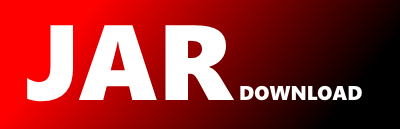
org.camunda.community.rest.client.dto.ProcessInstanceQueryDto Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.dto;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.camunda.community.rest.client.dto.ProcessInstanceQueryDtoSortingInner;
import org.camunda.community.rest.client.dto.VariableQueryParameterDto;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* A process instance query which defines a group of process instances
*/
@JsonPropertyOrder({
ProcessInstanceQueryDto.JSON_PROPERTY_DEPLOYMENT_ID,
ProcessInstanceQueryDto.JSON_PROPERTY_PROCESS_DEFINITION_ID,
ProcessInstanceQueryDto.JSON_PROPERTY_PROCESS_DEFINITION_KEY,
ProcessInstanceQueryDto.JSON_PROPERTY_PROCESS_DEFINITION_KEY_IN,
ProcessInstanceQueryDto.JSON_PROPERTY_PROCESS_DEFINITION_KEY_NOT_IN,
ProcessInstanceQueryDto.JSON_PROPERTY_BUSINESS_KEY,
ProcessInstanceQueryDto.JSON_PROPERTY_BUSINESS_KEY_LIKE,
ProcessInstanceQueryDto.JSON_PROPERTY_CASE_INSTANCE_ID,
ProcessInstanceQueryDto.JSON_PROPERTY_SUPER_PROCESS_INSTANCE,
ProcessInstanceQueryDto.JSON_PROPERTY_SUB_PROCESS_INSTANCE,
ProcessInstanceQueryDto.JSON_PROPERTY_SUPER_CASE_INSTANCE,
ProcessInstanceQueryDto.JSON_PROPERTY_SUB_CASE_INSTANCE,
ProcessInstanceQueryDto.JSON_PROPERTY_ACTIVE,
ProcessInstanceQueryDto.JSON_PROPERTY_SUSPENDED,
ProcessInstanceQueryDto.JSON_PROPERTY_PROCESS_INSTANCE_IDS,
ProcessInstanceQueryDto.JSON_PROPERTY_WITH_INCIDENT,
ProcessInstanceQueryDto.JSON_PROPERTY_INCIDENT_ID,
ProcessInstanceQueryDto.JSON_PROPERTY_INCIDENT_TYPE,
ProcessInstanceQueryDto.JSON_PROPERTY_INCIDENT_MESSAGE,
ProcessInstanceQueryDto.JSON_PROPERTY_INCIDENT_MESSAGE_LIKE,
ProcessInstanceQueryDto.JSON_PROPERTY_TENANT_ID_IN,
ProcessInstanceQueryDto.JSON_PROPERTY_WITHOUT_TENANT_ID,
ProcessInstanceQueryDto.JSON_PROPERTY_PROCESS_DEFINITION_WITHOUT_TENANT_ID,
ProcessInstanceQueryDto.JSON_PROPERTY_ACTIVITY_ID_IN,
ProcessInstanceQueryDto.JSON_PROPERTY_ROOT_PROCESS_INSTANCES,
ProcessInstanceQueryDto.JSON_PROPERTY_LEAF_PROCESS_INSTANCES,
ProcessInstanceQueryDto.JSON_PROPERTY_VARIABLES,
ProcessInstanceQueryDto.JSON_PROPERTY_VARIABLE_NAMES_IGNORE_CASE,
ProcessInstanceQueryDto.JSON_PROPERTY_VARIABLE_VALUES_IGNORE_CASE,
ProcessInstanceQueryDto.JSON_PROPERTY_OR_QUERIES,
ProcessInstanceQueryDto.JSON_PROPERTY_SORTING
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class ProcessInstanceQueryDto {
public static final String JSON_PROPERTY_DEPLOYMENT_ID = "deploymentId";
private JsonNullable deploymentId = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_DEFINITION_ID = "processDefinitionId";
private JsonNullable processDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_DEFINITION_KEY = "processDefinitionKey";
private JsonNullable processDefinitionKey = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_DEFINITION_KEY_IN = "processDefinitionKeyIn";
private JsonNullable> processDefinitionKeyIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_PROCESS_DEFINITION_KEY_NOT_IN = "processDefinitionKeyNotIn";
private JsonNullable> processDefinitionKeyNotIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_BUSINESS_KEY = "businessKey";
private JsonNullable businessKey = JsonNullable.undefined();
public static final String JSON_PROPERTY_BUSINESS_KEY_LIKE = "businessKeyLike";
private JsonNullable businessKeyLike = JsonNullable.undefined();
public static final String JSON_PROPERTY_CASE_INSTANCE_ID = "caseInstanceId";
private JsonNullable caseInstanceId = JsonNullable.undefined();
public static final String JSON_PROPERTY_SUPER_PROCESS_INSTANCE = "superProcessInstance";
private JsonNullable superProcessInstance = JsonNullable.undefined();
public static final String JSON_PROPERTY_SUB_PROCESS_INSTANCE = "subProcessInstance";
private JsonNullable subProcessInstance = JsonNullable.undefined();
public static final String JSON_PROPERTY_SUPER_CASE_INSTANCE = "superCaseInstance";
private JsonNullable superCaseInstance = JsonNullable.undefined();
public static final String JSON_PROPERTY_SUB_CASE_INSTANCE = "subCaseInstance";
private JsonNullable subCaseInstance = JsonNullable.undefined();
public static final String JSON_PROPERTY_ACTIVE = "active";
private JsonNullable active = JsonNullable.undefined();
public static final String JSON_PROPERTY_SUSPENDED = "suspended";
private JsonNullable suspended = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_INSTANCE_IDS = "processInstanceIds";
private JsonNullable> processInstanceIds = JsonNullable.>undefined();
public static final String JSON_PROPERTY_WITH_INCIDENT = "withIncident";
private JsonNullable withIncident = JsonNullable.undefined();
public static final String JSON_PROPERTY_INCIDENT_ID = "incidentId";
private JsonNullable incidentId = JsonNullable.undefined();
public static final String JSON_PROPERTY_INCIDENT_TYPE = "incidentType";
private JsonNullable incidentType = JsonNullable.undefined();
public static final String JSON_PROPERTY_INCIDENT_MESSAGE = "incidentMessage";
private JsonNullable incidentMessage = JsonNullable.undefined();
public static final String JSON_PROPERTY_INCIDENT_MESSAGE_LIKE = "incidentMessageLike";
private JsonNullable incidentMessageLike = JsonNullable.undefined();
public static final String JSON_PROPERTY_TENANT_ID_IN = "tenantIdIn";
private JsonNullable> tenantIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_WITHOUT_TENANT_ID = "withoutTenantId";
private JsonNullable withoutTenantId = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_DEFINITION_WITHOUT_TENANT_ID = "processDefinitionWithoutTenantId";
private JsonNullable processDefinitionWithoutTenantId = JsonNullable.undefined();
public static final String JSON_PROPERTY_ACTIVITY_ID_IN = "activityIdIn";
private JsonNullable> activityIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_ROOT_PROCESS_INSTANCES = "rootProcessInstances";
private JsonNullable rootProcessInstances = JsonNullable.undefined();
public static final String JSON_PROPERTY_LEAF_PROCESS_INSTANCES = "leafProcessInstances";
private JsonNullable leafProcessInstances = JsonNullable.undefined();
public static final String JSON_PROPERTY_VARIABLES = "variables";
private JsonNullable> variables = JsonNullable.>undefined();
public static final String JSON_PROPERTY_VARIABLE_NAMES_IGNORE_CASE = "variableNamesIgnoreCase";
private JsonNullable variableNamesIgnoreCase = JsonNullable.undefined();
public static final String JSON_PROPERTY_VARIABLE_VALUES_IGNORE_CASE = "variableValuesIgnoreCase";
private JsonNullable variableValuesIgnoreCase = JsonNullable.undefined();
public static final String JSON_PROPERTY_OR_QUERIES = "orQueries";
private JsonNullable> orQueries = JsonNullable.>undefined();
public static final String JSON_PROPERTY_SORTING = "sorting";
private JsonNullable> sorting = JsonNullable.>undefined();
public ProcessInstanceQueryDto() {
}
public ProcessInstanceQueryDto deploymentId(String deploymentId) {
this.deploymentId = JsonNullable.of(deploymentId);
return this;
}
/**
* Filter by the deployment the id belongs to.
* @return deploymentId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getDeploymentId() {
return deploymentId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_DEPLOYMENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getDeploymentId_JsonNullable() {
return deploymentId;
}
@JsonProperty(JSON_PROPERTY_DEPLOYMENT_ID)
public void setDeploymentId_JsonNullable(JsonNullable deploymentId) {
this.deploymentId = deploymentId;
}
public void setDeploymentId(String deploymentId) {
this.deploymentId = JsonNullable.of(deploymentId);
}
public ProcessInstanceQueryDto processDefinitionId(String processDefinitionId) {
this.processDefinitionId = JsonNullable.of(processDefinitionId);
return this;
}
/**
* Filter by the process definition the instances run on.
* @return processDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProcessDefinitionId() {
return processDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessDefinitionId_JsonNullable() {
return processDefinitionId;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_ID)
public void setProcessDefinitionId_JsonNullable(JsonNullable processDefinitionId) {
this.processDefinitionId = processDefinitionId;
}
public void setProcessDefinitionId(String processDefinitionId) {
this.processDefinitionId = JsonNullable.of(processDefinitionId);
}
public ProcessInstanceQueryDto processDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = JsonNullable.of(processDefinitionKey);
return this;
}
/**
* Filter by the key of the process definition the instances run on.
* @return processDefinitionKey
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProcessDefinitionKey() {
return processDefinitionKey.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessDefinitionKey_JsonNullable() {
return processDefinitionKey;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY)
public void setProcessDefinitionKey_JsonNullable(JsonNullable processDefinitionKey) {
this.processDefinitionKey = processDefinitionKey;
}
public void setProcessDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = JsonNullable.of(processDefinitionKey);
}
public ProcessInstanceQueryDto processDefinitionKeyIn(List processDefinitionKeyIn) {
this.processDefinitionKeyIn = JsonNullable.>of(processDefinitionKeyIn);
return this;
}
public ProcessInstanceQueryDto addProcessDefinitionKeyInItem(String processDefinitionKeyInItem) {
if (this.processDefinitionKeyIn == null || !this.processDefinitionKeyIn.isPresent()) {
this.processDefinitionKeyIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.processDefinitionKeyIn.get().add(processDefinitionKeyInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Filter by a list of process definition keys. A process instance must have one of the given process definition keys. Must be a JSON array of Strings.
* @return processDefinitionKeyIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getProcessDefinitionKeyIn() {
return processDefinitionKeyIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getProcessDefinitionKeyIn_JsonNullable() {
return processDefinitionKeyIn;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY_IN)
public void setProcessDefinitionKeyIn_JsonNullable(JsonNullable> processDefinitionKeyIn) {
this.processDefinitionKeyIn = processDefinitionKeyIn;
}
public void setProcessDefinitionKeyIn(List processDefinitionKeyIn) {
this.processDefinitionKeyIn = JsonNullable.>of(processDefinitionKeyIn);
}
public ProcessInstanceQueryDto processDefinitionKeyNotIn(List processDefinitionKeyNotIn) {
this.processDefinitionKeyNotIn = JsonNullable.>of(processDefinitionKeyNotIn);
return this;
}
public ProcessInstanceQueryDto addProcessDefinitionKeyNotInItem(String processDefinitionKeyNotInItem) {
if (this.processDefinitionKeyNotIn == null || !this.processDefinitionKeyNotIn.isPresent()) {
this.processDefinitionKeyNotIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.processDefinitionKeyNotIn.get().add(processDefinitionKeyNotInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Exclude instances by a list of process definition keys. A process instance must not have one of the given process definition keys. Must be a JSON array of Strings.
* @return processDefinitionKeyNotIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getProcessDefinitionKeyNotIn() {
return processDefinitionKeyNotIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY_NOT_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getProcessDefinitionKeyNotIn_JsonNullable() {
return processDefinitionKeyNotIn;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY_NOT_IN)
public void setProcessDefinitionKeyNotIn_JsonNullable(JsonNullable> processDefinitionKeyNotIn) {
this.processDefinitionKeyNotIn = processDefinitionKeyNotIn;
}
public void setProcessDefinitionKeyNotIn(List processDefinitionKeyNotIn) {
this.processDefinitionKeyNotIn = JsonNullable.>of(processDefinitionKeyNotIn);
}
public ProcessInstanceQueryDto businessKey(String businessKey) {
this.businessKey = JsonNullable.of(businessKey);
return this;
}
/**
* Filter by process instance business key.
* @return businessKey
**/
@javax.annotation.Nullable
@JsonIgnore
public String getBusinessKey() {
return businessKey.orElse(null);
}
@JsonProperty(JSON_PROPERTY_BUSINESS_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getBusinessKey_JsonNullable() {
return businessKey;
}
@JsonProperty(JSON_PROPERTY_BUSINESS_KEY)
public void setBusinessKey_JsonNullable(JsonNullable businessKey) {
this.businessKey = businessKey;
}
public void setBusinessKey(String businessKey) {
this.businessKey = JsonNullable.of(businessKey);
}
public ProcessInstanceQueryDto businessKeyLike(String businessKeyLike) {
this.businessKeyLike = JsonNullable.of(businessKeyLike);
return this;
}
/**
* Filter by process instance business key that the parameter is a substring of.
* @return businessKeyLike
**/
@javax.annotation.Nullable
@JsonIgnore
public String getBusinessKeyLike() {
return businessKeyLike.orElse(null);
}
@JsonProperty(JSON_PROPERTY_BUSINESS_KEY_LIKE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getBusinessKeyLike_JsonNullable() {
return businessKeyLike;
}
@JsonProperty(JSON_PROPERTY_BUSINESS_KEY_LIKE)
public void setBusinessKeyLike_JsonNullable(JsonNullable businessKeyLike) {
this.businessKeyLike = businessKeyLike;
}
public void setBusinessKeyLike(String businessKeyLike) {
this.businessKeyLike = JsonNullable.of(businessKeyLike);
}
public ProcessInstanceQueryDto caseInstanceId(String caseInstanceId) {
this.caseInstanceId = JsonNullable.of(caseInstanceId);
return this;
}
/**
* Filter by case instance id.
* @return caseInstanceId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getCaseInstanceId() {
return caseInstanceId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CASE_INSTANCE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCaseInstanceId_JsonNullable() {
return caseInstanceId;
}
@JsonProperty(JSON_PROPERTY_CASE_INSTANCE_ID)
public void setCaseInstanceId_JsonNullable(JsonNullable caseInstanceId) {
this.caseInstanceId = caseInstanceId;
}
public void setCaseInstanceId(String caseInstanceId) {
this.caseInstanceId = JsonNullable.of(caseInstanceId);
}
public ProcessInstanceQueryDto superProcessInstance(String superProcessInstance) {
this.superProcessInstance = JsonNullable.of(superProcessInstance);
return this;
}
/**
* Restrict query to all process instances that are sub process instances of the given process instance. Takes a process instance id.
* @return superProcessInstance
**/
@javax.annotation.Nullable
@JsonIgnore
public String getSuperProcessInstance() {
return superProcessInstance.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SUPER_PROCESS_INSTANCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSuperProcessInstance_JsonNullable() {
return superProcessInstance;
}
@JsonProperty(JSON_PROPERTY_SUPER_PROCESS_INSTANCE)
public void setSuperProcessInstance_JsonNullable(JsonNullable superProcessInstance) {
this.superProcessInstance = superProcessInstance;
}
public void setSuperProcessInstance(String superProcessInstance) {
this.superProcessInstance = JsonNullable.of(superProcessInstance);
}
public ProcessInstanceQueryDto subProcessInstance(String subProcessInstance) {
this.subProcessInstance = JsonNullable.of(subProcessInstance);
return this;
}
/**
* Restrict query to all process instances that have the given process instance as a sub process instance. Takes a process instance id.
* @return subProcessInstance
**/
@javax.annotation.Nullable
@JsonIgnore
public String getSubProcessInstance() {
return subProcessInstance.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SUB_PROCESS_INSTANCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSubProcessInstance_JsonNullable() {
return subProcessInstance;
}
@JsonProperty(JSON_PROPERTY_SUB_PROCESS_INSTANCE)
public void setSubProcessInstance_JsonNullable(JsonNullable subProcessInstance) {
this.subProcessInstance = subProcessInstance;
}
public void setSubProcessInstance(String subProcessInstance) {
this.subProcessInstance = JsonNullable.of(subProcessInstance);
}
public ProcessInstanceQueryDto superCaseInstance(String superCaseInstance) {
this.superCaseInstance = JsonNullable.of(superCaseInstance);
return this;
}
/**
* Restrict query to all process instances that are sub process instances of the given case instance. Takes a case instance id.
* @return superCaseInstance
**/
@javax.annotation.Nullable
@JsonIgnore
public String getSuperCaseInstance() {
return superCaseInstance.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SUPER_CASE_INSTANCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSuperCaseInstance_JsonNullable() {
return superCaseInstance;
}
@JsonProperty(JSON_PROPERTY_SUPER_CASE_INSTANCE)
public void setSuperCaseInstance_JsonNullable(JsonNullable superCaseInstance) {
this.superCaseInstance = superCaseInstance;
}
public void setSuperCaseInstance(String superCaseInstance) {
this.superCaseInstance = JsonNullable.of(superCaseInstance);
}
public ProcessInstanceQueryDto subCaseInstance(String subCaseInstance) {
this.subCaseInstance = JsonNullable.of(subCaseInstance);
return this;
}
/**
* Restrict query to all process instances that have the given case instance as a sub case instance. Takes a case instance id.
* @return subCaseInstance
**/
@javax.annotation.Nullable
@JsonIgnore
public String getSubCaseInstance() {
return subCaseInstance.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SUB_CASE_INSTANCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSubCaseInstance_JsonNullable() {
return subCaseInstance;
}
@JsonProperty(JSON_PROPERTY_SUB_CASE_INSTANCE)
public void setSubCaseInstance_JsonNullable(JsonNullable subCaseInstance) {
this.subCaseInstance = subCaseInstance;
}
public void setSubCaseInstance(String subCaseInstance) {
this.subCaseInstance = JsonNullable.of(subCaseInstance);
}
public ProcessInstanceQueryDto active(Boolean active) {
this.active = JsonNullable.of(active);
return this;
}
/**
* Only include active process instances. Value may only be true, as false is the default behavior.
* @return active
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getActive() {
return active.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ACTIVE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getActive_JsonNullable() {
return active;
}
@JsonProperty(JSON_PROPERTY_ACTIVE)
public void setActive_JsonNullable(JsonNullable active) {
this.active = active;
}
public void setActive(Boolean active) {
this.active = JsonNullable.of(active);
}
public ProcessInstanceQueryDto suspended(Boolean suspended) {
this.suspended = JsonNullable.of(suspended);
return this;
}
/**
* Only include suspended process instances. Value may only be true, as false is the default behavior.
* @return suspended
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getSuspended() {
return suspended.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SUSPENDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSuspended_JsonNullable() {
return suspended;
}
@JsonProperty(JSON_PROPERTY_SUSPENDED)
public void setSuspended_JsonNullable(JsonNullable suspended) {
this.suspended = suspended;
}
public void setSuspended(Boolean suspended) {
this.suspended = JsonNullable.of(suspended);
}
public ProcessInstanceQueryDto processInstanceIds(List processInstanceIds) {
this.processInstanceIds = JsonNullable.>of(processInstanceIds);
return this;
}
public ProcessInstanceQueryDto addProcessInstanceIdsItem(String processInstanceIdsItem) {
if (this.processInstanceIds == null || !this.processInstanceIds.isPresent()) {
this.processInstanceIds = JsonNullable.>of(new ArrayList<>());
}
try {
this.processInstanceIds.get().add(processInstanceIdsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Filter by a list of process instance ids. Must be a JSON array of Strings.
* @return processInstanceIds
**/
@javax.annotation.Nullable
@JsonIgnore
public List getProcessInstanceIds() {
return processInstanceIds.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getProcessInstanceIds_JsonNullable() {
return processInstanceIds;
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_IDS)
public void setProcessInstanceIds_JsonNullable(JsonNullable> processInstanceIds) {
this.processInstanceIds = processInstanceIds;
}
public void setProcessInstanceIds(List processInstanceIds) {
this.processInstanceIds = JsonNullable.>of(processInstanceIds);
}
public ProcessInstanceQueryDto withIncident(Boolean withIncident) {
this.withIncident = JsonNullable.of(withIncident);
return this;
}
/**
* Filter by presence of incidents. Selects only process instances that have an incident.
* @return withIncident
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getWithIncident() {
return withIncident.orElse(null);
}
@JsonProperty(JSON_PROPERTY_WITH_INCIDENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getWithIncident_JsonNullable() {
return withIncident;
}
@JsonProperty(JSON_PROPERTY_WITH_INCIDENT)
public void setWithIncident_JsonNullable(JsonNullable withIncident) {
this.withIncident = withIncident;
}
public void setWithIncident(Boolean withIncident) {
this.withIncident = JsonNullable.of(withIncident);
}
public ProcessInstanceQueryDto incidentId(String incidentId) {
this.incidentId = JsonNullable.of(incidentId);
return this;
}
/**
* Filter by the incident id.
* @return incidentId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getIncidentId() {
return incidentId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INCIDENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getIncidentId_JsonNullable() {
return incidentId;
}
@JsonProperty(JSON_PROPERTY_INCIDENT_ID)
public void setIncidentId_JsonNullable(JsonNullable incidentId) {
this.incidentId = incidentId;
}
public void setIncidentId(String incidentId) {
this.incidentId = JsonNullable.of(incidentId);
}
public ProcessInstanceQueryDto incidentType(String incidentType) {
this.incidentType = JsonNullable.of(incidentType);
return this;
}
/**
* Filter by the incident type. See the User Guide for a list of incident types.
* @return incidentType
**/
@javax.annotation.Nullable
@JsonIgnore
public String getIncidentType() {
return incidentType.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INCIDENT_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getIncidentType_JsonNullable() {
return incidentType;
}
@JsonProperty(JSON_PROPERTY_INCIDENT_TYPE)
public void setIncidentType_JsonNullable(JsonNullable incidentType) {
this.incidentType = incidentType;
}
public void setIncidentType(String incidentType) {
this.incidentType = JsonNullable.of(incidentType);
}
public ProcessInstanceQueryDto incidentMessage(String incidentMessage) {
this.incidentMessage = JsonNullable.of(incidentMessage);
return this;
}
/**
* Filter by the incident message. Exact match.
* @return incidentMessage
**/
@javax.annotation.Nullable
@JsonIgnore
public String getIncidentMessage() {
return incidentMessage.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INCIDENT_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getIncidentMessage_JsonNullable() {
return incidentMessage;
}
@JsonProperty(JSON_PROPERTY_INCIDENT_MESSAGE)
public void setIncidentMessage_JsonNullable(JsonNullable incidentMessage) {
this.incidentMessage = incidentMessage;
}
public void setIncidentMessage(String incidentMessage) {
this.incidentMessage = JsonNullable.of(incidentMessage);
}
public ProcessInstanceQueryDto incidentMessageLike(String incidentMessageLike) {
this.incidentMessageLike = JsonNullable.of(incidentMessageLike);
return this;
}
/**
* Filter by the incident message that the parameter is a substring of.
* @return incidentMessageLike
**/
@javax.annotation.Nullable
@JsonIgnore
public String getIncidentMessageLike() {
return incidentMessageLike.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INCIDENT_MESSAGE_LIKE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getIncidentMessageLike_JsonNullable() {
return incidentMessageLike;
}
@JsonProperty(JSON_PROPERTY_INCIDENT_MESSAGE_LIKE)
public void setIncidentMessageLike_JsonNullable(JsonNullable incidentMessageLike) {
this.incidentMessageLike = incidentMessageLike;
}
public void setIncidentMessageLike(String incidentMessageLike) {
this.incidentMessageLike = JsonNullable.of(incidentMessageLike);
}
public ProcessInstanceQueryDto tenantIdIn(List tenantIdIn) {
this.tenantIdIn = JsonNullable.>of(tenantIdIn);
return this;
}
public ProcessInstanceQueryDto addTenantIdInItem(String tenantIdInItem) {
if (this.tenantIdIn == null || !this.tenantIdIn.isPresent()) {
this.tenantIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.tenantIdIn.get().add(tenantIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Filter by a list of tenant ids. A process instance must have one of the given tenant ids. Must be a JSON array of Strings.
* @return tenantIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getTenantIdIn() {
return tenantIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TENANT_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getTenantIdIn_JsonNullable() {
return tenantIdIn;
}
@JsonProperty(JSON_PROPERTY_TENANT_ID_IN)
public void setTenantIdIn_JsonNullable(JsonNullable> tenantIdIn) {
this.tenantIdIn = tenantIdIn;
}
public void setTenantIdIn(List tenantIdIn) {
this.tenantIdIn = JsonNullable.>of(tenantIdIn);
}
public ProcessInstanceQueryDto withoutTenantId(Boolean withoutTenantId) {
this.withoutTenantId = JsonNullable.of(withoutTenantId);
return this;
}
/**
* Only include process instances which belong to no tenant. Value may only be true, as false is the default behavior.
* @return withoutTenantId
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getWithoutTenantId() {
return withoutTenantId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_WITHOUT_TENANT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getWithoutTenantId_JsonNullable() {
return withoutTenantId;
}
@JsonProperty(JSON_PROPERTY_WITHOUT_TENANT_ID)
public void setWithoutTenantId_JsonNullable(JsonNullable withoutTenantId) {
this.withoutTenantId = withoutTenantId;
}
public void setWithoutTenantId(Boolean withoutTenantId) {
this.withoutTenantId = JsonNullable.of(withoutTenantId);
}
public ProcessInstanceQueryDto processDefinitionWithoutTenantId(Boolean processDefinitionWithoutTenantId) {
this.processDefinitionWithoutTenantId = JsonNullable.of(processDefinitionWithoutTenantId);
return this;
}
/**
* Only include process instances which process definition has no tenant id.
* @return processDefinitionWithoutTenantId
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getProcessDefinitionWithoutTenantId() {
return processDefinitionWithoutTenantId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_WITHOUT_TENANT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessDefinitionWithoutTenantId_JsonNullable() {
return processDefinitionWithoutTenantId;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_WITHOUT_TENANT_ID)
public void setProcessDefinitionWithoutTenantId_JsonNullable(JsonNullable processDefinitionWithoutTenantId) {
this.processDefinitionWithoutTenantId = processDefinitionWithoutTenantId;
}
public void setProcessDefinitionWithoutTenantId(Boolean processDefinitionWithoutTenantId) {
this.processDefinitionWithoutTenantId = JsonNullable.of(processDefinitionWithoutTenantId);
}
public ProcessInstanceQueryDto activityIdIn(List activityIdIn) {
this.activityIdIn = JsonNullable.>of(activityIdIn);
return this;
}
public ProcessInstanceQueryDto addActivityIdInItem(String activityIdInItem) {
if (this.activityIdIn == null || !this.activityIdIn.isPresent()) {
this.activityIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.activityIdIn.get().add(activityIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Filter by a list of activity ids. A process instance must currently wait in a leaf activity with one of the given activity ids.
* @return activityIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getActivityIdIn() {
return activityIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getActivityIdIn_JsonNullable() {
return activityIdIn;
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_ID_IN)
public void setActivityIdIn_JsonNullable(JsonNullable> activityIdIn) {
this.activityIdIn = activityIdIn;
}
public void setActivityIdIn(List activityIdIn) {
this.activityIdIn = JsonNullable.>of(activityIdIn);
}
public ProcessInstanceQueryDto rootProcessInstances(Boolean rootProcessInstances) {
this.rootProcessInstances = JsonNullable.of(rootProcessInstances);
return this;
}
/**
* Restrict the query to all process instances that are top level process instances.
* @return rootProcessInstances
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getRootProcessInstances() {
return rootProcessInstances.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ROOT_PROCESS_INSTANCES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getRootProcessInstances_JsonNullable() {
return rootProcessInstances;
}
@JsonProperty(JSON_PROPERTY_ROOT_PROCESS_INSTANCES)
public void setRootProcessInstances_JsonNullable(JsonNullable rootProcessInstances) {
this.rootProcessInstances = rootProcessInstances;
}
public void setRootProcessInstances(Boolean rootProcessInstances) {
this.rootProcessInstances = JsonNullable.of(rootProcessInstances);
}
public ProcessInstanceQueryDto leafProcessInstances(Boolean leafProcessInstances) {
this.leafProcessInstances = JsonNullable.of(leafProcessInstances);
return this;
}
/**
* Restrict the query to all process instances that are leaf instances. (i.e. don't have any sub instances)
* @return leafProcessInstances
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getLeafProcessInstances() {
return leafProcessInstances.orElse(null);
}
@JsonProperty(JSON_PROPERTY_LEAF_PROCESS_INSTANCES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getLeafProcessInstances_JsonNullable() {
return leafProcessInstances;
}
@JsonProperty(JSON_PROPERTY_LEAF_PROCESS_INSTANCES)
public void setLeafProcessInstances_JsonNullable(JsonNullable leafProcessInstances) {
this.leafProcessInstances = leafProcessInstances;
}
public void setLeafProcessInstances(Boolean leafProcessInstances) {
this.leafProcessInstances = JsonNullable.of(leafProcessInstances);
}
public ProcessInstanceQueryDto variables(List variables) {
this.variables = JsonNullable.>of(variables);
return this;
}
public ProcessInstanceQueryDto addVariablesItem(VariableQueryParameterDto variablesItem) {
if (this.variables == null || !this.variables.isPresent()) {
this.variables = JsonNullable.>of(new ArrayList<>());
}
try {
this.variables.get().add(variablesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* A JSON array to only include process instances that have variables with certain values. The array consists of objects with the three properties `name`, `operator` and `value`. `name` (String) is the variable name, `operator` (String) is the comparison operator to be used and `value` the variable value. The `value` may be String, Number or Boolean. Valid operator values are: `eq` - equal to; `neq` - not equal to; `gt` - greater than; `gteq` - greater than or equal to; `lt` - lower than; `lteq` - lower than or equal to; `like`.
* @return variables
**/
@javax.annotation.Nullable
@JsonIgnore
public List getVariables() {
return variables.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VARIABLES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getVariables_JsonNullable() {
return variables;
}
@JsonProperty(JSON_PROPERTY_VARIABLES)
public void setVariables_JsonNullable(JsonNullable> variables) {
this.variables = variables;
}
public void setVariables(List variables) {
this.variables = JsonNullable.>of(variables);
}
public ProcessInstanceQueryDto variableNamesIgnoreCase(Boolean variableNamesIgnoreCase) {
this.variableNamesIgnoreCase = JsonNullable.of(variableNamesIgnoreCase);
return this;
}
/**
* Match all variable names in this query case-insensitively. If set to true variableName and variablename are treated as equal.
* @return variableNamesIgnoreCase
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getVariableNamesIgnoreCase() {
return variableNamesIgnoreCase.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VARIABLE_NAMES_IGNORE_CASE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getVariableNamesIgnoreCase_JsonNullable() {
return variableNamesIgnoreCase;
}
@JsonProperty(JSON_PROPERTY_VARIABLE_NAMES_IGNORE_CASE)
public void setVariableNamesIgnoreCase_JsonNullable(JsonNullable variableNamesIgnoreCase) {
this.variableNamesIgnoreCase = variableNamesIgnoreCase;
}
public void setVariableNamesIgnoreCase(Boolean variableNamesIgnoreCase) {
this.variableNamesIgnoreCase = JsonNullable.of(variableNamesIgnoreCase);
}
public ProcessInstanceQueryDto variableValuesIgnoreCase(Boolean variableValuesIgnoreCase) {
this.variableValuesIgnoreCase = JsonNullable.of(variableValuesIgnoreCase);
return this;
}
/**
* Match all variable values in this query case-insensitively. If set to true variableValue and variablevalue are treated as equal.
* @return variableValuesIgnoreCase
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getVariableValuesIgnoreCase() {
return variableValuesIgnoreCase.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VARIABLE_VALUES_IGNORE_CASE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getVariableValuesIgnoreCase_JsonNullable() {
return variableValuesIgnoreCase;
}
@JsonProperty(JSON_PROPERTY_VARIABLE_VALUES_IGNORE_CASE)
public void setVariableValuesIgnoreCase_JsonNullable(JsonNullable variableValuesIgnoreCase) {
this.variableValuesIgnoreCase = variableValuesIgnoreCase;
}
public void setVariableValuesIgnoreCase(Boolean variableValuesIgnoreCase) {
this.variableValuesIgnoreCase = JsonNullable.of(variableValuesIgnoreCase);
}
public ProcessInstanceQueryDto orQueries(List orQueries) {
this.orQueries = JsonNullable.>of(orQueries);
return this;
}
public ProcessInstanceQueryDto addOrQueriesItem(ProcessInstanceQueryDto orQueriesItem) {
if (this.orQueries == null || !this.orQueries.isPresent()) {
this.orQueries = JsonNullable.>of(new ArrayList<>());
}
try {
this.orQueries.get().add(orQueriesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* A JSON array of nested process instance queries with OR semantics. A process instance matches a nested query if it fulfills at least one of the query's predicates. With multiple nested queries, a process instance must fulfill at least one predicate of each query (Conjunctive Normal Form). All process instance query properties can be used except for: `sorting` See the [User guide](https://docs.camunda.org/manual/7.21/user-guide/process-engine/process-engine-api/#or-queries) for more information about OR queries.
* @return orQueries
**/
@javax.annotation.Nullable
@JsonIgnore
public List getOrQueries() {
return orQueries.orElse(null);
}
@JsonProperty(JSON_PROPERTY_OR_QUERIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getOrQueries_JsonNullable() {
return orQueries;
}
@JsonProperty(JSON_PROPERTY_OR_QUERIES)
public void setOrQueries_JsonNullable(JsonNullable> orQueries) {
this.orQueries = orQueries;
}
public void setOrQueries(List orQueries) {
this.orQueries = JsonNullable.>of(orQueries);
}
public ProcessInstanceQueryDto sorting(List sorting) {
this.sorting = JsonNullable.>of(sorting);
return this;
}
public ProcessInstanceQueryDto addSortingItem(ProcessInstanceQueryDtoSortingInner sortingItem) {
if (this.sorting == null || !this.sorting.isPresent()) {
this.sorting = JsonNullable.>of(new ArrayList<>());
}
try {
this.sorting.get().add(sortingItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Apply sorting of the result
* @return sorting
**/
@javax.annotation.Nullable
@JsonIgnore
public List getSorting() {
return sorting.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SORTING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getSorting_JsonNullable() {
return sorting;
}
@JsonProperty(JSON_PROPERTY_SORTING)
public void setSorting_JsonNullable(JsonNullable> sorting) {
this.sorting = sorting;
}
public void setSorting(List sorting) {
this.sorting = JsonNullable.>of(sorting);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ProcessInstanceQueryDto processInstanceQueryDto = (ProcessInstanceQueryDto) o;
return equalsNullable(this.deploymentId, processInstanceQueryDto.deploymentId) &&
equalsNullable(this.processDefinitionId, processInstanceQueryDto.processDefinitionId) &&
equalsNullable(this.processDefinitionKey, processInstanceQueryDto.processDefinitionKey) &&
equalsNullable(this.processDefinitionKeyIn, processInstanceQueryDto.processDefinitionKeyIn) &&
equalsNullable(this.processDefinitionKeyNotIn, processInstanceQueryDto.processDefinitionKeyNotIn) &&
equalsNullable(this.businessKey, processInstanceQueryDto.businessKey) &&
equalsNullable(this.businessKeyLike, processInstanceQueryDto.businessKeyLike) &&
equalsNullable(this.caseInstanceId, processInstanceQueryDto.caseInstanceId) &&
equalsNullable(this.superProcessInstance, processInstanceQueryDto.superProcessInstance) &&
equalsNullable(this.subProcessInstance, processInstanceQueryDto.subProcessInstance) &&
equalsNullable(this.superCaseInstance, processInstanceQueryDto.superCaseInstance) &&
equalsNullable(this.subCaseInstance, processInstanceQueryDto.subCaseInstance) &&
equalsNullable(this.active, processInstanceQueryDto.active) &&
equalsNullable(this.suspended, processInstanceQueryDto.suspended) &&
equalsNullable(this.processInstanceIds, processInstanceQueryDto.processInstanceIds) &&
equalsNullable(this.withIncident, processInstanceQueryDto.withIncident) &&
equalsNullable(this.incidentId, processInstanceQueryDto.incidentId) &&
equalsNullable(this.incidentType, processInstanceQueryDto.incidentType) &&
equalsNullable(this.incidentMessage, processInstanceQueryDto.incidentMessage) &&
equalsNullable(this.incidentMessageLike, processInstanceQueryDto.incidentMessageLike) &&
equalsNullable(this.tenantIdIn, processInstanceQueryDto.tenantIdIn) &&
equalsNullable(this.withoutTenantId, processInstanceQueryDto.withoutTenantId) &&
equalsNullable(this.processDefinitionWithoutTenantId, processInstanceQueryDto.processDefinitionWithoutTenantId) &&
equalsNullable(this.activityIdIn, processInstanceQueryDto.activityIdIn) &&
equalsNullable(this.rootProcessInstances, processInstanceQueryDto.rootProcessInstances) &&
equalsNullable(this.leafProcessInstances, processInstanceQueryDto.leafProcessInstances) &&
equalsNullable(this.variables, processInstanceQueryDto.variables) &&
equalsNullable(this.variableNamesIgnoreCase, processInstanceQueryDto.variableNamesIgnoreCase) &&
equalsNullable(this.variableValuesIgnoreCase, processInstanceQueryDto.variableValuesIgnoreCase) &&
equalsNullable(this.orQueries, processInstanceQueryDto.orQueries) &&
equalsNullable(this.sorting, processInstanceQueryDto.sorting);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(deploymentId), hashCodeNullable(processDefinitionId), hashCodeNullable(processDefinitionKey), hashCodeNullable(processDefinitionKeyIn), hashCodeNullable(processDefinitionKeyNotIn), hashCodeNullable(businessKey), hashCodeNullable(businessKeyLike), hashCodeNullable(caseInstanceId), hashCodeNullable(superProcessInstance), hashCodeNullable(subProcessInstance), hashCodeNullable(superCaseInstance), hashCodeNullable(subCaseInstance), hashCodeNullable(active), hashCodeNullable(suspended), hashCodeNullable(processInstanceIds), hashCodeNullable(withIncident), hashCodeNullable(incidentId), hashCodeNullable(incidentType), hashCodeNullable(incidentMessage), hashCodeNullable(incidentMessageLike), hashCodeNullable(tenantIdIn), hashCodeNullable(withoutTenantId), hashCodeNullable(processDefinitionWithoutTenantId), hashCodeNullable(activityIdIn), hashCodeNullable(rootProcessInstances), hashCodeNullable(leafProcessInstances), hashCodeNullable(variables), hashCodeNullable(variableNamesIgnoreCase), hashCodeNullable(variableValuesIgnoreCase), hashCodeNullable(orQueries), hashCodeNullable(sorting));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ProcessInstanceQueryDto {\n");
sb.append(" deploymentId: ").append(toIndentedString(deploymentId)).append("\n");
sb.append(" processDefinitionId: ").append(toIndentedString(processDefinitionId)).append("\n");
sb.append(" processDefinitionKey: ").append(toIndentedString(processDefinitionKey)).append("\n");
sb.append(" processDefinitionKeyIn: ").append(toIndentedString(processDefinitionKeyIn)).append("\n");
sb.append(" processDefinitionKeyNotIn: ").append(toIndentedString(processDefinitionKeyNotIn)).append("\n");
sb.append(" businessKey: ").append(toIndentedString(businessKey)).append("\n");
sb.append(" businessKeyLike: ").append(toIndentedString(businessKeyLike)).append("\n");
sb.append(" caseInstanceId: ").append(toIndentedString(caseInstanceId)).append("\n");
sb.append(" superProcessInstance: ").append(toIndentedString(superProcessInstance)).append("\n");
sb.append(" subProcessInstance: ").append(toIndentedString(subProcessInstance)).append("\n");
sb.append(" superCaseInstance: ").append(toIndentedString(superCaseInstance)).append("\n");
sb.append(" subCaseInstance: ").append(toIndentedString(subCaseInstance)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" suspended: ").append(toIndentedString(suspended)).append("\n");
sb.append(" processInstanceIds: ").append(toIndentedString(processInstanceIds)).append("\n");
sb.append(" withIncident: ").append(toIndentedString(withIncident)).append("\n");
sb.append(" incidentId: ").append(toIndentedString(incidentId)).append("\n");
sb.append(" incidentType: ").append(toIndentedString(incidentType)).append("\n");
sb.append(" incidentMessage: ").append(toIndentedString(incidentMessage)).append("\n");
sb.append(" incidentMessageLike: ").append(toIndentedString(incidentMessageLike)).append("\n");
sb.append(" tenantIdIn: ").append(toIndentedString(tenantIdIn)).append("\n");
sb.append(" withoutTenantId: ").append(toIndentedString(withoutTenantId)).append("\n");
sb.append(" processDefinitionWithoutTenantId: ").append(toIndentedString(processDefinitionWithoutTenantId)).append("\n");
sb.append(" activityIdIn: ").append(toIndentedString(activityIdIn)).append("\n");
sb.append(" rootProcessInstances: ").append(toIndentedString(rootProcessInstances)).append("\n");
sb.append(" leafProcessInstances: ").append(toIndentedString(leafProcessInstances)).append("\n");
sb.append(" variables: ").append(toIndentedString(variables)).append("\n");
sb.append(" variableNamesIgnoreCase: ").append(toIndentedString(variableNamesIgnoreCase)).append("\n");
sb.append(" variableValuesIgnoreCase: ").append(toIndentedString(variableValuesIgnoreCase)).append("\n");
sb.append(" orQueries: ").append(toIndentedString(orQueries)).append("\n");
sb.append(" sorting: ").append(toIndentedString(sorting)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `deploymentId` to the URL query string
if (getDeploymentId() != null) {
try {
joiner.add(String.format("%sdeploymentId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDeploymentId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processDefinitionId` to the URL query string
if (getProcessDefinitionId() != null) {
try {
joiner.add(String.format("%sprocessDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processDefinitionKey` to the URL query string
if (getProcessDefinitionKey() != null) {
try {
joiner.add(String.format("%sprocessDefinitionKey%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessDefinitionKey()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processDefinitionKeyIn` to the URL query string
if (getProcessDefinitionKeyIn() != null) {
for (int i = 0; i < getProcessDefinitionKeyIn().size(); i++) {
try {
joiner.add(String.format("%sprocessDefinitionKeyIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getProcessDefinitionKeyIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `processDefinitionKeyNotIn` to the URL query string
if (getProcessDefinitionKeyNotIn() != null) {
for (int i = 0; i < getProcessDefinitionKeyNotIn().size(); i++) {
try {
joiner.add(String.format("%sprocessDefinitionKeyNotIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getProcessDefinitionKeyNotIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `businessKey` to the URL query string
if (getBusinessKey() != null) {
try {
joiner.add(String.format("%sbusinessKey%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getBusinessKey()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `businessKeyLike` to the URL query string
if (getBusinessKeyLike() != null) {
try {
joiner.add(String.format("%sbusinessKeyLike%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getBusinessKeyLike()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `caseInstanceId` to the URL query string
if (getCaseInstanceId() != null) {
try {
joiner.add(String.format("%scaseInstanceId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCaseInstanceId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `superProcessInstance` to the URL query string
if (getSuperProcessInstance() != null) {
try {
joiner.add(String.format("%ssuperProcessInstance%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSuperProcessInstance()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `subProcessInstance` to the URL query string
if (getSubProcessInstance() != null) {
try {
joiner.add(String.format("%ssubProcessInstance%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSubProcessInstance()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `superCaseInstance` to the URL query string
if (getSuperCaseInstance() != null) {
try {
joiner.add(String.format("%ssuperCaseInstance%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSuperCaseInstance()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `subCaseInstance` to the URL query string
if (getSubCaseInstance() != null) {
try {
joiner.add(String.format("%ssubCaseInstance%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSubCaseInstance()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `active` to the URL query string
if (getActive() != null) {
try {
joiner.add(String.format("%sactive%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getActive()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `suspended` to the URL query string
if (getSuspended() != null) {
try {
joiner.add(String.format("%ssuspended%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSuspended()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processInstanceIds` to the URL query string
if (getProcessInstanceIds() != null) {
for (int i = 0; i < getProcessInstanceIds().size(); i++) {
try {
joiner.add(String.format("%sprocessInstanceIds%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getProcessInstanceIds().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `withIncident` to the URL query string
if (getWithIncident() != null) {
try {
joiner.add(String.format("%swithIncident%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getWithIncident()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `incidentId` to the URL query string
if (getIncidentId() != null) {
try {
joiner.add(String.format("%sincidentId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIncidentId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `incidentType` to the URL query string
if (getIncidentType() != null) {
try {
joiner.add(String.format("%sincidentType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIncidentType()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `incidentMessage` to the URL query string
if (getIncidentMessage() != null) {
try {
joiner.add(String.format("%sincidentMessage%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIncidentMessage()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `incidentMessageLike` to the URL query string
if (getIncidentMessageLike() != null) {
try {
joiner.add(String.format("%sincidentMessageLike%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIncidentMessageLike()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `tenantIdIn` to the URL query string
if (getTenantIdIn() != null) {
for (int i = 0; i < getTenantIdIn().size(); i++) {
try {
joiner.add(String.format("%stenantIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getTenantIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `withoutTenantId` to the URL query string
if (getWithoutTenantId() != null) {
try {
joiner.add(String.format("%swithoutTenantId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getWithoutTenantId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processDefinitionWithoutTenantId` to the URL query string
if (getProcessDefinitionWithoutTenantId() != null) {
try {
joiner.add(String.format("%sprocessDefinitionWithoutTenantId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessDefinitionWithoutTenantId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `activityIdIn` to the URL query string
if (getActivityIdIn() != null) {
for (int i = 0; i < getActivityIdIn().size(); i++) {
try {
joiner.add(String.format("%sactivityIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getActivityIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `rootProcessInstances` to the URL query string
if (getRootProcessInstances() != null) {
try {
joiner.add(String.format("%srootProcessInstances%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getRootProcessInstances()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `leafProcessInstances` to the URL query string
if (getLeafProcessInstances() != null) {
try {
joiner.add(String.format("%sleafProcessInstances%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getLeafProcessInstances()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `variables` to the URL query string
if (getVariables() != null) {
for (int i = 0; i < getVariables().size(); i++) {
if (getVariables().get(i) != null) {
joiner.add(getVariables().get(i).toUrlQueryString(String.format("%svariables%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `variableNamesIgnoreCase` to the URL query string
if (getVariableNamesIgnoreCase() != null) {
try {
joiner.add(String.format("%svariableNamesIgnoreCase%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getVariableNamesIgnoreCase()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `variableValuesIgnoreCase` to the URL query string
if (getVariableValuesIgnoreCase() != null) {
try {
joiner.add(String.format("%svariableValuesIgnoreCase%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getVariableValuesIgnoreCase()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `orQueries` to the URL query string
if (getOrQueries() != null) {
for (int i = 0; i < getOrQueries().size(); i++) {
if (getOrQueries().get(i) != null) {
joiner.add(getOrQueries().get(i).toUrlQueryString(String.format("%sorQueries%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `sorting` to the URL query string
if (getSorting() != null) {
for (int i = 0; i < getSorting().size(); i++) {
if (getSorting().get(i) != null) {
joiner.add(getSorting().get(i).toUrlQueryString(String.format("%ssorting%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy