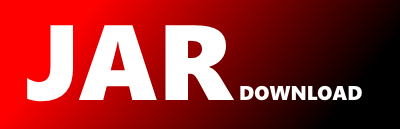
org.camunda.community.rest.client.dto.CompleteExternalTaskDto Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.dto;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.HashMap;
import java.util.Map;
import org.camunda.community.rest.client.dto.VariableValueDto;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* CompleteExternalTaskDto
*/
@JsonPropertyOrder({
CompleteExternalTaskDto.JSON_PROPERTY_WORKER_ID,
CompleteExternalTaskDto.JSON_PROPERTY_VARIABLES,
CompleteExternalTaskDto.JSON_PROPERTY_LOCAL_VARIABLES
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class CompleteExternalTaskDto {
public static final String JSON_PROPERTY_WORKER_ID = "workerId";
private String workerId;
public static final String JSON_PROPERTY_VARIABLES = "variables";
private JsonNullable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy