job invocation. The batch seed job is invoked until it has created all batch execution jobs required by the batch (see `totalJobs` property).
* @return batchJobsPerSeed
**/
@javax.annotation.Nullable
@JsonIgnore
public Integer getBatchJobsPerSeed() {
return batchJobsPerSeed.orElse(null);
}
@JsonProperty(JSON_PROPERTY_BATCH_JOBS_PER_SEED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getBatchJobsPerSeed_JsonNullable() {
return batchJobsPerSeed;
}
@JsonProperty(JSON_PROPERTY_BATCH_JOBS_PER_SEED)
public void setBatchJobsPerSeed_JsonNullable(JsonNullable batchJobsPerSeed) {
this.batchJobsPerSeed = batchJobsPerSeed;
}
public void setBatchJobsPerSeed(Integer batchJobsPerSeed) {
this.batchJobsPerSeed = JsonNullable.of(batchJobsPerSeed);
}
public BatchDto invocationsPerBatchJob(Integer invocationsPerBatchJob) {
this.invocationsPerBatchJob = JsonNullable.of(invocationsPerBatchJob);
return this;
}
/**
* Every batch execution job invokes the command executed by the batch `invocationsPerBatchJob` times. E.g., for a process instance migration batch this specifies the number of process instances which are migrated per batch execution job.
* @return invocationsPerBatchJob
**/
@javax.annotation.Nullable
@JsonIgnore
public Integer getInvocationsPerBatchJob() {
return invocationsPerBatchJob.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INVOCATIONS_PER_BATCH_JOB)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getInvocationsPerBatchJob_JsonNullable() {
return invocationsPerBatchJob;
}
@JsonProperty(JSON_PROPERTY_INVOCATIONS_PER_BATCH_JOB)
public void setInvocationsPerBatchJob_JsonNullable(JsonNullable invocationsPerBatchJob) {
this.invocationsPerBatchJob = invocationsPerBatchJob;
}
public void setInvocationsPerBatchJob(Integer invocationsPerBatchJob) {
this.invocationsPerBatchJob = JsonNullable.of(invocationsPerBatchJob);
}
public BatchDto seedJobDefinitionId(String seedJobDefinitionId) {
this.seedJobDefinitionId = JsonNullable.of(seedJobDefinitionId);
return this;
}
/**
* The job definition id for the seed jobs of this batch.
* @return seedJobDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getSeedJobDefinitionId() {
return seedJobDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SEED_JOB_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSeedJobDefinitionId_JsonNullable() {
return seedJobDefinitionId;
}
@JsonProperty(JSON_PROPERTY_SEED_JOB_DEFINITION_ID)
public void setSeedJobDefinitionId_JsonNullable(JsonNullable seedJobDefinitionId) {
this.seedJobDefinitionId = seedJobDefinitionId;
}
public void setSeedJobDefinitionId(String seedJobDefinitionId) {
this.seedJobDefinitionId = JsonNullable.of(seedJobDefinitionId);
}
public BatchDto monitorJobDefinitionId(String monitorJobDefinitionId) {
this.monitorJobDefinitionId = JsonNullable.of(monitorJobDefinitionId);
return this;
}
/**
* The job definition id for the monitor jobs of this batch.
* @return monitorJobDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getMonitorJobDefinitionId() {
return monitorJobDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_MONITOR_JOB_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getMonitorJobDefinitionId_JsonNullable() {
return monitorJobDefinitionId;
}
@JsonProperty(JSON_PROPERTY_MONITOR_JOB_DEFINITION_ID)
public void setMonitorJobDefinitionId_JsonNullable(JsonNullable monitorJobDefinitionId) {
this.monitorJobDefinitionId = monitorJobDefinitionId;
}
public void setMonitorJobDefinitionId(String monitorJobDefinitionId) {
this.monitorJobDefinitionId = JsonNullable.of(monitorJobDefinitionId);
}
public BatchDto batchJobDefinitionId(String batchJobDefinitionId) {
this.batchJobDefinitionId = JsonNullable.of(batchJobDefinitionId);
return this;
}
/**
* The job definition id for the batch execution jobs of this batch.
* @return batchJobDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getBatchJobDefinitionId() {
return batchJobDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_BATCH_JOB_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getBatchJobDefinitionId_JsonNullable() {
return batchJobDefinitionId;
}
@JsonProperty(JSON_PROPERTY_BATCH_JOB_DEFINITION_ID)
public void setBatchJobDefinitionId_JsonNullable(JsonNullable batchJobDefinitionId) {
this.batchJobDefinitionId = batchJobDefinitionId;
}
public void setBatchJobDefinitionId(String batchJobDefinitionId) {
this.batchJobDefinitionId = JsonNullable.of(batchJobDefinitionId);
}
public BatchDto suspended(Boolean suspended) {
this.suspended = JsonNullable.of(suspended);
return this;
}
/**
* Indicates whether this batch is suspended or not.
* @return suspended
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getSuspended() {
return suspended.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SUSPENDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSuspended_JsonNullable() {
return suspended;
}
@JsonProperty(JSON_PROPERTY_SUSPENDED)
public void setSuspended_JsonNullable(JsonNullable suspended) {
this.suspended = suspended;
}
public void setSuspended(Boolean suspended) {
this.suspended = JsonNullable.of(suspended);
}
public BatchDto tenantId(String tenantId) {
this.tenantId = JsonNullable.of(tenantId);
return this;
}
/**
* The tenant id of the batch.
* @return tenantId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getTenantId() {
return tenantId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TENANT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getTenantId_JsonNullable() {
return tenantId;
}
@JsonProperty(JSON_PROPERTY_TENANT_ID)
public void setTenantId_JsonNullable(JsonNullable tenantId) {
this.tenantId = tenantId;
}
public void setTenantId(String tenantId) {
this.tenantId = JsonNullable.of(tenantId);
}
public BatchDto createUserId(String createUserId) {
this.createUserId = JsonNullable.of(createUserId);
return this;
}
/**
* The id of the user that created the batch.
* @return createUserId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getCreateUserId() {
return createUserId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CREATE_USER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCreateUserId_JsonNullable() {
return createUserId;
}
@JsonProperty(JSON_PROPERTY_CREATE_USER_ID)
public void setCreateUserId_JsonNullable(JsonNullable createUserId) {
this.createUserId = createUserId;
}
public void setCreateUserId(String createUserId) {
this.createUserId = JsonNullable.of(createUserId);
}
public BatchDto startTime(OffsetDateTime startTime) {
this.startTime = JsonNullable.of(startTime);
return this;
}
/**
* The time the batch was started. Default format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. For further information, please see the [documentation] (https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/)
* @return startTime
**/
@javax.annotation.Nullable
@JsonIgnore
public OffsetDateTime getStartTime() {
return startTime.orElse(null);
}
@JsonProperty(JSON_PROPERTY_START_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getStartTime_JsonNullable() {
return startTime;
}
@JsonProperty(JSON_PROPERTY_START_TIME)
public void setStartTime_JsonNullable(JsonNullable startTime) {
this.startTime = startTime;
}
public void setStartTime(OffsetDateTime startTime) {
this.startTime = JsonNullable.of(startTime);
}
public BatchDto executionStartTime(OffsetDateTime executionStartTime) {
this.executionStartTime = JsonNullable.of(executionStartTime);
return this;
}
/**
* The time the batch execution was started, i.e., at least one batch job has been executed. Default format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. For further information, please see the [documentation] (https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/)
* @return executionStartTime
**/
@javax.annotation.Nullable
@JsonIgnore
public OffsetDateTime getExecutionStartTime() {
return executionStartTime.orElse(null);
}
@JsonProperty(JSON_PROPERTY_EXECUTION_START_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getExecutionStartTime_JsonNullable() {
return executionStartTime;
}
@JsonProperty(JSON_PROPERTY_EXECUTION_START_TIME)
public void setExecutionStartTime_JsonNullable(JsonNullable executionStartTime) {
this.executionStartTime = executionStartTime;
}
public void setExecutionStartTime(OffsetDateTime executionStartTime) {
this.executionStartTime = JsonNullable.of(executionStartTime);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BatchDto batchDto = (BatchDto) o;
return equalsNullable(this.id, batchDto.id) &&
equalsNullable(this.type, batchDto.type) &&
equalsNullable(this.totalJobs, batchDto.totalJobs) &&
equalsNullable(this.jobsCreated, batchDto.jobsCreated) &&
equalsNullable(this.batchJobsPerSeed, batchDto.batchJobsPerSeed) &&
equalsNullable(this.invocationsPerBatchJob, batchDto.invocationsPerBatchJob) &&
equalsNullable(this.seedJobDefinitionId, batchDto.seedJobDefinitionId) &&
equalsNullable(this.monitorJobDefinitionId, batchDto.monitorJobDefinitionId) &&
equalsNullable(this.batchJobDefinitionId, batchDto.batchJobDefinitionId) &&
equalsNullable(this.suspended, batchDto.suspended) &&
equalsNullable(this.tenantId, batchDto.tenantId) &&
equalsNullable(this.createUserId, batchDto.createUserId) &&
equalsNullable(this.startTime, batchDto.startTime) &&
equalsNullable(this.executionStartTime, batchDto.executionStartTime);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(id), hashCodeNullable(type), hashCodeNullable(totalJobs), hashCodeNullable(jobsCreated), hashCodeNullable(batchJobsPerSeed), hashCodeNullable(invocationsPerBatchJob), hashCodeNullable(seedJobDefinitionId), hashCodeNullable(monitorJobDefinitionId), hashCodeNullable(batchJobDefinitionId), hashCodeNullable(suspended), hashCodeNullable(tenantId), hashCodeNullable(createUserId), hashCodeNullable(startTime), hashCodeNullable(executionStartTime));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BatchDto {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" totalJobs: ").append(toIndentedString(totalJobs)).append("\n");
sb.append(" jobsCreated: ").append(toIndentedString(jobsCreated)).append("\n");
sb.append(" batchJobsPerSeed: ").append(toIndentedString(batchJobsPerSeed)).append("\n");
sb.append(" invocationsPerBatchJob: ").append(toIndentedString(invocationsPerBatchJob)).append("\n");
sb.append(" seedJobDefinitionId: ").append(toIndentedString(seedJobDefinitionId)).append("\n");
sb.append(" monitorJobDefinitionId: ").append(toIndentedString(monitorJobDefinitionId)).append("\n");
sb.append(" batchJobDefinitionId: ").append(toIndentedString(batchJobDefinitionId)).append("\n");
sb.append(" suspended: ").append(toIndentedString(suspended)).append("\n");
sb.append(" tenantId: ").append(toIndentedString(tenantId)).append("\n");
sb.append(" createUserId: ").append(toIndentedString(createUserId)).append("\n");
sb.append(" startTime: ").append(toIndentedString(startTime)).append("\n");
sb.append(" executionStartTime: ").append(toIndentedString(executionStartTime)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `id` to the URL query string
if (getId() != null) {
try {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `type` to the URL query string
if (getType() != null) {
try {
joiner.add(String.format("%stype%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getType()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `totalJobs` to the URL query string
if (getTotalJobs() != null) {
try {
joiner.add(String.format("%stotalJobs%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTotalJobs()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `jobsCreated` to the URL query string
if (getJobsCreated() != null) {
try {
joiner.add(String.format("%sjobsCreated%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getJobsCreated()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `batchJobsPerSeed` to the URL query string
if (getBatchJobsPerSeed() != null) {
try {
joiner.add(String.format("%sbatchJobsPerSeed%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getBatchJobsPerSeed()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `invocationsPerBatchJob` to the URL query string
if (getInvocationsPerBatchJob() != null) {
try {
joiner.add(String.format("%sinvocationsPerBatchJob%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getInvocationsPerBatchJob()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `seedJobDefinitionId` to the URL query string
if (getSeedJobDefinitionId() != null) {
try {
joiner.add(String.format("%sseedJobDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSeedJobDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `monitorJobDefinitionId` to the URL query string
if (getMonitorJobDefinitionId() != null) {
try {
joiner.add(String.format("%smonitorJobDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getMonitorJobDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `batchJobDefinitionId` to the URL query string
if (getBatchJobDefinitionId() != null) {
try {
joiner.add(String.format("%sbatchJobDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getBatchJobDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `suspended` to the URL query string
if (getSuspended() != null) {
try {
joiner.add(String.format("%ssuspended%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSuspended()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `tenantId` to the URL query string
if (getTenantId() != null) {
try {
joiner.add(String.format("%stenantId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTenantId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `createUserId` to the URL query string
if (getCreateUserId() != null) {
try {
joiner.add(String.format("%screateUserId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCreateUserId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `startTime` to the URL query string
if (getStartTime() != null) {
try {
joiner.add(String.format("%sstartTime%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getStartTime()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `executionStartTime` to the URL query string
if (getExecutionStartTime() != null) {
try {
joiner.add(String.format("%sexecutionStartTime%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getExecutionStartTime()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy