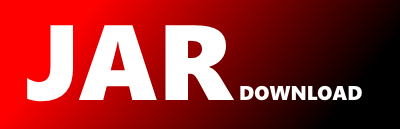
org.camunda.community.rest.client.dto.IncidentDto Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.dto;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* IncidentDto
*/
@JsonPropertyOrder({
IncidentDto.JSON_PROPERTY_ID,
IncidentDto.JSON_PROPERTY_PROCESS_DEFINITION_ID,
IncidentDto.JSON_PROPERTY_PROCESS_INSTANCE_ID,
IncidentDto.JSON_PROPERTY_EXECUTION_ID,
IncidentDto.JSON_PROPERTY_INCIDENT_TIMESTAMP,
IncidentDto.JSON_PROPERTY_INCIDENT_TYPE,
IncidentDto.JSON_PROPERTY_ACTIVITY_ID,
IncidentDto.JSON_PROPERTY_FAILED_ACTIVITY_ID,
IncidentDto.JSON_PROPERTY_CAUSE_INCIDENT_ID,
IncidentDto.JSON_PROPERTY_ROOT_CAUSE_INCIDENT_ID,
IncidentDto.JSON_PROPERTY_CONFIGURATION,
IncidentDto.JSON_PROPERTY_TENANT_ID,
IncidentDto.JSON_PROPERTY_INCIDENT_MESSAGE,
IncidentDto.JSON_PROPERTY_JOB_DEFINITION_ID,
IncidentDto.JSON_PROPERTY_ANNOTATION
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class IncidentDto {
public static final String JSON_PROPERTY_ID = "id";
private JsonNullable id = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_DEFINITION_ID = "processDefinitionId";
private JsonNullable processDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_INSTANCE_ID = "processInstanceId";
private JsonNullable processInstanceId = JsonNullable.undefined();
public static final String JSON_PROPERTY_EXECUTION_ID = "executionId";
private JsonNullable executionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_INCIDENT_TIMESTAMP = "incidentTimestamp";
private JsonNullable incidentTimestamp = JsonNullable.undefined();
public static final String JSON_PROPERTY_INCIDENT_TYPE = "incidentType";
private JsonNullable incidentType = JsonNullable.undefined();
public static final String JSON_PROPERTY_ACTIVITY_ID = "activityId";
private JsonNullable activityId = JsonNullable.undefined();
public static final String JSON_PROPERTY_FAILED_ACTIVITY_ID = "failedActivityId";
private JsonNullable failedActivityId = JsonNullable.undefined();
public static final String JSON_PROPERTY_CAUSE_INCIDENT_ID = "causeIncidentId";
private JsonNullable causeIncidentId = JsonNullable.undefined();
public static final String JSON_PROPERTY_ROOT_CAUSE_INCIDENT_ID = "rootCauseIncidentId";
private JsonNullable rootCauseIncidentId = JsonNullable.undefined();
public static final String JSON_PROPERTY_CONFIGURATION = "configuration";
private JsonNullable _configuration = JsonNullable.undefined();
public static final String JSON_PROPERTY_TENANT_ID = "tenantId";
private JsonNullable tenantId = JsonNullable.undefined();
public static final String JSON_PROPERTY_INCIDENT_MESSAGE = "incidentMessage";
private JsonNullable incidentMessage = JsonNullable.undefined();
public static final String JSON_PROPERTY_JOB_DEFINITION_ID = "jobDefinitionId";
private JsonNullable jobDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_ANNOTATION = "annotation";
private JsonNullable annotation = JsonNullable.undefined();
public IncidentDto() {
}
public IncidentDto id(String id) {
this.id = JsonNullable.of(id);
return this;
}
/**
* The id of the incident.
* @return id
**/
@javax.annotation.Nullable
@JsonIgnore
public String getId() {
return id.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getId_JsonNullable() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
public void setId_JsonNullable(JsonNullable id) {
this.id = id;
}
public void setId(String id) {
this.id = JsonNullable.of(id);
}
public IncidentDto processDefinitionId(String processDefinitionId) {
this.processDefinitionId = JsonNullable.of(processDefinitionId);
return this;
}
/**
* The id of the process definition this incident is associated with.
* @return processDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProcessDefinitionId() {
return processDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessDefinitionId_JsonNullable() {
return processDefinitionId;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_ID)
public void setProcessDefinitionId_JsonNullable(JsonNullable processDefinitionId) {
this.processDefinitionId = processDefinitionId;
}
public void setProcessDefinitionId(String processDefinitionId) {
this.processDefinitionId = JsonNullable.of(processDefinitionId);
}
public IncidentDto processInstanceId(String processInstanceId) {
this.processInstanceId = JsonNullable.of(processInstanceId);
return this;
}
/**
* The id of the process instance this incident is associated with.
* @return processInstanceId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProcessInstanceId() {
return processInstanceId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessInstanceId_JsonNullable() {
return processInstanceId;
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_ID)
public void setProcessInstanceId_JsonNullable(JsonNullable processInstanceId) {
this.processInstanceId = processInstanceId;
}
public void setProcessInstanceId(String processInstanceId) {
this.processInstanceId = JsonNullable.of(processInstanceId);
}
public IncidentDto executionId(String executionId) {
this.executionId = JsonNullable.of(executionId);
return this;
}
/**
* The id of the execution this incident is associated with.
* @return executionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getExecutionId() {
return executionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_EXECUTION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getExecutionId_JsonNullable() {
return executionId;
}
@JsonProperty(JSON_PROPERTY_EXECUTION_ID)
public void setExecutionId_JsonNullable(JsonNullable executionId) {
this.executionId = executionId;
}
public void setExecutionId(String executionId) {
this.executionId = JsonNullable.of(executionId);
}
public IncidentDto incidentTimestamp(OffsetDateTime incidentTimestamp) {
this.incidentTimestamp = JsonNullable.of(incidentTimestamp);
return this;
}
/**
* The time this incident happened. By [default](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/), the date must have the format `yyyy-MM-dd'T'HH:mm:ss.SSSZ`, e.g., `2013-01-23T14:42:45.000+0200`.
* @return incidentTimestamp
**/
@javax.annotation.Nullable
@JsonIgnore
public OffsetDateTime getIncidentTimestamp() {
return incidentTimestamp.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INCIDENT_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getIncidentTimestamp_JsonNullable() {
return incidentTimestamp;
}
@JsonProperty(JSON_PROPERTY_INCIDENT_TIMESTAMP)
public void setIncidentTimestamp_JsonNullable(JsonNullable incidentTimestamp) {
this.incidentTimestamp = incidentTimestamp;
}
public void setIncidentTimestamp(OffsetDateTime incidentTimestamp) {
this.incidentTimestamp = JsonNullable.of(incidentTimestamp);
}
public IncidentDto incidentType(String incidentType) {
this.incidentType = JsonNullable.of(incidentType);
return this;
}
/**
* The type of incident, for example: `failedJobs` will be returned in case of an incident which identified a failed job during the execution of a process instance. See the [User Guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/incidents/#incident-types) for a list of incident types.
* @return incidentType
**/
@javax.annotation.Nullable
@JsonIgnore
public String getIncidentType() {
return incidentType.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INCIDENT_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getIncidentType_JsonNullable() {
return incidentType;
}
@JsonProperty(JSON_PROPERTY_INCIDENT_TYPE)
public void setIncidentType_JsonNullable(JsonNullable incidentType) {
this.incidentType = incidentType;
}
public void setIncidentType(String incidentType) {
this.incidentType = JsonNullable.of(incidentType);
}
public IncidentDto activityId(String activityId) {
this.activityId = JsonNullable.of(activityId);
return this;
}
/**
* The id of the activity this incident is associated with.
* @return activityId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getActivityId() {
return activityId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getActivityId_JsonNullable() {
return activityId;
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_ID)
public void setActivityId_JsonNullable(JsonNullable activityId) {
this.activityId = activityId;
}
public void setActivityId(String activityId) {
this.activityId = JsonNullable.of(activityId);
}
public IncidentDto failedActivityId(String failedActivityId) {
this.failedActivityId = JsonNullable.of(failedActivityId);
return this;
}
/**
* The id of the activity on which the last exception occurred.
* @return failedActivityId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getFailedActivityId() {
return failedActivityId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_FAILED_ACTIVITY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getFailedActivityId_JsonNullable() {
return failedActivityId;
}
@JsonProperty(JSON_PROPERTY_FAILED_ACTIVITY_ID)
public void setFailedActivityId_JsonNullable(JsonNullable failedActivityId) {
this.failedActivityId = failedActivityId;
}
public void setFailedActivityId(String failedActivityId) {
this.failedActivityId = JsonNullable.of(failedActivityId);
}
public IncidentDto causeIncidentId(String causeIncidentId) {
this.causeIncidentId = JsonNullable.of(causeIncidentId);
return this;
}
/**
* The id of the associated cause incident which has been triggered.
* @return causeIncidentId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getCauseIncidentId() {
return causeIncidentId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CAUSE_INCIDENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCauseIncidentId_JsonNullable() {
return causeIncidentId;
}
@JsonProperty(JSON_PROPERTY_CAUSE_INCIDENT_ID)
public void setCauseIncidentId_JsonNullable(JsonNullable causeIncidentId) {
this.causeIncidentId = causeIncidentId;
}
public void setCauseIncidentId(String causeIncidentId) {
this.causeIncidentId = JsonNullable.of(causeIncidentId);
}
public IncidentDto rootCauseIncidentId(String rootCauseIncidentId) {
this.rootCauseIncidentId = JsonNullable.of(rootCauseIncidentId);
return this;
}
/**
* The id of the associated root cause incident which has been triggered.
* @return rootCauseIncidentId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getRootCauseIncidentId() {
return rootCauseIncidentId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ROOT_CAUSE_INCIDENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getRootCauseIncidentId_JsonNullable() {
return rootCauseIncidentId;
}
@JsonProperty(JSON_PROPERTY_ROOT_CAUSE_INCIDENT_ID)
public void setRootCauseIncidentId_JsonNullable(JsonNullable rootCauseIncidentId) {
this.rootCauseIncidentId = rootCauseIncidentId;
}
public void setRootCauseIncidentId(String rootCauseIncidentId) {
this.rootCauseIncidentId = JsonNullable.of(rootCauseIncidentId);
}
public IncidentDto _configuration(String _configuration) {
this._configuration = JsonNullable.of(_configuration);
return this;
}
/**
* The payload of this incident.
* @return _configuration
**/
@javax.annotation.Nullable
@JsonIgnore
public String getConfiguration() {
return _configuration.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CONFIGURATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getConfiguration_JsonNullable() {
return _configuration;
}
@JsonProperty(JSON_PROPERTY_CONFIGURATION)
public void setConfiguration_JsonNullable(JsonNullable _configuration) {
this._configuration = _configuration;
}
public void setConfiguration(String _configuration) {
this._configuration = JsonNullable.of(_configuration);
}
public IncidentDto tenantId(String tenantId) {
this.tenantId = JsonNullable.of(tenantId);
return this;
}
/**
* The id of the tenant this incident is associated with.
* @return tenantId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getTenantId() {
return tenantId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TENANT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getTenantId_JsonNullable() {
return tenantId;
}
@JsonProperty(JSON_PROPERTY_TENANT_ID)
public void setTenantId_JsonNullable(JsonNullable tenantId) {
this.tenantId = tenantId;
}
public void setTenantId(String tenantId) {
this.tenantId = JsonNullable.of(tenantId);
}
public IncidentDto incidentMessage(String incidentMessage) {
this.incidentMessage = JsonNullable.of(incidentMessage);
return this;
}
/**
* The message of this incident.
* @return incidentMessage
**/
@javax.annotation.Nullable
@JsonIgnore
public String getIncidentMessage() {
return incidentMessage.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INCIDENT_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getIncidentMessage_JsonNullable() {
return incidentMessage;
}
@JsonProperty(JSON_PROPERTY_INCIDENT_MESSAGE)
public void setIncidentMessage_JsonNullable(JsonNullable incidentMessage) {
this.incidentMessage = incidentMessage;
}
public void setIncidentMessage(String incidentMessage) {
this.incidentMessage = JsonNullable.of(incidentMessage);
}
public IncidentDto jobDefinitionId(String jobDefinitionId) {
this.jobDefinitionId = JsonNullable.of(jobDefinitionId);
return this;
}
/**
* The job definition id the incident is associated with.
* @return jobDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getJobDefinitionId() {
return jobDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_JOB_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getJobDefinitionId_JsonNullable() {
return jobDefinitionId;
}
@JsonProperty(JSON_PROPERTY_JOB_DEFINITION_ID)
public void setJobDefinitionId_JsonNullable(JsonNullable jobDefinitionId) {
this.jobDefinitionId = jobDefinitionId;
}
public void setJobDefinitionId(String jobDefinitionId) {
this.jobDefinitionId = JsonNullable.of(jobDefinitionId);
}
public IncidentDto annotation(String annotation) {
this.annotation = JsonNullable.of(annotation);
return this;
}
/**
* The annotation set to the incident.
* @return annotation
**/
@javax.annotation.Nullable
@JsonIgnore
public String getAnnotation() {
return annotation.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ANNOTATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getAnnotation_JsonNullable() {
return annotation;
}
@JsonProperty(JSON_PROPERTY_ANNOTATION)
public void setAnnotation_JsonNullable(JsonNullable annotation) {
this.annotation = annotation;
}
public void setAnnotation(String annotation) {
this.annotation = JsonNullable.of(annotation);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
IncidentDto incidentDto = (IncidentDto) o;
return equalsNullable(this.id, incidentDto.id) &&
equalsNullable(this.processDefinitionId, incidentDto.processDefinitionId) &&
equalsNullable(this.processInstanceId, incidentDto.processInstanceId) &&
equalsNullable(this.executionId, incidentDto.executionId) &&
equalsNullable(this.incidentTimestamp, incidentDto.incidentTimestamp) &&
equalsNullable(this.incidentType, incidentDto.incidentType) &&
equalsNullable(this.activityId, incidentDto.activityId) &&
equalsNullable(this.failedActivityId, incidentDto.failedActivityId) &&
equalsNullable(this.causeIncidentId, incidentDto.causeIncidentId) &&
equalsNullable(this.rootCauseIncidentId, incidentDto.rootCauseIncidentId) &&
equalsNullable(this._configuration, incidentDto._configuration) &&
equalsNullable(this.tenantId, incidentDto.tenantId) &&
equalsNullable(this.incidentMessage, incidentDto.incidentMessage) &&
equalsNullable(this.jobDefinitionId, incidentDto.jobDefinitionId) &&
equalsNullable(this.annotation, incidentDto.annotation);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(id), hashCodeNullable(processDefinitionId), hashCodeNullable(processInstanceId), hashCodeNullable(executionId), hashCodeNullable(incidentTimestamp), hashCodeNullable(incidentType), hashCodeNullable(activityId), hashCodeNullable(failedActivityId), hashCodeNullable(causeIncidentId), hashCodeNullable(rootCauseIncidentId), hashCodeNullable(_configuration), hashCodeNullable(tenantId), hashCodeNullable(incidentMessage), hashCodeNullable(jobDefinitionId), hashCodeNullable(annotation));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class IncidentDto {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" processDefinitionId: ").append(toIndentedString(processDefinitionId)).append("\n");
sb.append(" processInstanceId: ").append(toIndentedString(processInstanceId)).append("\n");
sb.append(" executionId: ").append(toIndentedString(executionId)).append("\n");
sb.append(" incidentTimestamp: ").append(toIndentedString(incidentTimestamp)).append("\n");
sb.append(" incidentType: ").append(toIndentedString(incidentType)).append("\n");
sb.append(" activityId: ").append(toIndentedString(activityId)).append("\n");
sb.append(" failedActivityId: ").append(toIndentedString(failedActivityId)).append("\n");
sb.append(" causeIncidentId: ").append(toIndentedString(causeIncidentId)).append("\n");
sb.append(" rootCauseIncidentId: ").append(toIndentedString(rootCauseIncidentId)).append("\n");
sb.append(" _configuration: ").append(toIndentedString(_configuration)).append("\n");
sb.append(" tenantId: ").append(toIndentedString(tenantId)).append("\n");
sb.append(" incidentMessage: ").append(toIndentedString(incidentMessage)).append("\n");
sb.append(" jobDefinitionId: ").append(toIndentedString(jobDefinitionId)).append("\n");
sb.append(" annotation: ").append(toIndentedString(annotation)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `id` to the URL query string
if (getId() != null) {
try {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processDefinitionId` to the URL query string
if (getProcessDefinitionId() != null) {
try {
joiner.add(String.format("%sprocessDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processInstanceId` to the URL query string
if (getProcessInstanceId() != null) {
try {
joiner.add(String.format("%sprocessInstanceId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessInstanceId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `executionId` to the URL query string
if (getExecutionId() != null) {
try {
joiner.add(String.format("%sexecutionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getExecutionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `incidentTimestamp` to the URL query string
if (getIncidentTimestamp() != null) {
try {
joiner.add(String.format("%sincidentTimestamp%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIncidentTimestamp()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `incidentType` to the URL query string
if (getIncidentType() != null) {
try {
joiner.add(String.format("%sincidentType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIncidentType()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `activityId` to the URL query string
if (getActivityId() != null) {
try {
joiner.add(String.format("%sactivityId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getActivityId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `failedActivityId` to the URL query string
if (getFailedActivityId() != null) {
try {
joiner.add(String.format("%sfailedActivityId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getFailedActivityId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `causeIncidentId` to the URL query string
if (getCauseIncidentId() != null) {
try {
joiner.add(String.format("%scauseIncidentId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCauseIncidentId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `rootCauseIncidentId` to the URL query string
if (getRootCauseIncidentId() != null) {
try {
joiner.add(String.format("%srootCauseIncidentId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getRootCauseIncidentId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `configuration` to the URL query string
if (getConfiguration() != null) {
try {
joiner.add(String.format("%sconfiguration%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getConfiguration()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `tenantId` to the URL query string
if (getTenantId() != null) {
try {
joiner.add(String.format("%stenantId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTenantId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `incidentMessage` to the URL query string
if (getIncidentMessage() != null) {
try {
joiner.add(String.format("%sincidentMessage%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIncidentMessage()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `jobDefinitionId` to the URL query string
if (getJobDefinitionId() != null) {
try {
joiner.add(String.format("%sjobDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getJobDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `annotation` to the URL query string
if (getAnnotation() != null) {
try {
joiner.add(String.format("%sannotation%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAnnotation()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy