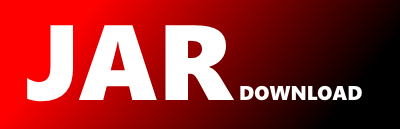
org.camunda.community.rest.client.dto.RestartProcessInstanceDto Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.dto;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.camunda.community.rest.client.dto.HistoricProcessInstanceQueryDto;
import org.camunda.community.rest.client.dto.RestartProcessInstanceModificationInstructionDto;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* RestartProcessInstanceDto
*/
@JsonPropertyOrder({
RestartProcessInstanceDto.JSON_PROPERTY_PROCESS_INSTANCE_IDS,
RestartProcessInstanceDto.JSON_PROPERTY_HISTORIC_PROCESS_INSTANCE_QUERY,
RestartProcessInstanceDto.JSON_PROPERTY_SKIP_CUSTOM_LISTENERS,
RestartProcessInstanceDto.JSON_PROPERTY_SKIP_IO_MAPPINGS,
RestartProcessInstanceDto.JSON_PROPERTY_INITIAL_VARIABLES,
RestartProcessInstanceDto.JSON_PROPERTY_WITHOUT_BUSINESS_KEY,
RestartProcessInstanceDto.JSON_PROPERTY_INSTRUCTIONS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class RestartProcessInstanceDto {
public static final String JSON_PROPERTY_PROCESS_INSTANCE_IDS = "processInstanceIds";
private JsonNullable> processInstanceIds = JsonNullable.>undefined();
public static final String JSON_PROPERTY_HISTORIC_PROCESS_INSTANCE_QUERY = "historicProcessInstanceQuery";
private HistoricProcessInstanceQueryDto historicProcessInstanceQuery;
public static final String JSON_PROPERTY_SKIP_CUSTOM_LISTENERS = "skipCustomListeners";
private JsonNullable skipCustomListeners = JsonNullable.undefined();
public static final String JSON_PROPERTY_SKIP_IO_MAPPINGS = "skipIoMappings";
private JsonNullable skipIoMappings = JsonNullable.undefined();
public static final String JSON_PROPERTY_INITIAL_VARIABLES = "initialVariables";
private JsonNullable initialVariables = JsonNullable.undefined();
public static final String JSON_PROPERTY_WITHOUT_BUSINESS_KEY = "withoutBusinessKey";
private JsonNullable withoutBusinessKey = JsonNullable.undefined();
public static final String JSON_PROPERTY_INSTRUCTIONS = "instructions";
private JsonNullable> instructions = JsonNullable.>undefined();
public RestartProcessInstanceDto() {
}
public RestartProcessInstanceDto processInstanceIds(List processInstanceIds) {
this.processInstanceIds = JsonNullable.>of(processInstanceIds);
return this;
}
public RestartProcessInstanceDto addProcessInstanceIdsItem(String processInstanceIdsItem) {
if (this.processInstanceIds == null || !this.processInstanceIds.isPresent()) {
this.processInstanceIds = JsonNullable.>of(new ArrayList<>());
}
try {
this.processInstanceIds.get().add(processInstanceIdsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* A list of process instance ids to restart.
* @return processInstanceIds
**/
@javax.annotation.Nullable
@JsonIgnore
public List getProcessInstanceIds() {
return processInstanceIds.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getProcessInstanceIds_JsonNullable() {
return processInstanceIds;
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_IDS)
public void setProcessInstanceIds_JsonNullable(JsonNullable> processInstanceIds) {
this.processInstanceIds = processInstanceIds;
}
public void setProcessInstanceIds(List processInstanceIds) {
this.processInstanceIds = JsonNullable.>of(processInstanceIds);
}
public RestartProcessInstanceDto historicProcessInstanceQuery(HistoricProcessInstanceQueryDto historicProcessInstanceQuery) {
this.historicProcessInstanceQuery = historicProcessInstanceQuery;
return this;
}
/**
* Get historicProcessInstanceQuery
* @return historicProcessInstanceQuery
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_HISTORIC_PROCESS_INSTANCE_QUERY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HistoricProcessInstanceQueryDto getHistoricProcessInstanceQuery() {
return historicProcessInstanceQuery;
}
@JsonProperty(JSON_PROPERTY_HISTORIC_PROCESS_INSTANCE_QUERY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHistoricProcessInstanceQuery(HistoricProcessInstanceQueryDto historicProcessInstanceQuery) {
this.historicProcessInstanceQuery = historicProcessInstanceQuery;
}
public RestartProcessInstanceDto skipCustomListeners(Boolean skipCustomListeners) {
this.skipCustomListeners = JsonNullable.of(skipCustomListeners);
return this;
}
/**
* Skip execution listener invocation for activities that are started as part of this request.
* @return skipCustomListeners
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getSkipCustomListeners() {
return skipCustomListeners.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SKIP_CUSTOM_LISTENERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSkipCustomListeners_JsonNullable() {
return skipCustomListeners;
}
@JsonProperty(JSON_PROPERTY_SKIP_CUSTOM_LISTENERS)
public void setSkipCustomListeners_JsonNullable(JsonNullable skipCustomListeners) {
this.skipCustomListeners = skipCustomListeners;
}
public void setSkipCustomListeners(Boolean skipCustomListeners) {
this.skipCustomListeners = JsonNullable.of(skipCustomListeners);
}
public RestartProcessInstanceDto skipIoMappings(Boolean skipIoMappings) {
this.skipIoMappings = JsonNullable.of(skipIoMappings);
return this;
}
/**
* Skip execution of [input/output variable mappings](https://docs.camunda.org/manual/7.21/user-guide/process-engine/variables/#input-output-variable-mapping) for activities that are started as part of this request.
* @return skipIoMappings
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getSkipIoMappings() {
return skipIoMappings.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SKIP_IO_MAPPINGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSkipIoMappings_JsonNullable() {
return skipIoMappings;
}
@JsonProperty(JSON_PROPERTY_SKIP_IO_MAPPINGS)
public void setSkipIoMappings_JsonNullable(JsonNullable skipIoMappings) {
this.skipIoMappings = skipIoMappings;
}
public void setSkipIoMappings(Boolean skipIoMappings) {
this.skipIoMappings = JsonNullable.of(skipIoMappings);
}
public RestartProcessInstanceDto initialVariables(Boolean initialVariables) {
this.initialVariables = JsonNullable.of(initialVariables);
return this;
}
/**
* Set the initial set of variables during restart. By default, the last set of variables is used.
* @return initialVariables
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getInitialVariables() {
return initialVariables.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INITIAL_VARIABLES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getInitialVariables_JsonNullable() {
return initialVariables;
}
@JsonProperty(JSON_PROPERTY_INITIAL_VARIABLES)
public void setInitialVariables_JsonNullable(JsonNullable initialVariables) {
this.initialVariables = initialVariables;
}
public void setInitialVariables(Boolean initialVariables) {
this.initialVariables = JsonNullable.of(initialVariables);
}
public RestartProcessInstanceDto withoutBusinessKey(Boolean withoutBusinessKey) {
this.withoutBusinessKey = JsonNullable.of(withoutBusinessKey);
return this;
}
/**
* Do not take over the business key of the historic process instance.
* @return withoutBusinessKey
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getWithoutBusinessKey() {
return withoutBusinessKey.orElse(null);
}
@JsonProperty(JSON_PROPERTY_WITHOUT_BUSINESS_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getWithoutBusinessKey_JsonNullable() {
return withoutBusinessKey;
}
@JsonProperty(JSON_PROPERTY_WITHOUT_BUSINESS_KEY)
public void setWithoutBusinessKey_JsonNullable(JsonNullable withoutBusinessKey) {
this.withoutBusinessKey = withoutBusinessKey;
}
public void setWithoutBusinessKey(Boolean withoutBusinessKey) {
this.withoutBusinessKey = JsonNullable.of(withoutBusinessKey);
}
public RestartProcessInstanceDto instructions(List instructions) {
this.instructions = JsonNullable.>of(instructions);
return this;
}
public RestartProcessInstanceDto addInstructionsItem(RestartProcessInstanceModificationInstructionDto instructionsItem) {
if (this.instructions == null || !this.instructions.isPresent()) {
this.instructions = JsonNullable.>of(new ArrayList<>());
}
try {
this.instructions.get().add(instructionsItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* **Optional**. A JSON array of instructions that specify which activities to start the process instance at. If this property is omitted, the process instance starts at its default blank start event.
* @return instructions
**/
@javax.annotation.Nullable
@JsonIgnore
public List getInstructions() {
return instructions.orElse(null);
}
@JsonProperty(JSON_PROPERTY_INSTRUCTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getInstructions_JsonNullable() {
return instructions;
}
@JsonProperty(JSON_PROPERTY_INSTRUCTIONS)
public void setInstructions_JsonNullable(JsonNullable> instructions) {
this.instructions = instructions;
}
public void setInstructions(List instructions) {
this.instructions = JsonNullable.>of(instructions);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RestartProcessInstanceDto restartProcessInstanceDto = (RestartProcessInstanceDto) o;
return equalsNullable(this.processInstanceIds, restartProcessInstanceDto.processInstanceIds) &&
Objects.equals(this.historicProcessInstanceQuery, restartProcessInstanceDto.historicProcessInstanceQuery) &&
equalsNullable(this.skipCustomListeners, restartProcessInstanceDto.skipCustomListeners) &&
equalsNullable(this.skipIoMappings, restartProcessInstanceDto.skipIoMappings) &&
equalsNullable(this.initialVariables, restartProcessInstanceDto.initialVariables) &&
equalsNullable(this.withoutBusinessKey, restartProcessInstanceDto.withoutBusinessKey) &&
equalsNullable(this.instructions, restartProcessInstanceDto.instructions);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(processInstanceIds), historicProcessInstanceQuery, hashCodeNullable(skipCustomListeners), hashCodeNullable(skipIoMappings), hashCodeNullable(initialVariables), hashCodeNullable(withoutBusinessKey), hashCodeNullable(instructions));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RestartProcessInstanceDto {\n");
sb.append(" processInstanceIds: ").append(toIndentedString(processInstanceIds)).append("\n");
sb.append(" historicProcessInstanceQuery: ").append(toIndentedString(historicProcessInstanceQuery)).append("\n");
sb.append(" skipCustomListeners: ").append(toIndentedString(skipCustomListeners)).append("\n");
sb.append(" skipIoMappings: ").append(toIndentedString(skipIoMappings)).append("\n");
sb.append(" initialVariables: ").append(toIndentedString(initialVariables)).append("\n");
sb.append(" withoutBusinessKey: ").append(toIndentedString(withoutBusinessKey)).append("\n");
sb.append(" instructions: ").append(toIndentedString(instructions)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `processInstanceIds` to the URL query string
if (getProcessInstanceIds() != null) {
for (int i = 0; i < getProcessInstanceIds().size(); i++) {
try {
joiner.add(String.format("%sprocessInstanceIds%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getProcessInstanceIds().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `historicProcessInstanceQuery` to the URL query string
if (getHistoricProcessInstanceQuery() != null) {
joiner.add(getHistoricProcessInstanceQuery().toUrlQueryString(prefix + "historicProcessInstanceQuery" + suffix));
}
// add `skipCustomListeners` to the URL query string
if (getSkipCustomListeners() != null) {
try {
joiner.add(String.format("%sskipCustomListeners%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSkipCustomListeners()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `skipIoMappings` to the URL query string
if (getSkipIoMappings() != null) {
try {
joiner.add(String.format("%sskipIoMappings%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSkipIoMappings()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `initialVariables` to the URL query string
if (getInitialVariables() != null) {
try {
joiner.add(String.format("%sinitialVariables%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getInitialVariables()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `withoutBusinessKey` to the URL query string
if (getWithoutBusinessKey() != null) {
try {
joiner.add(String.format("%swithoutBusinessKey%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getWithoutBusinessKey()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `instructions` to the URL query string
if (getInstructions() != null) {
for (int i = 0; i < getInstructions().size(); i++) {
if (getInstructions().get(i) != null) {
joiner.add(getInstructions().get(i).toUrlQueryString(String.format("%sinstructions%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy